How to build the foundation of a WordPress theme using React - (r)
>
>
The code within the index.php file defines the primary HTML structure of the WordPress theme. It also contains hooks to allow WordPress to include the necessary headers (wp_head) and footers (wp_footer) as well as a div containing the ID app where it is where the React application is mounted.
Create React using @wordpress/scripts
- Open your terminal and browse to your theme's directory:
cd wp-content/themes/my-basic-theme
- Create a fresh Node.js project:
npm init -y
- Install
@wordpress/scripts
and@wordpress/element
:
Install npm @wordpress/scripts and @wordpress/element --save-dev
Note that this step can take a few minutes.
- Modify your package.json File to contain a
start
andbuild
script:
"scripts":
"start": "wp-scripts start",
"build": "wp-scripts build"
,
The '@wordpress/scripts'
can be used to begin an application development server that is hot reloading in order to facilitate development ( start
) and also to build the React application into static assets that can be used for the production ( build
).
Create a React project
- Create a new directory named src for the React source files within the theme.
- In in the folder src folder, you can create two files new: index.js and App.js.
- Input the code below into index.js :
import render from '@wordpress/element';
import App from './App';
render(, document.getElementById('app'))
The above code is importing rendering functions from the rendering
function that is in @wordpress/element
and the App
component. It then mounts the App
component to the Document Object Model (DOM).
- After that, paste this code into App.js file:
import Component from '@wordpress/element';
export default class App extends Component
render()
return (
Hello, WordPress and React!
/* Your React components will go here */
);
Make sure you activate and finalize your theme
To activate your theme:
- Run the development server with
npm start
. - Then, activate the theme from the WordPress dashboard by going to the Appearanceand then Themes choosing the theme you want to activate, then clicking to activate.
- Make sure the React project's build processes are set up so that it outputs to the appropriate theme directory, allowing WordPress to render your React components.
- Visit your WordPress site's frontend to see the latest changes.
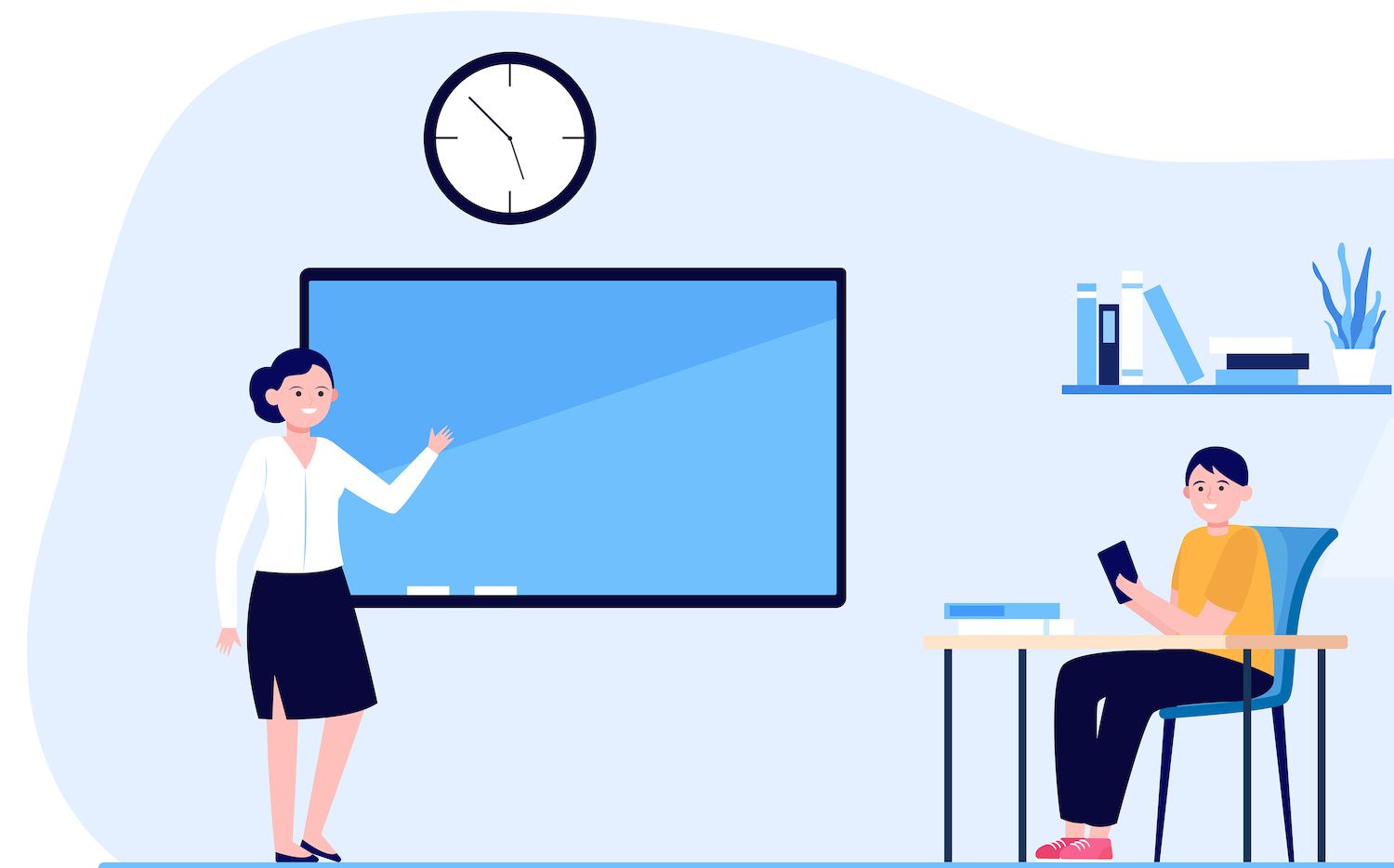
Create React elements for your theme
Then, you can follow the component-based method to enhance the basic React configuration for your WordPress theme by adding specific elements such as the header.
