Laravel API: Create and Test an API for Laravel -- (r)
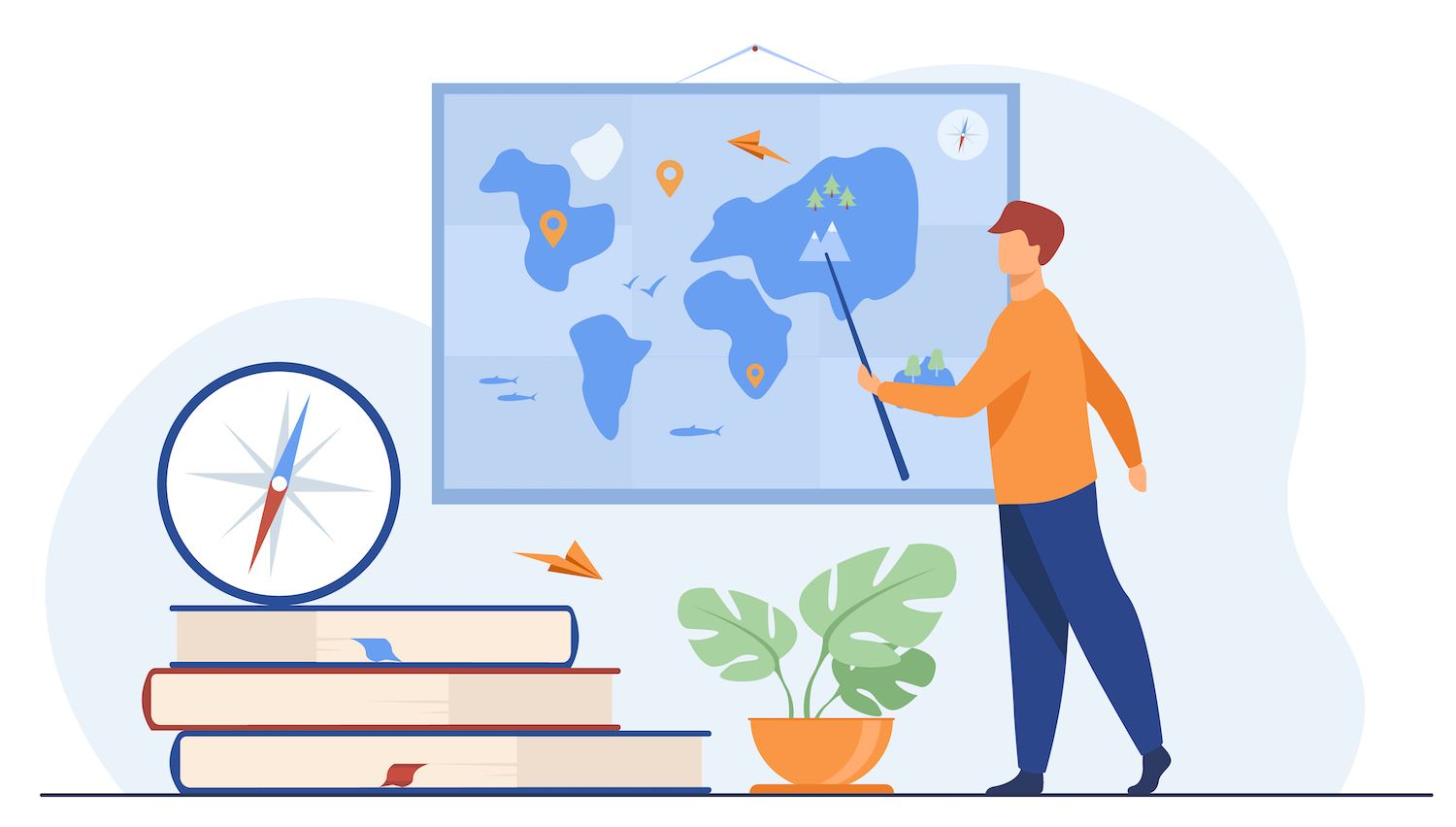
Share the news on
Laravel Eloquent is an easy way to interact to your databases. It is an object-relational mapper (ORM) which simplifies the complexity of databases through providing a framework that allows you to communicate with tables.
In this way, Laravel Eloquent has excellent tools for creating and testing APIs that can help you develop. In this hands-on article you'll discover how easy it is to build and test APIs using Laravel.
