Build an App With FastAPI for Python - (r)
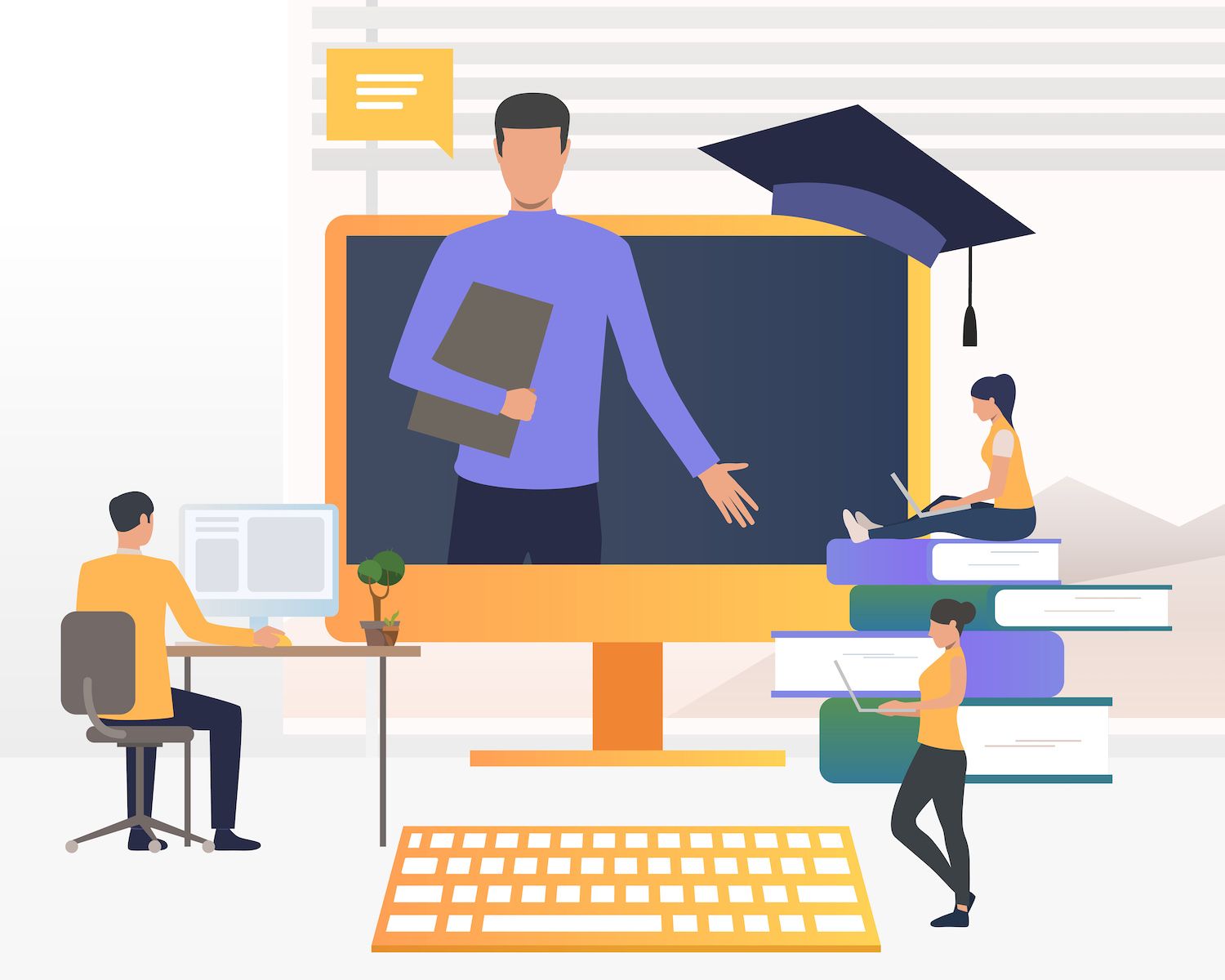
We should first learn the fundamentals.
The advantages of FastAPI
Below are some benefits that the FastAPI Framework provides to a project.
- Easy to learn and code: FastAPI has already discovered almost all the information you'll need to build an API for production. If you are a developer who uses FastAPI it is not necessary to code all of it from scratch. With only a few pages of code you can create a RESTful API that is ready for use.
- Documentation that is comprehensive: FastAPI uses the OpenAPI documentation standards, so documentation can be dynamically generated. This documentation provides detailed information on FastAPI's Endpoints, Responses to parameters, return codes.
- APIs with fewer bugs: FastAPI supports customized data validation that allows developers to build APIs with less bugs. FastAPI's developers boast that the framework results in fewer human-induced bugs -- as much as 40% lower.
- Type hints: The types module was added in Python 3.5. This allows you to specify the
nature
of a variable. When the type of a variable is defined, IDEs are able to offer better assistance and anticipate errors with greater accuracy.
What to Do To Start Using FastAPI
For this guide to follow and begin using FastAPI, you'll have to do a few things first.
It is a standard practice to keep your Python applications as well as their instances running on virtual machines. Virtual environments enable different package sets and configurations to run simultaneously, and avoid conflict due to inconsistent package versions.
$ python3 -m venv env
You'll also need to activate your virtual world. The command to do that will vary depending on your operating system and the shell you're working with. Below are CLI activation examples that work in a variety of different environments:
# On Unix or MacOS (bash shell):
/path/to/venv/bin/activate
# On Unix or MacOS (csh shell):
/path/to/venv/bin/activate.csh
# On Unix or MacOS (fish shell):
/path/to/venv/bin/activate.fish
# On Windows (command prompt):
\path\to\venv\Scripts\activate.bat
# On Windows (PowerShell):
\path\to\venv\Scripts\Activate.ps1
(Some Python-aware IDEs are also able to be set to activate the current virtual environment.)
Then, download FastAPI:
$ pip3 install fastapi
The framework FastAPI can be used for creating APIs. However, to evaluate your APIs, you'll require an internet connection locally. Uvicorn is a lightning-fast Asynchronous Server Gateway Interface (ASGI) web server that runs Python which is perfect for development. For installation of Uvicorn, run this command:
$ pip3 install "uvicorn[standard]"
Following successful installation, you can make a new file called main.py within your application's directory of work. The file is your entry point into your application.
