How to Create and Implement the Developer Portfolio with Next.js - (r)
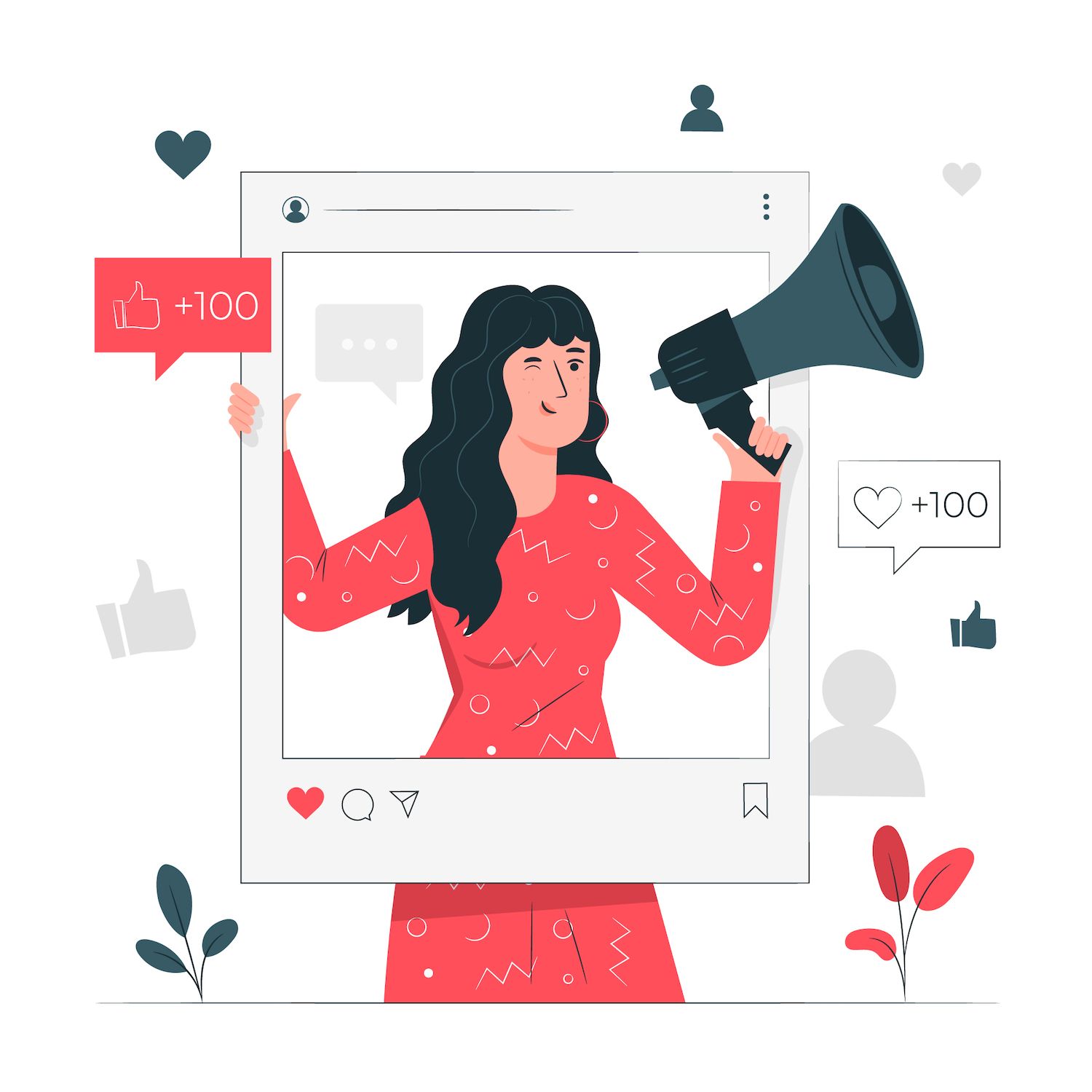
A developer's portfolio is an accumulation of examples of work and projects showcasing your skills and knowledge. An impressive portfolio can set you apart from other candidates in the search for jobs. It's not just that. A portfolio can also be beneficial for networking as well as making sure you are keeping track of your education as well as establishing yourself as an expert on the subject.
