How To Create a Blog using Laravel - (r)
@yield('content') Mini-Blog (c) 2023 [email protected]<\/a>">[email protected]<\/a>/dist/js/bootstrap.bundle.min.js" integrity="sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4" crossorigin="anonymous">
By using this HTML codes, it is possible to can import Bootstrap version 5.2.3 and Vite to combine the JavaScript as well as CSS assets. The page you create is a header that includes a navbar and a footer, with scripts below. The body contains dynamic content is rendered from different Blade files using the power from @yield('content')
.
postsdirectory postsdirectory is where you can find the Blade files needed to perform the read and create actions.
- Inside within the postsdirectory, create inside the postsdirectory, create a Blade file named index.blade.php and add the below code:
@extends('layouts.app')
@section('content')
Blog list
The Blog 1 - $post
@endsection
The code is extended beyond that app.blade.php file on the layouts page. When it is rendered in your browser, it displays the blog's content article and also the navigation bar and footer inherited from the app.blade.phpfile in the layouts folder. Between the tag sections, you can pass the contents from the controller to render within the browser after you run the app.
- Create the route using the routesdirectory. This allows to load the route automatically of the
RouteServiceProvider
located in the App/Providers directory. TheRouteServiceProvider
will be the main class responsible for loading the application's route files. - Inside the routes/web.php file, import PostController using
use AppHttpControllersPostController
. - Then, set the route by adding
Route::resource('posts', PostController::class);
to the routes/web.php file. - With the Vite development server running Use
PHP artisan server
to run the program in your terminal. - With your browser, open
http://127.0.0.1:8000/posts
to see your new blog post list.
The webpage should appear as these:
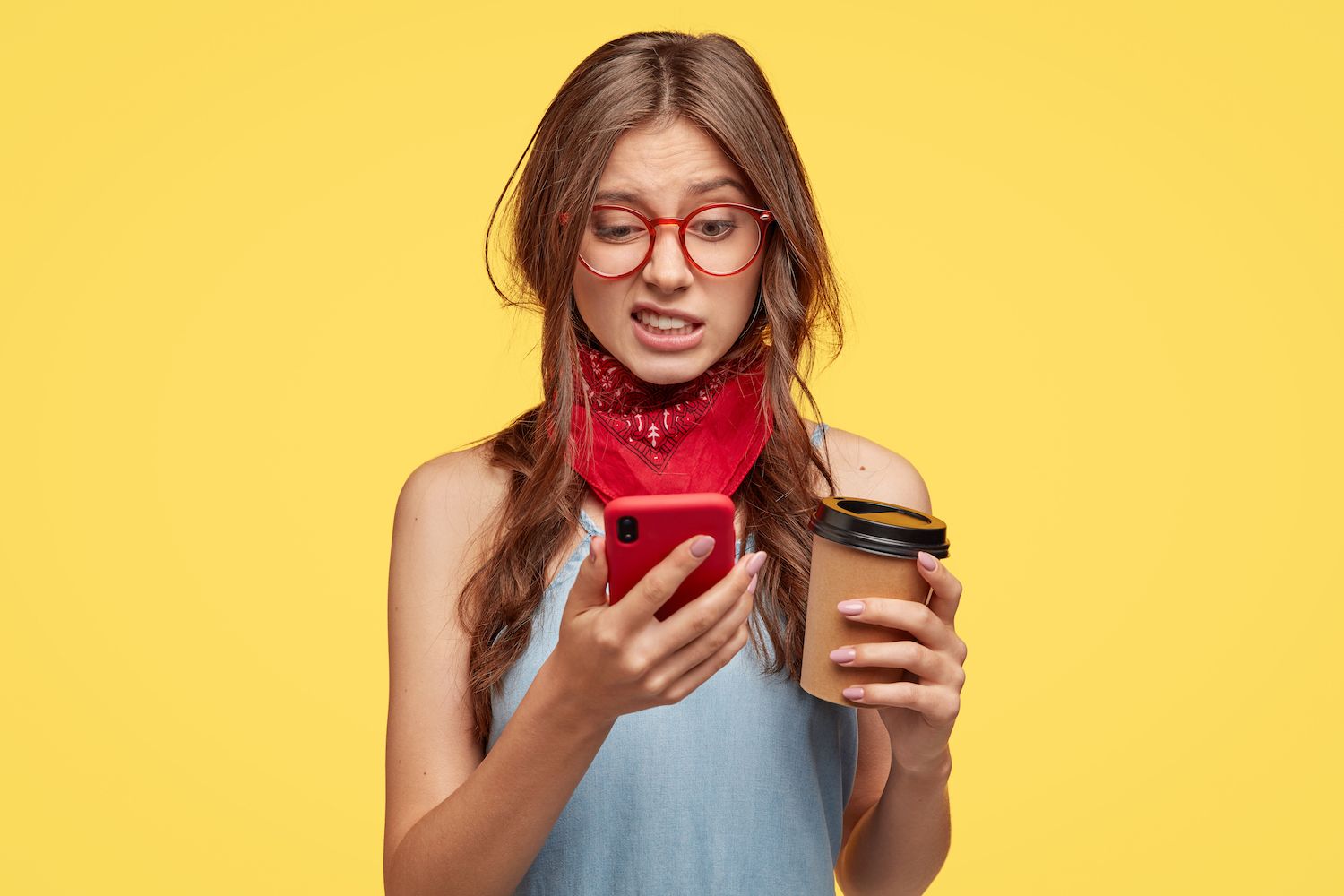
In the next section In the following section, we'll define the controller methods for displaying the entire posts, creating posts, and then storing the post. Add their routes, and then create Blade file in the appropriate sections.
