How to Generate and Utilize fake records using the Model Factories in Laravel - (r)
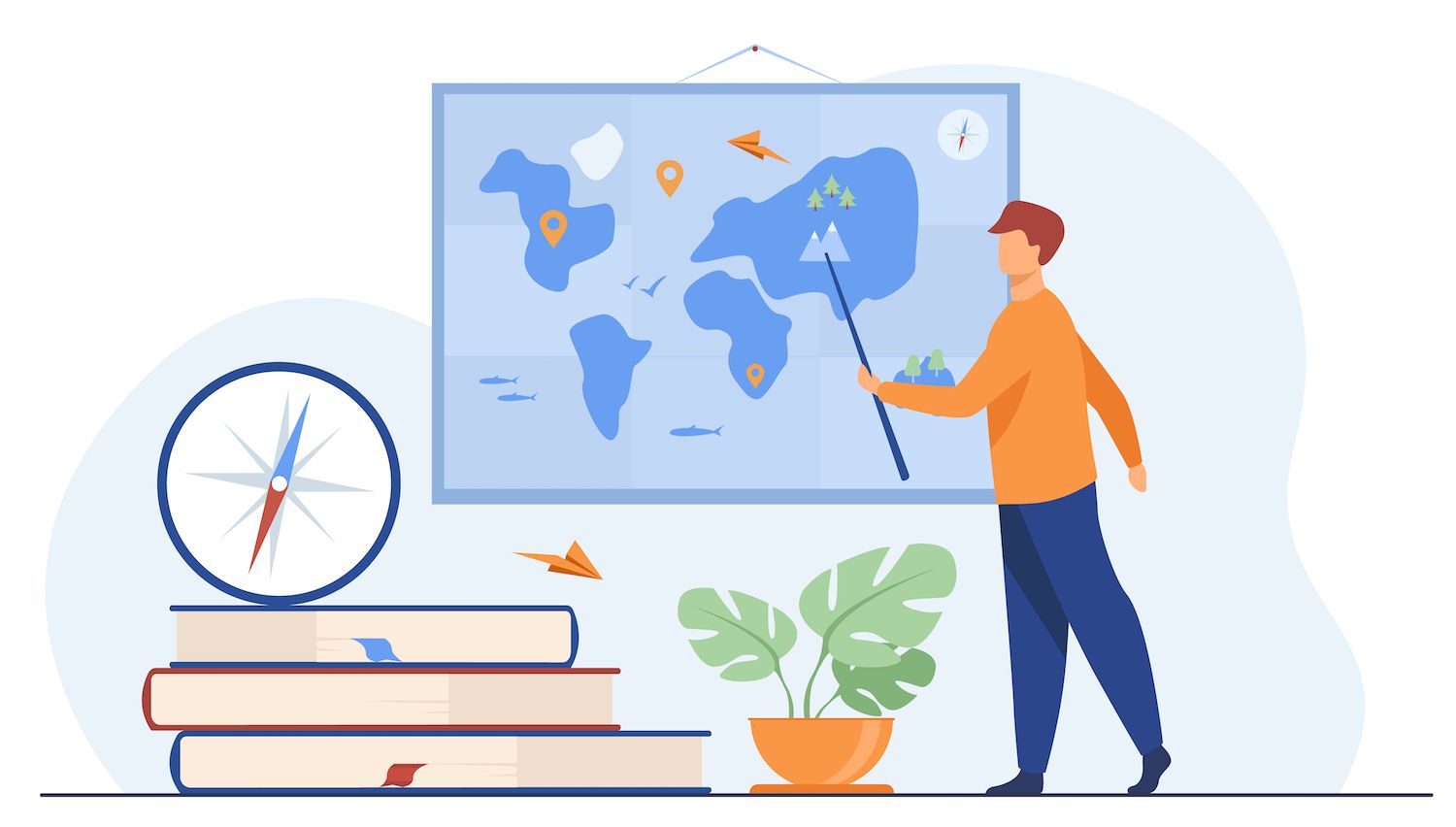
Share the news on
In the case of developing a web-based blogging application that permits authors and moderators to approve comments before they go live, you'd need to test if the function works properly before deploying it to your users. The test needs information.
This article explains how to get comments data without the comments of real users.
The prerequisites
To complete this tutorial it is necessary to be acquainted to the following topics:
- XAMPP
- Composer
Download the complete code for the project so that you can follow along.
How To Set Up the Project
In this part in this section, you'll build a Laravel project and connect it to an online database. We'll look over what it takes and the steps to accomplish this.
Install Laravel Installer
To create an Laravel project quickly, install the Laravel installer.
composer global require laravel/installer
This code installs the Laravel installer on a global basis. device.
Create the Laravel Project
laravel new app-name
The code bootstraps a brand new Laravel project and installs all the dependencies:
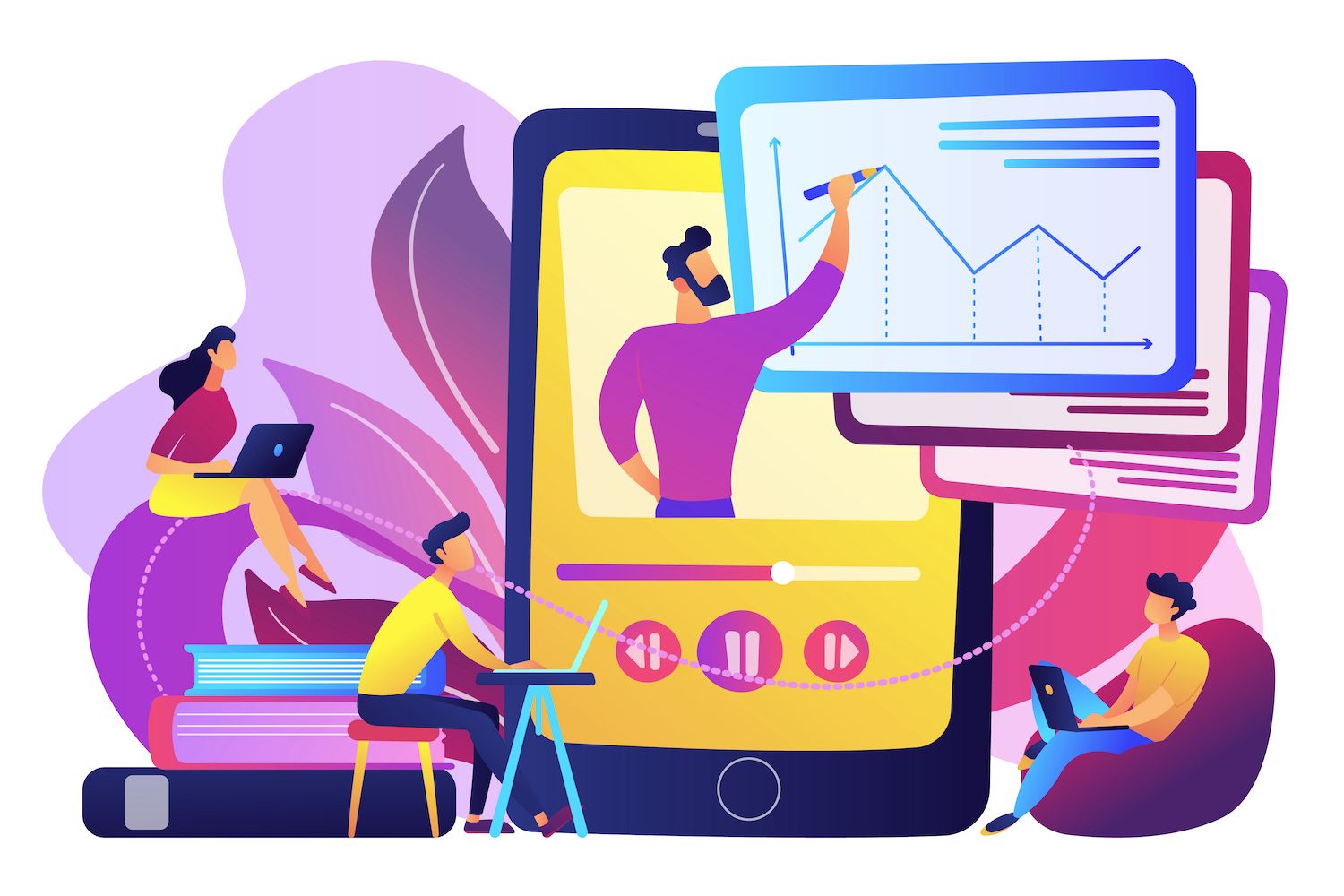
Another easier way to install Laravel is to make use of Composer direct.
composer create-project laravel/laravel app-name
It is not necessary to download Laravel's installer. Laravel installer when following the method above.
