Create a -Hosted To-Do List Using Jira API & React
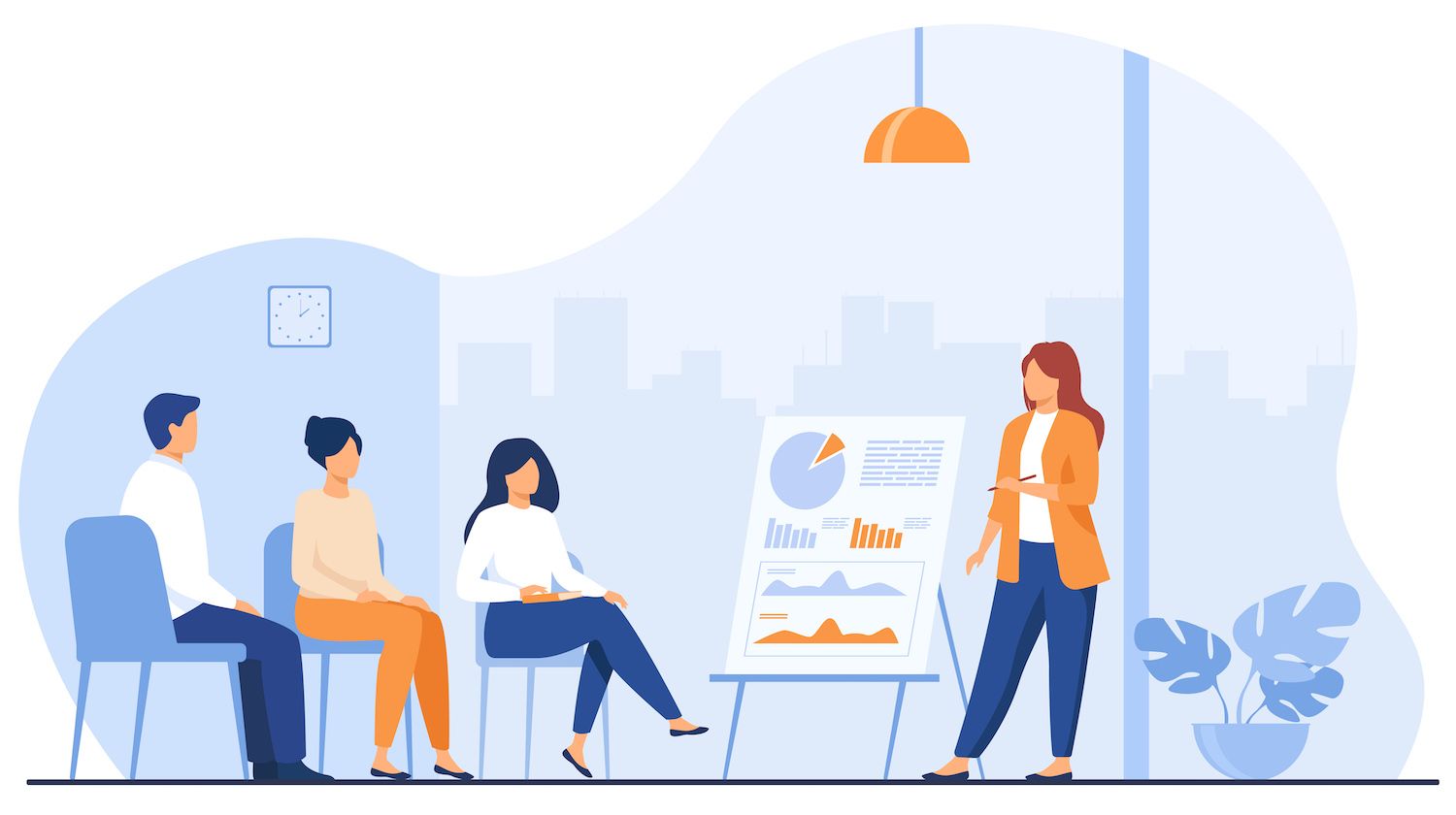
-sidebar-toc>
Jira is a popular project management tool that helps to keep track of the tasks within a project. When you are working on a big project, your Jira dashboard may become overloaded as the number of tasks and team members increases.
In order to address this issue To address this issue, use to address this issue, you can use the Jira REST API to build a simple To-Do List application that displays your tasks as well as their due dates. The API lets you programmedly interact with Jira to create, retrieve updates, delete, or create issues as well as view user information as well as project information.
