Display WooCommerce order details using order ID (r)
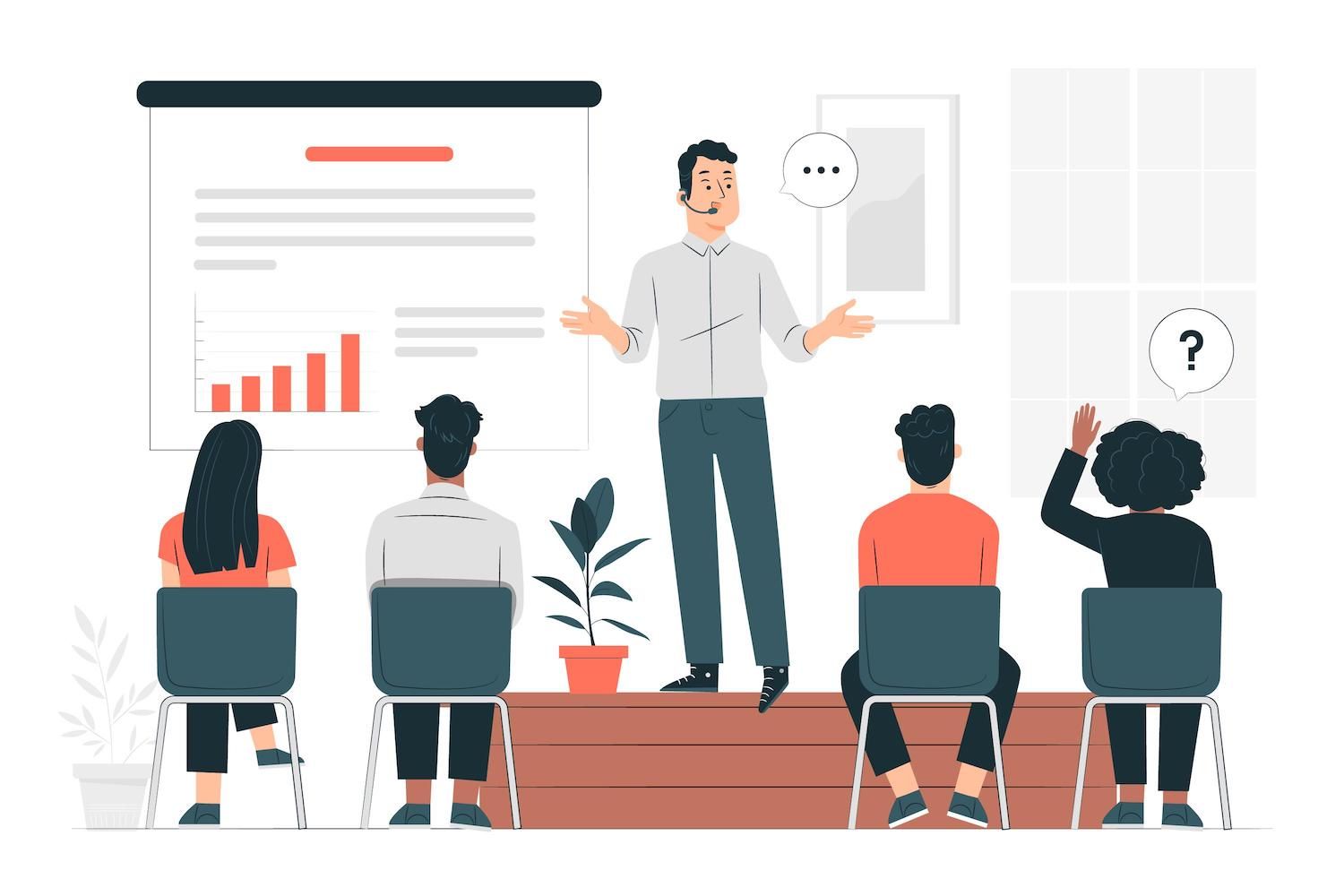
-sidebar-toc> -language-notice>
One standout feature of WooCommerce is the ability to store detailed information on orders. It meticulously stores every aspect of a purchase, including the quantity of the order, its currency, details about shipping tax details, as well as other information.
