React Best Practices to up your Game by December 9 2022.
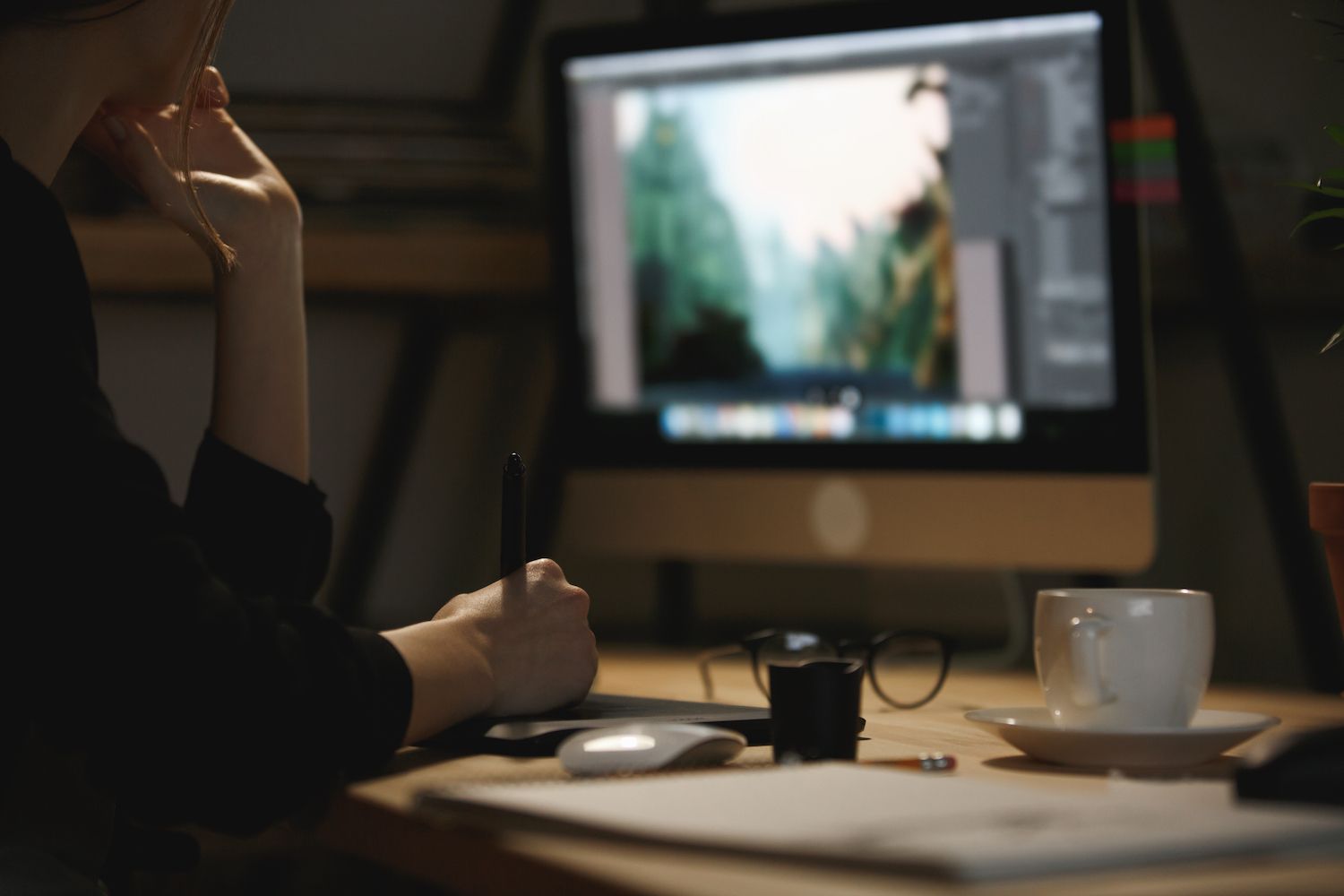
As as a React developer, learning the way that React functions isn't the only thing that you have to do in order to develop projects that are user-friendly, easy to scale, and manageable.
Additionally, you must be aware of some conventions to enable you to write clear React code. It will help you better serve your clients and help you and other developers working for the project to manage the code base.
In this video tutorial in this tutorial, we'll begin by speaking about some of the common challenges React developers have to face. We'll then dive into some of the most effective practices to apply to writing React code in a better way.
Let's get started!
Challenges React Developers Face
The challenges that you'll encounter in this article can be avoidable by following guidelines, which we'll discuss in detail later on.
Let's begin with one of the basic issues that affects beginners.
