How To Build and Deploy an Developer Portfolio Using Next.js (r) (r)
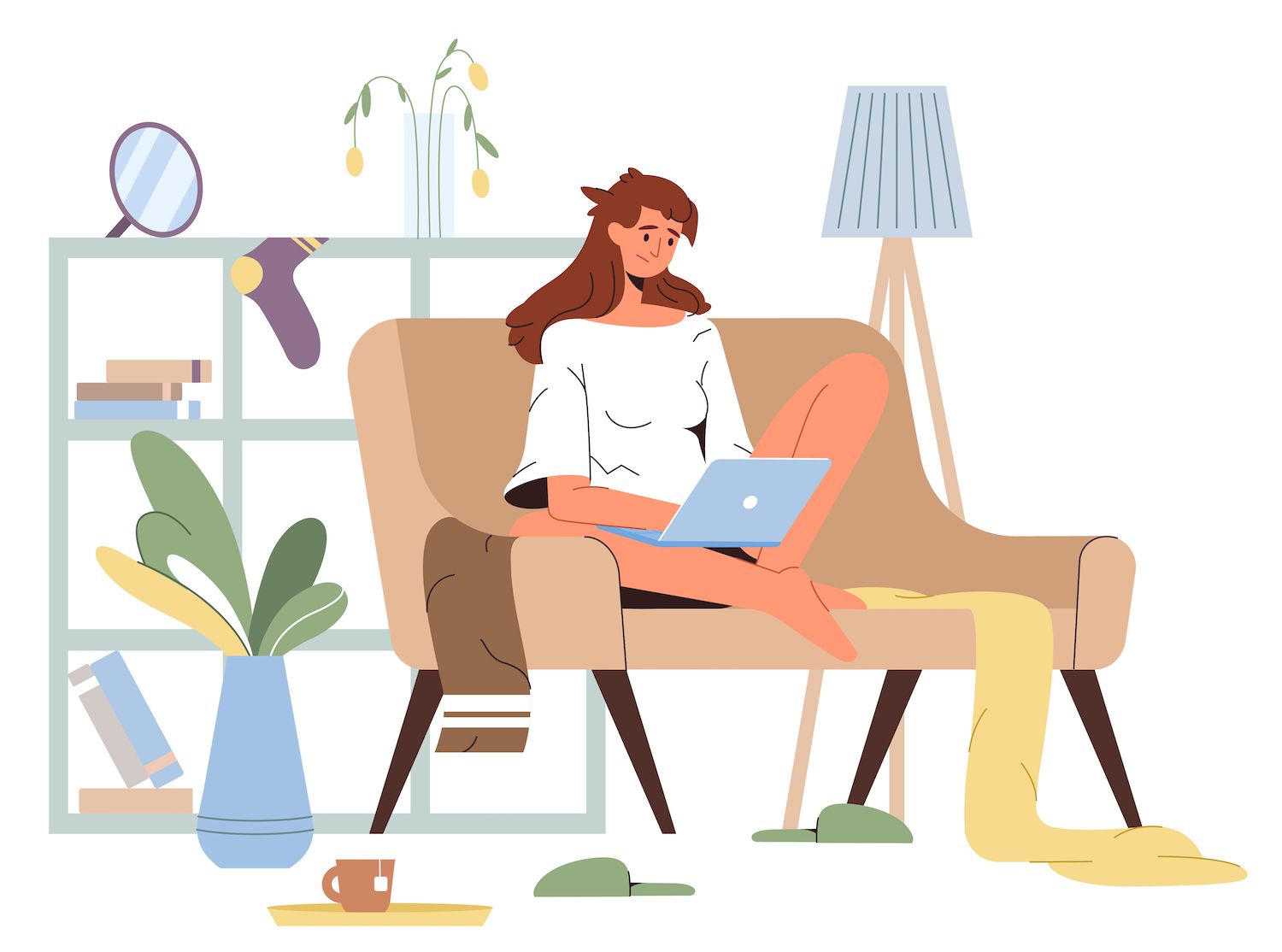
A developer portfolio is an accumulation of examples of work and work projects that demonstrate your abilities as well as experience. A strong portfolio sets you apart from other candidates to get a job. However, it's not just about that. A portfolio can also be a useful tool for networking, making sure you are keeping track of your education and ultimately building yourself into an expert in your field.
