Master state management with Vue.js using Pinia - (r)
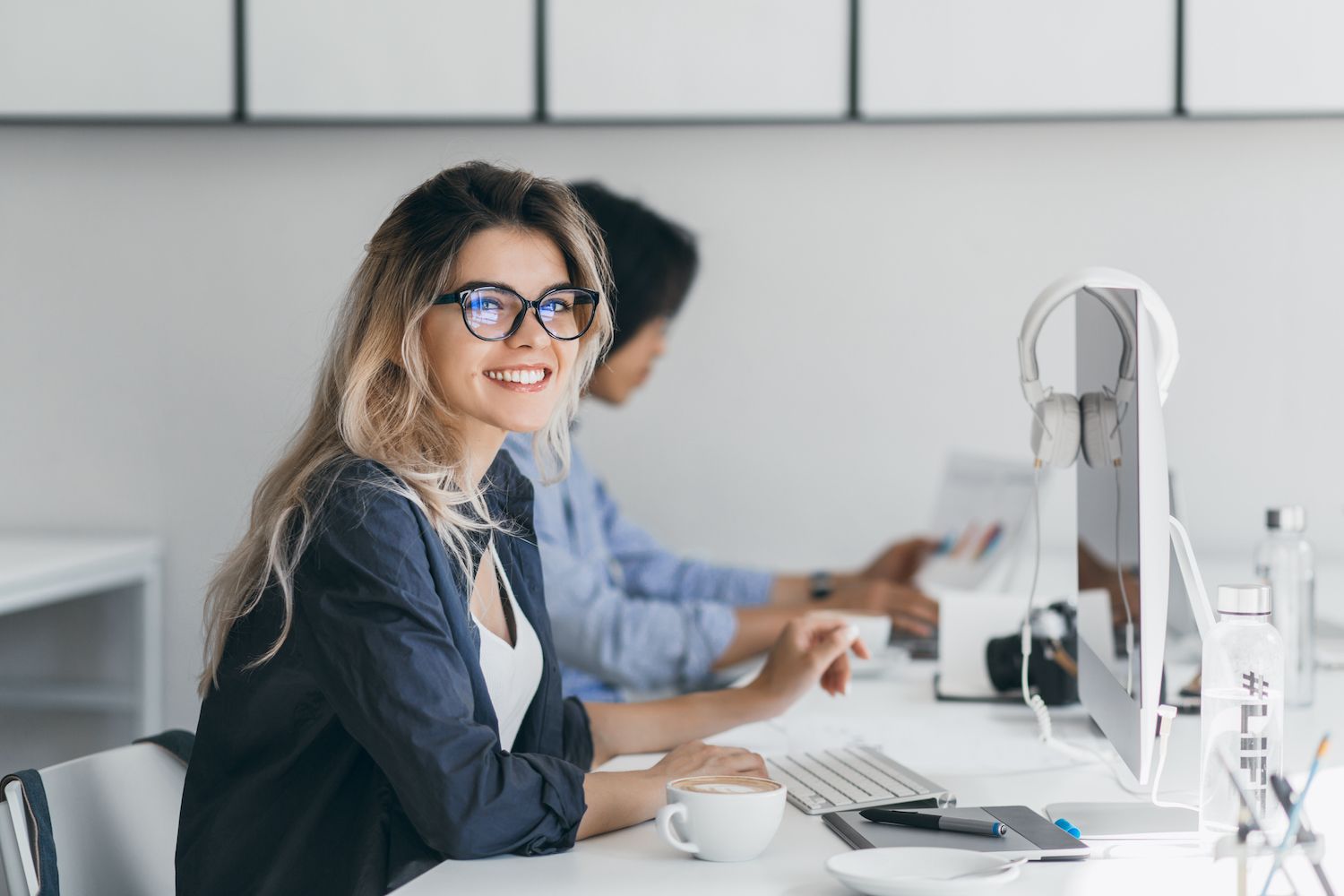
-sidebar-toc>
In Vue, state management has been a staple of Vuex which is a library that provides a centralized store of all the components used by an application. However, recent advancements within the Vue framework have led to Vuex's successor Pinia.
Pinia provides a lighter, modular, and intuitive management approach. It seamlessly integrates with Vue's Reactivity System and Composition API which makes it easy for developers to control and manage shared data in a flexible and manageable manner.