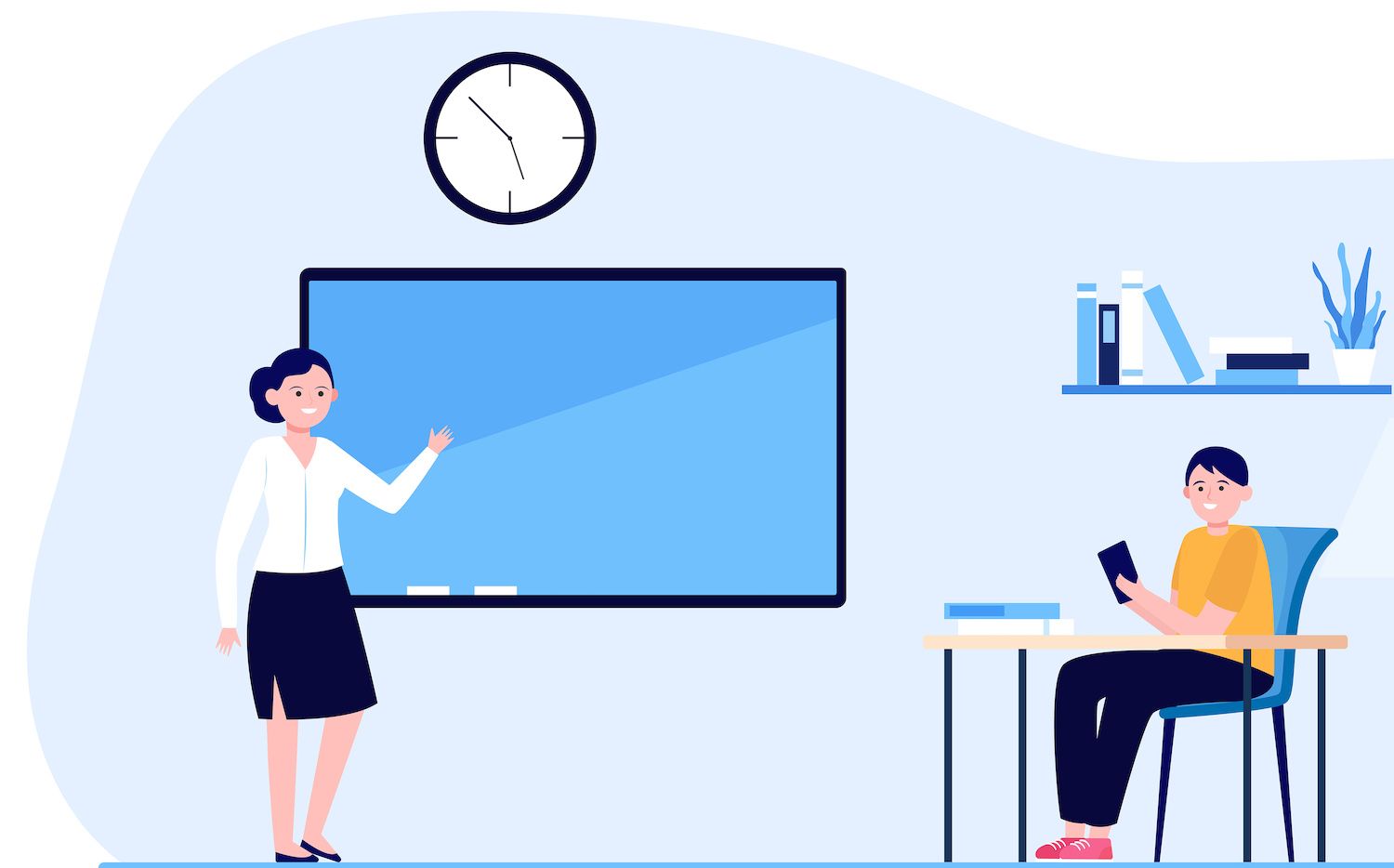
A Fast FastAPI Example
You will test your FastAPI installation by swiftly configuring an example endpoint. In the main.py file, insert the following code. Finally, save the file
# main.py
by importing fastapi FastAPI
application = FastAPI()
FastAPI()@app.get ("/")
async root() Return "greeting":"Hello world"
The above snippet creates an initial FastAPI endpoint. The following is an overview of what each line does:
from Fastapi import FastAPI
: The function of your API is provided via the FastAPI Python class.app is FastAPI()
: This creates the FastAPI instance.@app.get ("/")
: This is a python decorator that specifies to FastAPI it is the task that follows it is in charge of processing requests.@app.get ("/")
: This is a decorator that specifies the route. This creates aGET
method on the site's route. The results are then returned via the wrap function.- Other operations that could be utilized to send messages could include @app.post(), @app.put(), @app.delete(), @app.options(), @app.head(), @app.patch(), and @app.trace().
In the files directory, run the following command from your terminal, to launch the API server:
$ uvicorn main:app --reload
In this command, main
is the module's name. The app
object represents an instance of your program that is loaded to the ASGI server. The --reload
flag tells the server that it should reload when you make any changes.
It is possible to see something similar to this on your terminal
$ uvicorn main:app --reload
INFO: Will watch for changes in these directories: ['D:\\WEB DEV\\Eunit\\Tests\\fast-api']
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
INFO: Started reloader process [26888] using WatchFiles
INFO: Started server process [14956]
INFO: Waiting for application startup. The application has started successfully.
In your browser, navigate to http://localhost:8000
to confirm that your API is working. It should show "Hello": "World" as a JSON object displayed on the page. This shows how simple it is to develop an API with FastAPI. All you had to do was create an API route, and then return the Python dictionary as shown in the sixth line of the snippet above.