This is a live demonstration that shows your developer portfolio which you'll create using Next.js. The portfolio is accessible on the development project's repository on GitHub if you'd like to take a closer look or fork it Next.js portfolio-building beginning project I designed. The portfolio starter contains the simple codes, such as the styles and the Font Awesome CDN link, pictures, and the basic structure.
Requirements/Prerequisites
This is a "follow along" style of tutorial. It will be simpler to code along if you've got:
- Some knowledge of React as well as Next.js
What is the reason behind Next.js?
- Making static web pages: Next.js can build static sites that are speedy, easy to deploy, and need minimal maintenance similar to the portfolio site for developers which we'll create through this entire tutorial.
- Making dynamic websites: Next.js allows you to build dynamic websites that can change content based on users' interactions, or on server-side data downloading.
- Enhancing the performance of websites: Using server-side rendering, Next.js could improve web site speed by cutting down on the amount of time required for the page to load.
- Building ecommerce websites: Next.js is well-suited to build e-commerce sites which require rendering on the server for better SEO and speed.
- The development of progressive web applications (PWAs): Next.js allows the development of PWAs that are web applications that operate as native applications that can be placed on devices of users.
How to Setup The Environment For Your Next.js Development Environment
npx [email protected]est my-portfolio
An alert will pop up asking for confirmation of additional dependents. After that, you'll be able to execute an npm-based run
to make your app available at the localhost address 3000.
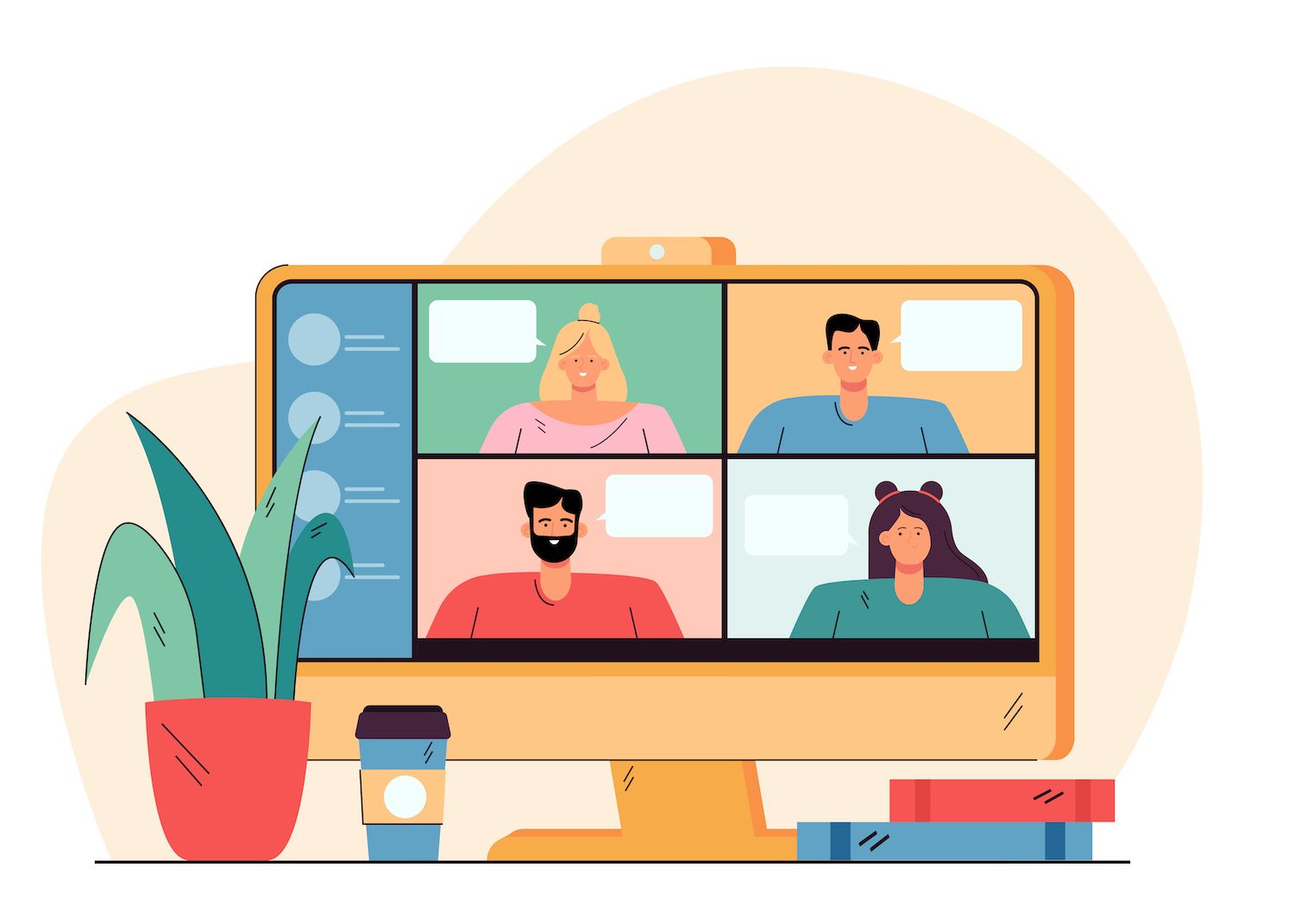
If you create an Next.js project using the npx
command, it automatically scaffolds one's folder structure by using these directories as the main ones:
- pages The folder in question contains the application's pages, which are automatically routed based on their file name. For example, pages/index.js would be the homepage page, while pages/about.js would be the about page.
