There are six things you must know to master Laravel Eloquent (r)
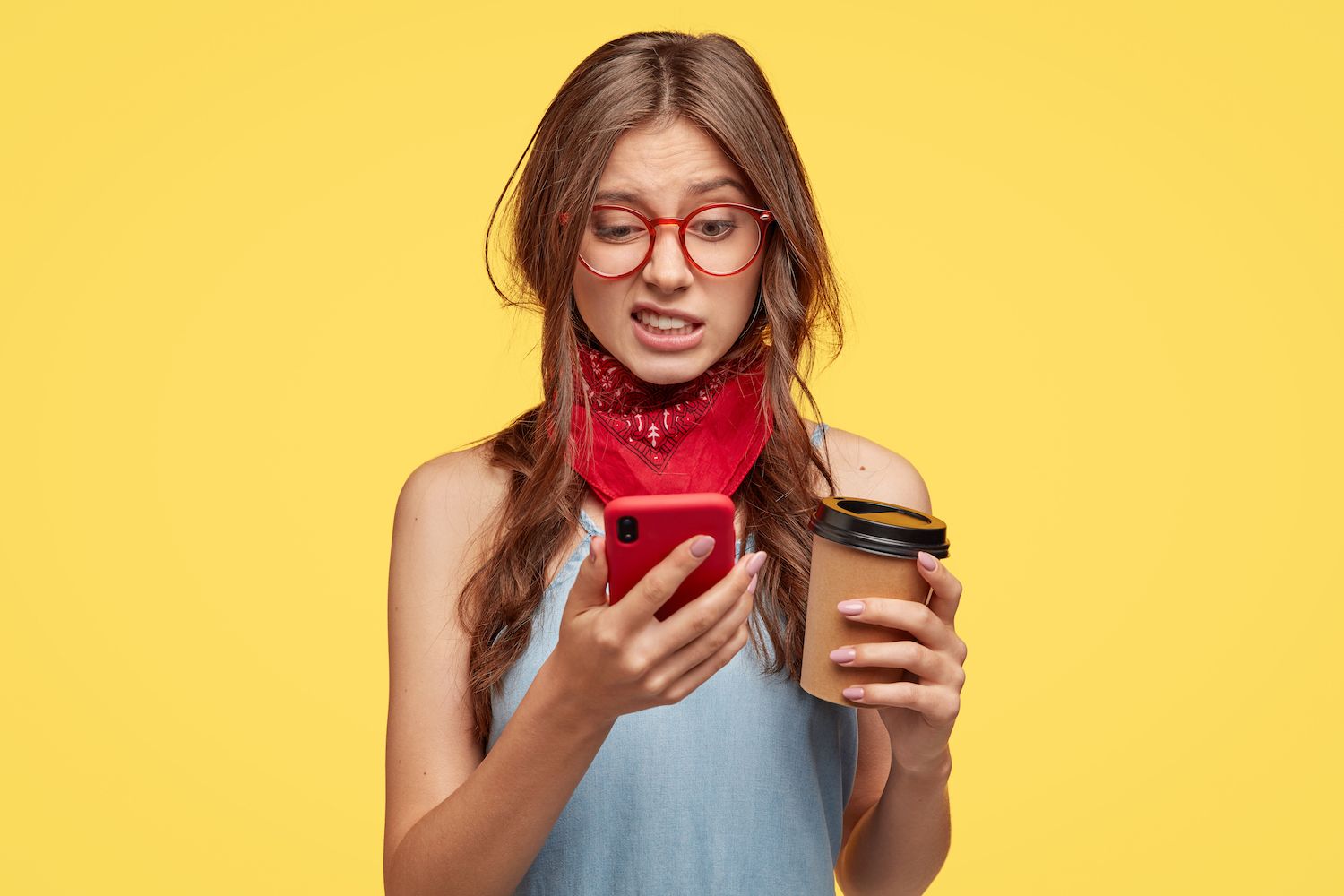
-sidebar-toc>
Eloquent speedily creates the ability to read, write, update and delete operations on databases from applications. In Eloquent you create models that mirror database tables using those models build your queries.
The article focuses on six aspects that make Eloquent's powerful capabilities: query scopes relationships, mutators and accessors models, collections, deletion of model and factories. It covers what each feature does with practical examples. We hope that you will be able to take these lessons to kick-start your understanding of Laravel Eloquent.