Open the app
It is now possible to modify the directory's application-name and begin the project using Laravel's own Command-Line Interface (CLI) tool, Artisan:
php artisan serve
This code begins the project by connecting it to localhost:8000 or another open port, if port 8000 is used. If you connect to localhost:8000, you will find something similar to this:
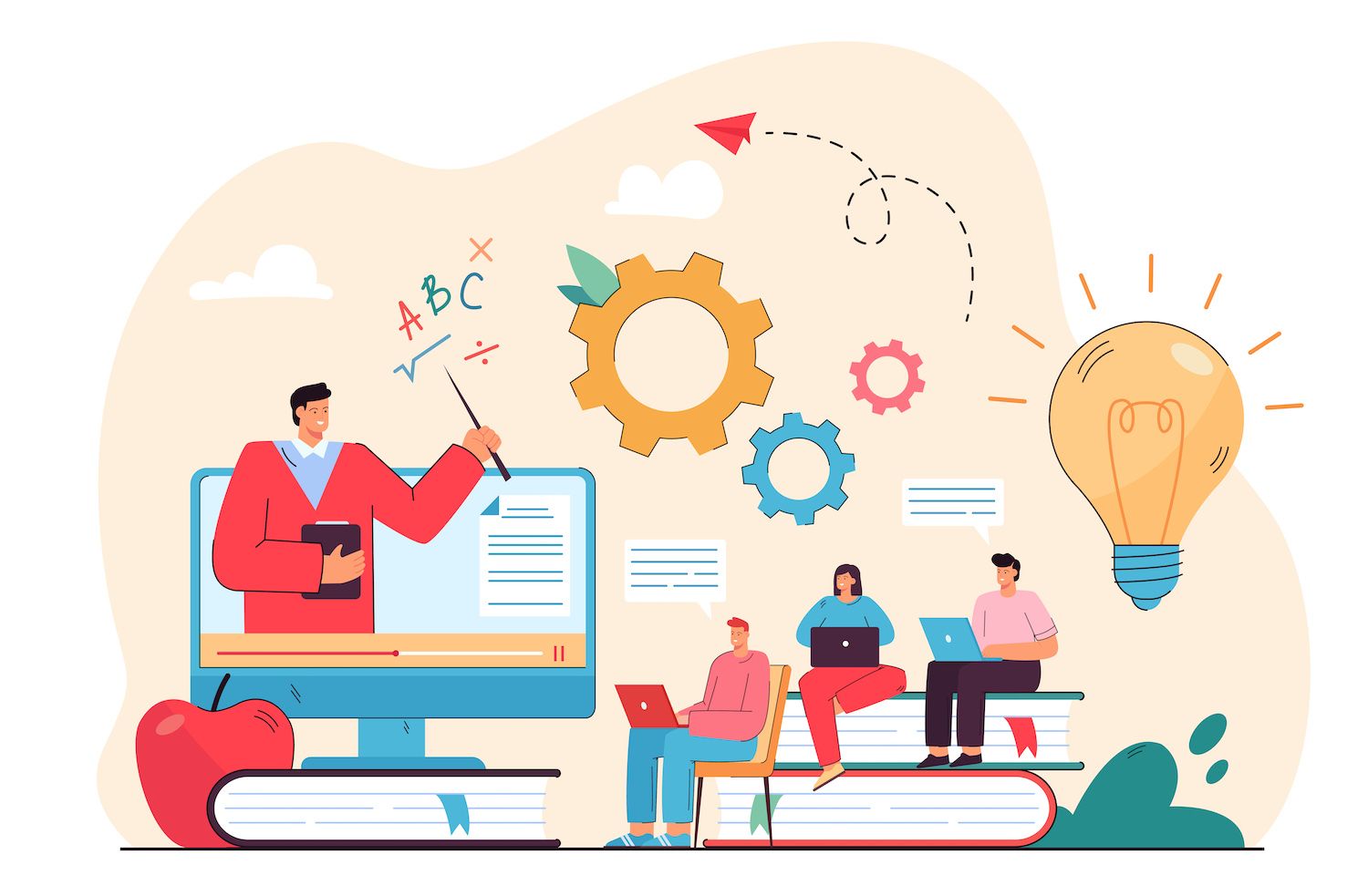
Create a Database
To connect your app to a database, you must make a database from scratch using the XAMPP PHPMyAdmin graphic user interface. Go to http://localhost/phpmyadmin and select New on the sidebar:
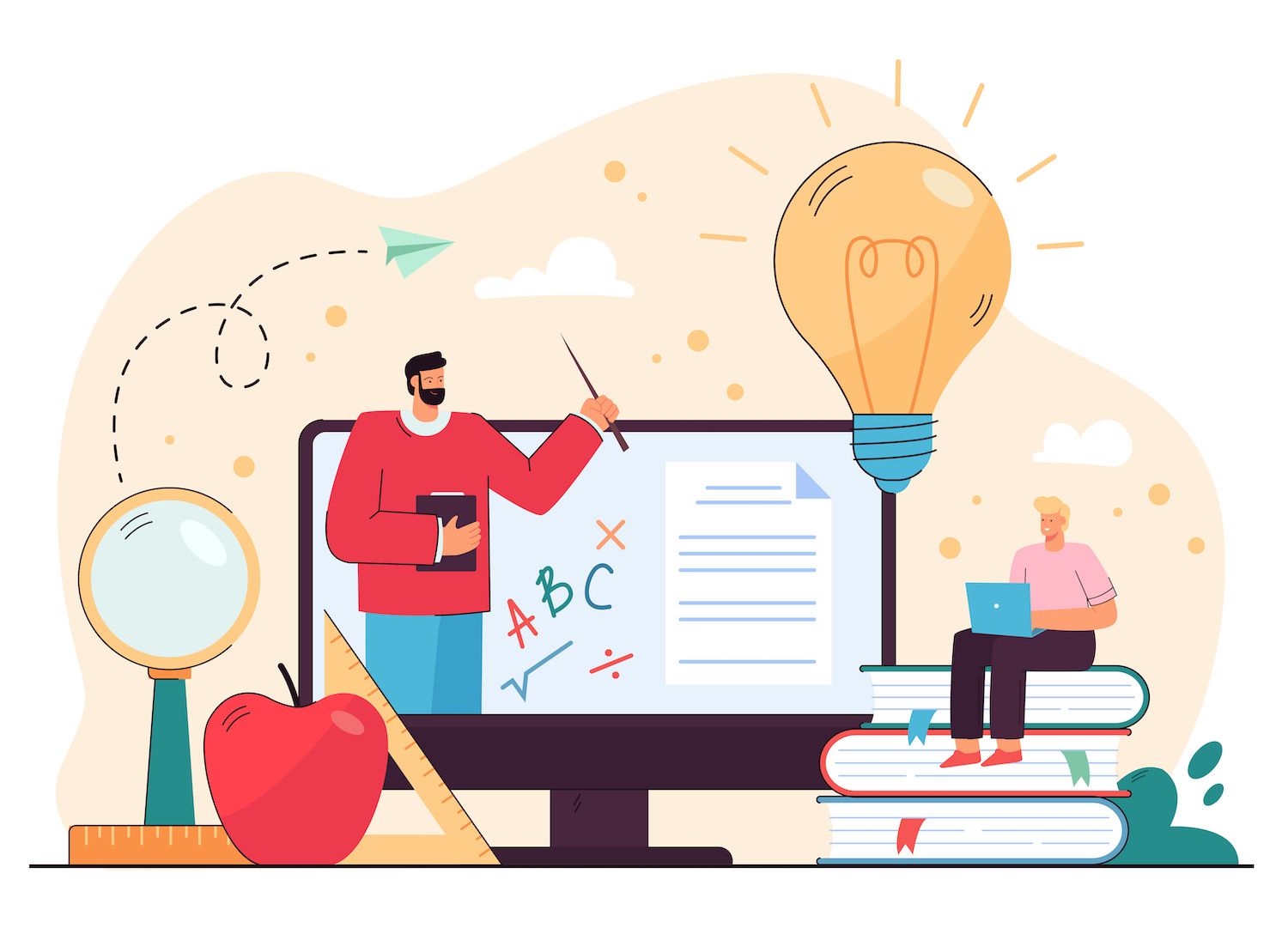
This image shows how to fill out the Create Database form that uses the app_name as the name of the database.
