How To Design a headless WordPress Site With React.js (r) (r)
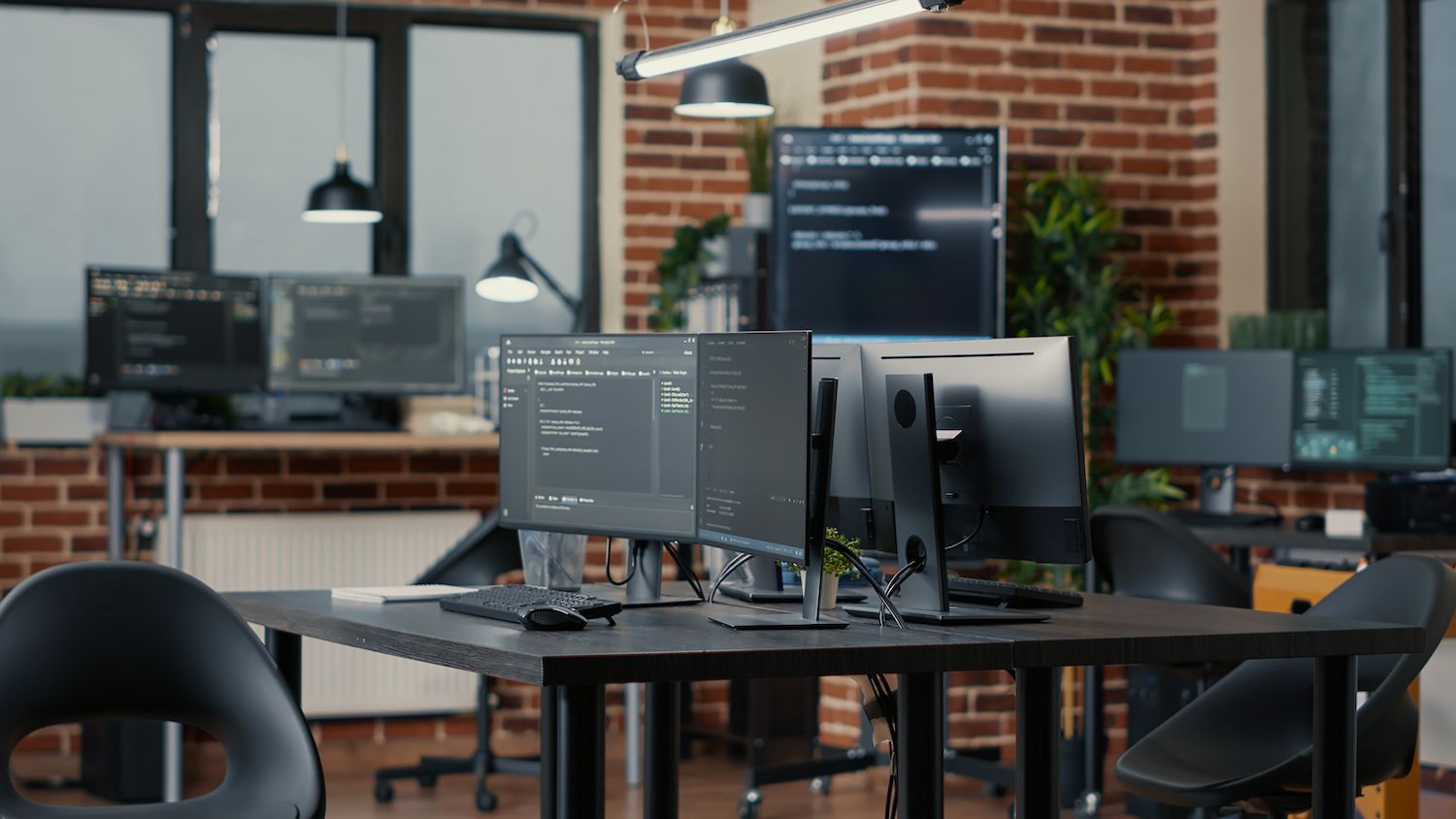
Share on
One method to get the most of WordPress is by going completely headless.
