OOP in PHP transforms WordPress development (r)
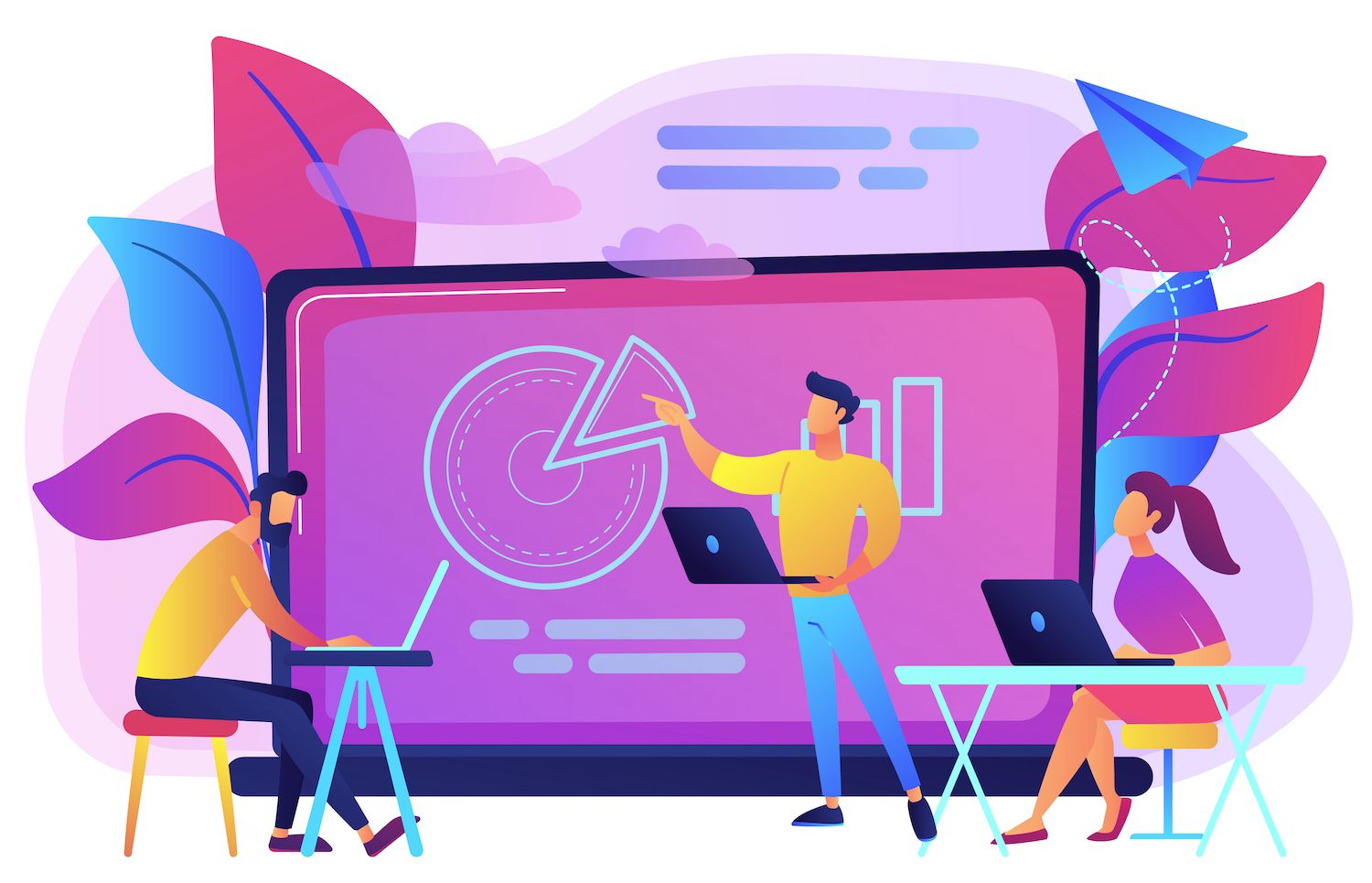
-sidebar-toc>
Object-oriented programming (OOP) one of the most important method of software development that is focused on "objects" -- instances of classes which contain data and behaviors rather than "actions."
