How to Create A WordPress Website Using API (r)
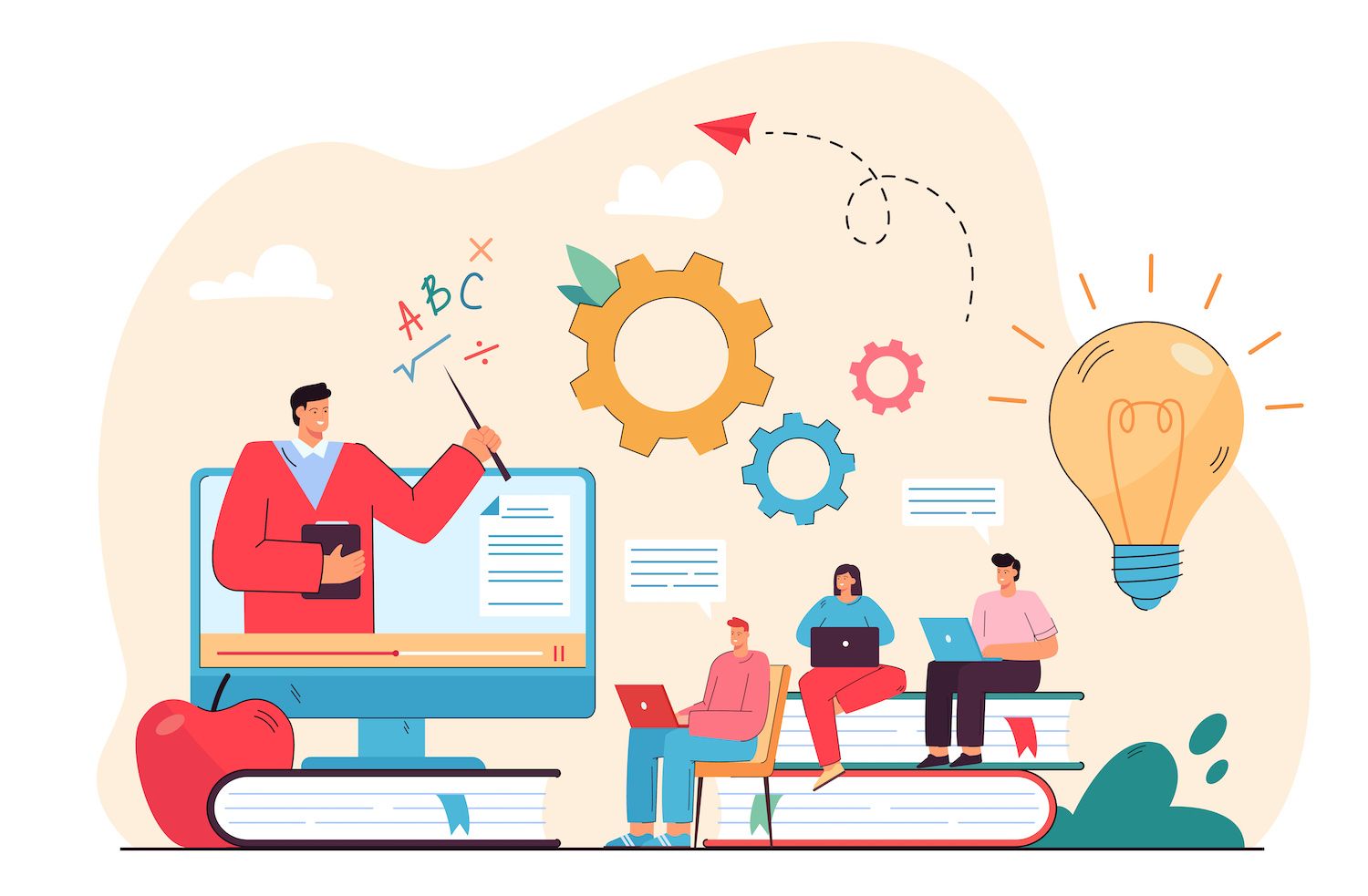
Share on
Here's a live demo of the site builder application.
