How to Build and deploy static websites using Gatsby and (r) (r)
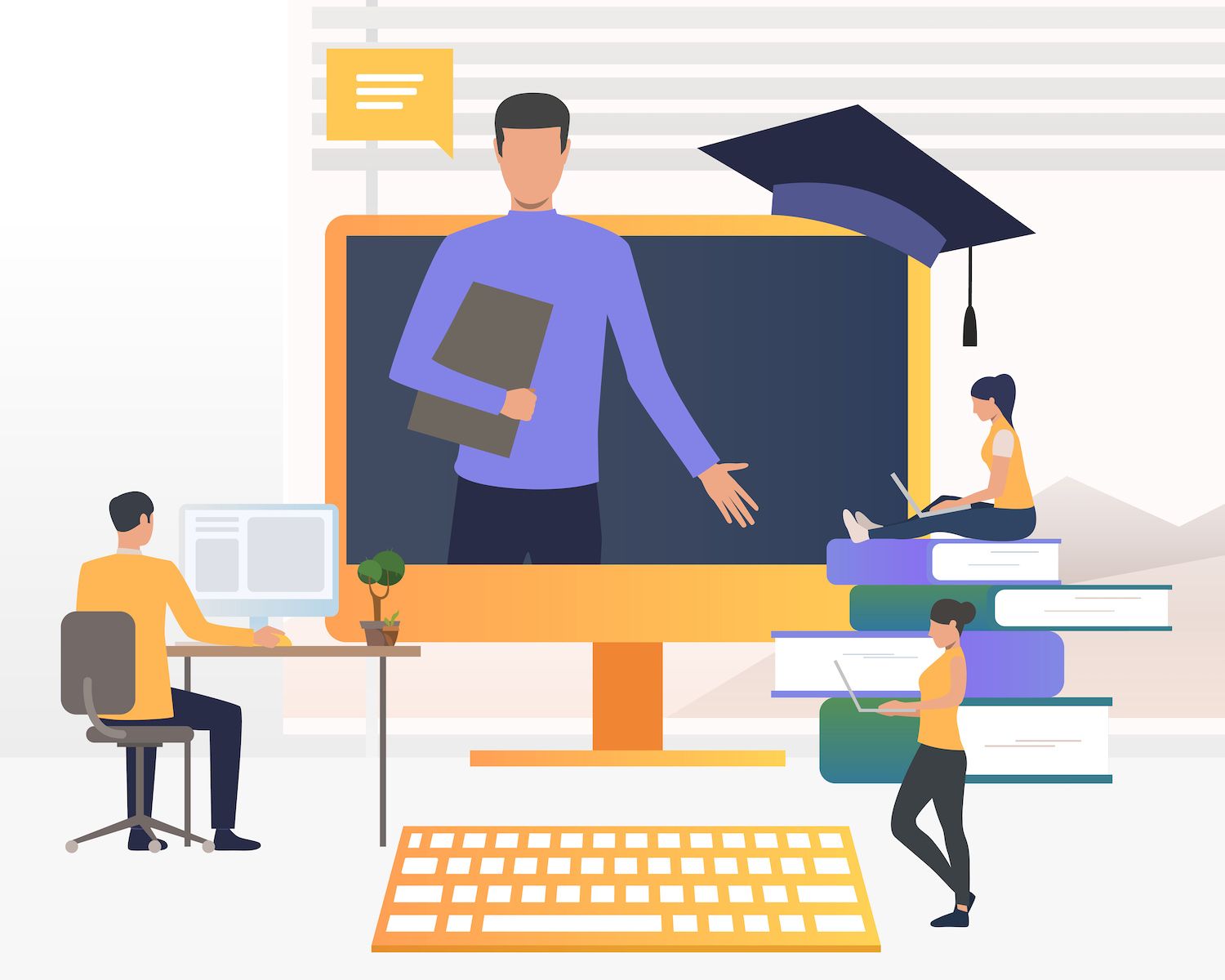
-sidebar-toc>
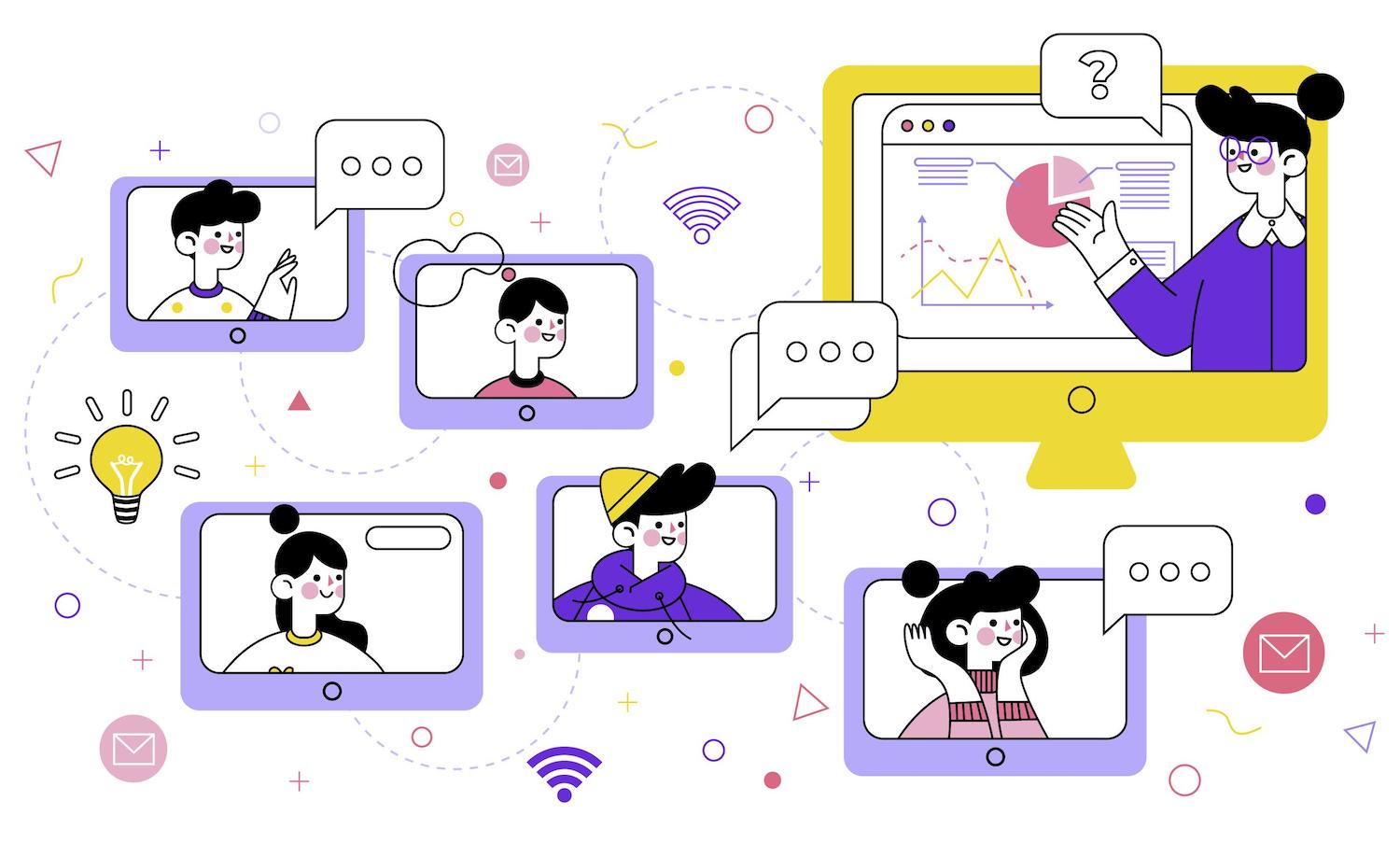
Understanding Static Sites
- Speed: Static sites load quickly since there's no server-side processing.
- Security Since there is no server side executable code, static sites are less vulnerable to security-related threats.
- Scalability: It's easy to distribute and cache static web pages via Content Delivery Networks (CDNs).
- Simplicity: They are easier to design, implement and manage.
After you have a better understanding of what static sites are and their benefits let's dive into Gatsby.
What is Gatsby?
- Rapidly Speedy: Gatsby optimizes your website for speed by using methods like code splitting and lazy loading. The result is almost instantaneous loading of pages.
- Flexible Data Sourcing: It's able to get data from many sources such as Markdown files, APIs, and databases.
- Rich Plugin Ecosystem: Gatsby's large ecosystem of plugins lets you extend its functionality effortlessly.
- SEO and performance: Gatsby automatically produces optimized HTML that is better suited to SEO and performance.
Beginning to Get Started with Gatsby
In order to follow along with this guide, you should possess:
- Basic understanding of HTML, CSS, and JavaScript
- Basic knowledge of React
For a start in Gatsby and create a project, try any of the numerous examples in the Gatsby Starter Library or build a idea from beginning to finish.
To follow this procedure, let's use GatsbyJS's Hello World starter for GatsbyJS since it offers us a plain project with no extensions or additional files.
- First, install Gatsby's CLI on your computer using the below command:
NPM install -g gatsby cli
Try running gatsby --version
to determine if installation is successful.
- Next, navigate into the directory you want to create your project then run the following command:
npx gatsby new https://github.com/gatsbyjs/gatsby-starter-hello-world
Make the change the project-name>
above to the desired title for the project you are working on.
- After this has been completed, navigate into the project folder and start the server for development:
cd gatsby develop
The local development server will start at http://localhost:8000, where you can access your Gatsby site.
