Mastering React Conditional rendering Deep Dive (r)
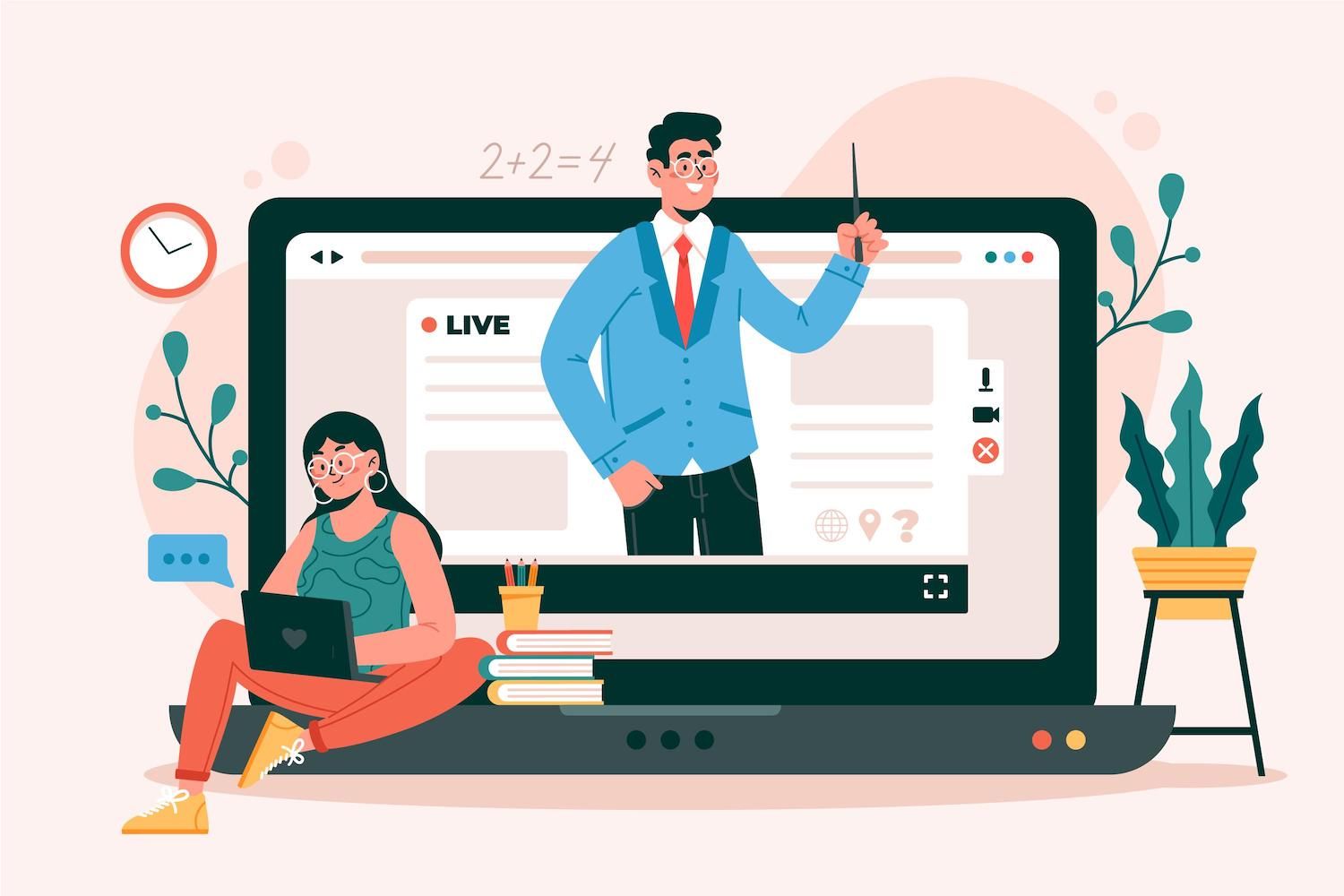
This information should be sent to
Conditional rendering is described as an essential characteristic of React which allows developers to render elements in response to certain circumstances.
It's a crucial concept that's crucial to building websites that are dynamic and intriguing.
In this comprehensive guide, we will dive into the subject of conditional rendering utilizing React. The guide will cover the fundamentals as well as advanced techniques and will provide examples that will help in understanding.
React's view of Conditional Rendering
It's highly useful in situations where you need to block or deactivate certain UI components, modify the look of your website or provide different variations of your site in response to the interaction with users.
