Logging slow queries with Active Support Notifications (r)
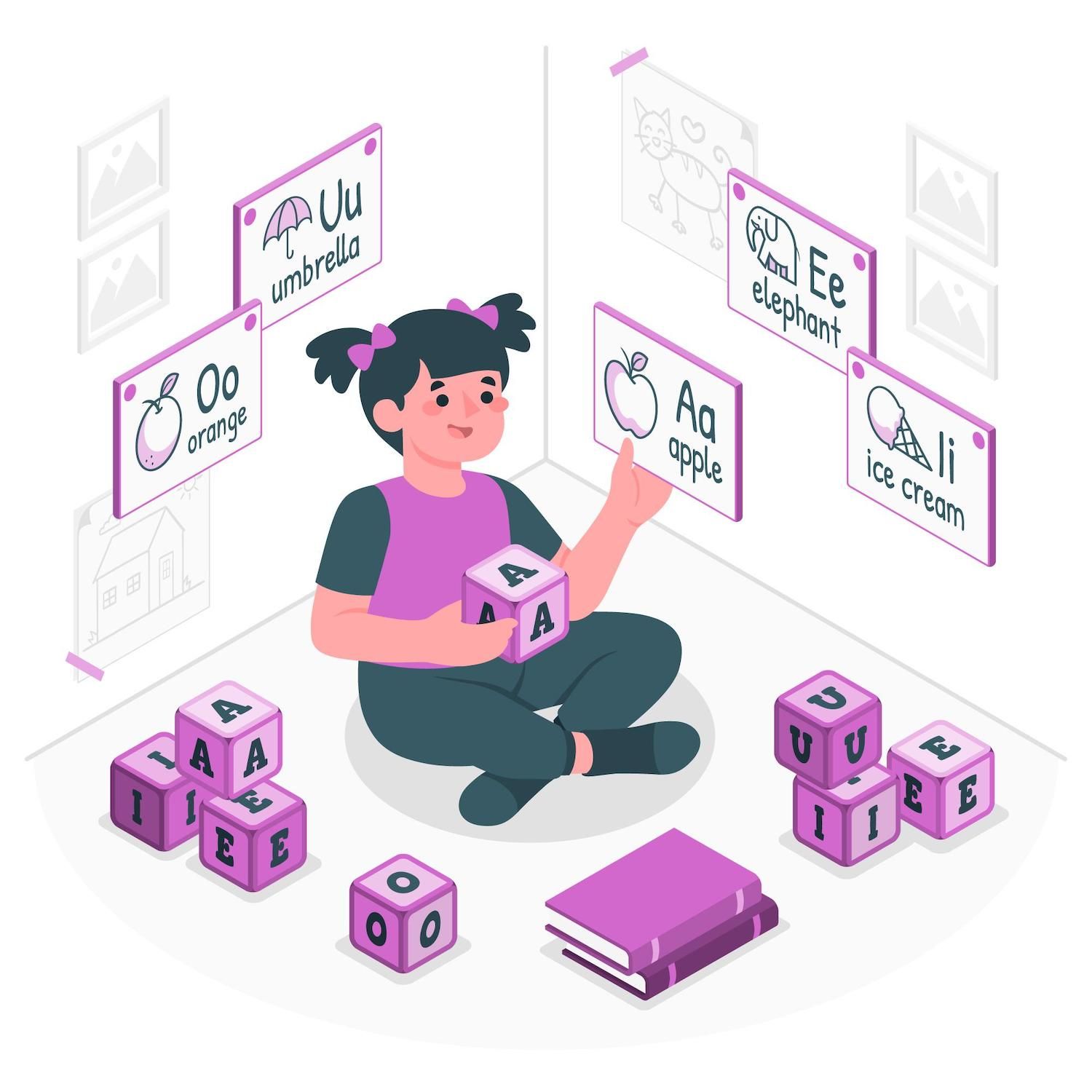
-sidebar-toc>
This article will demonstrate how to utilize Active Support Notifications to record slow queries in the Rails application. In addition, we'll provide advice on how to analyze the slow query logs in order to find and address performance issues.
What's a slow question?
A slow query is an application that takes more than a specified time to execute. What constitutes slow queries can differ depending on the application and its performance requirements. A good standard is to judge any query taking more than 100 milliseconds as slow.
Why is it necessary to log slow queries?
There are many reasons why it's important, and the reason it is important to record slow queries.
First, slow queries can be a sign of underlying performance problems in your application. If you record slow queries, you'll be able to spot these issues quickly and then take action to correct these issues. Prevention is more effective than treating.
