20+ Must-Know Python Commands You Should Know
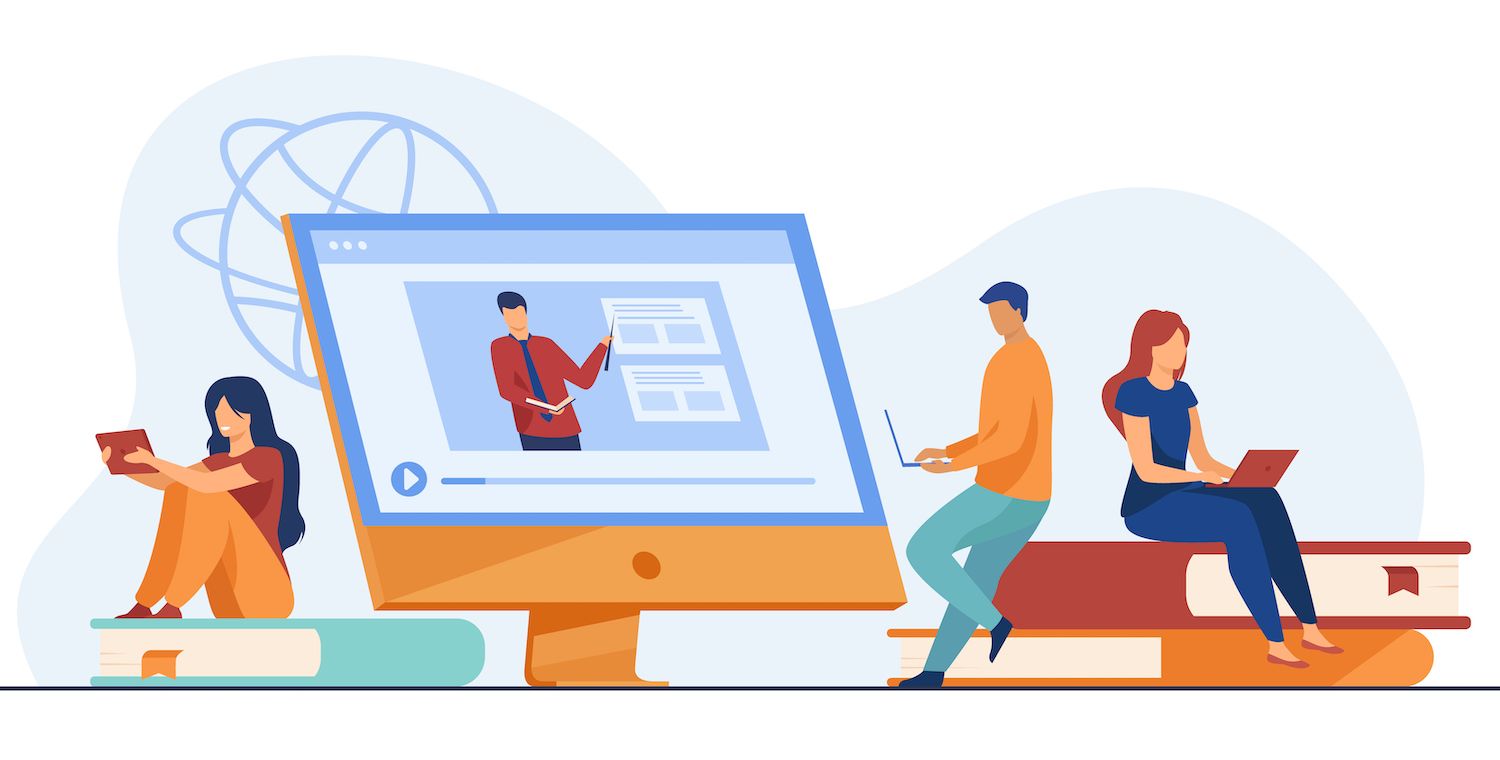
An 2021 Stack Overflow survey shows Python as the preferred programming language that most developers want to develop with most.
