Design and Launch an Node.js app in five minutes using Express - (r)
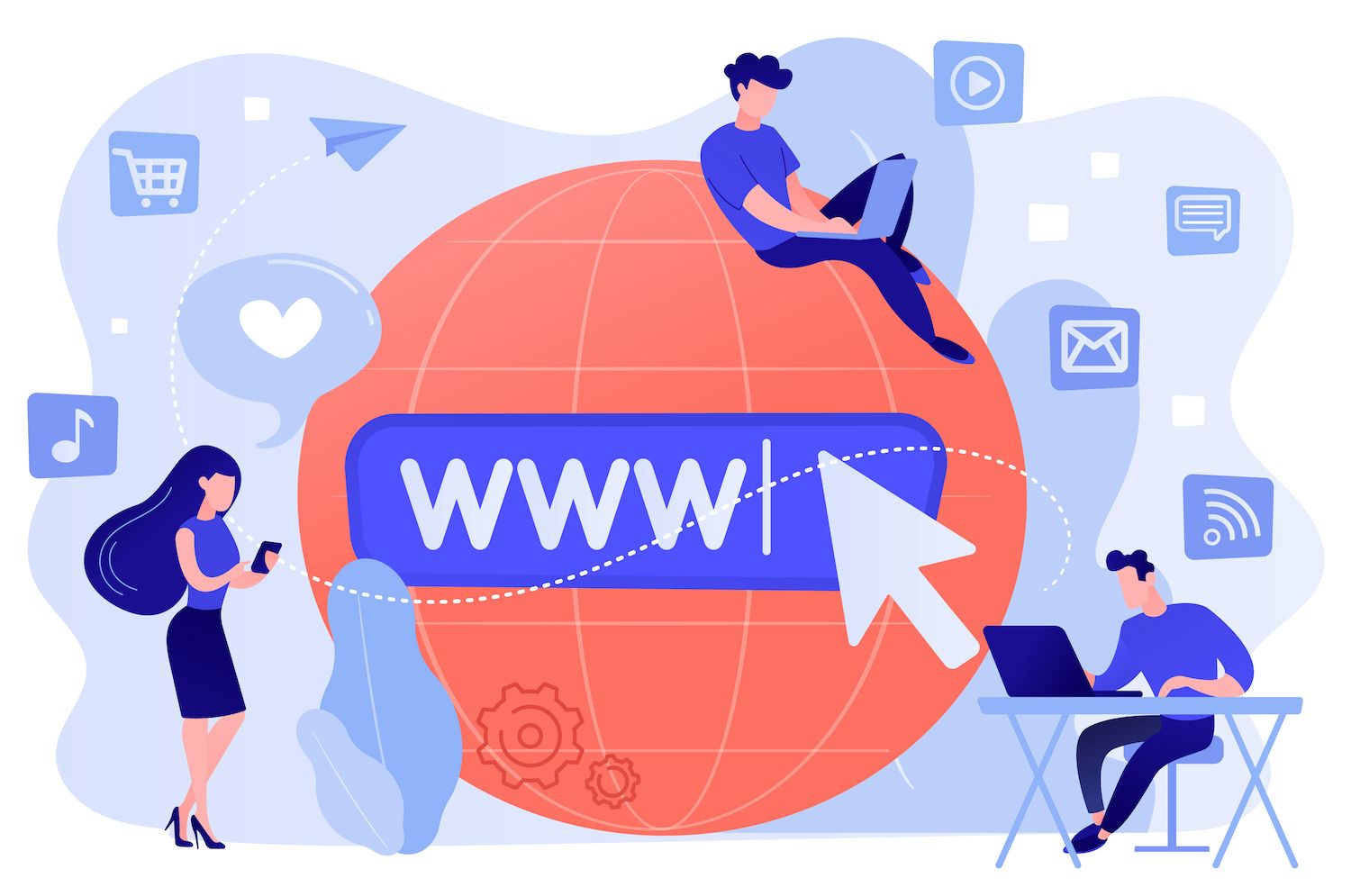
-sidebar-toc>
Express The world's most used Node.js framework lets developers develop backend web servers using JavaScript. The framework offers the majority of the backend development tools needed out of the box making routing easier and faster in answering web-based requests.