This means that the frontend must be managed separately. This is why an API comes in.
In this article we'll discuss the concept of a headless CMS is, what it does, and why you might want to build one, and the best way to set one up to work with WordPress.
What is Headless WordPress?
Simply put the term headless architecture implies that the CMS is used only for the storage and management of your information, and it doesn't care about the frontend of the website.
The frontend's main job however, is to display the contents, and in turn doesn't care what the location of content or controlled, so long as it can reach it.
The pros and cons of headless WordPress
Like all options for development that are available, there are benefits and disadvantages of using a headless WordPress solution. It's important to understand the pros and cons before making a final decision.
The advantages of Headless WordPress
A few of the main advantages of an headless WordPress implementation are as follows:
- Security: Through the separation of frontend from the backend, headless WordPress could provide an additional layer of security by limiting access to the base WordPress codebase and database.
Pros and Cons of Headless WordPress
The disadvantages of WordPress that are headless WordPress may include:
What exactly is The WordPress REST API?
The API supports many different data formats, including JSON that makes it simple to communicate with the system.
WordPress REST API can be a useful tool for developers to build, modify or erase data, as well as create custom functionality for sites or integrating with another service. Furthermore, there are plugins available that extend the functionality of the API, such as integrating additional authentication methods.
What is React?
React produces an interactive user interface and renders the component when the information changes. This library is extremely popular with developers seeking to develop complex, reusable components; effectively manage state as well as easily modify the user interface in real time.
React's active developer community has created a set of libraries, tools, as well as resources designed to improve the capabilities of the library. Many organizations and businesses have adopted React as a technology to assist in building complex, dynamic, and high-performance web applications.
Why should you use React?
React provides many benefits that make it an excellent choice to build complicated and dynamic web applications.
Here are some of the key advantages of React: React:
- Declarative syntax React employs a method of building declarative UI components. This makes it easy to design reusable and highly efficient components.
- A large community and an ecosystem: React has a large and active developer community that has resulted in the creation of a wide range of tools and libraries that enhance the functionality of React.
- Virtual DOM: React uses virtual DOM in order to modify the interface at a real-time rate. That means, any time the state changes It only updates the components required to change instead of re-displaying the entire page.
- Reusability React.js provides reusable elements that are able to be used in different applications, which significantly decreases the time spent developing and work.
How Do You create a headless WordPress Website Using React
It's now time to get our hands dirty and learn how to create and launch an unidirectional WordPress website using React.
Continue reading for more information.
Prerequisites
Before you start with this tutorial, make sure you're equipped with:
- A good grasp of React
Step 1. Setup an WordPress Website
Let's start by setting up your WordPress site, since this serves as the database source for the React application. If you already have a WordPress site then you may skip this section; otherwise you can follow the steps.
Once the installation is completed, open the Dev dashboard and select New WordPress website to create a new WordPress website.
Input the necessary information such and press the button to create the site button to continue.
It could take several minutes, but once the website has been created you will be able to access it as well as its admin panel by clicking "Open Site" and WP Admin options respectively.
To enable the JSON API, you'll need to change the permalinks on your website.
There are also tools such as Postman to easily test and submit request via WordPress REST APIs.
Step 2: Setting up a React Application
Now that we have your WordPress website up and running and running, it is time to start developing the front end. As mentioned above, in this tutorial we will utilize React to serve as the frontend of the application.
In order to begin, execute the below code in your terminal and create React. React application.
npm create [email protected] my-blog-app
cd my-blog-app
npm install
The above commands will create an React application, and then install the necessary dependencies.
It is also necessary to install Axios also, which is which is a JavaScript library to make HTTP requests. Run the below command to install it.
NPM install Axios
Now, in order to launch the server for development it is possible to run npm run dev
within the terminal. The server should then initialize your app at http://127.0.0.1:5173.
It is also possible to replace the code within main.jsx and App.jsx with the following codes:
// main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App'
ReactDOM.createRoot(document.getElementById('root')).render(
,
)
/http://www.html0.com/ App.jsx
import React using'react'export the default method App()
return (
)
Step 3: Fetching Posts From WordPress
The time has come to pull all the content from the WordPress website.