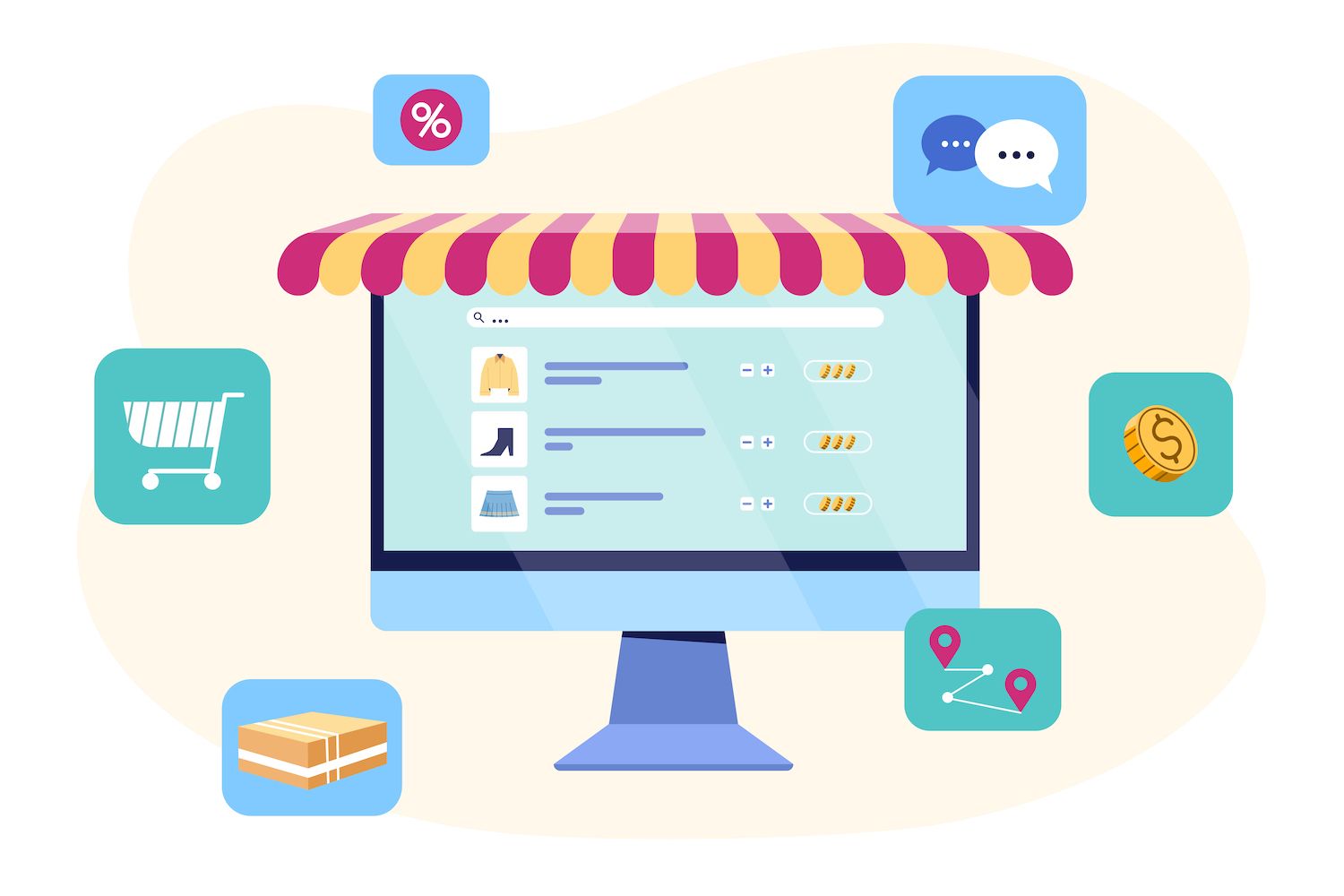
With the help of tools such as Jupyter Notebook, Google Colaboratory and online compilers you are able to start using this programming language without having to worry about installing anything.
In this guide, you'll learn some of the most important commands that are useful that are useful for Python development.
What Is the Command-Line Interface?
Command-line interface, often abbreviated CLI -- is a text-based application that is used to execute programs and perform things that are related to the OS (OS) that include creating and managing documents.
A CLI can execute different shells. The screenshot below illustrates two CLIs, one with the Python shell and one that uses Bash:
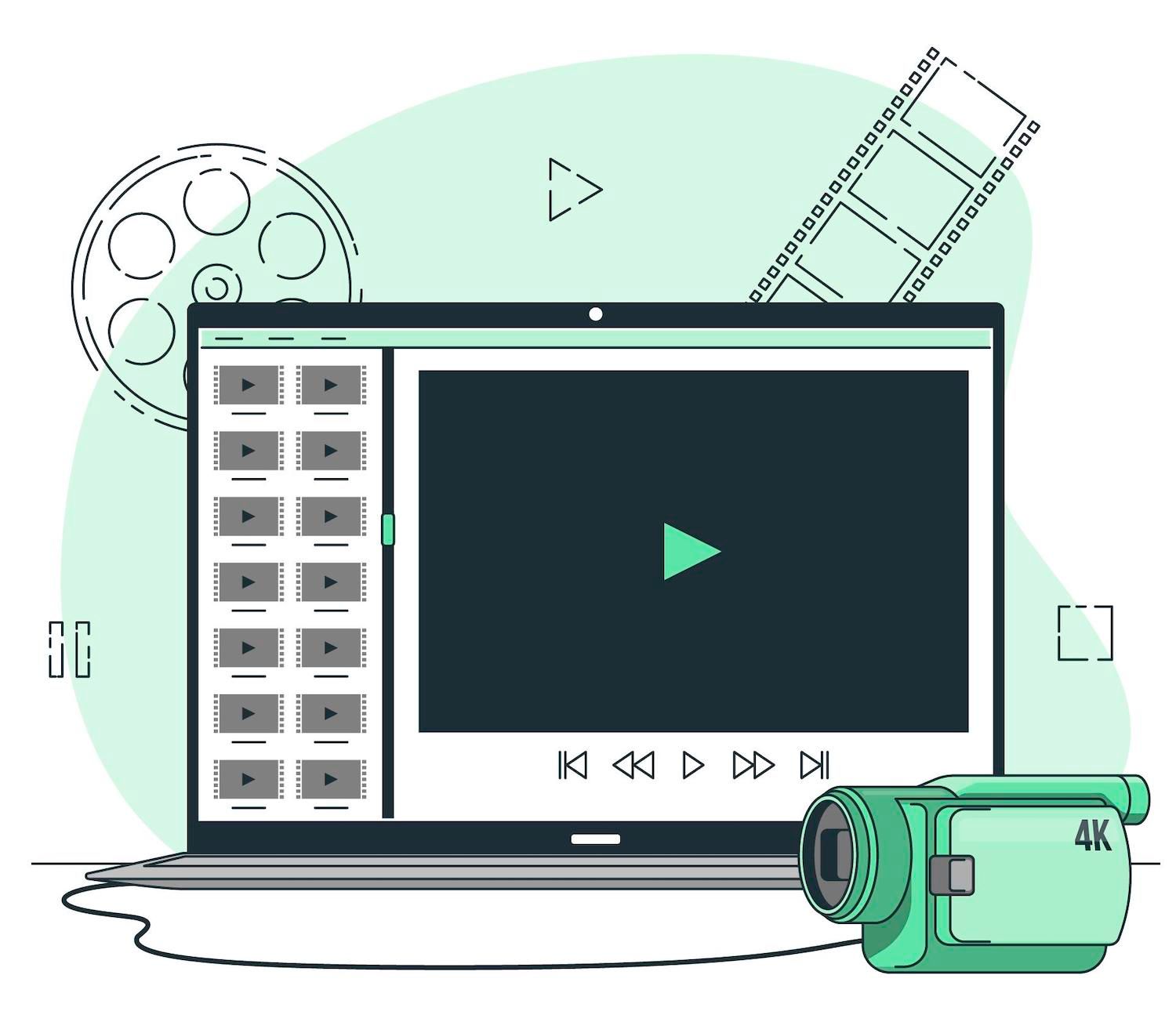
These two concepts are often confused, so this is the way to break them down:
- CLI is the text-based interface that allows you to type commands and get the output of those commands. It can execute different commands.
- A shell is a command interpreter that is able to interact with the operating system.
Believe it or not, every application you are running is controlled by commands. Your windows ( GUI) you interact with every day are made up of commands that are triggered by bindings which allow you to interact with your operating system.
Do you need the CLI for Python Development?
Actually, Python has loads of libraries to build CLI applications such as Typer, Argsparse, and Click. There is the possibility of going from only a CLI user to becoming a designer of your own CLI apps! This showcases the strong connection between CLI environments and Python.
The CLI: Introduction
Based on the OS you're running There are differences depending on the OS you're running. the way you interact with the command line. Every OS comes with its own method of opening and interacting with the CLI because of the different files organization as well as default command shells.
Let's look at three of the operating systems that are most commonly used by programmers: Windows, Mac, and Linux.
Windows
Keep in mind that Windows makes use of the letter
for paths instead of /
. Keep this in mind when navigating through directories.
Also on Windows there is the option of installing Git Bash, a command line that emulates the behavior of the Bash shell in Windows. This will make the majority of Unix commands listed below compatible with your system.
Mac
As for Mac the commands are accessible via an integrated application known as "Terminal". It is searchable using the Launchpad and locate it in the "Utilities" folder under "applications".
Linux
On Linux, you have loads of choices depending on which distro you are using However, the method "Ctrl + Alt + T" usually activates the default terminal of the system.
