Next.js vs React? It's a Partnershipand Not an Competition (r) (r)
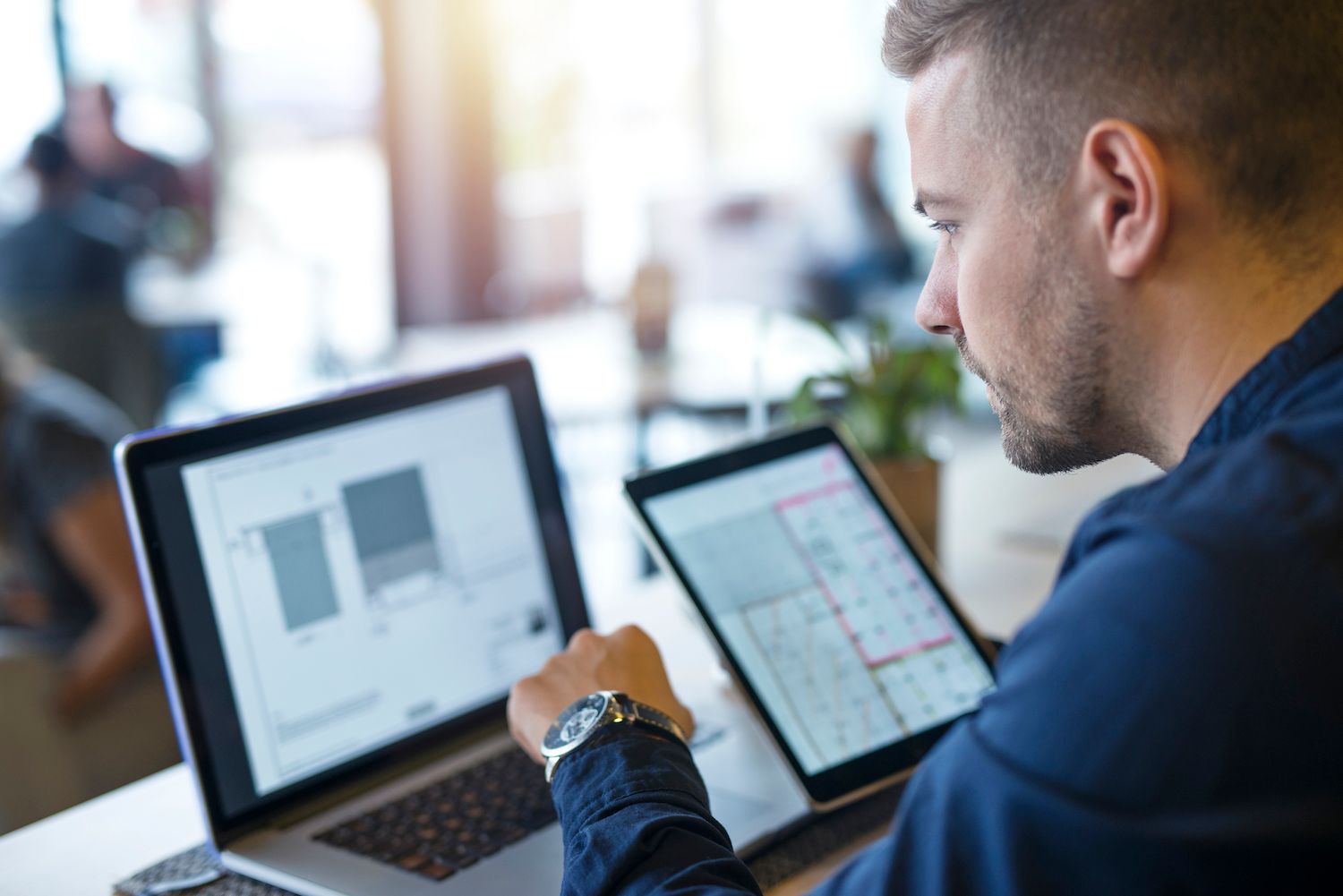
In this post we'll examine Next.js as well as React to help you decide if they're right for the next task you're working on.