Select "Create" to make an account database.
Modify the .env File
To connect your app to the database, you have to modify the DB portion of your .env file:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=app_name
DB_USERNAME=root
DB_PASSWORD=
The code will fill in the data in your database with the database's name, username, port, password, and host. You're now ready to start creating factories and models.
NOTE: Replace the values by entering your credentials for your database. In case you get the "Access denied for user," error, make sure you put the data for the DB_USERNAME
and the DB_PASSWORD
in double quotation marks.
How To Generate Fake Data
After creating the app and connecting it to the database, you can now create the necessary files for creating fake data within the database.
Create the Comment Model
Create the model file to interact with the databases tables. To create a model, use Artisan:
php artisan make:model Comment
This code generates an Comment.php file inside the app/M odels folder with some boilerplate code. Add the following code below the use HasFactory;
line:
protected $fillable = [
'name',
'email',
'body',
'approved',
'likes'
];
The code below lists the fields that you would like to be able to accept mass assignments since Laravel secures your database against massive assignments automatically. Your comment model file must now look like this:
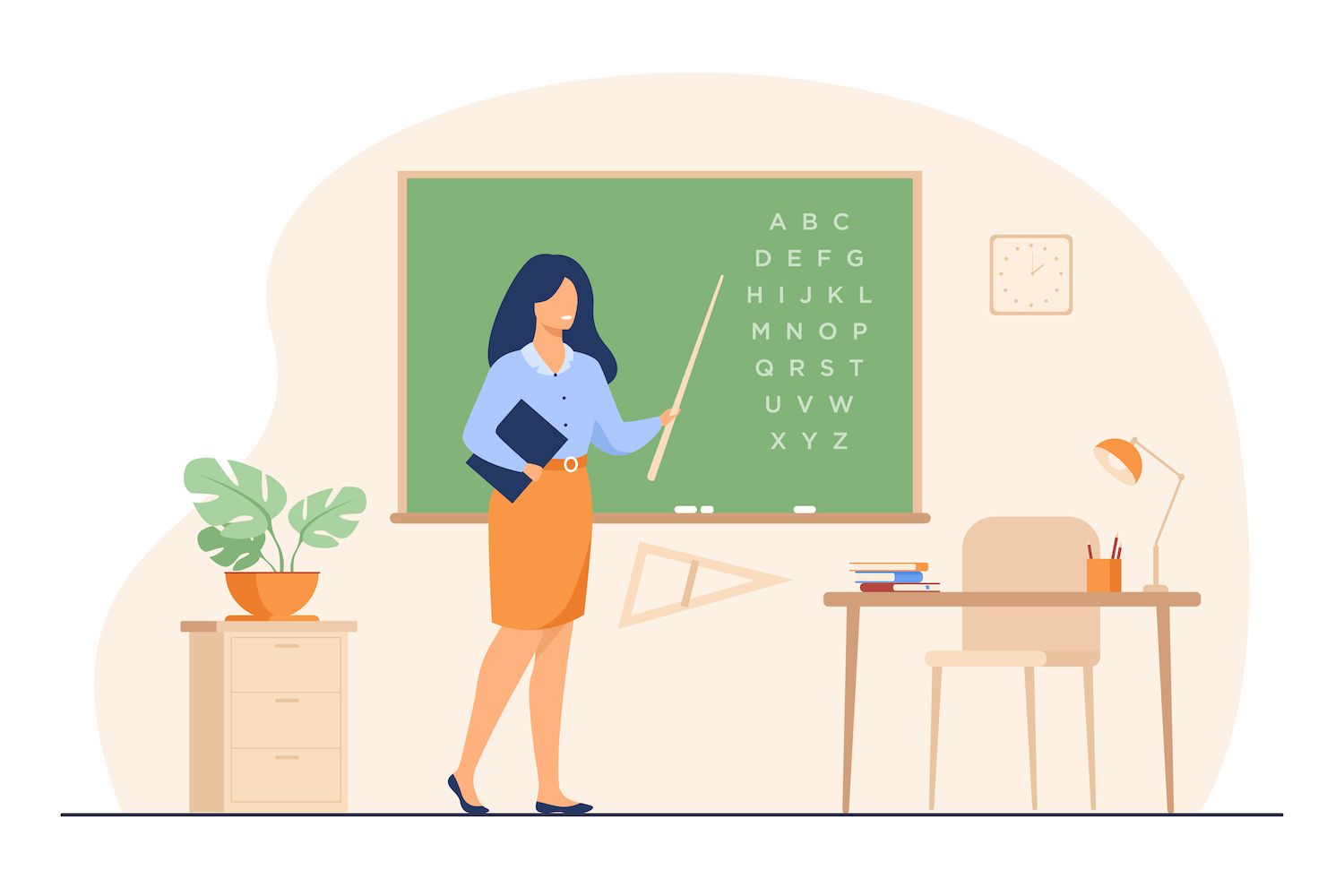
Create the Migration File
After you've created your model file, and declaring the $fillable
array, you will need to create the migration file using the command below:
php artisan make:migration create_comments_table
Note: The naming convention for creating migrations in Laravel is usually the snake_case
which is also referred to as underscore_case
. The first word refers to the action, the second word is a plural of the model and the last word is the feature that gets created inside the application. It is the reason you enter create_books_table
when creating a Migration for the Book model.
This code creates a file named yyyy_mm_dd_hhmmss_create_comments_table inside the database/migrations folder.
Next, edit the up function inside yyyy_mm_dd_hhmmss_create_comments_table:
public function up()
Schema::create('comments', function (Blueprint $table)
$table->id();
$table->string('name');
$table->string('email');
$table->longText('body');
$table->boolean('approved');
$table->integer('likes')->default(0);
$table->timestamps();
);
This code creates the schema for an array of columns id, name, email, body, approval, likeds, as well as timestamps.
Run the Migrations
Making and editing the migrations file won't do anything until you run them through the command line. If you go to the database manager, you'll see that it's still empty.
