Build a Simple URL Shortener Utilizing Python (r) (r)
URL Shortener
Enter an https:// URL: Submit
Created URL"/form>" Body> /html>
The code above creates a form with two labels as well as two input fields as well as one button.
The first input field, dubbed URL
, is for writing the long URL, and the other field is for generating the shorter URL.
The URL
input field contains these attributes:
name
: To denote this elements ( e.g. URL)placeholder
: To show a URL for an example- pattern: To specify the pattern of a URL that is https ://. *
is required
: To give an input URL prior to sendingvalue
For a look at the URL that was previously used
The second input field is equipped with a value
attribute that is set for new_url
. New_url is new_url
is an uncomplicated URL created by the pyshorteners library from the main.py file (shown in the section below).
The entry form can be seen by the following image:
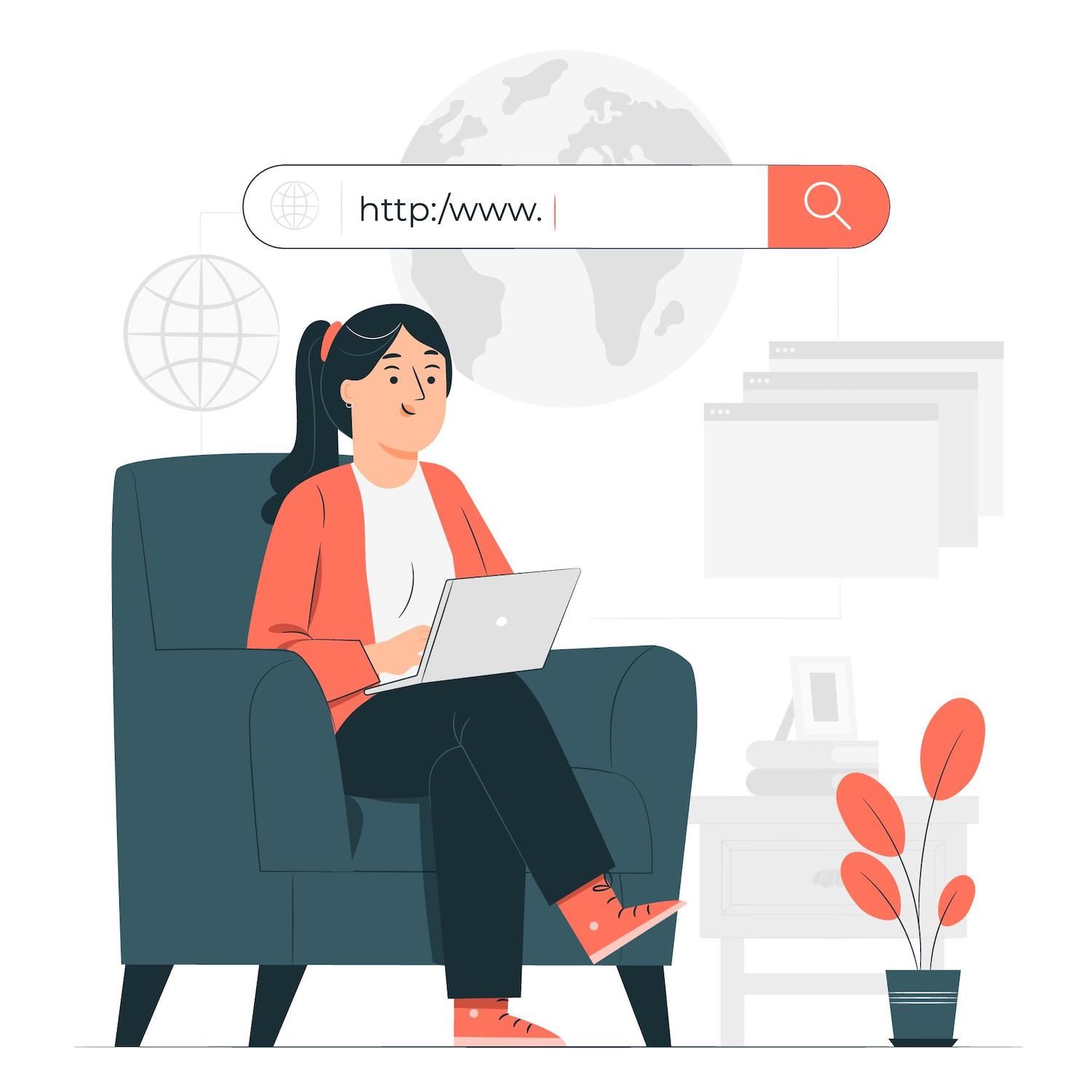
URL Shortening Code Using pyshorteners
After you've built the form, you're able to make it more functional using Python and pyshorteners.