- public This folder includes static files that can be served directly, such as images, fonts, as well as other resources.
- components The folder can be used for optional purposes and includes reusable UI components that are able to be utilized across applications.
- styles : This folder is not required and also contains global styles that can be applied across the application.
The other directories and file types can also be generated depending on the particular configuration and feature, however, these are the core directories of a basic Next.js project.
The website that we build will appear on the index page (our single-page site) It will include components for various sections , including the hero about, the projects as well as others.
How to Create an Responsive Portfolio of Developers Using Next.js
A portfolio typically consists of these components:
- Navbar component
- Hero component
- About component
- Skills component
- Projects are a component of
- Contact component
- Footer component
The Navbar and Footer components should be displayed on every page if the portfolio is multiple pages. It can be accomplished by using Next.js by creating the layout.
Definition of Layouts using Next.js
In Next.js Layouts are the method of defining an uniform structure for components that appear on every webpage of a site. The layout typically contains elements like a header along with a navigation menu and a footer displayed across all site pages.
Create a components folder within the Src (source) directory in the Next.js project. Then, you need to create your Navbar and Footer components which will use in the Layout component.
Below is the Navbar component in Navbar.jsx:
// components/Navbar.jsx
import Link from "next/link";
const Navbar = () =>
return (
Joe's Portfolio
Resume
)
export default Navbar;
The Footer component is in Footer.jsx:
// components/Footer.jsx
const Footer = () =>
return (
(c) new Date().getFullYear() Joel's Portfolio
>
)
export default Footer;
Note: For the Font Awesome icons to work you need to either download Font Awesome into your project or utilize its CDN. Add the CDN hyperlink to the _document.js file like the following:
/pages/_document.js pages/_document.js
import Html, Head, NextScript, and Main from 'next/document';
Export default function Document()
return (
);
Note: If you link to a different version of Font Awesome via the CDN, you will need change it above the correct quality
hash to match the version you are using.
After creating all the necessary components for your layout, you can create the Layout component and include it on your webpages by wrapping the page content within it.
Layout will be able to take a prop >code>children which allows you access to the contents of Your Next.js pages.
// components/Layout.jsx
import Navbar from './navbar';
import Footer from './footer';
const Layout = ( children ) =>
return (
children
>
)
export default Layout;
At this point, you are able to create the Layout component, which houses the Footer and Navbar along with the children props positioned properly. It is now possible to add the Layout component to your webpages by wrapping the contents of the page in it. The Layout component will be added to the _app.js file.
// pages/_app.js
import '@/styles/globals.css';
import Layout from '../components/layout';
export default function App( Component, pageProps )
return (
);
Now you have succeeded in making a layout of your portfolio of developers. For this portfolio, we are focusing more on Next.js and how to deploy your website to . Therefore, you are able to copy the styles in the styles/globals.css file into your project. If you launch your portfolio site in development mode, you'll be able to see your app's layout.