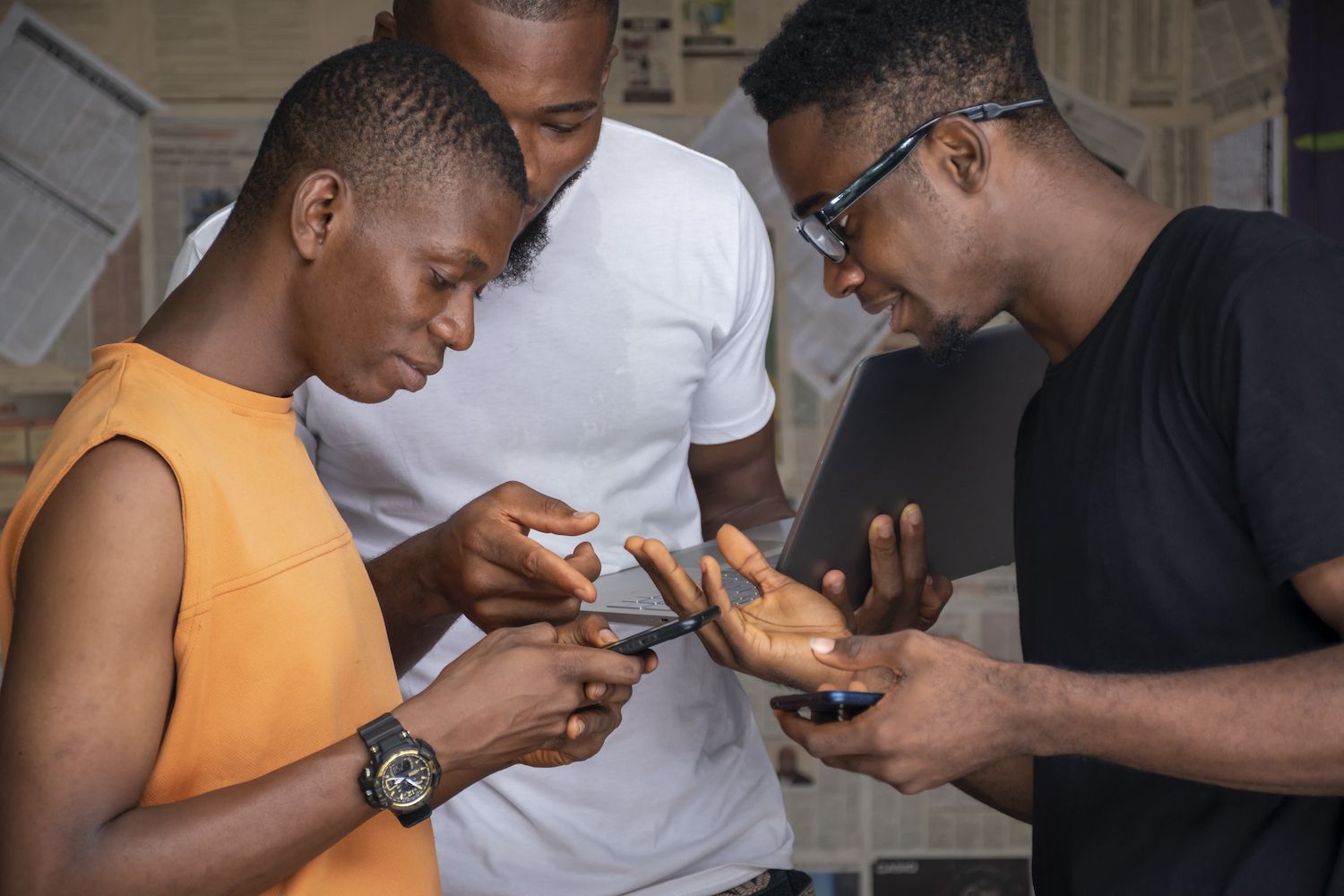
Understanding Gatsby File Structure
If you launch your project in an editor for code and you'll be able to see the following structures:
/
|-- /public
|-- /src
|-- /pages
|-- index.js
|-- /static
|-- gatsby-config.js
- Public: This directory contains the results of your Gatsby build procedure. It's where the generated HTML, CSS, JavaScript as well as other files are stored.
- /src: This is the heart of your Gatsby project. It's which is where you'll be spending the majority the time. There are several subdirectories as well as documents:
- /pages: This is where every page for your website are kept. Every JavaScript file here corresponds to the route you have on your site.
- static: This directory is utilized for static files that are required to be included on your site, such as images, fonts, or downloadable files. These files are served as-is and will not be processed by Gatsby.
- gatsby-config.js: This configuration file is where you specify various settings for your Gatsby site. You can specify plugins or site metadata as well as various other settings here.
Creating Pages and Components
In Gatsby, building web pages is a straightforward process. Each JavaScript files you make in the pages/src directory automatically transforms into an actual page, with the appropriate route, thanks to Gatsby's automated page creation.
You are able to make as many pages as you'll need on your website through the addition of additional JavaScript files to the pages folder in the /src/pages folder. You can, for instance, build the about.js file for the "About" page.
Although you're able to create separate JavaScript files for each page straight in the pages/src/pages folder, it's possible to arrange your pages further. You can create subfolders to separate related pages. As an example, you could create the blog folder that will organize all your blog-related pages.
For this project, this is what the page structure will look like:
|-- /src
|-- /pages
|-- about.js
|-- index.js
|-- /blog
|-- index.js
Utilizing JSX for Pages
In this case, for instance, you could make the contents for the home Page ( index.js) in the following way:
import React from'react";the default export function is Home()
return (
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
>
);
Links Pages within Gatsby
For creating a link to another website, you can use to use the Link
element in the manner is:
import React from'react';
import Link from 'gatsby'
export default function home()
return (
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
to="/about">About Us
to="/blog">Blog
>
);
In this example in the above example, we've imported Link
element from the gatsby website
and used it to build links for our "About Us" page and blog. For the "About Us" page, as an example, has the route about
. After users click on"About Us," for instance "About Us" button, they'll be taken to the page /about
page.
To create links to external sites, you may utilize ordinary anchor ( ) tags with an HREF
attribute:
import React using'react";Export default functions Home()
return (
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
Read more
>
);
In this case it opens an external website in a new browser tab due to being able to use the target="_blank"
and rel="noreferrer"
attributes.
Creating components in Gatsby
Gatsby's modular architecture enables you to design reusable building components for your website pages. Instead of duplicating code across several pages, you are able to encapsulate common elements into components, making your codebase more efficient, manageable, and efficient.