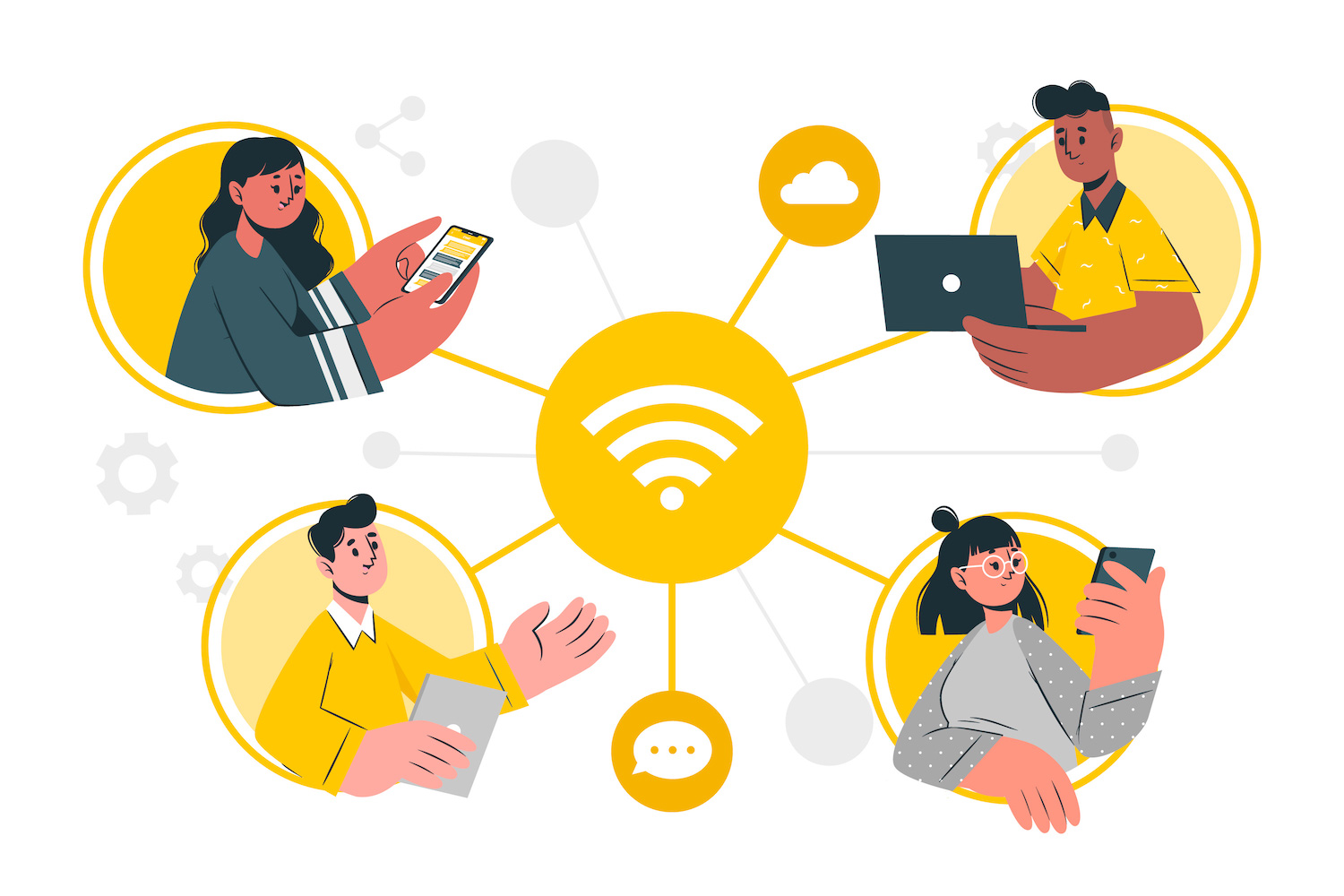
Learning the API
To generate an API key
- Log into your My Dashboard.
- Visit your API Keys page ( Your name > Corporate settings >> API Keys).
- Click to to Create API Key.
- Select an expiration date or choose an individual start date and number of hours for the key to run out.
- Make sure the key has a distinct name.
- Click Generate.