The code will convert the lengthy URL into a short one and run the web application. Go to the main.py file you created earlier, enter the following code, and save it:
from flask import Flask, render_template, request
import pyshorteners
app = Flask(__name__)
@app.route("/", methods=['POST', 'GET'])
def home():
if request.method=="POST":
url_received = request.form["url"]
short_url = pyshorteners.Shortener().tinyurl.short(url_received)
return render_template("form.html", new_url=short_url, old_url=url_received)
else:
return render_template('form.html')
if __name__ == "__main__":
app.run()
The code above imports the shorteners pyshorteners library and the following modules from the Flask framework, all of which will be required to shorten URLs:
Flask
the Flask framework, itself, was previously introduced.render_template
The HTML0 render template is a rendering package used to generate HTML files. HTML documents from directorytemplate files
.request
A class of the Flask framework, which holds all the data that an individual user transmits from the frontend of the application to the backend, as in the HTTP request.
Next, it creates an application called home()
that takes a URL submitted in the form, and generates an shortened URL. The app.route()
decorator is used to bind the function to the particular URL route to run this application. The POST/GET methods handle the request.
Within the "home()
function, there's an in-between
conditional statement.
For the if
statement, if request.method=="POST"
, a variable called url_received
is set to request.form["url"]
, which is the URL submitted in the form. This is because URL
is the title of the input field that is defined within the HTML form that was created previously.
Then, a variable called short_url
is set to pyshorteners.Shortener().tinyurl.short(url_received)
.
Two methods use the pyshorteners library: .Shortener()
and .short()
. It is the .Shortener()
function creates an pyshorteners class instance and the .short()
function takes in the URL for an input, and will shorten it.
The brief()
function, tinyurl.short()
is among the shorteners in the pyshorteners libraries many APIs. osdb.short()
is a different API that could be used to accomplish the same purpose.
The render_template()
function is employed to create the HTML template file form.html and send URLs back to form via arguments. The new_url
argument is set to short_url