Design the header component.
Within your theme's src directory, you can create an entirely new file to house the header component. Name it like Header.js, and add the following code:
import Component from '@wordpress/element';
class Header extends Component
render()
const toggleTheme, darkTheme = this.props;
const headerStyle = returning (
Header Style = headerStyle>My theme for WordPress
OnClick button = toggleTheme>arkTheme ? 'Light Mode' : 'Dark Mode'
);
export default Header;
This code defines a header component by using the '@wordpress/element'
that is dynamically styling the header using darkTheme prop
. The component has a button that allows users to toggle between light and dark themes using toggleTheme. toggleTheme
method passed as a prop.
Make sure to include the footer component
Within your theme's src directory, you can create an entirely new file to house the footer component. Give it a name -- for example, Footer.js -- and add the following code:
import Component from '@wordpress/element';
class Footer extends Component
render()
const darkTheme = this.props;
const footerStyle =
backgroundColor: darkTheme ? #EEE: '#333',
color darkTheme? 'white' : '#333',
padding: '20px',
textAlign: 'center',
;
return (
style=footerStyle>
(c) new Date().getFullYear() My WP Theme
);
export default Footer;
This code defines a footer component that creates a footer using dynamic styling based on the darkTheme
prop. The footer displays the current year.
Refresh the App.js file
In order to make use of the brand new header and footer styles, simply replace the content of the App.js file with the following codes:
import Component from '@wordpress/element';
import Header from './Header';
import Footer from './Footer';
export default class App extends Component
state =
darkTheme: true,
;
toggleTheme = () =>
this.setState(prevState => (
darkTheme: !prevState.darkTheme,
));
;
render()
const darkTheme = this.state;
return (
);
The builder process for development, which watches for changes in your code and compiles it, should still be active. So, your last version of your template must be similar to the following:
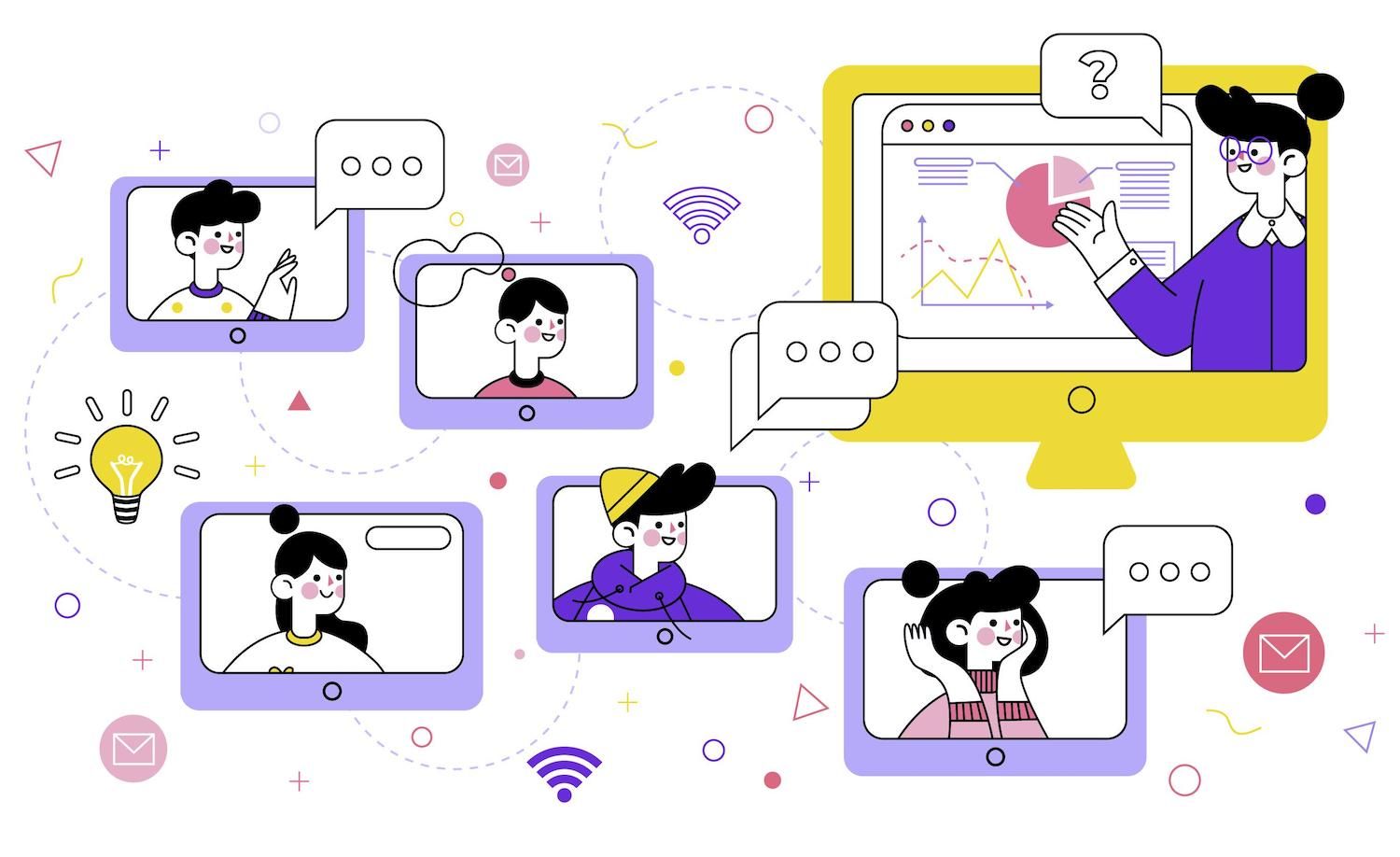
What is the best way to deal with WordPress information in React
In your theme's src directory, make an additional file called Posts.js and add the following code:
import Component from '@wordpress/element';
class Posts extends Component
state =
posts: [],
isLoading: true,
error: null,
;
componentDidMount()
fetch('http://127.0.0.1/wordpress_oop/wp-json/wp/v2/posts')
.then(response =>
if (!response.ok)
throw new Error('Something went wrong');
return response.json();
)
.then(posts => this.setState( posts, isLoading: false ))
.catch(error => this.setState( error, isLoading: false ));
render()
const posts, isLoading, error = this.state;
if (error)
return Error: error.message;
if (isLoading)
return Loading...;
return (
posts.map(post => (
))
);
export default Posts;
The fetch('http://127.0.0.1/wordpress_oop/wp-json/wp/v2/posts')
URL could be slightly different depending on the WP deployment name -- that is, the folder where you installed WP. Alternatively, you can take the site hostname from the Dev dashboard and append the suffix /wp-json/wp/v2/posts
.
The Posts
component is an excellent example of this integration, demonstrating the process of fetching and controlling WordPress information -- particularly posts using WordPress REST API. WordPress REST API.
By initiating a network request inside the component's lifecycle process, componentDidMount
, the component is able to efficiently fetch content from a WordPress website and saves it in its current state. This technique demonstrates a method for dynamically incorporating WordPress data, such as posts or custom types of post, into React components.
For the use of the new component, change the content in App.js file App.js file with the following code:
import Component from '@wordpress/element';
import Header from './Header';
import Footer from './Footer';
import Posts from './Posts'; // Import the Posts component
export default class App extends Component "
You can now check the latest and final version of your theme. Alongside the header and footer it consists of an interactive content area where you can see the content.
