Mastering React Conditional Rendering: A Deep Dive - (r)
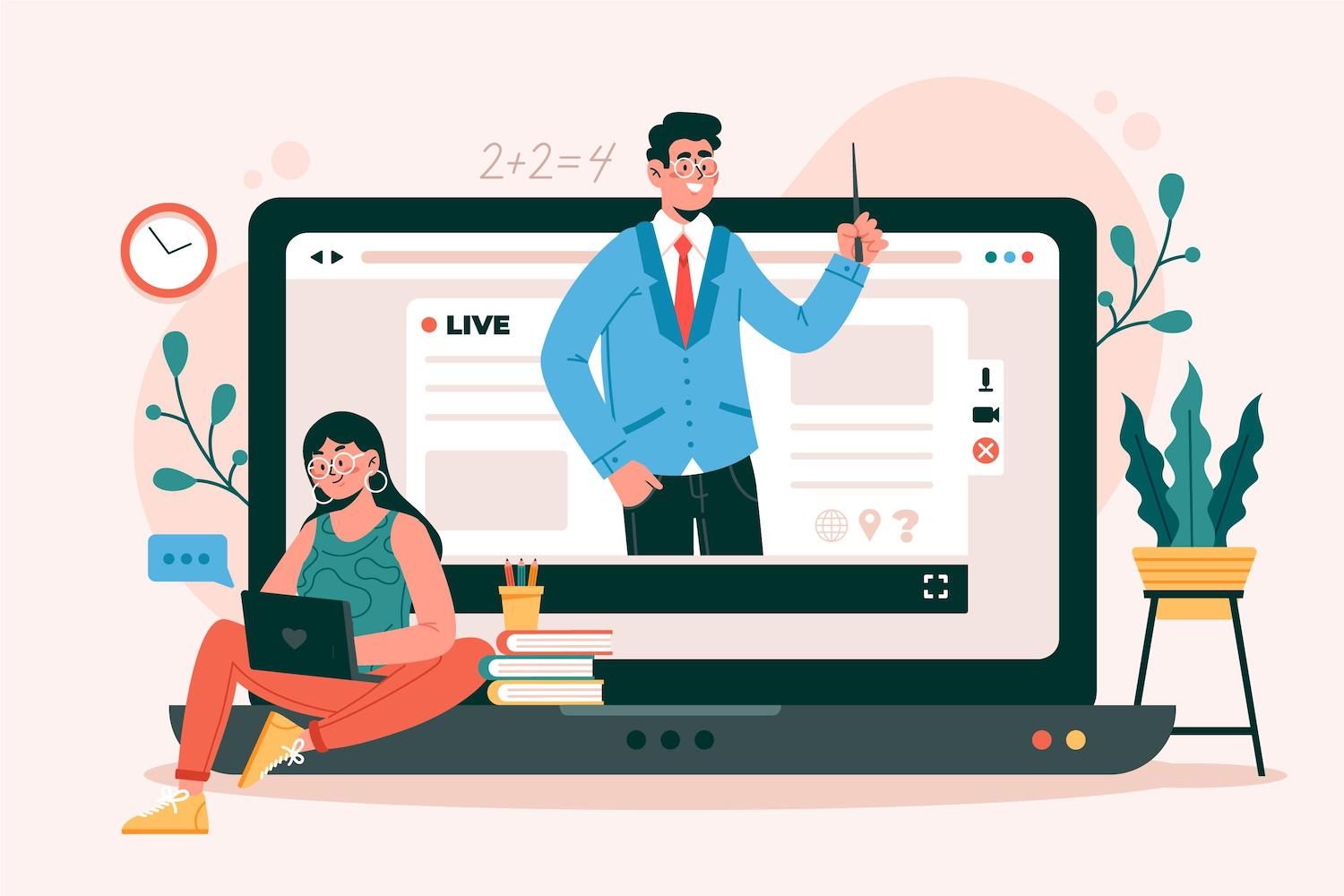
Share this on
Conditional rendering is a powerful feature in React which allows programmers to render components based on certain circumstances.
It's an essential notion that plays a vital role in building websites that are dynamic and interactive.
In this comprehensive guide, we will dive deep into the world of conditional rendering with React by covering both the basics and advanced methods, with examples for proper understanding.