A live example of the developer portfolio that you'll build using Next.js. The portfolio is accessible on the project's GitHub repository for more information. take a closer look or fork it Next.js portfolio beginning project I designed. The portfolio starter contains the simple codes, such as the styles and the Font Awesome CDN link, pictures, and the basic structure.
Requirements/Prerequisites
This is a "follow along" kind of tutorial. It is easier for you to follow along with code if you've got:
- A basic understanding of React and perhaps Next.js
Why Next.js?
- Building static web pages: Next.js can build static sites that are speedy, easy to deploy, and need minimal maintenance like the developer portfolio website that we'll build throughout this tutorial.
- Designing web pages that are dynamic: Next.js allows you to develop dynamic websites which modify content according to user interactions or server-side data downloading.
- Enhancing the performance of websites Utilizing rendering on the server side, Next.js can improve website performance by decreasing the amount of time it takes for a page to load.
- The building of an e-commerce sites: Next.js is well-suited to build e-commerce sites that need server-side rendering to ensure improved SEO and performance.
- Building progressive web applications (PWAs): Next.js supports the creation of PWAs that are web apps that work as native apps that can be installed on a user's device.
How To Set Up Your Next.js Development Environment
npx [email protected] my-portfolio
A prompt will appear asking for confirmation of additional dependencies. You can then start npm run dev
to make your app available at localhost:3000.
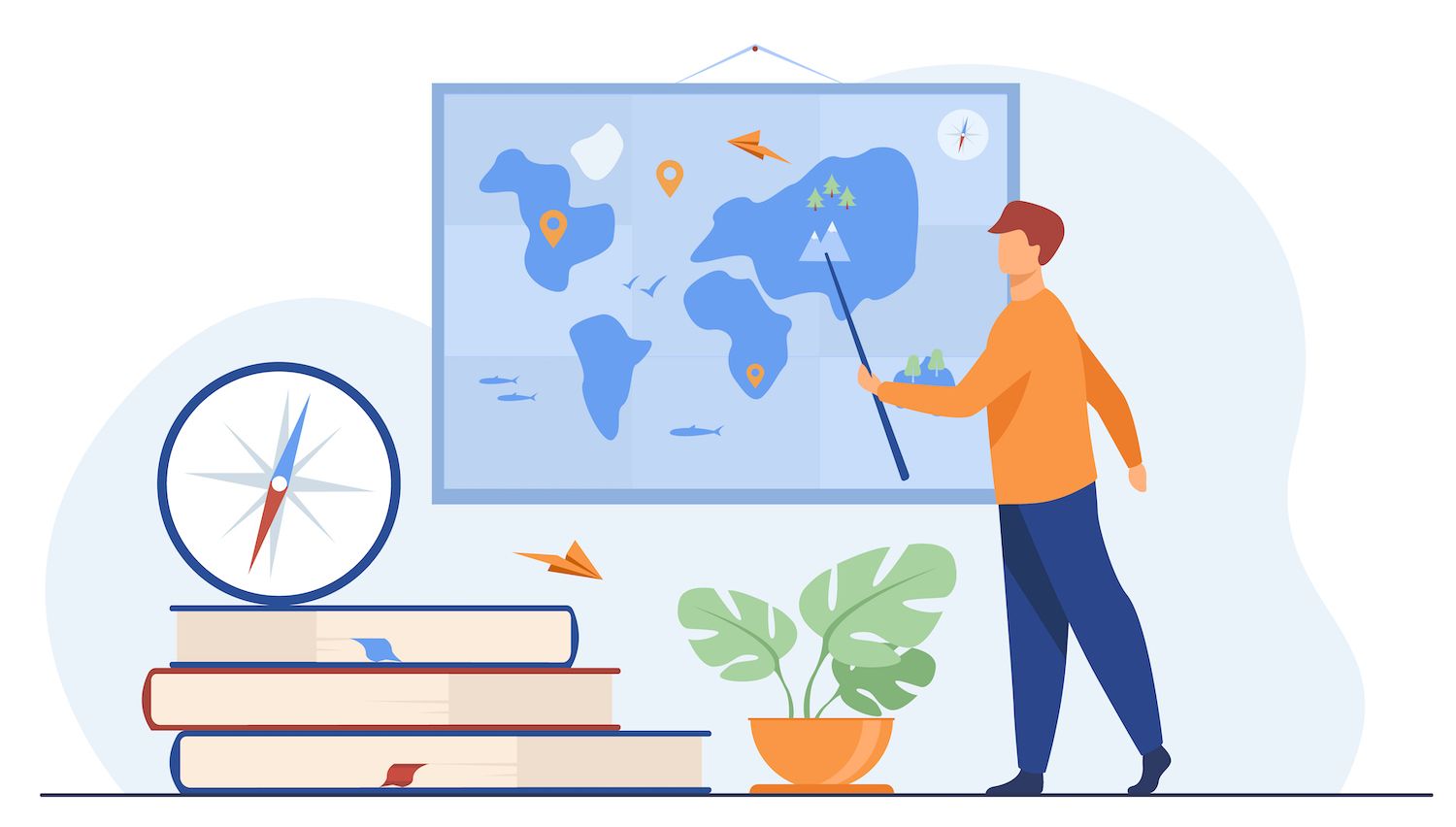
When creating the Next.js project with the npx
command that automatically creates one's folder structure by using the following main directories:
- pages The folder in question contains the application's pages, which are routed automatically based on their file names. For example, pages/index.js would be the homepage page, in contrast, pages/about.js would be the page about.