The prerequisites
In order to get started, here's what you'll need:
API Basics
Begin by establishing a brand new Laravel project with composer
:
composer create-project laravel/laravel laravel-api-create-test
To begin the server run the following command. This runs the application server on port 8000:
cd laravel-api-create-test
php artisan serve
It is recommended that you look at the following screen:
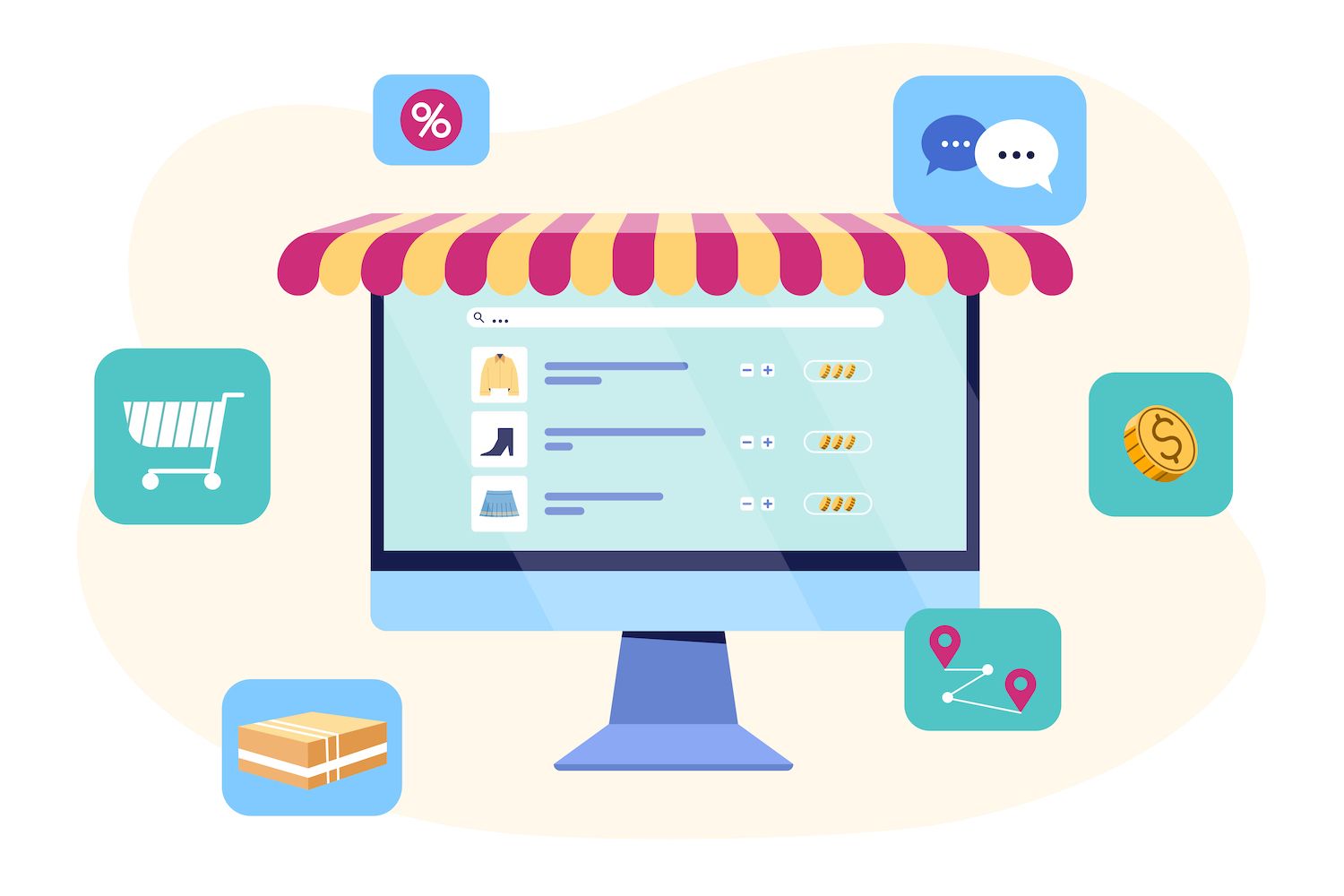
Then, create a model with a "-m
flag to allow the migration with the code in the following:
php artisan make:model Product -m
Then, upgrade the file to incorporate the necessary field. Add title and description fields for the product model and these two table fields inside the database/migrations/date_stamp_create_products_table.php file.