Perform the migrations with Artisan:
php artisan migrate
This command runs all the changes within the databases/migrations because it's the first time a migration has been run since the app:
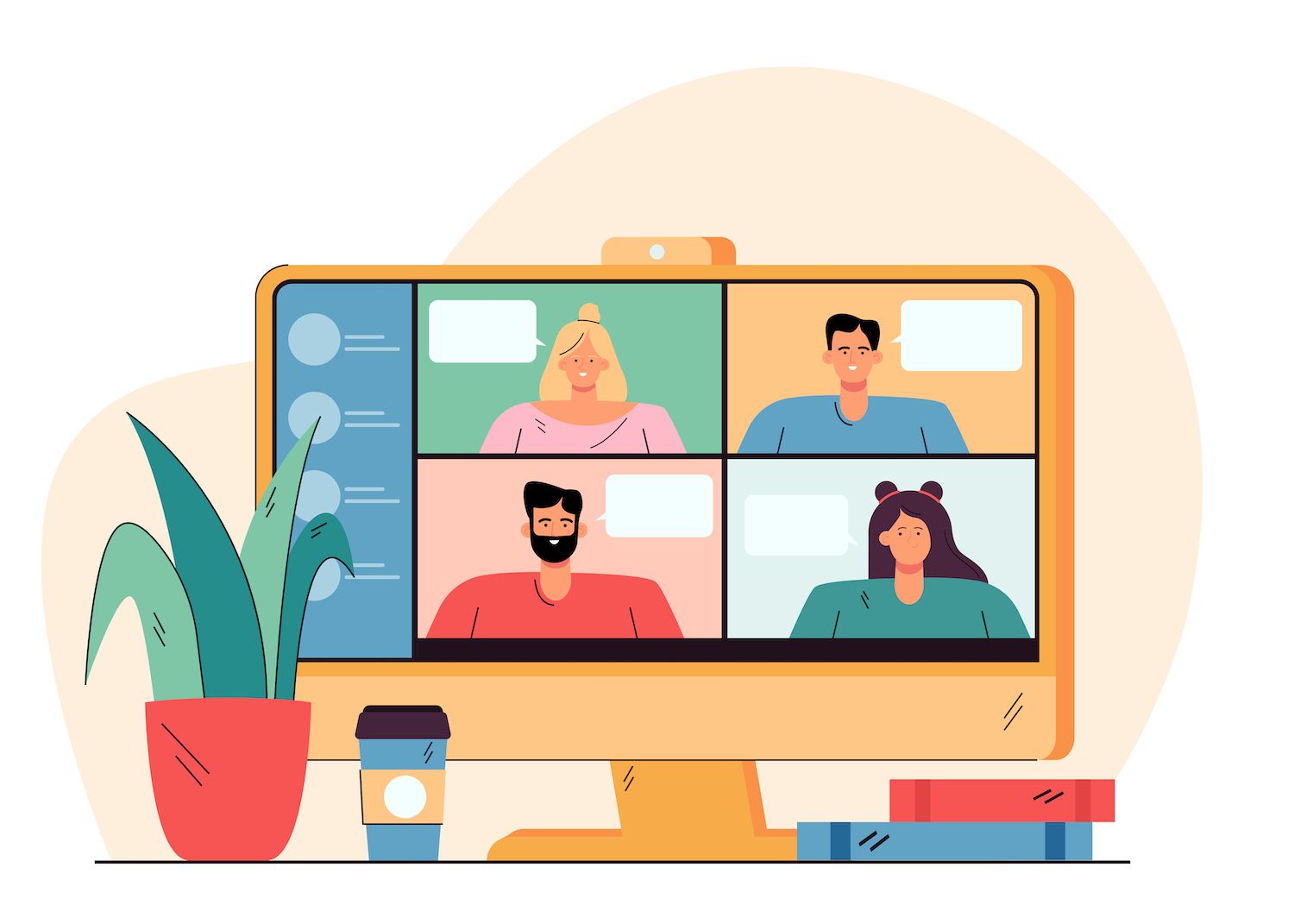
The image below shows all the migration files that you executed. Each file represents a table of the database:
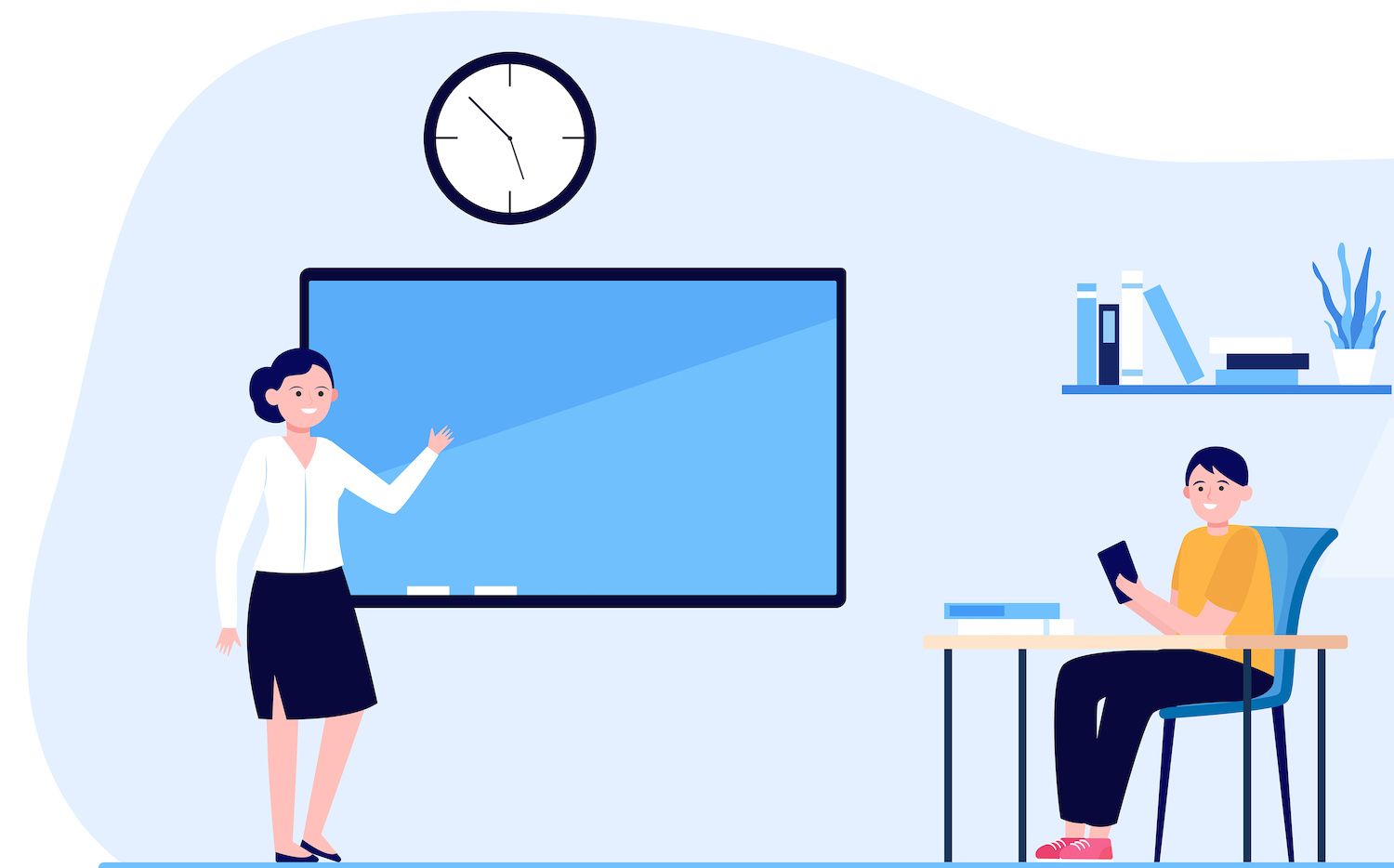
Create the CommentFactory File
Make a factory with your definition function. In this tutorial, you'll build a factory with Artisan:
php artisan make:factory CommentFactory.php
This code makes an CommentFactory .php file inside the directory database/factories folder.