- public The folder is comprised of static files that can be downloaded directly including fonts, images, and other assets.
- components: This folder is optional and contains the reusable UI components that may be used across the application.
- styles The folder styles can also be used for optional styles and has global styles that can be utilized across applications.
Other directories and files may also be generated depending on the specific configuration and feature, but they are the main directories in the base Next.js project.
In this tutorial, all the components that we build will appear at the top of the page (our single-page site), and you will find components for different sections , including the hero information, project, as well as others.
How To Build A Responsive Developer Portfolio using Next.js
A typical portfolio consists of these components:
- Navbar component
- Hero component
- About component
- Skills component
- The Projects component
- Contact component
- Footer component
It is expected that the Navbar and Footer components should be displayed on every page if the portfolio has multiple pages. It can be accomplished in Next.js by defining an layout.
Definition of Layouts using Next.js
In Next.js Layouts are an approach to create a consistent structure for the elements that are displayed on each web page. The layout typically contains elements such as a header along with a navigation menu and a the footer that is displayed on all pages.
Begin by creating a component folder within the Src (source) directory within your Next.js project. Then, you need to create your Navbar Footer and Footer components to use in the Layout module.
This is the Navbar component of Navbar.jsx:
// components/Navbar.jsx
import Link from "next/link";
const Navbar = () =>
return (
Joe's Portfolio
Resume
)
export default Navbar;
The Footer component is in Footer.jsx:
// components/Footer.jsx
const Footer = () =>
return (
(c) new Date().getFullYear() Joel's Portfolio
>
)
export default Footer;
Notice: For the Font Awesome icons to work you need to either install Font Awesome in your project or make use of its CDN. It is possible to add the CDN link to your _document.js file like this:
// pages/_document.js
import Html, Main, NextScript, and Head in 'next/document'
document export default function()
return (
);
NOTE: If you link in a different version of Font Awesome using the CDN You will have change it above the correct integrity
hash of the release.
Once you have created all the required elements for your layout you are able to create the Layout component itself and add this component to your pages by wrapping your pages' content inside it.
The Layout component will accept a >code>children prop, allowing you to access the content that you want to display on Your Next.js pages.
// components/Layout.jsx
import Navbar from './navbar';
import Footer from './footer';
const Layout = ( children ) =>
return (
children
>
)
export default Layout;
You have created the Layout component that holds the Navbar and the Footer along with the children props positioned properly. Now, you can incorporate the Layout component into your pages by wrapping contents of the page in it. This will be done in the _app.js file.
// pages/_app.js
import '@/styles/globals.css';
import Layout from '../components/layout';
export default function App( Component, pageProps )
return (
);
You have now succeeded in designing a layout for your portfolio of developers. In this portfolio, we focus in more detail on Next.js and how to deploy your website . You can then take the style styles from your styles/globals.css file into your project. If you start your portfolio's website using dev mode, you will observe the layout for your app.