Then, you'll see an identical window like the one below:
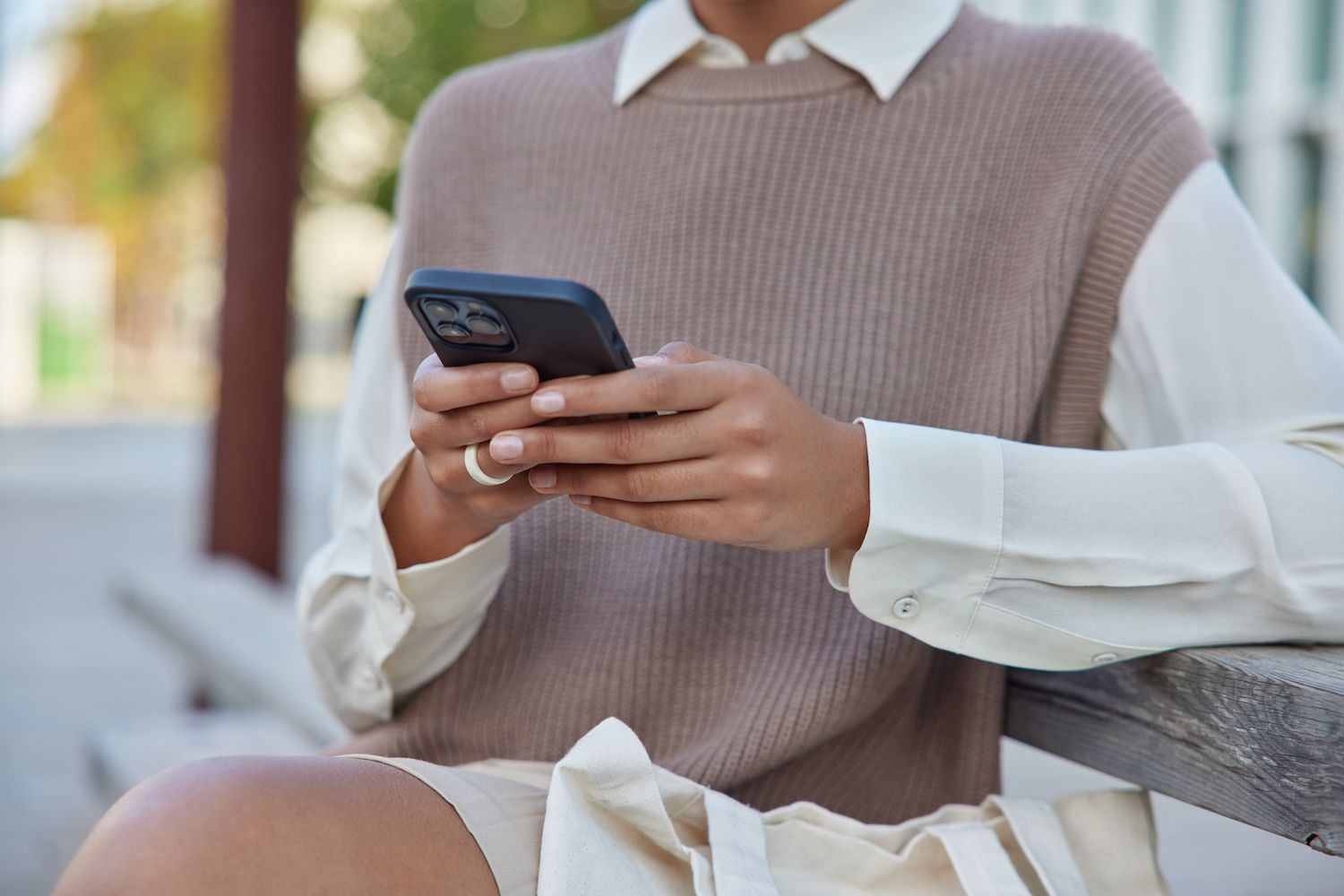
20+ of the Most Utilized CLI Commands for Python Development
After you've successfully got your CLI running, you're ready to explore the most popular command-line options that can make your life as an Python developer significantly easier.
Installation Commands
You've probably stumbled across numerous methods for installing Python. But sometimes, it's much simpler to accomplish it with just a command.
Here are the different commands that'll help you to install Python on different operating systems.
1. Chocolatey
When you run Windows, you have no standard package manager. One option to get past this problem is Chocolatey that provides users with the ability to install programs directly from the command line -- obviously including Python.
Be sure to Install Chocolatey prior to running the following command:
choco install python --pre
2. Homebrew and Pyenv
macOS includes Python 2.7 as a default. However, Python 2.7 is now deprecated. The whole community has shifted towards Python 3. For managing your Python versions in a way that is efficient it is possible to use an application such as Python.
Open a command line and download the most recent version Homebrew (a package manager similar to Chocolatey) using the following instructions:
/bin/bash -c "$(curl -fsSL
https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Then you can install pyenv by using this instruction:
brew install Python
It is possible to install a particular Python version and then set it to be the default Python executable in place of Python 2.
Python install 3.10 The version you'd like to install is
to install. pyenv globally 3.10.4 # Sets this version as default
When you run Python, it will be the Python version that you have set using pyenv:
python# Python 3.10.4 ....
# >>
3. 3. and dnf
In Debian-based distributions (Ubuntu, Linux Mint, Kali Linux) You'll utilize apt. It stands as "advanced tools for package development":
sudo apt update
sudo apt install python3
In addition, if you would like to make Python 3 as your preferred Python interpreter, utilize the following command
sudo apt install python-is-python3
For Arch-based distros, you can use the official package manager pacman:
sudo pacman -S python
For Fedora as well as RPM-based Linux distributions (Red Hat, CentOS), you make use of dnf
sudo dnf install python3
Interpreter Commands
Review the key flags -- command line options -- of the Python command, as well as its packaging manager, pip.
4. python
The python
command comes with a variety of flags. These are options which alter the way in which the execution of code.