Design blog post Page
Make blog posts by inputting the title, providing a description as well as uploading images. Then, display your posts in a sequential manner.
- In the app/Models directory, open in the app/Models directory, open Post.php file.
- In the
Post
class, below it,use HasFactory;
code block, insertthe protected fillable value ['title', 'description', 'image'];
.
This program protects your model's features from being assigned to mass.
- In your app/Http/Controllers/PostController.php file, import the
Post
model usinguse AppModelsPost;
. - Replace the
index
andcreate
controller methods previously created within thePostController
class by using this code:
// Show all posts
public function index()
$posts = Post::orderBy('created_at', 'desc')->get();
return view('posts.index', ['posts' => $posts]);
// Create post
public function create()
return view('posts.create');
With the index
method that you have just developed in the index method you just created, the PHP application collects all post, puts them in chronological order, and then stores them into the posts
variable. The return view shows the posts pass into the index.blade.php file as an instance variable within the views/posts directory. The create
method generates the create.blade.php file and puts it into the views/posts directory, if the user tries to make the post.
- Create the
store
controller using the following code (to keep blog entries in the database). Incorporate this code into thePostController
class beneath index. Add this code to theindex
anddevelop
controller methods.
// Store post
public function store(Request $request)
// validations
$request->validate([
'title' => 'required',
'description' => 'required',
'image' => 'required
The storage
method handles client requests regarding the data inside its body. As such, it will take a the request
as an argument. After that, you check the fields that are used to create posts and create an instance of a Post
instance by using the Post
model. Field data that you enter is transferred to the newly created instance and then saved. The site redirects users back to index
page, which displays a flash text that says, "Post created successfully."
Create Routes for Your Posts
To add the routes to your web.php file:
- Within the routes directory of your project's root directory, you can open web.php. web.php file.
- Register the routes for the controller methods, replacing the existing code with this:
names([
'index' => 'posts.index',
'create' => 'posts.create',
'store' => 'posts.store',
'show' => 'posts.show',
]);
The controller utilizes these routes to build, store, and display the data objects you have created.
Make Blade Files
To create the views, return in the class PostController
class:
- In the resources/views/posts directory, make a Blade file called create.blade.php and add the code below:
@extends('layouts.app')
@section('content')
Add Post
@csrf
@if ($errors->any())
@foreach ($errors->all() as $error)
$error
@endforeach
@endif
Title
Description
Add Image
Save
@endsection
In this code, create.blade.php inherits the contents of app.blade.php in the layouts directory using @extends('layouts.app')
. This includes a heading, navigation bar and footer. After adding the Add Post
text within the h1
tag, you created a form with the post
method that contains the route('posts.store')
action.
The code enctype="multipart/form-data"
allows for image uploads, and csrf
protects your form from cross-site attacks. Then, the error messages display invalid field entries, and uses fields attributes
to make labels and inputs for the form.
- Replace your code inside your index.blade.php file with this code, which will display all the blog posts:
@extends('layouts.app')
@section('content')
Add Post
Mini post list
@if ($message = Session::get('success'))
$message
@endif
@if (count($posts) > 0)
@foreach ($posts as $post)
$post->title
$post->description
@endforeach
@else
No Posts found
@endif
@endsection
This adds the "Add Post" button. If clicked, it generates a post and passes any information into the body of the page. The if
condition determines for data within the database. If it is the condition passes. Otherwise, the screen will display "No Posts found."