Understanding Conditional Rendering in React
This can be extremely useful in scenarios where you want to display or block specific UI elements, change the layout of a page or display different types of pages based on the user's interactions.
Conditional rendering is essential for React applications since it allows the creation of interactive and dynamic user interfaces that respond to changes in data or users' interactions in real time.
The Basics of Conditional Rendering
There are a variety of basic methods that you can use for conditional rendering within React. We'll look into each in detail.
Using the if Statement for conditional rendering
One of the easiest methods to use conditional rendering within React is to use the standard if
statement.
if (condition)
return Expression 1;
else
return Expression 2;
Its if
statement is a good option inside your component's render()
method to conditionally render content based on an underlying specific.
For example, you can make use of the if statement in order to show a spinning spinner in the meantime of waiting for data to load:
import useState, useEffect from 'react';
import Spinner from './Spinner';
const MyComponent = () =>
const [isLoading, setIsLoading] = useState(true);
const [data, setData] = useState(null);
useEffect(() =>
// Fetch data from an API
fetch('https://example.com/data')
.then((response) => response.json())
.then((data) =>
setData(data);
setIsLoading(false);
);
, []);
if (isLoading)
return ;
return /* Render the data here */;
;
export default MyComponent;
In this instance, MyComponent
fetches information from an API by using useEffect. utilizeEffect
hook. While waiting for the data to be loaded, we display a Spinner component by using the If
statement.
A different example is rendering as a fallback interface if an error occurs while rendering your component.
const MyComponent const MyComponent ( data ) •
export default MyComponent
In this example it is possible to use the MyComponent
which takes in a data
prop. If the data
prop is not true and we show an error message using the If
statement.
In addition, you can show various content to different users roles with the if
clause:
const MyComponent = ( user ) • Export default MyComponent;
In this example it is possible to create an MyComponent
that uses an person as a
prop. Depending on the user.role
property, we show different contents using the if
statement.
Utilizing the Ternary Operator to perform Conditional Rendering
A more concise method to implement conditional rendering with React is by using the operator ternary (?) inside JSX.
The ternary operator permits you to write a compact inline if-else by specifying 3 operands. The primary operand is called the condition, while the second and third operands represent the expressions. If the conditions are correct
then the first expression will be executed; otherwise, the second expression will be executed.
You can, for instance, render various components in the context of a prop:
import ComponentA from './ComponentA';
import ComponentB from './ComponentB';
const ExampleComponent = ( shouldRenderComponentA ) =>
return (
shouldRenderComponentA ? :
);
;
export default ExampleComponent;
In this code, we have an ExampleComponent
that takes a prop called shouldRenderComponentA
. The ternary operator is used to conditionally render either ComponentA
or ComponentB
based on the prop's value.
There is the option of rendering other text depending on a condition:
import useState from 'react';
const ExampleComponent = () =>
const [showMessage, setShowMessage] = useState(false);
return (
setShowMessage(!showMessage)>
showMessage ? 'Hide message' : 'Show message'
showMessage ? Hello, world! : null
);
;
export default ExampleComponent;
In this case, we use the ternary operator to render different text depending on the value of the showMessage
state. When the button is clicked, showMessage's value showMessage
can be toggled and text will be displayed or hidden accordingly.
You can also create a loading spinner as data is being fetched:
import useState, useEffect from 'react';
import Spinner from './Spinner';
const ExampleComponent = () =>
const [isLoading, setIsLoading] = useState(true);
const [data, setData] = useState(null);
useEffect(() =>
const fetchData = async () =>
const response = await fetch('https://jsonplaceholder.typicode.com/todos/1');
const jsonData = await response.json();
setData(jsonData);
setIsLoading(false);
;
fetchData();
, []);
return (
isLoading ? : data.title
);
;
export default ExampleComponent;
In this example it is used to use the ternary operator to render a loading spinner as the data is being downloaded from an API. After the data is fetched then we render the property title
property using the operators ternary.
Utilizing Logical AND/OR Operators to perform Conditional rendering
You can also utilize the and ( &&
) as well as OR ( ||
) operators to implement conditional rendering in React.