Third, slow queries may aid in improving your application's performance.
By analyzing slow query logs, you can identify the queries that are causing the most performance problems and make steps to optimize these. You can record slower queries within our application using Active Support Notifications.
What are Active Support Notifications?
Understanding their functions and effectively utilizing them, you can significantly improve the performance, maintainability, and overall health of your Rails app.
This article provides an outline of Active Support Notifications and their key benefits.
Functionality
- Instrumentation: You can instrument specific events in your Rails application using the ActiveSupport::Notifications.instrument method. This method requires the name of the user, along with the payload (optional information), and an element of code that needs to be executed.
- Payload: It allows users to send additional data that is relevant to the event monitored. It could be things such as timestamps, user IDs, data query information for databases and any other pertinent information.
- Subscribers When the block is executed, Active Support sends a message with the user's name as well as the payload to all registered subscribers. Subscribers can examine the message and perform specific actions in response to the event.
Benefits
- Performance monitoring: By instrumenting important events, you are able to monitor their time to execution and identify performance bottlenecks within the application. This helps you improve your application's code as well as improve the overall performance of your Rails application.
- Debugging: Notifications may provide valuable insights into the operation of your application and assist in identifying issues. Data from the payload can indicate unanticipated behavior or issues during runtime.
- Custom instrumentation: You can extend the functionality that comes with Active Support Notifications by creating your own instruments that you can customize and subscribers. This lets you track certain events specific to your specific application's needs.
- Integration with other libraries: A number of well-known Rails libraries, including Action Cable and ActiveJob, leverage Active Support Notifications to provide performance data and event tracking capabilities.
How can you record slow queries by using Active Support Notifications
Active Support Notifications is a built-in Rails mechanism for logging instances that happen within the application. To use Active Support Notifications to log slow queries, the example code below would be placed in an initializer (e.g., config/initializers/error_notifications.rb
) to ensure it runs on application startup. Alternatively, add the code into a particular model or controller to enable targeted logging.
config.active_support.Notifications.subscribe('sql.active_record') do |*args|
event = ActiveSupport::Notifications::Event.new(*args)
if event.duration > 100
Rails.logger.info "Slow query: #event.payload[:sql]"
end
end
This code will log all SQL queries that require more than 100 milliseconds.
The logged information will include details of the SQL statement, the time of the query, and the names of the files and the line number on which the query was executed.
How can you analyze query slow logs
Once you have started logging slow queries, you can look over the logs and identify the queries that are causing the biggest performance issues. There are many methods to analyse the logs of slow queries.
One way is to use tools similar to the Rails Performance Dashboard. Its Rails Performance Dashboard offers a visual interface for analyzing and viewing the slowness of query logs.
Another option to study slow query logs is to use a text editor or spreadsheet program. It is possible to use a text editor or spreadsheet program to filter and sort slow query logs by length, SQL statement, or file name.
This will help you pinpoint those queries that cause the greatest issues with performance.
What can you do to speed up your queries?
After you've identified those queries causing the most performance problems, you can take steps to fix the issue. There are many methods to improve the performance of your queries.
One way is to optimize one way is to optimize the SQL statement. The ability to optimize your SQL statement using indexes, adding constraints, or using more efficient query methods.
The implementation of pagination and breaking up large results sets into smaller pages can improve the performance of your system and help reduce memory use.
Also, consider running Background Jobs and offloading long-running queries to background jobs (e.g. with Sidekiq, Resque) to block web requests.
Summary
Active Support Notifications is an inbuilt Rails feature to log slow queries. Logging slow queries is an important element of the performance tuning process for every Rails application. Through logging these queries it is possible to spot issues with performance early and make steps to address the issue.
It is possible to use Active Support Notifications to log slow queries. Then, you can examine the logs to determine those queries that cause the greatest performance issues. Once you have identified the ones that cause the most performance problems, you can take steps to fix them.
If you're operating a well-established business and have the capacity to incorporate junior developers into teams, this type of efficiency will help them in establishing better methods through participation with the work. In addition, it will help with the team that monitors and senior members and collaborating to create a stronger and performant platform. Give your slow query project to a junior developer, and they will benefit tremendously by it.
Don't let the issue to exist. Be proactive and prevent slow queries before they can slow your performance. Take a proactive approach, not reactive!
Lee Sheppard
Lee is an Agile certified full stack Ruby on Rails developer. Over six years in the tech industry he enjoys teaching, coaching Agile and guiding others. Lee also speaks in tech related conferences as well as has an education in graphic design and illustration.