The Laravel Built-In Client to communicate with External APIs (r)
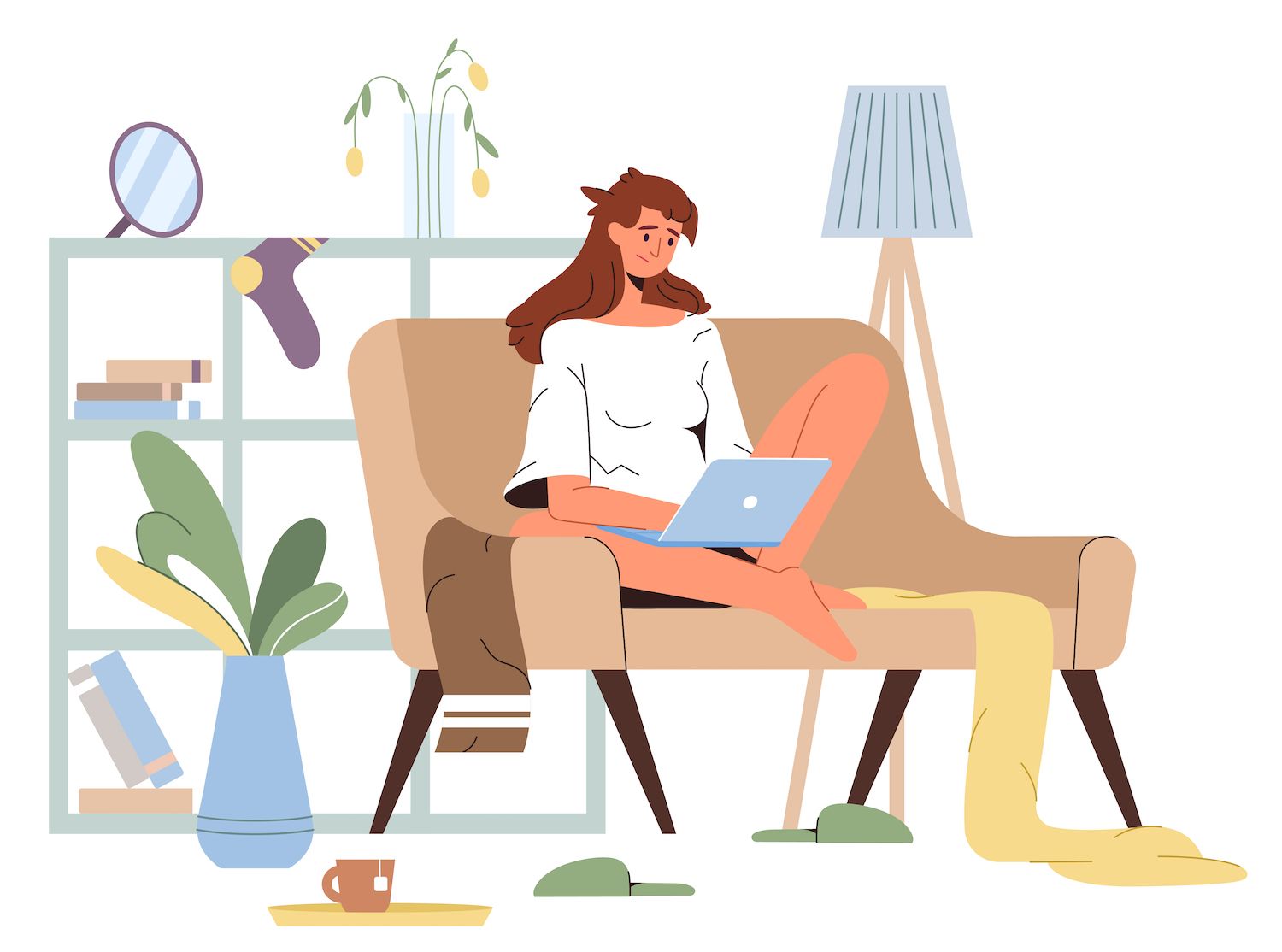
Share on
This article will look at how to use Laravel's HTTP client to make requests, analyze responses, create middleware and macros, and more.
