Iterate like a Pro The Complete How-to Guide for Python Iterables (r)
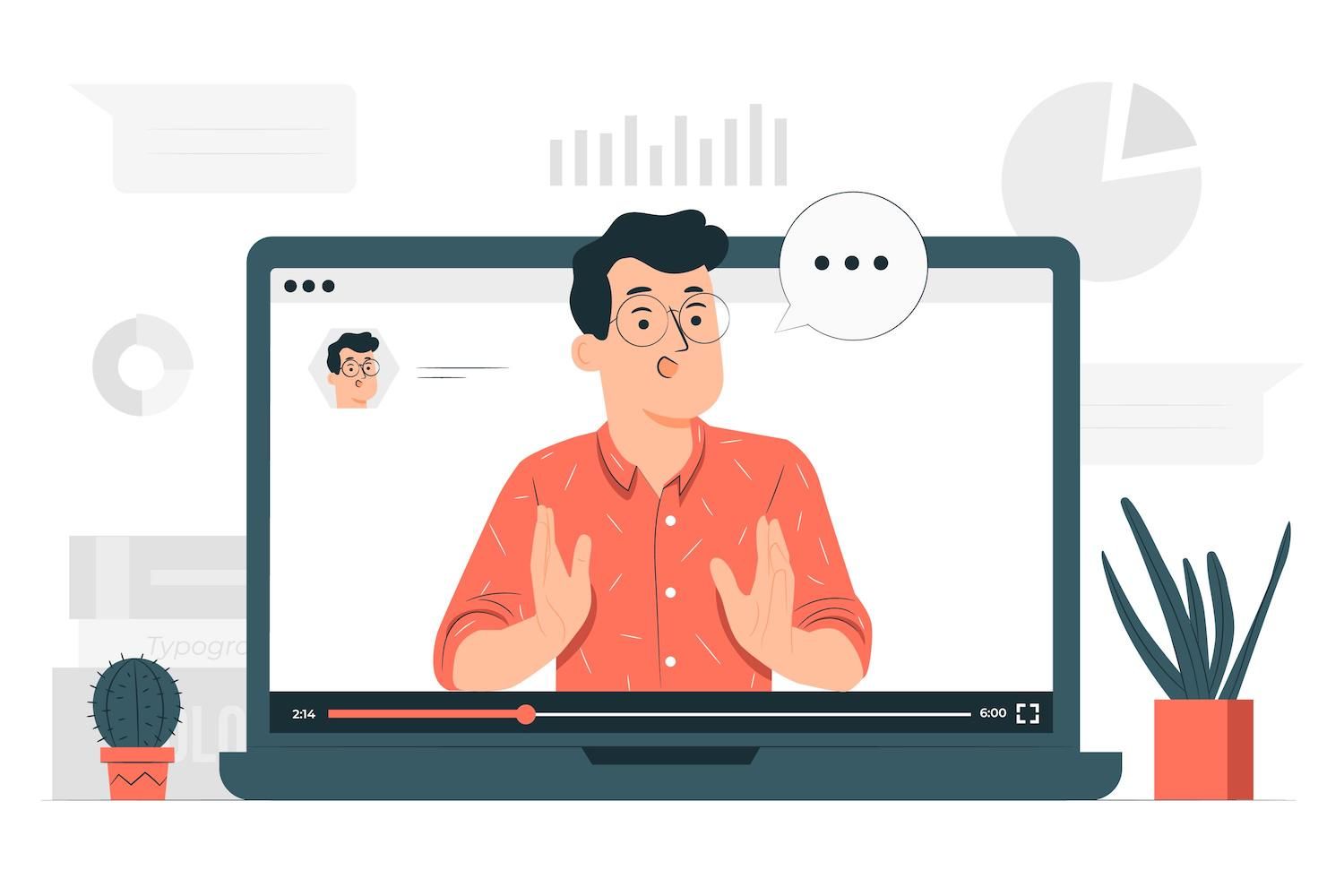
-sidebar-toc>
In Python programming learning and using iterables effectively is the most important aspect to be able to code effectively. Iterables are the objects that which you can loop through. They facilitate the sequential movement of the elements inside their boundaries, which makes them a critical tool for accessing and manipulating elements within objects or data structures.