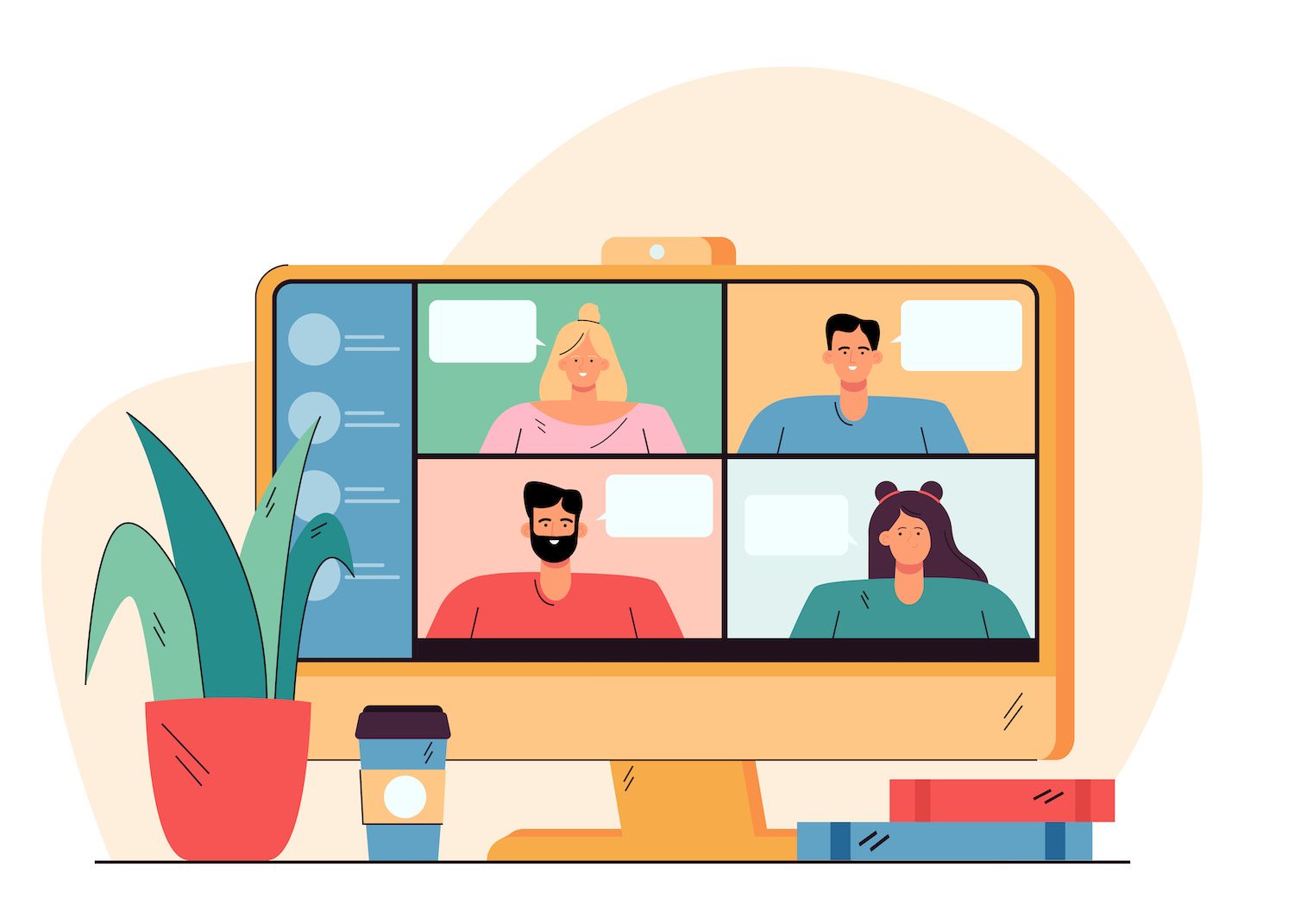
Making a WordPress Website Using the API
After you have you've got your API key is in place, let's create a WordPress site with the API. To do this, we'll make use of the /sites
endpoint. It is expecting a payload with the following information:
- firm: This parameter expects an unique ID for the company that can be found in the My settings. It allows you to determine the name of the company associated with the WordPress website.
- install_mode: This parameter determines the kind of WordPress installation. Its default value will be "plain," which sets the standard WordPress website. There are other options, including "new" for a brand new installation and additional modes depending on the specific needs.
- is_subdomain_multisite: This boolean (true/false) parameter specifies whether the WordPress site should be configured as a multisite using subdomains.
- admin_email This parameter is based on an email address from the WordPress administrator. It is used to manage administrative tasks and also receive important messages.
- admin_password: This parameter can be used to create the password for the WordPress administrator user account. Choose a secure password to secure your website.
- admin_user This parameter sets the username used for the WordPress administrator account. It is used to log in to the WordPress dashboard and to manage the site.
- is_multisite: Similar to
is_subdomain_multisite
, this boolean parameter determines whether the WordPress site should be configured as a multisite. - site_title: This parameter is used to determine the name of your WordPress website. It is displayed at the top of every page on the website. You can always change it later.