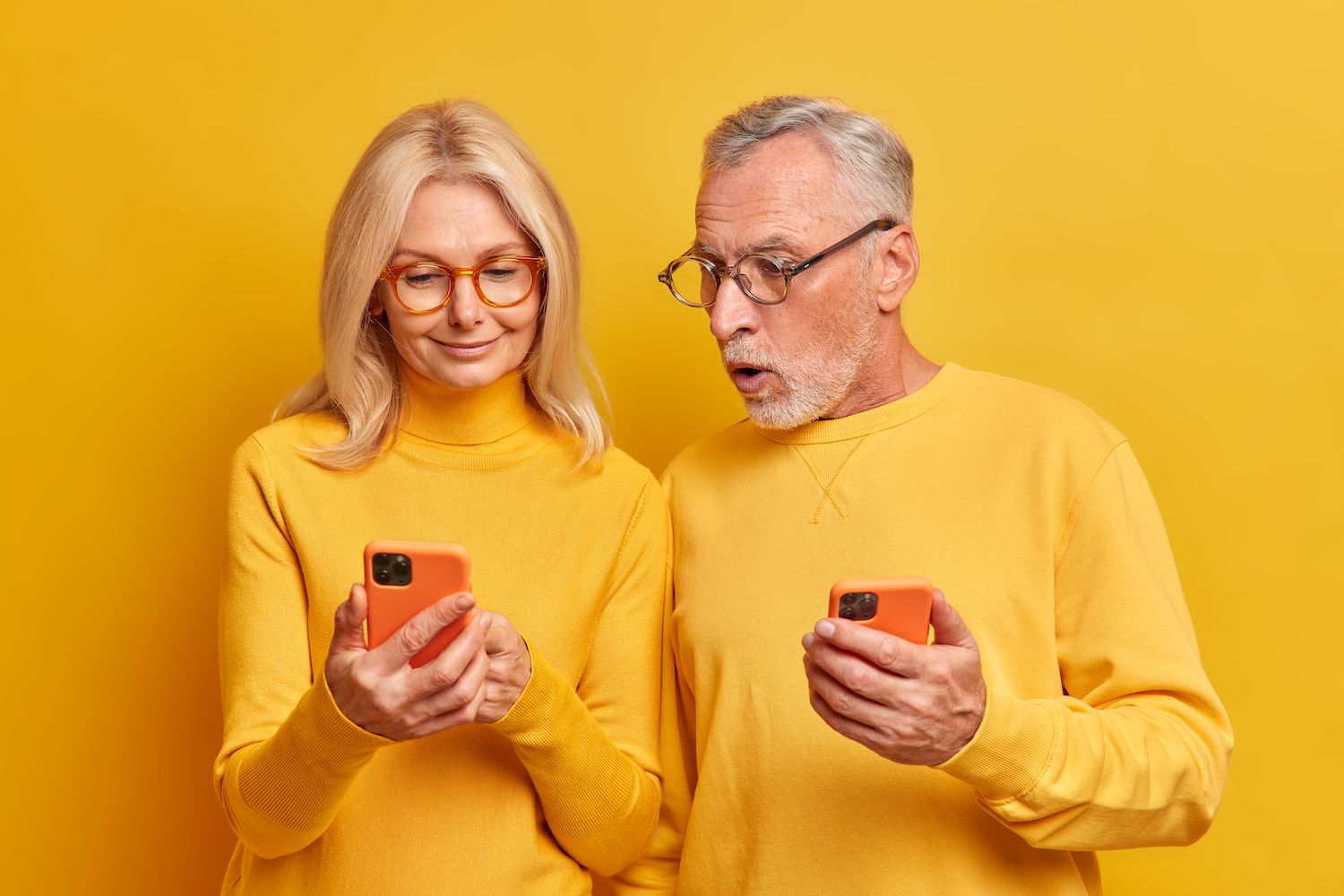
Using Type Hints
If you're using Python then you're used to annotating variables with simple data types like int
, str
, float
, and the bool
. In Python version 3.9 new advanced data structures were introduced. This allows you to work on data structures, such as dictionaries
, tuples
, and list
. With FastAPI's type hints allows you to organize the data schema using Pythonic models. Then, utilize the pydantic model to provide hints for typing and benefit of the validation for data that is provided.
In the below example you will see how the usage of types of hints within Python is illustrated using an easy meal cost calculator, calculate_meal_fee
:
def calculate_meal_fee(beef_price: int, meal_price: int) -> int:
total_price: int = beef_price + meal_price
return total_price
print("Calculated meal fee", calculate_meal_fee(75, 19))
Be aware that type hinting does not change how your code is executed.
FastAPI Interactive API Documentation
FastAPI uses Swagger's user interface to offer automatic, interactive documentation for APIs. To access it, navigate to http://localhost:8000/docs
and you will see a screen with all your endpoints, methods, and schemas.
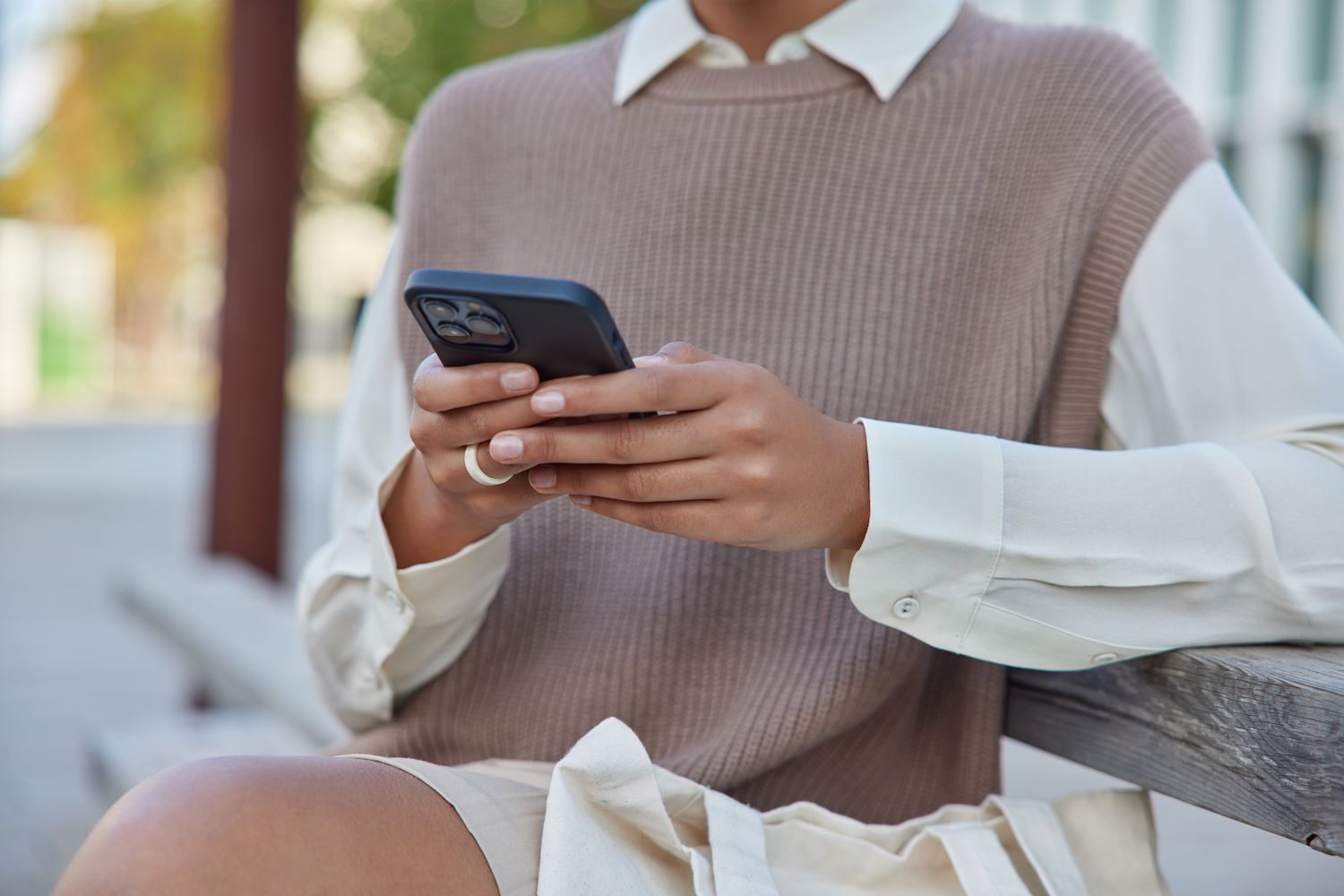
This browser-based, automatic API document is offered by FastAPI. It is free, so you don't need to do anything else to benefit from it.