The prerequisites
In order to follow the hands-on sections of this article, ensure you have:
- and XAMPP (or similar) to Apache and MySQL
- An environment for PHP in Version 7.4 or greater
- A code editor, like Visual Studio Code
- Expertise in PHP and WordPress
The advantages of OOP when it comes to PHP development
In this light we'll look into two distinct advantages that come with OOP in PHP by highlighting the ways it can transform the process of development.
1. Reusability of code and its maintenance
OOP in PHP makes it straightforward to reuse code due to Polymorphism as well as inheritance. Classes can use properties and methods from other classes. This allows you to reuse old methods in new ways and without much change.
OOP is also a great way to manage your code. It is a way to keep objects' information private, and share only the information that is needed. This can be done using specific methods known as getters and setters. This method helps to prevent the changes made to one component of your program from creating issues in other parts, which makes the code simpler to update and maintain.
Also, because objects are complete on their own, finding and fixing issues in specific areas of the system becomes much easier. This increases overall quality of code and reliability.
2. Enhanced clarity and structure
OOP makes PHP code more efficient and organised by using classes and objects. Classes act like template objects that keep all of the information that is needed all in one location.
OOP can also allow classes to utilize features from other classes. That means that you don't have to write the same code over and over. These features help to make code cleaner, easier to repair, and also more organized.
Clear code from OOP allows teams to work more effectively together. It's much easier for all to comprehend what code is doing, meaning less time explaining issues and more time to do the work. This also helps reduce errors and helps the project remain on course. When code is clean and well-organized, new team members can quickly make progress.
Implementing OOP within PHP
When you use OOP with PHP You organize your code with classes and objects, like blueprints and houses. Create classes for all things (like the books or users) with their attributes and their actions. You then make use of inheritance to create new classes by combining existing classes, saving time in the process of not having to duplicate your code. Since encapsulation protects certain part of the class private, your code is safer.
1. Define a class with the properties and methods
Start with an Article
class which contains titles, content and the status property -- together with the methods used to show and control these properties.
class Article
private $title;
private $content;
private $status;
const STATUS_PUBLISHED = 'published';
const STATUS_DRAFT = 'draft';
public function __construct($title, $content)
$this->title = $title;
$this->content = $content;
$this->status = self::STATUS_DRAFT;
public function setTitle($title)
$this->title = $title;
return $this;
public function setContent($content)
$this->content = $content;
return $this;
public function setStatus($status)
$this->status = $status;
return $this;
public function display()
echo "$this->title
$this->content
Status: $this->status";
2. Create objects and implement method chaining
Create an object called article and use the method of chaining to set its properties:
$article = new Article("OOP with PHP", "Object-Oriented Programming concepts. ");
$article->setTitle("Advanced OOP in PHP")->setContent("Exploring advanced concepts in OOP. ")->setStatus(Article::STATUS_PUBLISHED)->display();
3. Increase the strength of inheritance and encapsulation
Improve encapsulation using the getter and setter functions and create a FeaturedArticle
class that inherits from Article
:
class FeaturedArticle extends Article
private $highlightColor = '#FFFF00'; // Default highlight color
public function setHighlightColor($color)
$this->highlightColor = $color;
return $this;
public function display()
echo " style='background-color: $this->highlightColor;'>";
parent::display();
echo "";
$featuredArticle = new FeaturedArticle("Featured Article", "This is a featured article. ");
$featuredArticle->setStatus(FeaturedArticle::STATUS_PUBLISHED)->setHighlightColor('#FFA07A')->display();
4. Interfaces and polymorphism
Design an interface for publishing content, and then implement it into the Article
class, demonstrating polymorphism:
interface Publishable
public function publish();
class Article implements Publishable
// Existing class code...
public function publish()
$this->setStatus(self::STATUS_PUBLISHED);
echo "Article '$this->title' published. ";
function publishContent(Publishable $content)
$content->publish();
publishContent($article);
5. Utilize traits to help with common behavior
PHP allows you to use traits to include functions in classes, without having to take a class's inheritance. Utilizing the following code create a trait to allow logging activities within CMS: CMS:
trait Logger
public function log($message)
// Log message to a file or database
echo "Log: $message";
class Article
use Logger;
// Existing class code...
public function publish()
$this->setStatus(self::STATUS_PUBLISHED);
$this->log("Article '$this->title' published. ");
OOP within WordPress development
This section explains how to implement OOP to WordPress development. There are examples that you can cut and paste into your WordPress deployment to test.