Laravel HTTP Client does the hard work for you for APIs
Guzzle is an easy HTTP client designed for PHP. Guzzle supports different forms of requests like the GET
, POST
, PUT
, and DELETE
along with streaming features as well as multipart request. Utilizing the Guzzle HTTP client, sending both synchronous and asynchronous request to servers is feasible. Additionally, it includes a good middleware tool to alter the behavior of the client.
Laravel's HTTP client is a wrapper that is built upon Guzzle but with extra functionalities. It includes support for trying to retry failed requests, as well as some helper functions with JSON information. The majority of functions of Laravel HTTP clients is comparable to Guzzle.
Prerequisites
In the following sections we'll provide more information about the Laravel HTTP client. In order to follow the tutorial it will require:
- PHP and Composer are both installed
- Postman
How To Make Requests
For a better understanding of how to utilize an HTTP client to make an request, utilize a wide range of hosted APIs for example, like ReqRes.
Begin by installing the HTTP package when you are creating the application. Inside the App/Http/Controllers/UserController.php file, add the following code, starting with the use statement at the beginning of the file and the remaining code inside the index function.
use Illuminate\Support\Facades\Http;
return Http::get("https://reqres.in/api/users?page=2");
Note: For complex use cases, you can also transmit the request using headers via withHeaders method. usingHeaders
method.
In the same file make a new by using the code below:
function post()
$response = Http::withHeaders([
'Content-Type' => 'application/json',
])->post('https://reqres.in/api/users', [
'name' => 'morpheus',
'job' => 'leader',
]);
return $response;
Add a route to it in your routes/web.phpfile:
Route::get('post',[UserController::class,'post']);
Now, Postman can be used to check this method. Open Postman and add http://127.0.0.1:8000/post as the URL, with the type of request as GET
. After you hit send and you'll get the following response:
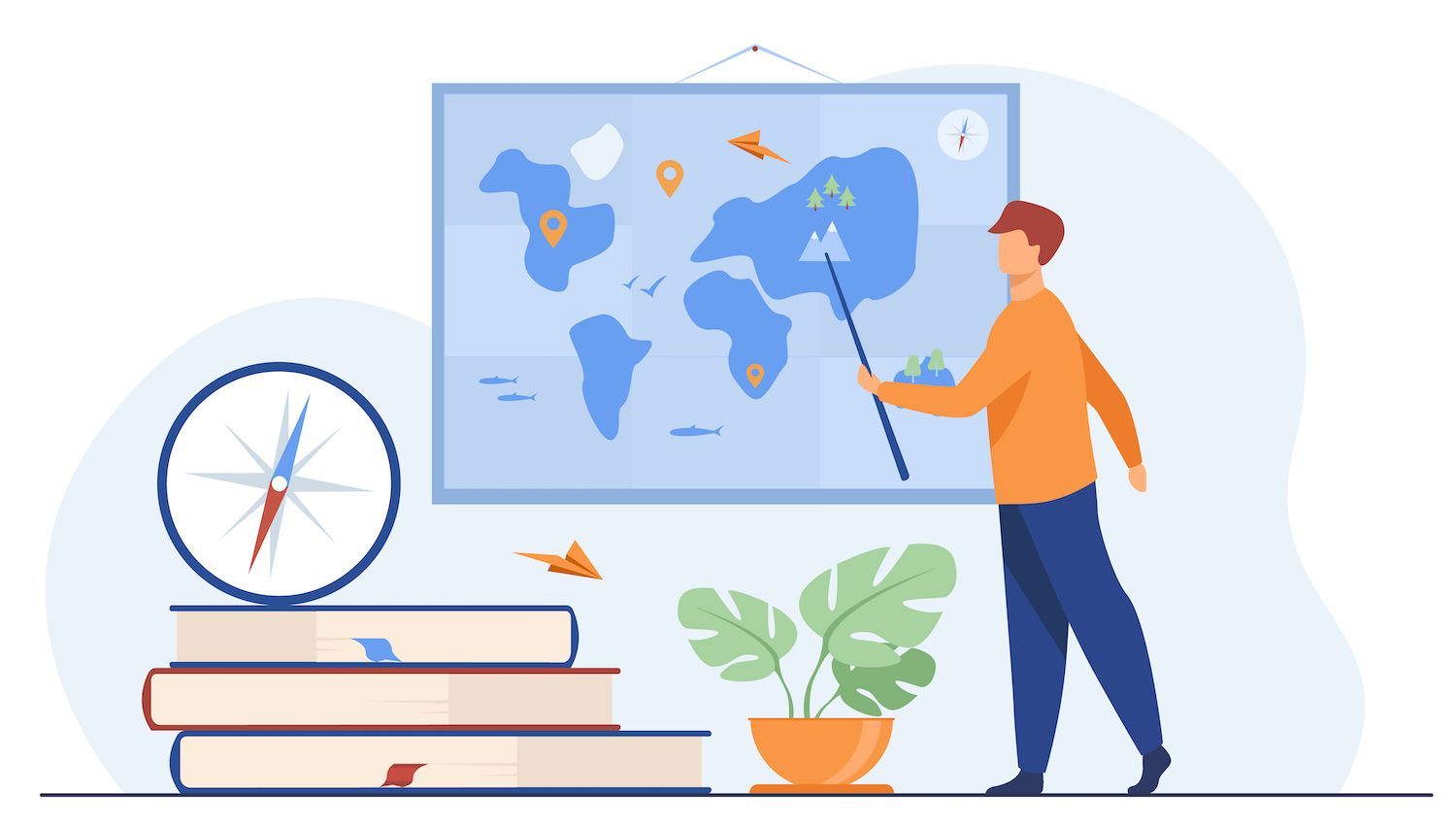