The scene is set: Pinia vs Vuex
A popular library to use for state management in Vue application, Vuex provided a centralized storage for all of an application's components. With the development in Vue, Pinia represents a more modern option. It is worth examining what makes it different from Vuex.
- API variations The HTML0 API has some differences the Pinia Composition API offers an easier and more intuitive API as compared to Vuex, which makes it more simple to handle the application's state. Additionally, its design resembles Vue's Options API, familiar to many Vue developers.
- Typing support -- The past has seen a lot of Vue developers have been frustrated by the limited support for type in Vuex. Contrarily, Pinia is a fully managed by type which eliminates the concerns. Its type safety helps prevent any runtime error, helps to code readability, and helps facilitate smoother scaling capabilities.
- Reactivity technology -- Both libraries use Vue's reactivity system, but Pinia's approach aligns more closely in line with Vue 3's Composition API. Although the API for reactivity is effective, managing the complexity of states in large applications can be challenging. The simple and adaptable architecture alleviates the burden of managing state in your Vue 3 applications. Store patterns in Pinia let users define a storage space for managing a specific portion of the application state, simplifying its organization and sharing it across components.
- Its lightweight design -- With just 1 KB, Pinia integrates seamlessly into your dev environment, and its light weight can improve your application performance and load times.
How do you set up an Vue project with Pinia
In order to integrate Pinia within the Vue project, initialize your project with Vue CLI and Vite. Once the project is initialized, you can install Pinia using npm or yarn as dependencies.
- Create your own Vue project by using Vue CLI or Vite. Then, follow the prompts to set up your project.
// Using Vue CLI
vue create my-vue-ap
// Using Vite
npm create vite@latest my-vue-app -- --template vue
- Switch your directory to the new project folder:
Cd my-vue app
- Install Pinia as a dependency on your project.
// Using npm
npm install pinia
// Using yarn
yarn add pinia
- The main entry file (usually main.js or main.ts ), import Pinia and tell Vue to use the following:
import createApp from 'vue';
import createPinia from 'pinia';
import App from './App.vue';
const app = createApp(App);
app.use(createPinia());
app.mount('#app');
With Pinia installed and set up inside the Vue project, you're now ready to designate and implement the state-management stores.
How to create a store in Pinia
The store forms the basis of state management in the pinia powered Vue application. It assists you in managing application-wide data in a cohesive and coordinated manner. The store is where you decide to define the data, manage it, and store the information you want to share with all the components of your application.
This centralization is critical because it organizes the state of your application and manages changes, making data flow easier to predict and analyze.
Moreover, a store in Pinia does more than hold the state of things: its included features allow you to modify the state via actions and determine derived states using getters. These built-in capabilities help to create a more efficient and maintainable codebase.
The following illustration shows how to build the basic Pinia store in a projects src/store.js file.
import defineStore from 'pinia';export const useStore = defineStore('main', state: () => (count: 0,),
actions: thegetters:
doubleCount: (state) => state.count * 2,
,
);
What is the best way to get access to state stored in the components
Comparatively with Vuex, Pinia's approach to state access and management is easier to understand, particularly when you're already familiar with Vue 3's Composition API. This API is a set of APIs that allow the inclusion of reactive and composable functions in your component.
Look at the code.
>
import store from './store' and export the
The store
function gives access to the Pinia store, so you download it from store.js using import useStore from './store';
.
As a feature of Vue3's Composition API, the setting
function specifies your component's state of reactive and its function. Inside this function, you use utilizeStore()
to access the Pinia store.
Following, const count = store.count
accesses the store's count
property and makes it accessible in the component.
Then, setup
returns the count
and allows the template to display it. Vue's reactivity system means that your component's template changes the value of count
when it is changed in the store.
Below is a screen shot of the results.