The prerequisites
For following along, you need:
- Node.js and Node Package Manager (npm) installed on your machine for development.
- An account on Jira account that allows you to view the tasks.
How to build the backend Utilizing Node.js and Express
Follow these steps for setting up your server:
- Create a new directory and navigate to it. After that, you can start Node.js by running the command as follows:
NPM init"y"
This command generates an package.json file with default settings located at the root folder of your application's folder.
- Then, you must install the required dependencies needed to your project using the following command:
npm install express dotenv Axios
The command above installs the following:
express
is a simple Node.js framework to build APIs.- dotenv is a module which is loaded with
.env
variables toprocess.env
to let you securely access them. Axios
is a promise-based HTTP client for Node.js. The client is designed to allow API call to Jira.
- After the installation has been successful, create the .env The file should be located in the root your project. Add the
PORT
number:
PORT=3000
This is the port number that the server is listening to. It is possible to change it to any port you want.
- Create the index.js The file is located in the root folder and add the following code to load Express to your project. Create an instance of the Express application and then launch your server:
const express = require('express');
require('dotenv').config()
const app = express();
const PORT = process.env.PORT;
// Define routes here
app.listen(PORT, () =>
console.log(`Server is running on port $PORT`);
);
- In the final, you should include package.json File, include a script to start the server.
"scripts":
"start": "node index"
,
You can now run the start script in the terminal you are using:
NPM run begin
This command starts your server. You should see the following text logged in the terminal:
Server is running on port 3000
Once the server is up and in operation, you can configure the settings for your Jira application.
How To configure an Jira App
For access to the Jira REST API requires you to create an account user with your Jira website. The to-do app API you build uses simple authentication using the Atlassian account email address and API token.
This is how you can set it up:
- Create a Jira login or login when you already have one.
- Navigate to your Security section on your Atlassian profile, and then select the Create API token button..
- In the dialogue that opens you will be asked to enter a label to identify your token (e.g., "jira-todo-list") and then click Create.
- Copy the token to your clipboard.
- Then, save the API token in your .env file:
JIRA_API_TOKEN="your-api-token"
Now, you can use the Jira API using basic authentication.
Create Routes to Fetch Issues From Jira
Now that you have configured a Jira application. Set up the routes that fetch issues from Jira within your Node.js server.
In order to initiate a request through the Jira API, you must use your Jira API token you saved within the .env file. Retrieve the API token using process.env
and assign it to a variable named JIRA_API_TOKEN
within your index.js file:
const JIRA_API_TOKEN = process.env.JIRA_API_TOKEN
Now, you need to specify the endpoint URL to your API request. This URL is comprised of your Jira domain, as well as a Jira Query Language (JQL) statement. The Jira domain is a URL for your Jira organisation and appears like org.atlassian.net
, where the word "org"
is the name of your company. JQL, on the other hand is a query language for interacting with problems in Jira.
The first step is to add your Jira domain in your .env file:
JIRA_DOMAIN="your-jira-domain"
It is also necessary to save your Jira email in your .env file as it would be used for the authorization needed when you submit a request to Jira:
JIRA_EMAIL="your-jira-email"
Then, you must add the two environment variables. Then, build the endpoint URL by using the domain and the next JQL query. This query filters issues with "In the process" or "To complete" statuses of the user. It then sorts them in order of status.
const jiraDomain = process.env.JIRA_DOMAIN;
const email= process.env.JIRA_EMAIL;
const jql = "status%20in%20(%22In%20progress%22%2C%20%22To%20do%22)%20OR%20assignee%20%3D%20currentUser()%20order%20by%20status";
const apiUrl = `https://$jiraDomain/rest/api/3/search?jql=$jql`;
Before creating a route, you must also add Axios into your index.js file:
const axios = require("axios")
You can now create a route create a GET request to the Jira API to return issues. In index.js add the below code:
app.get('/issues/all', async (req, res) =>
)
Use the axios.get
method to make a GET request to Jira's Jira REST API. Create an Authorization header. authorization
header using base64 encryption of your Jira email as well as API token:
const response = await axios.get(apiUrl,
headers:
Authorization: `Basic $Buffer.from(
`$email:$JIRA_API_TOKEN`
).toString("base64")`,
Accept: "application/json",
,
);
Await the response from the Jira API and store it as an array of variables. The response contains the property issues
and it contains an array of issues objects:
const data = await response.json();
const issues = data;
Then, you'll go through the issues
array, extract only pertinent information regarding the items to be completed, and send it back in JSON response:
let cleanedIssues = [];
issues.forEach((issue) =>
const issueData =
id: issue.id,
projectName: issue.fields.project.name,
status: issue.fields.status.name,
deadline: issue.fields.duedate,
;
cleanedIssues.push(issueData);
);
res.status(200).json( issues: cleanedIssues );
If you make a request to http://localhost:3000/issues/all
, you should receive a JSON object containing the issues:
curl localhost:3000/issues/all
You can even take this further by using a provider like SendGrid and a cron job to deliver daily emails with your assigned tasks.
