Learning to master Laravel Routes
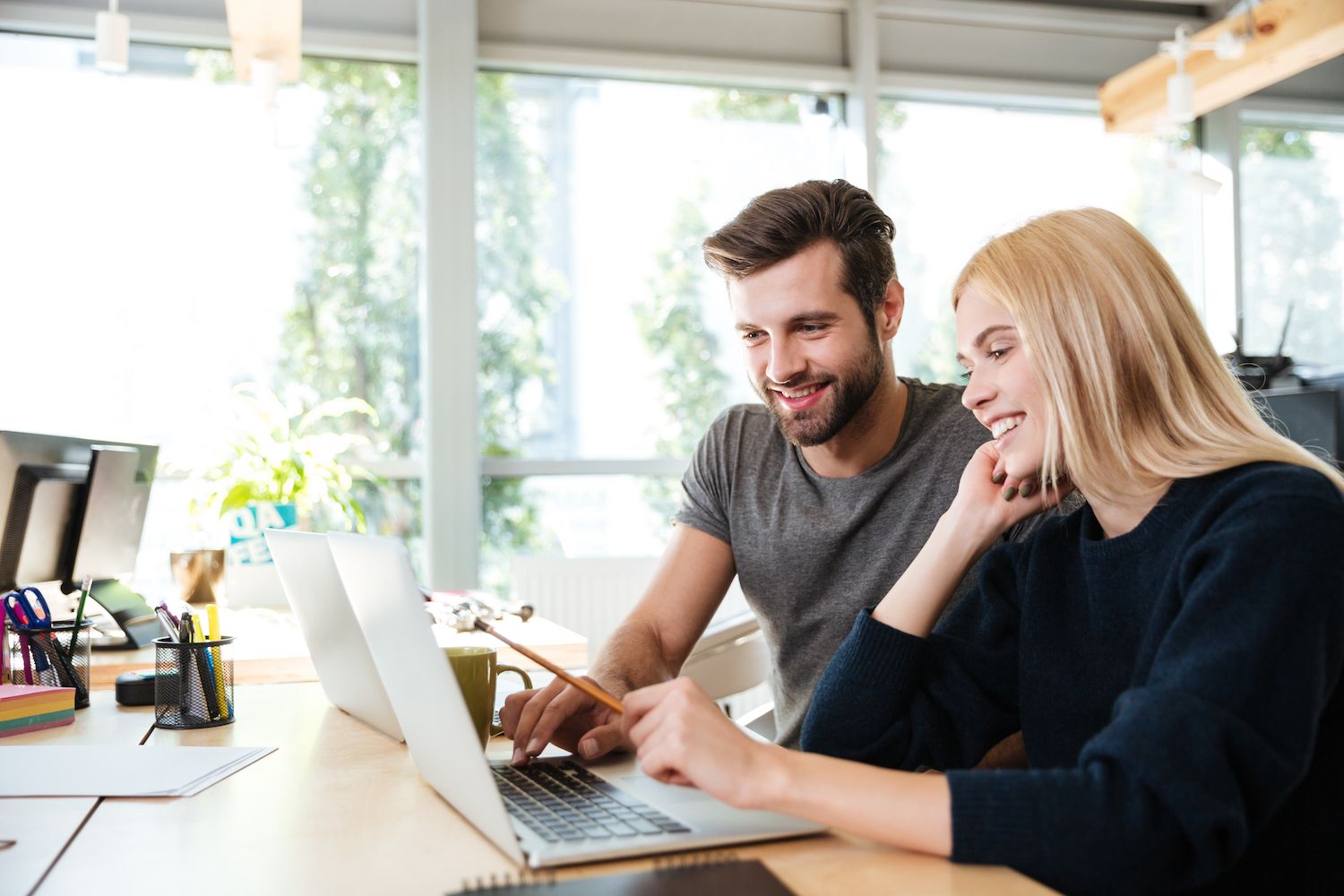
If we look at the backend, we eventually meet routes. This can be regarded as the backbone of the backend since each request that the server gets is directed to a controller via the routing list, which connects requests to the controller or action.