The Function of Definition
The mechanism in CommentFactory describes the way in which Faker produces fake information. Edit it to look as follows:
public function definition()
return [
'name' => $this->faker->name(),
'email' => $this->faker->email(),
'body' => $this->faker->sentence(45),
'approved' => $this->faker->boolean(),
'likes' => $this->faker->randomNumber(5)
];
This code tells Faker to produce the following:
- The name
- An email address
- A paragraph that contains 45 phrases
- A valid value which is only valid if it's real or not
- A random number that ranges between zero and 9999.
Connect the Comment Model to CommentFactory
Attach the Comment
model with the CommentFactory
by declaring a protected $model
variable above the definition of:
protected $model = Comment::class;
Additionally, you should add app ModelsComment
to the file dependencies. The CommentFactory file should now look like:
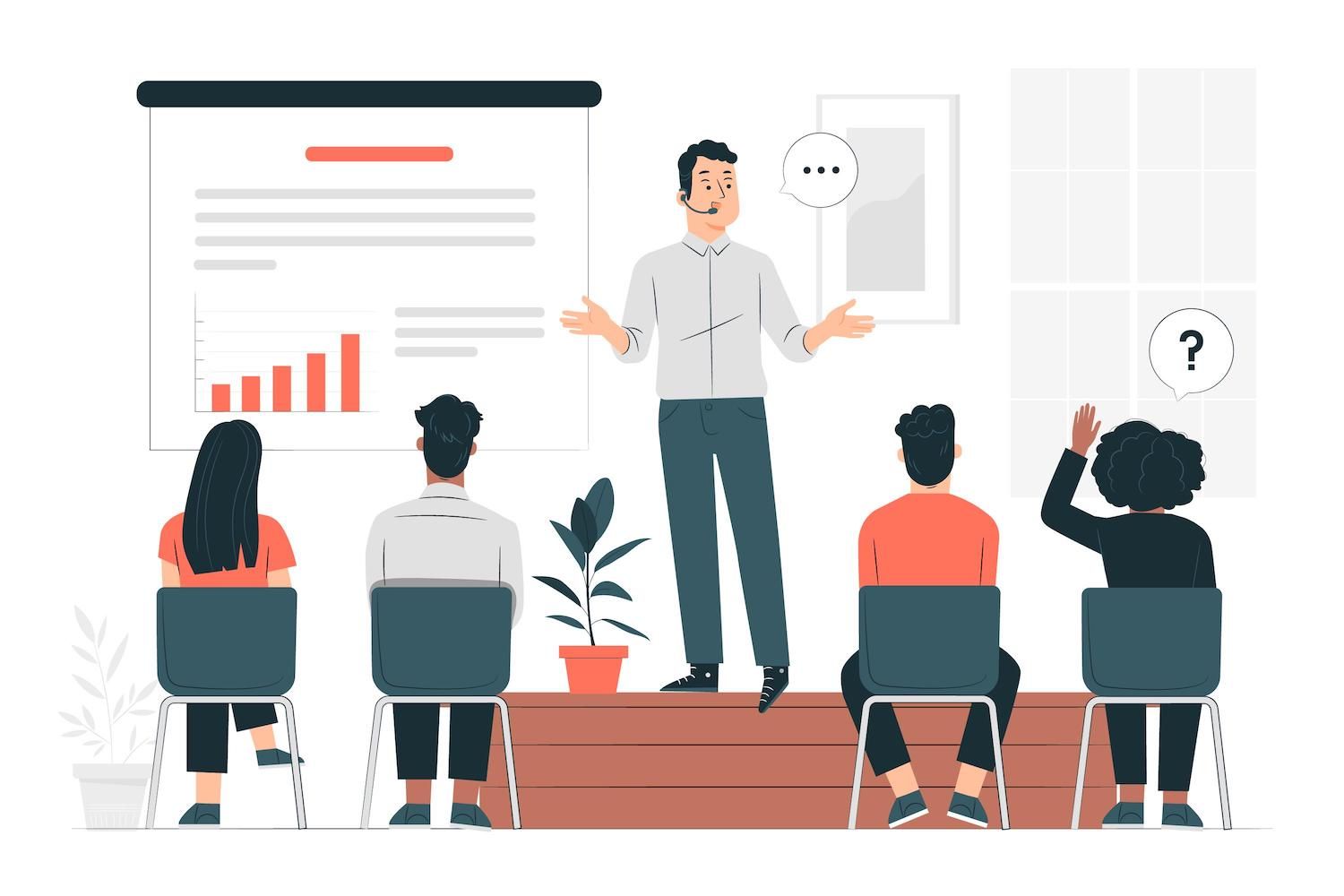
How To Begin Seeding the Database
Seeding in programming means generating random fake data to be stored in databases for testing purposes.
Now that you've created the model, run modifications, and added the definition within CommentFactory. run the seeder using the DatabaseSeeder file.
Create the CommentSeeder File
Create an seeder file that uses factory data to produce it:
php artisan make:seeder CommentSeeder.php
This code creates the CommentSeeder .php file inside the databases/seeders folder.