How To Make Apps Quickly By Using Express.js
This tutorial demonstrates how you can build a web-based application that accepts queries to an endpoint. It then utilizes a parameter from the request to make an SQL database call, then gives the details from the database in JSON.
The prerequisites
To follow this tutorial be sure to are running the following software on your PC:
Express Application Generator
Express.js is the official Express generator by Express.js is a Node package that allows you to generate a an entirely new application skeleton. You can do this through the creation of a folder for your application after which you can run the the npx
command (available within Node.js 8.2.0):
mkdir express-application
npx express-generator
After successful generation after successful generation, the terminal shows the list of files and folders that have been created, along with commands to install dependencies, and then running the program. Install the dependencies by running the following command:
npm install
Next, launch your web server:
DEBUG=myapp:* npm start
The skeleton application comes with an pre-built index route that displays a standard homepage. It is possible to view it within your browser by going to http://localhost.3000
.
Investigating how to use the Skeleton Express Application
When you open your Express application in your preferred code editor, you'll discover a fundamental structure that creates the basis of your website application.
/
|-- /node_modules
|-- /public
|-- /routes
|-- index.js
|-- users.js
|-- /views
|-- error.jade
|-- index.jade
|-- layout.jade
|-- app.js
|-- package.json
- node_modules This directory contains all dependencies that are installed as well as libraries for the project.
- public: Contains static assets like CSS, JavaScript, images, etc. The files are directly served to a browser on the client.
- routes holds files that are responsible for defining various routes and handling requests coming from different URLs.
- view: Contains templates or views that the server renders in order to build the user interface. In this case, error.jade, index.jade, and layout.jade are templates that are written in the Jade the templating language. They help structure and render dynamic content to users.
- app.js: This is typically the entry point for an Express application. It's where the server is installed and middleware is installed, routes are defined in the app.js file, and both requests and replies are processed.
- package.json: This document contains information about the app. It helps manage dependencies and project configuration.
Understanding Route Handling
In your Express application In your Express application, the routes directory contains the locations where routes are defined as separate files. The first route, usually known as the index route is located in the routes/index.js file.
This route handles an GET
request and responds with a web page generated with HTML using the framework. Below is the code snippet illustrating the way an GET
request will be processed to render a basic welcoming page.
var express is require('express');
variable router = express.Router();
* GET the homepage. */
router.get('/', function(req, res, next)
res.render('index', title: 'Express' );
);
module.exports = router;
If you change this res.render()
function to res.send()
, the response type changes between HTML to JSON
expression = require('express');
var router = express.Router();
Express.Router()router.get ('/', function(req, new) (req, res, next)res.send( key: 'value' );
);module.exports = router
To expand the possibilities, a new option is added to the identical file, which introduces the possibility of a new destination that can accept a parameter. This code sample shows how your application can handle the traffic from a different endpoint, and extract a parameter, and respond by storing its value in JSON:
/* GET a new resource */
router.get('/newEndpoint', function(req, res, next)
res.send( yourParam: req.query.someParam );
);
Sending a GET
request to localhost:3000/newEndpoint?someParam=whatever
will yield JSON output containing the string "whatever".