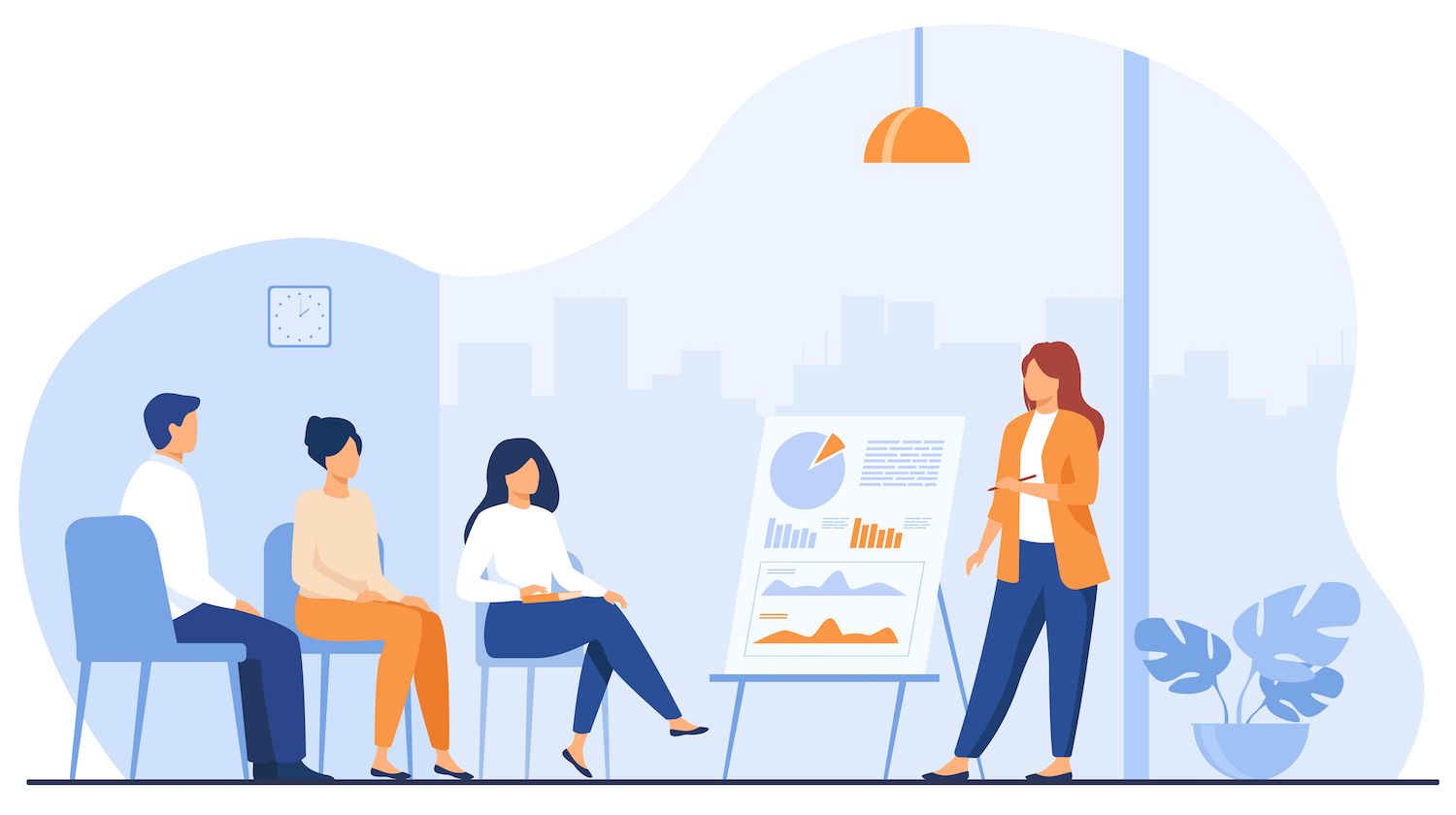
The time has come to update your portfolio website the appropriate content.
Building Portfolio Components
You can now create individual components for every section of your developer's portfolio. All these components will be imported into the index page for your Next.js project. They will be displayed when you start your project using npm run dev
.
The Hero Component
The Hero component is the first section beneath the Navbar The primary purpose is to draw the user's attention and give an idea of the purpose of the site or app will be about.
// components/Hero.jsx
import Image from "next/image";Const Hero = () •export the default Hero
In the code above, you will notice that you will notice that the Next.js image component replaces using the HTML img
tag. This is to include the image because it enables the automatic optimization of images, scaling as well as many other.
Within the About section, you'll also notice that a simple line to describe the creator was included along with the social icons of Font Awesome to add social hyperlinks.