This detailed information is valuable for store owners and customers. Imagine designing a website that allows customers to easily check their order details and then customize the page to show more details.
What is the reason you would want to design a unique order information page?
- Enhances user experience The custom pages for order details can present the order details in a way that best suits your customers' needs. You can customize the layout and content of your page so you are able to highlight key information such as delivery status, the specifics of your product and dates for delivery, helping buyers to identify what they're looking for quickly.
- Interactivity Custom order details page allows you to include interactive elements like timers for tracking your order and links to other products, or direct access to customer support, providing a richer, more engaging experience for your users.
- Flexibility and adaptability The creation of the custom order information page allows users to use custom-designed logic for your business and adjust to changing needs. You can display different information based on criteria unique to your store, such as your customer's address or kind of items ordered.
How do I get the order information from the object $order
In WooCommerce, the $order
object is the central element that holds all data about an individual order. Utilizing this object, you can retrieve various details concerning an order including the order number and date of the order, as well as shipping and billing information along with the purchased products.
Let's go through how to make use of and access these various pieces of data from the $order
object. We'll go over each one in depth.
1. Retrieve the order object
The first step is to obtain an order object by using the function wc_get_order.
function. This function will take in an order ID, and then returns the corresponding order object.
$order_id = 123 // Example order ID
$order is the wc_get_order function( the order ID );
2. Information about the basic order
After you've obtained an purchase
object, you will be able to find the most basic information regarding the order. These are some of the examples:
- Purchase ID Unique identifier to identify the purchase.
$order_id = $order->get_id();
echo 'Order ID: ' . $order_id;
- Order dat e • The day when the order was created.
$order_date = $order->get_date_created();
echo 'Order date: ' . wc_format_datetime( $order_date );
- Order total The amount for the order.
$order_total = $order->get_formatted_order_total();
echo 'Order total: ' . $order_total;
3. Billing information
The information regarding billing is supplied by the buyer at the time of checkout. These details can be retrieved using the following methods:
- Billing address:
$billing_address = $order->get_formatted_billing_address();
echo 'Billing address: ' . $billing_address;
- Email for billing:
$billing_email = $order->get_billing_email();
echo 'Billing email: ' . $billing_email;
- Billing phone:
$billing_phone = $order->get_billing_phone();
echo 'Billing phone: ' . $billing_phone;
4. Shipping Information
Shipping information includes the details of where the order will be shipped. Similar to billing information You can get this information via an object called "$order"
object:
- Address for shipping:
$shipping_address = $order->get_formatted_shipping_address();
echo 'Shipping address: ' . $shipping_address;
5. Place an order for items
You can retrieve the items that are included in your order, this is especially useful when you would like to show bought items. How do you get ordered items:
$items = $order->get_items();
foreach ( $items as $item_id => $item )
$product_name = $item->get_name();
$product_quantity = $item->get_quantity();
$product_total = $item->get_total();
echo 'Product name: ' . $product_name . '';
echo 'Quantity: ' . $product_quantity . '';
echo 'Total: ' . wc_price( $product_total ) . '';
6. Methods for payment and shipping
Additionally, you can get information about the payment and shipping methods used for the purchase:
- The payment method is:
$payment_method = $order->get_payment_method_title();
echo 'Payment method: ' . $payment_method;
- Shipping method :
$shipping_methods = $order->get_shipping_methods();
foreach ( $shipping_methods as $shipping_method )
echo 'Shipping method: ' . $shipping_method->get_name();
7. Status of the order
The status of the order is useful for many purposes, including keeping track of the progress of the order
$order_status = wc_get_order_status_name( $order->get_status() );
echo 'Order status: ' . $order_status;
Creating a page to display the order information by order ID
In order to provide an easy experience for your customers by creating a customized webpage where customers can see the details of their orders by adding their order ID can be beneficial.