Concurrent Requests
Parallel requests significantly improve performance because you are able to access more data in the same amount of time. Laravel's HTTP client allows to carry out simultaneous requests by through the pool method.
Inside App/Http/Controllers/UserController.php, add the following code:
use Illuminate\Http\Client\Pool;
function concurrent()
$responses = Http::pool(fn (Pool $pool) => [
$pool->get('https://reqres.in/api/users?page=2'),
$pool->get('https://reqres.in/api/users/2'),
$pool->get('https://reqres.in/api/users?page=2'),
]);
return $responses[0]->ok() &&
$responses[1]->ok() &&
$responses[2]->ok();
After that, you can add the route inside in the routes/web.phpfile.
Route::get('concurrent',[UserController::class,'concurrent']);
The web browser displays this message when you visit the site:
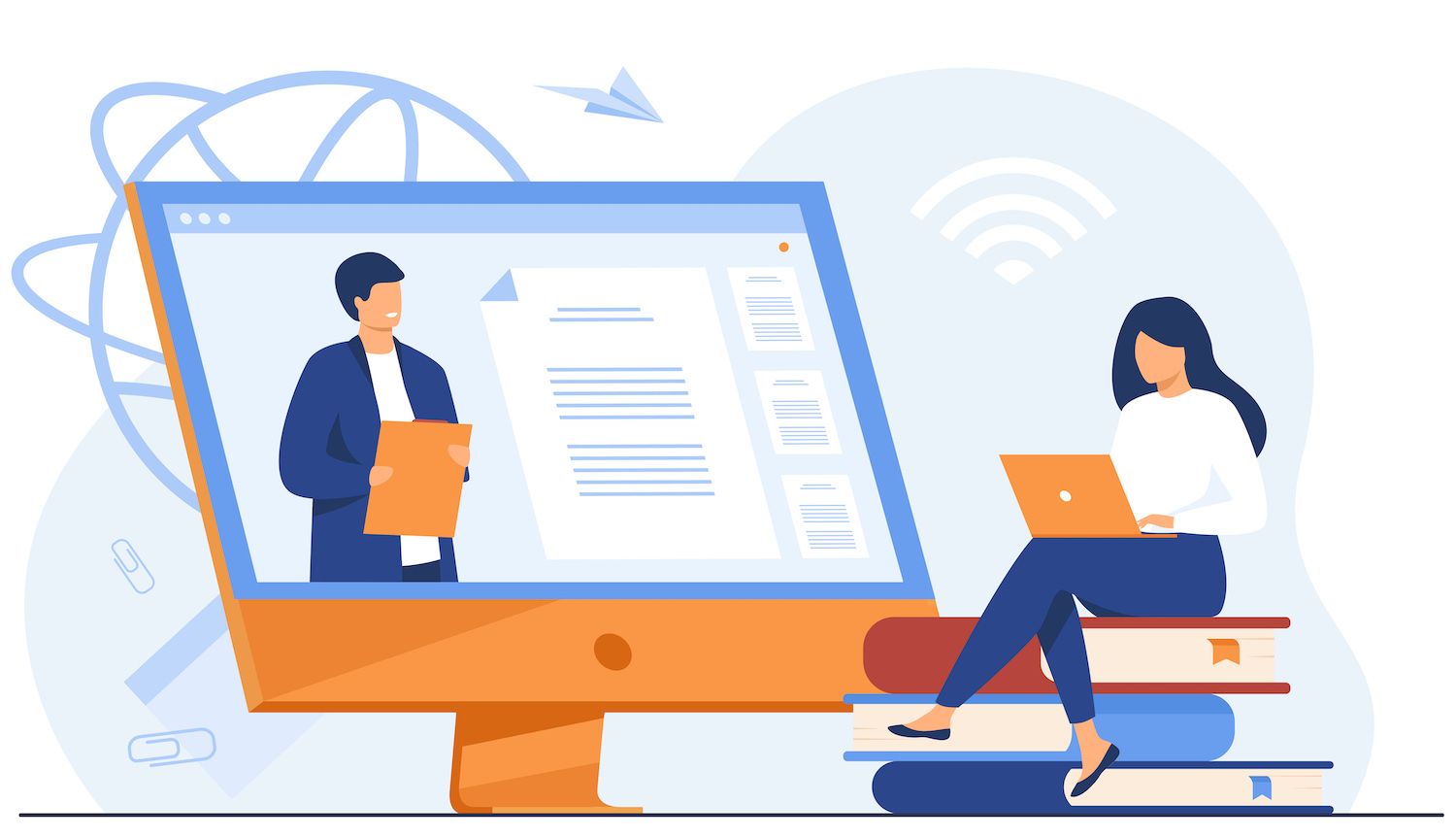
Macros Request Macros
Request macros are useful when interacting with common API routes.
To create the macro, you need to define the macro inside the boot method of the app/Http/Providers/AppServiceProvider.php file using the code below:
use Illuminate\Support\Facades\Http;
Http::macro('reqres', function ()
return Http::baseUrl('https://reqres.in/api');
);
NOTE: Make sure to include the use statement in the first line of your file.
You can then use the macro inside the UserController
by adding the below code:
function macro()
$response = Http::reqres()->get('/users?page=2');
return $response;
You can see that since the macro is already in place so you do not need to add the full URL each time.
Finally, you can add a route in the routes/web.php file using the code below:
Route::get('macro',[UserController::class,'macro']);
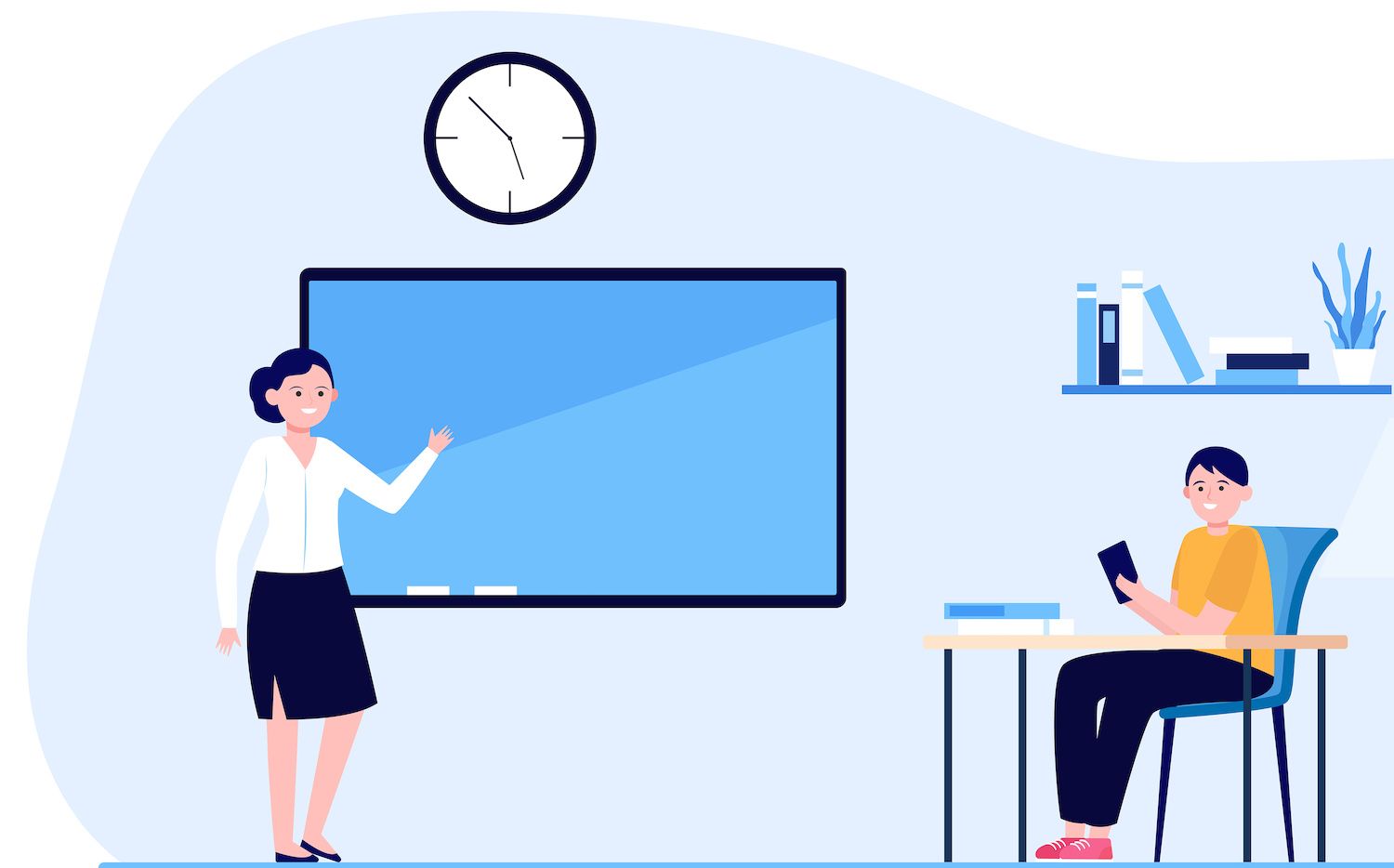
How To Decode Responses
To decode a response and ensure that an API request was valid, you can utilize the status option within the client. This method retrieves the status message by the server, and then display it.
To test this out, replace the previous macro code with the code below inside the App/Http/Controllers/UserController.php file:
function macro()
$response = Http::reqres()->get('/users?page=2');