OOP in WordPress themes: Custom post type registration
Place the following code in your theme's functions.php file. Your themes are available in the themes/wp-content directory.
class CustomPostTypeRegistrar
private $postType;
private $args;
public function __construct($postType, $args = [])
$this->postType = $postType;
$this->args = $args;
add_action('init', array($this, 'registerPostType'));
public function registerPostType()
register_post_type($this->postType, $this->args);
// Usage
$bookArgs = [
'public' => true,
'label' => 'Books',
'supports' => ['title', 'editor', 'thumbnail'],
'has_archive' => true,
];
new CustomPostTypeRegistrar('book', $bookArgs);
This code dynamically registers an individual post type book
and passes its information via bookArgs array. bookArgs
array. You can see the new custom post type in the WordPress administration sidebar that is labeled Book.
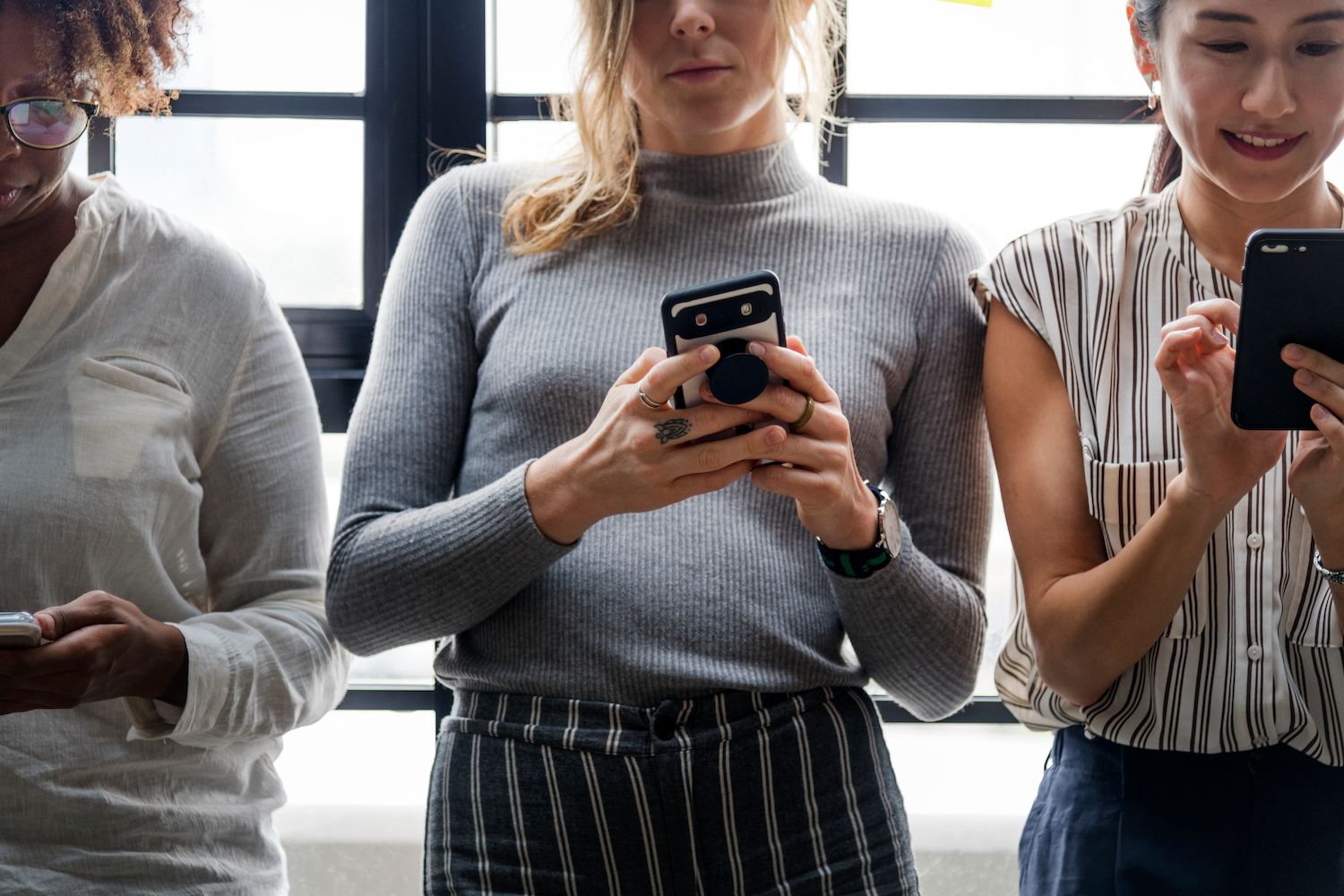
This example illustrates how OOP could be used to provide the capability of registering custom post types, making it reusable for different types of posts.