To execute the Python file, just call the interpreter and add the name of the file, including the .py
extension:
python helloworld.py
If you're trying to recall the meaning of a flag then you could use the aid
flag to any of these 3 presentations:
Python -? Python -H python
In order to print (see) the version of Python you're running, you can use the following:
Python -V Python version
If you want to run Python code without opening and editing a .py
file, you can run it directly from your terminal with the command flag:
# Hello, World! Python # Hello, World! python "print('Hello, World! ')"
The flag m
flag runs a Python module using script. This is really beneficial when you wish to make a virtual space with the built-in venv module.
Python -m Venv .venv
5. pip
Its pip command scans for packages in the Python Package Index (PyPI) It also resolves dependencies and installs the program you've selected.
For installing an Python package, simply enter pip
as well as your name. Then, enter the package you'd like to install.
This command installs the latest version of the program:
pip install django
If you're looking for a specific version, run this command:
# pip install package==version
pip install django==4.0.4
When working on collaborative projects, you need to track dependencies, usually with the requirements file. With the flag r
flag the user can access and install programs in a text-based file
pip install with -r requirements.txt
A different feature that is commonly utilized is the the freeze
flag. This flag is used to generate an inventory of all the packages versions that you've put on your computer. It is also able to write your dependencies in a requirements file:
pip freeze >> requirements.txt
Permission Commands
Python is a great tool for creating scripts and managing files. For these functions, you need to have some knowledge of how the permissions are implemented on your OS.
6. sudo, runas
In Unix-based systems (macOS, Linux, BSD) You must be granted superuser privileges in order to carry out specific tasks like installing a software program, like you did in the previous example.
The sudo command allows you to temporarily gain admin permissions in order to perform any of these commands.
Below is an example of installing ueberzug (an images review Python package) worldwide:
sudo pip install ueberzug
The Windows equivalent of Runas is Runas, which executes scripts with a different user, or administrator
runas /noprofile /user:Administrator cmd
There are also other projects like Gsudo Gsudo, which helps to make the procedure of increasing permission significantly easier than using other integrated Windows commands.
Installation of gsudo
choco install gsudo
:: Reads a text file known as MySecretFile.txt
Gsudo enter MySecretFile.txt
7. Chmod
chmod
is used to change the permissions of files and directories on Unix.
A common usage is making an Python script executable:
# Creates mypythonscript.py executablechmod +x
mypythonscript.py
Once you've created an executable script and you're able to run it directly using the .Note: The
note:
# Executes the script./mypythonscript.py
Navigation Commands
Utilizing the file system through commands-line-interface is an everyday task for Python programmers. These are the most important actions you can use for navigating your system developing using Python.
8. Ls, dir
For listing the contents of a directory (folder) to list the contents of a folder, you need to use the ls
(Unix) or dir
(Windows) command. This was likely the first command you were taught upon first encountering the CLI.
Here's the syntax used:
Ls # Displays the contents of the directory that is used for work
mydirectory with ls
This is an example of the contents of a folder in a local file system:
ls test_python/# classes_error.py radius.py test-Django
This command is a great tool with many options. It's actually used in conjunction with the command ls -al
to view hidden files (those that have a dot at the start) and the sizes, mode and the date for each document:
alias ls="ls -al"
# Results
total 20
drwx------ 3 daniel daniel 4096 ene 16 19:13 . drwxr-xr-x 36 daniel daniel 4096 may 17 22:18 ..
-rw------- 1 daniel daniel 32 nov 17 2020 classes_error.py
-rw------- 1 daniel daniel 327 nov 10 2020 radius.py
drwx------ 4 daniel daniel 4096 ene 16 01:07 test-Django
As for Windows it is possible to use ls
using Git Bash, or you could use the integrated director
commands:
dir
9. pwd
PWD
is a reference to "print working directory" and does precisely it: provides you with all the path to the directory you're in:
pwd
# /home/daniel/github/HTML-site/images
If you've ever been lost on your computer, this command is invaluable.
You can achieve the same output in Windows by using the command cd
command without parameter (note that the command for Unix would take you to the Home directory):
# Available only on Windows
cd
# D:\Folder\subFolder
10. cp
Transferring files using a visual file manager is intuitive however, it is not efficient. By using this option, you are able to copy any data over the system.
cp old_file.txt copy_old_file.txt
To copy all the contents of a directory, you must utilize Cp -R
:
cp -r originaldirectory/ newdir
A similar word to cp
in Windows is the following: copy
:
Copy old_file.txt copy_old_file.txt /a
11. cat Type
To print the content of text files in the terminal, without opening the file in an editor, you can use to type cat
, more
than, and less
on Unix or type
on Windows:
cat old_file.txt # Unix
type old_file.txt # Windows
# Content
Hi there I hope you're enjoying my piece ...
I hope you enjoy itas much as I've loved creating it! The end of the example.