$table->string('title');
$table->longText('description');
The next step is making these fields fillable. Inside app/Models/Product.php, make title
and description
fillable fields.
protected $fillable = ['title', 'description'];
How Do You Make the Controller
Create a controller file for the product by executing the following command. This will create the app/Http/Controllers/Api/ProductController.php file.
php artisan make:controller Api\\ProductController --model=Product
Add the logic to create and retrieving products. Inside index method index
method, you can add the following code to retrieve each product
Products = $products = All:();
return the response ()->json(["status" = false and 'products'more than $products);
After that, you must add a StoreProductRequest
class to store your new items in your database. The following class should be added at the top of the same file.
public function store(StoreProductRequest $request)
$product = Product::create($request->all());
return response()->json([
'status' => true,
'message' => "Product Created successfully! ",
'product' => $product
], 200);
Now, you'll create the request. You can do by executing the below command:
php artisan make:request StoreProductRequest
If you want to add validations, you can use the app/Http/Requests/StoreProductRequest.php file. This is a demonstration where there is no validation.
How To Create an Route
The last step prior to trying out the API is adding a route. To do so, add this code in the routes/api.php file. Add to the file a use
declaration at the start of the file and the the Route
statement in the body:
use App\Http\Controllers\Api\ProductController;
Route::apiResource('products', ProductController::class);
Before beginning to test the API, make sure that you have the Products table exists in your database. If not then create it with a control panel like XAMPP. You can also execute the following command to migrate the database:
php artisan migrate
How to Test an API
Before testing the API, ensure that the authorize
method inside the app/Http/Requests/StoreProductRequest.php is set to return true
.
You can now create a new product using Postman. Start by hitting a POST
request to this URL: http://127.0.0.1:8000/api/products/. Because this is an request to POST a
request to create an entirely new product that you need to provide a JSON object with a title and description.
