The power of WordPress with Python and Redis - (r)
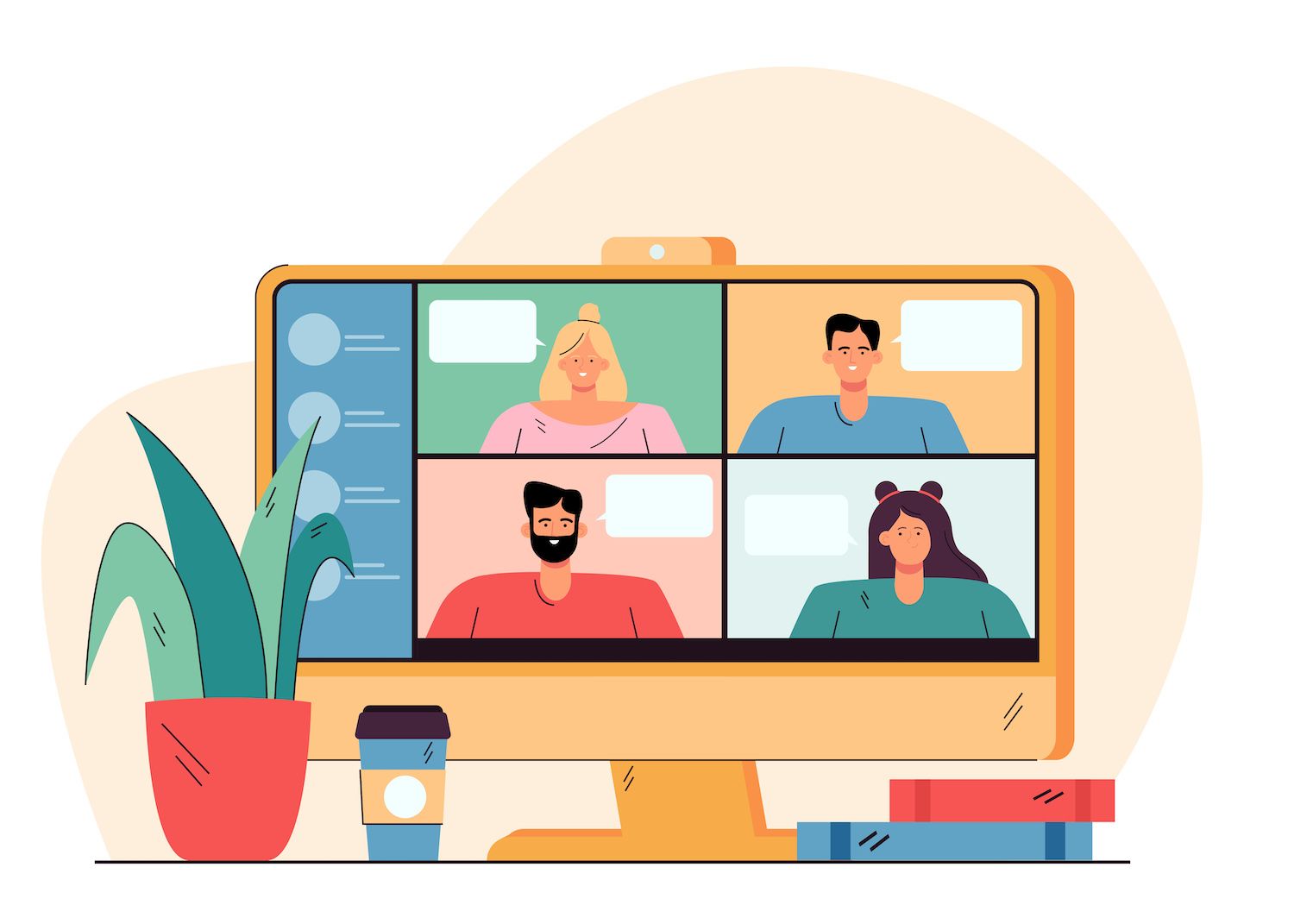
-sidebar-toc>
It is possible to bring the two worlds together when you manipulate that WordPress content with a Python software.
In this video, we demonstrate how to upload content directly onto Redis by creating an Python app that uses the popular redis-py library. We also show how to post through Redis using WordPress REST API.
What is Redis?