Design Your Pages
Now you can use PHP artisan server
to publish and show blog posts. Open http://127.0.0.1:8000
, and the page should look like this:
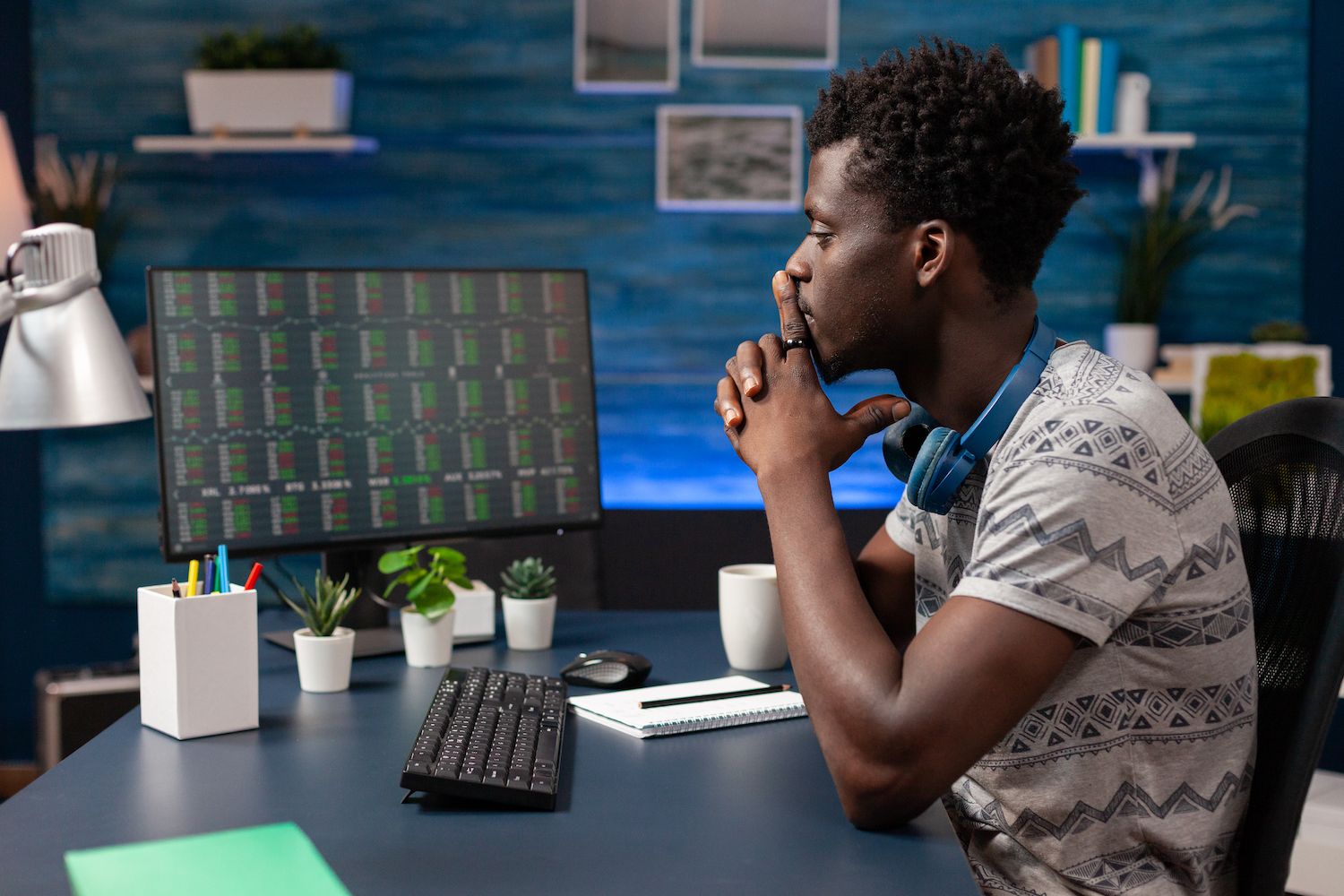
If you add a blog, it will look in the following manner:
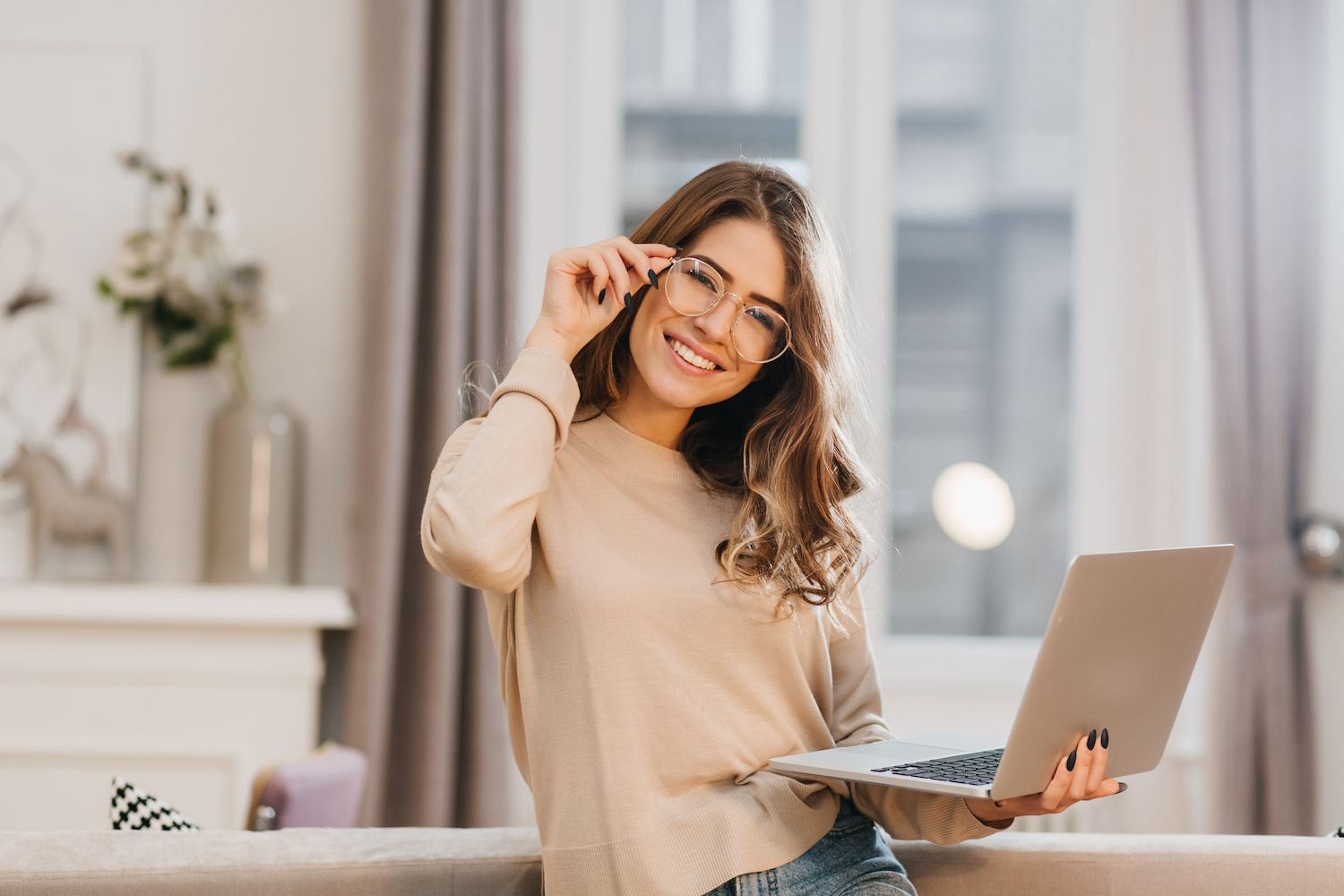
Deploy Your Laravel Blog on
- Make a .htaccess file.
- Push the code to a repository.
- Set up an online database.
- Set up a project on My.
- Create and publish your blog.
Make an .htaccess file.
Within the root directory of your project make a new file named .htaccess, and include the following code in it:
RewriteEngine On
RewriteRule ^(. *)$ public/$1 [L]
This code redirects the application's requests via public/index.php in the deployment.
Send Your Code into the Repository
Make a repository of your project and publish your code. It is possible to use GitHub, GitLab, or Bitbucket to place your code on the web and to upload it to My.
Create the database in Your My Dashboard
Create a database of My:
- Click on the "Add Service button, and then select the Database option..
- Input the information for your database in the following format. Make sure that, for the installation to succeed, you must leave the Database username as the default value.