12. Mv, move
It is the mv
command is used to move directories and files from one location to the next (basically a copy and paste. Or, it alters the file's name if it's not in the directory:
# Rename files
mv source_file.txt renamed_file.txt
# Copy file to another directorymove renamed_file.txt thenewdir/
It is also possible to employ pattern matching for the transfer of files. In this case, you can move all .py
files to another folder:
MV *.py mypythondir/
A similar command for Windows is move
it, which offers nearly the same capabilities as the above:
# Windows
move source_file.txt renamed_file.txt
13. rm, del
It is possible to use the Rm
command to eliminate files and directories.
For deleting a file that is not an entire directory, you'd need to use:
rm file_to_remove.txt
If you want to delete any empty directories, you can use the"recursive" ( -r
) flag:
rm -r dir_to_remove/
To remove a directory with contents inside, employ the force ( -f
) and the recursive flags
Rm with contentThen, /
Similar to the above, you can find del
in Windows. Be even more cautious since the command isn't equipped with preventing flags seen previously:
del \mywindowsdir
14. 14.
Once you're done with the Python programming you should be capable of exiting the shell session. Most of the time, you will also shut down the program you're running in:
exit
Note that this command works both on Windows as well as Unix.
Command-Line Editors
Once you get used to the command line, it will be slower to change windows or even using the mouse to edit the code.
The ability to write code even while on the command line isn't only a great option to cut down on time, it can help you appear like an ace among your colleagues!
These are the most popular command-line editors.
15. Vim/Neovim
Vim and its variant, Neovim, are keyboard-based text editors which are mainly used in the Command Line. Based on an 2021 Survey by Stack Overflow survey, they rank 4th and first among the top loved editors by developers.
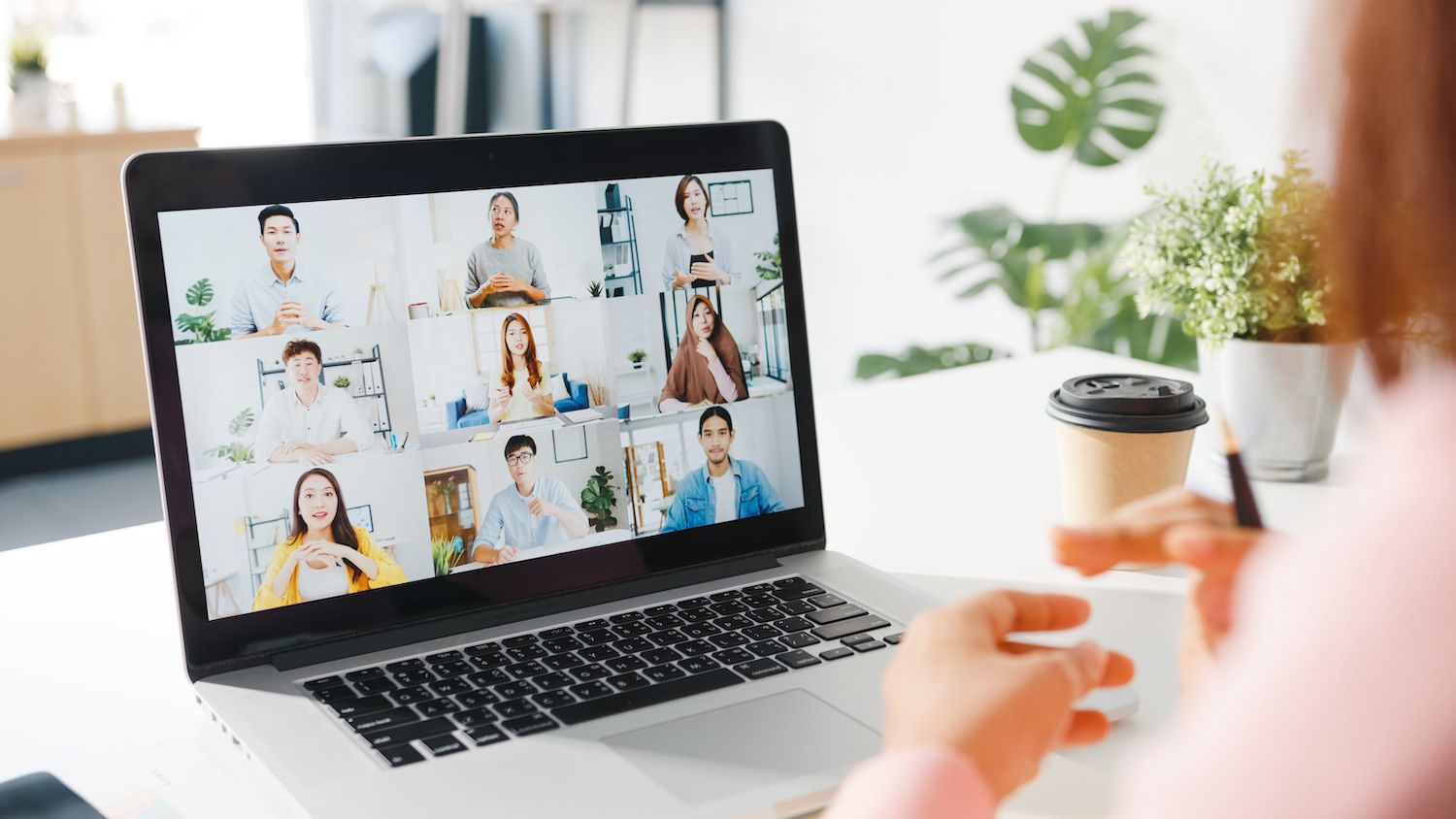
Now, you can enjoy the power of Vim simply by typing its name on your command line
vim
This will trigger an interface that is text-based, with multiple keyboard combinations for every step you'll need to perform when writing code using Python.