How to Loop Through Python Iterables
In Python is a programming language that allows you to loop across a variety of iterative types with a for
loop. This lets you navigate the sequences of operations and specific items in sets, lists, and dictionaries.
1. Looping Through a List
Lists are ordered collections of items that allow simple iteration with an for
loop.
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"]
for fruit in fruits_list:
print(fruit)
In the code above, fruit
acts as an iterator which it uses to loop through each list element while simultaneously printing them. The loop is terminated after the evaluation of the last element in the list. This code should produce the output below:
Apple
Mango
Peach
Orange
Banana
2. Then, iterating through a Tuple
They are similar to lists but they're immutable. You can iterate through them just like lists.
fruits_tuple = ("Apple", "Mango", "Peach", "Orange", "Banana")
for fruits in fruits_tupleprint(fruit)
In this case it is the for
loop cycles through the tuple, and in each iteration, iterations of the variable fruit
changes to match the values of the current element in the tuple. The code should give the following output:
Apple
Mango
Peach
Orange
Banana
3. Looping Through Sets
Sets are unordered collections of unique elements. It is possible to traverse them using the for
loop.
fruits_set = "Apple", "Mango", "Peach", "Orange", "Banana"
for fruit in fruits_set:
print(fruit)
In this example in this example, the for
loop iterates through the set. However, since sets are unordered and unsorted, the order of iteration might not be the exact similar to the sequence in which elements were defined in the set. In each iteration the fruit variable fruit
will take on the values of the element currently in the set. The code will produce an output like the one below (the number of elements may be different):
Mango
Banana
Peach
Apple
Orange
4. Repetition of Strings
Strings are characters in a series that can be looped one character at a time.
string = ""
for string char print(char)print(char)
The code below iterates over"" in the text "" and then prints every character on a separate line. Every time iteration is repeated the variable char
is set to the value of the currently displayed character in the string. The code will produce this output:
KN s T
an
5. The Traversing of a Dictionary
Utilizing using the for
loop can be used to using the for loop in sets, lists or tuples as well as strings, but this is different with dictionaries because they make use of key-value pairs for storing things. Dictionarys are a distinct case for looping, as they can be looped using different approaches. These are the many methods you could use to traverse a Python dictionary:
- The keys are being manipulated:
countries_capital =
"USA": "Washington D.C.",
"Australia": "Canberra",
"France": "Paris",
"Egypt": "Cairo",
"Japan": "Tokyo"
for country in countries_capital.keys():
print(country)
The above code creates the dictionary countries_capital
with country names, in which they are the keys, and their respective capitals are the value. This for
loop cycles through the keys of the countries_capital
dictionary, using keys() method. keys()
method. This method generates a view object that displays a list of the keys that are in the dictionary. This lets you easily cycle through all the keys. Every time you repeat the loop, the variable country
will take on the value of the currently active key. The code will produce the following outputs:
USA
Australia
France
Egypt
JapanJapan
- The process of transferring values
countries_capital =
"USA": "Washington D.C.",
"Australia": "Canberra",
"France": "Paris",
"Egypt": "Cairo",
"Japan": "Tokyo"
for capital in countries_capital.values():
print(capital)
In the above code, in the code above, for
loops through the value in the countries_capital
dictionary using values(). the values()
method. The method produces an object with a view, which displays a list of the values that are in the dictionary. This makes it easy to loop through all values. Every time you repeat the loop the capital variable capital
will take on the the current value in the list. This code should give this output:
Washington D.C.