and the the old_url argument
is set to the URL that is received
. The is
statement's scope ends here.
For the else
statement, in the event that the method of request is different than POST, the form.html HTML template will be displayed.
Demonstration of URL Shortener Web App built with the Python Shorteners Library
To demonstrate the pyshorteners URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
Enter a hyperlink you like into the first field of the form for web:
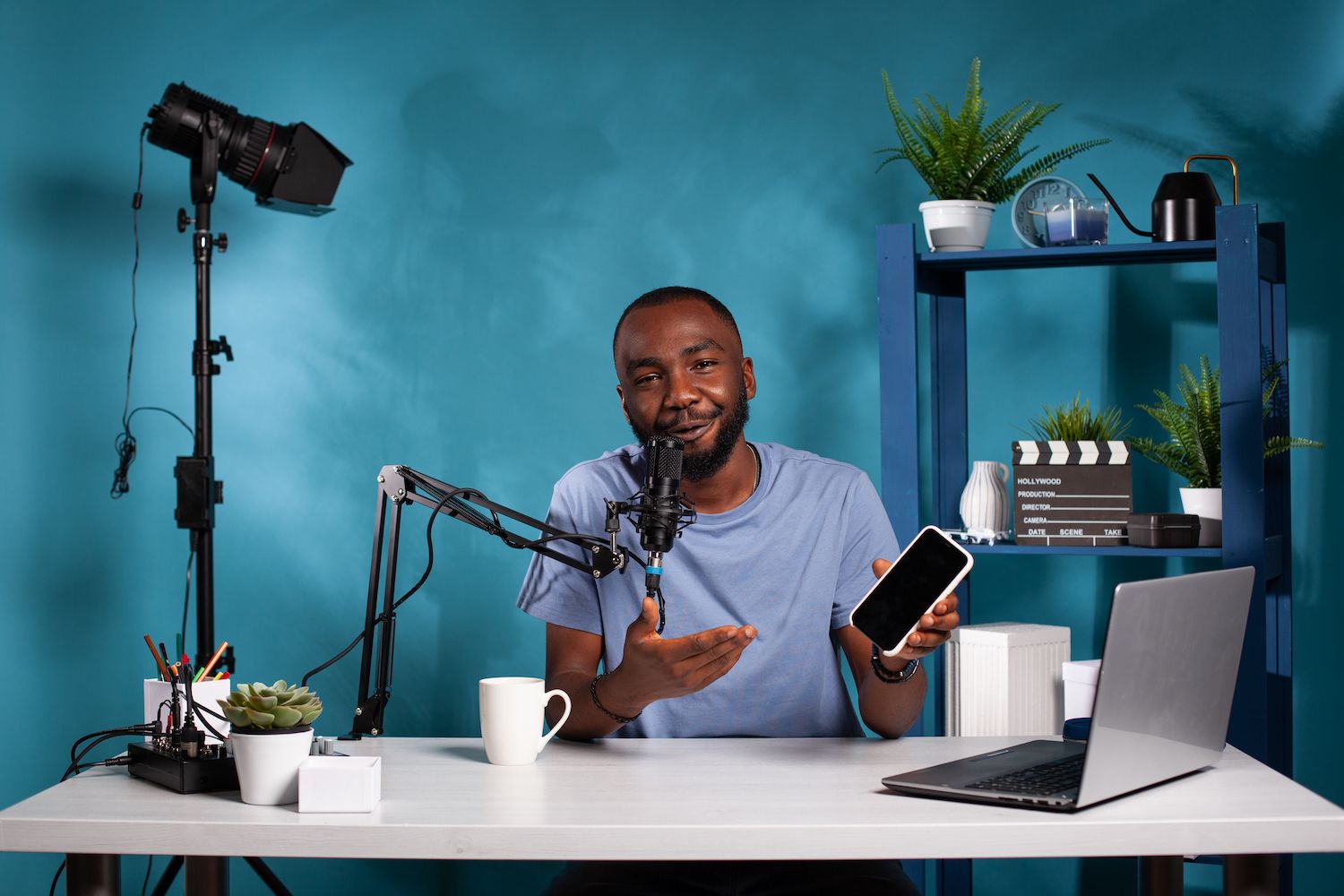
Hit on the Submit button to output the URL in a shorter form, with tinyurl
as the domain in the field for Generated URL
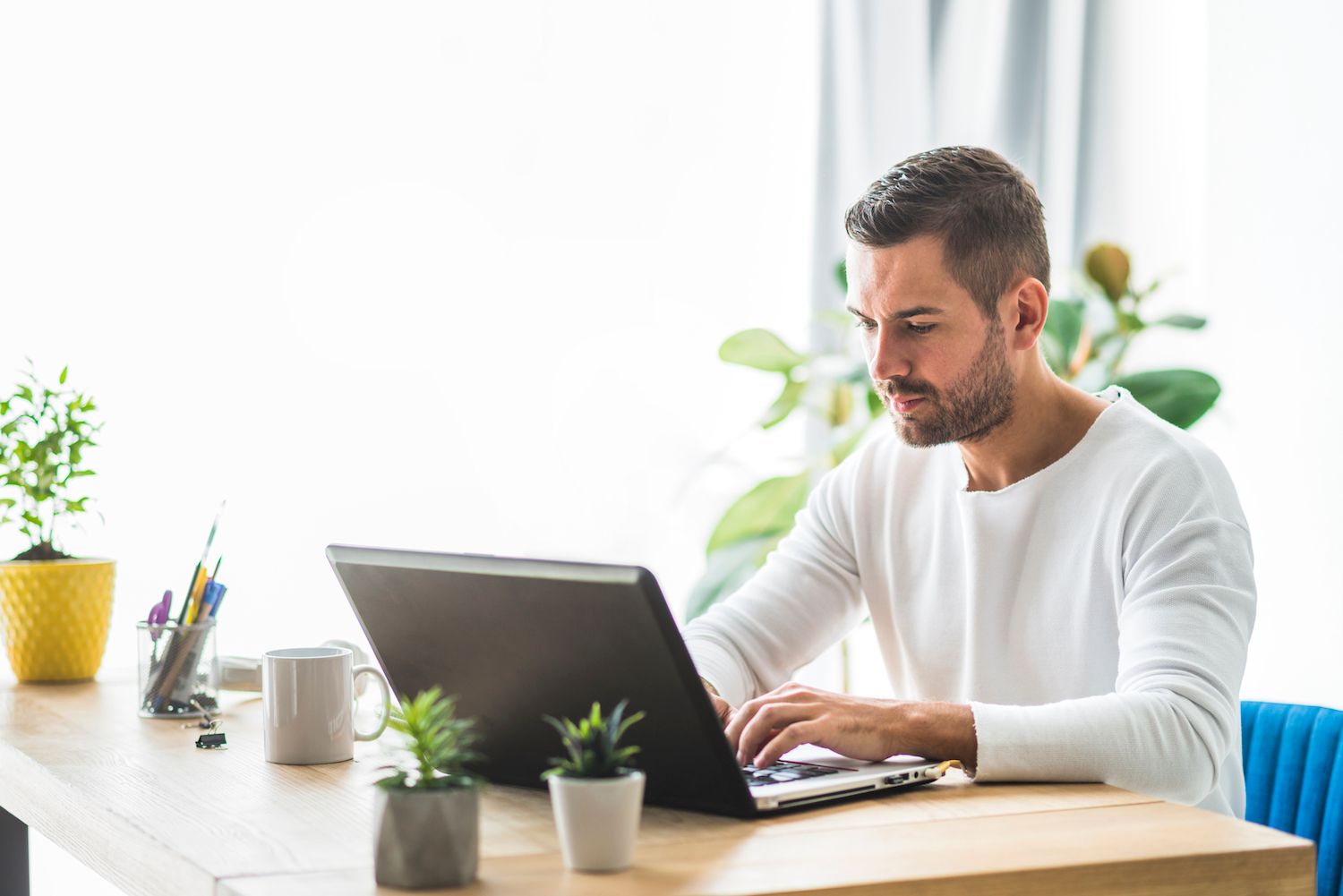
Using Bitly API Module Bitly API Module, you can build a URL Shortener Web App
In this section, you'll develop a URL-shortening application using Bitly API. Bitly API. The Bitly API module is another method for shortening URLs and additionally provides comprehensive analytics of clicks, the location as well as the type of device that are used (such as desktop or mobile).
Download the Bitly API using the following instruction:
pip to install bitly API-py3
Access tokens are required for access to the Bitly API. You are able to obtain by signing in with Bitly.