return $response->status();
The status code 200 indicates that the request was completed successfully.
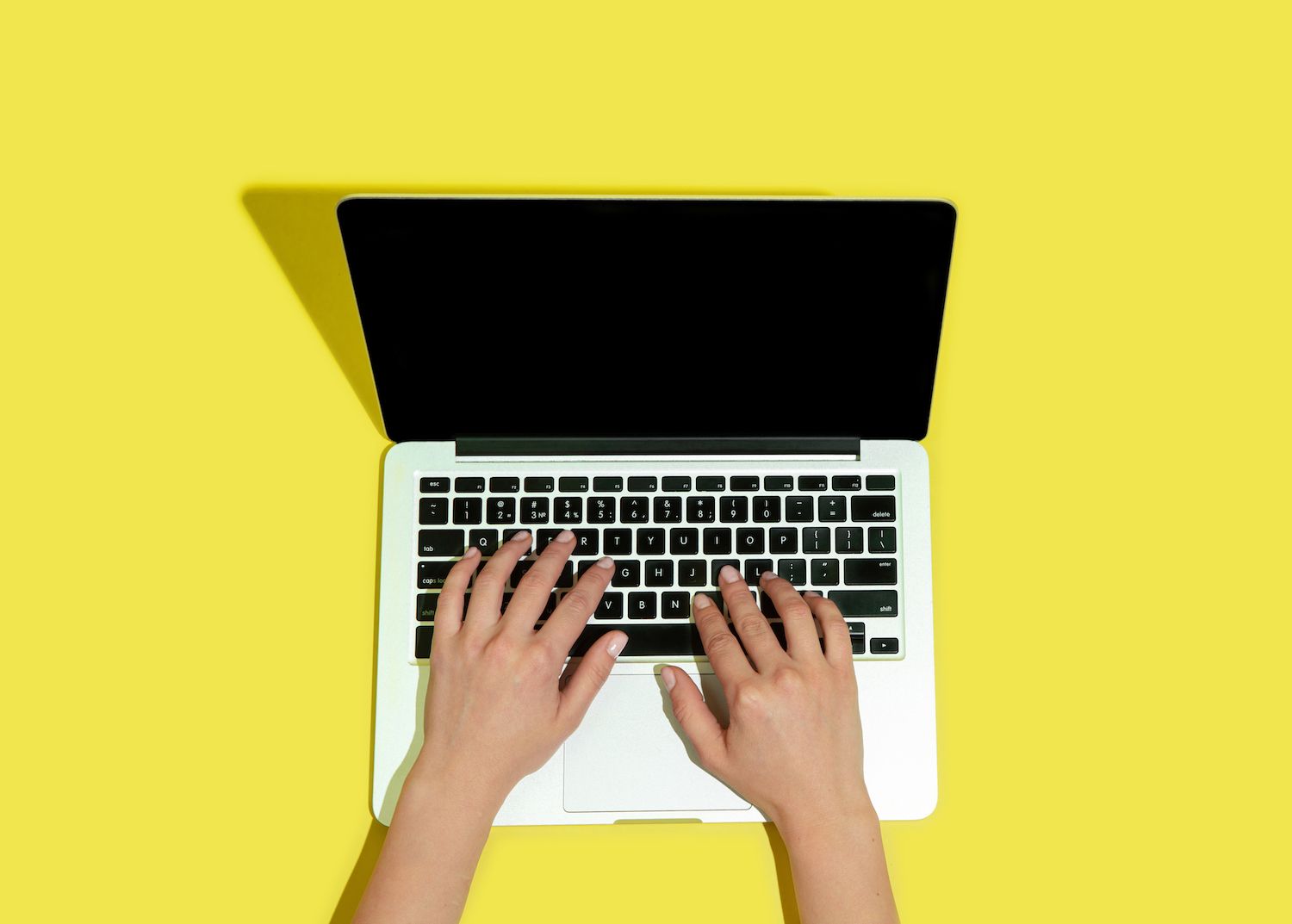
What is the best way to test JSON APIs
Laravel has several helpers to check the JSON APIs and their responses. The helper functions include JSON, GetJson, PostJson putJson and patchJson. deleteJson, and so on.
To comprehend the Testing better, create a test scenario that follows this GET
user's route. When you bootstrap the Laravel application then the Example Test is already created. Inside the tests/Feature/ExampleTest.php file, replace the existing code with the following:
getJson('/users');
$response->assertStatus(200);
The additional code retrieves the JSON information from the user's location and then checks whether the status code is 200 or not.
After adding the test code, execute this command inside your terminal in order to run the tests:
./vendor/bin/phpunit
When the tests are complete after the tests are completed, you'll find that the test was conducted twice and both were successful.