Conditions To React
Particularly, there are the core JavaScript concepts and functionalities to be aware of prior to diving into React. A few of them, that mostly belong to ES6 are:
- Arrow serves a purpose
- Rest operator
- Spread operator
- Modules
- Destructuring
- Array methods
- Template literals
- Promises
Let
as well asconst
variables
The JavaScript topics listed above will help you understand the basics of the way React operates.
In addition, you'll learn about the latest concepts in React such as:
- Components
- JSX
- State management
- Props
- Rendering elements
- Event handling
- Conditional rendering
- Lists and keys
- Forms and validation for forms
- Hooks
- Styling
A solid grasp of React concepts and the prerequisites in using the library can enable you to use its capabilities effectively.
State Management
In JavaScript changing a variable can be as simple as assigning a brand new value by using the equal to operator ( =
). Here's an example
var x = 300;
function updateX()
x = 100;
updateX();
console.log(x);
// 100
In the code above, we made a variable named x
that has the beginning value in the range of 300
.
Utilizing the equal operator, we assigned a number of a new value, 100
to it. The code was written in an updateX
function.
In React it is possible to update the value of variables is done differently. Here's how:
import useState from 'react';
function App()
const [x, setX] = useState(300)
let updateX =()=>
setX(100);
return (
x Update X); x Update X); setX, x)
SetX
setX);
export the default App
In order to update the state of a variable in React, you utilize the utilizeState
Hook. There are three points to note when using this Hook:
- The variable name
- The function allows you to update the variable
- The initial value/state of the variable.
In our example in our example,"x" is the title of the variable. SetX is the function for updating the value of x in contrast to the initial number (300) of x is given as a parameter to the function useState:
const [x setX, x[x, setX useState(300)
To update the state of the x
to reflect the current state of x, we utilized the setX
function:
import useState from'react';
let updateX =()=> (100);
Thus, the function updateX
function invokes setX function. setX
function. It is then used to set the value for x
to 100.
.
Though this method seems to perform perfectly in making changes to the state of your variables, it adds to the amount of code for very large projects. A lot of State Hooks make the code extremely difficult to keep track of and to comprehend, especially when the project grows.
Another issue that comes with this State Hook is that these variables created are not shared across the different elements that comprise the app. You'd still have the Props in order to transfer the information from one variable to another.
Luckily for us, there are libraries designed to manage state management in a way that is efficient with React. They even allow you to define a variable one time and use it anywhere you'd like in your React app. These libraries comprise Redux, Recoil, and Zustand.
The problem with choosing the third-party software for state management is that it means you'll need to understand new concepts foreign to what you've already learned in React. Redux is a good example. It was well-known for its large amount of boilerplate codethat caused it to be difficult for newcomers to understand (although this has been fixed through the Redux Toolkit, which allows you to write less code than you would with Redux).
Maintainability and Scalability
When the needs of users for products continue to shift There is always a need to make changes to the software which makes up the product.
It's often difficult to scale the code you write when it doesn't make it easy for the team to keep up. Difficulties like these arise from using bad practice when writing your code. These practices may seem to be working perfectly initially providing you with the desired result, but everything that works "for now" is inefficient for the future and development of your program.
Reacting to best practices
In this section this section, we'll go over some of the best practices to follow when writing code for React code. Let's dive right in.
1. Make sure that the Folder Structure is clear
Folder structures help the developers and you to understand the structure of files and assets being employed in projects.
When you've got a proper folder structure, it's easy to find your way around which saves time as well as helping avoid confusion. Folder structures differ with each team's preferences, but here are a selection of most commonly-used folders within React.
Grouping Folders by Features or Routes
Sorting your files by their route and capabilities helps to keep everything about a particular feature in the same space. If, for instance, there is a dashboard that you use for your users, you can have the JavaScript, CSS, and test files relating to the dashboard within the same folder.
Here's an example to demonstrate that:
dashboard/
index.js
dashboard.css
dashboard.test.js
home/
index.js
Home.css
HomeAPI.js
Home.test.js
blog/
index.js
Blog.css
Blog.test.js
As can be seen above the core features of the app has all its files and assets stored in the same location.
Gruping similar files
You can also group similar files in the same folder. There is also the option of having separate folders to store Hooks components, Hooks as well as other components. See this case study:
hooks/
useFetchData.js
usePostData.js
components/
Dashboard.js
Dashboard.css
Home.js
Home.css
Blog.js
Blog.css
It is not necessary to follow these folder structures in coding. If you've got a particular way to order your files, then go with the method you prefer. So long as the developers and you have an accurate understanding of format of the files, you're all set to start!
2. Institute a Structured Import Order
When your React application continues to grow, you're bound to make extra imports. The format of your imports go a long way in helping you understand what makes up your components.