A different API documentation that is accessible via a browser which is provided by FastAPI, can be found in Redoc. To access Redoc, navigate to http://localhost:8000/redoc
, where you will be presented with a list of your endpoints, the methods, and their respective responses.
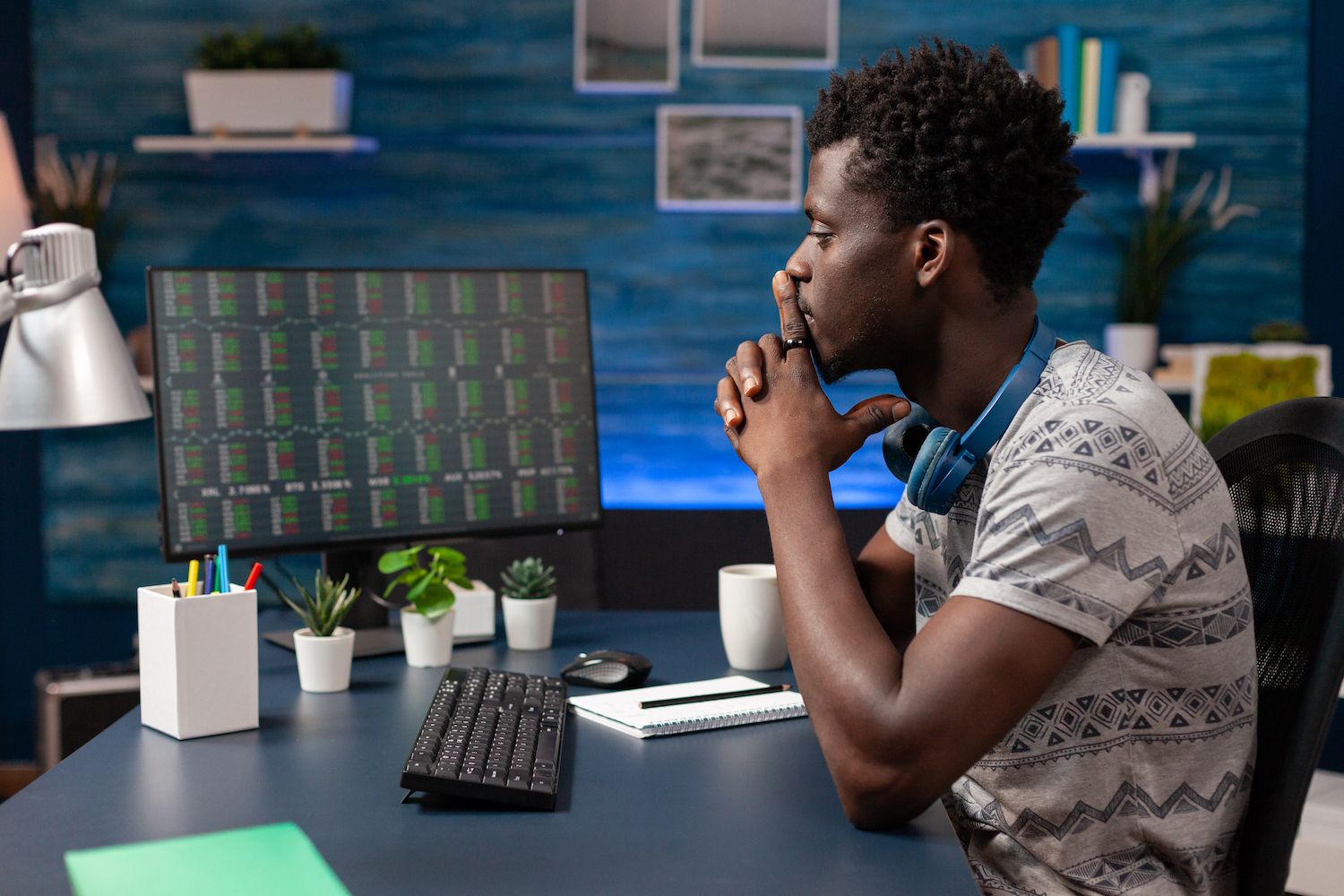
Setting Up Routes in FastAPI
The decorator for @app lets you to specify the way to use the route like @app.get or @app.post, and supports GET, POST, PUT, and DELETE, along with more obscure options, PATCH, HEAD and TRACE.
Building Your App With FastAPI
In this course we'll walk you through building a CRUD application using FastAPI. This application is able to:
Do you want to know what we did to increase our volume by more than 1000%?
Join 20,000+ others who get our weekly newsletter with insider WordPress tips!
- Create a user
- Access a database entry of a user
- Update for an existing user
- Remove a specific user
In order to execute these CRUD actions, you will create ways to expose API endpoints. It will create an in-memory data store that will keep a user list.