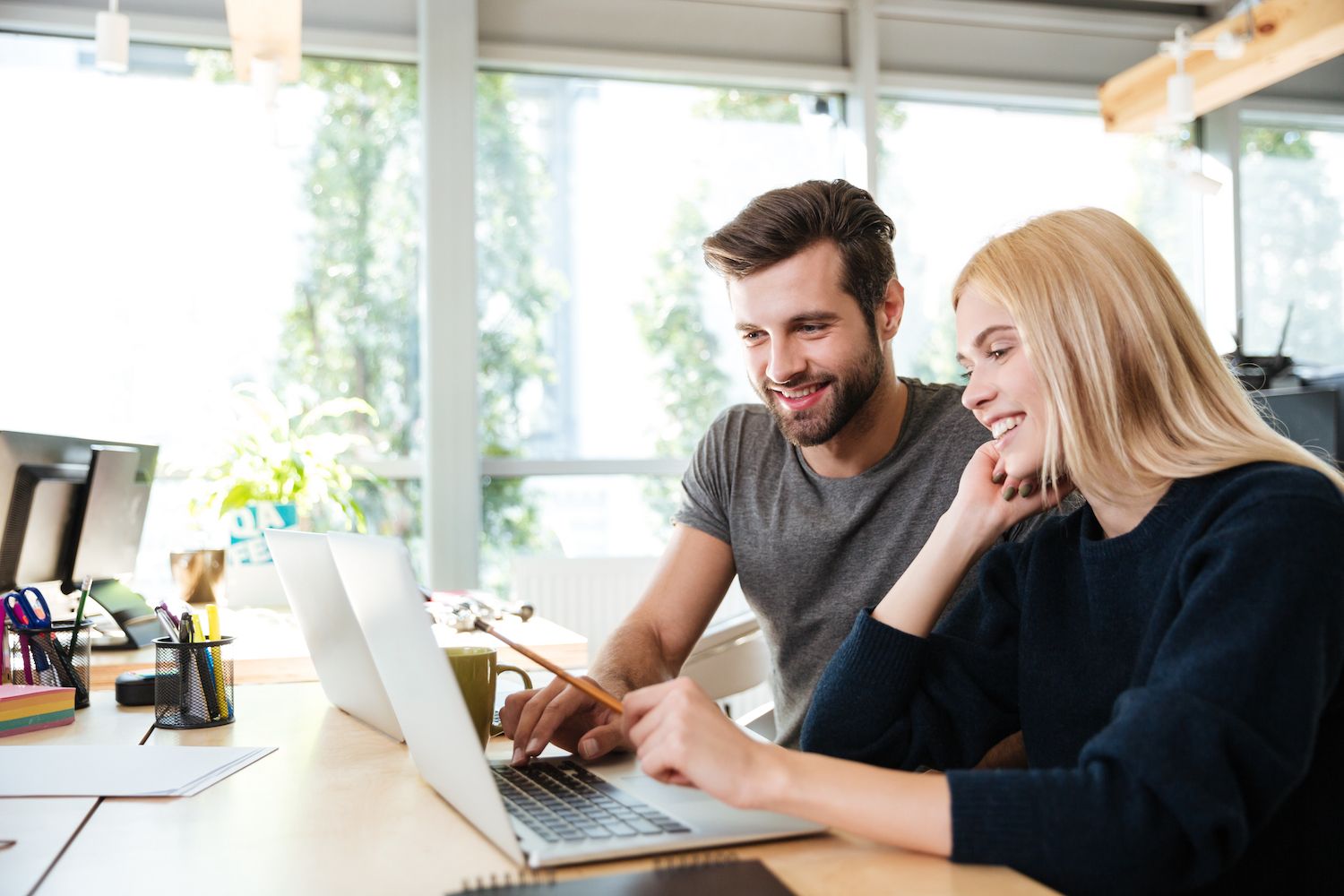
The details include the Database Name, Display Name, Database type, Version, Database username, Data Center location and the size. This example uses MariaDB as the database and the dimension can be described as Db3 (1CPU / 4GB RAM/10GB Disk Space, 65 USD per month). It is possible to select the type of database and size to meet your particular requirements.
- Click Continue.
- Verify your monthly costs as well as the payment method. Then select Create database.
Set Up the Project to My
In order to deploy your application My:
- Click the Dashboard panel.