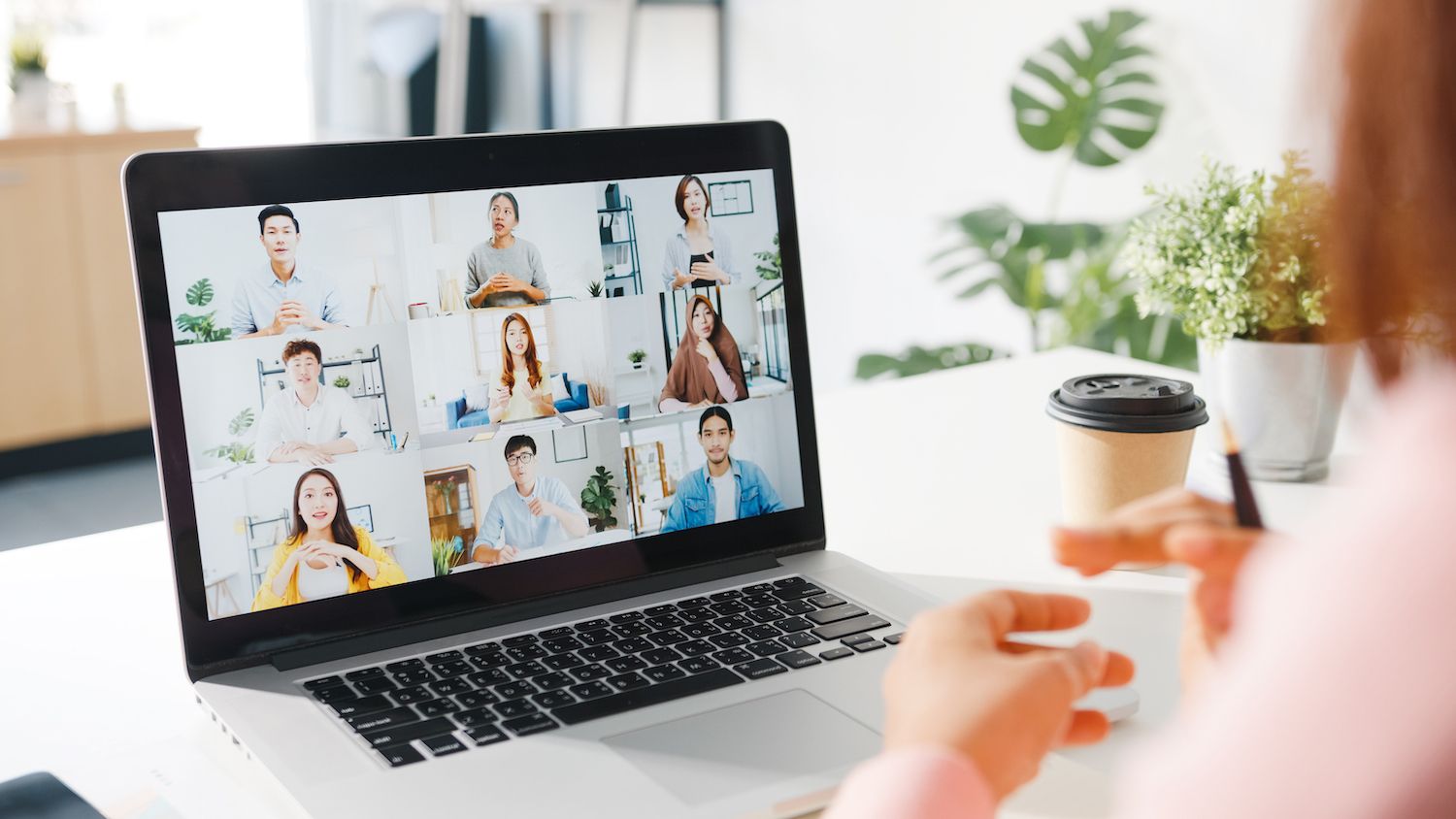
This illustration illustrates the advantages of Pinia:
- Simple It is Pinia provides direct access to the store's state without mapping functions. Contrarily, Vuex needs
mapState
(or similar tools) for the same access. - Direct store access -- Pinia lets you access state properties (like
store.count
) in a direct manner, which makes the code more understandable and understandable. Meanwhile, Vuex often requires getters to get access to even the most basic properties. This adds complexity and hinders understanding.
Modify state using Pinia
In Pinia it is possible to alter an item's state by taking actions that are more flexible than Vuex mutations. Think about the function below that increments an state's count
property:
store.increment() // Increases the count
On the other hand, a Vuex equivalent involves the creation of a mutation that is in addition to at least one of the following actions:
action and
The Pinia action and its equivalent Vuex code illustrate a significant difference between the libraries' complexity of their code. We'll explore the differences in more detail:
- Direct or indirect state modification -- As the
increment
actions demonstrates, Pinia actions directly mutate the store's state. In Vuex it is possible to change the state using mutations, which you must commit with actions. This separation of the process ensures that changes to state can be tracked however, it's more complicated and rigid than similar Pinia actions. - Asynchronous and synchronous processes While Vuex mutations remain synchronous and cannot contain asynchronous processes, Pinia actions can contain both synchronous and asynchronous codes. This means that you can perform API calls and other synchronous actions directly inside actions, making for a leaner and more concise code base.