Conditional rendering is crucial in React applications since it allows the development of interactive, dynamic user interfaces that respond to any changes made in information or interactions, in real-time.
The Fundamentals of Conditional rendering
There are a variety of fundamental techniques that you could employ to render conditional graphics with React. In this article, we will go over every one of them thoroughly.
With the help of the if expression to create the conditional rendering
One of the simplest ways to make use of the conditional rendering function in React is to make use of the traditional "when"
statement.
If (condition) returns Expression 1. If not, you should return Expression 2.
The the If
clause is a good choice in rendering(). rendering()
method to make sure that the content you have uploaded will be displayed in a specific fashion that aligns with the specifics of the base.
If you want to illustrate the concept you're trying to convey Use the expression if to illustrate the spinning wheel in the meantime you are waiting for data to be loaded:
import useState, useEffect from 'react'; import Spinner from './Spinner'; const MyComponent = () => const [isLoading, setIsLoading] = useState(true); const [data, setData] = useState(null); useEffect(() => // Fetch data from an API fetch('https://example.com/data') .then((response) => response.json()) .then((data) => setData(data); setIsLoading(false); ); , []); if (isLoading) return ; return /* Render the data here */; ; export default MyComponent;
In this scenario, MyComponent
fetches information from an API by using. utilizeEffect
hook. In the meantime, you are waiting for information to be loaded, you are able to utilize the Spinner component. It is controlled by an When
clause.
A different example is rendering for a fail-safe option in the event that rendering does not work because of an error. your component.
const MyComponent const MyComponent ( data ) * export default MyComponent
It is possible to utilize MyComponent. MyComponent
is a component that accepts prop for information as a prop-for-information
prop. If the prop isn't valid, and the component displays an error message.
prop isn't valid it will display an error message and the "If"
statement.
Furthermore, you may show different types of content to multiple user roles using your anytime
clause:
const MyComponent = ( user ) * Export default MyComponent;
It's possible to create MyComponent. It's possible to build MyComponent
which makes use of the role of a human as it to operate the
device. On the basis of user.role
property, the content is displayed using an "If"
clause.
Utilizing the Ternary Operator to perform conditional rendering
The most effective method of using conditional rendering inside React is using the operator the ternary (?) inside JSX.
The ternary operator allows users to create an Inline If-Else using three operands. The first operand can be referred to by the term condition. Third and second operands make up the expressions. When the condition is acceptable,
then the first expression will be performed. If there's no need, the second one is executed.
You can, for instance, render a frame of a prop
import ComponentA from './ComponentA'; import ComponentB from './ComponentB'; const ExampleComponent = ( shouldRenderComponentA ) => return ( shouldRenderComponentA ? : ); ; export default ExampleComponent;
In this code, we have an ExampleComponent
that takes a prop called shouldRenderComponentA
. The operator will render component A
or ComponentB
according to the prop's values.
You can create different texts that are based on:
import useState from 'react'; const ExampleComponent = () => const [showMessage, setShowMessage] = useState(false); return ( setShowMessage(!showMessage)> showMessage ? display message" message" message" Welcome to the world! : null ); ; export default ExampleComponent;
In this case, it's the ternary operator that renders the text in a specific manner based on the showMessage's showMessage
status. If the button is clicked, and showMessage is selected, the display of showMessage
can be changed and the text could be displayed or hidden based on circumstance.
It is also possible to create the loading spinner when you download data:
import useState, useEffect from 'react'; import Spinner from './Spinner'; const ExampleComponent = () => const [isLoading, setIsLoading] = useState(true); const [data, setData] = useState(null); useEffect(() => const fetchData = async () => const response = await fetch('https://jsonplaceholder.typicode.com/todos/1'); const jsonData = await response.json(); setData(jsonData); setIsLoading(false); ; fetchData(); , []); return ( isLoading ? : data.title ); ; export default ExampleComponent;
In this example it is employed to make use of the ternary operator rendering an elongated spinner while the data is downloaded via an API. Once the data is download and rendered we then render the name of the property
property with the help of operators in the ternary.
Utilizing Logical OR Operators to perform Conditional rendering
In addition, it's possible to use also and ( &&
) as well as OR ( ||
) operators to perform rendering of conditional elements in React.
The operator logic and allows the rendering of a component only when one of the prerequisites is satisfied, whereas the logic OR operator allows rendering of an element when either or both of the conditions are met.