Change the run function to edit
Connect the Comment model to the CommentSeeder. Include the following code in the run function:
Comment::factory()->count(50)->create();
The code will instruct the CommentSeeder to use the Comment model and CommentFactory's definition function to create 50 comments within the database. Add the Use AppModelsComment
to the file dependencies. CommentSeeder's file should now look like this: CommentSeeder file should now look like this:
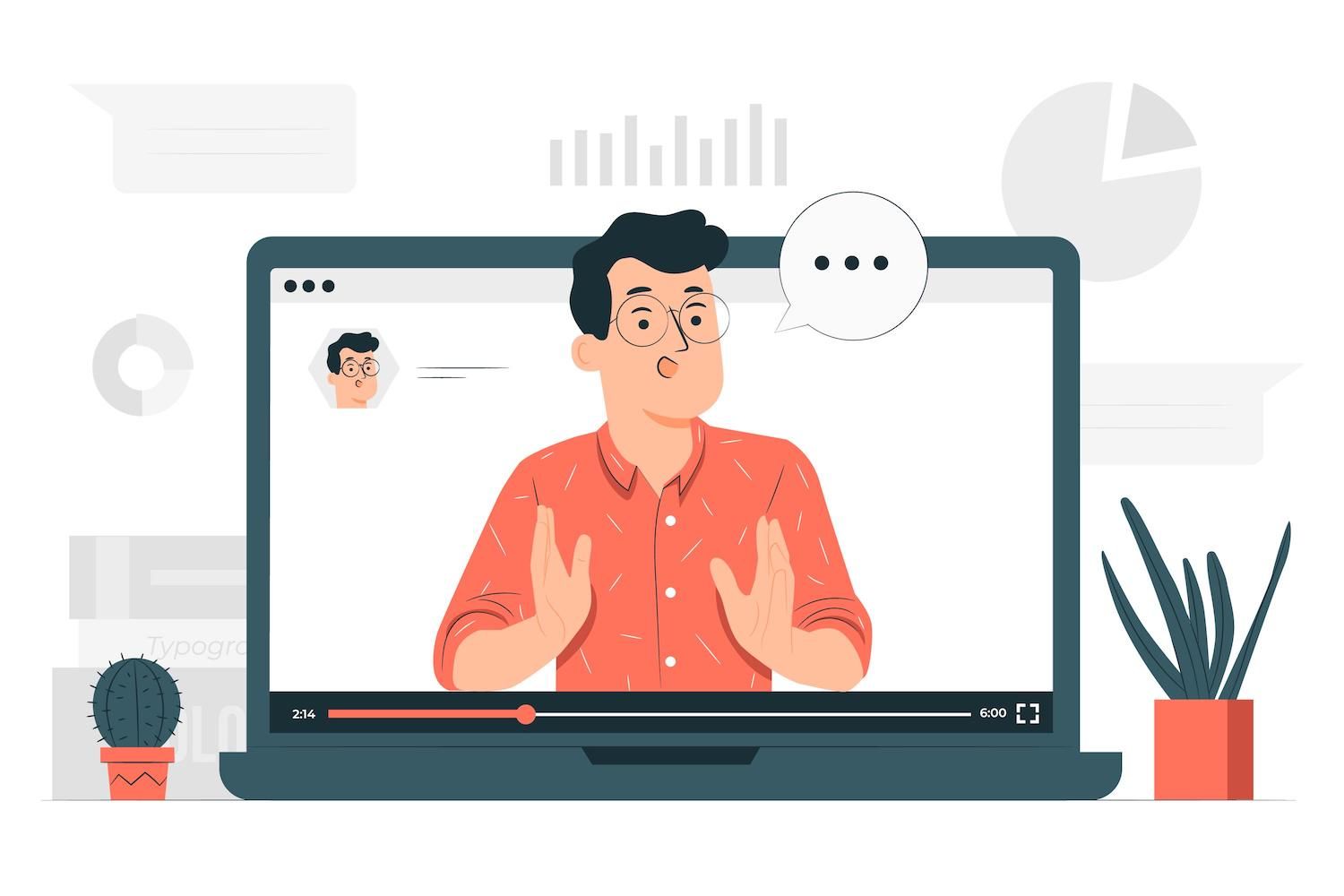
Note: You can configure Faker to create local information. For example, you can configure it to create Italian names rather than random names by changing the faker_locale
inside the app/config.php file to it_IT
. For more information, read regarding Faker Locales within this tutorial.
Run the Seeder
Then, you can run the file to seeder application using Artisan:
php artisan db:seed --class=CommentSeeder
This program runs the Seeder file and produces 50 rows of fake data in the database.