1. Eloquent query scopes
When building an application, it is not uncommon to encounter situations that require you to use the same condition more than once. Rewriting code in each case could increase the risk of mistakes and render your code untidy. Laravel helps solve this issue by wrapping these types of conditions into modular statements known as scopes.
Queries are methods of creating database logic in a model and reusing query logic.
Here is an example of the query scope. Let's say you are planning to build a software development platform for your company that records completed and ongoing capabilities. This condition can be used to track only features that are ongoing:
$onGoing = Project::where('ongoing', 1)->get();
You may need this condition on other application pages for example, the statistics page. The query scopes enable a page to reuse the above condition which makes your query easier and making the code simpler.
Here's how to use a query scope for this particular scenario:
class Features extends Model
public function scopeOngoing($query)
return $query->where('ongoing', 0);
Use the following code to implement that scope:
$onGoing = Feature::ongoing()->get();
There are two kinds of scopes: global and local.
Global scopes
Global scopes allow the addition of restrictions to any query in the model. For instance, you can include a filtering condition that options based on your team leader's name in every query within your model.
Local areas of coverage
Local scopes permit the creation of common constraints for reusability. In particular, you might require the program to provide any features with bugs. The code could implement one of these local scopes:
namespace AppModels;
use IlluminateDatabaseEloquentModel;
class User extends Model
public function scopeBugged($query)
return $query->where('bugged', '>', 1);
The above code will return all the features that have unfixed issues.
2. Relationships with eloquence
Relations in Eloquent enable you to connect to tables in a simple manner. As an example, a particular item on an online store may have inventory, price views, reviews and views included. With Eloquent, you can easily manage these relationships even when their records are in different tables.
It is possible to define relationships as methods on model classes just as the Eloquent model. Some commonly used Eloquent relationships comprise one-to-1, inverse and polymorphic.
One-to-one
Here's an example of a basic one-to-one relationship that associates a product model with an inventory.
public function Inventory()
return $this->hasOne('AppInventory');
In the example code above In the code above, the Inventory()
method calls the hasOne()
method on the product model. The method checks whether the product is in stock.
Inverse
Eloquent can also allow users to establish an inverse relation. In the case of, for example, if you wish to retrieve items based on their view counts. The reverse relationships will give you those products that generate the highest interest from web site's viewers. You can use is the isTo()
method, which is the reverse of hasOne()
. This code illustrates the concept.
public function product()
return $this->belongsTo('AppProduct');
In the code above, Eloquent matches the product_id
to the number of pages it's passed. The product_id
will then be used to retrieve other parameters, such as the price or inventory.
Polymorphic
When it comes to application development, the relationships can be complex. There are times when you've got a model that belongs to several types of model. For instance, product and inventory models can both be polymorphic to a model of images.
Class Image extends Modelclass Product extends Modelclass Product extends Model
/**• Get the image to use on the page of the product. */
public function image()
return $this->morphOne(Image::class, 'myimage');
class Inventory extends Model
/**
* Get the image to use on the inventory page. */
public function image()
return $this->morphOne(Image::class, 'myimage');
The code above uses the morphTo()
method to retrieve the parent of the polymorphic model.
3. Mutators, accessors and other eloquently mutators
Accessors and mutators permit you to alter data while storing or retrieving it. Mutators modify data before saving it. Accessors alter data when retrieving it.
If you want to store names in lowercase within your database, then you could make a mutator that will perform that conversion. If you wish to show the user's first name and their last name on your app pages it is possible to build an accessor that accomplishes that.
Below is an example of an obliterator which capitalizes names before making them available for saving.
Class User extends Model
/*** Mutators capitalize first and last name. */
public function setFirstNameAttribute($value)
$this->attributes['first_name'] = ucfirst($value);
public function setLastNameAttribute($value)
$this->attributes['last_name'] = ucfirst($value);
Here is an example of accessors that combine the first name and the last initial of the name used by the user.
class User extends Model
/**
* Accessor combining both names. */
public function getFullNameAttribute()
return ucfirst($this->first_name) . ' ' . ucfirst($this->last_name);
4. Collections of eloquent words
Eloquent collections handle procedures that provide different model-specific results. This class is found in IlluminateDatabaseEloquentCollection
.
Similar to arrays, it is possible to traverse collection. Here's a quick iteration.
use AppModelsProduct;
$products = Product::where('availability', 1)->get();
foreach ($products as $product)
echo $product->name;
Collections are more powerful than arrays, as they allow you to do more complicated operations with these types of arrays because you can do more sophisticated operations with them. For instance, you can display the list of all the available products and skip all that are not "active."