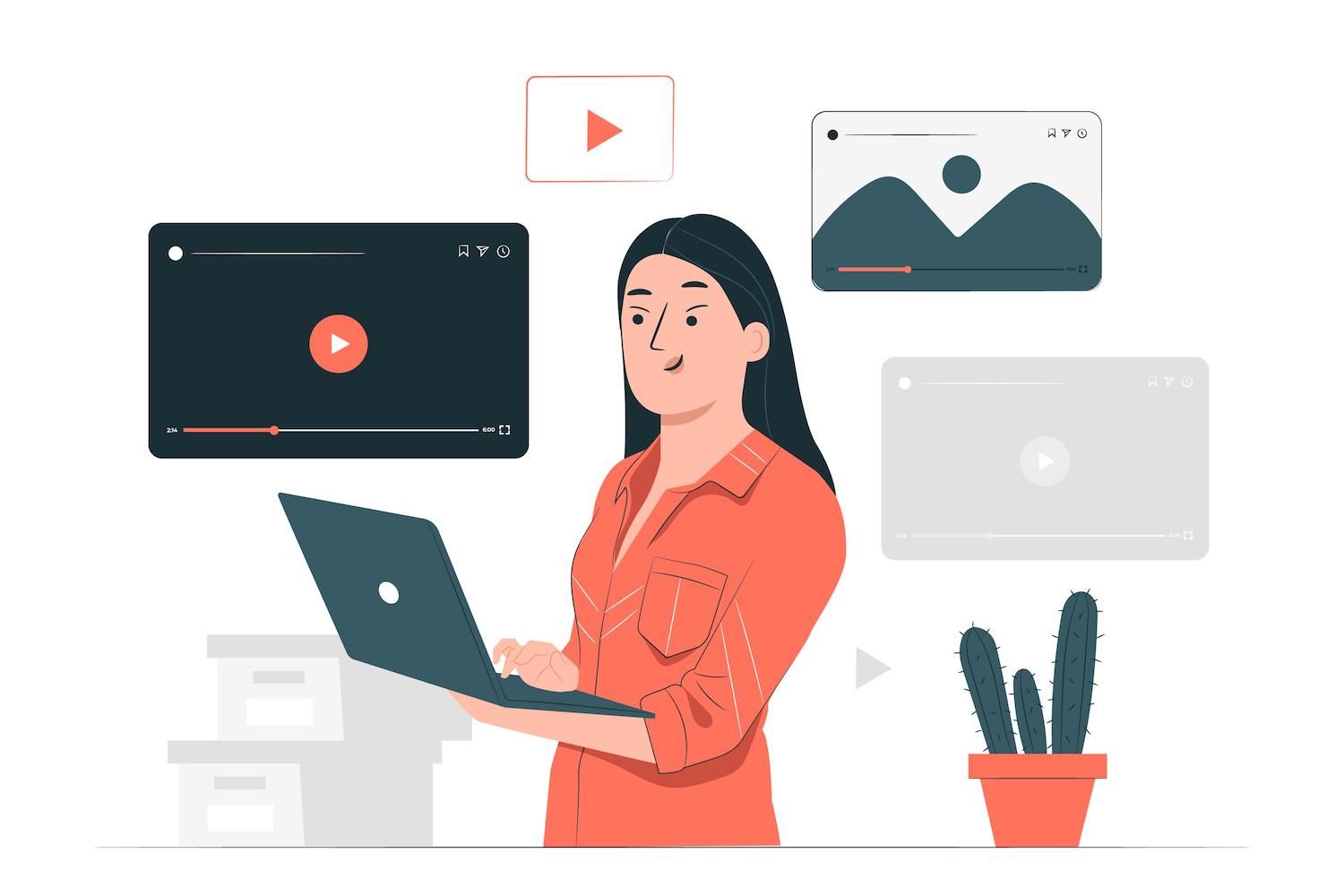
Application and Express Hosting
Making web requests from your computer to your laptop is cool however, web development won't be finished until you've got off localhost. It is a good thing that it makes the deployment of applications on the internet easy, even if you need a database.
Let's begin by the capabilities of your application by integrating database functionality and deploying both your application and database on the internet to allow access from any computer.
Once your repository is set, follow these steps to create your Express application in :
- Log in or create an account to view Your dashboard. My dashboard.
- Authorize Git with the service you use.
- Click Applications on the left sidebar, and then click Add a new application.
- Choose the repository you want to deploy from and also the branch that you want to deploy from.
- Create a unique name for your application and select a Data center location.
- Make use of all the default settings and select to create the application.