After you've completed the sign-up process After completing the signup process, login to Bitly to view your dashboard:
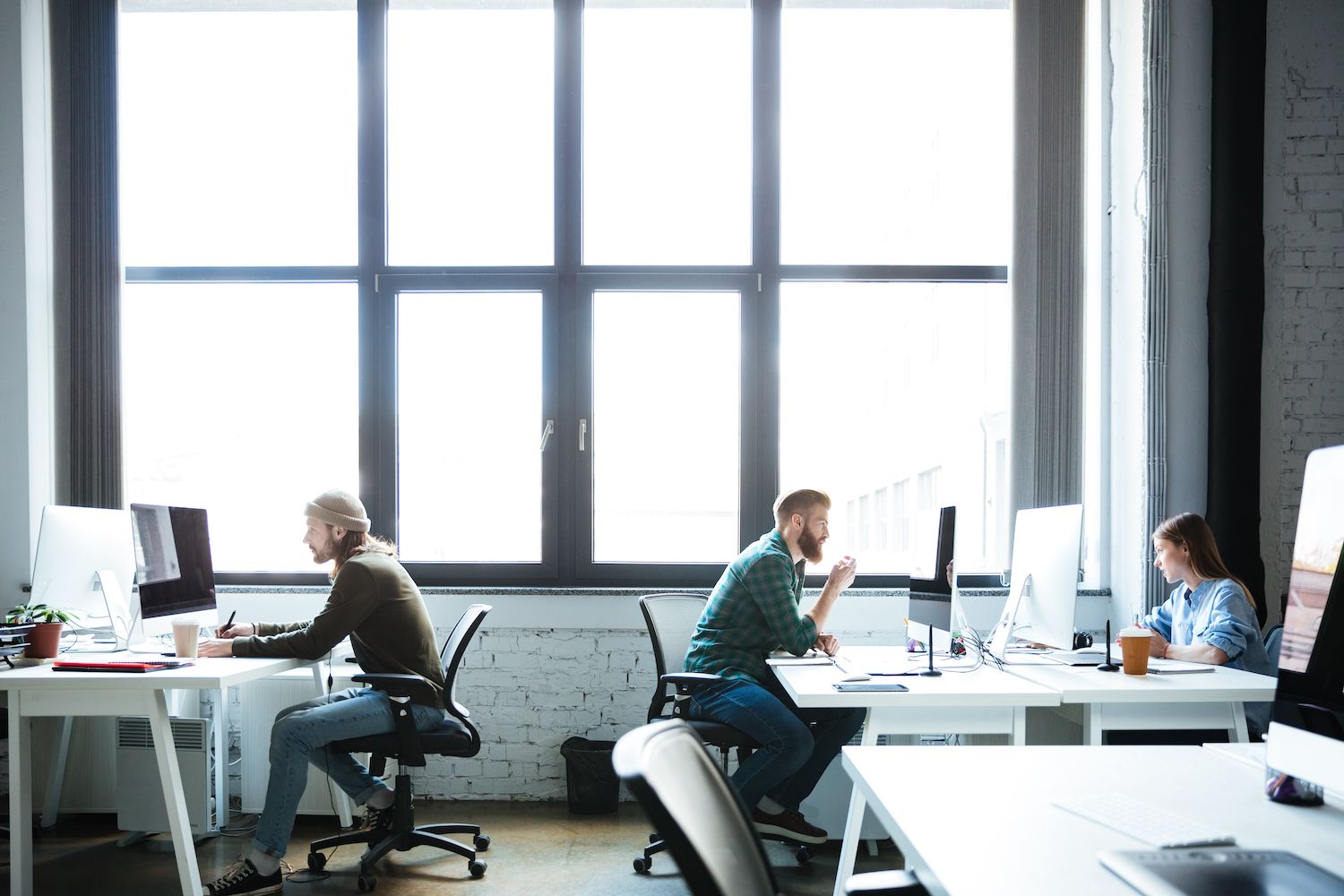
Select Settings on the left sidebar, then go to the API section located in Developer settings.
Generate an access token by entering your password into the area just above button Generator token button, which is shown in the picture below. save the token for use in the code for your app:
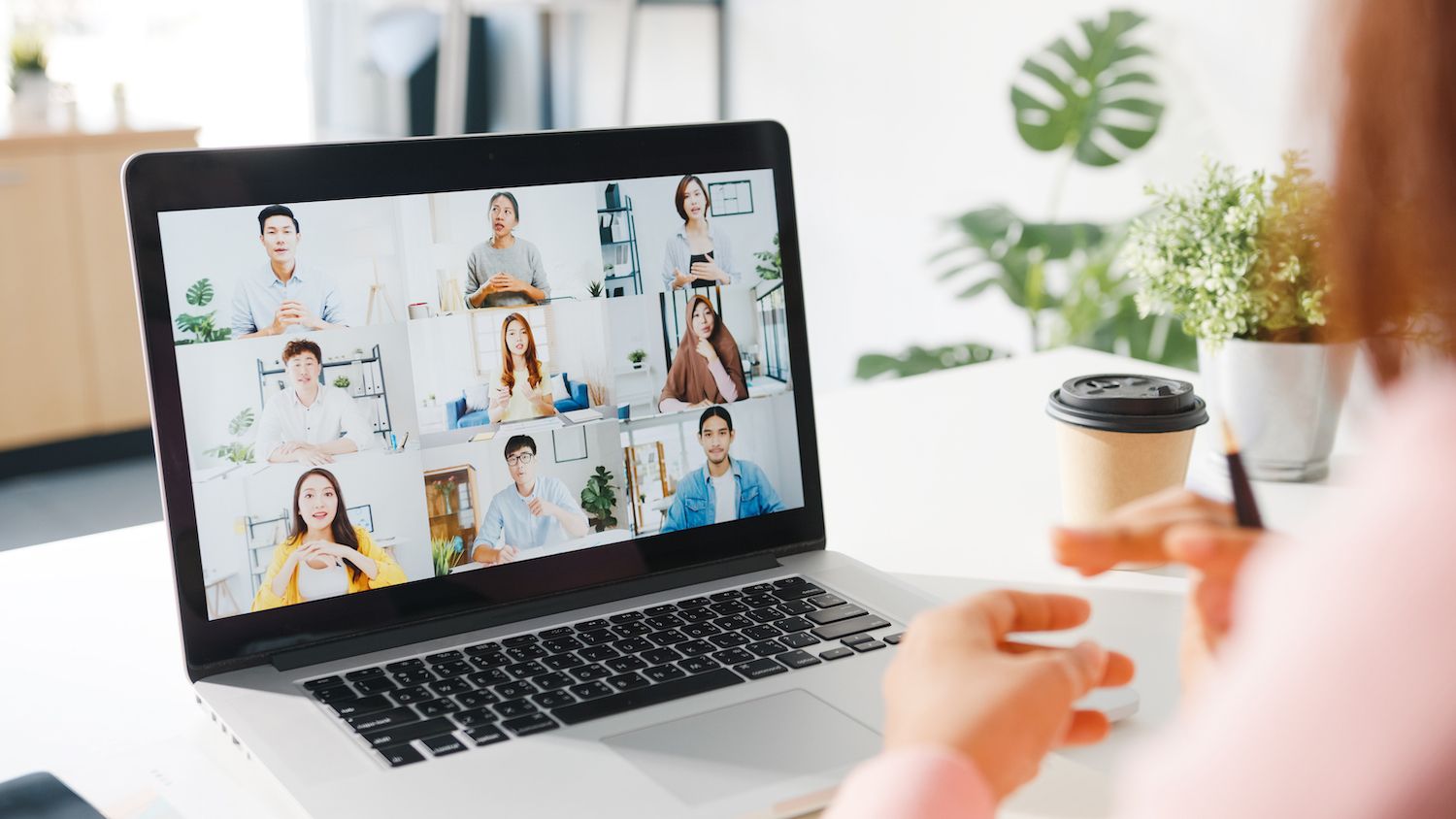
URL Shortening Code Using the Bitly API
Once you've received the token from Bitly You can then program your web application to shorten the URL using the Bitly API.
You'll use the same form you created to create the shorteners section, however with a few changes to your main.py file:
from flask import Flask, render_template, request
import bitly_api
app = Flask(__name__)
bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxxxx"
@app.route("/", methods=['POST', 'GET'])
def home():
if request.method=="POST":
url_received = request.form["url"]
bitly = bitly_api.Connection(access_token=bitly_access_token)
short_url = bitly.shorten(url_received)
return render_template("form.html", new_url=short_url.get('url'), old_url=url_received)
else:
return render_template('form.html')
if __name__ == "__main__":
app.run()
In the code above, bitly_api
is loaded using import bitly_api
. The access token is then saved in a variable called bity_access_token
, as in bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxx"
.
The "home"()
function shortens the URL. It contains an in-case-of-nothing
conditional expression.
In the If
statement when the method is POST, or the request has the format POST
, then the URL that is entered in the form will be changed to the URL_received
variable.
The bitly_api.Connection(access_token=bitly_access_token)
function connects to the Bitly API and passes it the access token you saved earlier as an argument.
To reduce the URL, it is possible to use the bitly.shorten()
function can be used by passing the URL_received
variable as an argument, and saving the value in a variable named short_url
.
After that, the form is rendered, and URLs are then sent back to display in the form using the render_template()
function. The if
statement completes the form.
In the other
clause for the else statement, the model is rendered with render_template() function. render_template()
function.