Vim comes with a steep learning curve, but when you've mastered the program, you'll never change to something else.
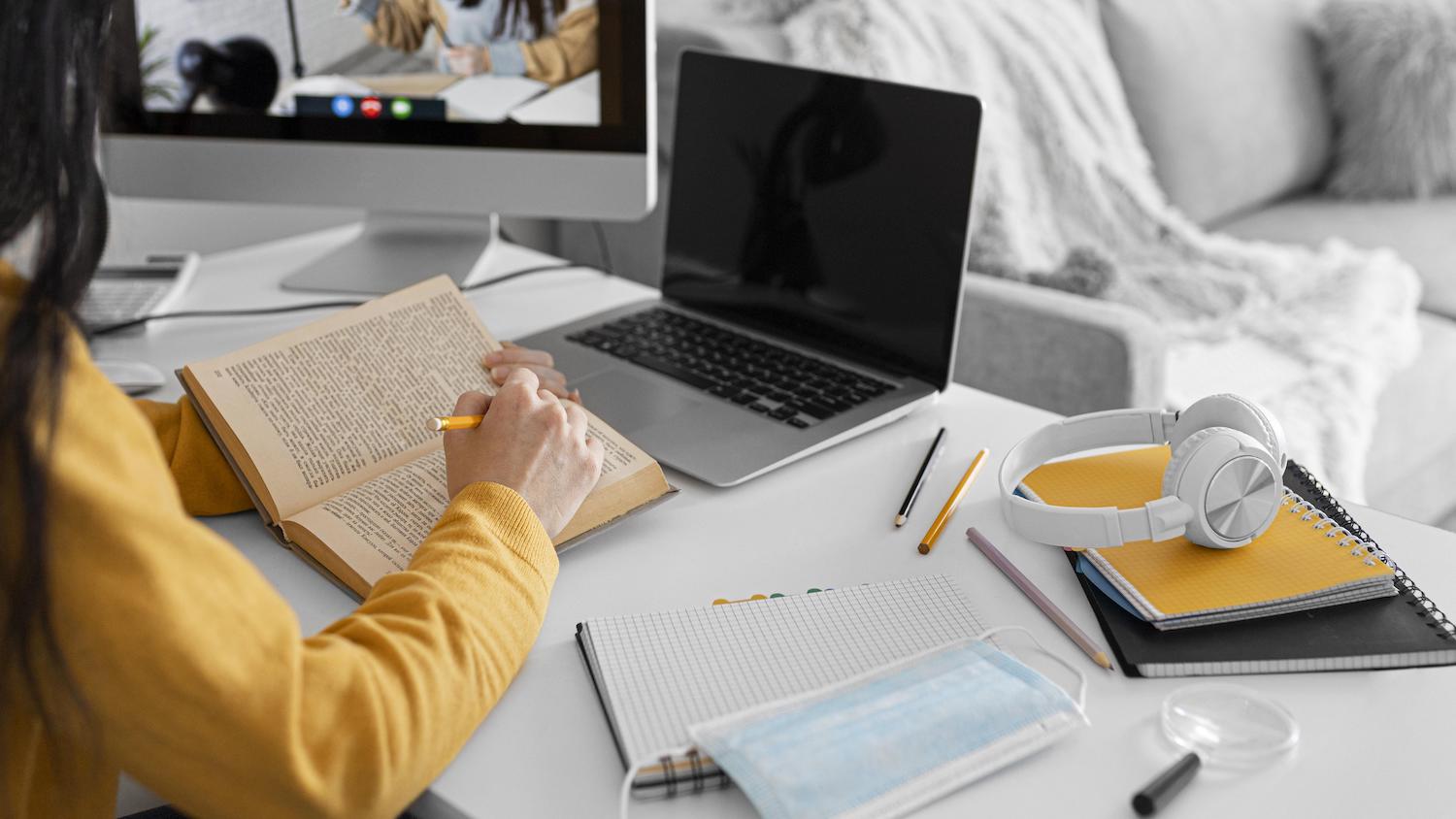
16. Nano
Nano is a command line text editor that's most often used for editing quick changes.
If you've made an error in your syntax code, but you don't want to open your editor to correct the issue. Nano assists you in fixing the problem right on your command line:
nano
17. Emacs
Emacs is one of the most extensible and customizable text editors that you will find. There's a whole area specifically devoted for Python programming where you'll find tons of extensions to improve your development experience.
