An introduction to WordPress coding standards - (r)
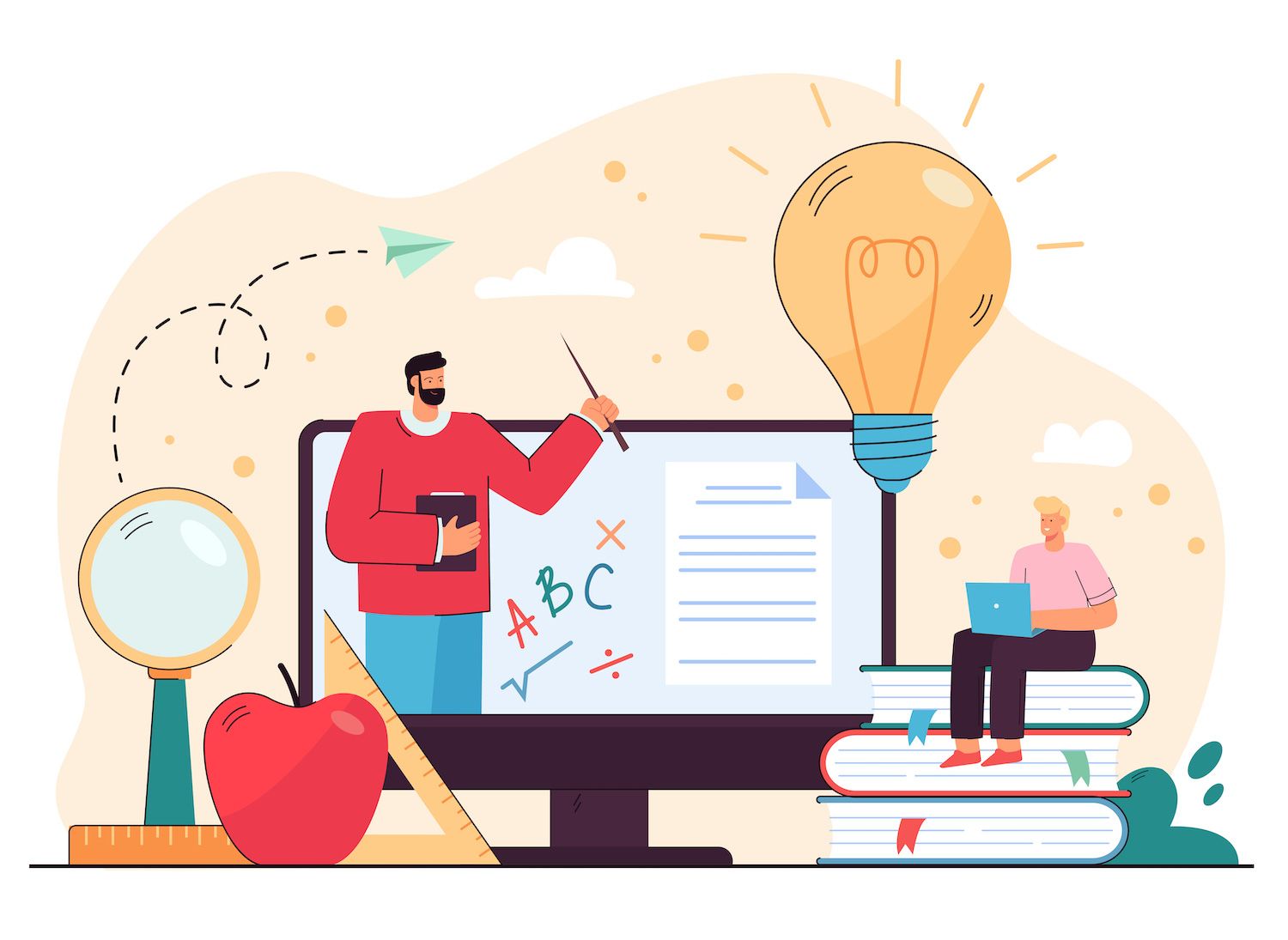
-sidebar-toc>
Moreover, coding standards safeguard against typical errors and mistakes as well as improve code quality. For WordPress development, where multiple contributors are often working together on a single project Coding standards support effective teamwork. They facilitate communication, mitigate possible conflicts and contribute to an efficient the development process.
The adhering to of coding standards helps ensure the consistency of your projects and makes it simpler to change between various codebases seamlessly. This consistency extends to code readability and maintainability and fosters a shared trust among your team members.