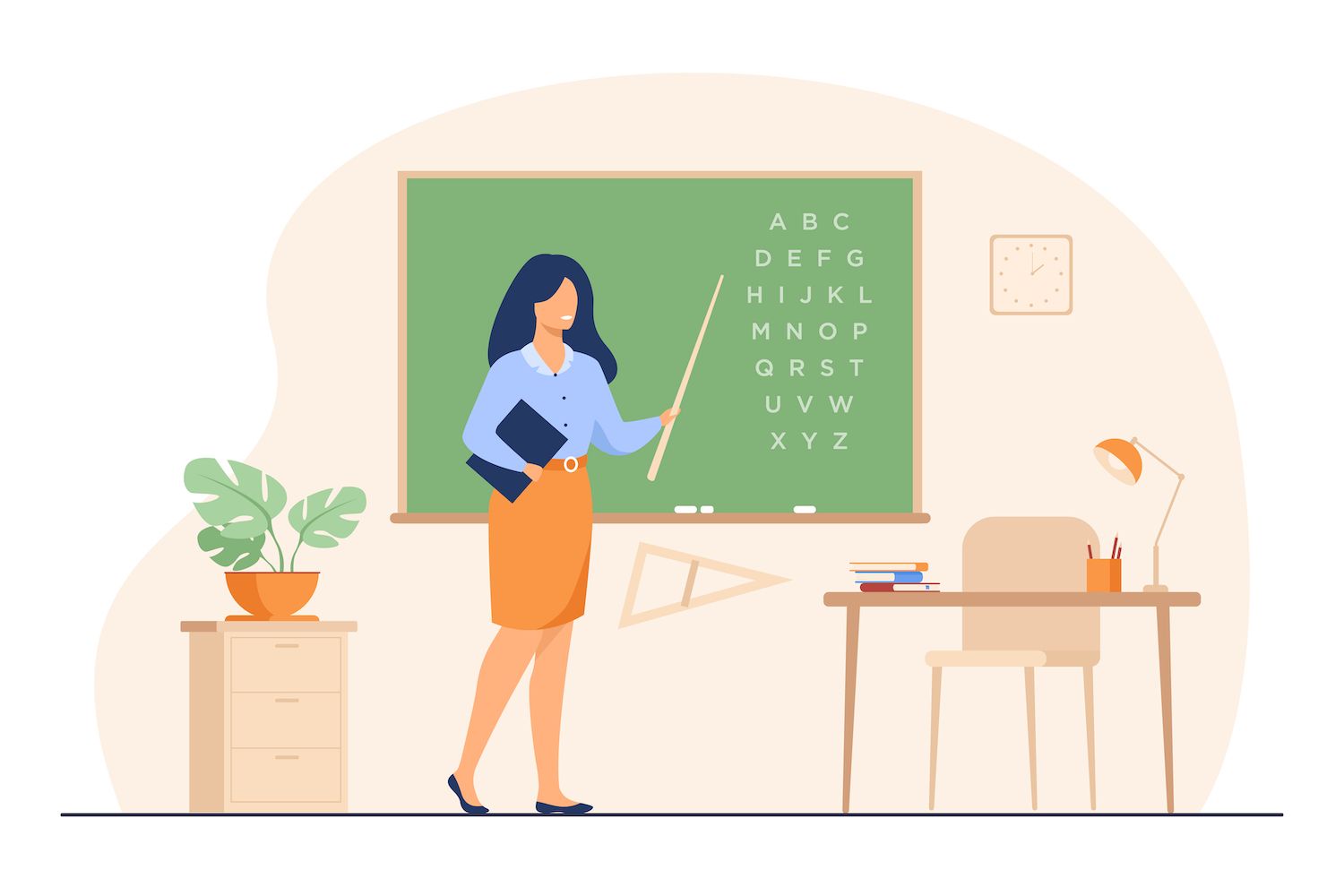
It's now time to provide your portfolio site the proper content.
Building Portfolio Components
It is now possible to create separate components for every section of your portfolio as a developer. These components will all be imported into the index page for your Next.js project, so they are displayed whenever you launch your project with NPM run dev
.
The Hero Component
The Hero component is the initial portion just below the Navbar The primary purpose is to draw the user's attention and give them a sense of what the application or website is about.
/components/Hero.jsx components/Hero.jsx
import Image from "next/image" //const Hero = () •Export default Hero
In the code above, you will notice that you will notice that the Next.js image component is utilized instead of using the HTML img
tag. This is to include images since it allows automated image optimization, resizing as well as many other.
In the About section, you'll also notice that a simple sentence to introduce the developer was added alongside some social icons from Font Awesome to add social links.