Host Your Node.js Application on
- Install the cors npm package By running this command on your terminal:
npm install cors
- Once you have imported the package, it can be opened then, you can import the package index.js .
const cors = require('cors')
- Then, you can configure CORS as a middleware, enabling it to handle every request that comes in. Add the following code at the beginning of your index.js file:
app.use(cors());
Now you can send HTTP requests to your server via an alternative domain and not encounter CORS errors.
- Log in or create an account to see your My dashboard.
- Authorize your Git service provider.
- Select Applications on the left sidebar, and then click Add application.
- Choose the repository you want to deploy from and also the branch you would like to make available from.
- Create a unique brand name for your app and choose an appropriate data center place.
- Add the environment variables. It is not necessary to include the PORT as an environment variable, as does it in a way that is automatic. Make sure to check the boxes beside Available during runtime and Available during build process :
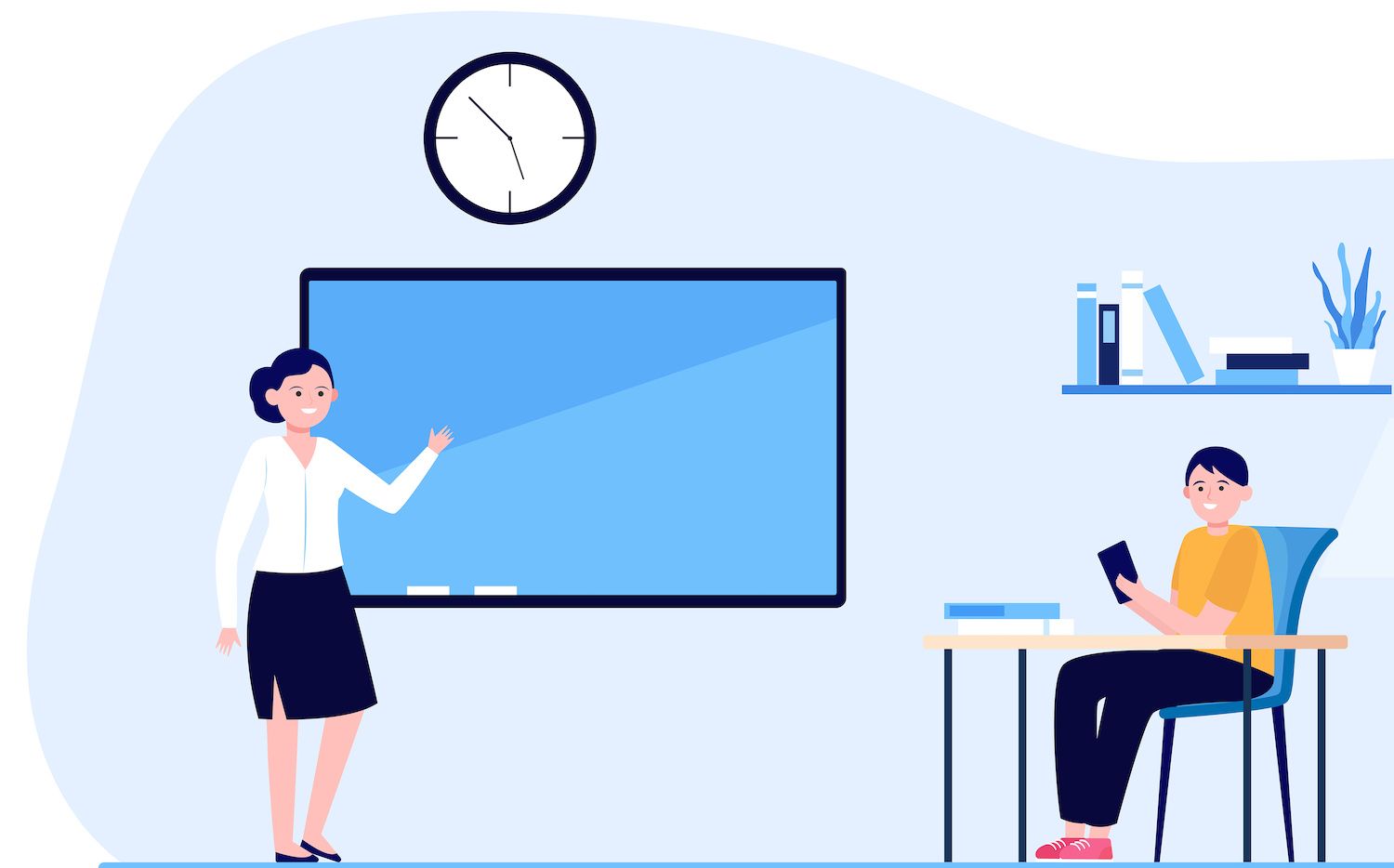
- Review other information (you may change the defaults) and click to create an application.