The official WordPress Coding standards encompass five important areas to ensure an efficient and cohesive the development process:
In this post this article, we look into the coding guidelines to assist you in getting going with building websites that are compliant or even contributing to WordPress development. WordPress development community.
Standards for PHP in WordPress development
WordPress-specific PHP codes ensure consistency and readability within WordPress code. They're mandatory to use for WordPress Core and strongly recommended for plugins and themes. They cover a range of issues, including naming conventions, indentation, as well as the structure of code to make it easier for users to read and ease collaboration.
WordPress PHP standards span the following categories:
- General This standard include placing the opening and closing PHP tags on a single line in their own when embedding a multi-line PHP snippet in an HTML block. Also, avoid using the use of shorthand PHP tags if you are using double and single quotes. Guidelines to write
are
andare required
the following statements:
// Closing and opening PHP tags inside HTML:
// Use open/close tags in their own lines. ## DO
function foo()
?>
Do not use shorthand PHP tags
## DO
do the following:
# DON'T
... ?>
esc_html( $x ); ?>
Write include/require statement:
// Avoid include_once because it will continue execution regardless of whether it isn't able to locate the file. Don't use brackets in the path to the file. Do not require once ABSPATH . 'file-name.php'
## DON'T
require_once __DIR__ . '/file-name.php Include_once ( ABSPATH . 'file-name.php' );
Naming The standards for naming comprise names conventions, as well as interpolation and interpolation when naming dynamic hooks:
#DOMake use of lowercase letters in variables and function names. Function my_function( $some_variable ) { /Make use of uppercase letters to describe name constants. define('MAX_AGE', 60) •# DON'T
// Make use of the camelCase. function myFunction( the variable $someVariable )
Whitespace -- Whitespace standards provide guidelines on space use, indentation, and removing space trails. (If you're planning to launch an enthusiastic debate among developers, ask them what they would prefer: tabs or spaces for indenting code. Whatever your preference The official suggestion of WordPress web developers are tabs -as is JavaScript and CSS, in addition to PHP. So, keep that in mind when working on collaborative projects.)
## DO
// Put spaces after commas. Colors are ['red green', blue']
// Put spaces on both sides of the opening and
// closing brackets of controls. foreach( $foo and $bar ) {// ...// Defining theFunction my_function() { ...
//// Logical comparisons:
if ( ! $foo ) { ...
// Accessing array items:
$a = $foo['bar']
$a = $foo[ $bar ]
## DON'T
$colors = ['red','green','blue']
foreach($foo as $bar){ ...
function my_function(){ ...
if (!$foo) { ...
$a = $foo[ 'bar' ]
$a = $foo[$bar]
Formatting -- Formatting standards to be used in WordPress PHP development include brace styles and array declarations. They also provide guidelines for multi-line function calls, type declarations magical constants, the spread operator:
// DOMake use of the brace style as follows. If ( condition )
action();
else if ( condition2 )
action2();
otherwise
default_action();
// Declare arrays with longer syntax. $numbers_long = array(1, 2, 3 5 5, 5) * In multi-line function calls, each parameter must be a single line. Multi-line parameter values should be assigned a variable, and that variable should be passed to functions. */
$data = array(
'user_name' => 'John Doe',
'email' => '[email protected]',
'address' => '123 Main Street, Cityville',
);
$greeting_message = sprintf(
/* translation function. %s maps to User's name */*/( 'Hello %s! ' yourtextdomain' )$data['user_name']) The $result is one of the functions (
$data,
$greeting_message
function to translate The %s mapping is based on the name of the city*/sprintf( * __( "User lives in %s.' ), 'Cityville' )
( ', 'yourtextdomain' ), 'Cityville');
/Magical constants should be in uppercase. The constant ::class must be lowercase and there should not be spaces surrounding the resolution operator (::). add_action( my_action, array( __CLASS__, my_method ) ) Add_action( my_action ), array( My_Class::class, my_method ) ) ();• Make sure to add a space or a new line with appropriateindentation before a spread operator. There should be:
* No space between the spread's operator and any variable or function it applies to. There should be no space between the spread operator and the referrers when they are combined. *///DOfunction one of the functions( &...$arg1 )
bar( ...$arg2 );
bar(
array( ...$arg3 ),
...array_values( $array_vals )
);
//DONTcall some function( and ... $arg1 )
bar (...