Canberra
Paris
Cairo
TokyoTokyo
- The process of generating key-value pairs
countries_capital =
"USA": "Washington D.C.",
"Australia": "Canberra",
"France": "Paris",
"Egypt": "Cairo",
"Japan": "Tokyo"
for country, capital in countries_capital.items():
print(country, ":", capital)
The above code shows how to loop through the key and value of the countries_capital
dictionary using the items()
method. This items()
method returns a view object which displays an array of keys-value tuples within the dictionary. In the for
loop, each iteration unpacks a key-value pair from the current item in the list. The variables country
capital and capital
have the same keys and values, in turn. This code should give the output below:
USA : Washington D.C.
Australia : Canberra
The city of CanberraFrance : Paris
Egypt : Cairo
Japan : Tokyo
Advanced Iteration with enumerate() Python Python
Another option to iterate on Python iterables and return both the index as well as the value of elements is through the iterate()
function. See this example
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"]
for index, fruit in enumerate(fruits_list):
print(fruit, index)
Here's the output:
Apple 0
Mango 1
Peach 2
Orange 3
Banana 4
The Enumerate
function lets you define the index that will be used as the starting point, besides zero
in the iteration operation. You can modify the example below as follows:
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"]
for index, fruit in enumerate(fruits_list, start = 2):
print(fruit, index)
This is the output:
Apple 2
Mango 3
Peach 4
Orange 5
Banana 6
This example indicates the beginning index of the enumeration process, enumerate
does not use zero-based indexing on the iterable like is the case for native lists. It simply appends the start value to the first element of the list all way to the last one.
How to Implement Python Generators
Generators are specialized Python iterables that allow users to create generator objects without explicitly creating kinds that are built-in, such as lists sets or dictionaries. Generators can be used to produce values as you go based on the generation logic.
Generators make use of the yield
statement to return generated values each one at a. Here's an example of generator iterables
def even_generator(n) Counter = 0
while counter is n
when counter %2 == 0:yield counter
counter= 1for num in even_generator(20):print(num)
The code provided defines the even_generator
function which generates a sequence of even numbers between 0
to a specified number
by using the yield
expression. The loop used for generating these values, and then repeats the process using the num
iterator, ensuring the evaluation of all values within the specified area. This program outputs a table of odd numbers that range from to 20.
up to 20
which is as follows:
0
2
4
6
8
10
12
14
16
18
20
It is possible to achieve greater efficiency when you work with generator expressions. As an example, you could design a generator function that can also include loop logic
cube = (num * 3 for the number in range(1, 6,))
to print c in cube (print(c) in cubeprint(c)
This is where you can assign this cube as a variable cube
to the outcome of a program that calculates the cube of values in the range 1 to 6. Then, it loops through the values within the given range and publishing the results of your computation in sequence, one after another. Its output will be as follows:
1
8
27
64
125
How To Build Custom Iterables
Python allows you to further customize iterable operations with the help of iterators. Iterator objects are a part of the iterator protocol, and have 2 options: __iter__()
and __next__()
. The method __iter__()
method returns an object that is an iterator, whereas the method __next()
returns the next value in the iterable container. Here's an example of iterators using Python:
even_list = [2, 4, 6, 8, 10]
my_iterator = iter(even_list)
print(next(my_iterator)) # Prints 2
print(next(my_iterator)) # Prints 4
print(next(my_iterator)) # Prints 6
In this example, you use to use iter()
method to create an iterator ( my_iterator
) from the following list. To gain access to all items in the list you create an iterator object using the next()
method. Since lists are ordered collections, the iterator returns each element in a sequential fashion.
Customized iterators are great for operations involving large datasets that you can't load into memory concurrently. Because memory is costly and subject to limitations on space and space constraints, it's possible to utilize an iterator to process data elements individually without loading the whole dataset in memory.
Multiple Functionalities
Python uses functions to traverse the elements of a list. Common list functions are:
sum
returns the sum of an iterable, as long as the collection is made up of numerical types (integers floating point numbers, integers as well as complicated numbers)all
-- Returnstrue
when any of the elementable elements is true. In the absence of any, it returnsthe value of false.
