How do you install TypeScript using Express (r) (r)
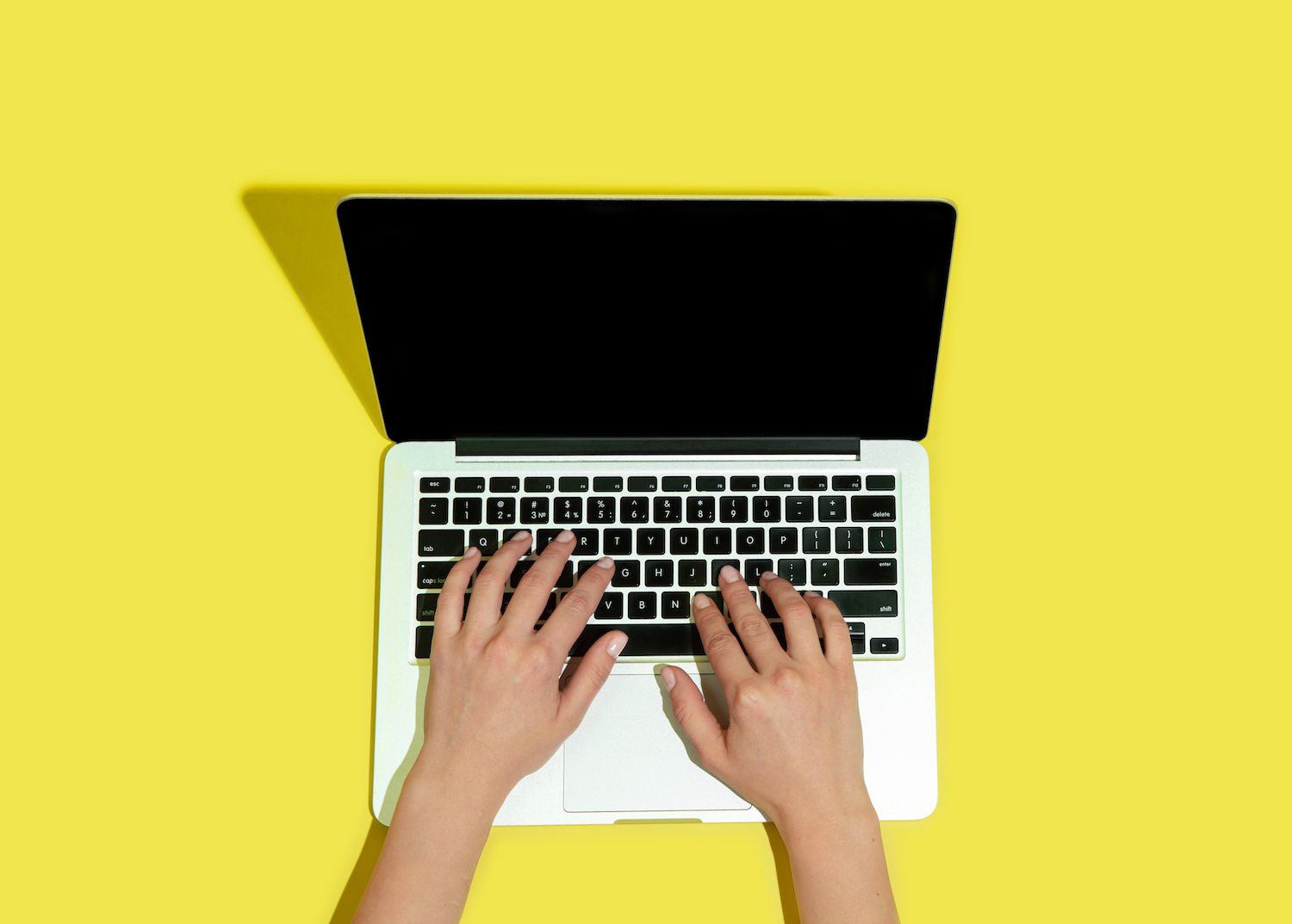
-sidebar-toc>
TypeScript is a written programming language that expands JavaScript capabilities. It offers a range of tools to assist you create scalable apps using Node.js and Express.
How to create an Express server?