If your code for your homepage includes the navigation section, main content, and a footer:
import React from 'react';
import Link from 'gatsby';
export default function Home()
return (
to="/">Home
to="/about">About
to="/blog">Blog
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
Read more
Hosted with by 's' '
href="https://.com/static-site-hosting">
Static Site Hosting
.
>
);
Imagine having to copy the footer and navbar codes for every page on your website. This is where components come into play. You can create reusable components for the navbar, footer, and every element of code that could be reused across different elements and pages.
To work with components in Gatsby to work with them, you need to make components folders in Gatsby. Create a component folder within the src folder. This will store all components. Next, create your components, e.g. Navbar.js and Footer.js. Inside the Navbar.js file, divide the code in this manner:
import Link from 'gatsby';import React using'react";const Navibar () =
return (
Home
About
Blog
);
;
export default Navbar
Also, the Footer.js:
import React from 'react';
const Footer = () =>
return (
Hosted with by 's' '
href="https://.com/static-site-hosting">Static Site Hosting
.
);
;
export default Footer;
Then, you can import your component documents into your pages or components and make use of this method:
import React from 'react';
import Navbar from '../components/Navbar';
import Footer from '../components/Footer';
export default function Home()
return (
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
Read more
>
);
Designing Layout Components
An etiquette that is common when developing websites is the creation of an layout element which reflects the general design of the site. The layout component usually includes elements that are displayed on each page, such as headers, footers and sidebars and navigation menus.
Create a file named Layout.js in your component's /src/components directory. After that, determine the layout's structure. This guide will define the layout structure should only comprise the footer and navbar:
import React from 'react';
import Navbar from './Navbar';
import Footer from './Footer';
const Layout = ( children ) =>
return (
className="content">children
);
;
export default Layout;
With this layout component, we make use of the components to wrap page contents (provided as children
). To use the layout component within your pages, you need to import it and wrap your page contents with it. For example, in your index.js page:
import React from 'react';
import Layout from '../components/Layout';
export default function Home()
return (
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
Read more
);
With a layout element, you ensure a consistent design and layout across your pages while keeping your code organised and up-to-date. This is a great method of managing the most common elements of your site efficiently.
Styling Pages and Other Components in Gatsby
Styling your Gatsby site can be a bit more flexible, and allows you to use different approaches like basic CSS as well as CSS-in-JS or CSS preprocessors like Sass. We'll show you how to make standard and module-styled pages.
CSS Styling
In Gatsby there is a way to create a CSS file, and then link it to whatever part or web page you want that you wish to, and it should perform flawlessly. In this case, for instance, you can make the the styles folder inside the src folder and then create the global.css file with your CSS codes.
For example, here are some general styles for components that were created earlier:
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500&display=swap');
*
margin: 0;
padding: 0;
box-sizing: border-box;
body
background-color: #ddd;
font-family: 'Poppins',
sans-serif;
width: 1200px;
margin: 0 auto;
a
text-decoration: none;
img
width: 100%;
nav
display: flex;
justify-content: space-between;
height: 200px;
align-items: center;
nav .logo-img
width: 100px;
nav .nav-links a
padding: 0 20px;
font-size: 18px;
@media (max-width:700px)
body
width: 100%;
padding: 0 20px;
nav .nav-links a
padding: 0 18px;
.footer
width: 100%;
text-align: center;
margin: 100px 0 20px;
After that, add the CSS file into the components that you would like to style in this case, you can add it to your Layout component so it applies to all components:
import React from 'react';
import Navbar from './Navbar';
import Footer from './Footer';
import '../styles/global.css';
const Layout = ( children ) =>
return (
className="content">children
);
;
export default Layout;
Module CSS Styling
CSS Modules permit you to scope your styles to specific parts or pages. This avoids conflicts between styles and makes it easier to manage your code. In the styles folder, create your CSS modules with the structure (pageName>.module.css and add the specific style into the files.
In this case, for example, make home.module.css for the homepage, and include the below code:
.home_hero
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
text-align: center;
.home_hero h1
font-size: 60px;
width: 70%;
.home_hero p
color: #6E7076;
font-size: 20px;
.btn
background-color: #5333ed;
padding: 20px 30px;
margin-top: 40px;
border-radius: 5px;
color: #fff;
@media (max-width:700px)
.home_hero h1
font-size: 40px;
.home_hero p
font-size: 16px;
In order to use module CSS styles on the Gatsby webpage or components, you must import the styles from the CSS module as an object at the top of your page or file and use in this manner:
import React from 'react';
import Layout from '../components/Layout';
import * as styles from '../styles/home.module.css';
export default function Home()
return (
Enjoy Static Site Hosting With StSH.
Fast, Secure, Reliable Hosting Solution.
Read more
);
Utilizing Static Files in Gatsby
In Gatsby static files are referred to assets like pictures, fonts and CSS files and many other assets that are sent directly to the client's web browser without the need for server-side processing. These files are added to the the /static directory in the root of your project.
For example, if you upload an image -logo.png to the directory /static directory may show it on your component like this:
import Link from 'gatsby';import React from 'react';const Navbar = () =>
return (
to ="/">
to ="/">Home
to="/about">About
to="/blog">Blog
);
;
export default Navbar
Gatsby will automatically convert these relative paths to the correct URL once your website is created. Further in this tutorial, you will learn how to optimize images in Gatsby.
Integrations and Plugins
Gatsby offers a wide range of plugins, which allow you to extend the capabilities of. It has plugins to help with SEO, analytics images, markdown optimization, image transformation, and much more. Configuring and installing the plugins is straightforward and can significantly improve the functionality of your website.