This is how should be the Hero component must look to look like:
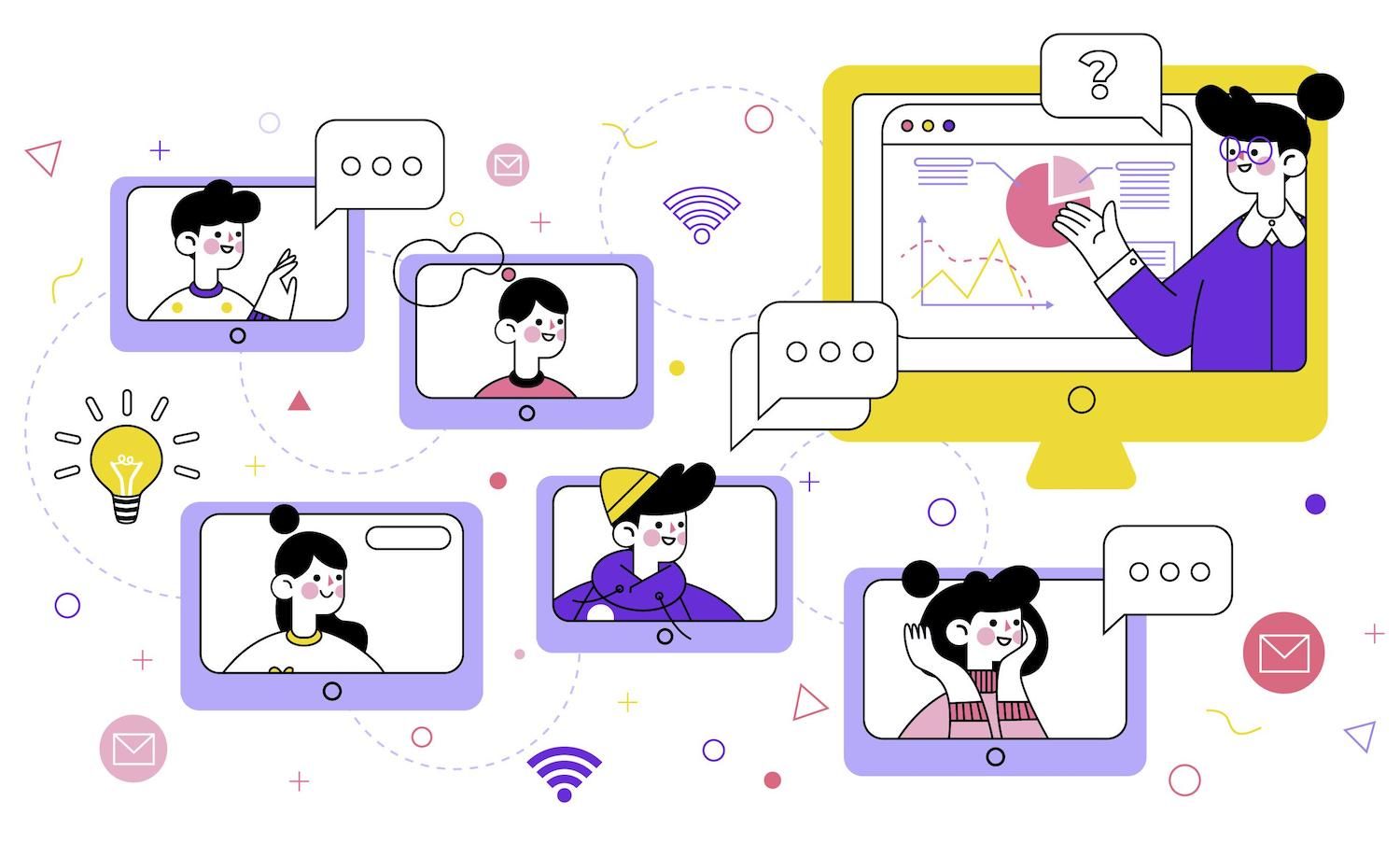
It is possible to add additional content in the Hero component, tweak the stylings in the styles/globals.css file or perhaps recreate the section to your liking.
The About Component
The About part is intended to provide readers or those who visit your portfolio details about yourself in as many paragraphs as you'd like. If you wish to tell your story then you could create a dedicated "About Me" page. Add an option on the page for more information about you.