Redis is distinct from other NoSQL databases by the database storage system. It's usually called a data structure store as it can hold files that are similar to the kinds of data that are used in many programming languages. This includes sets, strings lists, dictionaries, and sets (or hashes). Apart from supporting basic data structures, Redis supports advanced data structures to perform tasks such as geolocation and stream processing.
Python app prerequisites
Prior to making your application it is necessary to download the following three things locally.
- Redis -Read the Redis official Redis installation instructions in case you require direction.
- Python along with pip -- As of Python 3.4 pip, an installer for the Python installation program, is added in default.
Once you have the necessary components installed then it's time to create the things work. Particularly, you're developing a Python app that takes the user's WordPress post in dictionary format and saves it to an Redis cache.
Create the Python app to store an article in the Redis cache
Redis cache can be described as a powerful caching mechanism for websites. It stores frequently requested information to provide faster, easier access. The cache is organized in a key-value data arrangement.
pip Install redis
Begin by installing the newly installed redis-py library. Set the Redis host and port address:
import redis
redis_host = 'localhost'
redis_port = 6379
Next, determine the key and value of the WordPress post as key/value pairs in an index. Here's an example
posting =
'ID': 1,
'post_author': 1,
'post_date': '2024-02-05 00:00:00',
'post_date_gmt': '2024-02-05 00:00:00',
'post_content': 'Test Post related blog post',
'post_title': 'My first post',
'post_excerpt': 'In this post, I will ...',
'post_status': 'publish',
'comment_status': 'open',
'ping_status': 'open',
'post_password': 'my-post-pwd',
'post_name': 'my-first-post',
Add to the code with an redis_dict()
function that will allow you to connect to your Local Redis server, and save the above post to the Redis cache, and then print the created values successfully to the console:
def redis_dict():
try:
r = redis.StrictRedis(host = redis_host, port = redis_port, decode_responses=True)
r.hset("newPostOne", mapping=post)
msg = r.hgetall("newPostOne")
print(msg)
except Exception as e:
print(f"Something went wrong e")
# Runs the function:
if __name__ == "__main__":
redis_dict()
If you did not launch Redis from within Docker or Docker itself, you can invoke Redis' command line interface. Redis Command Line Interface by using these commands:
redis-cli
Run your Python script:
Python main.py
When you execute the script, it will add the script in the Redis value store for key values. It should show the following response in the console of your terminal:
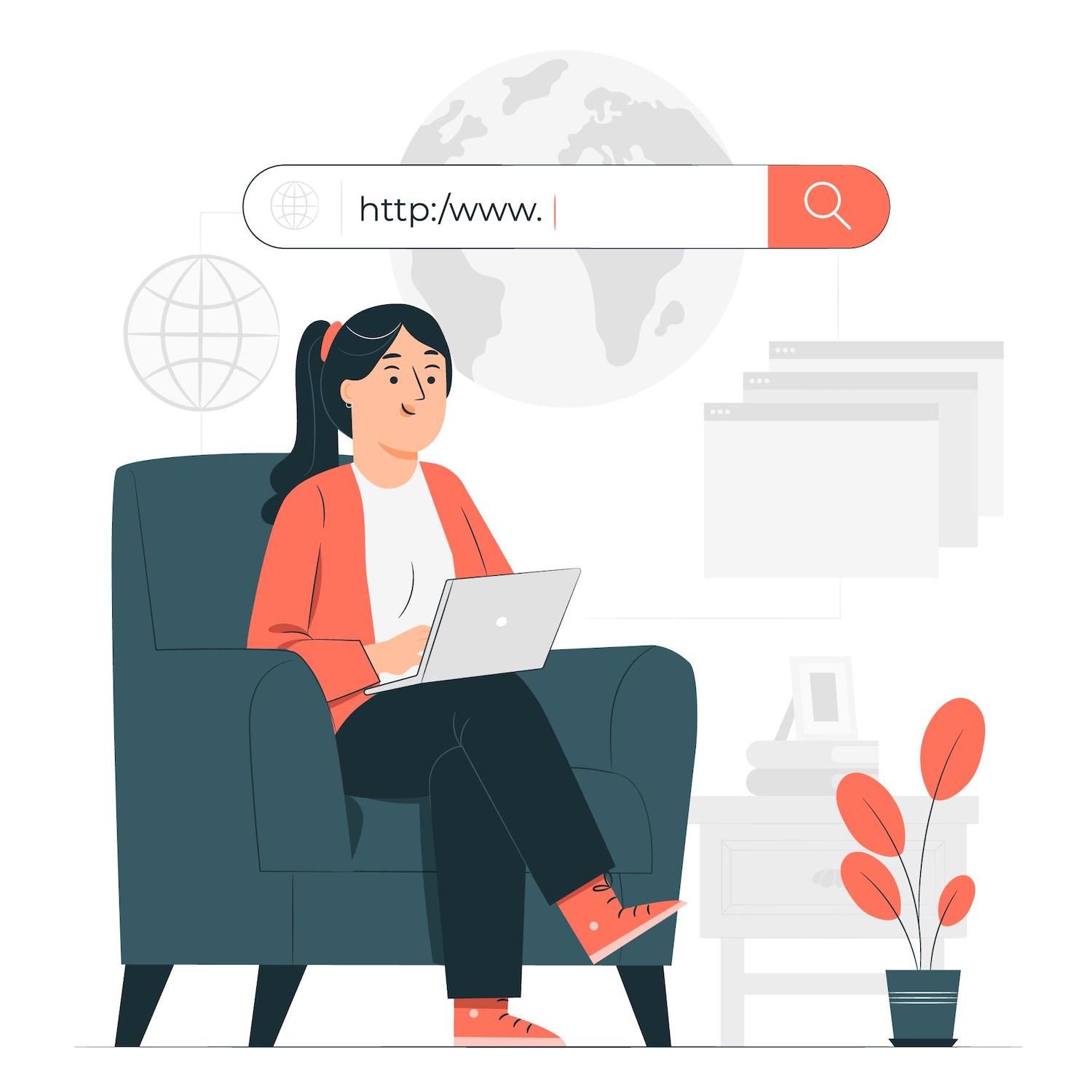
The post has been saved on your home Redis database.
Upload a post to WordPress by using the REST API