In this tutorial using four plug-ins:
- gatsby-transformer-remark: This plugin allows you to transform Markdown files into HTML content, making it easy to create and manage blog posts, documentation, or any text-based content.
- gatsby-transformer-sharp and gatsby-plugin-sharp: These plugins work together to optimize and manipulate images in your Gatsby project.
- gatsby-source-filesystem: This plugin enables you to source files from your project directory and make them available to query with GraphQL.
For these plugins to be used in your Gatsby project, run the following command within the project's root directory in order to install them:
npm install gatsby-transformer-remark gatsby-transformer-sharp gatsby-plugin-sharp gatsby-source-filesystem
Then, you can configure them in your gatsby-config.js file. This is an illustration of the way you can install the plugins
module.exports =
plugins: [
// ...other plugins
// Transform Markdown files into HTML
'gatsby-transformer-remark',
// Optimize and manipulate images
'gatsby-transformer-sharp',
'gatsby-plugin-sharp',
// Source files from your project directory
resolve: `gatsby-source-filesystem`,
options:
name: `posts`,
path: `$__dirname/src/posts/`,
,
,
resolve: `gatsby-source-filesystem`,
options:
name: `images`,
path: `$__dirname/src/images/`,
,
,
],
;
Two gatsby-source-filesystem configurations are created, pointing to two folders: posts and images. Posts will store some markdown files (blog posts) that would be transformed with gatsby-transformer-remark, and images will store images for the blog and other images you wish to optimize.
Remember to restart the local development server whenever you make changes to the gatsby-config.js file.
Creating Blog Posts
Once we've installed our plugins create posts folders in the post folder inside the src directory and then create two Markdown files with the following contents:
post-1.md:
---
title: "Introduction to Gatsby"
date: "2023-10-01"
slug: "introduction-to-gatsby"
description: "Learn the basics of Gatsby and its features." featureImg: ../images/featured/image-1.jpeg
---
Welcome to the world of Gatsby! In this article, we will guide you through the basic concepts of Gatsby and its powerful features.
And post-2.md:
---
title: "Optimizing Images in Gatsby"
date: "2023-10-05"
slug: "optimizing-images-in-gatsby"
description: "Explore how to optimize images in your Gatsby project." featureImg: ../images/featured/image-2.jpeg
---
Images play a crucial role in web development. In this post, we'll dive into how to optimize images in Gatsby with plugins.