Next.js along with React JavaScript on the Next Level
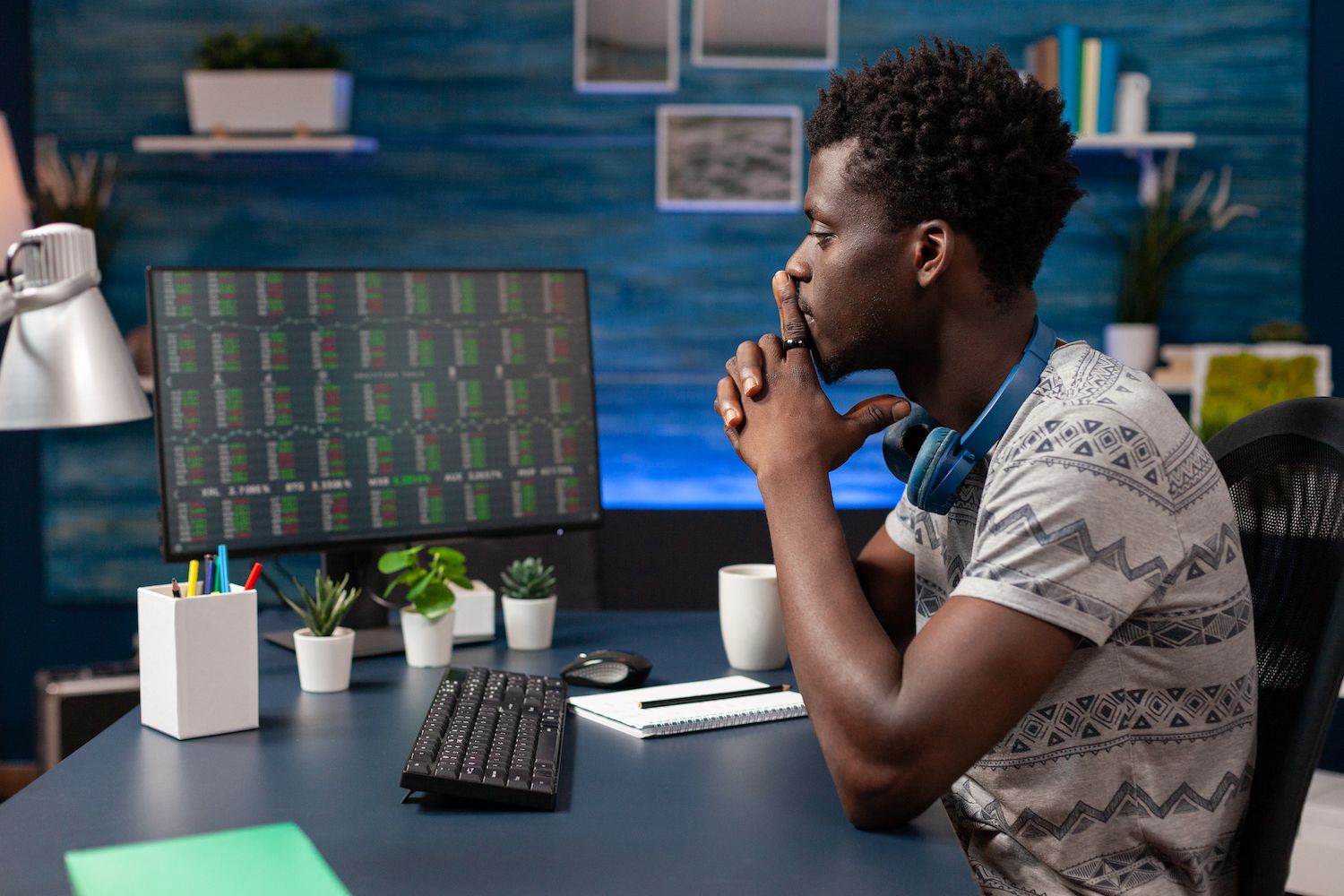
The broadness of JavaScript is the reason that a complete grasp of its most commonly used tools are essential to your success as web developers.