.all
Returnstrue
when all elements that can be iterated are valid. If not, it returnsFalse
.max
-returns the highest value that can be obtained from an iterable collectionmin
-- Returns the minimum value of an iterable collectionlen
-- Returns the length of an iterableappend
-- Appends an additional value at the bottom of a list iterable
The example below demonstrates these functions with this list:
even_list = [2, 4, 6, 8, 10]
print(sum(even_list))
print(any(even_list))
print(max(even_list))
print(min(even_list))
even_list.append(14) # Add 14 to the end of the list
print(even_list)
even_list.insert(0, 0) # Insert 0 and specified index [0]
print(even_list)
print(all(even_list)) # Return true only if ALL elements in the list are true
print(len(even_list)) # Print the size of the list
Here's the result:
30
True10
2[2, 4 6, 8, 10, 14]
[0 2, 4 7 8 14]
False7
In the above example, append is the append
function is used to add a single number ( 14
) at the bottom of the listing. Insert function allows you to specify the index for insertion. function insert
function allows specifying the index for insertion. Thus, even_list.insert(0, 0)
inserts 0
at index [0insert[0, 0]
.
The statement print(all(even_list))
returns false
because there is a 0
value in the list, interpreted as false
. Finally, print(len(even_list))
outputs the length of the iterable.
Advanced Iterable Operations
Python provides advanced capabilities that promote conciseness in iterable processes. Listed below are some of these features.
1. List Comprehensions
List comprehensions allow you to create new lists by adding an action to each item in existing lists. Here's an example
my_numbers = [11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
even_number_list = [num for num in my_numbers if num%2 == 0]
print(even_number_list)
In this code snippet, a list named my_numbers
is generated using numbers ranging from 11
up to 20
. Its goal is to create an entirely new list, even_number_list
that contains only even integers. For this to be achieved, use a list comprehension that returns the number of integers from my_numbers
only when the integer is even. The if
clause contains the logic that returns the even numbers.
Here's the result:
[12, 14, 16, 18, 20]
2. Zip
Python's zip()
function combines many iterables to create Tuples. Tuples can store a variety of values in one variable, and they are unchangeable. Here's how to combine iterables by using zip()
:
fruits = ["apple", "orange", "banana"]
rating = [1, 2, 3]
fruits_rating = zip(rating, fruits)
print(list(fruits_rating))
In this example, fruits_rating
pairs every fruit with its rating making a single iterable. Its output will be:
[(1, 'apple'), (2, 'orange'), (3, 'banana')]
The code functions as a system of rating for different fruits, with the first lists ( fruits
) representing the fruits while the second one presenting ratings on a scale from 1 up to 3.
3. Filter
Another function that is more advanced, filter, is a function that takes two arguments that are functions and an iterable. The function is applied to every element of the iterable, then returns a new iterable containing only the elements that are for which the function gives an true
value. The example below is a filtering of an array of integers within the specified range, returning only those that are even:
def is_even(n):
return n%2 == 0
nums_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_list = filter(is_even, nums_list)
print(list(even_list))
In the code above in the code above, you begin by creating an algorithm, is_even
which computes an even number passed to it. You then create an integer list between 1 and 10-- the nums_list
. Finally, you define a new list, even_list
, which uses it's filter()
function to apply the method that you have defined on the existing list to provide only those elements of the list that are even. The result is:
[2, 4, 6, 8, 10]
4. Map
Similar to filter()
, Python's map()
function takes an iterable as well as an argument as the arguments. However, instead of returning all elements that were in the original iterable it creates a new iterable containing the result of the function applied to the elements of the previous iterable. For a squared list of numbers, make use of this mapping()
function:
my_list = [2, 4, 6, 8, 10]
square_numbers = map(lambda x: x ** 2, my_list)
print(list(square_numbers))
In this code, x
is the iterator which traverses the list and transforms it by using the square algorithm. The map()
function executes this process by using the list's original argument, and then a mapping function. The result is like this:
[4, 16, 36, 64, 100]
5. Sorted
The sorted
function arranges the elements of an iterable in an order that is specific (ascending or decresing) and then returns the result in an array. It takes in the maximum three parameters -- iterable
, reverse
(optional) as well as iterable, reverse (optional), and key
(optional). Then, reverse
defaults to False
when you set it at True
the elements are ordered in ascending order. key
is a function that computes a value in order to determine the sorting order of an element that is an iterable, and this defaults to the value None
.