A common practice is to group similar programs together appears to be a good idea. In particular, it is possible to separate external or third party imports separately from local imports.
Check out this example:
import Routes, Routes from "react-router-dom" toimport the createSlice from "@reduxjs/toolkit";
import the Menu from "@headlessui/react" Import the Home folder from "./Home" andimport logos from "./logo.svg";
import "./App.css";
In the example code above, we first grouped third party libraries together (these are libraries we had to install prior).
Then, we imported the files we had created locally, such as images, stylesheets, and components.
To ensure simple understanding and ease of use the example we've chosen to use doesn't show a very large codebase however, following this style of imports will help the other developers to understand the structure of your React application more clearly.
You can go further grouping your local files according to the types of files if it is what you prefer -- in other words grouping images, components, stylesheets, Hooks, and so on separately within your local imports.
This is an example:
home import from "./Home";
import about from "./About"
to import contact from "./Contact"
import the logo file from "./logo.svg" andimport closeBtn in "./close-btn.svg"
load "./App.css" toimport "Home.css"
3. Be Affirmative To Naming Conventions
Naming conventions can improve code readability. This applies not just to component names but even your variable names, all the way to your Hooks.
The React documentation does not offer an official format for the naming of your components. The most used naming conventions can be found in camelCase or PascalCase.
PascalCase is mostly used to describe name of component:
import React from 'react Function StudentList()
return (
StudentList
)
export default StudentList
The above component is called StudentList
, which is much more readable than studentlist
as well as Studentlist
.
Want to know how we increased our visitors by 1000%?
Join the 20,000+ who receive our newsletter every week with insider WordPress tricks!
In contrast on the other hand, the camelCase naming convention is mostly employed to name hooks, variables, functions, arrays, and so on:
const [firstName, setFirstName] = useState("Ihechikara");
const studentList = [];
const studentObject = ;
const getStudent = () =>
4. Use an Linter
The program analyzes the code you have written and informs the user when a rule has been violated. The word or phrase which violates the rule will typically be highlighted in red.
As every developer is different in their style of coding, linter tools will help to ensure that code is uniform.
Linter tools can also help in resolving bugs quickly. We can see spelling errors as well as variables that were declared , but never used, and other such functionalities. Some of these bugs can be solved automatically by modifying your program.
5. Employ Snippet Libraries
Snippet libraries help developers develop easier by providing prebuilt code that developers use often.
A good example is the ES7+ React/Redux/React-Native snippets extension, which has a lot of helpful commands for generating prebuilt code. In the case of, say, if you want to create the React functional component without typing out the entire code, all you need to do is type "rfce"
and press enter.
The command above will go on to generate a functional component with an alias that matches the file's name. We generated the code below using the ES7+ React/Redux/React-Native snippets extension:
import React from 'react studentList' function()
return (
StudentList
)
export default StudentList
Another tool for snippets of code that can be useful is the Tailwind CSS IntelliSense extension, which makes it simpler to creating web pages that are styled with Tailwind CSS. This extension will assist you to autocompletion by suggesting utility classes such as syntax highlighting and functions for linting. The extension lets you even view what your colors look like while coding.
6. Combining CSS and JavaScript
When working on large project, using separate styles of stylesheet files for different components can make your file arrangement clunky and difficult navigate around.
One solution is to blend your CSS with JSX code. It is possible to use libraries or frameworks such as Tailwind CSS and Emotion for this.
Here's how styling using Tailwind CSS looks like:
edge of resource
The code below gives the element of paragraph a bold font and adds some margin to the right. It is possible to accomplish this with the help of the framework's utility classes.
Here's how you'd style an element using Emotion:
Hello World!
7. Limit Component Creation
One of the main characteristics of React is code reusability. It is possible to create components and then reuse the logic of it in as many instances as you want without having to rewrite the logic.
Keep this in mind when you create your files it is important to limit the number of components you build. Not doing so bloats the file structure with unnecessary file types that aren't needed at all.
We'll use a very easy instance to show this:
Function UserInfo()
return (
My name is Ihechikara.
);
export the default UserInfo
The image above displays the name of a user. If we had to make one file per user, we'd eventually have an unreasonable number of documents. (Of course the fact that we're using information about users to make things simpler. In a real life situation there could be a need to work with a different type of reasoning.)
In order to make our part usable, we can employ props. Here's how:
Function UserInfo(UserName) •Export default UserInfo
Once we have that done, we may then import this component and utilize it for as many times as we'd like.
transfer UserInfo to "./UserInfo" Function App()
return (
);
export default App;
We now have three distinct instances for the UserInfo
element that is derived from the code that is created in one file instead of having separate files per individual user.