"title":"Apple",
"description":"Best Apples of the world"
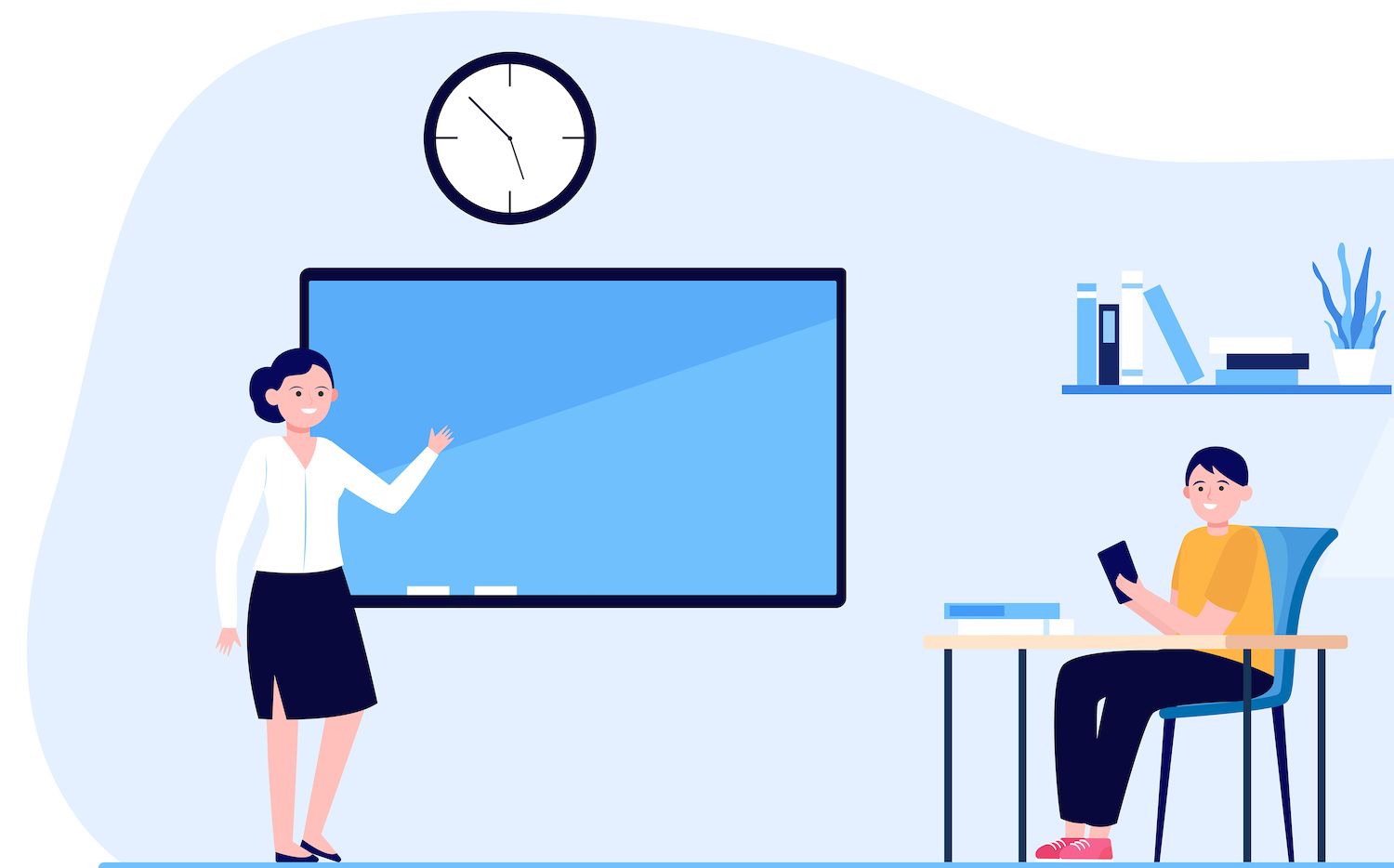
After clicking to click the "Send" button, you will get the following information:
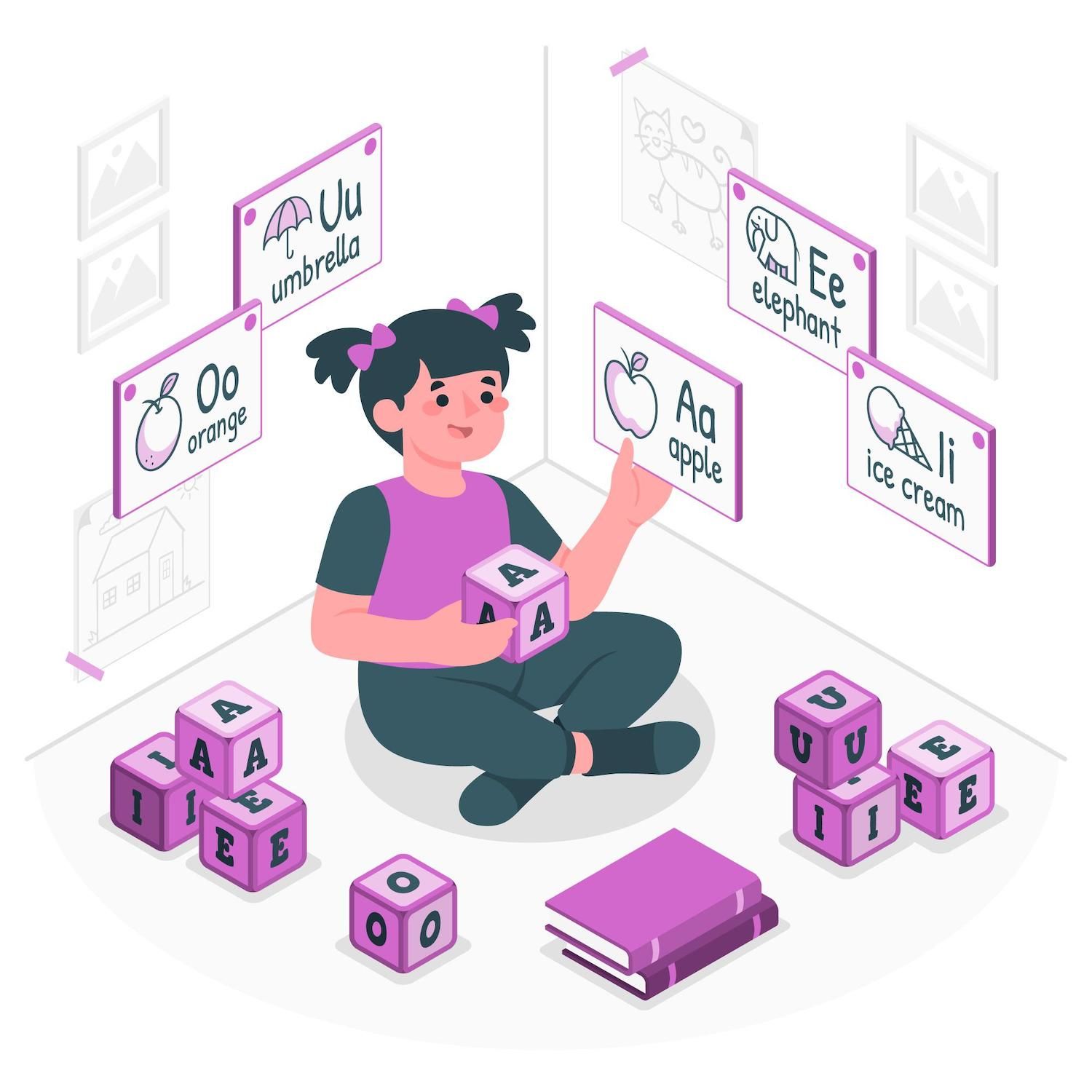
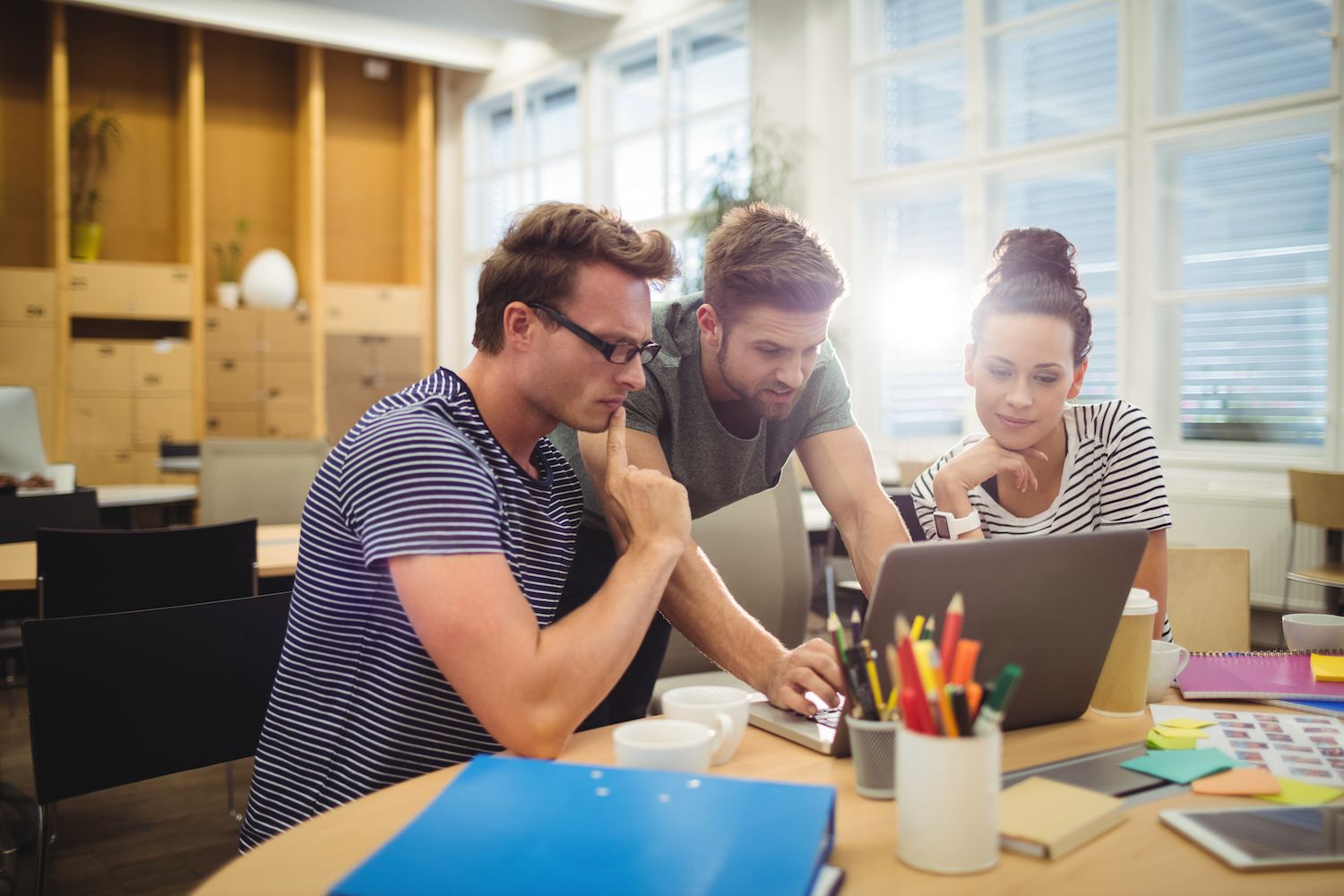
What is the best method to authenticate APIs by using Sanctum
Authentication is crucial when securing the security of an API. Laravel makes it easy through the function that comes with the Sanctum token that could be used as a middleware. It protects the API using tokens generated when users log in with the correct username and password. Be aware that the user cannot access the secure API without the token.
The first step for adding authentication is to include a Sanctum package by using the code as follows:
composer require laravel/sanctum
Then, publish the Sanctum configuration file:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Following that, include Sanctum's token to middleware. In the app/Http/Kernel.php file, make use of the following class, and replace middlewareGroups
with the code below in the protected middleware groups' API.