Here's an example of how you can implement the sorted
function to different iterables:
# set
py_set = 'e', 'a', 'u', 'o', 'i'
print(sorted(py_set, reverse=True))
# dictionary
py_dict = 'e': 1, 'a': 2, 'u': 3, 'o': 4, 'i': 5
print(sorted(py_dict, reverse=True))
# frozen set
frozen_set = frozenset(('e', 'a', 'u', 'o', 'i'))
print(sorted(frozen_set, reverse=True))
# string
string = ""
print(sorted(string))
# list
py_list = ['a', 'e', 'i', 'o', 'u']
print(py_list)
This gives you the following output:
['u', 'o', 'i', 'e', 'a']
['u', 'o', 'i', 'e', 'a']
['u', 'o', 'i', 'e', 'a']
['a', 'i', 'k', 'n', 's', 't']
['a', 'e', 'i', 'o', 'u']
What to do with Errors and Edge Cases Iterables
Edge cases are frequently encountered in many programming scenarios, and you should anticipate them in iterables. Let's explore a few possibilities you may encounter.
1. Incomplete Iterables
You may run into issues when an iterable is empty but a program logic is trying to navigate it. You can address this programmatically to avoid inefficiencies. This is an example of using an If not
expression to verify that an item is not empty.
fruits_list = []
If the list is not fruit-related If not,print("The list is empty")
This is the result:
The table is not empty
2. Nested Iterables
Python is also able to support nested Iterables, which are iterable objects containing other iterables within them. You can, for instance, create a list of foods containing an array of food categories that are nested like vegetables, meats and grains. Here's how to model such a scenario using nested iterables
food_list = [["kale", "broccoli", "ginger"], ["beef", "chicken", "tuna"], ["barley", "oats", "corn"]]
for inner_list in food list:to list food items in the inner list:print(food)
In the code above, you can see that the food_list
variable contains three nested lists, representing different food groups. Its outer loop ( for inner_list in food_list
) loops through the main list and inside the loop ( for food in the inner list:
) iterates through each nested list, printing each food items. The output is as follows:
Kale broccoligingerchicken
tunabarley
oats,corn
3. Exception Handling
In Python iterables, they also allow exception-handling operations. For example, you may traverse a list, but come across an index error
. This means that you're trying to reference something that is beyond the iterable's bounds. How do you deal with such an error using a attempt-to-exclude
block:
fruits_list = ["apple", "mango", "orange", "pear"]
try:
print(fruits_list[5])
except IndexError:
print("The index specified is out of range. ")
The code below shows that the fruits_list
iterable is comprised of five elements with indices of 0
to five
within the collection of list. Try phrase test
phrase includes the print
function which attempts to display the value at index five
in the iterable that isn't there. This executes the except clause and returns the error message. The console returns the error:
The specified index is not in the the range.
Summary
Learning how to iterate in Python is vital to write efficient and readable programming. Learning the many ways to create different types of types of data structures by using comprehensions, generators, and leveraging built-in functions makes you a proficient Python programmers.
Have we missed anything from the guide? Do let us know in the comments section!
Jeremy Holcombe
Content and Marketing Editor at , WordPress Web Developer, and Content writer. Apart from all things WordPress I love playing golf, at the beach as well as movies. I also have tall people issues ;).