What Is Next.js?
Next.js is one of the best developers experiences when it comes to creating fast, SEO-friendly applications. Below are some characteristics of Next.js which create it as a superior production framework
- Hybrid rendering capabilities
- Automatic code-splitting
- Image optimization
- Built-in support for CSS preprocessors as well as CSS-in-JS libraries.
- Built-in routing
Those features assist Next.js developers save considerable time in the process of configuration and tooling. It is possible to start building your application, which may be used to support projects like these:
- Ecommerce stores
- Blogs
- Dashboards
- Single-page application
- Interact user interfaces
- Websites with static content
What's the React?
React is an JavaScript library that is used to create dynamic user interfaces. In addition to making web interfaces, it is also possible to build mobile applications with React Native.
Some benefits of using React include:
- Performance improvements: Instead of updating each component in the DOM React makes use of a virtual DOM that updates only the changed components.
- Component-driven:Once you create a component, you can use it again and again.
- Quick debugging React applications employ a unidirectional data flow - between parent and child components only.
Next.js vs React
Though developers frequently use Next.js and React for the same purpose, there are some fundamental difference between the two.
Ease of Use
To use Next.js the most basic command is:
Create-next-App
If there are no other arguments to create-next-app
, the installation is carried out in an interactive manner. It will ask you for an appropriate name for your project (which will be used for the directory of your project), and whether you wish to provide the ability to support TypeScript as well as the code linter ESLint.
It'll look something like this:
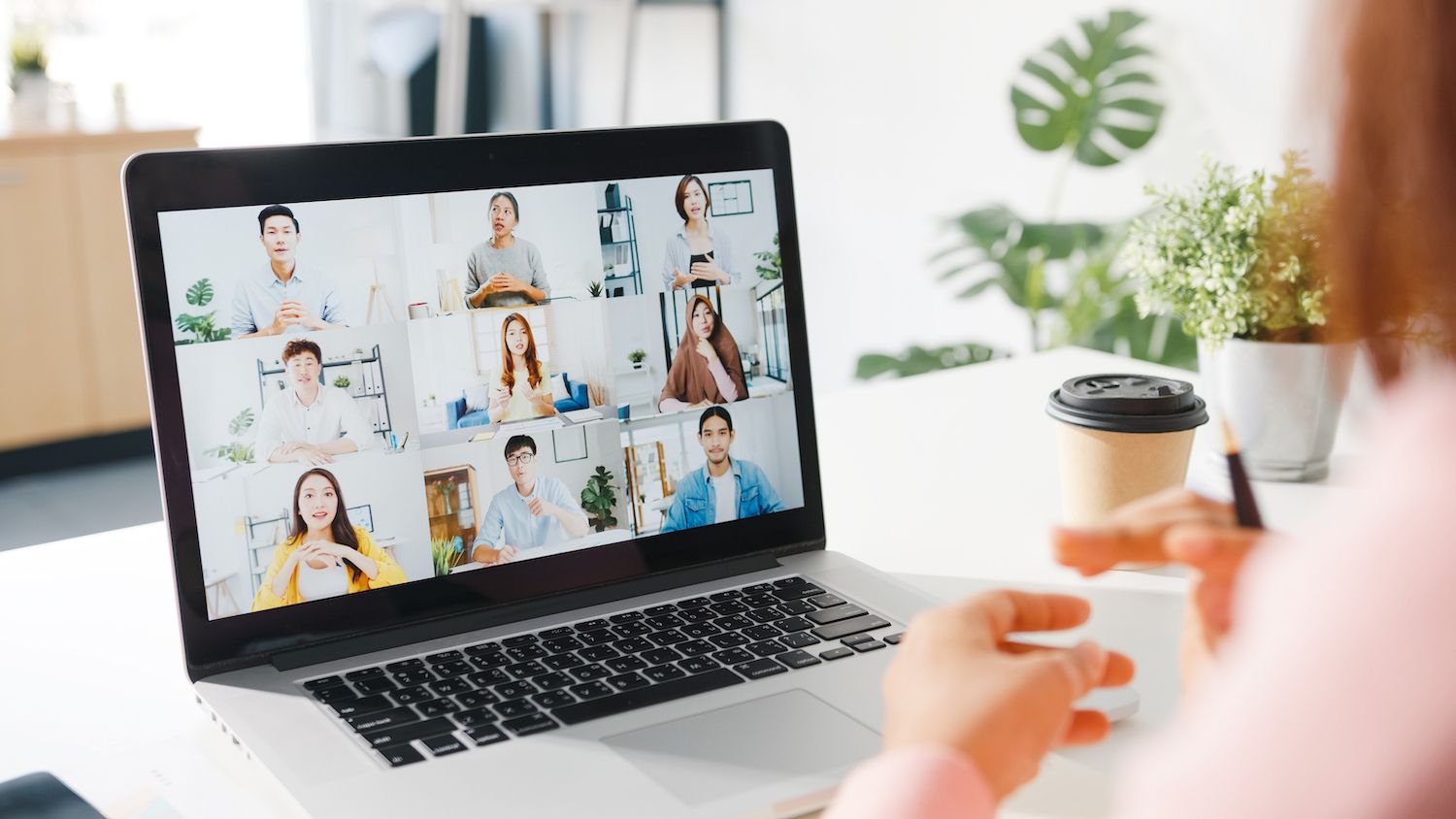
When initializing the React instance, the simplest procedure is to include a name for the project's directory:
npx create-react-app new-app
The result is a folder with all the necessary initial configurations and dependencies:
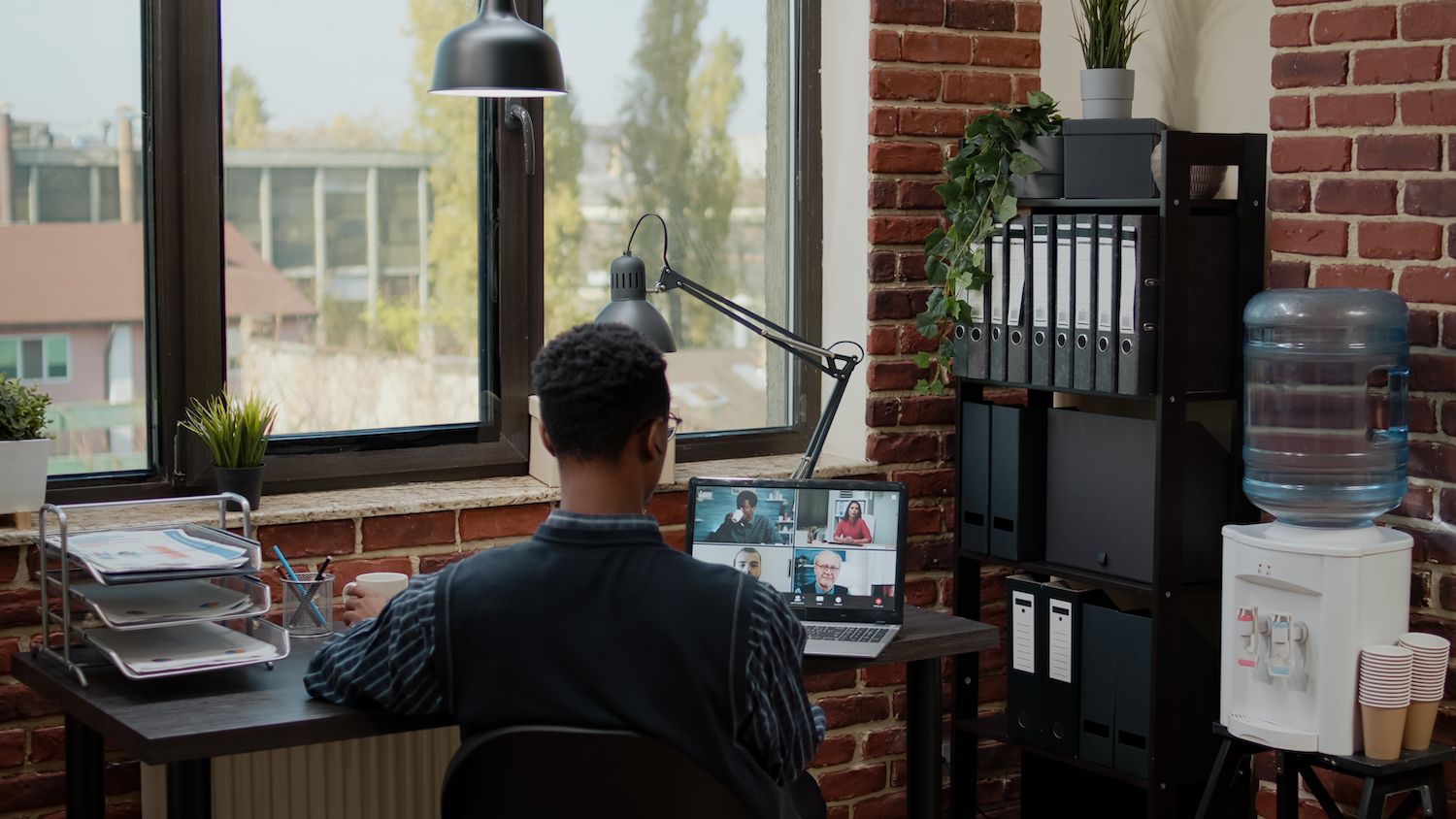
Both make it simple to start, keep in mind that Next.js is built on top of React. This means that you cannot master Next.js without learning React and understanding how it operates. It's a good thing, React is a relatively easy to learn curve that is ideal for novices.
Also, it is important to remember that React is not structured. You must install and set up a React router, and then decide on how to handle data fetching, image optimization, and code-splitting. This setup requires you to install and configure further libraries and other tools.
By contrast, Next.js comes with these tools installed and ready-to-use. When using Next.js, any file added to it's pages
folder automatically serves as a route. With this support built in, Next.js is easier to use every day, allowing you to start coding your app's logic right away.
Next.js and React features
Because Next.js is built on React, the two have some similarities in features. But, Next.js goes a step beyond and offers additional functions like routing, code-splitting, pre-rendering and API support out of the box. These are the features are necessary to setup yourself when using React.
Data Fetching
React displays data from the client's side. It sends static data to the browser. after that, the browser retrieves the information from APIs in order to fill the app with data. This decreases performance and creates a bad customer experience because the app is slow to load. Next.js can solve this issue by the pre-rendering process.
With pre-rendering, the server makes the necessary API calls and fetches the information prior to sending an application to the browser. So, when a browser opens, it gets ready-to-render pages from the web server.
Here is an example of the way Next.js creates pages that are not based on any data:
function App()
return Welcome
export default App
To create static pages that use external data, use to use the GetStaticProps()
function. After you have exported the getStaticProps()
from a page, Next.js will pre-render the page using the props it returns. The function runs always in the server. Therefore, use to getStaticProps()
when the data the page uses is available at build time. For example, you can utilize it to pull blog posts from the CMS.
const Posts= (Posts) •
export const GetStaticProps for async () •
When the page paths depend on other data sources, utilize to use the obtainStaticPaths()
function. To create a path based on the ID of the post, you can export the staticPaths()
from the page.