This is how the Hero component ought to appear like:
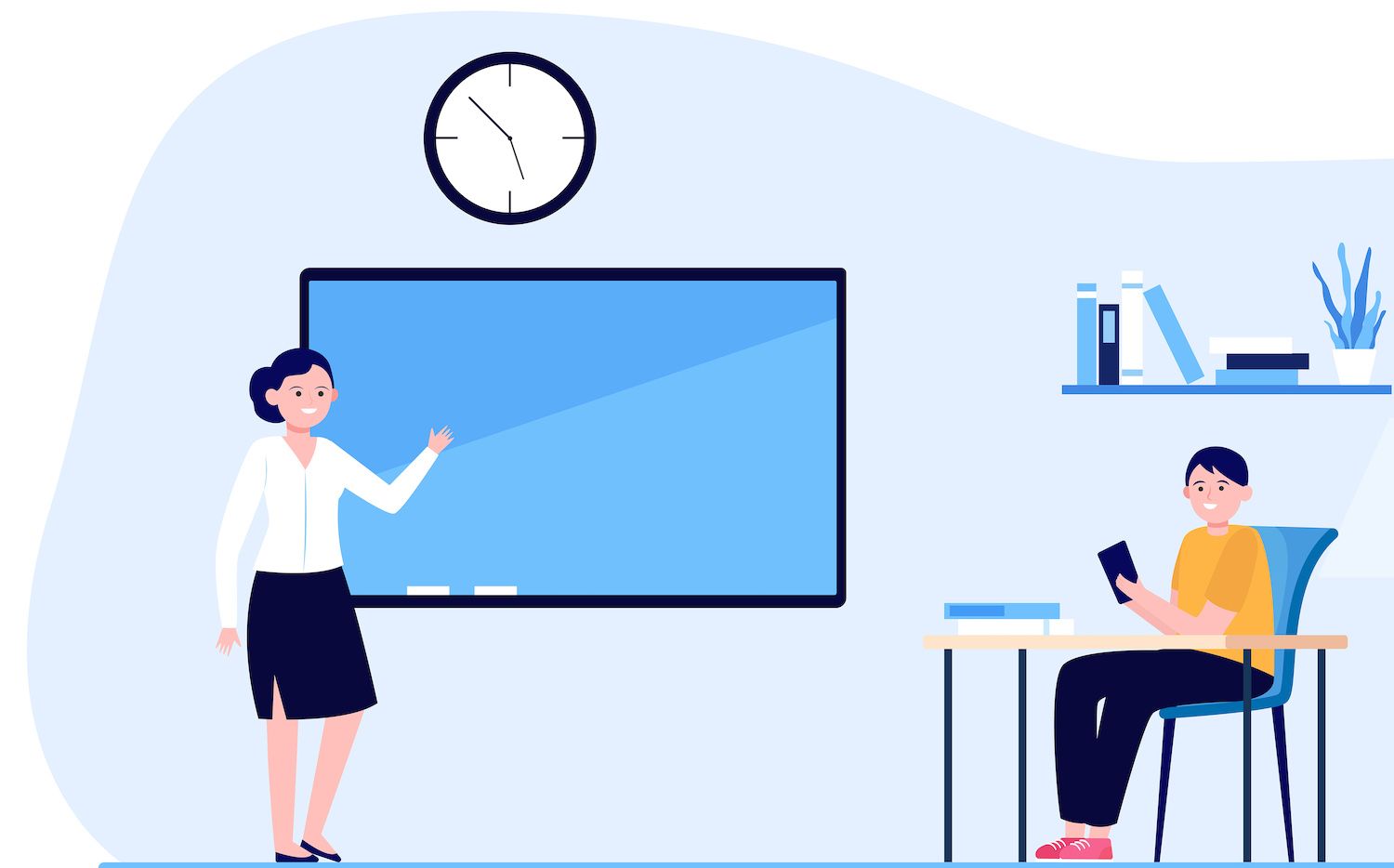
It is possible to add additional content to this Hero component, alter the styles in the styles/globals.css file or perhaps recreate the section according to your preferences.
The About Component
The About section is designed to provide readers or those that visit your portfolio more information about you in the number of paragraphs you want. If you want to share your story it is possible to create a dedicated "About Me" page. Add a button on this section to learn more about yourself.