Step 1: Create a custom page template
No order ID provided.';
?>
Invalid order ID.';
return;
echo 'Order Details';
echo '';
echo 'Order ID: ' . $order->get_id() . '';
echo 'Order Date: ' . wc_format_datetime( $order->get_date_created() ) . '';
echo 'Order Total: ' . $order->get_formatted_order_total() . "/li>'echo '
Billing Address ' . $order->get_formatted_billing_address() . '';
echo 'Shipping Address: ' . $order->get_formatted_shipping_address() . "/li>";echo "Billing Email . $order->get_billing_email() . '';echo "Billing Phone . $order->get_billing_phone() . '';
echo 'Payment Method: ' . $order->get_payment_method_title() . '';
echo 'Order Status: ' . wc_get_order_status_name( $order->get_status() ) . '';
echo '';
echo 'Order Items';
echo '';
$items = $order->get_items();
foreach ( $items as $item_id => $item )
echo '';
echo 'Product Name: ' . $item->get_name() . '';
echo 'Quantity: ' . $item->get_quantity() . '';
echo 'Total: ' . wc_price( $item->get_total() ) . '';
echo '';
echo '';
Let's break down this code in order to make it easier for you to understand.
if ( isset( $_GET['order_id'] ) )
$order_id = intval( $_GET['order_id'] );
display_order_details( $order_id );
else
echo 'No order ID provided.';
What it does is that if an order_id
exists, it sanitizes the input using intval()
and calls the display_order_details
function. If there is no order_id
is available then it displays an error message to indicate the absence of an order.
The display_order_details
function is the function declared below get_footer()
. This function takes the order ID as an input and retrieves the corresponding order object using wc_get_order()
.
If the ID of an order is invalid, it outputs an error message. Otherwise, it retrieves and displays the order's details such as number of orders, dates the total amount, the shipping and billing addresses telephone, email payment method and order status in an unordered listing.
It also goes through the ordered items and shows the name of the item as well as the quantity and price of each item in another unorganized list.
Step 2: Apply the template to your theme. Create an entirely new page on WordPress
Save the page-order-details.php file in your child theme directory. The template will be available for selection when creating a new page on WordPress.
If you don't see the Page Attributes section, you can go back to Pages and you'll be able to see the list of pages, with a few options available by hovering over each page. Click Quick Edit and then you will find the page attributes section.
Create the page then you are able to try the test page.
For testing, open your preferred browser and add the?order_id=ORDER_ID
in the URL, replacing the ORDER_ID
with a valid WooCommerce order ID. For example, https://yourwebsite.com/order-details/?order_id=70
This should display the order information for the specific order ID.