Works seamlessly with integrates with Express application generator straight from the beginning! Once you complete these steps, the application will start the creation and deployment process.
The screen that will be used to deploy your application will display an URL that will allow you to deploy the application. You can append /newEndpoint?someParam=whatever
to test out the endpoint built in the previous section of this article.
How to Include a Database in Express Application
Here's how you can build a database on :
- Navigate through The Databases section on the sidebar of My's dashboard.
- Click on the Create Database button. Configure your database details by entering a name and selecting the database type.
- Select the PostgreSQL option. A Database username and password It is generated automatically:
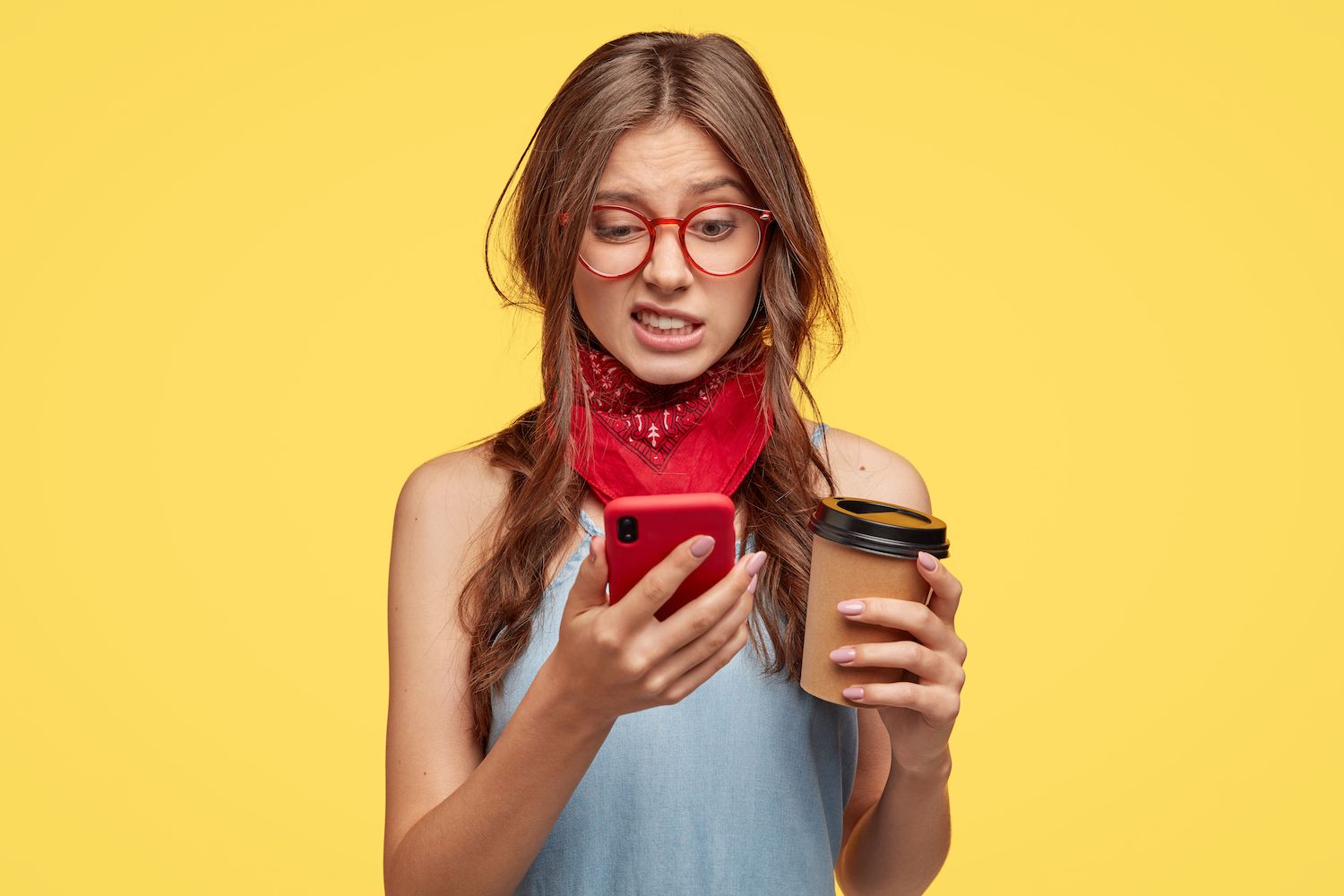
- Choose the exact Data center site where you hosting your Express application. Configure your desired size.