- woocommerce: This boolean parameter indicates whether you want to set up the WooCommerce plug-in when you are creating your WordPress site creation.
- wp_language This parameter is a string value that represents the language/locale of your WordPress site (discover the locale of your WordPress locale here).
Once you have a better understanding of each parameter. Here is an example of what the payload you send to the API API will look like:
"company": "54fb80af-576c-4fdc-ba4f-b596c83f15a1",
"display_name": "First site",
"region": "us-central1",
"install_mode": "new",
"is_subdomain_multisite": false,
"admin_email": "[email protected]",
"admin_password": "vJnFnN-~v)PxF[6k",
"admin_user": "admin",
"is_multisite": false,
"site_title": "My First Site",
"woocommerce": false,
"wordpressseo": false,
"wp_language": "en_US"
const createWPSite = async () =>
const resp = await fetch(
`https://api..com/v2/sites`,
method: 'POST',
headers:
'Content-Type': 'application/json',
Authorization: 'Bearer '
,
body: JSON.stringify(
company: '54fb80af-576c-4fdc-ba4f-b596c83f15a1',
display_name: 'First site',
region: 'us-central1',
install_mode: 'new',
is_subdomain_multisite: false,
admin_email: '[email protected]',
admin_password: 'vJnFnN-~v)PxF[6k',
admin_user: 'admin',
is_multisite: false,
site_title: 'My First Site',
woocommerce: false,
wordpressseo: false,
wp_language: 'en_US'
)
);
const data = await resp.json();
console.log(data);
This code makes use of using the JavaScript Fetch API to send a POST request to the API for creating an WordPress site. Its CreateWPSite
function manages the operation. Within the function, a Fetch request is sent to the API's site
endpoint, containing the required details.
The response is parsed as JSON with the help of resp.json()
which is logged to the console. Make sure you substitute your YOUR_KEY_HERE>
by your API key, adjust the payload values as needed, then use CreateWPSite()
to create a WordPress website using the API.
This is what the response looks like:
"operation_id": "sites:add-54fb80af-576c-4fdc-ba4f-b596c83f15a1",
"message": "Adding site in progress",
"status": 202
Monitoring Operations using the API
Once you initiate a site design using the API it is essential to monitor the progress of your operation. It's possible to do this in a programmatic manner without needing to verify My by using an API's /operations
endpoint.
For monitoring operations, you can use to monitor operations, use the operation_id
that you get when starting an operation such as making the WordPress website. Send the operations_id
as a parameter the operation's
endpoint.
const operationId = 'YOUR_operation_id_PARAMETER';
const resp = await fetch(
`https://api..com/v2/operations/$operationId`,
method: 'GET',
headers:
Authorization: 'Bearer '
);
const data = await resp.json();
console.log(data);
If the data response is received through the API It is then logged to the console. The data provided by the response is valuable about the status and progress of the operation. If, for example, the WordPress site creation is still taking place, the reply appears like this:
"status": 202,
"message": "Operation in progress",
"data": null
Similar to the way, after the operation is completed successfully, this is the response:
"message": "Successfully finished request",
"status": 200,
"data": null
Now it is possible to create an WordPress site, and then check its performance through the API. For further enhancements we can go higher and create a custom User Interface (UI) capable of handling these operations. This way, even individuals who aren't technical experts can take advantage of the API's capabilities.
Building a React Application To Make a WordPress Website Using API
Prerequisite
Getting Started
For a simpler project set-up process, a starter project is available for your use. Follow these steps to get going:
2. Download the repository onto your computer locally and then switch it to the starting-files branch using the following command:
Git checkout starter-files
3. Install the necessary dependencies using the command to install npm
. After the installation has been completed, you can launch the project locally on your PC using npm run begin
. This opens the project at http://localhost:3000/.