Exhibit of the URL Shortener Web Application built with the Bitly API
To demonstrate the Bitly API URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
Enter a hyperlink of your choice into the first field of the form for web:
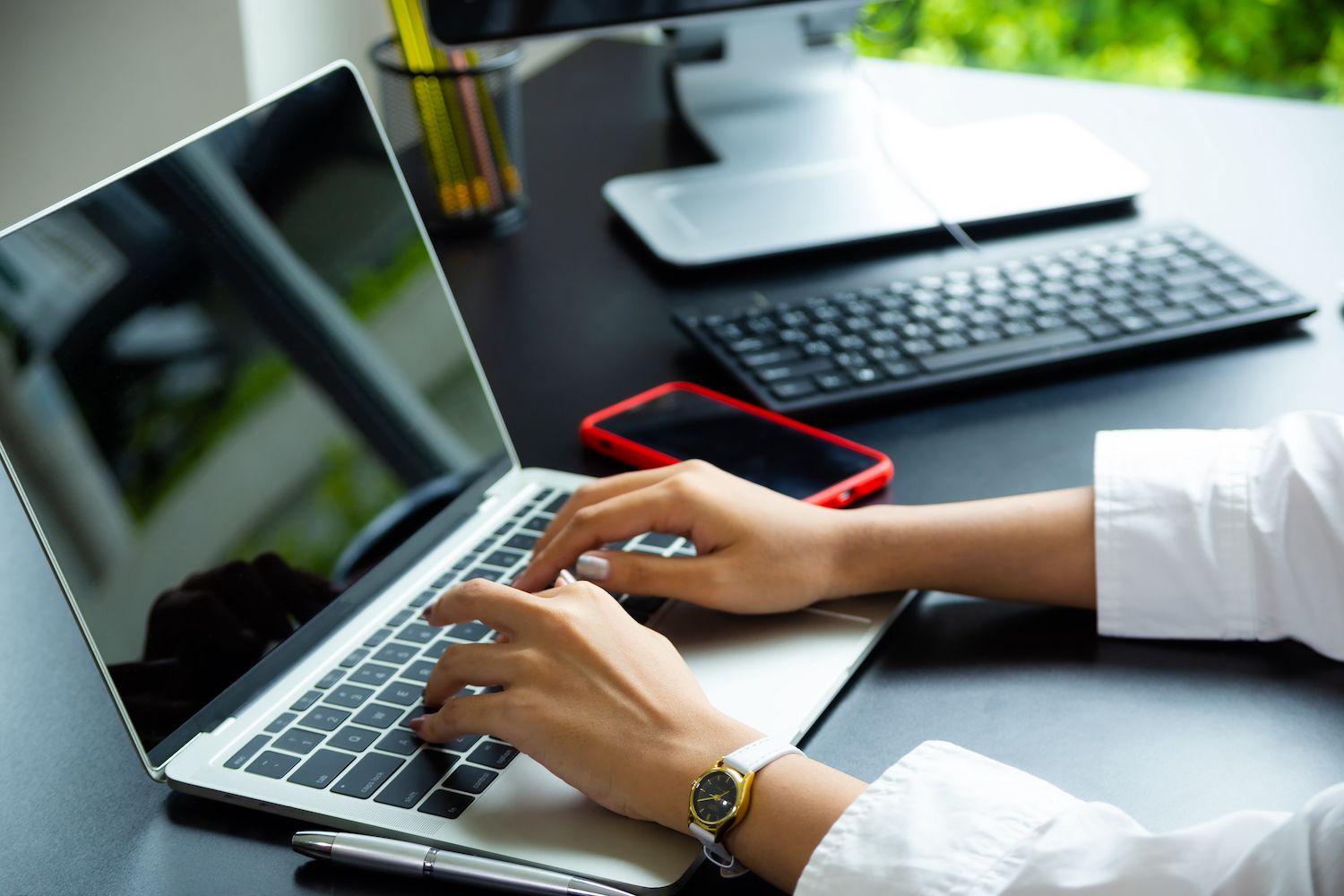
Hit "Submit" to generate a short URL using bit.ly
as the domain. The second area of the web application:
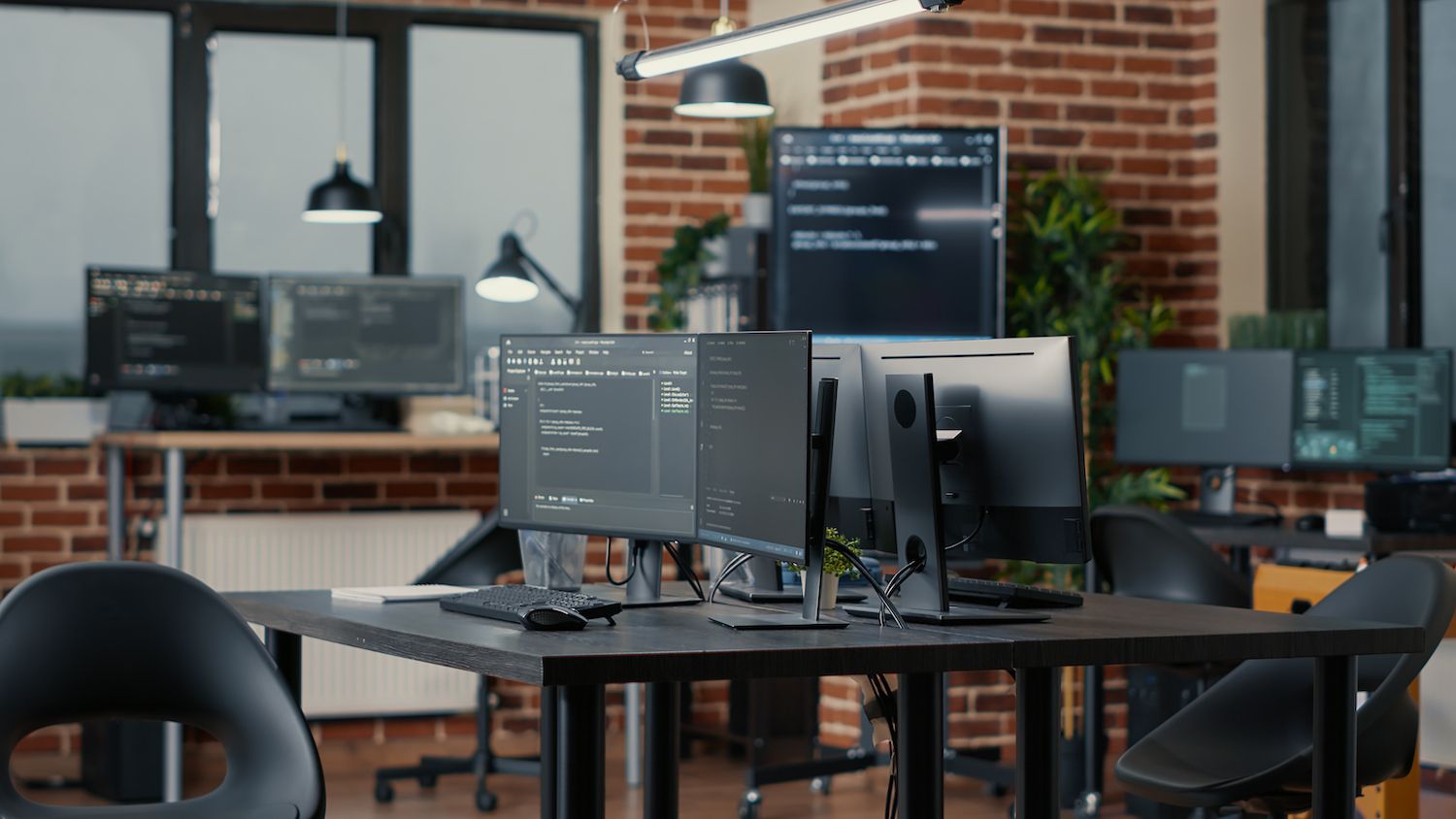
Using Bitly API to use the Bitly API to shorten URLs inside your Python program is as simple as that.
Summary
URL shorteners offer you shorter URLs that you can easily use, are cleaner looking, and require less space. In this article you will learn about URL shorteners and their benefits and ways to make a URL shortener-related web app using Python using Pyshorteners along with the Bitly API. The Python shorteners library provides short URLs, while the Bitly API provides detailed analytics along with short URLs.
Adarsh Chimnani
Adarsh is a Web developer (MERN stack), enthusiastic about game-level design (Unity3D), and an anime enthusiast. He is awed by learning out of curiosity, implementing the knowledge he's acquired in the real world and then sharing his information with other.