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
protected $middlewareGroups = [
'web' => [
\App\Http\Middleware\EncryptCookies::class,
\Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class,
\Illuminate\Session\Middleware\StartSession::class,
// \Illuminate\Session\Middleware\AuthenticateSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\App\Http\Middleware\VerifyCsrfToken::class,
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
'api' => [
EnsureFrontendRequestsAreStateful::class,
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
];
The following step is to build a UserController
and then add the code that will generate the token needed to authenticate.
php artisan make:controller UserController
After creating the UserController
, navigate to the app/Http/Controllers/UserController.php file and replace the existing code with the following code:
Before you test the authentication, create an account for a user using seeders. Following command generates a users table file.
php artisan make:seeder UsersTableSeeder
Inside the database/seeders/UsersTableSeeder.php file, replace the existing code with the following code to seed the user: insert([ 'name' => 'John Doe', 'email' => '[email protected]', 'password' => Hash::make('password') ]);
Run the seeder now with this command:
php artisan db:seed --class=UsersTableSeeder
The last step left to complete the authentication process is to utilize the middleware created to secure the routing. Browse through the routes/api.php file and add the products route inside the middleware.
use App\Http\Controllers\UserController;
Route::group(['middleware' => 'auth:sanctum'], function ()
Route::apiResource('products', ProductController::class);
);
Route::post("login",[UserController::class,'index']);
Once you've added a route the middleware, you'll get an internal server error if you try to fetch the products.
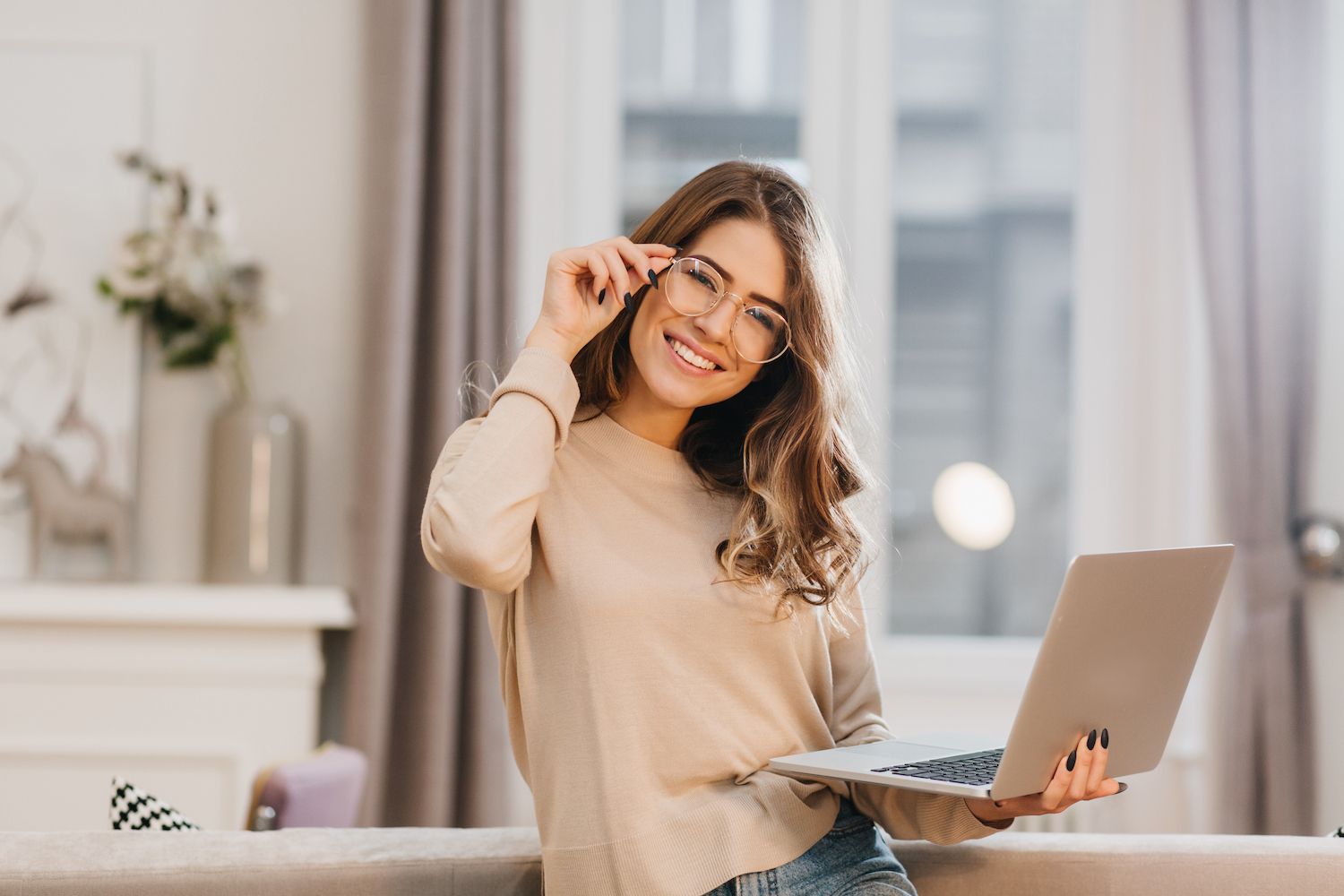
After you've logged into your account, you will receive an account token and put it as a header it will authenticate you and begin working. You can send a POST request to http://127.0.0.1:8000/api/login with the following body:
"email":"[email protected]",
"password":"password"
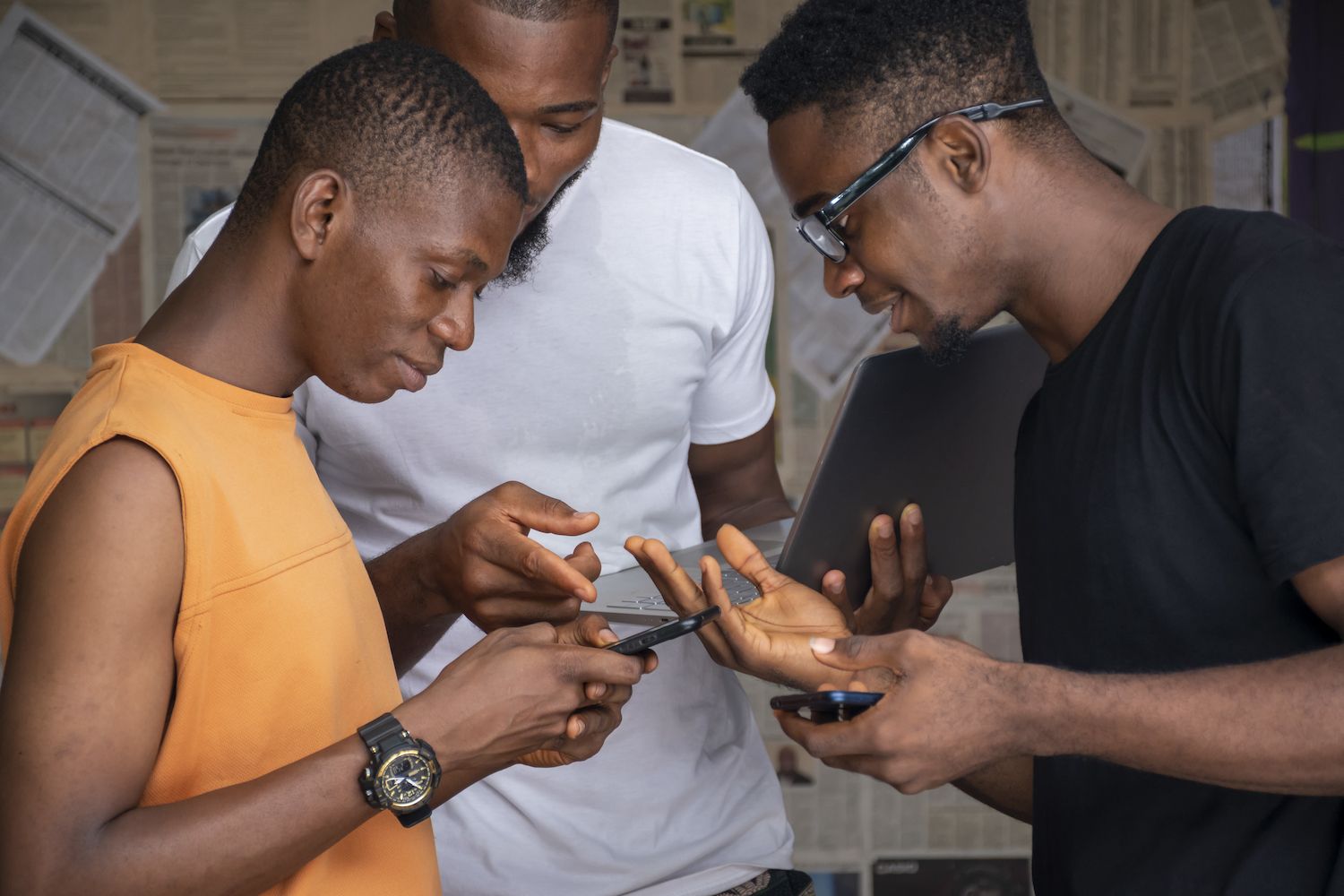
Utilize the token you obtained as a Bearer token, and then add it to the header for Authorization.