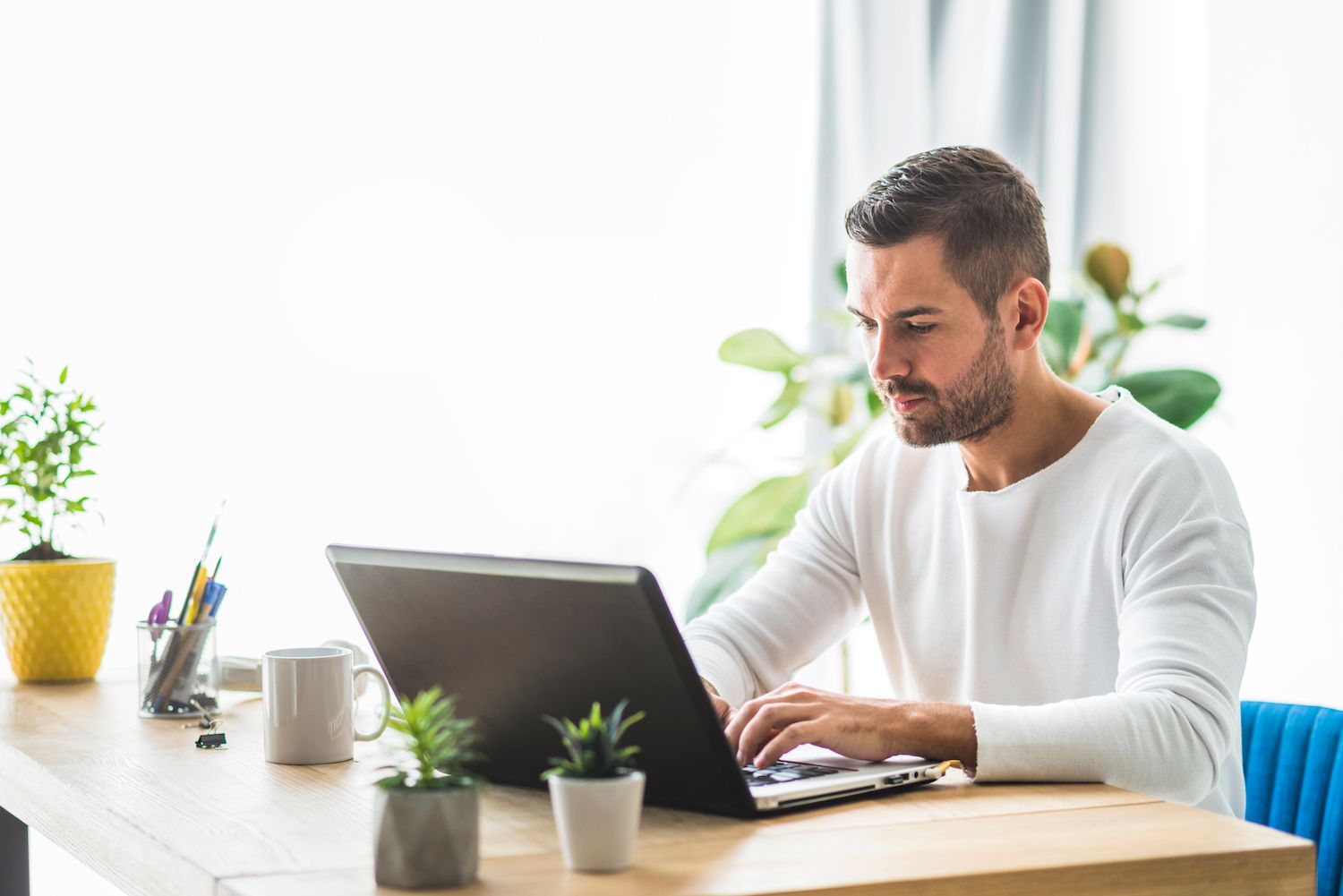
You can then add styling to the template however you'd would like.
Creating an order details page using WooCommerce order tracking shortcode
A different option that you may not know about is an alternative to the WooCommerce order tracking shortcode that provides an interface for users to find information about their purchase by entering the number of their order and their email address.