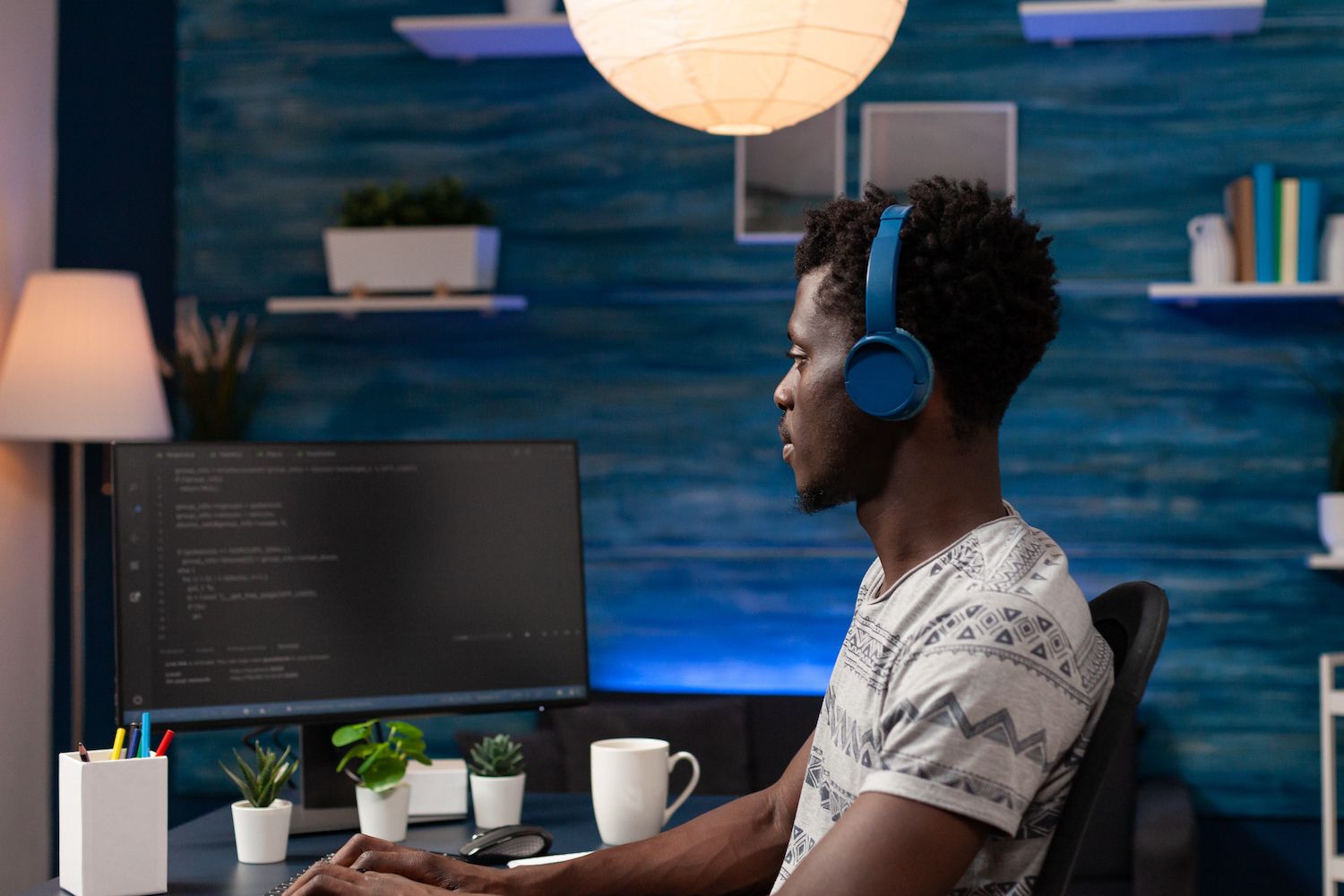
You'll use the Python library to perform the validation of data and manage settings by using Python annotations of type. To complete this course, you'll define the data's shape by defining classes and attributes.
The building of the App
Start by creating an account model for your users. The model of the user will include the following attributes:
id
: A Universal Unique Identifier (UUID)first_name
: The initial name given to the userlast_name
The name that is the last of the usergender
is the person's gender.roles
, which is an array ofroles for admin
androles for users.
roles
Create a new file called models.py in your working directory, then paste the following code into models.py to create your model:
In the code above:
- The
user
class is an extension ofBaseModel
that is transferred fromPythonic.
. - You defined the attributes of the user, as discussed earlier.
The next step is creating your database. Change the content of the main.py file with the following code:
# main.py
from typing import List
from uuid import uuid4
from fastapi import FastAPI
from models import Gender, Role, User
app = FastAPI()
db: List[User] = [
User(
id=uuid4(),
first_name="John",
last_name="Doe",
gender=Gender.male,
roles=[Role.user],
),
User(
id=uuid4(),
first_name="Jane",
last_name="Doe",
gender=Gender.female,
roles=[Role.user],
),
User(
id=uuid4(),
first_name="James",
last_name="Gabriel",
gender=Gender.male,
roles=[Role.user],
),
User(
id=uuid4(),
first_name="Eunit",
last_name="Eunit",
gender=Gender.male,
roles=[Role.admin, Role.user],
),
]
Within main.py:
- You created
db
by using the typeList
then passed it theUser
model - You created an in-memory database that has four users possessing the essential characteristics like
the first name, last name
,last_name
,gender
, as well asroles
. The Eunit userEunit
is given the role ofadmin
as well asuser
as well as user. The other three users are assigned only the position user.user
.
Read Database Records
The database has been set up successfully. your in-memory database and populated it with users, so the next step is to set up an endpoint that gives a complete listing of all users. This is the point where FastAPI is in.
Within your main.py file, paste the following code just beneath the Hello World endpoint. Hello World
endpoint:
# main.py
@app.get("/api/v1/users")
async def get_users():
return db
This code defines the endpoint users/api/v1/users
, and generates an async operation, get_users
, that returns the entire contents of the database db
.