Backend Routing and Cross-Site Scripting in Laravel
On a server there exist the private and public routes. Public routes could pose a risk for XSS.
It's a problem that occurs because a user may be diverted to a destination that doesn't require a session token to one that does, and have access without the token.
One way to solve the issue is to implement an entirely new HTTP header and add "referrer" in the path in order to avoid this situation.
'main' => [
'path' => '/main',
'referrer' => 'required,refresh-empty',
'target' => Controller\DashboardController::class . '::mainAction'
]
Laravel Basic Routing
In Laravel, routes are created inside the web.php and api.php files, and Laravel includes two routes by default, one for the WEB as well as one that is for API. API.
These routes reside in the routes/ folder, but they are loaded in the Providers/RouteServiceProvider.php
Instead of doing this we can load the routes directly inside the RouteServiceProvider.php skipping the routes/ folder altogether.
Redirects
// Simple redirect
Route::redirect("/class", "/myClass");
// Redirect with custom status
Route::redirect("/home", "/office", 305);
// Route redirect with 301 status code
Route::permanentRedirect("/home", "office");
Inside the redirect routes we are forbidden to use the "destination" as well as "status" keywords for parameters as they are protected by Laravel.
// Illegal to use
Route::redirect("/home", "/office/status");
Views
Views are the . blade.php files that we use to render the frontend for our Laravel application. They use the blade templating engine. it is the default way to create a full stack application with just Laravel.
If our route is to will return a view you can use the view method on the Route facade. It accepts a route parameter, a view name, as well as an list of value to be sent to the view.
// When the user will enter the 'my-domain.com/homepage
// the homepage.blade.php file will be rendered
Route::view("/homepage", "homepage");
// Let's assume our view wants to say "Hello, name",
// by passing an optional array with that parameter
// we can do just that and if the parameter is missing but
// it is required in the view, the request will fail and throw an error
Route::view('/homepage', 'homepage', ['name' => ""]);
Route Liste
When the application grows in size, so does the amount of requests that must be directed. And with a great volume of data comes a lot of confusion.
That's where the art route:list command is able to help. It provides an overview of the various routes that are defined in the application, their middlewares and controllers.
php artisan route:list
The program will show a listing of all possible routes, without middlewares. For this, we have to utilize the flag with '-v'
php artisan route:list -v
In a situation where you may be employing Domain Driven Design and your routes could have specific names, you could make use of the filters available with this command.
php artisan route:list -path=api/account
This will show only the routes that start with API/Account..
On the other hand you can tell Laravel to exclude or include the third party defined routes using options like the -except-vendor / -only-vendor choices.
Route Parameters
Sometimes we will have to capture specific portions of the URI with the route, for example, a userID or token. We can do so by defining a route parameter, which is always encased within the ' ' braces and should only contain alphabetic characters.
If our routes include dependencies in the callbacks, the Laravel Service Container will automatically add them.
use Illuminate\Http\Request;
use Controllers/DashboardController;
Route::post('/dashboard/id, function (Request $request, string $id)
return 'User:' . $id;
Route::get('/dashboard/id, DashboardController.php);
Mandatory Parameters
The parameters that are required are those of the call that we are not allowed to bypass when making an attempt to call. If we do the error could be raised.
Route::post("/gdpr/userId", GetGdprDataController.php");
Now inside the GetGdprDataController.php we will have direct access to the $userId parameter.
public function __invoke(int $userId)
// Use the userId that we received...
A route could have any numbers of parameters and they are added to route callbacks / controllers according to their order of entry:
Are you interested in knowing what we did to increase our visitors by 1000 per cent?
Join the 20,000+ who receive our weekly newsletter that contains insider WordPress tips!
// api.php
Route::post('/gdpr/userId/userName/userAge', GetGdprDataController.php);
// GetGdprDataController.php
public function __invoke(int $userId, string $userName, int $userAge)
// Use the parameters...
Optional Parameters
In a situation where we want to do something within a specific route only if a parameter is present and nothing otherwise without impacting the whole application or the entire application, we could create an optional parameter.
These are denoted by the letter '?' appended to these.