A logical AND operator permits you to render a component only if one of the conditions is met, whereas the logic OR operator allows the rendering of a component if either or both of the conditions are true.
These operators can be useful for situations where you need to define simple criteria that decide if a particular component ought to be rendered or not. As an example, if you need to render a button only if a form is valid then you could utilize the logic AND operator as follows: this:
import useState from 'react';
const FormComponent = () =>
const [formValues, setFormValues] = useState( username: "", password: "" );
const isFormValid = formValues.username && formValues.password;
const handleSubmit = (event) =>
event.preventDefault();
// Submit form data
;
return (
setFormValues( ...formValues, username: e.target.value )
/>
setFormValues( ...formValues, password: e.target.value )
/>
isFormValid && Submit
);
;
export default FormComponent;
In this example it's the FormComponent
which has a form with two input fields for username
as well as password
. We're using useState
hook to handle the values of the form and isFormValid
variable to determine if each field is filled with values. Utilizing the logical AND operator, (&&), we render the submit button only when the isFormValid variable
is the case. This makes sure that the button will only be enabled if the form is validated.
Similar to that, you could use also the OR operator to display an error message when data is still loading or an error message if there is an error:
import React, useEffect, useState from 'react';
const DataComponent = () =>
const [data, setData] = useState(null);
const [isLoading, setIsLoading] = useState(true);
const [errorMessage, setErrorMessage] = useState('');
useEffect(() => to fetch data, []);
return (
>ErrorMessage ? (
errorMessage
) : (
data.map((item) => (
item.name
))
)
>
);
;
export default DataComponent;
In this instance it is a DataComponent
collects data through an API using fetch and shows it as a list. We're using the useState
hook to manage the state of data, load state as well as the error message. Using the logic OR operator (||), we are able to display either a loading or error message if either of its conditions is true. The user sees a message indicating what is happening with the process of fetching data.
Using the logical AND as well as OR operators for conditional rendering in React is an efficient and understandable way to deal with basic conditions. But, you should utilize other strategies such as switches
declarations for more intricate reasoning.
Advanced Methods to Conditional Rendering
Conditional rendering in React can be more complex depending on the requirements of the application. These are advanced methods that can be used for conditional rendering for more complicated situations.
Utilizing Switch Statements for Conditional rendering
While if statements and ternary operators are common approaches for conditional rendering Sometimes, switching to a switch
statement is appropriate, especially when dealing with multiple conditions.
Here's an example:
import React from'react";const MyComponent = (userType) => =switch (userType)
case 'admin':
return Welcome, admin user!;
case 'user':
return Welcome, regular user!;
default:
return Please log in to continue.;
;
export default MyComponent;
In this program, a switch
statement is utilized for rendering content in accordance with the userType
prop conditionally. This method can prove useful when dealing with multiple conditions and can provide a better structured and understandable way to handle complicated algorithms.
Conditional Rendering With React Router
React Router can be described as a well-known application that manages routing for clients for React applications. React Router lets you render elements based on the current route conditionally.
Here's an example for implementing conditional rendering with React Router:
import useState from 'react';
import BrowserRouter as Router, Route, Switch from 'react-router-dom';
import Home from './components/Home';
import Login from './components/Login';
import Dashboard from './components/Dashboard';
import NotFound from './components/NotFound';
const App = () =>
const [isLoggedIn, setIsLoggedIn] = useState(false);
return (
isLoggedIn ? (
) : (
)
);
;
export default App;
In the code below in this example, we use the isLoggedIn
state to render one of the components, either the Dashboard
component only if the user is logged in, or the NotFound
component if the user is not logged in. Login component login
component changes its state of isLoggedIn
condition to be true
when users successfully sign in.
We are utilizing the component's children prop to pass in the Login
component as well as the setIsLoggedIn
function. This lets us add props to Login without having to specify it in the Login
component without specifying the the path
prop.
Summary
Conditional rendering is an effective method in React that allows you to change the user interface dynamically depending on various conditions.
With respect to the degree of sophistication the app's UI algorithm, you are able to select the method most suitable to your specific needs.
Be sure to keep your code neat, tidy, and readable, and always thoroughly check your conditional rendering algorithm to verify that it functions as expected in different scenarios.