Backup your data, then you can test your user endpoint. Run the following command in your terminal to launch the API server:
$ uvicorn main:app --reload
In your browser, navigate to http://localhost:8000/api/v1/users
. This should return an overview of all your users, as seen below:
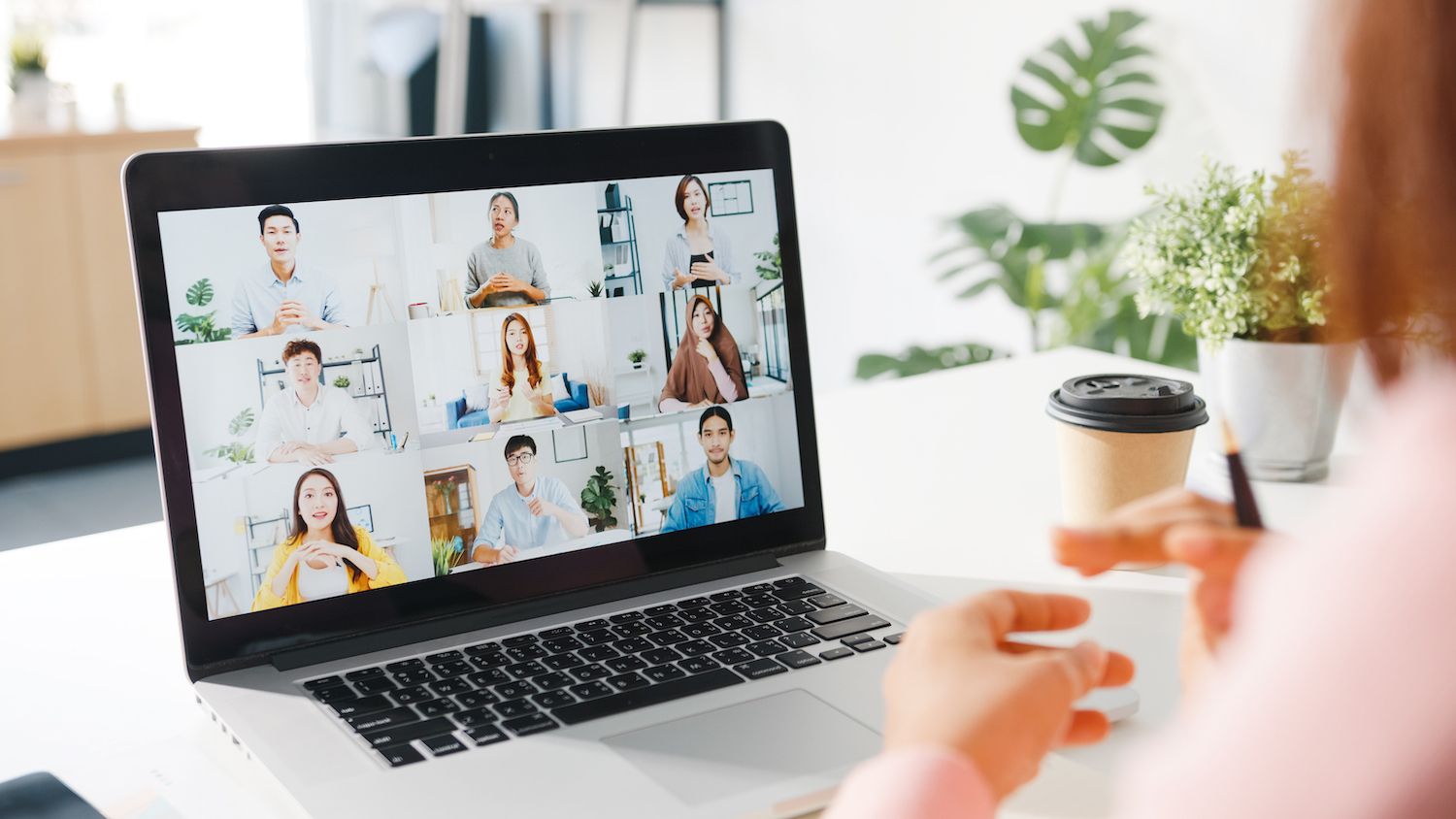
At this stage, the main.py file will appear like:
# main.py
from typing import List
from uuid import uuid4
from fastapi import FastAPI
from models import Gender, Role, User
app = FastAPI()
db: List[User] = [
User(
id=uuid4(),
first_name="John",
last_name="Doe",
gender=Gender.male,
roles=[Role.user],
),
User(
id=uuid4(),
first_name="Jane",
last_name="Doe",
gender=Gender.female,
roles=[Role.user],
),
User(
id=uuid4(),
first_name="James",
last_name="Gabriel",
gender=Gender.male,
roles=[Role.user],
),
User(
id=uuid4(),
first_name="Eunit",
last_name="Eunit",
gender=Gender.male,
roles=[Role.admin, Role.user],
),
]
@app.get("/")
async def root():
return "Hello": "World",
@app.get("/api/v1/users")
async def get_users():
return db
Create Database Records
The next step is to create an endpoint that will create a new user in your database. Incorporate the following text into the main.py file:
# main.py
@app.post("/api/v1/users")
async def create_user(user: User):
db.append(user)
return "id": user.id
In this code snippet it is clear that you set the goal to create a brand new user. You used an @app.post
decorator to generate the Post
method.
Also, you created the function create_user that accepts the users from the User model and appended (added) the newly-created user into the database db. The endpoint then provides a JSON object that contains the newly created user's id
.
You'll need to follow the automatic API documentation that is provided by FastAPI for testing your API endpoint, as seen earlier. This is because it is not possible to create a post-request using the web browser. Navigate to http://localhost:8000/docs
to test using the documentation provided by SwaggerUI.