They are Markdown files contain frontmatter with metadata for blog post, which includes names and dates, as well as slugs, descriptions and images.
In Gatsby, GraphQL can query the Gatsby novel.
When you run gatsby develop
in your terminal, you'll notice that, in addition to the link gatsby-source-filesystem, which opens your project on the web, you also see the http://localhost:8000/___graphql URL. This link gives access to the GraphiQL editor that you can use for your Gatsby project.
When you open the editor, you see the following interface:
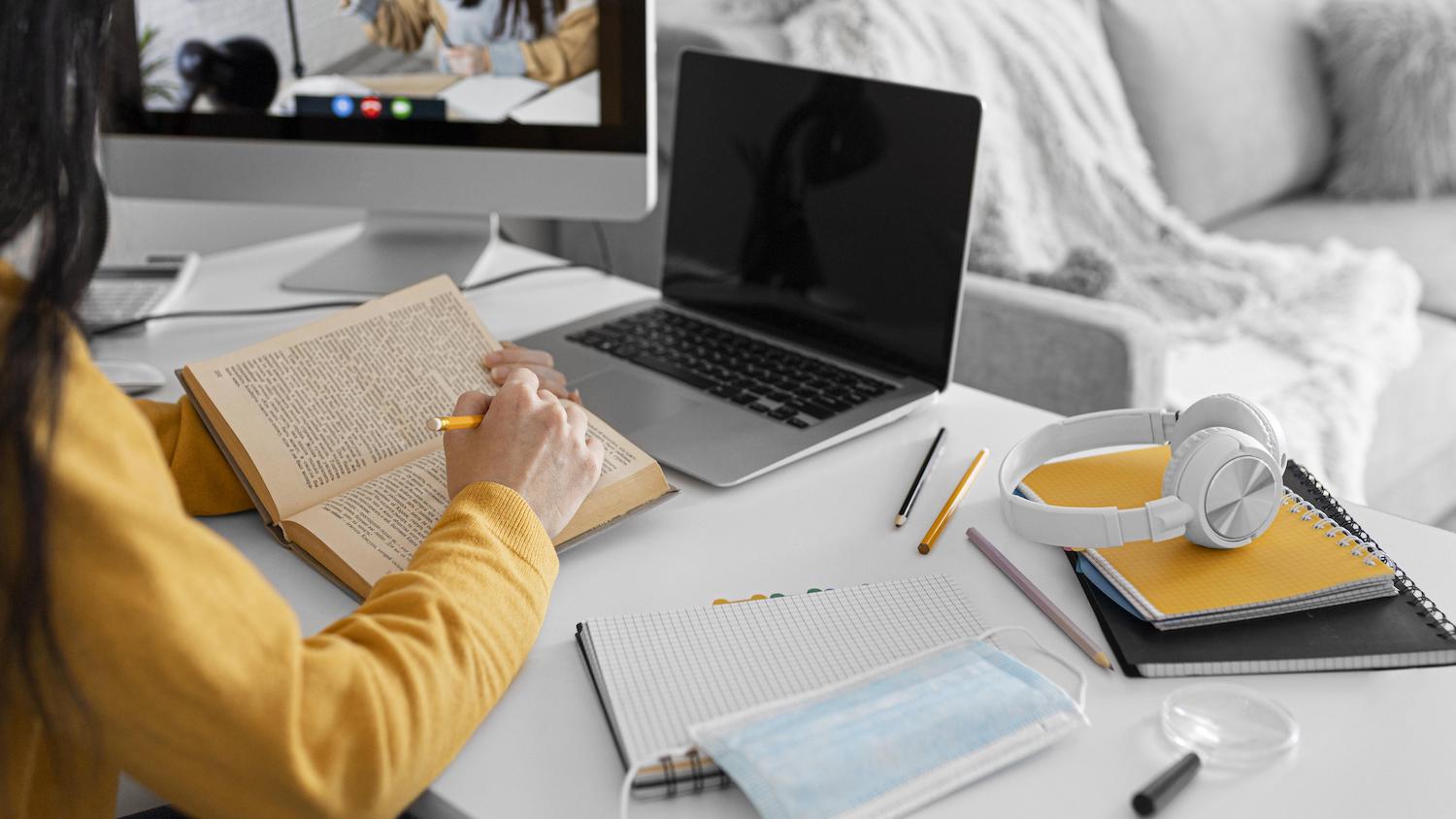
It is possible to get as much information about your site from this editor. It is because you've made markdown files and completed the configurations in your gatsby-config.js file. We can query the Markdown files and their content by running the following query within the editor:
query BlogList •
This query retrieves information from all Markdown files by using allMarkdownRemark
. It extracts the name
, slug
, and description
from the front matter of each Markdown file as well as their id
.
After writing your query, hit after writing your query, click the "Play" button (a left-facing triangle) to perform the query. The result will be shown in the upper right corner of the editors.
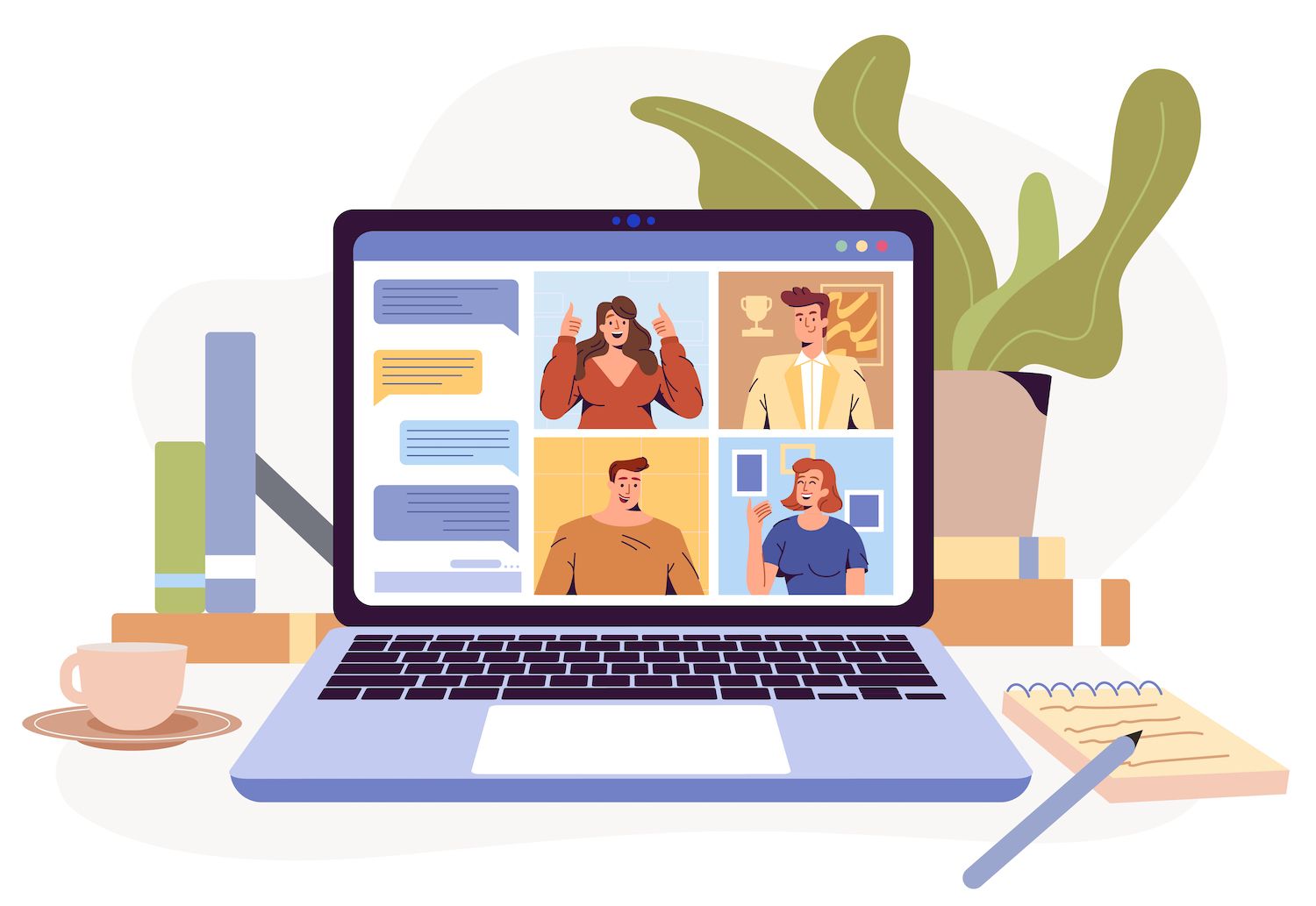
It is then possible to use GraphQL to access the Markdown data within your components or pages. For querying this data on blog/index.js, on the blog/index.js page, first install graphql
from the gatsby package
. Then, at the bottom of your JSX code, add the following information:
export const query = graphql`
query BlogList
allMarkdownRemark
nodes
frontmatter
title
slug
description
id
`;
In the above code it uses this graphql
tag to generate a GraphQL query, which is referred to as query
. What your blog/index.js file should look in the following format:
import graphql, Link from 'gatsby';
import React from 'react';
import Layout from '../../components/Layout';
import * as styles from '../../styles/blog.module.css';
const blog = ( data ) =>
const posts = data.allMarkdownRemark.nodes;
return (
Blog
posts.map((post) => (
post.frontmatter.title
post.frontmatter.description
))
);
;
export default blog;
export const query = graphql`
query BlogList
allMarkdownRemark
nodes
frontmatter
title
slug
description
id
`;
In the above code, you use the query
results using your information
prop inside your component. After that, go through the post
data with the JavaScript method. Then, show the posts' titles as an order.