OOP is a feature of WordPress plugins shortcode handler
The plugin's name is: OOP Shortcode Handler* Description: Handles the creation of custom shortcodes using OOP. * Version: 1.0
* Author: Name
*/
class OOPShortcodeHandler
public function __construct()
add_shortcode('oop_message', array($this, 'displayCustomMessage'));
public function displayCustomMessage($atts)
$attributes = shortcode_atts(['message' => 'Hello, this is your OOP
message! '], $atts);
return "$attributes['message']";
new OOPShortcodeHandler();
Save this as my-oop-shortcode-handler.php in the wp-content/plugins directory. Finally, activate the plugin.
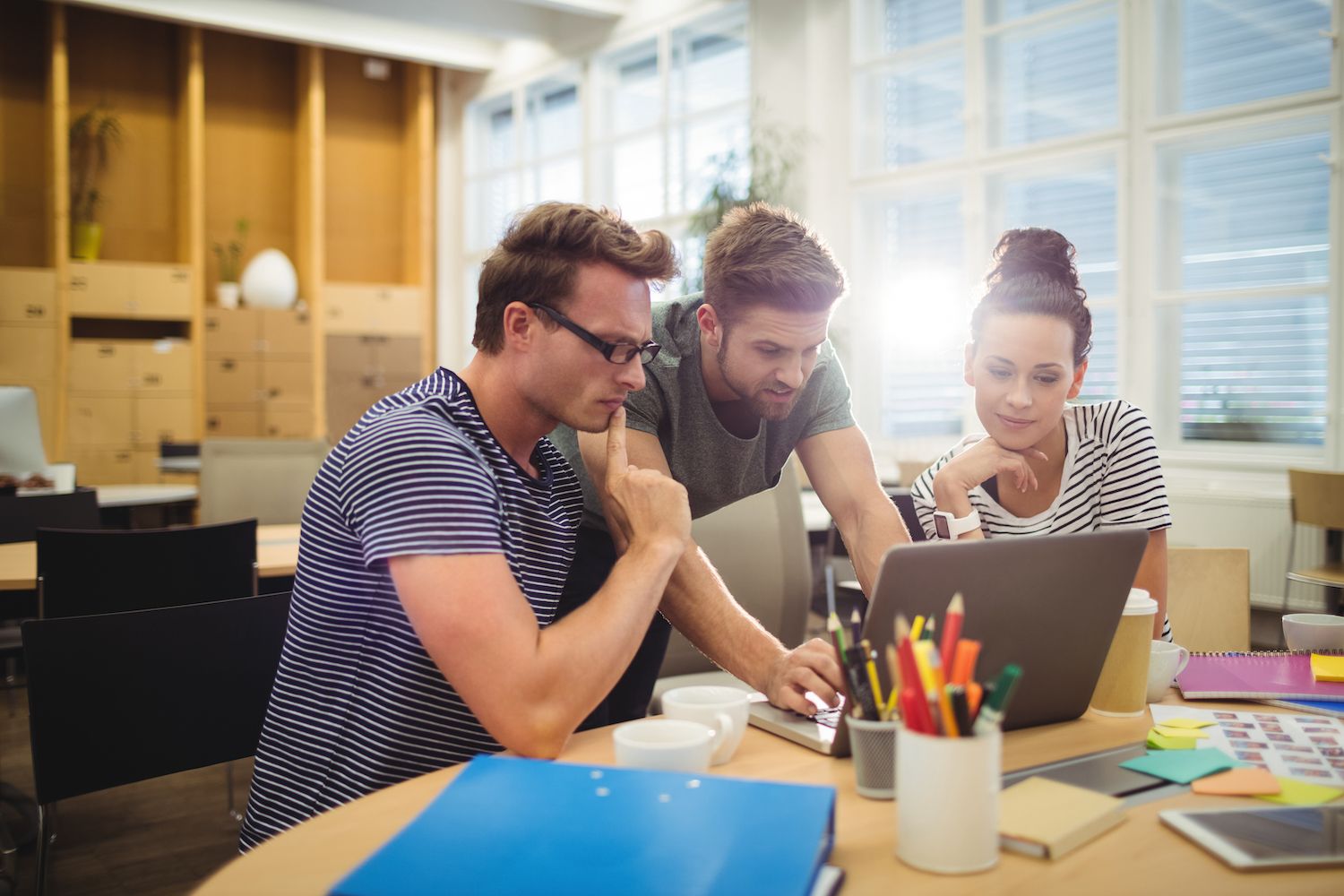
Then, in the editor for your page or blog prior to publishing or modifying, use those shortcodes [oop_messageand [oop_message
and [oop_message] to display the message="Custom Message Here"[oop_message message="Custom Message Here"]
, as shown below:
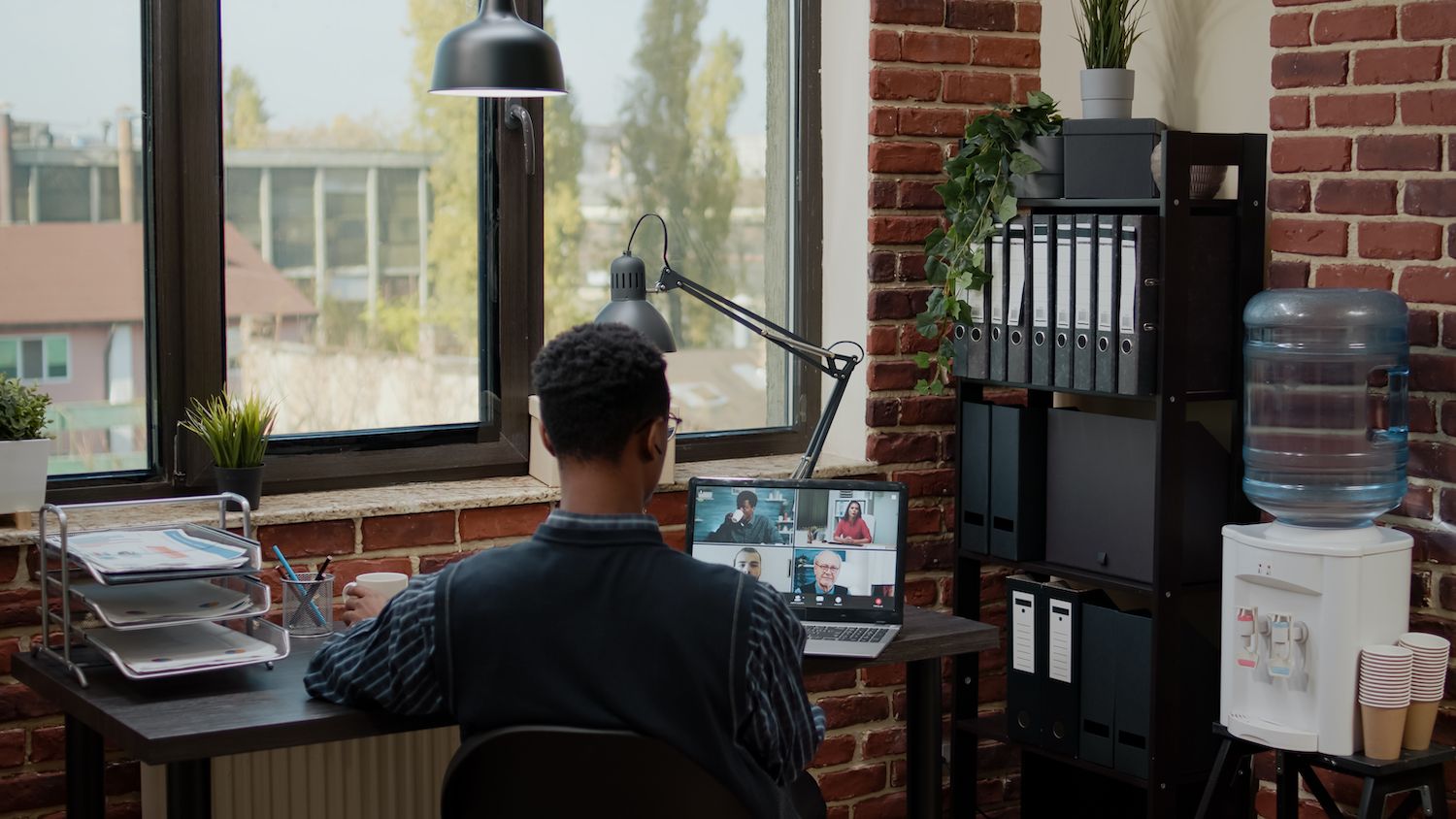
Following the publication or update, page/post , you see the message that the shortcodes displayed denote.