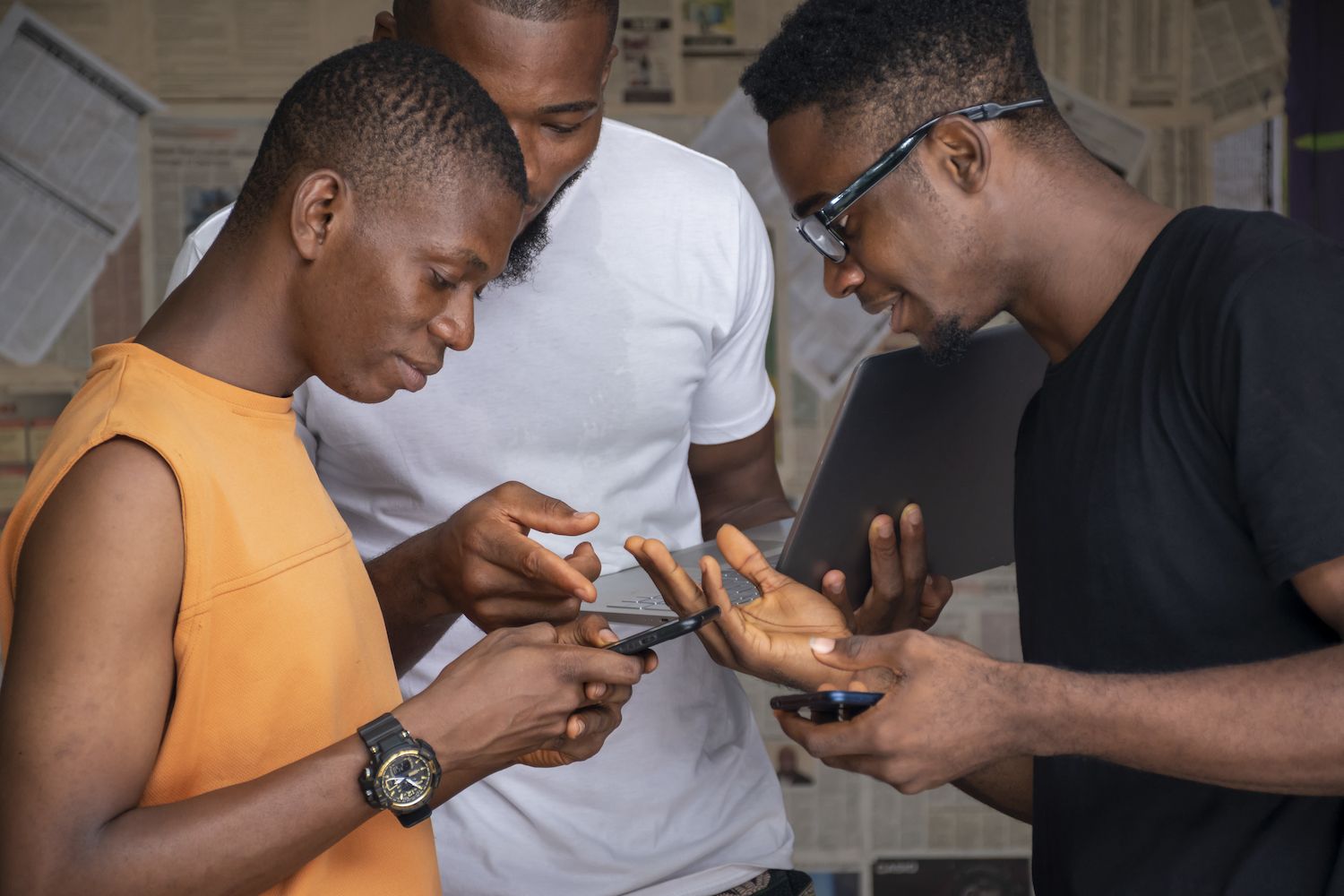
Removing Database Records
Since you're building an CRUD application the application you build will have to have the capability to remove a specified resource. This tutorial are creating an endpoint that will erase the user.
Incorporate the following code in your main.py file:
# main.py
from uuid import UUID
from fastapi HTTPException
@app.delete("/api/v1/users/id")
async def delete_user(id: UUID):
for user in db:
if user.id == id:
db.remove(user)
return
raise HTTPException(
status_code=404, detail=f"Delete user failed, id id not found." )
This is a breakdown of line-by-line how that code works:
@app.delete("/api/v1/users/id")
: You created the delete endpoint using the@app.delete()
decorator. It's still/api/v1/users/id
, but then it retrieves theid
, which is associated with the id of the user.async def delete_user(id UUID):
: Creates thedelete_user
function that retrieves theid
in the web address.for user's db:
: This tells the application to go through the users in the database and determine whether theid
that was retrieved matches with the person in the database.
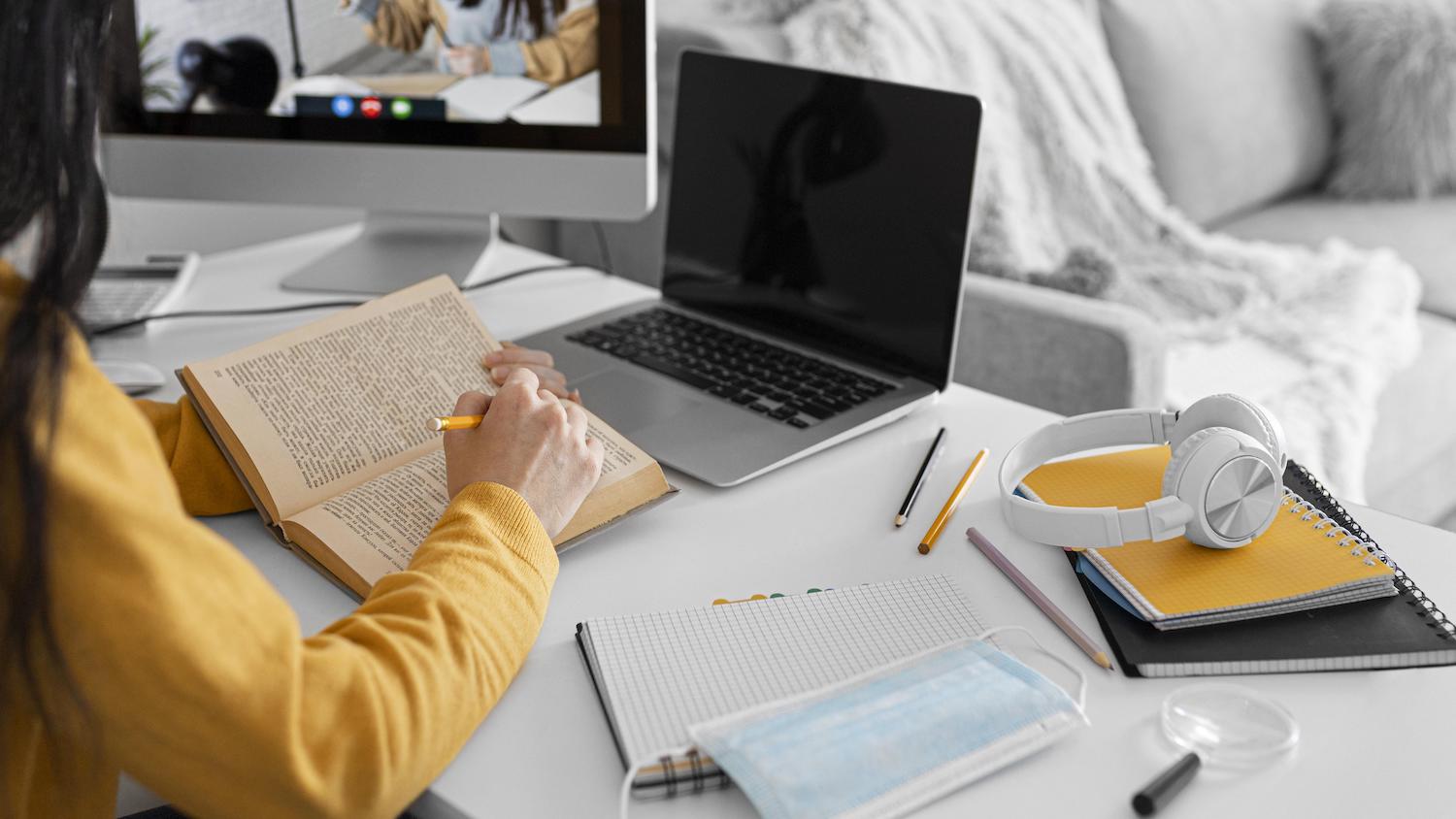
Update Database Records
You are going to create an endpoint to update a user's personal information. The details that can be updated comprise the below parameters: first_name
, last_name
as well as the roles
.