For example, you might export staticPaths.getStaticPaths.()
from pages/posts/[id].js as shown below.
export GetStaticPaths = Async() *
getStaticPaths()
is often paired with GetStaticProps()
. In this case it is possible to make use of the getStaticProps()
to fetch information about the ID in the path.
export const getStaticProps = async ( params ) =>
const post = await getSinglePost(params.id);
return
props: post
;
;
In SSR the data is downloaded at the requested time and sent to the web browser. To use SSR simply convert the the getServerSide()
props function from the page that you would like to render. The server invokes this function each time a request is made, which allows SSR suitable for sites that use dynamic data.
In particular, you could utilize it to pull information from an API for news.
const News = ( data ) =>
return (
// render data
);
;
export async function getServerSideProps()
const res = await fetch(`https://app-url/data`)
const data = await res.json()
return props: data
The data is fetched on every request and passed to the News component through props.
Code Splitting
Code splitting involves splitting code into chunks which the browser is able to load at any time. This reduces the volume of code transmitted to the browser in the initial load because the server sends only what the user needs. Bundlers like Webpack, Rollup, and Browserify support code-splitting within React.
Next.js lets code-splitting be done straight out of the box.
With Next.js the pages are code-split. Adding pages to the app does reduce the size of the bundle. Next.js additionally supports dynamic imports. This allows users to import JavaScript modules and load them dynamically during runtime. Dynamic imports help speed up speed of pages since bundles are loaded lazy.
In the Home section below the server won't include the hero component within the bundle that was created.
const DynamicHero = dynamic(() => import('../components/Hero'),
suspense: true,
)
export default function Home()
return (
)
In this case, the suspense's fallback element will be rendered before the hero component is loaded.
API Support for Next.js against React
You can, for instance, build an API route called pages/api/user.js API route which returns the user's name like this:
export default function user(req, res)
res.status(200).json( username: 'Jane' );
If you visit the https://app-url/api/user URL, you will see the username object.
username: 'Jane'
API routes are helpful when you want to mask the URL of a service you are accessing or want to keep environment variables private without having to program an entire backend application.
Performance
Next.js is definitely superior in its ability to create better-performing apps with a simplified procedure. SSR as well as SSG Next.js applications are more efficient than the client-side rendering (CSR) React applications. By fetching data on the server and transferring everything necessary to the browser in order to render it, Next.js eliminates the need for a data-fetch request to APIs. This means faster load times.
Next.js offers font optimizations, smart route prefetching and bundling optimizations. These features aren't immediately available in React.
Assistance
Since React has existed for longer than Next.js and has an larger community. However, many React developers are adopting Next.js, so that development of the community continues to grow. Developers are more easily finding already-existing solutions to the problems they run into instead of having to build the solutions themselves.
Next.js is also a great documentation with comprehensive examples that are simple to follow. In spite of its popularity, React documentation is not nearly as user-friendly as.
Summary
The choice of Next.js or React comes down to an application's requirements.
Next.js increases the power of React through providing structure and tools that enhance performance. The tools such as code splitting, routing and image optimization, are built into Next.js and developers don't require manual configuration of anything. Thanks to these features, Next.js is easy to use, and developers can begin coding the business logic as soon as they are ready.
Due to the various rendering options, Next.js can be utilized for rendering server-side applications as well as applications that blend static generation with Node.js rendering on the server. Also, thanks to the optimization features offered by Next.js, it's perfect for sites that must be quick, such as e-commerce stores.
React is a JavaScript library that will help you create and scale powerful front-end apps. Its syntax is easy to understand, especially for developers with a JavaScript background. Furthermore, you have control over the tools you use in your application and the way you set them up.
- Simple setup and management on My dashboard. My dashboard
- Support is available 24/7.
- The top Google Cloud Platform hardware and network, that is powered by Kubernetes for maximum scalability
- Enterprise-level Cloudflare integration for speed and security
- The reach of the global audience is as high as up to 35 data centers, and more than 275 PoPs across the globe