/"/ components/About.jsx
Import Image from "next/image" //const About = () => >
return (
About me
As as a software developer, I've always had a passion for finding elegant, efficient solutions to
difficult problems. I have a strong foundation in the field of software development with a focus on webtechnologies like HTML, CSS, and JavaScript. I enjoy working on both the front and back end of applications, and I am always looking for ways to increase efficiency, enhance the userexperience and guarantee the highest level of code quality. Through my career, I have been involved in a variety of tasks, ranging from basic static websites, to morecomplex enterprise-level applications. I am experienced in using a range of development
tools and frameworks that include React, Angular, Vue.js, Node.js, and Laravel. I'm always lookingto discover and experiment with new technologies, and I always seek out new ways to enhancemy abilities and knowledge.
> The
)
export default About;
The code below has two paragraphs about the developer and an image showing the creator. The About section will be:
About component
You can always tweak your style to include more images and lots more.
The Skills Component
The skills component is meant to highlight how the developer has the most popular technologies, or techniques that the developer used in the past.
Skills component
You can make this simpler to manage by creating an array within an external file, and later upload it into the skill component. That way you'll be able to repeat the loop instead of duplicate similar code.
// components/Skills.jsx
const Skills = () =>
return (
Skills
HTML
CSS
JavaScript
React
Node
Python
)
export default Skills;
In the code above, a card is created for each skill. And every card is adorned with the technology icon from font-awesome as well as the name of the technology. You can also add more styles and tweak the code to make it look more appealing and distinctive.
The Projects Component
The component of a project is one essential elements in a developer's portfolio. The projects are tangible proof of the developer's abilities and capabilities and demonstrate their capacity to put their expertise into actual issues.
The Projects component
It is possible to create an array to hold the details of each project and import it into your component so that you don't have to code these details.
Let's create an data.js file to store the array of project data. It can be stored within the folder of components or in the pages/api folder. This demo put it into the components folder. The array will contain the project's object It holds the name of the project, description, and GitHub hyperlink.
/components/data.js components/data.js
export projectData const
ID: 1,
title: 'Todo List App' Description: A simple Todo List app built using JavaScript. All datas are stored within local storage. It allows users to list the tasks they have planned and then tick when they complete them. ',
gitHubLink: 'https://github.com/olawanlejoel/Todo-List-App',
,
id: 2,
title: 'Books Library App',
description:
'A simple Book Library App built with JavaScript. It helps readers have an extensive list of books they are either studying or finished reading. ',
gitHubLink: 'https://github.com/olawanlejoel/Book-Library',
,
id: 3,
title: 'Quotes Generator',
description:
'Helps you generate quotes from about 1600 quotes written by different authors . Quotes are copied automatically onto your clipboards. ',
gitHubLink: 'https://github.com/olawanlejoel/random-quote-generator',
,
id: 4,
title: 'Password Generator',
description:
'Helps you generates random passwords, you can select what you want your password to entail and also you can copy generated password to clipboard. ',
gitHubLink: 'https://github.com/olawanlejoel/Password-Generator',
,
id: 5,
title: 'Twitter UI Clone',
description:
'Simple Twitter UI clone built with TailwindCSS and Vue Js. It only covers the homepage of Twitter UI. This is cool to get started with TailwindCSS because it can help you understand basic concepts. ',
gitHubLink: 'https://github.com/olawanlejoel/TwitterUI-clone',
,
];
It is now possible to develop a component for your project to benefit from this data by looping through easily. You can use any JavaScript method of iteration, but for this particular tutorial, you can utilize the JavaScript maps() array method to look through the data array following the import of data into the Projects component.
// components/Projects.jsx
import projectData from './data.js';
const Projects = () =>
return (
Projects
projectData && projectData.map((project) => (
project.title
project.description
))
)
export default Projects;
In the code above you've successfully prevented repetition by looping through the array to output each project into the Projects component. This makes it easier to manage and create more projects.
The Contact Component
The reason you should create the portfolio of a developer is to ensure that prospective clients are able to get in touch with them. Another option is to allow people to send you an email. This is exactly what we'll facilitate in this Contact element.
// components/Contact.jsx
const Contact = () =>
return (
Get In Touch
If you want us to work together, have any questions or want me to speak at your event, my inbox is always open. Even if I simply want to meet you or have a question, I'll be sure to reach you! Cheers!