- Simple syntax - Simplified syntax - Vuex typically requires the definition of changes and the steps to make them happen. Pinia eliminates this need. Its ability to alter the state within actions reduces boilerplate code and keeps your current code simple. In Vuex simple state updates require defining an action and a mutation, even if the act is simply performing the mutation.
The transition to Vuex to Pinia
Transitioning to Pinia could bring numerous advantages in terms of simplicity, flexibility and maintainability, but it requires thoughtful planning and consideration in order for a successful transition.
Before making the switch, make sure you
- Be familiar with the distinctions between Pinia's and Vuex's architecture states management patterns and APIs. It is important to know these differences in order to refactor your code to take full benefit of the features offered by Pinia.
- Refactor and analyze your Vuex states, actions change, and getters to fit into Pinia's structure. Remember, in Pinia it is possible to define state as a function. You can directly change states through actions and can implement getters more smoothly.
- Consider how you can transition to Vuex module store. Pinia uses modules not exactly the same way as Vuex, but you can organize your store to fulfill similar functions.
- Review your testing methods to adapt to changes in state management. This process might involve updating how you mock stores or actions in your unit and integration tests.
- Take note of how the change will affect the project's structure and organizational system. Be aware of things like name conventions, and how you integrate and manage stores across elements.
- Make sure that the library is compatible with other Libraries. Ensure compatibility with the other. Check for any required updates or dependency changes which could impact.
- Evaluate any performance changes. Pinia is generally more lightweight than Vuex however, it is important to continue observing your application's performance during and following the change to be sure that there aren't issues.
Converting a store that was created on Vuex to Pinia involves several steps to adapt their structure and API. Think about the Pinia store which was previously.
The store that is identical in a Vuex store.js file appears as follows.
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Store(
state:
count: 0,
,
mutations:
increment(state)
state.count++;
,
,
actions:
increment(context)
context.commit('increment');
,
,
getters:
doubleCount(state)
return state.count * 2;
,
,
);
Like the Pinia store as well, this Vuex instance contains an state
object, which has only a count
property, which is set at the value of 0
.
The mutations
object has methods that directly mutate the state, and the actions
object's methods commit the increment
transformation.
The getters
object contains the doubleCount
method that multiply each number
condition by two