- Confirm payment information and select on Create Database.
When the database is successfully created
- You can access the database's details through clicking the link. In the Overview page, click on the internal connection section.
- Select the appropriate application.
- Check the option to include environment variables in this application.
- Click Add connection to join the newly created database and your application.
After that take a copy of the connection string for the new database and connect to it with the database software. Any SQL connection tool can be used, but this demonstration uses Beekeeper. Start the application, click Import from URL, insert the connection string and click Import. You can then run SQL in the database you just created.
Next, create an elementary table with a single record by executing a couple of SQL statements against the database hosted by the tool for creating databases:
CREATE TABLE "States"
( state_pk ID INTEREST CONSTRAINT PRIMARY KEY,
state_name varchar(100),
capital varchar(100),
state_bird varchar(100),"createdAt" TimestampTZ NOT NULL DEFAULT ANYMORE(),"updatedAt" TIMESTAMPTZ IS NOT Null DEFAULT Now()"updatedAt" TIMESTAMPTZ NOT NULL DEFAULT NOW) INSTALL INTO "States"
INTO "States"VALUES(1 1, 'ohio "columbus", "cardinal");
Add the following database packages into your project:
NPM install sequelize pg
This sequelize
dependency functions as an ORM for Node.js, and it
is the PostgreSQL client that allows interaction between Node.js applications as well as PostgreSQL databases.
After that, create the program that receives a GET
request with an id
parameter and returns the information within the database that is associated with the id
. To do so, alter your index.js file accordingly:
var express = require('express');
var router = express.Router();
const Sequelize, DataTypes = require('sequelize');
const sequelize = new Sequelize(process.env.CONNECTION_URI,
dialect: 'postgres',
protocol: 'postgres',
);
const State = sequelize.define('State',
// Model attributes are defined here
state_name:
type: DataTypes.STRING,
allowNull: true,
unique: false
,
capital:
type: DataTypes.STRING,
allowNull: true,
unique: false
,
state_bird:
type: DataTypes.STRING,
allowNull: true,
unique: false
,
,
// Other model options go here
);
async function connectToDB()
try
sequelize.authenticate().then(async () =>
// await State.sync( alter: true );
)
console.log('Connection has been established successfully. ');
catch (error)
console.error('Unable to connect to the database:', error);
connectToDB();
/* GET a new resource */
router.get('/state', async function(req, res, next)
const state = await State.findByPk(req.query.id);
if (state)
res.send(state)
else
res.status(404).send("state not found");
);
/* GET home page. */
router.get('/', function(req, res, next)
res.render('index', title: 'Express' );
);
/* GET a new resource */
router.get('/newEndpoint', function(req, res, next)
res.send( yourParam: req.query.someParam );
);
module.exports = router;
Change the code, commit them and push the changes to your Git repository. Then, proceed to deploy manually or wait for the automatic deployment.
When you access the /states
endpoint using ID=1
and you'll be able to retrieve states from the database.