WordPress REST API WordPress REST API is an assortment of endpoints that to call via your application in order to connect with WordPress. We utilize the the post-endpoint for creating a blog post within WordPress.
Step 1: Create the password for your application in WordPress
The WordPress API needs an application password that allows your application access to data on the WordPress website. The password consists of a 24-character secret key, which you need to include with every call to the REST API.
Create an application's password using the User Profile page of the WordPress Dashboard. You are able to assign a user-friendly name for each password you create, but you won't be able to view the password once you have created it (so take a photocopy now):
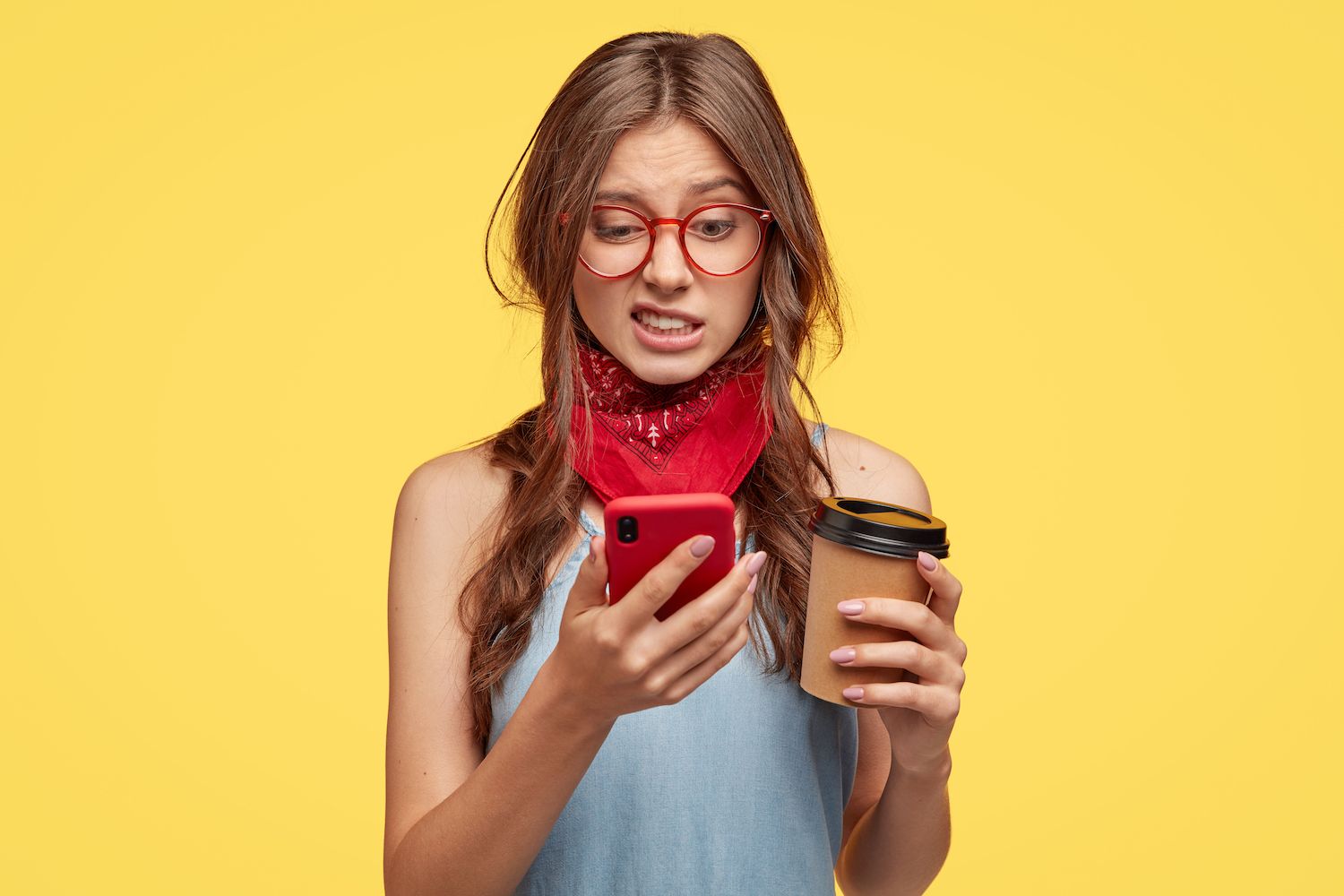
Step 2: Post on WordPress with your Python app
The first step is to install first the Python Requests library for making the HTTP connection to WordPress API. For this, you must run this command in the terminal:
request for pip installations
In the Python-redis folder, create an app.py file. app.py. After that, you can open the file using the text editor you have installed.
Start by installing the requests, json and bases64 modules:
import requestsImport JSON import Base64
Determine the API base URL as well as your WordPress username as well as your password. For the password variable, select the password for your application that you generated using WordPress:
url = 'http://localhost/wp-json/wp/v2'
user = ''
password = ''
Then, sign in with your the user
to create a password
, encode the result and pass it to the Request headers:
creds = user + ":" + password
token = base64.b64encode(creds.encode())
header = 'Authorization': 'Basic ' + token.decode('utf-8')
Here's the body of the post:
blog =
'author': 1,
'date': '2024-02-05 00:00:00',
'date_gmt': '2024-02-05 00:00:00',
'content': 'Test Post related blog post',
'title': 'My second post',
'excerpt': 'In this post, I will ...',
'status': 'publish',
'comment_status': 'open',
'ping_status': 'open',
'password': 'my-post-pwd',
'slug': 'my-second-post',
Create your POST request to the API and a command to show the status of the response
r is requests.post(url + '/posts' headers=header, json=post)
print(r)
You can run your script by using this command inside the terminal
Python app.py
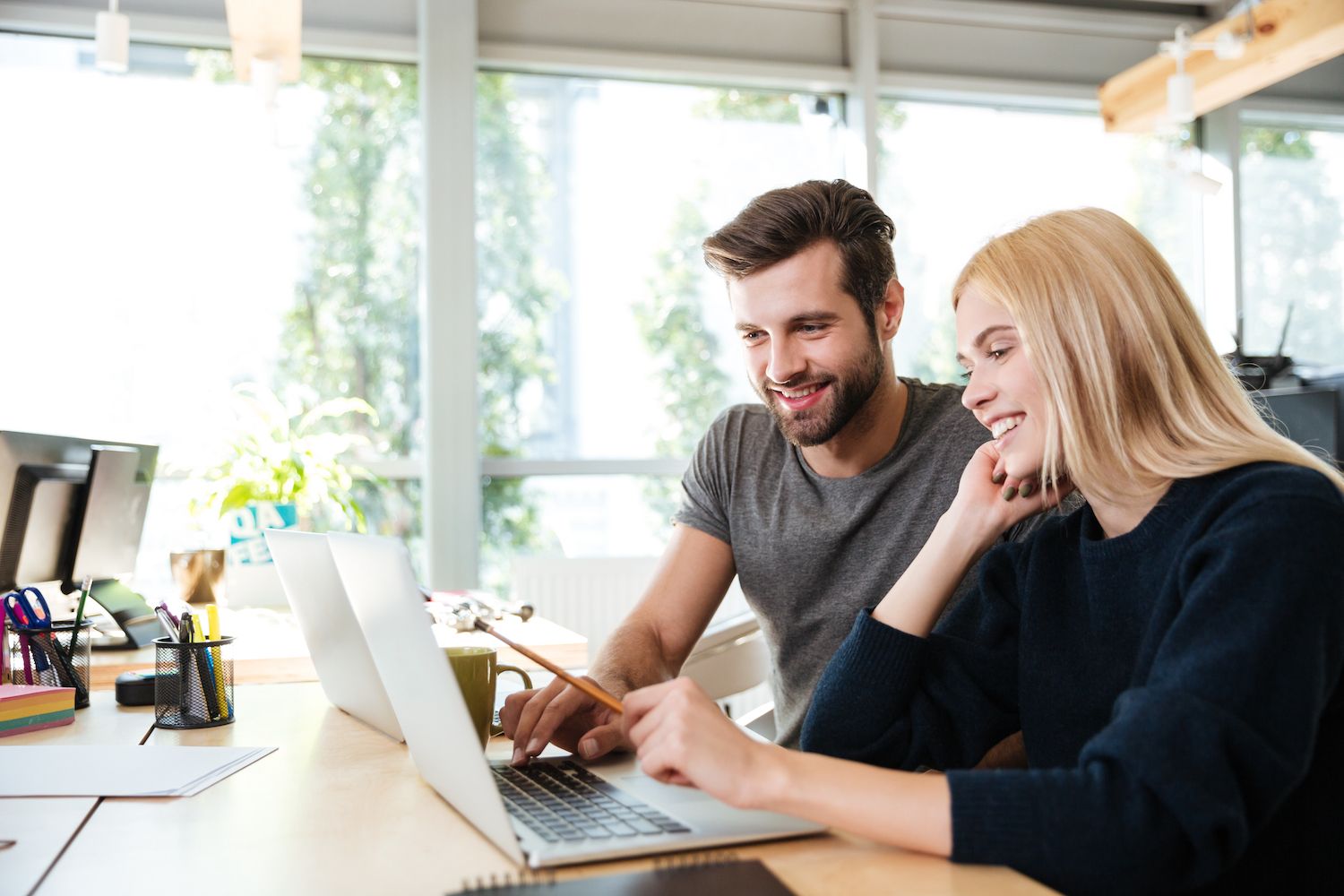
Make use of Redis cache directly within WordPress
WordPress websites are able to use Redis cache. Redis cache to temporarily save items, like Pages, posts, or even users. This object is then accessible from the cache whenever it is required. This saves precious time, decreases latency and increases the capacity of the website to handle more traffic.
Customers can use Redis to purchase
Installation of the Redis plugin
Let's say, for example, we download Redis Object Cache, for example. Redis Object Cache plugin on your local WordPress site.