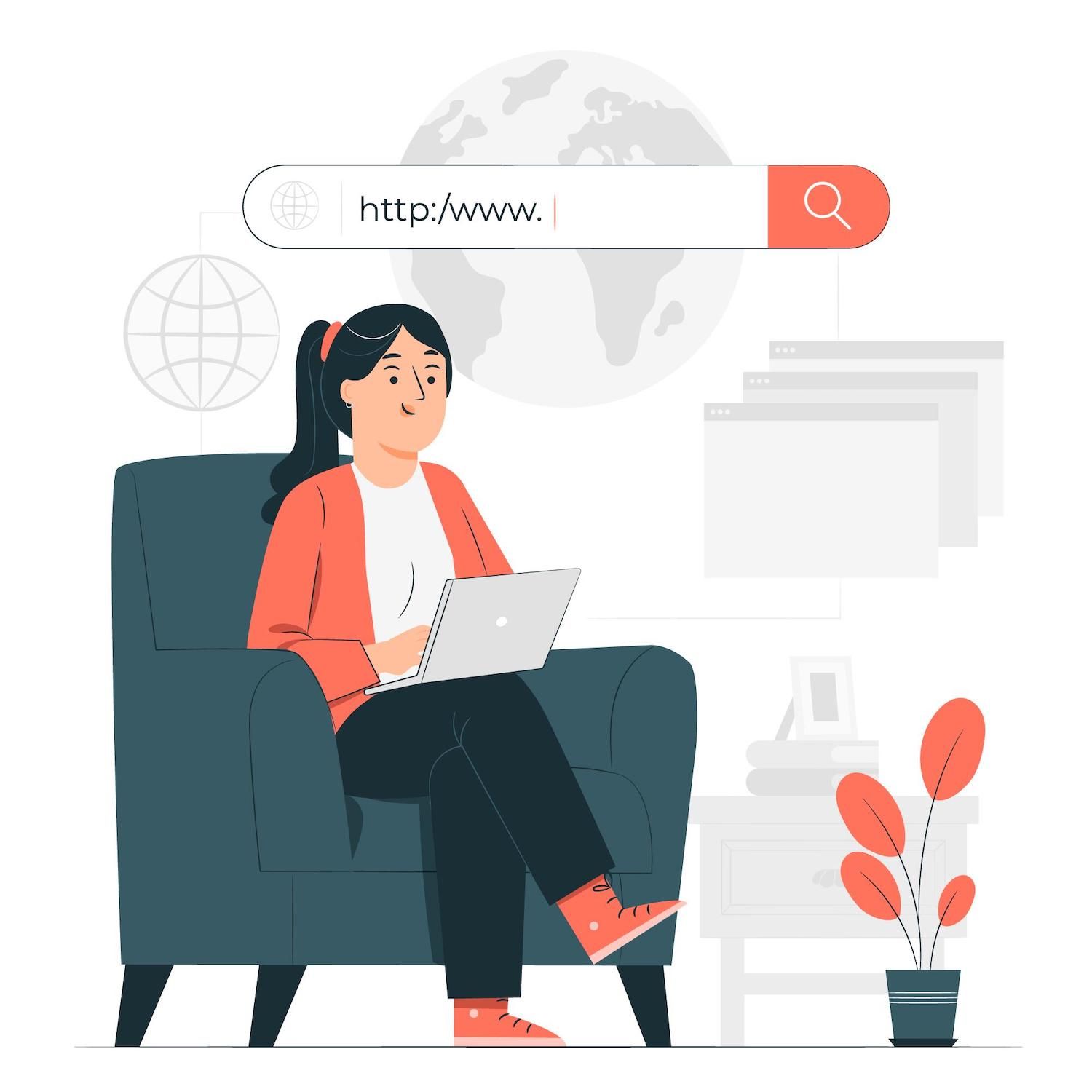
Understanding the Project Files
Within within the the src directory of this project are two main subfolders: components as well as pages. The folder for components contains reused components, such as pages, such as the header and footer that are used on both the Homepage as well as the Details pages.
In this project, your primary focus is implementing the logic on the home page and the Details pages since the style and routing is already implemented.
The Home page has a form that gathers data from various fields which can be passed on onto the API. The response from the page is saved in the localStorage (you can explore alternative ways to store the operation ID, which is crucial for checking the operation status).
In the Detail page, the operation ID is pulled from loaclStoage, and then passed through the API's /operation
endpoint as a parameter for checking the status that the process is in. In this application, we provide a button to allow users to check the status on a regular basis (you can utilize the setInterval
method to automate this procedure).