- Make a directory with the code in the following:
mkdir sample_app && cd sample_app
- Start a Node.js application in the directory by running this command:
Init.npm"y"
The "-y"
flag of the command allows standard prompts while creating a package.json file populated with the following text:
"name": "sample_app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts":
"test": "echo \"Error: no test specified\" && exit 1"
,
"keywords": [],
"author": "",
"license": "ISC"
- Make an .env File at the base of the sample_app directory, and then add the variable below.
PORT=3000
- Create an express application which responds to a
Hello World
Text when users go tohttp://localhost:3000
.
const express = require("express");
const dotenv = require("dotenv");
// configures dotenv to work in your application
dotenv.config();
const app = express();
const PORT = process.env.PORT;
app.get("/", (request, response) =>
response.status(200).send("Hello World");
);
app.listen(PORT, () =>
console.log("Server running at PORT: ", PORT);
).on("error", (error) =>
// gracefully handle error
throw new Error(error.message);
)
dotenv.config()
will populate the Node application's environment ( process.env
) with variables defined in a .env file.
- Get started with your Node.js application using this command:
node index.js
Check if the application works by visiting http://localhost:3000 on your browser. The response you should receive is like this.
UWRHNhfrGsYzVSVTMHbg Hello World on http:localhost:3000.
Enable TypeScript inside an Express application.
Follow the steps below to use TypeScript within the Express application:
- Install TypeScript with this command:
npm i -D typescript
- One of the strengths that is a strength of this TypeScript community is the DefinitelyTyped GitHub repository . It stores documentation of type definitions for the various NPM packages. Users are able to easily integrate npm packages into their projects without having to worry about issues related to type just by installing the appropriate package's type definition with npm.DefinitelyTyped is a vital tool to TypeScript creators. It allows them to write cleaner and more efficient codes and lessen the risk of mistakes. It is necessary to install the type definitions of both
Express
anddotenv
By running this command:
Install npm # @types/express # @types/dotenv
- To start initializing TypeScript to start TypeScript, execute this command.
TSC --init
The created tsconfig.json file indicates your TypeScript Application's main directory. The file provides options for configuration to define the manner in which TypeScript compilers will function. It provides a variety of config
options that can be disabled or turned on and a description of each option.
- Incorporate an
outDir
The property belongs to theConfigured
object for defining the directory for output.
"compilerOptions":
// ...
"outDir": "./dist"
// ...
How to create the TypeScript server
To set up an TypeScript server, switch to change the .js
extension to .ts
and update the code using these type definitions:
import express, Request, Response from "express";
import dotenv from "dotenv";
// configures dotenv to work in your application
dotenv.config();
const app = express();
const PORT = process.env.PORT;
app.get("/", (request: Request, response: Response) =>
response.status(200).send("Hello World");
);
app.listen(PORT, () =>
console.log("Server running at PORT: ", PORT);
).on("error", (error) =>
// gracefully handle error
throw new Error(error.message);
);
NpX tsc
After that, start the application with the command.
node dist/index.js
Visiting http://localhost:3000 on your browser should provide a "Hello World" response.
How to deploy your TypeScript server to
// ...
"script":
"start": "npx tsc && node dist/index.js",
// ...
Create a new directory in the root of your application's directory. Include node_modules and .env to prevent pushing these files onto the Git service provider.
When your repository has been set then follow these steps to make your application available to:
- Sign in or create an account to see the dashboard of your My dashboard.
- Authorize your Git service provider.
- Click Applications in the left-hand sidebar, and then click Add application.
- Choose the repository as well as the branch that you want to deploy from.
- Give a unique title to the app, and then select an data center address.
- Click Create application.
After deployment, My provides a URL for you to view your application's deployment publically. You can visit the page to confirm it displays "Hello World."
Summary
This guide demonstrated how to develop and set up the Express Application using TypeScript and launch the application using . TypeScript offers additional capabilities which JavaScript doesn't -- including type classes, type safety, refactoring tools as well as auto-completion to assist you in creating large-scale applications as well as catch mistakes when you are developing.
allows you to deploy your app quick, while ensuring security and reliability. There are 21 data centres, you can deploy your application quickly. Google's C2 machine, which operates on Google's top-tier network.
Have you ever used TypeScript previously? What do you think about the use of it on the Express server?
Jeremy Holcombe
Content & Marketing Editor , WordPress Web Developer, and Content writer. In addition to everything related to WordPress I love playing golf, at the beach, and movies. Also, I have height problems ;).