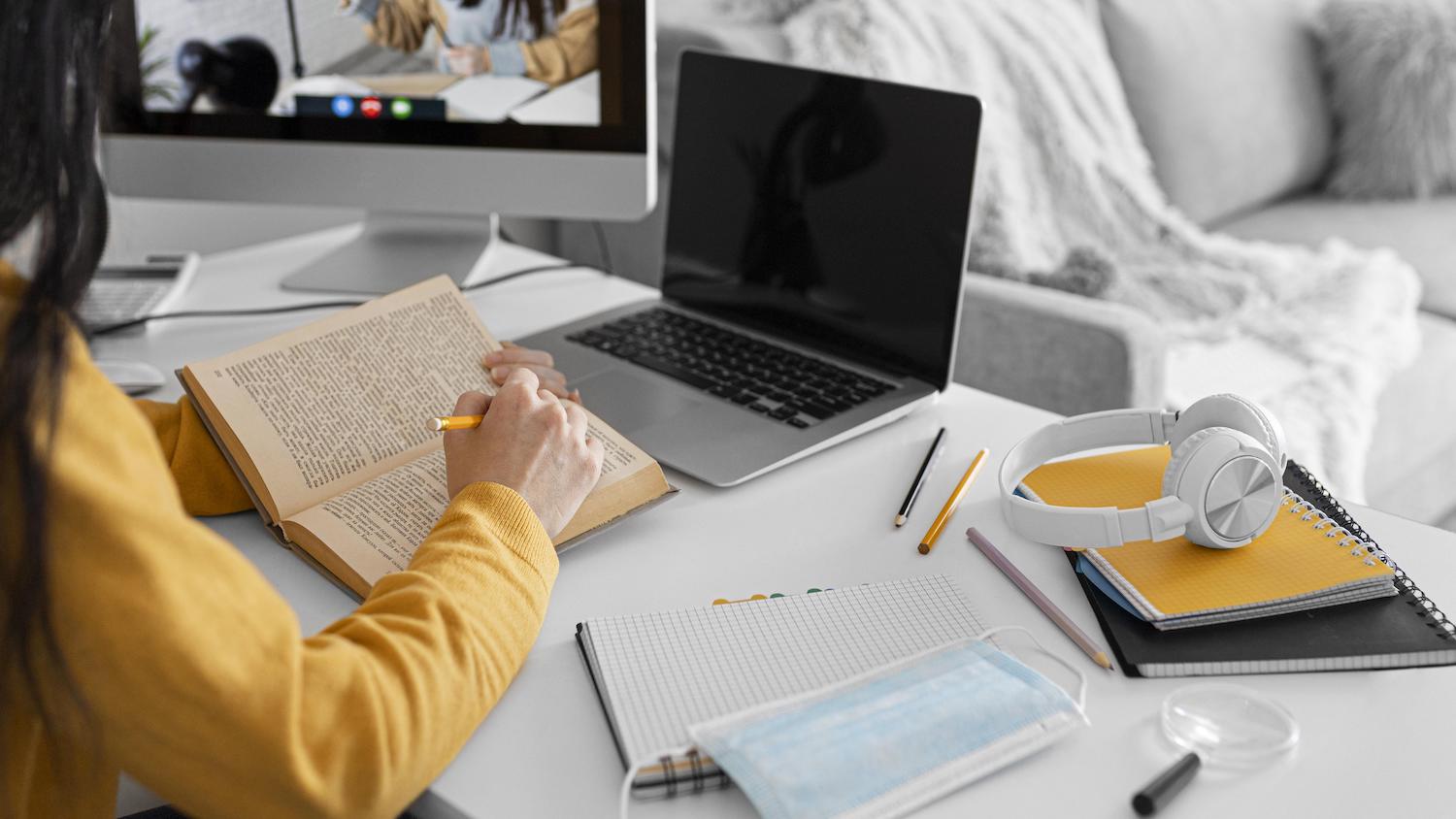
The page shows the purchase number, date and status of the order, as well as items delivery address and billing. This can be useful if you do not intend to show more detailed details.
Creating an order tracking page using the WooCommerce order tracking shortcode can be a simple way to enhance customer experience without requiring custom programming.
To accomplish this, you must take these steps:
- Log into your WordPress dashboard.
- Next, create a new page by going to Pages > Create New.
- The page should be given a name, and in the editor on your page, include the shortcode below:
[woocommerce_order_tracking]
- Then, press the button to publish. button to bring the website live.
This is as easy as that. This may not provide you the freedom you want.
Summary
Making an order information page with WooCommerce can enhance the experience for customers by allowing simple access to information about orders while increasing transparency and satisfaction.
Joel Olawanle
Joel is a Frontend Developer working as a Technical Editor. He is a passionate educator who is a lover of open source. He has written more than 300 technical pieces, mainly on JavaScript and it's frameworks.