- Simply click Add Service and choose the Application like the following:
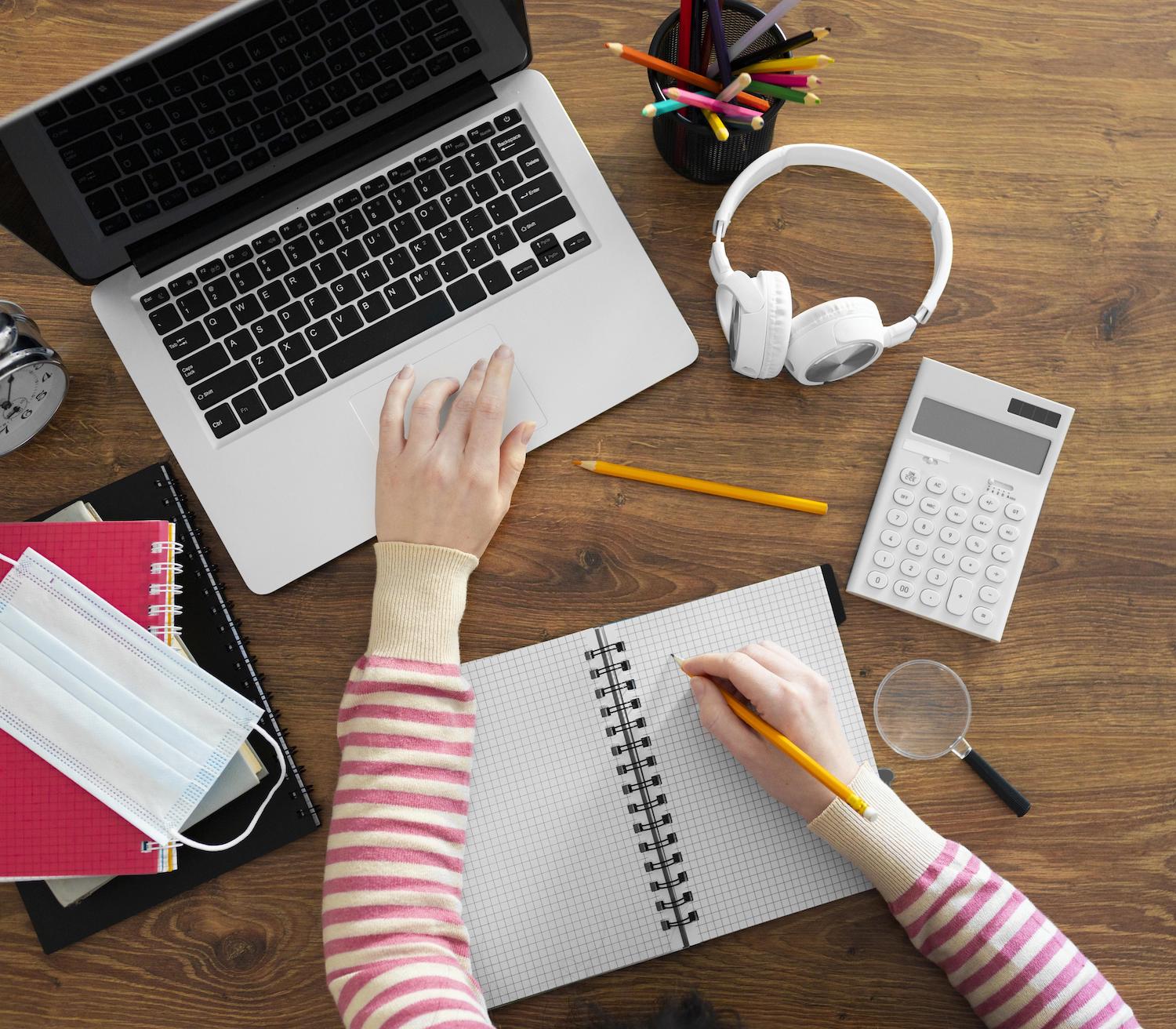
My will take users to Apply Application page.
- Under the Select branch card section, click your repository on GitHub, and then click the Add deployment to commit checkbox.
- In the Basic information in the basic details section, type in your application name and select the location of your data center for the application.
- As Laravel requires an app-specific key during deployment, in the environment variables card, include an app key as Key 1. The key can be used as the APP_KEY defined in your locally stored .env file or make use of an online key generator for Laravel to get one.
- Click Continue.
- Pick the appropriate build resource (CPU and RAM) for your application. This demonstration employs the typical build machine (1CPU / 4 GB RAM) + 0.02USD per minute.
- Make sure to leave the Image of the container to be set up in place by pressing the radio button.
- Click to continue.
- In the Setting up your process webpage, you can modify the pod size as well as the type of instance, by choosing these boxes. This example uses the defaults.
- Click to Continue.
- Then, press then the "Confirm Payment Method" button to initiate your application's deployment. Clicking this also directs you to the information about your deployment page, where you will be able to see your progress on your deployment.
Develop and Launch Your Application
Once the application and database are hosting, connect the database to your application, and then build it the application to be deployed.