8. Implement Lazy Loading
As a default, React packs and deploys the entire application. But we can change the behavior by using lazy loading, otherwise called code splitting.
In essence, you are able to limit the content of your application that will be loaded at a specific moment. This is accomplished by splitting your bundles and only loading what is relevant to the needs of the user. In this case, for instance, you could initially load just the necessary logic for users to sign up, then load the logic for the user's dashboard only after the user has successfully signed in.
9. Utilize Reusable Hooks
Hooks included in React allow you to harness the additional capabilities of React such as interacting with your component's state and running effects in relation to specific state changes within the component. It is possible to do without having to write class components.
We can also make Hooks reuseable so that we don't have to retype the logic in every file they're used. The way to do this is through the creation of unique Hooks that can be imported any time within the application.
In the below example in the example below, we'll build a Hook for fetching information from APIs that are external:
import useState, useEffect in "react";function useFetchData(url) Const [data setDatadata, setData useState(null);
useEffect(() = useEffect(()) =fetch(url)
.then((res) => res.json())
.then((data) = setData(data))
.catch((err) => console.log(`Error in $err `));
, [url]);
return data ;
export the default useFetchData
The Hook was created that can fetch data from APIs listed above. This hook can now be transferred to any part of the system. It eliminates the hassle having to write all that process in each component that we have to fetch external information.
The type of custom Hooks you can design in React is endless, therefore it's up to you choose how you will use them. Be aware that if you're creating a functionality that has to be used across various components, it's best to ensure that it is reusable.
10. Log and Manage Errors
There are different ways of handling errors in React like using error boundaries, try and catch blocks or using external libraries like react-error-boundary
.
The built in error boundaries which were introduced in React 16 was a functionality that was designed for class components, so we will not discuss it since it's recommended to use functional components in place of class components.
However the use of the try
and catch
block is only suitable to execute imperative code but it is not a declarative method. This means that it's not an option to use with JSX.
Our best recommendation would be to use a library like react-error-boundary. The library offers functions that are wrapped around your component, which can help you detect errors while your React application is rendered.
11. Monitor and Test Your Code
Although many may claim that testing isn't important when it comes to developing an app for the internet, it comes with innumerable benefits. Here are just some of them:
- Finding bugs results in improved code quality.
- Unit tests can be documented for data collection and future reference.
- A quick bug fix can save users from paying developers to eliminate the fire that the bug might result in if not addressed.
12. Utilize Functional Components
Utilizing functional components in React offers a variety benefits: There is less writing required and it's much easier to understand, and the beta version of the Official React documentation is currently being revised by using functional parts (Hooks), so you should definitely get used to using functional components.
Functional components mean that there is no need to fret about how to use the
or by using classes. It is also possible to manage your component's state easily by writing less code thanks to Hooks.
Most of the updated information you'll come across on React make use of functional components. This makes it easy to grasp and follow helpful guides and other resources produced by React's community members when you encounter issues.
13. Be Up-to-Date with Updates to React Version
In the coming years, new functionalities will be introduced, and older ones will be altered. One of the best ways to keep track of this is to keep an eye on the official documentation.
You can also join React communities on social media to get information about developments as they occur.
Staying current with the most current version of React will allow you to determine which times to optimize or alter your base code for most efficient performance.
Additionally, there are other libraries that are built on React that you should stay current and using including React Router, which is used for routing in React. Understanding the changes that these libraries can make will assist you in making the necessary modifications to your application as well as make it easier for everyone working on the project.
Furthermore, certain functions can become deprecated and certain keywords could be altered as new versions become available. To be on the safe side, you should always review the manual and guide in the event of changes.
14. Use a Fast, Secure Hosting Provider
If you'd like to make your web app available to everybody after you've built it, then you'll have to host it. It's essential to use a fast and secure hosting service.
Hosting your website gives you access to various tools that help you manage and scale your website easy. The web server on which your site is hosted allows that the data locally on your machine to be safely stored on the server. The overall benefit of hosting your site is that other people get to see the awesome stuff that you've put together.
There's a myriad of hosting platforms offering free hosting to developers such as Firebase, Vercel, Netlify, GitHub Pages, or more expensive services like Azure, AWS, GoDaddy, Bluehost, and so on.
Summary
When you are building your next web app with React take these top methods in mind in order to make using and managing the product effortless for both users and your developers.
What other React good practices do have that you don't know about but weren't included in this piece? Comment below. Have fun Coding!
- It is easy to set up and manage My dashboard. My dashboard
- Support is available 24/7.
- The top Google Cloud Platform hardware and network, powered by Kubernetes for maximum scalability
- A high-end Cloudflare integration that improves speed and security
- Reaching a global audience with as many as 35 data centers, and more than 275 POPs across the globe