// components/About.jsx
import Image from "next/image" •const About = () => =
return (
return (
About me
About Me"flex-about""p> an engineer, I've always had a passion for developing elegant and efficient solutions to
difficult problems. I'm well-versed in the field of software development with an emphasis on webtechnologies like HTML, CSS, and JavaScript. I am a fan of working on the front-end and
back-end of the applications. I am always looking for ways to optimize efficiency, improve the userexperience and guarantee the highest level of code quality.
Throughout my career, I've been involved in a variety of projects, ranging from static websites, to morecomplex enterprise-level applications. I'm proficient in working with a variety of tools for development and frameworks such as React, Angular, Vue.js, Node.js, and Laravel. I am always eagerto learn and explore new technologies, and I am constantly seeking out opportunities to increasemy abilities as well as my knowledge.
> (/div>
•)
export default About
The code below includes two paragraphs of text on the developer, as well as an image of the developer. This is what the About section will appear like:
About component
You are able to tweak the styles to add pictures and more.
The Skills Component
The skills component is meant to highlight how the developer has the most popular technologies, or techniques that the developer used in the past.
Skills component
You can make this simpler to manage with an array created on an external file. Then, import into the skills component. That way you'll be able to continue to loop around instead of creating duplicates of similar code.
// components/Skills.jsx
const Skills = () =>
return (
Skills
HTML
CSS
JavaScript
React
Node
Python
)
export default Skills;
In the code above it is possible to create cards to represent each skill. each card will hold the font-awesome technology icon as well as the name of the technology. Additionally, you can add other styles or tweak the code to make it more attractive and distinct.
The Projects Component
The project component is one essential elements in a developer's portfolio. The projects are tangible proof of a developer's skills and capabilities and demonstrate their capacity to put their expertise into real-world problems.
Projects component
It is possible to create arrays to store each project's details and then add it to your component to avoid hard-coding these details.
We will create a data.js file to keep the project's array of data. It can be stored in the components folder or the pages/api folder. In this demonstration, I will store it in the component folder. The array will contain the project's object The object holds the name of the project as well as the description and GitHub hyperlink.
/• components/data.js
export const projectData = [
id: 1,
title: 'Todo List App',
description: A simple Todo List app built using JavaScript. All information is saved locally in local storage. This app helps users to check of their goals and mark them as they do them. ',
gitHubLink: 'https://github.com/olawanlejoel/Todo-List-App',
,
id: 2,
title: 'Books Library App',
description:
'A simple Book Library App built with JavaScript. The app allows users to keep excellent lists of books that they are in the process of reading or are currently reading. ',
gitHubLink: 'https://github.com/olawanlejoel/Book-Library',
,
id: 3,
title: 'Quotes Generator',
description:
'Helps you generate quotes from about 1600 quotes written by different authors . Quotes automatically copy into your clipboards. ',
gitHubLink: 'https://github.com/olawanlejoel/random-quote-generator',
,
id: 4,
title: 'Password Generator',
description:
'Helps you generates random passwords, you can select what you want your password to entail and also you can copy generated password to clipboard. ',
gitHubLink: 'https://github.com/olawanlejoel/Password-Generator',
,
id: 5,
title: 'Twitter UI Clone',
description:
'Simple Twitter UI clone built with TailwindCSS and Vue Js. It only covers the homepage of Twitter UI. This is a fantastic way to begin using TailwindCSS since it makes it easier to grasp basic concepts. ',
gitHubLink: 'https://github.com/olawanlejoel/TwitterUI-clone',
,
];
It is now possible to create a project component to make use of this data, using a looping. You can use any JavaScript iteration method, but in this case, you can make use of the JavaScript map() array method to browse through the data array after importing it to The Projects component.
// components/Projects.jsx
import projectData from './data.js';
const Projects = () =>
return (
Projects
projectData && projectData.map((project) => (
project.title
project.description
))
)
export default Projects;
In the example code above you've kept from repeating yourself by looping the array and outputting each project into the Projects component making it easy to manage and create more projects.
The Contact Component
Another reason for creating a developer's portfolio is so potential clients can get in touch with them. One way would be for individuals to reach out to you by email, which is what we'll facilitate in this Contact element.
// components/Contact.jsx
const Contact = () =>
return (
Get In Touch
If you want us to work together, have any questions or want me to speak at your event, my inbox is always open. Whether I just want to chat with you and say hello, I'll do my best to get back to you! Cheers!