To connect to your database, use the external connections of your hosted database. In the Infotab of your hosted database there are External connections which are as follows:
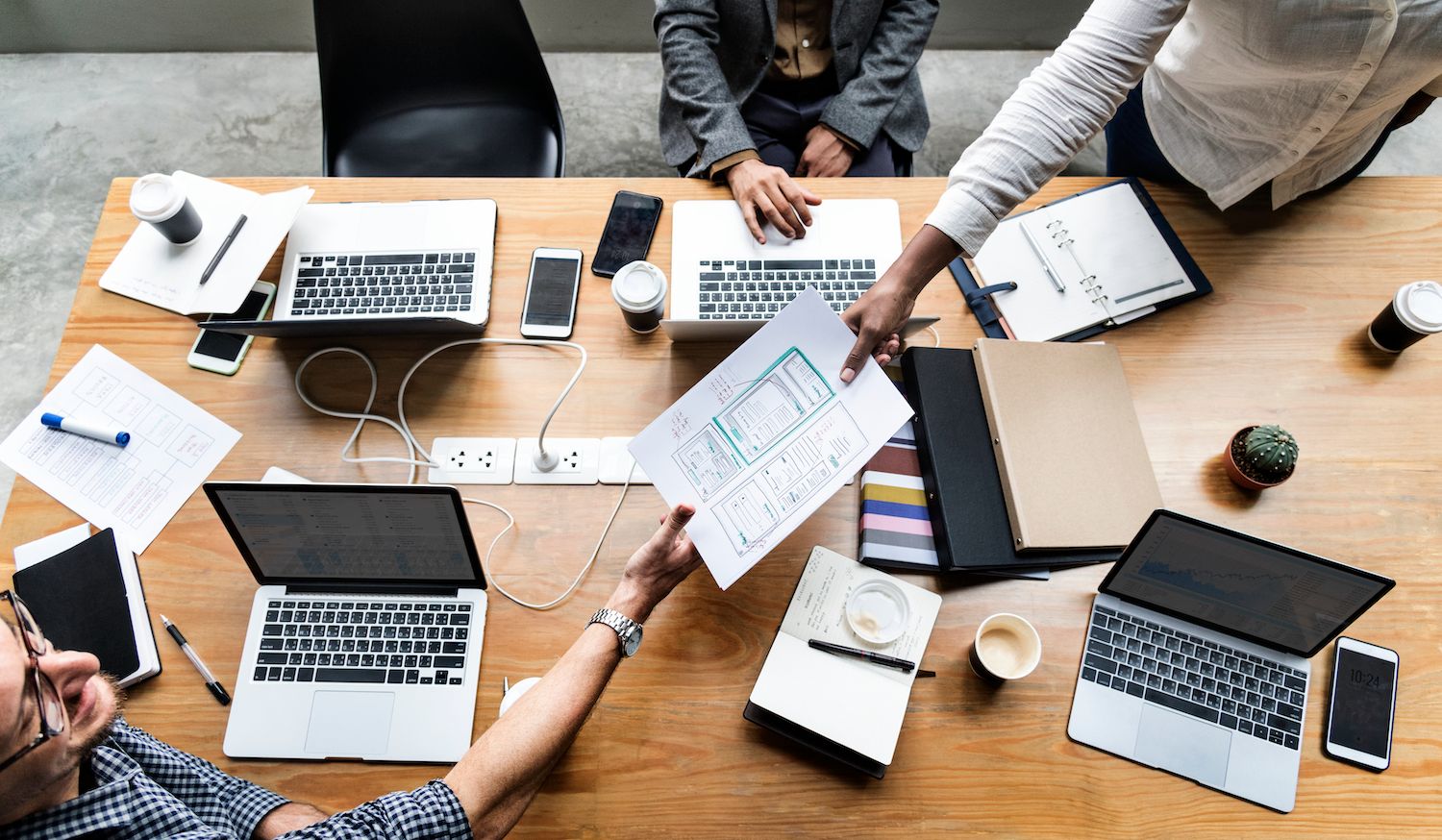
- In the app's Settingspage, navigate towards the Environment variable card.
- Click Add an environment variable to add the external connections to your database hosted by the value that corresponds to it. Use the same variables found included in the .env file.