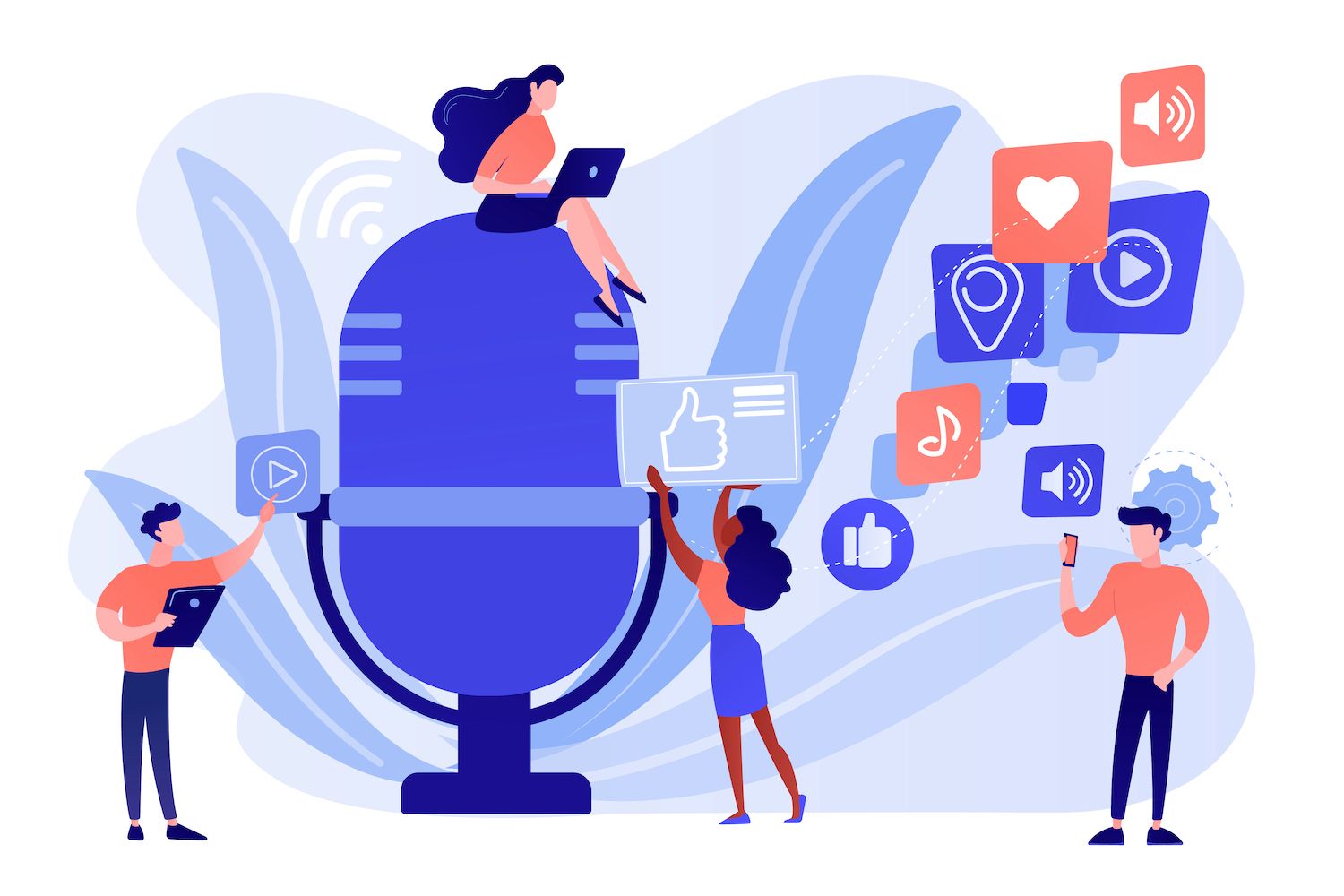
That's all there is to it! Check out the complete project code via GitHub.
Summary
The power and ease of using the Express framework for Node.js development of applications are huge. By using the framework, you are able to carry the momentum Express and Node.js give you into the implementation phase of your application without having to spend time with configuration.
What are your opinions on the Express generator for applications? Have you used the tool to create any apps previously? Feel free to discuss your experience in the comments below!
Jeremy Holcombe
Content and Marketing Editor at , WordPress Web Developer, and Content Writer. Apart from everything related to WordPress I like the ocean, golf and movies. Additionally, I'm tall and have issues ;).