To avoid errors, create a blog.module.css file in the styles folder. Then, add the following code:
.blog_cont h2
font-size: 40px;
margin-bottom: 20px;
.blog_grid
display: grid;
grid-template-columns: 1fr 1fr 1fr;
gap: 20px;
@media (max-width:700px)
.blog_grid
grid-template-columns: 1fr;
.blog_card
background-color: #bfbfbf;
padding: 20px;
border-radius: 5px;
color: #000;
transition: all .5s ease-in-out;
.blog_card:hover
background-color: #5333ed;
color: #fff;
.blog_card h3
margin-bottom: 15px;
.blog_card p
margin-bottom: 15px;
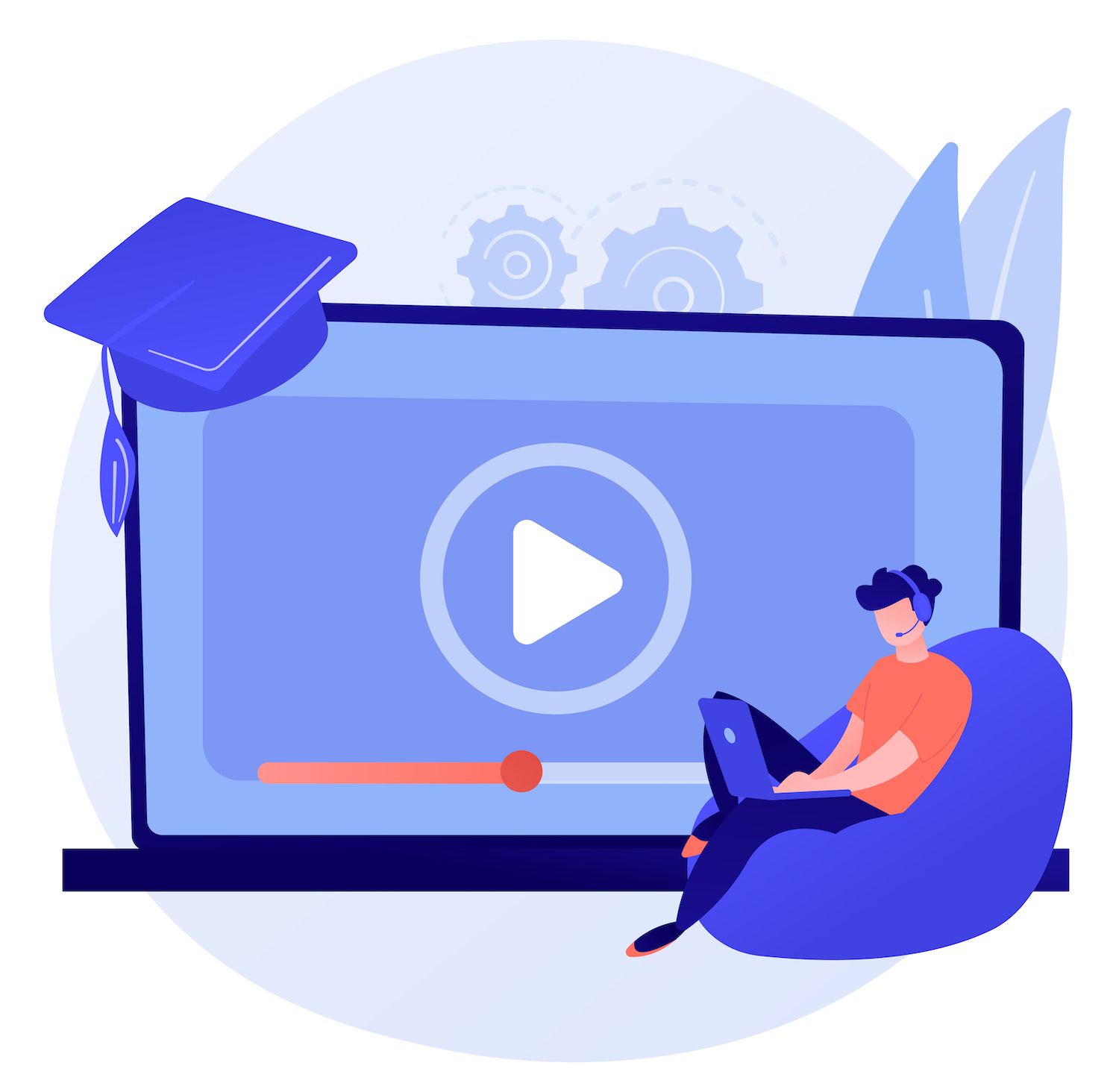
Learning Templates and Generating Dynamic Pages In Gatsby By Using GraphQL
In Gatsby the Gatsby, templates and dynamic pages are essential principles that let you create flexible and data-driven websites. Templates allow you to define the layout and structure of your pages. GraphQL assists you in obtaining information to fill these templates automatically.