Route::get('/user/>' (function (int age = null) *if (!$age) Log::info("User doesn't have the age of");
else Log::info("User's Age is " . $age);
Route::get('/user/"; method (int"$name" is "John Doe") Log::info("John Doe")Log::info("User's name begins with " . $name);
Route Wildcard
"Where Method "where method" accepts the parameter's name as well as the regex rule which is used for the verification. By default, it takes the first parameter, but if we have many options, it is possible to pass an array that contains names of parameters as keys, and the rule's value. Laravel will sort them out to us.
Route::get('/user/age', function (int $age)
//
->where('age', '[0-9]+');
Route::get('/user/age', function (int $age)
//
->where('[0-9]+');
Route::get('/user/age/name', function (int $age, string $name)
//
->where(['age' => '[0-9]+', 'name' => '[a-z][A-z]+');
We can take this a step further by applying a validation on all the routes in our application by employing the pattern method on the Route facade
Route::pattern('id', '[0-9]+');
It will cause every ID parameter validated using this regex expression. Once we have defined it, it is automatically applied to all possible routes that have that name.
Route::get('/find/query', function ($query)
//
)->where('query', , '. *');
Named Routes
How Do You Create Named Routes
A simple way to do this is through the name method chained on the Route facade. Names should be unique.
Route::get('/', function ()
)->name("homepage");
Route Groups
Route groups permit you to share route attributes like middlewares on a variety of routes, without having to define it for each and every route.
Middleware
When assigning a middleware to any of the methods, we must include them in a group first using groups method. Another thing to take into consideration is the fact that middlewares will be performed in the same order as they are added in the group.
Route:middleware(['AuthMiddleware', 'SessionMiddleware'])->group(function ()
Route::get('/', function() );
Route::post('/upload-picture', function () );
);
Controllers
When a group utilizes the same controller, it is possible to use the controller method to identify the common controller for each route within the same group. Now we have to specify what method the route will use to call.
Route::controller(UserController::class)->group(function ()
Route::get('/orders/userId', 'getOrders');
Route::post('/order/id', 'postOrder');
);
Subdomain Routing
The routes can be utilized for subdomain routing. We could capture the domain, as well as some of the subdomain to be used for our controller as well as routes. With the help of the domain method on the Route façade, we can classify the routes we use under a domain:
Route::domain('store.enterprise.com')->group(function()
Route::get('order/id', function (Account $account, string $id)
// Your Code
);
Name Prefixes and Prefixes
Whenever we have a group of routes, instead of changing them all at once, we can make use of the utils provided by Laravel provides, such as the name and prefix on the Route façade.
Prefix methods can be employed to prefix every routes in the group by the given URI as well as the name method may be used to prefix every route's name using a specific number of characters.
This allows us to create something like Admin routes without having to alter each and every name or prefix in order to distinguish them.
Route::name('admin. ")->group(function()
Route::prefix("admin")->group(function()
Route::get('/get')->name('get');
Route::put('/put')->name(put');
Route::post('/post')->name('post');
);
);
In the future, the URI for these routes will be admin/get and admin/put. as well as the names admin.get, admin.put, admin.post.
Route Caching
What Is Route Caching?
Route caching will decrease the time required to record all application routes.
Running php artisan route:cache an instance of Illuminate/Routing/RouteCollection is generated and after being encoded, the serialized output is written to bootstrap/cache.routes.php.
The reason it's important to use Route Caching
By not using the feature of route cache that Laravel has, we're slowing down our website more than it needs to be, and reducing the user's retention as well as overall enjoyment the website.
Based on the size of the project and the amount of routes In the case of a single command, it can speed up your application by 1.3x times up to 5x times.
Summary
Routing is the backbone of Backend development. Laravel excels at this through its lucid method of managing and defining routes.
- Easy setup and management in My dashboard. My dashboard
- Support is available 24/7.
- The most efficient Google Cloud Platform hardware and network, that is powered by Kubernetes for the highest scalability
- Enterprise-level Cloudflare integration that improves speed as well as security
- Global audience reach with as many as 35 data centers as well as more than 275 PoPs in the world.