Inside of the App.jsx, add the state below and include the useState
from React:
const [posts setPosts, useState] = ([])
posts
is used to get the post's data from state. setPosts
is used to add more data to the post. Also, we have given an empty array back to state by default.
After that, insert the following code after the state in order to retrieve posts from the WordPress REST API:
const fetchPosts = () =>
// Using axios to fetch the posts
axios
.get("http://headless-wordpress-website.local/wp-json/wp/v2/posts")
.then((res) =>
// Saving the data to state
setPosts(res.data);
);
// Calling the function on page load
useEffect(() =>
fetchPosts()
, [])
The above code is running in the fetchPosts()
function on page load. In the fetchPosts()
function, we send a request for a GET
call to WordPress API via Axios to find posts. We after that save them to the state we previously declared.
Step 4: Creating the Blog Component
Inside of the root directory, make the folder components and within it, create two new documents: blog.jsx and blog.css.
First, add the following code into blog.jsx:
import axios from "axios";
import React, useEffect, useState from "react";
import "./blog.css";
export default function Blog( post )
const [featuredImage, setFeaturedimage] = useState();
const getImage = () =>
axios
.get(post?._links["wp:featuredmedia"][0]?.href)
.then((response) =>
setFeaturedimage(response.data.source_url);
);
;
useEffect(() =>
getImage();
, []);
return (
new Date(Date.now()).toLocaleDateString("en-US",
day: "numeric",
month: "long",
year: "numeric",
)
post.title.rendered
);
In the above code, we have created a card component that takes the blog post
property that contains the data about the blog's post. It is obtained taken from WordPress API. WordPress API.
In using the obtainImage()
function, we utilize Axios to get the URL of the picture that was featured, and save that information to state.
Then, we added a UseEffect
hook to call to call the obtainImage()
function once the component is been mounted. Additionally, we have added a return declaration in which we are rendering the data for the post, including title excerpt, image, and post data.
After that, apply the style below to blog.css. blog.css file:
@import url("https://fonts.googleapis.com/css?family=Poppins");
.blog-container
width: 400px;
height: 322px;
background: white;
border-radius: 10px;
box-shadow: 0px 20px 50px #d9dbdf;
-webkit-transition: all 0.3s ease;
-o-transition: all 0.3s ease;
transition: all 0.3s ease;
img
width: 400px;
height: 210px;
object-fit: cover;
overflow: hidden;
z-index: 999;
border-bottom-left-radius: 10px;
border-bottom-right-radius: 10px;
.blog-title
margin: auto;
text-align: left;
padding-left: 22px;
font-family: "Poppins";
font-size: 22px;
.blog-date
text-align: justify;
padding-left: 22px;
padding-right: 22px;
font-family: "Poppins";
font-size: 12px;
color: #c8c8c8;
line-height: 18px;
padding-top: 10px;
.blog-excerpt
text-align: justify;
padding-left: 22px;
padding-right: 22px;
font-family: "Poppins";
font-size: 12px;
color: #8a8a8a;
line-height: 18px;
margin-bottom: 13px;
Then, in your App.jsx file, you should add the follow code in the return
statement to create the blog component:
posts.map((item) => (
))
;
Then, here's what your App.jsx should appear like:
import React, useEffect, useState from 'react'
import axios from "axios"
import Blog from './components/Blog';
export default function App()
const [posts, setPosts] = useState([]);
const fetchPosts = () =>
axios
.get("http://my-awesome-website.local/wp-json/wp/v2/posts")
.then((res) =>
setPosts(res.data);
);
useEffect(() =>
fetchPosts()
, [])
return (
posts.map((item) => (
))
)
Save the file and refresh your browser tab. You should now be able to view the blog post you wrote in the webpage.
Summary
Headless WordPress provides a fantastic way to create fast-performing websites that have a flexible design. With the use of React and using the WordPress REST API, it is simpler than ever before to design engaging and dynamic websites using WordPress as the CMS system.
Have you created or are you considering building an headless WordPress site using React? Please let us know via the comment section below!