Say Hello
)
export default Contact;
Put your email address inside the tag. The tag in such a way that clicking it launches an email app that will send a message to you.
Contact component
It is now possible to create all the components for your portfolio application. The next thing to do is to include them on the index page. Navigate to pages/index.js file -that is the one created by default -- and replace it with this code.
// pages/index.js
import Hero from '@/components/Hero';
import About from '@/components/About';
import Skills from '@/components/Skills';
import Projects from '@/components/Projects';
import Contact from '@/components/Contact';
import Head from 'next/head';
const Home = () =>
return (
Joel's Portfolio
>
);
;
export default Home;
Image optimization is handled with the Next.js Image component. Before deploying an application into production using the Next.js Image component, it is strongly suggested that you set up sharp. You can do this via your terminal, ensuring you're within your project's directory, and then using the following command:
npm i sharp
It is now possible to install your app, and your images will function perfectly with the complete optimization that Next.js provides.
How to deploy the Next.js Application to
When you're satisfied with the portfolio you have created that highlights the best work you've done in development and important information, you'll likely want to share it with other people, don't you? Let's explore ways to accomplish it by using GitHub as well as's App Hosting Platform.
Send Your Code to GitHub
Upload your code on GitHub using the following instructions:
The first step is to create a brand new repository (just like a local folder for storing your source code). You can do this by signing in to your GitHub account, clicking on the + button at the top right corner of the screen and selecting New repository from the dropdown menu as seen in the image below.
Make a brand completely new GitHub repository.
The next step is to assign your repository a name, add an explanation (optional), and choose whether you would like your repository to be publicly accessible or private. Then click the Create button. You can now transfer your code into the newly created GitHub repository.
All that is needed to upload your code using Git is the repository URL You can locate it on the repository's main page, under the Clone or download button, or on the steps that appear when you create the repository.
Access your GitHub repository's URL
The best way to do this is to make sure you are ready to publish your code through a console or command prompt and going to the directory which includes your code. Follow the steps below to start your local Git repository:
the git process
Now add your code into the local Git repository by using this commands:
Add git .
The above command adds every file in the current directory along with subdirectories of it to the brand new Git repository. You can now commit changes by via the command below:
commit to git with a -m "my very first commit"
Note: You can replace "my first commit" by a short text describing the changes that you made.
Last but not least, upload your code onto GitHub by using these commands:
Remote git Add origin URL [repository URL]
git push -u origin master
Note: Ensure you replace "[repository URL"[repository URL]" with the URL for your personal GitHub repository.
When you've finished the steps above, your code will be uploaded to GitHub and accessible through your repository's URL. Now you can move your repository's data to .
Deploy Your Portfolio to
It will be deployed in just minutes. You can start by visiting My dashboard to log in or create an account. My dashboard and sign in or create an account.
Simply click Applications to the left of the sidebar
Simply click "Add service"
From the dropdown menu, select the Application as you wish to deploy a Next.js application onto .
Create an application project in My
A modal will appear through where you are able to select the repository that you want to publish.
Automatically detect the start command
At this point, your application will start in the process of deploying. Within hours, a link will be provided to access the deployed version of your application. In this case, it is: https://-developer-portfolio-ir8w8..app/
Deployment link on
Notice: Automatic deployment was disabled, and it will automatically re-deploy the application every time you update your codebase and push the changes onto GitHub.
Summary
There are many advantages to the use of Next.js for web-based projects. It is the first to provide optimized performance out-of-the-box, with features such as pre-fetching and code splitting that help reduce loading times for pages. Additionally, it offers a familiar development experience for React developers. It also supports well-known tools like stylized components and React hooks.
Next.js is also able to support a variety of deployment choices, from traditional server-based hosting to modern serverless platforms like 's. This allows developers to choose the deployment option that best matches their requirements, while profiting of the framework's optimizations for performance and many other benefits.
The next step is to add more options to your new portfolio site. A few ideas to get your creative energy flowing: include additional pages that provide detailed information, add blogs with MDX and implement animation.
Tell us about your work and experiences by leaving a comment below.
Simple setup and management on My dashboard. My dashboard
24 hour expert assistance
The most efficient Google Cloud Platform hardware and network, that is powered by Kubernetes to ensure maximum capacity
An enterprise-level Cloudflare integration for speed as well as security
Reaching a global audience with up to 35 data centers, and around 275 POPs in the world.