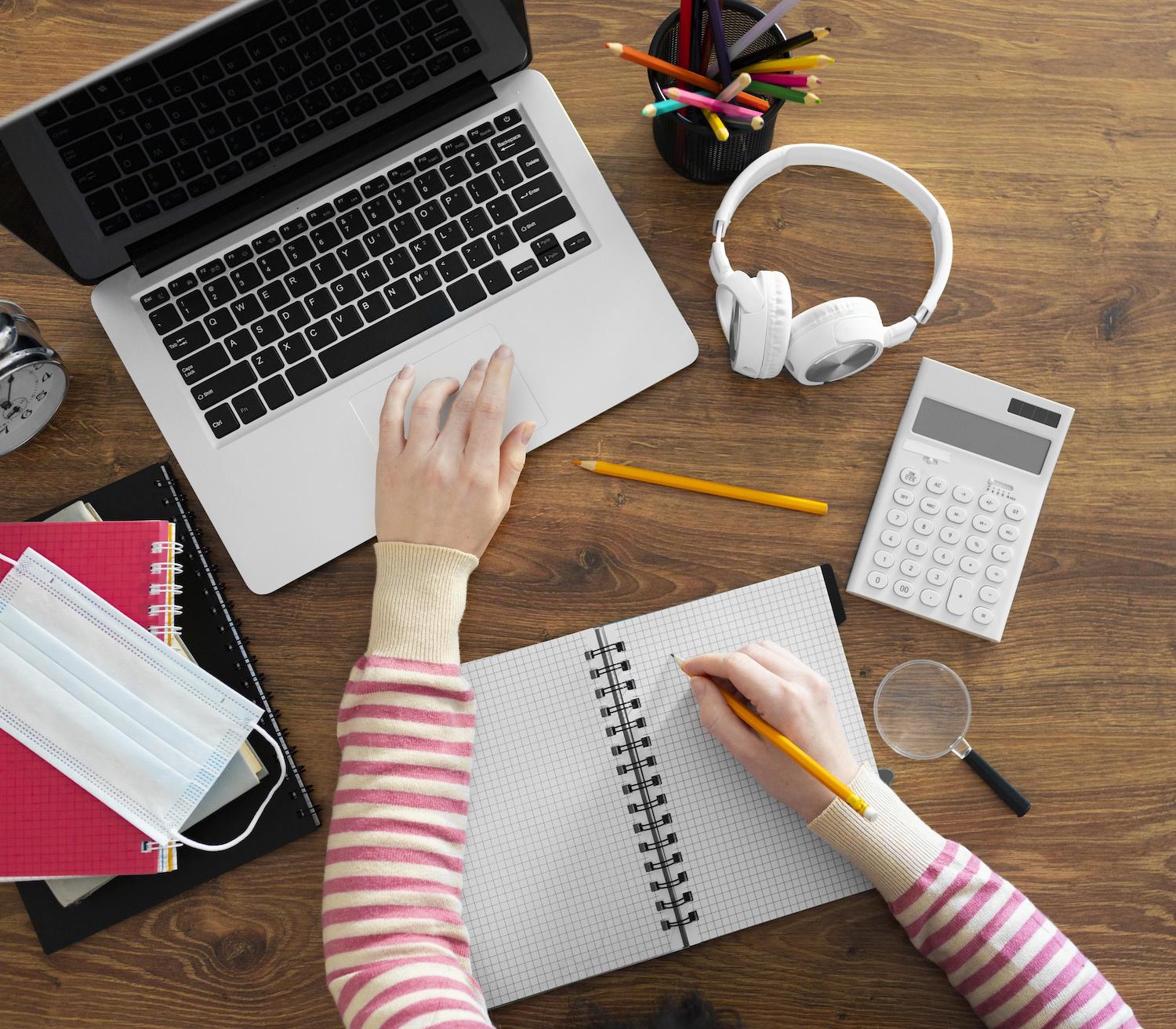
OOP in WordPress widgets: dynamic content widget
OOP is also beneficial for widgets because it encapsulates their functions inside classes. WordPress core itself uses OOP for widgets. This is where you build an individual widget which allows users to display interactive material with titles and the text.