The server has been successfully deployed to . In the menu on the left, click Domains and copy the domain that you want to use as your primary. This is your server's URL endpoint.
Develop a React application that displays the problems
- A fresh React project called
jira-todo
:
npx create-vite jira-todo --template react
- Navigate to the project directory and install the necessary dependencies:
Install npm
- Begin the development server
npm run dev
Issues with Fetching from the Server
- Remove the contents of App.jsx and add the below code:
function Application() *;
the default app to export;
- Before you can begin fetching issues, you must save the URL for your server inside a .env file at the root of your app's directory:
VITE_SERVER_BASE_URL="your-hosted-url"
- Get the URL from App.jsx by adding this line to the very top of the file:
const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL
- In your component, create an async function that is named
fetchData
and then send a GET call to the/issues/all
endpoint on the Express server. If you are able to receive an answer, you need to parse it as JSON and store the data as a state value calleddata
:
import useCallback, useEffect, useState from "react";
function App()
const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL
const [data, setData] = useState([]);
const fetchData = useCallback(async () =>
try
const response = await fetch(`$SERVER_BASE_URL/issues/all`);
if (!response.ok)
throw new Error('Network response was not ok');
const data = await response.json();
setData(data.issues);
catch (error)
console.error('Error fetching data:', error);
,[SERVER_BASE_URL]);
useEffect(() =>
// Fetch data when the component mounts
fetchData();
,[fetchData]);
return (
What's on my list today?
);
export default App;
Be aware that you must use the useEffect
hook to perform the fetchData
function whenever the component starts to mount.
Display the issues from Jira in the Browser
- Then, you are able to modify the return statement of the component so that it runs through the problems and display these in the browser.
return (
What's on my list today?
data && data.map(issue =>
return
issue.summary
issue.deadline
className="status">issue.status
)
);
- For styling this app to your liking, you can add the below CSS code into App.css:
h1
text-align: center;
font-size: 1.6rem;
margin-top: 1rem;
section
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
margin-top: 2rem;
.issues
display: flex;
min-width: 350px;
justify-content: space-between;
padding: 1rem;
background-color: #eee;
margin-bottom: 1rem;
small
color: gray;
.status-btn
padding: 4px;
border: 1px solid #000;
border-radius: 5px;
- After that, you import App.css in index.js to apply the styles:
import './App.css'
Now, when you start your application, you will see a list of tasks assigned to you with their status and deadline within your browser.