$arg2 );
bar(
array( ...$arg3 ),...array_values( $array_vals )
);
Declare declarations, namespaces, and import statements The Coding standards deal with namespace declarations and use statements:
The namespace declaration should contain // capitalized words that are separated with underscores. namespace My_CompanyProject_ProjectUtilities;
// Import use statements can use aliases
// to prevent name collisions. use Project_NameFeatureClass_C as Aliased_Class_C;
Obscure-oriented programming (OOP) -- The standards require just one object structure for each file, providing guidelines for using traits use statements, making sure visibility is always declared by describing the specific order of the visibility modifier and its order guidelines for object instantiation:
"Trait Use" statements should be on the first page of a class. It is recommended that the trait use statement contain at minimum one line prior to and following
the first and last statements. // Always declare visibility. class Foo
use Bar_Trait;
public $baz = true;
...
// Always use parentheses whenever you create a new
// object instance. Make sure you don't put an extra space between the name of a class and the first bracket. $foo = new Foo ();
Control structures -- Control structures use elseif and not otherwise if, and guidelines for Yoda conditions.Yoda statements: When mixing variables with literals, constants, or function calls in logic-based comparisons, put the variable to the left to prevent accidental assignment such as:
// A "legal" evaluation:If ( True= $result ) * // Perform something using $result/ But a typo like it could slip through your fingers in the event that ( $result = true ) Then we will end up in this situation.
Operators The Operators standards include ternary operators, such as the error control operator ( @), and increment/decrement operators:
• Always use Ternary Operators // test to see if the statement is correct or False. $programming_language = ( 'PHP' === $language ) ? 'cool' : 'meh';
// Favor pre-increment/decrement over post-increment/decrement
// for stand-alone statements. // DO
$a// DON'Tuse $a --;
Database --HTML0 Database Database codes provide guidelines to perform database queries as well as the formatting of SQL statements.
Additional recommendations -- Additional guidelines include the use of self-explanatory flag values for function arguments, clever code, closures (anonymous functions) regular expressions shell commands and directions to stay clear of extraction().
WordPress inline documentation standards for PHP code
Alongside the rules above, WordPress provides inline documentation standards to PHP code. WordPress utilizes a custom schema for documentation that is derived from PHPDoc syntax. It is an ever-changing method of providing documentation to PHP code that is maintained by phpDocumentor. The standards simplify the process of creating external documentation, and help the broader WordPress developer community by fostering common understanding of structure of the codebase.
PHP documentation for WordPress mostly appears as block-styled comments or as inline blocks. Document the following in WordPress documents:
Functions and class methods
Classes
Class members include properties and constants
Requires and includes
Hooks (actions and filters)
Inline comments
Headers of files
Constants
HTML and CSS standards in WordPress
WordPress themes and plug-ins conform to the strict HTML coding standards for consistency, accessibility, and maintenance. These guidelines focus on semantic markup and encourage designers to employ HTML elements for their intended purposes. This practice enhances content structure and enhances the search engine optimization (SEO) efficiency. Additionally, you're encouraged to validate your HTML for compatibility with all different browsers.
HTML code standards provide guidelines for:
ValidationYou must validate your HTML pages using the Validator from the W3C to make sure that the markup you use is properly formatted.
Self-closing elements The forward slash in self-closing components should be accompanied by a space preceding it.
Indentation Indentation for HTML0. HTML indentation must reflect the structure of the HTML. When combining PHP with HTML, indent the PHP blocks according to that of HTML code.
?php when ( ! "have_articles"() ) is:>
"Not Found" Not Found
Not FoundNot Found Results were not found.
Get_error_msg();?>
endif;
Additionally, to in addition to HTML standard, WordPress' CSS standards assist you in creating clean modular and flexible stylesheets. They establish a standard for review and collaboration starting with core code, through themes to plugins. These guidelines help ensure the code you write is understandable as well as consistent and useful.
CSS standards for WordPress focus on using specific classes to target elements and promote a uniform and well-structured structure. Specifically, they define specifications that apply to:
Structure:
/* DO Each selector should be in its own line, and end with
a comma or curly brace. The brace that closes it should be at the same indentation level as the opening selector. */
#selector-1,
#selector-2
property: value;
Selectors:
/* DO
Use lowercase and separate words using hyperbolas. Double quotes are used around the value for attribute selectors. Beware of overqualified selections for example, div.container. */
#contact-form
property: value;
input[type="text"]
property: value;
Property (ordering and vendor prefixes):
/* Append properties with an apostrophe and a space. Property names should be in lowercase- except font names as well as vendor-specific property names -- and use shorthand. */
#selector
property: value;
Values:
* Insert a space before the value, and then a semicolon after. Use double quotes. 0 values should not contain units. Use a leading zero for decimal values. Separate multiple comma-separated value values in a single property using a space or new line. */
#contact-form
font-family: "Helvetica Neue", sans-serif;
opacity: 0.9;
box-shadow:
0 0 0 1px #5b9dd9,
0 0 2px 1px rgba(20, 120, 170, 0.9);
Media queries:
The rules for media queries must be indented at least one step within. Keep media queries grouped by media at the bottom of the stylesheet. */
@media every and (max-width: 1024px) and (min-width: 780px)
$selector
property: value;
Commenting:
Since the beginning of 2003, WordPress coding standards for HTML and CSS are in line with the World Wide Web Consortium (W3C) guidelines regarding HTML as well as CSS. The focus is on the fusion of the principles of responsive design and semantic markup, W3C standards have affected the design of themes as well as plugins. This began with the release of HTML5 and CSS3.