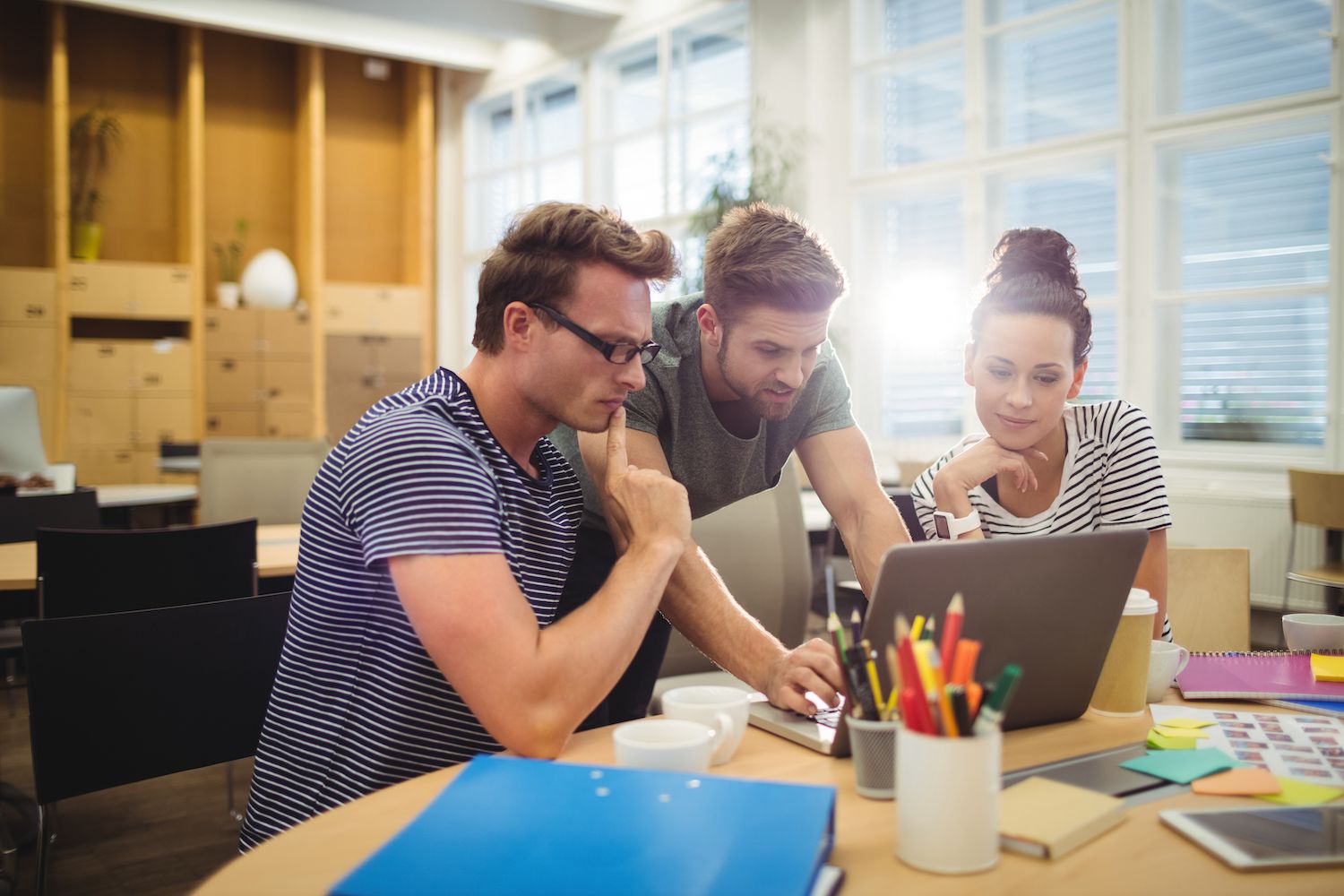
Interacting With the API in React
The user interface (UI) now in place now, the next step is to handle the form submission through the homepage and then send your POST request through the API's http://sites
endpoint. This request contains the form data as the payload, enabling the creation of a WordPress website.
For interaction with the API, you'll need the business ID and API key. After you've obtained the required credentials, you should try to store them securely as environment variables in your React application.
In order to set your environment variables create the .env file within the root directory of your project. Inside this file, add the following line:
REACT_APP__COMPANY_ID = 'YOUR_COMPANY_ID'
REACT_APP__API_KEY = 'YOUR_API_KEY'
To access these environment variables within your project, you can use the syntax process.env.THE_VARIABLE
. For example, to access the REACT_APP__COMPANY_ID
, you would use process.env.REACT_APP__COMPANY_ID
.
Retrieving Form Data using React
Within Home.jsx Home.jsx file, there's an application form. It is necessary to add an algorithm to your form in order to collect data and verify it when submitted. For retrieving the data from your form within the React application, you can use the controlled component approach and the hook. This method lets you create a state for each form field and update it as users input data.