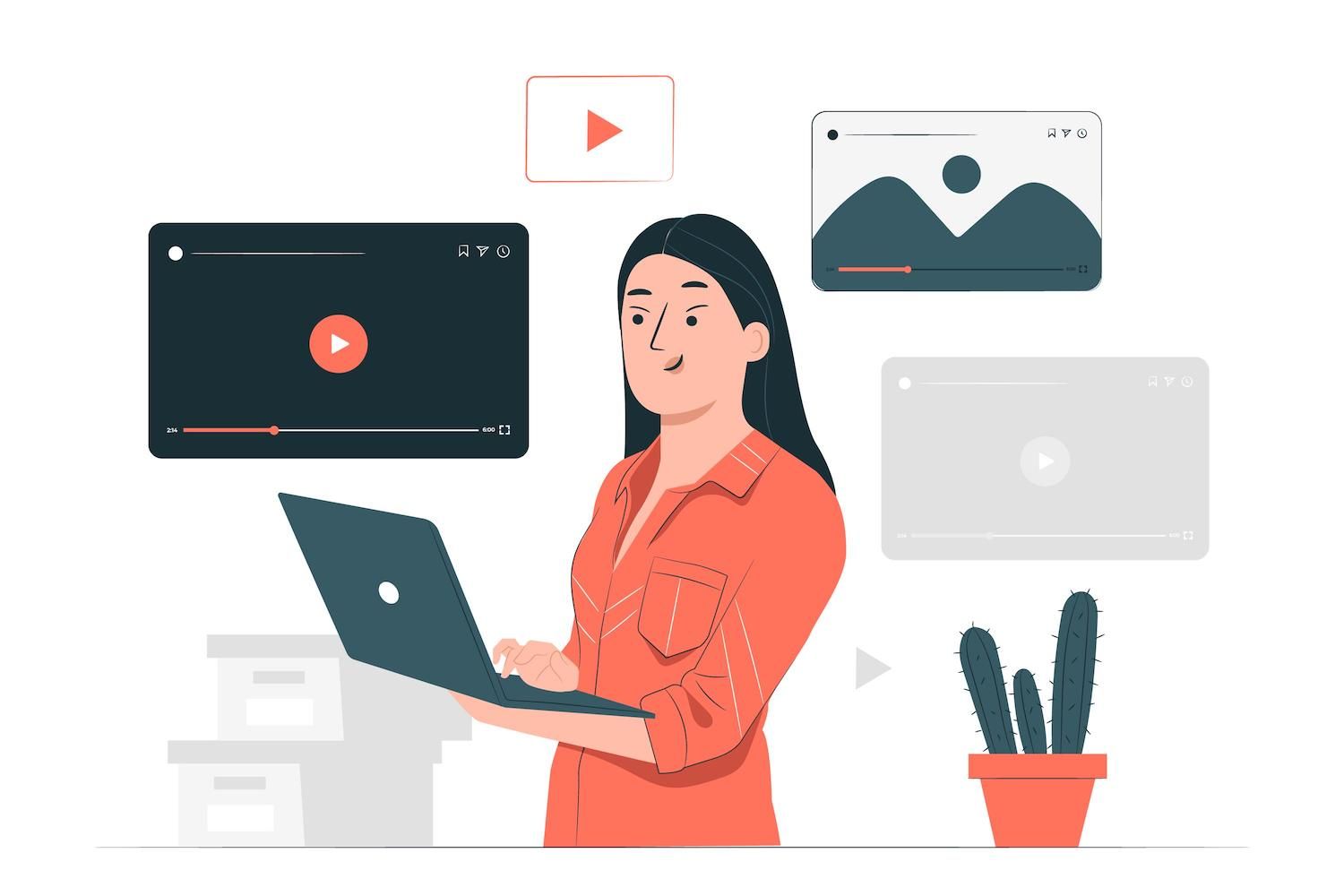
You can deploy the application on
- Sign in or create an account in order to access the My dashboard.
- Authorize Git with the service you use.
- Select Static Sites on the left sidebar and then select Add Site. Add site.
- Choose the repository you want to deploy from and also the branch you wish to use for deployment.
- Assign a unique name to your site.
- My detects the build settings of the React Project automatically. You see the following settings prefilled:
- Builder command
NPM run build
- Node version:
18.16.0
- Publish directory:
dist
- Add the URL of your server as an environment variable using
VITE_SERVER_BASE_URL
. - Then you can click to create your site..
Summary
In this tutorial in this guide, you will learn how you can create an Express app that retrieves identified Jira problems using Jira's Jira REST API. Additionally, you connected an React frontend application to your Express application in order to show those issues within the browser.
This app is a rudimentary illustration of the possibilities you do with the Jira REST API. It is possible to enhance the app with functionality that lets you make notes of completed tasks, carry out sophisticated filtering and more.
Jeremy Holcombe
Content and Marketing Editor at , WordPress Web Developer, and Content writer. Outside of everything related to WordPress I love the beach, golf, and watching movies. Also, I have height difficulties ;).