Making a template for Blog Posts
Let's say you want to build a blog in which each post on the blog has an identical structure that includes an introduction and a contents. Create a BlogDetails
template to create this layout. Within the src folder, you can create an template folder. Then, create blog-details.js file: blog-details.js file:
import React from 'react';
import Layout from '../components/Layout';
import * as styles from '../styles/blog-details.module.css';
const BlogDetails = () =>
return (
Title
className=styles.html
dangerouslySetInnerHTML=
/>
);
;
export default BlogDetails;
In this instance, the BlogDetails template defines the format for blog post. Next, let's use GraphQL to fetch data for particular blog posts, and then pass it as props to the template.
Generating Dynamic Pages
To create dynamic pages make a gatsby-node.js file in your project's root directory. This file will allow you to specify how pages are generated.
In the gatsby-node.js file, utilize GraphQL to access the information you want to use for dynamic websites. In the case of, for instance, if you've Markdown blog posts, you can query their slugs:
const path = require(`path`);
exports.createPages = async ( graphql, actions ) =>
const data = await graphql(`
query Articles
allMarkdownRemark
nodes
frontmatter
slug
`);
data.allMarkdownRemark.nodes.forEach((node) =>
actions.createPage(
path: '/blog/' + node.frontmatter.slug,
component: path.resolve('./src/templates/blog-details.js'),
context: slug: node.frontmatter.slug ,
);
);
;
In this example in this example, we search the slugs for all Markdown posts and create dynamic pages for each article making use of this BlogDetails template. The context object is used to provide data to the template. The data (slug) is what the template is using to get other data that aligns with the slug.
We must first know how image optimization works in Gatsby prior to adding a GraphQL query to your template's page.
Image Optimization in Gatsby
Earlier, you installed and configured the gatsby-transformer-sharp and gatsby-plugin-sharp along with gatsby-source-filesystem for sourcing images.
By using these plugins, you can query and optimize images using GraphQL. Here's an example of how to query and display an optimized image using the gatsby plugin-sharp:
export const query = graphql`
query
file(relativePath: eq: "example.jpg" )
childImageSharp
fluid
...GatsbyImageSharpFluid
`;
In the code above in the above code, you're requesting an image named example.jpg from the images source and then using the fluid property of the image to show it in a the most responsive and optimized rendering.
Then, you can import the image into images-gatsby to create optimized images.
import React from 'react';
import graphql from 'gatsby';
import Img from 'gatsby-image';
const ImageExample = ( data ) =>
const fluid = data.file.childImageSharp;
return (
);
;
export default ImageExample;
Queuing Dynamic Pages
Gatsby is using the template to build distinct pages for every blog post. Let's now include a GraphQL query on the template page to fetch the relevant data, based on the URL:
import graphql from 'gatsby';
import Img from 'gatsby-image';
import React from 'react';
import Layout from '../components/Layout';
import * as styles from '../styles/blog-details.module.css';
const BlogDetails = ( data ) =>
const html = data.markdownRemark;
const title, featureImg = data.markdownRemark.frontmatter;
return (
title
);
;
export default BlogDetails;
export const query = graphql`
query ProjectDetails($slug: String)
markdownRemark(frontmatter: slug: eq: $slug )
html
frontmatter
title
featureImg
childImageSharp
fluid
...GatsbyImageSharpFluid
`;
In the above code, you will notice it is querying for the optimized image and querying for the blog entry that matches the slug.
Check out the complete source code of the Gatsby project at GitHub.
Install Gatsby static websites
When your repo is done then follow these steps for deploying your static website to :
Sign in or create an account to see the dashboard of your My dashboard.
Authorize with your Git provider.
Go to Static Sites in the sidebar left, then select Add Site. Add Site.
Choose the repository you want to deploy from and also the branch you wish to deploy from.
Assign a unique name to your site.
My will recognize the build settings for this Gatsby project automatically. You will see the below settings already filled in:
Command to build: npm run build
Node version: 18.16.0
Publish directory: public
Then, click on the Create Site button..
Summary
This book explains the basic concepts of data source, routing, the styling of images, image optimization plug-ins, deployment and plenty more.
Gatsby's speed, flexibility and diverse ecosystem makes it a powerful option for creating static websites. Whether you're creating an individual blog, a portfolio site or even a corporate website, Gatsby has you covered.
Now it's time to put your knowledge into practice and build your very own Gatsby website. Have you utilized Gatsby for building anything? Feel free to share your projects and experiences with us in the comments in the section below.
Joel Olawanle
Joel is an Frontend developer working at as an Editor for Technical Issues. He is a fervent educator with a love of open source software and has written more than 200 technical pieces, mainly on JavaScript and its frameworks.
Website
LinkedIn
Twitter