Then, you must import the useState
hook that is located at the top of your file.
import React and useState from "react'
Create an instance of a state variable per form field within your functional component. As an example, if for instance you've got an input field that is for "Site title" You can set up the state variable siteTitle
:
const [siteTitle ] and setSiteTitle] ="useState" ('');
In this case, the siteTitle
will be the value of a state variable which holds what's in"Site title" input field "Site title" input field. setSiteTitle
is the state updater function.
For the purpose of binding form fields with their appropriate state values Add to the values
in addition to the onChange
attributes to every input element. For example, in"Site title" input field "Site title" input field:
setSiteTitle(event.target.value)
/>
In this instance in this example, the values
attribute is set to the websiteTitle
state variable. This ensures that the input field shows the current value of title
. OnChange is the change
event handler is set for use the setSiteTitle
function. This update on the siteTitle
status to reflect the latest value whenever the user types in the input field.
By following this approach for each form field, you can create the necessary state variables and modify these as users interact through the form. This allows you to easily get the data entered once the form has been submitted, and perform further operations or verify the data on the form.
If you follow this procedure for each of the fields on your form Your Home.jsx file looks as follows:
import Header from '../components/Header';
import Footer from '../components/Footer';
const Home = () =>
return (
className="title">Site Builder with API
className="description">
This is a React app that uses the API to create WordPress sites, without needing to access My dashboard. The form is
Form-container
input-divdisplay the name
helps you identify your site. Only used in My and temporary domain
className='error-message'>Ensure this has more than 4 characters
WordPress site title
Appears across the top of every page of the site. You can always change it later.
className='error-message'>Ensure this has more than 4 characters
WordPress admin username
WordPress admin email
className='error-message'>Ensure this is a valid email
WordPress admin password
Ensure you remember this password as it is what you'll use to log into WP-admin
Data center location
Allows you to place your website in a geographical location closest to your visitors.
value="">
/* add options */
Install WooCommerce
Install Yoast SEO
className='btn'>Create Site
)
export default Home;
Implementing Form Field Validation Using useRef Hook
To enable validation of form fields in React it is possible to take these steps. Focus on creating validation of fields like the "Display name" and "WordPress admin email" fields.
In the beginning, we must make references with the hook to control the display of warning messages. Import useRef hook into the UseRef hook and generate references for each field:
import useRef' from'react';displayNameRef const = useRef(null) Const wpEmailRef = useRef(null);
After that, you attach these refs to element of error messages in the form fields. For instance, for"Display Name" for example, in the "Display Name" field, you add this ref to the span tag. It is where you will find the error message
Name of display
Helps identify your site. Only used in My and temporary domain
setDisplayName(e.target.value) />
Ensure this has more than 4 characters
The same applies to the "WordPress admin email" field:
WordPress admin email
setWpAdminEmail(e.target.value)
/>
className='error-message' ref=wpEmailRef>Ensure this is a valid email
Now, we can design validation methods which check input values and decide whether to display the error messages. These are the functions that can be used to use for "Display name" and "WordPress admin email":
const checkDisplayName = () =>
if (displayName.length
let regex = /^\w+([.-]?\w+)*@\w+([.-]?\w+)*(\.\w2,3)+$/;
if (!wpAdminEmail.match(regex))
wpEmailRef.current.style.display = 'block';
else
wpEmailRef.current.style.display = 'none';
The functions are invoked at the time that the relevant input fields change. They compare the input values with the validation requirements and update the display of the error message by manipulating the style.display property of the error message elements.
Site builder form that is validated.
Feel free to implement additional validations or customize the validation logic as per your requirements.
Handling Form Submission in React
If we want to manage the form submission event for creating the site, we will need to perform several tasks. First, we attach the onSubmit event handler to the form element. Inside the createSite function, we prevent the default form submission behavior by calling event.preventDefault(). This allows us to manage the form submission process programmatically.
To ensure the form data is accurate prior to submitting the submission process, we call the validation techniques CheckDisplayName along with checkWpAdminEmail. These techniques verify that the fields are in line with the specified criteria.
const createSite = (e) =>
e.preventDefault();
checkDisplayName();
checkWpAdminEmail();
// Additional logic
;
In the event that all validations have been completed and that the fields required contain valid data, proceed with clearing localStorage to make sure that there is an uncluttered state for the storage of the API response as well as the display name.