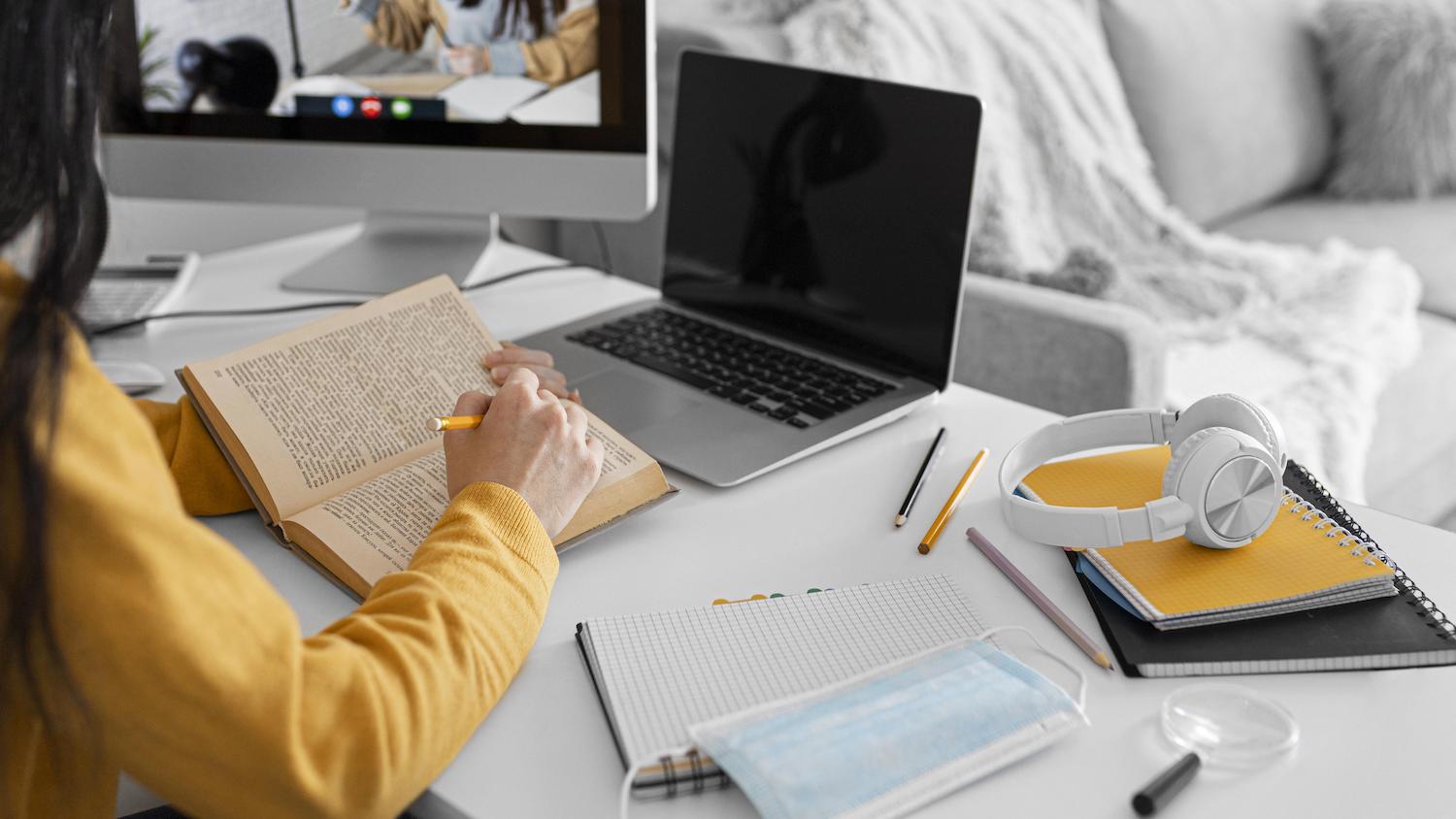
In the same way, you could check for different types of inquiries and employ other helper methods for more sophisticated testing.
How To Handle Events
- RequestSending that is prior to the request is sent.
- ResponseReceived, which is when a response is received.
- ConnectionFailed, which is when no response is received.
All three events include the $request
property to inspect the Illuminate\Http\Client\Request
instance, and ResponseReceived
has an additional $response property
. These properties are extremely useful for taking actions following an event. For example, you might need to send an email following a successful response.
To create an event and listener, navigate to the app/Providers/EventServiceProvider.php file and replace the listen array with the following code.
protected $listen = [
Registered::class => [
SendEmailVerificationNotification::class,
],
'Illuminate\Http\Client\Events\ResponseReceived' => [
'App\Listeners\LogResponseReceived',
],
];
Run the following command on your terminal:
php artisan event:generate
The above command will create the app/Listeners/LogResponseReceived.php listener. Replace the code from this file by the following above code:
info($response->status());
/**
* Handle the event. *
* @param \Illuminate\Http\Client\Events\ResponseReceived $event
* @return void
*/
public function handle(ResponseReceived $event)
The information log for the status code appears in the terminal.