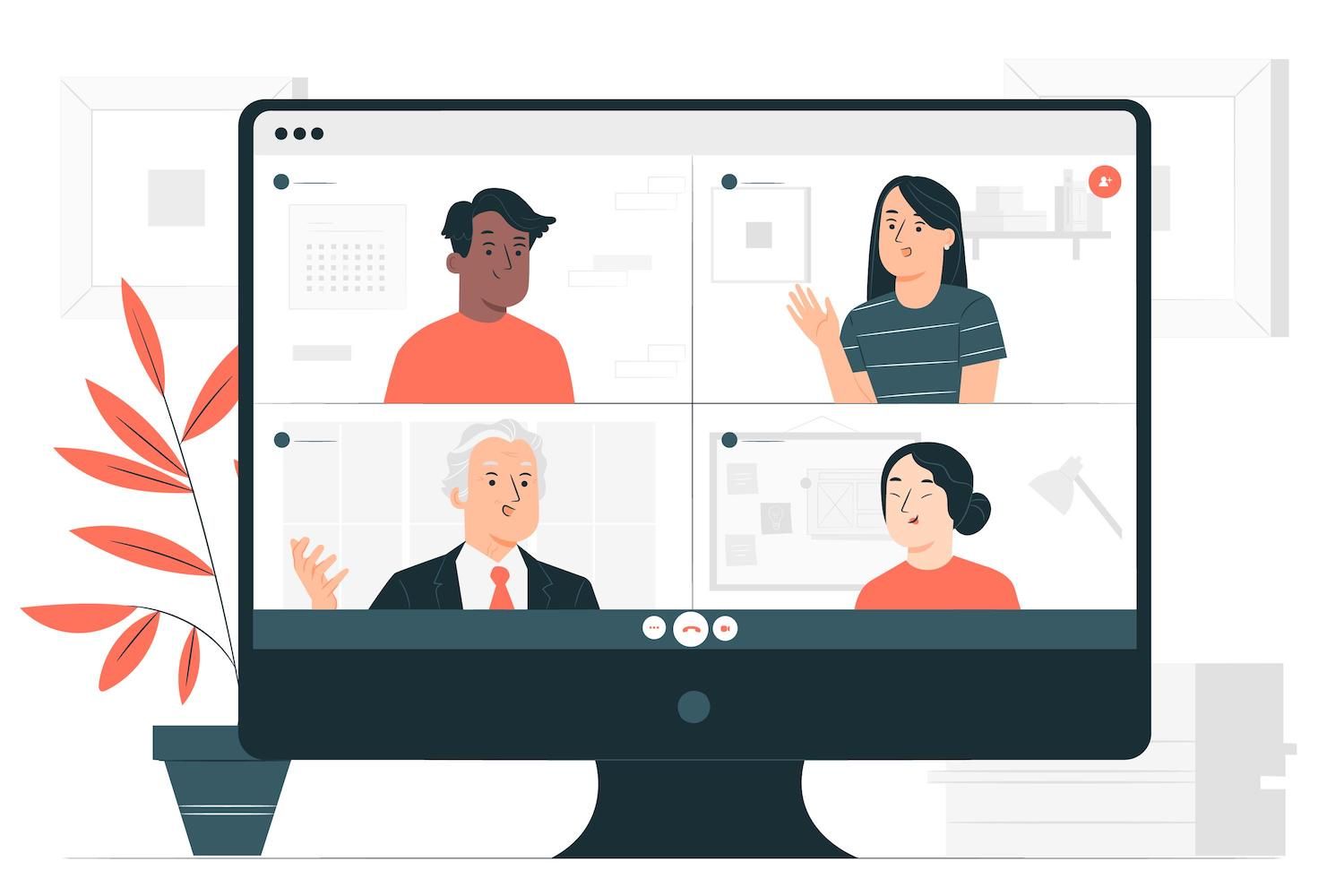
Utilize React WordPress as the React WordPress theme in a WordPress project
Integration of a React-based theme in an WordPress project starts by converting React code into a WordPress compatible format using packages such as @wordpress/scripts
. This tool simplifies the build process, letting you compile React programs into static assets that WordPress can enqueue.
It is easy to build the theme by using the npm command provided from @wordpress/scripts
. When you run npm run build
in the directory of your theme creates the React program into static JavaScript and CSS documents.
After that, place the assembled assets into the proper directory in the theme. This is to ensure that WordPress can properly load and render the React components as part of the theme. Once integrated, you can start using React as a React WordPress theme like any other, instantly bringing an app-like, modern user experience on the WordPress website.
Summary
When you build and integrate the theme in WordPress by using React's robust UI features, you can create flexible, highly interactive, and experience-driven web pages.
Do you think of creating an WordPress theme that uses React? Do you have any suggestions for the reasons you believe it's the best and how to go about the process.
Jeremy Holcombe
Content & Marketing Editor , WordPress Web Developer, and Content Writer. In addition to everything related to WordPress I like playing golf, at the beach and watching movies. Additionally, I'm tall and have issues ;).