$names = Product::all()->reject(function ($product)
return $product->active === false;
)->map(function ($product)
return $product->name;
);
Below are a few ways that the collection class gives you.
Includes
The contains()
method checks whether the code is in a specified mode, as shown in the code below:
$products->contains(1);
$products->contains(Product::find(1));
All
The all()
method returns all models that are within the collection as shown below:
$collection = Product::all();
Other methods are also supported by the collection class.
5. Eliminate the models that have been criticized.
With Eloquent you can create models that aid in the creation of queries. But, there are times when you have remove models in order in order to make your application more effective. To accomplish this, simply use delete
on the model's instance.
use AppModelsStock;
$stock = Stock::find(1);
$stock->delete();
The above code removes the Model Stock
in an application. This is a permanent removal that cannot be undone.
Soft delete
Another function that Eloquent has is the ability to soft delete models. When you remove the model, it doesn't delete it from your database.
Then, you flag it with deleted_at
to show the date and date of the delete. It is crucial in cases where you wish to remove certain database's records, like the ones that are not complete, without permanently removing them. It assists in cleaning Eloquent's query results without adding extra conditions.
It is possible to enable the soft delete feature by including softDeletes as softDeletes
trait to a model, and then adding an deleted_at
column on the related database table.
Adding soft delete to a model
You enable model soft deletes by adding the IlluminateDatabaseEloquentSoftDeletes
trait, as shown below.
namespace AppModels;
use IlluminateDatabaseEloquentModel;
use IlluminateDatabaseEloquentSoftDeletes;
class Flight extends Model
use SoftDeletes;
How can I include a delete_at column
Before you can start using soft delete, your database should include a delete_at
column. You add this column with the Laravel Schema
builder assister method as shown below:
use IlluminateDatabaseSchemaBlueprint;
use IlluminateSupportFacadesSchema;
Schema::table('users', function (Blueprint $table)
$table->softDeletes();
);
Schema::table('users', function (Blueprint $table)
$table->dropSoftDeletes();
);
The addition of an delete_at
column that is modified with respect to the dates and times, in case of a successful soft delete operation.
What is the best way to add soft deleted models
If you want query results to include soft-deleted models, you should add to the query usingTrashed()
method to the query. The following example demonstrates how:
$stocks = Stock::withTrashed()->where('stock_id', 20)->get();
The query above will also incorporate models using the deleted_at
attribute.
How do I retrieve only soft deleted models
Eloquent also allows you to download soft-deleted models only. You can do this by calling the OnlyTrashed()
method, such as:
$Stock = Stock::onlyTrashed()->where('stock_id', 1)->get();
How do I restore models that have been soft deleted?
It is also possible to restore deleted soft models by using the restore()
method.
$stocks = Stock::withTrashed()->where('stock_id', 20)->restore();
The delete_at
field of models that have been soft deleted to null. If the model isn't soft deleted, it will leave the field in its original state.
6. Eloquent Factories
Model factories within Laravel create dummy data that is used to test your app or create a database seed. To implement this, you create a model in the factory class like the one shown below. The code snippet makes a model factory, which creates fake suppliers of products and their prices.
namespace DatabaseFactories;
use IlluminateDatabaseEloquentFactoriesFactory;
use IlluminateSupportStr;
class StockFactory extends Factory
public function definition()
return [
'supplier_name' => fake()->name(),
'price' => fake()->numberBetween($min = 1500, $max = 6000),
];
The definition()
method in the example above returns a set of attribute values that Laravel utilizes when creating the model. The fake helper helps the factory access PHP's library, Faker.
Summary
Eloquent makes the tasks of developing applications in Laravel easier. Eloquent is equally efficient in the creation of complex or simple queries due to the implementation like relationships. The ease of creating functional dummy data using factories allows developers who want to create robust tests for their applications. In addition, Eloquent Scopes assist simplify complex queries by keeping the code neat.
While this article has covered just six principal features, it offers other useful features. Its use of shareable and reusable models is a widely-used tool for developers. Furthermore, the ease of using Eloquent query creates Laravel a developer-friendly framework -even for those who are new to the field.
Steve Bonisteel
Steve Bonisteel is a Technical Editor at who began his writing career as a print journalist who chased ambulances and fire engines. The journalist has covered Internet-related technology since the late 1990s.