The use of W3C guidelines guarantees that WordPress websites conform to international standards for web design, improving accessibility and usability while showing a dedication to remaining updated, safe, and compatible with the larger web-based ecosystem.
The adherence to these rules within WordPress emphasizes HTML quality verification using W3C HTML markup validator. W3C HTML markup validation tool.
These HTML as well as CSS standards ensure an appealing and user-friendly, and efficient presentation for WordPress websites across different platforms. They facilitate a seamless user experience and facilitate the collaboration of the developers that work on various aspects of the WordPress community.
JavaScript codes and standards are used in WordPress
WordPress Coding standards provide guidelines on formatting and styling JavaScript code in themes and plugins. Furthermore, they help promote code consistency alongside the core PHP, HTML, and CSS code.
The WordPress JavaScript coding standards are built on the JQuery JavaScript Style Guide it was created in 2012 and was a complete list of conventions for coding which improves consistency in code and readability. At first, it was geared to projects using jQuery, however the success of this guideline led to widespread adoption beyond the framework.
Although the guidelines for jQuery inform WordPress guidelines, there are some notable distinctions for WordPress development:
WordPress makes use of single quotation marks for string declarations.
Case statements are enclosed within the switch blocks.
Contents of the function are indented consistently as well as wrappers that are full-file closures.
Some whitespace rules differ to conform to PHP WordPress's standards. For instance, the use of tabs or indenting.
JQuery's hard-line of 100 characters though it's encouraged, isn't strictly enforced.
WordPress JavaScript codes are covered by the following topics:
Code refactoring.
Code spacing, including object declarations arrays, functions, and calls:
// Object declarationsDOvar obj = name: John, height: 179; age: 27/ DON'Tuse var obj to mean 'John'. Age: 27, Height:
// / Arrays and functions
include extra spaces around elements and arguments. array = [ 1, 2 ];foo( arg1, arg2 );
Semicolon use:
// Always use semicolons
array = [ 1, 2 ];
Line breaks and indentation which include blocks and curly braces. Multi-line statements, and chained method calls:
Tabs are used for indentation
( function ( $ ) Expressions indented )( jQuery );
/If, else for, while and try blocks must span multiple lines
if ( condition ) Expressions that are otherwise valid when ( ( condition && condition ) |Condition ) Expressions
// Line break are required after the operator in the event that a statement// is too long to fit on one line. Variable html = " The sum of '+ a + in addition to "' + B + " and'+ c
' is ' + ( A + B + c ) + '';
If a sequence of method calls are too large to be fit on a single line, make the method on a line with only one call. The initial call must be on a separate line separate fromthe device on which methods are called. */
elements.addClass( "foo' )
*/ elements.addClass( 'foo' ).children()"hello.html( 'hello' )
.end()
.appendTo( body) );
Assignments as well as globals, including declaring variables with const and let, declaring variables with var, globals, and common libraries.
Naming conventions like abbreviations and acronyms, class definitions, and constants
// Abbreviations must be typed in camelCase. // All letters of acronyms must be capitalized. const userId = 1;
const currentDOMDocument = window.document;
// Class definition must use UpperCamelCaseConvention. class Human
...
// Constants must use SCREAMING_SNAKE_CASE convention. const SESSION_DURATION =60
Equality:
Make sure to use strict equality and inequality tests (=== in conjunction with !==)
use them in place of abstract check (== and !=). if ( name is "John" )
...
when ( result == "John")if ( resultsequals false )
...
// Also, with negative: if ( result == false )
...