Add the following code to your theme's functions.php file or inside an application plugin. It creates a custom widget that shows the text "Hello World From My Custom Widget!"
class My_Custom_Widget extends WP_Widget
Public function called __construct()
parent::__construct(
My_Custom_Widget' // Base ID'My Custom Widget",namearray('description' = __('A basic custom widget. ',
'text_domain'),) // Args
);
public function widget($args, $instance)
echo $args['before_widget'];
if (!empty($instance['title']))
echo $args['before_title'] . apply_filters('widget_title',
$instance['title']) . $args['after_title'];
// Widget content
echo __('Hello World From My Custom Widget! ', 'text_domain');
echo $args['after_widget'];
public function form($instance)
// Form in WordPress admin
public function update($new_instance, $old_instance)
// Processes widget options to be saved
function register_my_custom_widget()
register_widget('My_Custom_Widget');
add_action('widgets_init', 'register_my_custom_widget');
If you edit the theme by clicking by clicking the Customize button under the Appearance in the Administration area, you can include a brand new widget where you want.
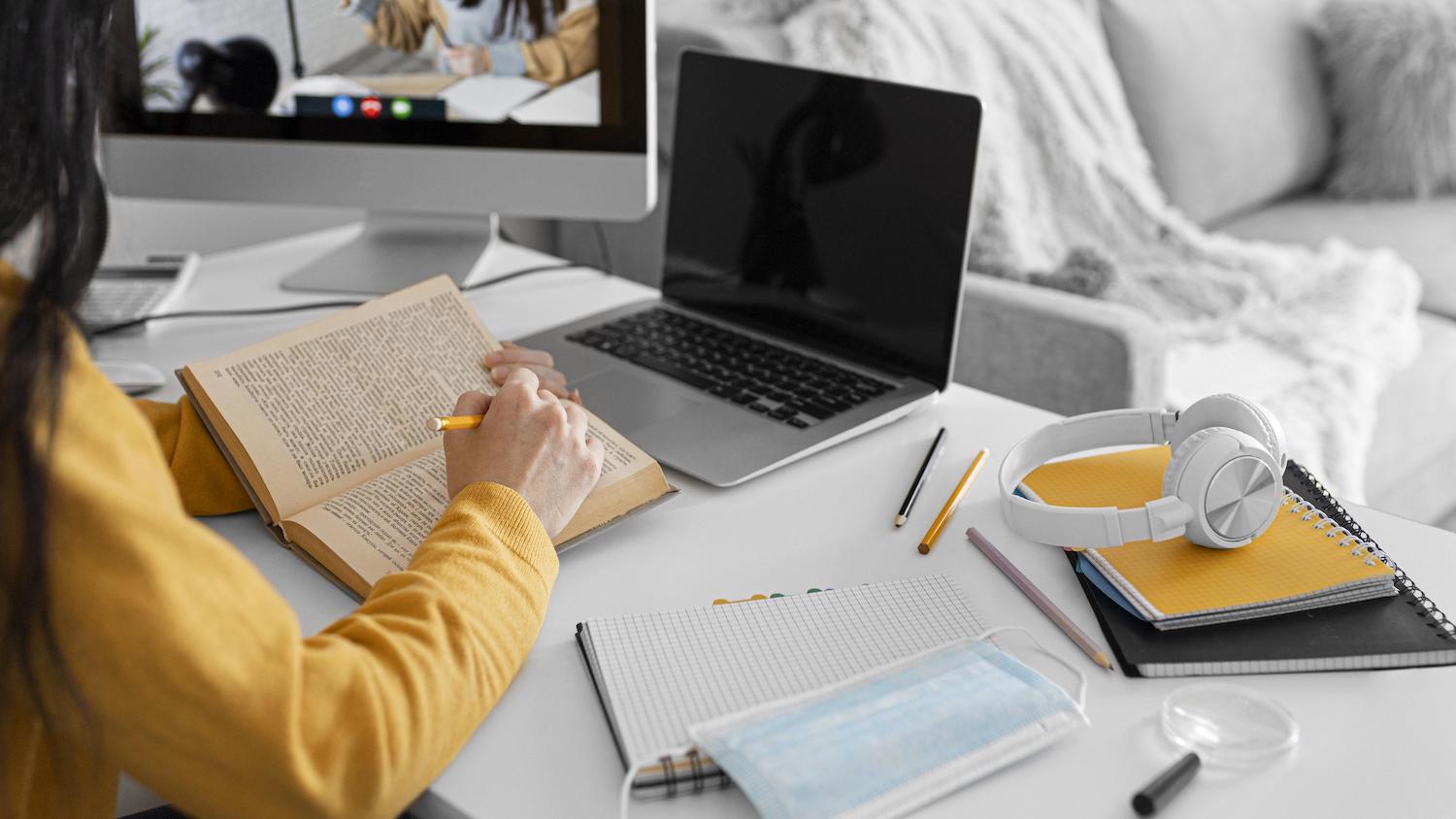
Utilizing WordPress classes
WordPress provides various classes that allow you to communicate with the CMS's core capabilities. Two classes that are included in this class are WP_User
and WP_Post
that represent the user as well as posts, respectively.
Save this as my-oop-shortcode-handler-extended.php in the wp-content/plugins directory. Then, you must activate the plugin.
$post->ID, // Default to the current post ID
], $atts);
$postDetails = get_post($attributes['post_id']); // Getting the WP_Post
object
if (!$postDetails)
return "Post not found. ";
$authorDetails = new WP_User($postDetails->post_author); // Getting the
WP_User object
$output = "";
$output .= "Author Information";
$output .= "Name: " . esc_html($authorDetails->display_name) . "";
$output .= "Post Information";
$output .= "Title: " . esc_html($postDetails->post_title) . "";
$output .= "Content: " . esc_html(wp_trim_words($postDetails->post_content, 20, '...')) . "";