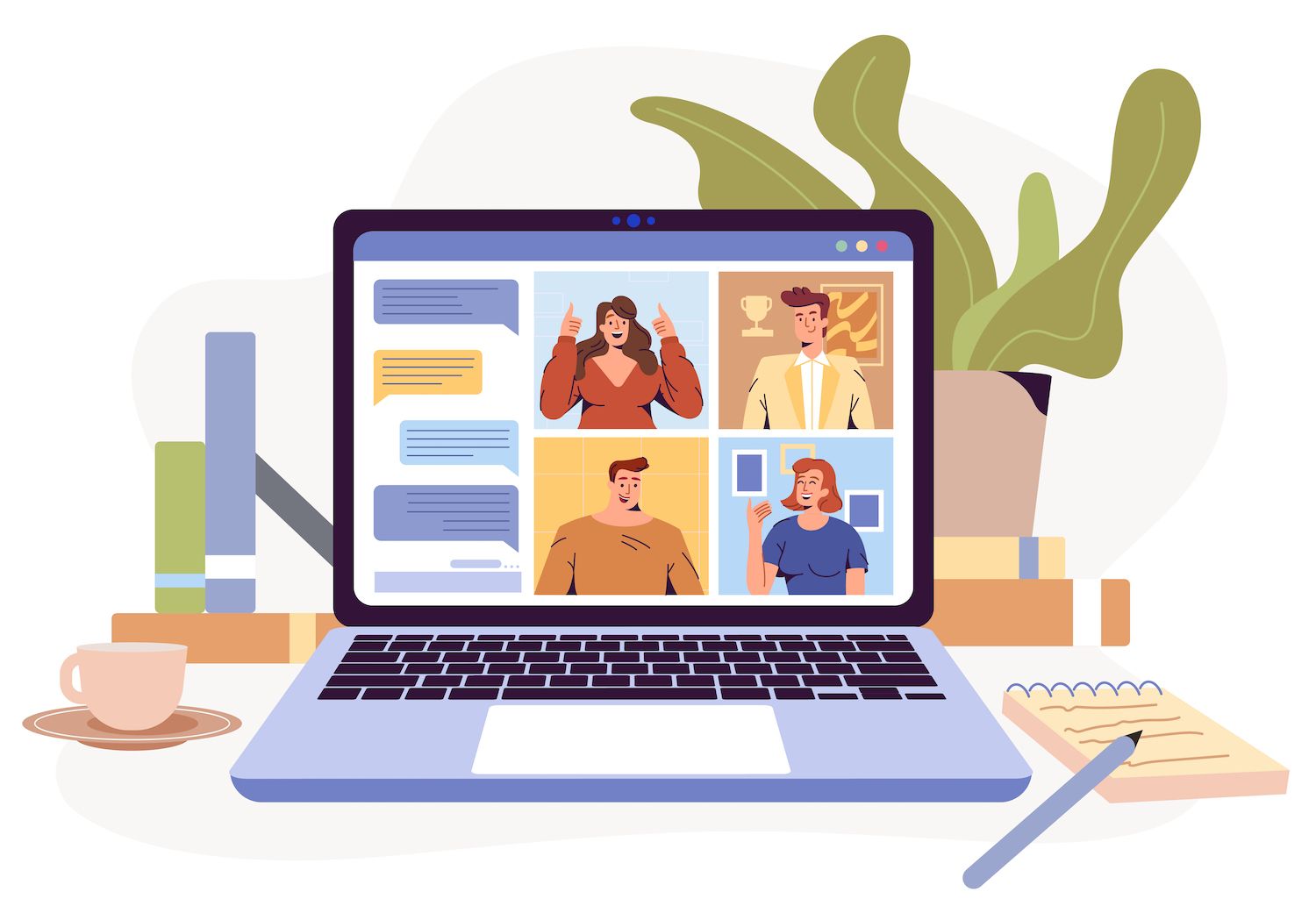
This picture is helpful to consider marking env
variables you edited manually in order to distinguish them from other ones.
The app_urlis the URL for the application you host, and DB_CONNECTION is mysql
.
- Visit your app's settings page.
- Within the Buildpackcard, add PHP as well as Node.js to build packs. Since it is a PHP application, it is necessary to install the PHP build pack after Node.js.
- Click Install now to build your application.
Then, you can add a procedure to migrate your database.
- Visit the Processestab of your host application's page.
- Choose the the Create process on the process card.
- Input Migration as the name, Background worker as the kind and
PHP artisan migrate
to initiate the command. You can leave the pod dimensions and the number of instances using the defaults. - Choose Continueto begin the application. This will trigger a brand new build and redeploys the application.
- In your domainstab of your application, click the link for your application. You can see that it's currently running.
- Note that the blog application deployed to My displays no blog posts. Create a new post by typing the title of your post, adding a description, selecting an image.
Summary
Laravel lets you make a basic blog in a short time. Its rapid page loading and robust controller design and a dependable security system make improving an application's performance easy. In addition, My lets you release and deliver your online applications quickly and effectively. My's pricing structure is based upon usage which eliminates any hidden costs.
Marcia Ramos
I'm the Editororial Team Leader at . I'm a fan of open source and am a huge fan of coding. Over the past seven years of technical writing and editing for the tech industry, enjoy working with colleagues to create short and precise pieces of content and improve processes.