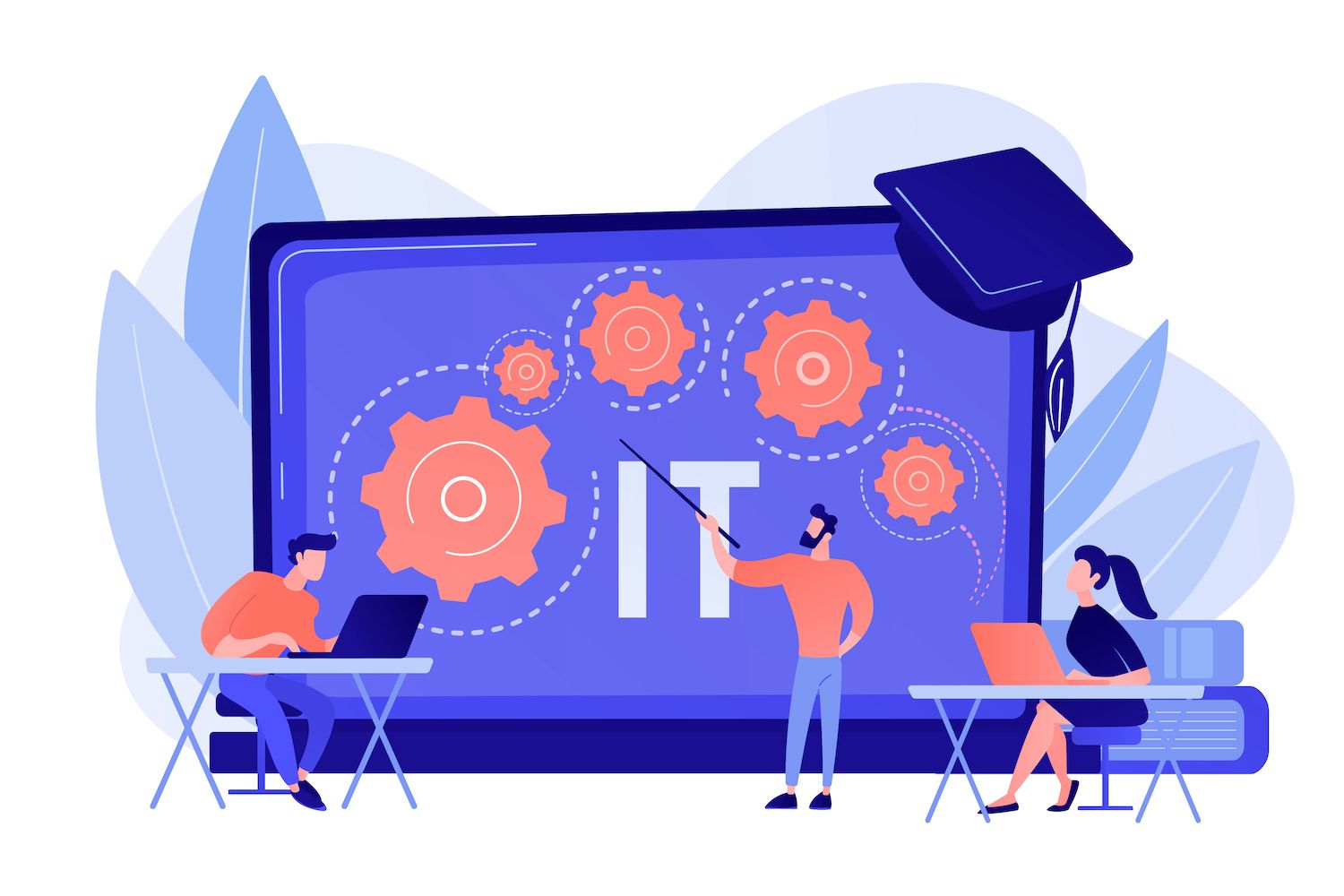
Start the wp-config.php file in a text editor and add this code into the section for customizing variable configurations:
define('WP_REDIS_CLIENT', 'predis');
define('WP_REDIS_HOST', 'localhost');
define('WP_REDIS_PORT', '6379');
Note: The address of your Redis host will depend on the server configuration.