Say Hello
)
export default Contact;
Put your email address inside the a tag, so that it launches an email application that will send a message to you.
Contact component
You have now successfully created each of the elements for your portfolio application. The next thing to do is to include them on the index page. Browse to the pages/index.js file -that is the one created as default -- then then replace its code with the following.
// pages/index.js
import Hero from '@/components/Hero';
import About from '@/components/About';
import Skills from '@/components/Skills';
import Projects from '@/components/Projects';
import Contact from '@/components/Contact';
import Head from 'next/head';
const Home = () =>
return (
Joel's Portfolio
>
);
;
export default Home;
Image optimization is performed using the Next.js Image component. Prior to deploying an app into production, that makes use of the Next.js Image component it is recommended that you set up Sharp. It is done via your terminal, ensuring you're in the project's directoryand using the following command:
npm i sharp
It is now possible to install your app, and your images will function well with the maximum optimization provided by Next.js gives you.
How to deploy the Next.js Application
Once you're happy with your portfolio showcasing your most impressive work in development as well as important information, you'll likely want to share it to others. Let's explore ways to accomplish it with GitHub and the Application Hosting platform.
You can push your Code to GitHub
Upload your code to GitHub using the following steps:
Create a new repository (just as a local directory to save your files). This can be done by signing in to your GitHub account, then clicking the + button located in the upper right corner of the screen and then choosing the option to create a new repository from the dropdown menu. This is shown in the image below.
Make a brand fresh GitHub repository.
The next step is to provide your repository with a name, add a description (optional), and choose whether you would like the repository to be open or private. Click the Create button. Then, you are able to transfer your code into the newly created GitHub repository.
All you need to push your code with Git is the repository URL that you will find on the repository's main page beneath the Clone or download button, or on the next steps following the creation of a repository.
Connect to your GitHub repository URL
The best way to do this is to make sure you are ready to publish your code by opening your console or command prompt and going to the directory which is home to your project. Use the following command to initialize your Git repository locally: Git repository:
Git init
Add your code now to the local Git repository by using the following command:
git add .
The command above adds all files in the current directory as well as its subdirectories to the new Git repository. It is now possible to make changes permanent using the following command:
Git commit"my first commit "my very first commit"
Note: You can replace "my first commit" with your own brief text describing the changes that you implemented.
Finally, push your code onto GitHub by using these commands:
Remote git Add origin URL [repository URL]
git push -u origin master
NOTE: Ensure you replace "[repository URL" by the URL of your own GitHub repository.
After you've completed these steps, your code will be pushed to GitHub and accessible through the URL for your repository. You can now move your repository's data to .
Deploy Your Portfolio to
The deployment will take just minutes. Begin by going to your My dashboard and sign in or create an account.
Select Applications to the left of the sidebar
Simply click "Add service"
In the dropdown menu, select App because you want to deploy the Next.js application to .
Create an application project in My
A pop-up window will be displayed in where you are able to select the repository you would like to publish.
Automatically detect start command
After this your application will start deploying. In just a couple of hours, a link will be sent to allow access to the deployed version of your application. In this case, it is: https://-developer-portfolio-ir8w8..app/
Deployment link on
Notice: Automatic deployment was activated, which means that it automatically deploys the application every time you make changes to your codebase . Then, it pushes it to GitHub.
Summary
There are a number of reasons developers should think about making use of Next.js for web-based projects. It is the first to provide optimized performance out-of-the-box, with features such as pre-fetching and code splitting, which help to reduce page load times. Additionally, it offers an easy development environment for React developers, supporting popular tools such as styled components and React hooks.
Next.js can also be used to implement a wide range of deployment options, ranging from traditional servers-based hosting to contemporary servers like's. This allows developers to choose the option of deployment that best matches their needs, while profiting of the framework's optimizations for performance as well as other advantages.
The next step is to add new options to your new portfolio website. A few ways to get your imagination juice flowing: add pages that contain more information, integrate a blog with MDX, implement animation.
Tell us about your work and experiences in the comments section below.
Easy setup and management in My Dashboard. My dashboard
24 hour expert assistance
The top Google Cloud Platform hardware and network, powered by Kubernetes for maximum scalability
An enterprise-level Cloudflare integration to speed up and security
The reach of the global audience is as high as more than 35 data centers as well as more than 275 PoPs across the globe