Emacs is available in almost all operating systems, so If you're still not installed, you can follow the downloading directions.
To launch Emacs using the command line type, use the no window system flag ( -nw
):
Emacs -nw
Development Tools
Python development implies not only programming, but also the handling of additional tools such as virtual environments, version control systems, and deployment tools.
18. virtualenv/venv
Virtual environments are a crucial technique utilized to aid in Python development. They allow you to separate the software that are used in different projects into a lightweight folder, most commonly called .venv
.
When you use Python 3.3 or greater then you are able to utilize the built-in venv module create a virtual environment:
# .venv is the name given to the Python virtual environment -m"venv .venv
virtualenv is an external project which is faster and extensible compared to the built-in option. For creating a virtual world you must first install the virtualenv software:
# Installs the virtualenv pip --user virtualenv
# Creates the .venv virtual environmentvirtualenv .venv
Next, you'll need to activate the virtual environment. On Windows, run one of these commands depending on whether or not you're using cmd, or PowerShell (recommended):
:: PowerShell
.venv\Scripts\Activate.ps1
:: Cmd
.venv\Scripts\activate.bat
On Linux and macOs:
source .venv/bin/activate
19. Git
Once you have installed it, open a terminal and get an initial glimpse of all the available options with this command:
Help git
To create a repository, use git init
and then type in the name of the repo:
git init name_repository
Initialized empty Git repository in /home/daniel/name_repository/.git/
git clone [email protected]:DaniDiazTech/HTML-site.git ... Cloning into 'HTML-site'... remote: Enumerating objects: 24, done. remote: Counting objects 100 percent (24/24), done. remote: Compressing objects: 100% (18/18) completed. remote Total 24 (delta 6) Reused 21 (delta 4) Pack-reused zeroReceiving objects 100% (24/24), 4.98 MiB | 438.00 KiB/s, done. Resolving deltas: 100% (6/6), done.
20. Docker
Docker allows you to package and ship your Python apps in lightweight and portable containers that are self-sufficient. It assists in both development and deployment, making it possible for each collaborator to utilize the same settings.
To use Docker You must carefully follow the installation process shown for your operating system at the Get Docker page.
To display Docker commands available, run the following:
docker help
It's hard to explain how to run Docker compose in this narrow section. So be sure to review the official documentation.
21. Grep
Grep is an indispensable command-line utility used for pattern matching in plain text files.
Commonly, it is used to find how many times words repeat in a file:
grp -ic Python pythondocument.txt
2.
In the example above you can find the amount of instances Python (case insensitive) can be found within the pythondocument.txt
file.
The Windows equivalent of grep is findstr. It's however not the same thing. There is a Git Bash for the command grep on Windows:
istr/i /C Python pythondocument.txt
2
22. HTTPie
HTTPie is a command line HTTP client, which allows you to easily communicate with web-based services. It can be used, for example, to test your Python APIs or to interact with websites of third parties.
The CLI tool is accessible in almost every package manager according to the official documentation of HTTPie. But, it's also offered as a Python package. You could install it using pip.
pip installation httpie
Here's how you query an external API -In this instance, GitHub API:
http GET https://api.github.com/users/danidiaztech
HTTP/1.1 200 OK
Accept-Ranges: bytes
Access-Control-Allow-Origin: *
...
23. ping
This command is mainly used to confirm the connection between two machines such as your own machine as well as your Python application on a web server:
ping .com
PING .com(2606:4700:4400::ac40:917d (2606:4700:4400::ac40:917d)) 56 data bytes
64 bytes from 2606:4700:4400::ac40:917d (2606:4700:4400::ac40:917d): icmp_seq=1 ttl=50 time=686 ms
Command Reference Table
Below, you'll get a quick guide to the commands we've talked about:
Command | Usage |
---|---|
|
Installs package on Windows |
|
macOS package manager |
|
Package manager on different Linux distros |
|
Runs Python interpreter |
|
Python package manager |
|
Unix and Windows application used to expand permissions |
|
Changes file permissions |
|
The directory's content is listed. directory |
|
Prints the directory of operations |
|
Directories, files and copies |
|
Contents of the print file |
|
Changes (renames) files and directories |
|
Remove files and directories |
|
exits the current shell session |
|
Efficient text editing |
|
Text editor for quick edits |
|
The most flexible editor |
|
Virtual environment generators |
|
System for controlling version |
|
Containerize apps |
|
Pattern matching utility |
|
Web Service Testing Utility |
|
Tests the network's connectivity |
|
Terminates programs |
Summary
What other commands have you found useful for Python development? Comment them below!
Save time, costs and improve site performance by:
- Help is available immediately from WordPress hosting experts, 24/7.
- Cloudflare Enterprise integration.
- Global audience reach with 34 data centers around the world.
- Optimization using the built-in Application Performance Monitoring.