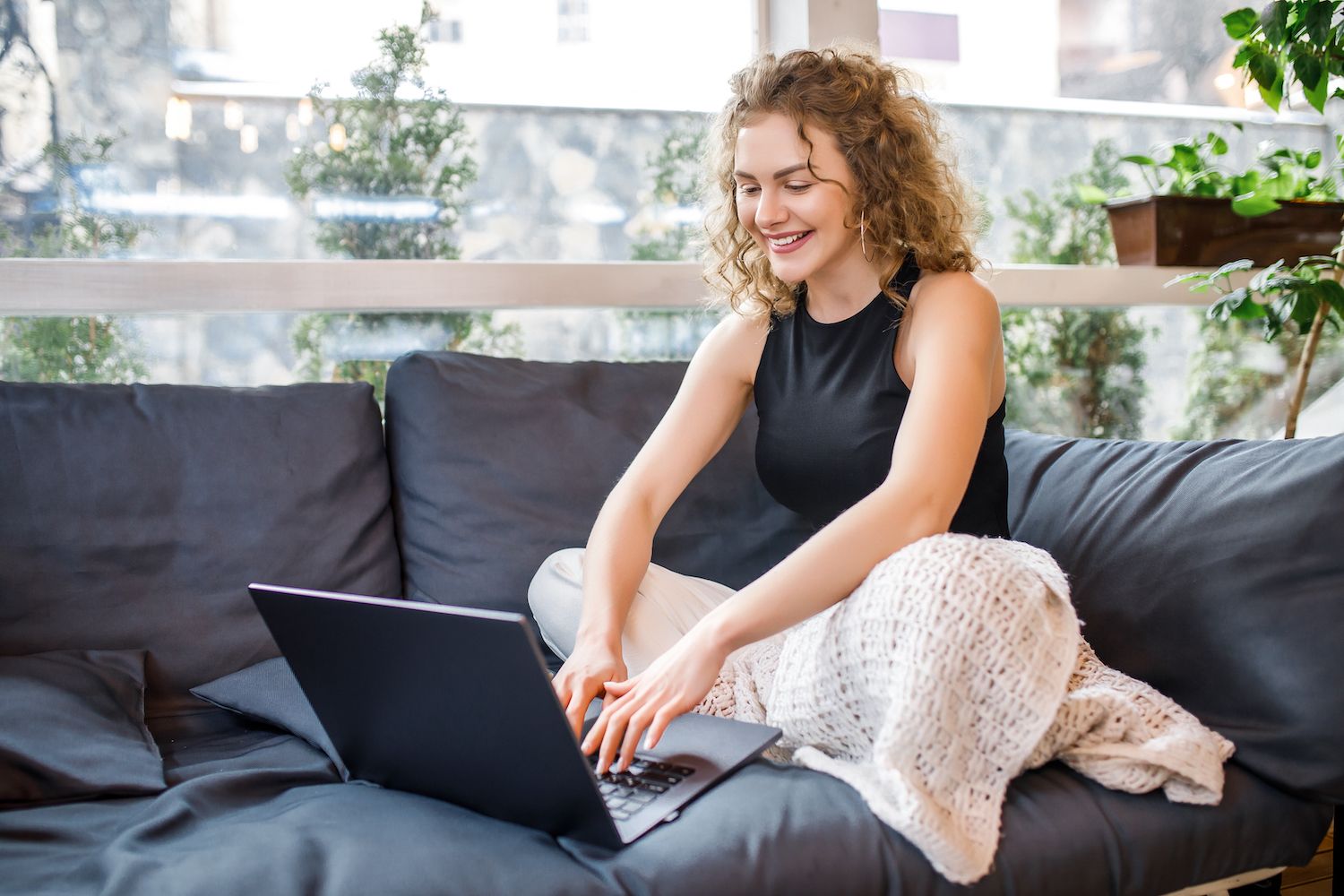
The database should now have 50 rows of fake information which you could use to check the functions of your program:
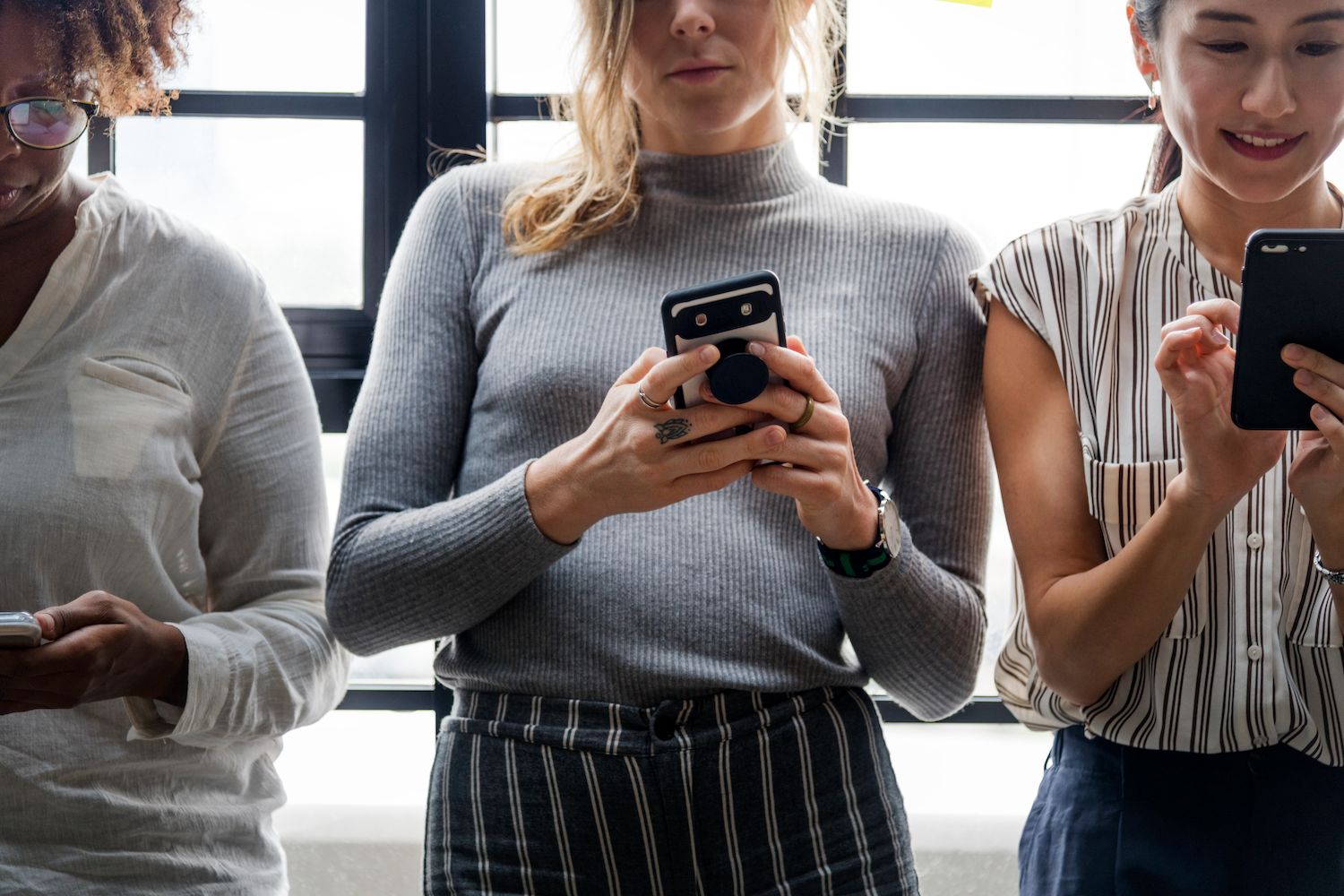
How To Reset the Database
If you are using generated data for testing you can reset your database every whenever you conduct a test. Suppose you wanted to test the comment toggle feature that is approved. Refresh the database after every test to make sure that the previous data generated won't cause problems in the future tests.
Utilize RefreshDatabase
Refresh the database with this RefreshDatabase
trait inside the test file.
Navigate to ExampleTest.php inside the tests/Feature folder to the comment use Illuminate\Foundation\Testing\RefreshDatabase;
and add the following line of code above the test_the_application_returns_a_successful_response
function:
make use of RefreshDatabase
Its ExampleTest.php file should be looking like:
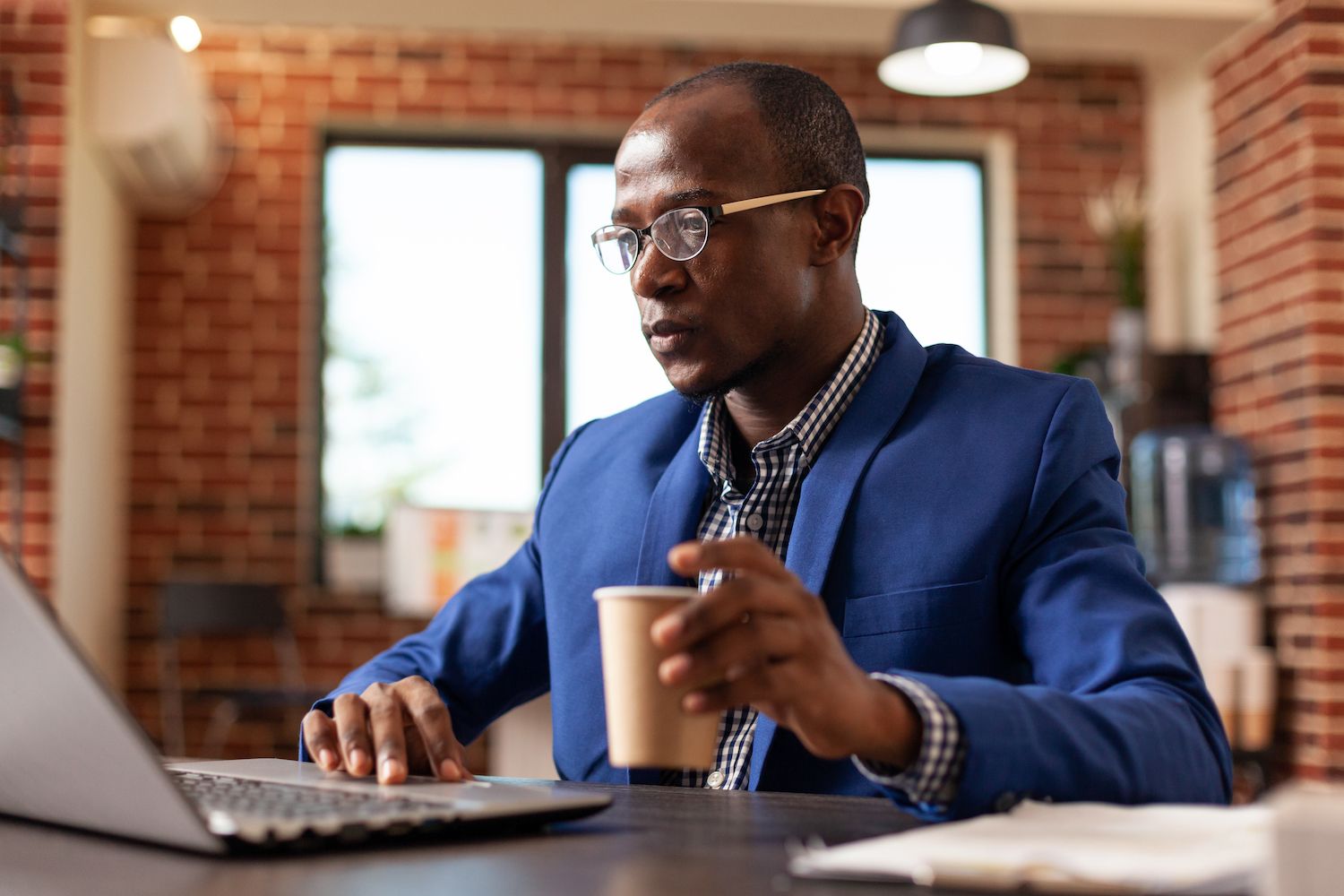