$output .= "";
return $output;
new ExtendedOOPShortcodeHandler();
In this expanded version the shortcode you've made is: [post_author_details post_id="1"]
.
![Screenshot of the Sample Page. It opens with the "[post_author_details post_id="1"]" code snippet, with text that reads, "This is the rest of the article..." below it. Under this is a "Type / to choose a block" message](https://crealanta.com/content/images/2024/07/shortcode-smaple-page.png)
When added to a post or page, it displays information about the author of the post (using the WP_User
class) and the post itself (using the WP_Post
class).
OOP as well as OOP and the REST API for WordPress
In stark contrast to OOP concepts, a large portion of WordPress's core, especially the earlier parts, such as the API for plugins and themes, is written in a procedural programming format.
As an example, whereas a procedural approach might directly modify global variables, relying on a sequence of tasks, OOP in the REST API encapsulates the logic within classes. This is why specific procedures inside these classes manage the tasks of making, fetching, or changing, or even deleting posts.
This is clear and makes the codebase easier to modify and test.
By defining classes to define the endpoints, and handles requests, such as fetching posts using the GET http://wp.json.com/wp.v2/posts
request, the REST API gives a structured and scalable method of interfacing with WordPress data, returning JSON formatted responses.
Utilizing the leverage for PHP along with WordPress hosting
Summary
As you've seen in this post, OOP offers unparalleled flexibility, scalability, and maintainability when it comes to PHP as well as WordPress development.
Have you experienced the benefits of OOP within your projects? Perhaps you are interested in the ways it could transform the development process? Comment below.
Jeremy Holcombe
Content and Marketing Editor at , WordPress Web Developer, and Content writer. Outside of everything WordPress I like the beach, golf, and watching movies. Also, I have height issues ;).