Navigate to the Settings > Redis within Redis on the WordPress dashboard. You should see something similar to this:
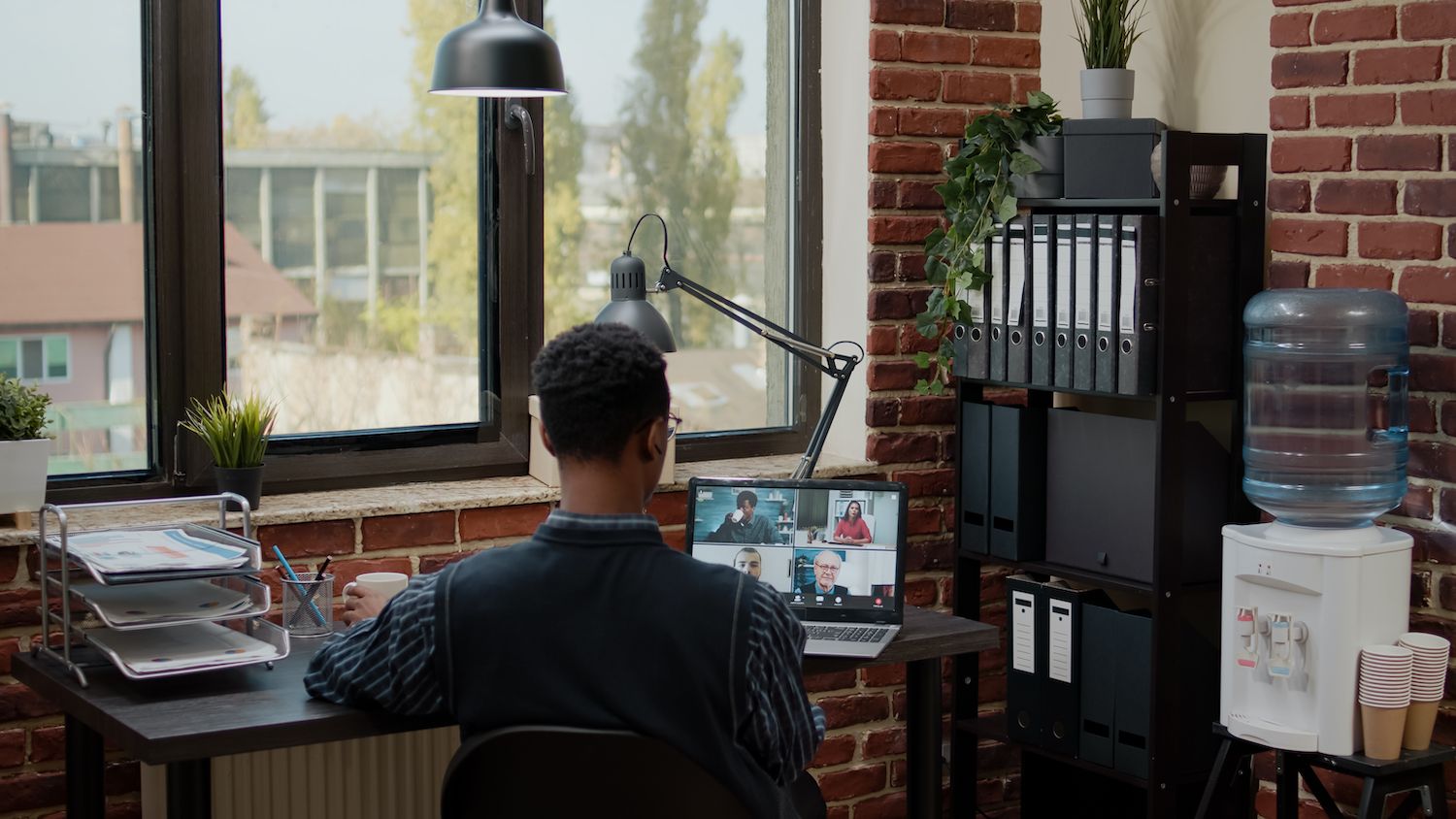
Redis cache has been replaced. Redis cache has since successfully replaced the previous MySQL database.