Strings:
• Use single quotes to represent string literals. var myString = 'Hello world! '
Switch statements:
// Make a break for each case other than default. Case statements should be indented on one tab in the switch. switch ( event.keyCode )
// ENTER and SPACE both trigger x()
case $.ui.keyCode.ENTER:
case $.ui.keyCode.SPACE:
x();
break;
case $.ui.keyCode.ESCAPE:
y();
break;
default:
z();
Furthermore, WordPress coding standards outline several guidelines for writing JavaScript code.
As with PHP, WordPress provides standard documentation inline to JavaScript code. These standards for inline documentation, which are either formatted blocks of documentation or inline comments, adhere to those of JSDoc 3 standard to provide inline JavaScript documentation. The standards inline cover functions, class methods, objects, closures, object properties, events, and headers for files.
How can we ensure accessibility for WordPress development
Standards for accessibility are essential to ensuring that all digital content such as websites created on platforms like WordPress are accessible to anyone of any ability. By adopting the accessibility standards of W3C, you can ensure the websites built using WordPress are accessible and inclusive to individuals with disabilities.
The W3C accessibility guidelines, particularly those referred to as the Web Content Accessibility Guidelines (WCAG), provide a comprehensive framework for making web content more accessible. Being aware of the importance of inclusion, WordPress has incorporated these guidelines into the core functionality of its.
Catering to diverse needs involves the use of design and features like screen reader compatibility, the keyboard, and alternatives to non-text information.
Some practical examples of implementing accessibility features in your plugins and themes are the following:
Utilize semantic HTML -- Ensure proper usage of semantic HTML tags. For instance, use to create navigation menus. Similarly, use header> for main content. the header> tag to display headers on your site and to display the main content. The tags assist screen readers as well as other assistive technology understand the structure of the webpage.
Text alternatives are available to images, videos, and audio content• Provide descriptive alt text for images so that they can convey the meaning for those who can't view them. In WordPress you can add descriptions alt attributes to your library of media when you add images. Add captions and transcriptions to videos and provide text alternatives in audio-based content, so that people who are deaf and difficult to hear can get the content.
Develop with responsive design in mind Make sure your plugin or theme has a responsive design and is able to adjust to the different sizes of screens. This method is beneficial to users on diverse devices, as well as ensuring the same experience across different platforms.
Forms that are accessible -- Provide explicit labels and directions on field fields. Use the appropriate input types, like email or phone in order to select the right keyboard for mobile devices as well as assistive technologies.
Utilize the keyboard to navigate -- Ensure that the interactive elements can be navigated via keyboards. The user should be able navigate through buttons, links and forms fields. Make sure that keyboard accessibility is improved by avoiding reliance on mouse-only interactions.
The tools for ensuring that you adhere to WordPress coding standards
There are many code-sniffing tools accessible to assist you in ensuring that users adhere to the code standards as described in the previous paragraph. Here's a brief overview of the tools for validation available to verify for WordPress codes that meet the requirements.
PHP_CodeSniffer
It is a tool that PHP_CodeSniffer analyzes the PHP codebase for deviations from the established norms. This helps you write cleaner and faster code, by detecting the code's infractions and styles that are not compatible. This improves the performance for WordPress websites as well as ensuring seamless compatibility with future versions and plugins.
The CSS Validation Service of W3 Org. CSS Validation Service
The W3Org's CSS Validation Service scans CSS style sheets, identifying the potential flaws that may hinder optimal site performance. It plays a crucial role in ensuring consistency and adhering to W3C standards to ensure an enjoyable user experience on various platforms. In the end, websites experience faster loading speeds and meet the stringent CSS coding standards set by WordPress.
JSHint
JSHint analyses JavaScript code to identify possible errors, stylistic inconsistencies as well as adherence to the best guidelines. It assists you in writing clean, efficient code and ultimately optimizes your web's performance. With its steadfast focus on WordPress standardization ensures that JavaScript code seamlessly integrates with WordPress's overall structure. WordPress and helps you to ensure a uniform and consistent coding environment.
WebAIM Contrast Checker
WebAIM's Contrast Checker aids you assess and improve accessibility for the accessibility of your WordPress websites. The tool helps simplify the difficult process of creating optimal color contrast to promote accessibility. With the help of real-time feedback, it can help you determine areas where you can increase the readability and legibility of text for all visitors.
Summary
Coding standards form the foundation for efficient and collaborative software development. They assure accuracy and consistency in the code, streamline the process of coding, improve maintainability, and facilitate teamwork. In the case of WordPress developers adhere to the coding standard is essential to create robust and scalable websites.
Steve Bonisteel
Steve Bonisteel is a Technical Editor at who began his writing career as print journalist, chasing ambulances and fire trucks. He's been writing about internet-related technologies since the mid 1990s.