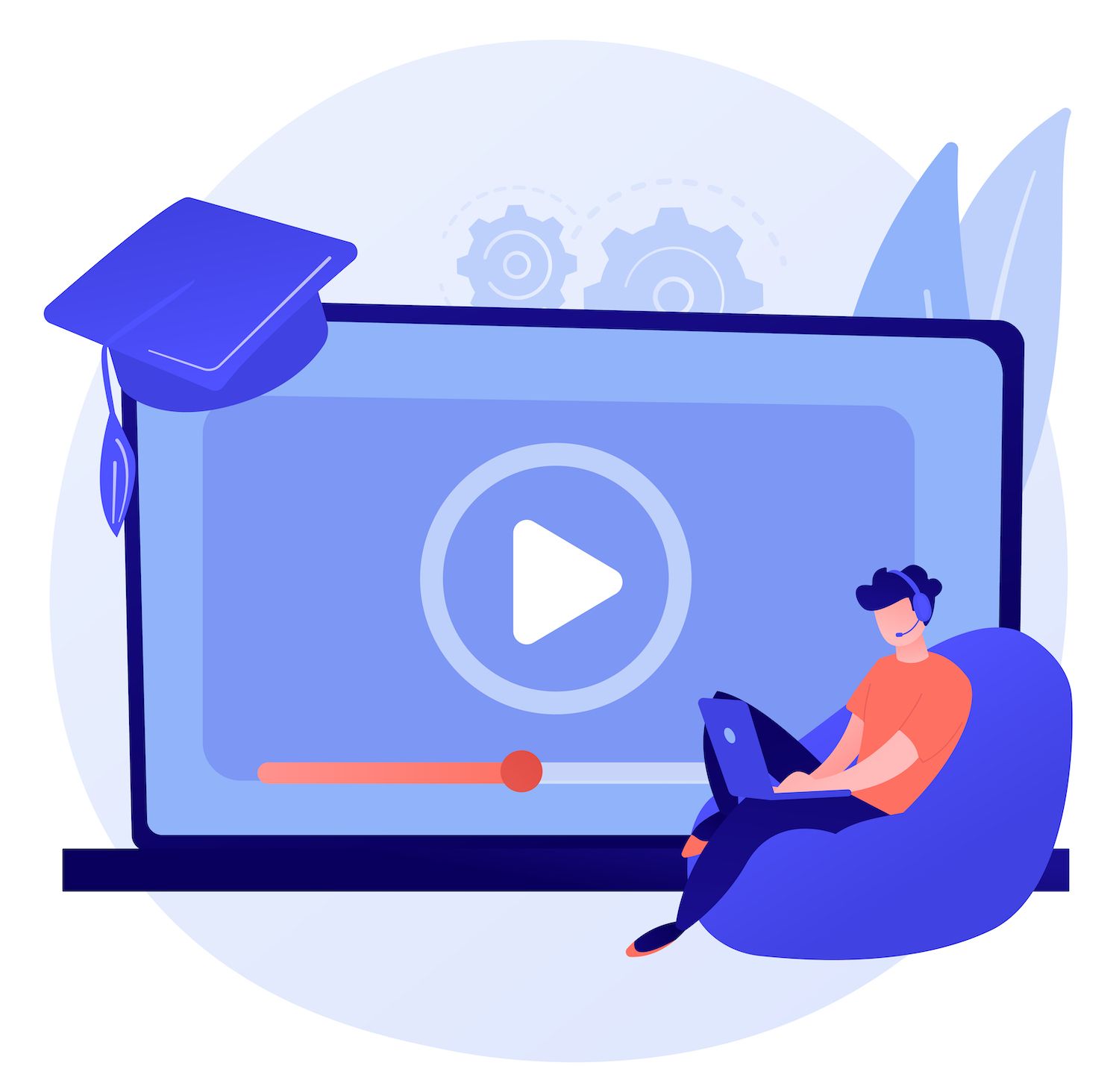
How to Handle API Errors
A status code alone is not enough. An error message that is human-readable is needed. Laravel has a number of options to address the issue. Try-catch block, fallback technique, or send a custom response. The following code you added to UserController UserController
shows this.
if (!$user || !Hash::check($request->password, $user->password))
return response([
'message' => ['These credentials do not match our records.'] ], 404);
Summary
The Laravel Eloquent Model makes it effortless to design, verify, and test APIs. The mapping of objects provides easy way to interact with databases.
Additionally, by acting as middleware, the token of Sanctum from Laravel helps you to protect your APIs quickly.
- Easy setup and management in the My dashboard
- 24/7 expert support
- The best Google Cloud Platform hardware and network, powered by Kubernetes for maximum scalability
- A high-end Cloudflare integration to speed up and security
- Reaching a global audience with up to 35 data centers as well as more than 275 PoPs across the globe