Furthermore, the frontend WordPress site uses the same cache that is used in that used by the backend Python application. It is possible to test this using a different terminal, and then running this command:
Redis-cli Monitor
As you navigate your site your website, the requests from your site will be result in a command prompt
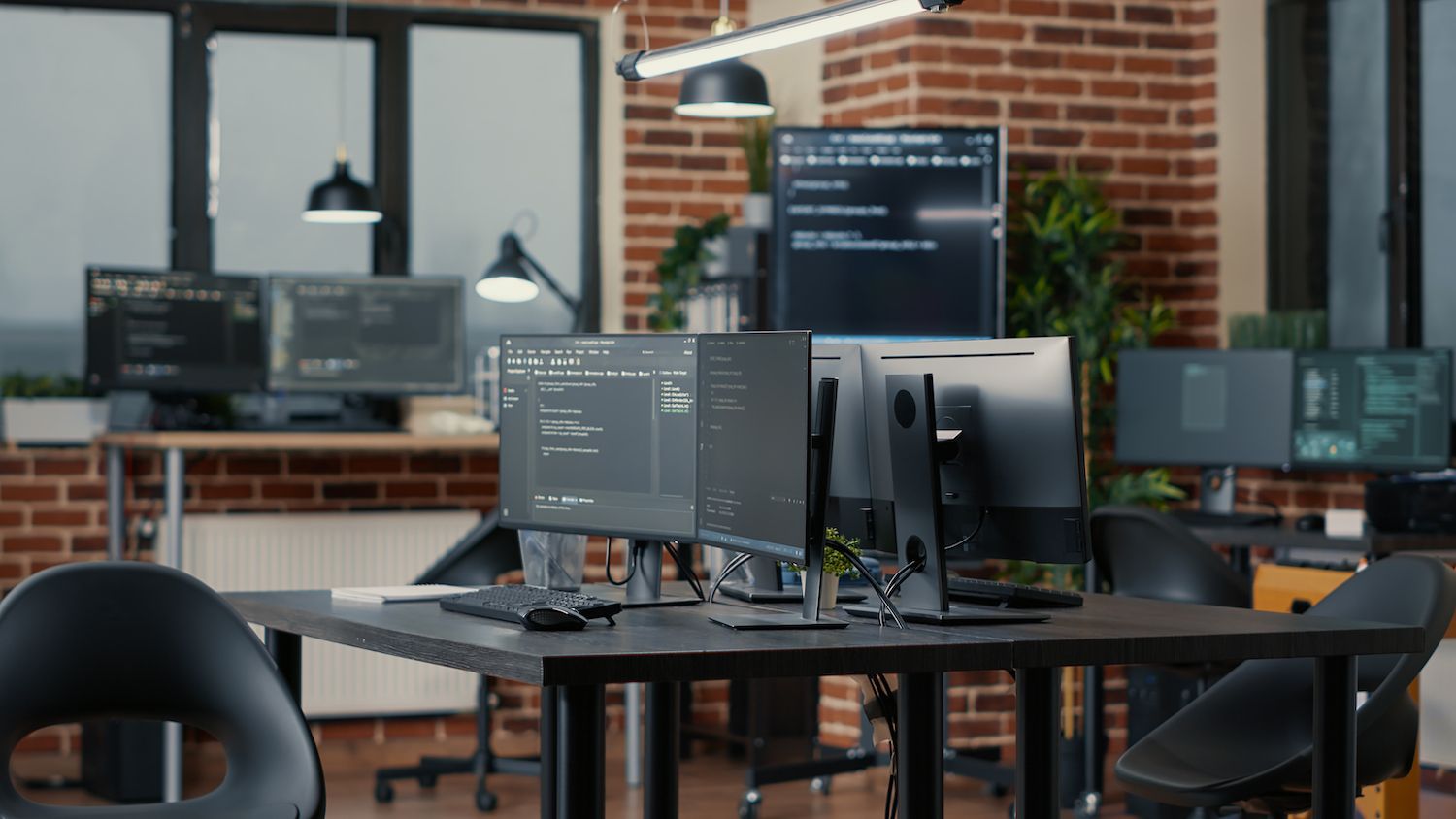
Now that both the front and back end are aligned, you are able to add a new post to WordPress using the Python app through the API for REST.
In order to do this, change the POST object within app.py to include the new post. Then, execute python app.py
to add the post to the cache.
Summary
In this tutorial, we learned how to connect an Redis database to the Python application using the Redis Python client. The client is compatible with a range of formats for Redis data stores including lists, sets dictionary, and various other command data types.
We also saw how it is possible to incorporate Redis into a WordPress site via the REST API and Redis Object Cache. Redis Object Cache plugin.
The possibility of using Redis memory cache to power your site makes it a potent and flexible software for developing. Redis is extraordinarily effective at increasing the speed of your database queries as well as the performance of your site as well as the overall satisfaction of users.
Steve Bonisteel
Steve Bonisteel is a Technical Editor for the site. He began his career in writing as a print journalist, chasing ambulances and fire engines. The journalist has covered internet-related technologies since the mid 1990s.