If you have a models.py file, add the following code to your user
model. This is after the User(BaseModel):
class:
# models.py
class UpdateUser(BaseModel):
first_name: Optional[str]
last_name: Optional[str]
roles: Optional[List[Role]]
In this example In this example, this Class UpdateUser
extends BaseModel
. Then, you set up the parameters for updatable users like initials
, last_name
as well as roles
that are optional.
Create an endpoint for updating a particular user's details. Within the main.py file, paste the following code after @app.delete
decorator:
# main.py
@app.put("/api/v1/users/id")
async def update_user(user_update: UpdateUser, id: UUID):
for user in db:
if user.id == id:
if user_update.first_name is not None:
user.first_name = user_update.first_name
if user_update.last_name is not None:
user.last_name = user_update.last_name
if user_update.roles is not None:
user.roles = user_update.roles
return user.id
raise HTTPException(status_code=404, detail=f"Could not find user with id: id")
In the above code, you've completed the following steps:
- Created
@app.put("/api/v1/users/id")
, the update endpoint. It is a variable parameterid
which is the id of the user. - A method was created called
update_user
that takes into UpdateUser class and ID.UpdateUser
class andID
. - Utilized as a
loop called a for
loop to determine if the account that is associated with theID
is in the database. - Verified that any of the user's parameters are
are not none
(not non-null). If some parameter, for examplenames, first_name
,last_name
, as well asroles
that are non-null, it will be updated. - If the operation goes well, the user id is given back.
- If the user isn't found in the database, an
HTTPException
warning with a status code of 404 and a message ofCould not find an individual with the ID: id
is raised.
To check this particular endpoint, be sure your Uvicorn server is running. If it is not running you can use this command:
uvicorn main:app --reload
Below is a screen shot of the test.
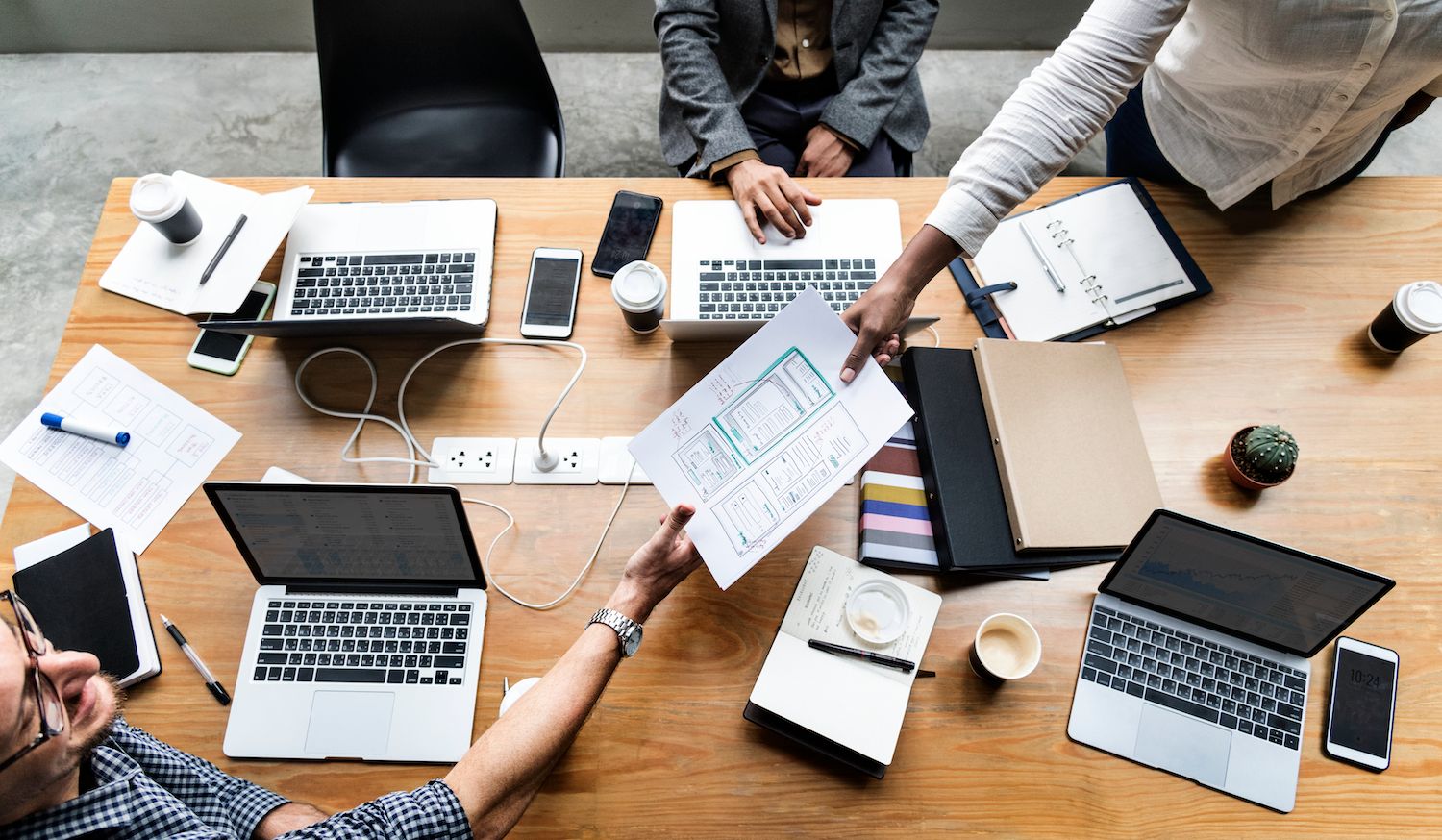
Summary
In this video you've been introduced to the FastAPI framework designed for Python and experienced how fast you could get an application using FastAPI running. It was a great opportunity to learn how to construct CRUD API endpoints using the framework which includes creating, reading, updating, and deleting database records.
Cut down on time, expenses and improve site performance by:
- Help is available immediately 24/7 support from WordPress experts in hosting, 24 hours a day.
- Cloudflare Enterprise integration.
- Global audience reach with 35 data centers worldwide.
- Optimization using the integrated Application Performance Monitoring.