Then, you can make an API request for the API by using the fetch function. The request is a POST method to the https://api..com/v2/sites endpoint. Be sure to include all the relevant headers and payload as JSON.
const createSiteWithAPI = async () =>
const resp = await fetch(
`$APIUrl/sites`,
method: 'POST',
headers:
'Content-Type': 'application/json',
Authorization: `Bearer $process.env.REACT_APP__API_KEY`
,
body: JSON.stringify(
company: `$process.env.REACT_APP__COMPANY_ID`,
display_name: displayName,
region: centerLocation,
install_mode: 'new',
is_subdomain_multisite: false,
admin_email: wpAdminEmail,
admin_password: wpAdminPassword,
admin_user: wpAdminUsername,
is_multisite: false,
site_title: siteTitle,
woocommerce: false,
wordpressseo: false,
wp_language: 'en_US'
)
);
// Handle the response data
;
The payload is comprised of various information fields that the API requires, such as your company's ID, display name, region configuration mode, admin email, admin password etc. The values come from the corresponding state variables.
After making the API request we await the response using await resp.json() and take the data relevant to our request. We create a new object newData, with the operation ID and display name, which is then stored in the localStorage using localStorage.setItem.
const createSiteWithAPI = async () =>
const resp = await fetch(
// Fetch request here
);
const data = await resp.json();
let newData = operationId: data.operation_id, display_name: displayName ;
localStorage.setItem('state', JSON.stringify(newData));
navigate('/details');
In the end, we use the createSiteWithAPI function to ensure that, when users fill out the form and clicks the button to create a WordPress website is built with the help of API. Additionally, in the code, it's stated that the user is redirect to the details.jsx page to keep track of the operation by using the API. To enable the navigation functionality to be used, you need to install UseNavigate into react-router.dom and then initialize it.
Reminder: You can find the entire source code of this page in the GitHub repository.
Implementing Operation Status Check with the API
In order to check the status of the operation with the API, we use the operation ID that was saved within the localStorage. This operation ID is retrieved from the localStorage using JSON.parse(localStorage.getItem('state')) and assigned to a variable.
To check the operation status, make another API request to the API by sending a GET request to the /operations/operationId endpoint. This request includes the necessary headers such as the Authorization header, which contains an API Key.
const [operationData, setOperationData] = useState( message: "Operation in progress" );
const APIUrl = 'https://api..com/v2';
const stateData = JSON.parse(localStorage.getItem('state'));
const checkOperation = async () =>
const operationId = stateData.operationId;
const resp = await fetch(
`$APIUrl/operations/$operationId`,
method: 'GET',
headers:
Authorization: `Bearer $process.env.REACT_APP__API_KEY`
);
const data = await resp.json();
setOperationData(data);
Once we receive an answer, we extract all relevant information from the response by using await resp.json(). The operation data is then updated in the state using setOperationData(data).
In the return statement of the component, we display the operation message using operationData.message. We also provide a button that allows the user to set up the check of status for operation using the operation check.
Furthermore, if the operational status is that it has successfully finished then the user is able to use the hyperlinks to get access to the WordPress administrator and site itself. The links are constructed using the stateData.display_name obtained from localStorage.
Open WordPress admin
Open WordPress admin Open URL Opening URL
Open WordPress admin Open URL
Clicking on these links opens the WordPress admin and the site URL, respectively, in a brand new tab. This allows users to get them directly without having go to My.
Now you can build a WordPress website with ease using an application that you've designed for you.
How To Deploy Your React-Based Application
To make your repository available to , comply with these steps:
Create or log in to an account using the My dashboard.
On the left sidebar On the right sidebar, click "Applications" then click "Add service".
Select "Application" from the dropdown menu to deploy the React application to .
The modal window that pops up select the repository that you wish to set up. If you've got several branches, you can select the branch you want to deploy and assign the application a name.
Choose one of the Data center locations from the available 25 possibilities. The system automatically recognizes the starting command of your application.
Configure environment variables on My before deploying.
After you have initiated the installation of your application then the deployment process begins and usually completes in a few minutes. A successful deployment generates a link to your application, like https://site-builder-ndg9i..app/.
Summary
In this tutorial, you have learned how to use the API to build a WordPress website and then integrate the API into a React application. Always remember to ensure that you keep your API keys secure. If you feel you have shared it publicly, revoke the key and make a fresh one.
What are the endpoints you would like to see included in the API in the near future? And what would you love to see us build with the API? Tell us via the comments.