and returns the result.
The code in this example demonstrates that implementing a store in Pinia involves several noticeable differences from Vuex:
- Initialization -- Pinia doesn't require
Vue.use()
. - Structure -- In Pinia the state, it is a function which returns an object, allowing for better TypeScript support as well as reactivity.
- Actions -- Actions in Pinia are techniques that direct mutate the state without needing changes, which simplifies the programming.
- Getters Getters HTML0Although similar to Vuex Getters in Pinia are defined within the definition of the store and are able to directly connect to the state.
How do you use the store's components in component stores?
If you are using Vuex You can make use of the store in this way:
import mapGetters, mapActions from 'vuex';
export default
computed:
...mapGetters(['doubleCount']),
,
methods:
...mapActions(['increment']),
,
;
To use Pinia this is the case, and it's a matter of:
>
import useStore from '/src/store';
export default
setup()
const store = useStore();
return
store,
;
,
;
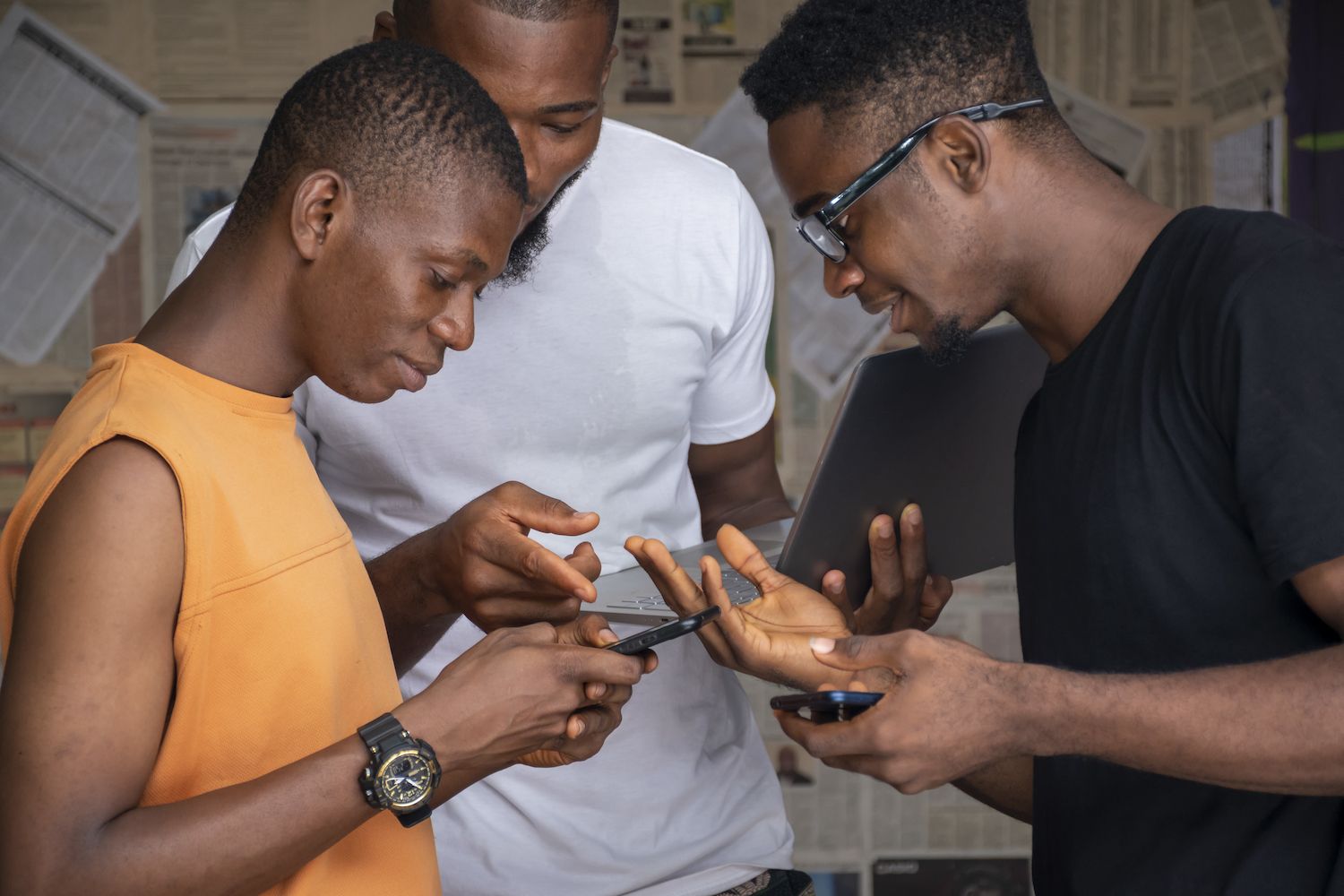
This example covers a basic conversion. If you want to convert more complicated Vuex stores, especially ones that use modules, the conversion could be more extensive in its overhaul to conform to the architecture of Pinia.
How to deploy your Vue application
Create the Dockerfile at your project's root and then paste the following contents:
From node:latest WorkDIR /app
Copy package*.json .Run NPM installCOPIY ./ . CMD ["npm", "run", "start"]
- Sign in or create an account to see Your dashboard. My dashboard.
- Authorize your Git service provider.
- Click on the Application on the left sidebar and click the "Add App" button.
- In the modal that appears in the modal, select the repository you want to deploy. If you have more than one branch, select the branch that you wish to use and assign a name the application.
- Pick one of the data center locations.
- Choose the build environment you prefer and choose the Dockerfile option to set the container image.
- If your Dockerfile isn't in your repo's root folder, click Context to identify its location and then click Proceed.
- You may just leave the start command field empty. Uses
npm start
to begin your program. - Select the pod size and the number of times that best suit your needs and then click Continue.
- Complete the details of your credit card and click Create Application.
Summary
The shift to Vuex to Pinia is a major change in state management inside the Vue ecosystem. Pinia's ease of use, enhanced TypeScript support, and its alignment with Vue 3.0's Composition API make it a an ideal choice for the contemporary Vue applications.
Jeremy Holcombe
Content and Marketing Editor at , WordPress Web Developer, and Content Writer. In addition to all things WordPress, I enjoy playing golf, at the beach and watching movies. Also, I have height difficulties ;).