Run the Test
Once you have added the RefreshDatabase
trait to the test file, execute the test with Artisan:
php artisan test
This code performs all tests within the app, and will refresh the database in the aftermath of the tests are completed, as seen in the image below:
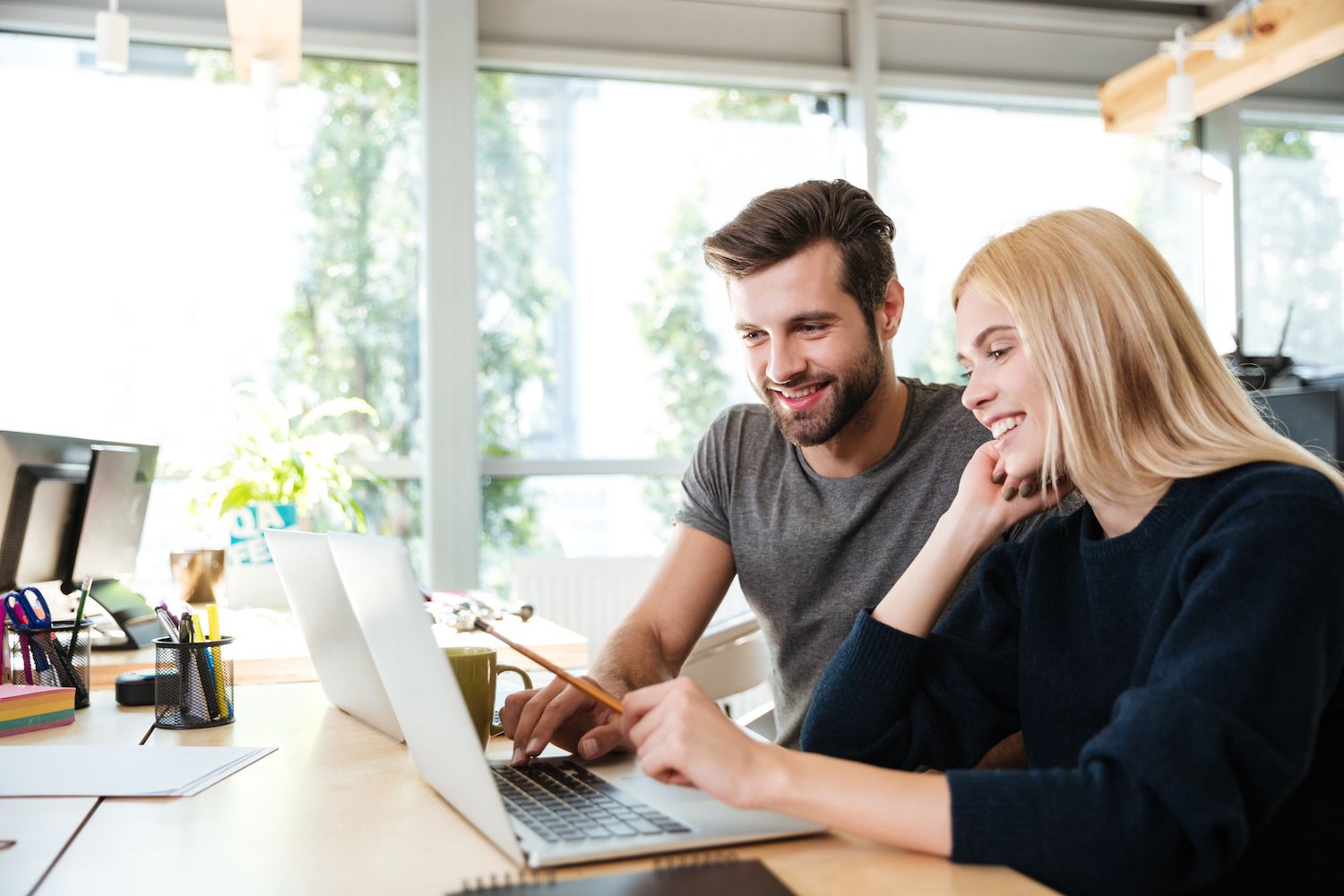
Now, check the database to see the empty comments table:
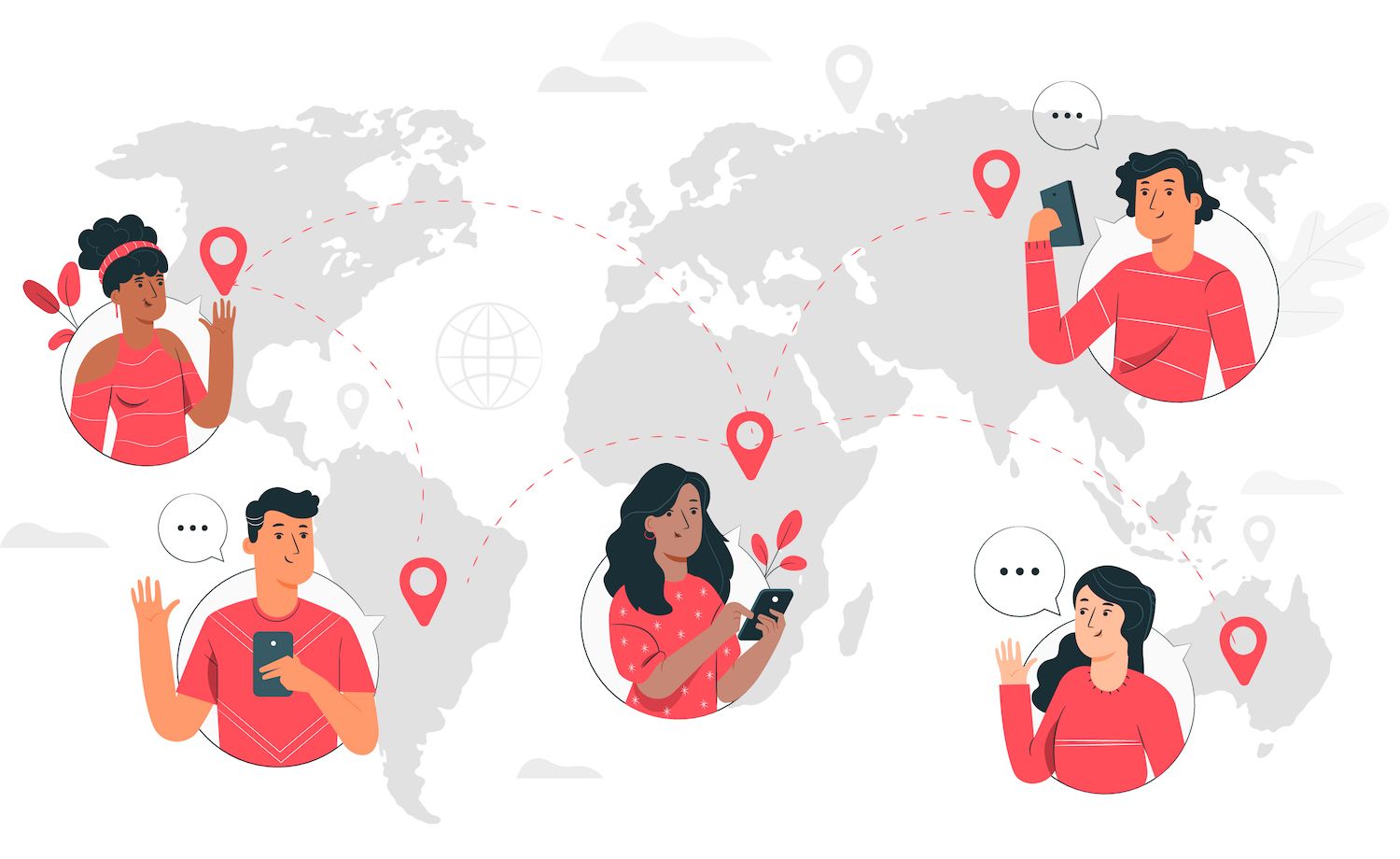
Summary
You've now seen how Laravel Factories and Faker make it possible to produce the entire amount of test data in minutes to test an application or even as placeholder data with minimal setting up.
- Easy setup and management in the My dashboard
- 24 hour expert assistance
- The best Google Cloud Platform hardware and network that is powered by Kubernetes to provide the highest scalability
- A high-end Cloudflare integration to speed up and security
- Global audience reach with up to 35 data centers, and more than 275 PoPs across the globe