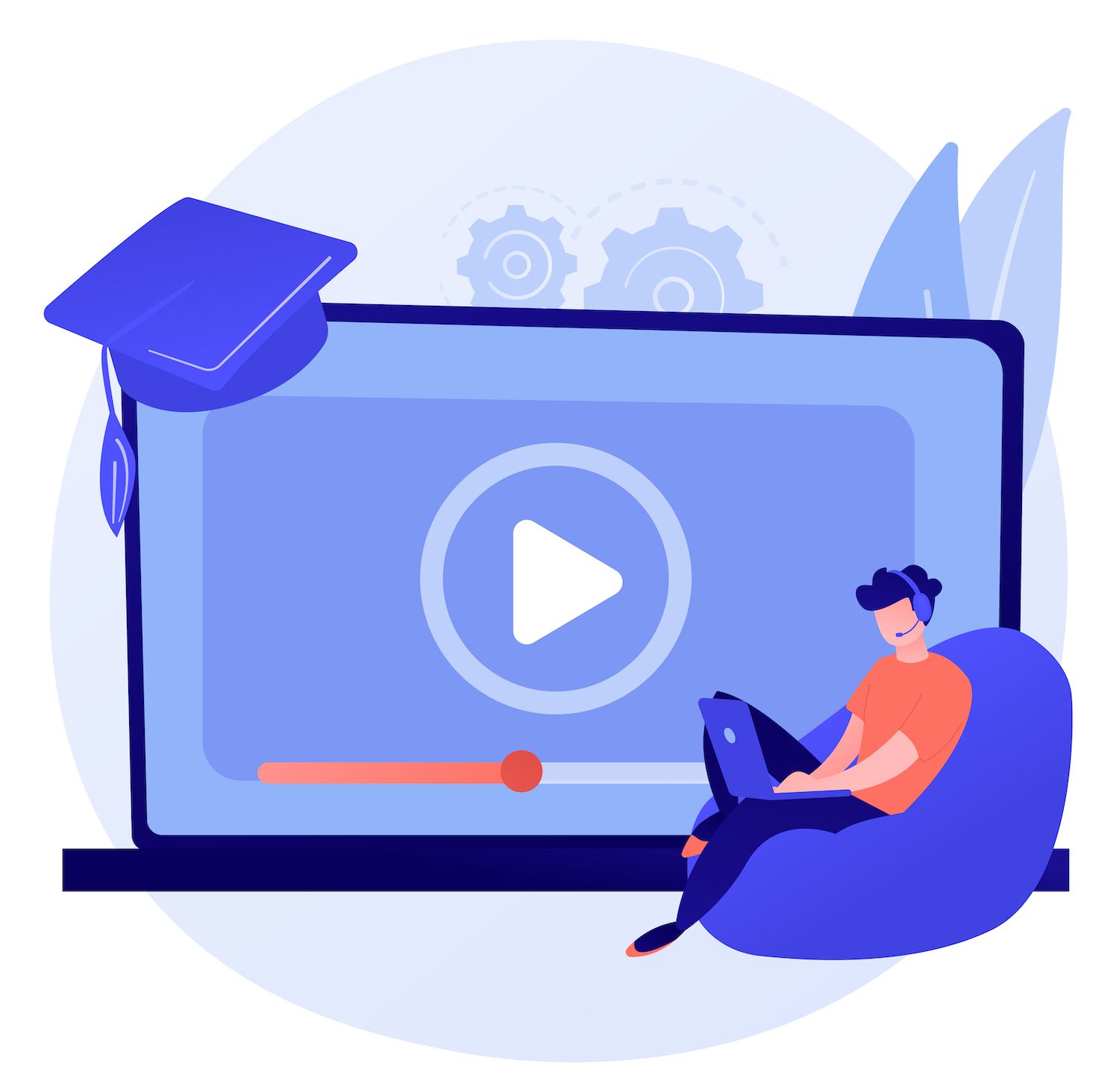
Summary
If a web site or application is made by an organisation or an individual developer, APIs play a crucial role in their success. However, using them can be a challenge.
- Simple setup and management on the My dashboard
- 24/7 expert support
- The best Google Cloud Platform hardware and network driven by Kubernetes to ensure maximum capacity
- A high-end Cloudflare integration that improves speed as well as security
- Global audience reach with the possibility of 35 data centers and 275 PoPs worldwide