These are helpful in situations in which you must establish easy rules that determine which elements are acceptable to render or not. In the case of rendering a button, if it is in a format that you can accept, and the layout is suitable, you could use the logic and operator and render the button in the following manner:
import useState from 'react'; const FormComponent = () => const [formValues, setFormValues] = useState( username: "", password: "" ); const isFormValid = formValues.username && formValues.password; const handleSubmit = (event) => event.preventDefault(); // Submit form data ; return ( setFormValues( ...formValues, username: e.target.value ) /> setFormValues( ...formValues, password: e.target.value ) /> isFormValid && Submit ); ; export default FormComponent;
This is a good example of FormComponent
using a form which includes two input fields that permit users to enter details about an username
as well as the password
. This form utilizes useState as the utilizeState
hook to handle the field's value as well as its isFormValid
variable that determines how much each field is within the form that has values. By using the logic AND operator, (&&), this will make the button submit only in the event that the value of the variable
is the case. This will ensure that the button is only capable of being enabled when the verification of forms has been successfully completed.
As an alternative, you may also use the OR operator to show an error message whenever the load of data isn't completed or to display a message about an error if you experience an error
import React, useEffect, useState from 'react'; Const DataComponent = () => const [data setData, data] = useState(null) Const [isLoading isLoading, setIsLoadingisLoading, setIsLoading] = useState(true) setErrorMessage, const, setErrorMessage] = the useState (''); UseEffect(() to fetch data, return to retrieve the data, []); return ( >ErrorMessage ? ( errorMessage ) : ( data.map((item) => ( item.name )) ) > ); ; export default DataComponent;
In this case, DataComponent. DataComponent
is able to collect data using an API using fetch. The data is presented in a list. It utilizes an UtilizeState
hook to control the status of the data. This includes its load status and error message. By using the logic OR operator, (||), we are capable of displaying the loading or error message in the event that one of the conditions are met. The message will be displayed to the user to explain the process of obtaining data.
Using this logic AND as well as OR operators to generate conditions, React is reliable and an easy way for dealing with simple scenarios. But it is advised to utilize other approaches for tackling complex situations like the changing of
declarations, to create more complexity in the way you think.
Advanced Techniques for Conditional Rendering
Conditional rendering in React is more complicated based on the specifications of the software. These are advanced methods that could be utilized to render conditionally in more complex circumstances.
Making use of Switch Statements to render conditional content
Statements and operators are the most common method of rendering conditionsal statements by with switches switch
phrase, they could work for situations involving multiple scenarios.
Here's a visual
Import React from'react";const MyComponent = (userType) =switch (userType) userType) "admin": Return"welcome admin user!" The user case returned"welcome to all regular users!" The default is to return. Log in to find out more. ; ; export default MyComponent;
It can be seen that it is the switch
declaration that creates the contents based on the userType
prop's conditional. This method can prove useful in a myriad of situations and can provide a better-organized and understandable way to manage complicated algorithmic procedures.
Conditional rendering by React Router
React Router is described as an application widely used by people of all ages and manages routing of clients who use React applications. React Router permits you to have the component perform rendering according with the specifications of your current routing.
Here's a simple illustration of how to implement the feature for conditional rendering with React Router.
import useState from 'react'; import BrowserRouter as Router, Route, Switch from 'react-router-dom'; import Home from './components/Home'; import Login from './components/Login'; import Dashboard from './components/Dashboard'; import NotFound from './components/NotFound'; const App = () => const [isLoggedIn, setIsLoggedIn] = useState(false); return ( isLoggedIn ? ( ) : ( ) ); ; export default App;
In the below code in the example above, it'll utilize its logged-in
status to display a component when it's part of the Dashboard
component. But, it'll perform this action only when users are logged into but not if it's the missing
component when users have not yet signed into their account. The Login Login
component can change its state to indicate it's in the
state to change to the current condition
when users log into their account.
The child prop within the component is passed to Login via the Login
component as well as employing Login's settingIsLoggedIn
function. It is possible to add the Login component to use props without declaring it in the Login
component, without indicating the exact location for the
prop.
Summary
Conditional rendering is a dependable technique in React which lets you change the user interface depending on various situations.
In the event that you're contemplating how complex the app's UI algorithm is, you're in a place to pick the one that is most appropriate to your specific needs.
Make sure you keep your code tidy, neat and neat, as well as ensuring that you examine thoroughly your conditional rendering algorithm to make sure it is working exactly as you want it to depending on the situation.
The post was published on this blog.
This post was first published here. this website
This article was originally posted here
Article was posted on here