Utilizing Node.js Utilizing WordPress for Building Dynamic APIs (r)
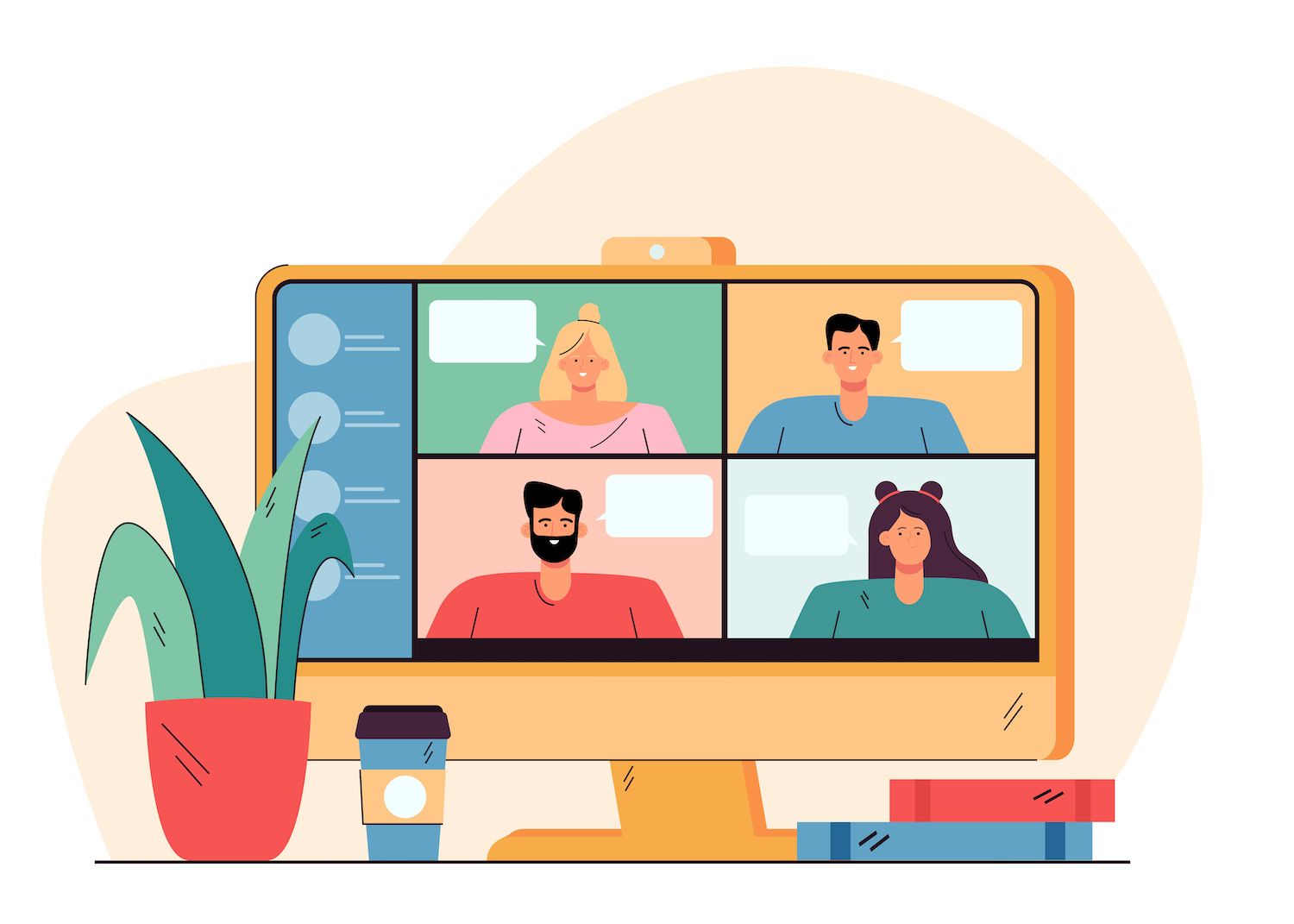
-sidebar-toc>
However, what's the point in mixing Node.js with WordPress?
Imagine you've got a WordPress website, and would like to design a unique dashboard. It could show real-time data from your site, such as recent posts, comments numbers, as well as user activity.
Here's where Node.js has a crucial role to play. This tutorial walks you through the process of setting to set up Node.js endpoints. They use the WordPress REST API to perform various tasks such as posting new posts, moderating comments, website customization and customized WordPress website administration.
Prerequisites
To follow along with this tutorial, we presume you've got:
- Basic understanding of Node.js.
- WordPress along with Thunder Client VS Code. Thunder Client VS Code extension installed.
Control WordPress Posts with Node.js
In WordPress The primary tasks include creating, updating, and the removal of posts. This guide will show you how to create specific endpoints for the above actions. It also explains ways to send demands to the the /posts
endpoints for each action.
Making the New Post in WordPress
For creating a new post in WordPress with the REST API. Make a POST call to the /posts
endpoint. Within the body of the request, you need to give the information for your WordPress post in JSON format.
After that, you can create a new route in your server file to create an additional post. The route's code looks like this:
app.post("/add-post", async (req, res) =>
try
const postID = req.body.id
const resp = await axios.post(`https://yourdomain.com/wp-json/wp/v2/posts/$postID`, req.body)
if(resp.status !== 200) throw "Something went wrong"
catch (err)
console.log(err)
)
This code creates an endpoint /add-post
in your app. When a request is sent to this endpoint, it detaches the post ID from the request body, and sends a POST request to your WordPress website. Remember to replace https://yourdomain.com
with your WordPress domain.
You can test this using tools like Thunder Client in Visual Studio Code. Make sure your JSON content of the request is correct for you to avoid any error.
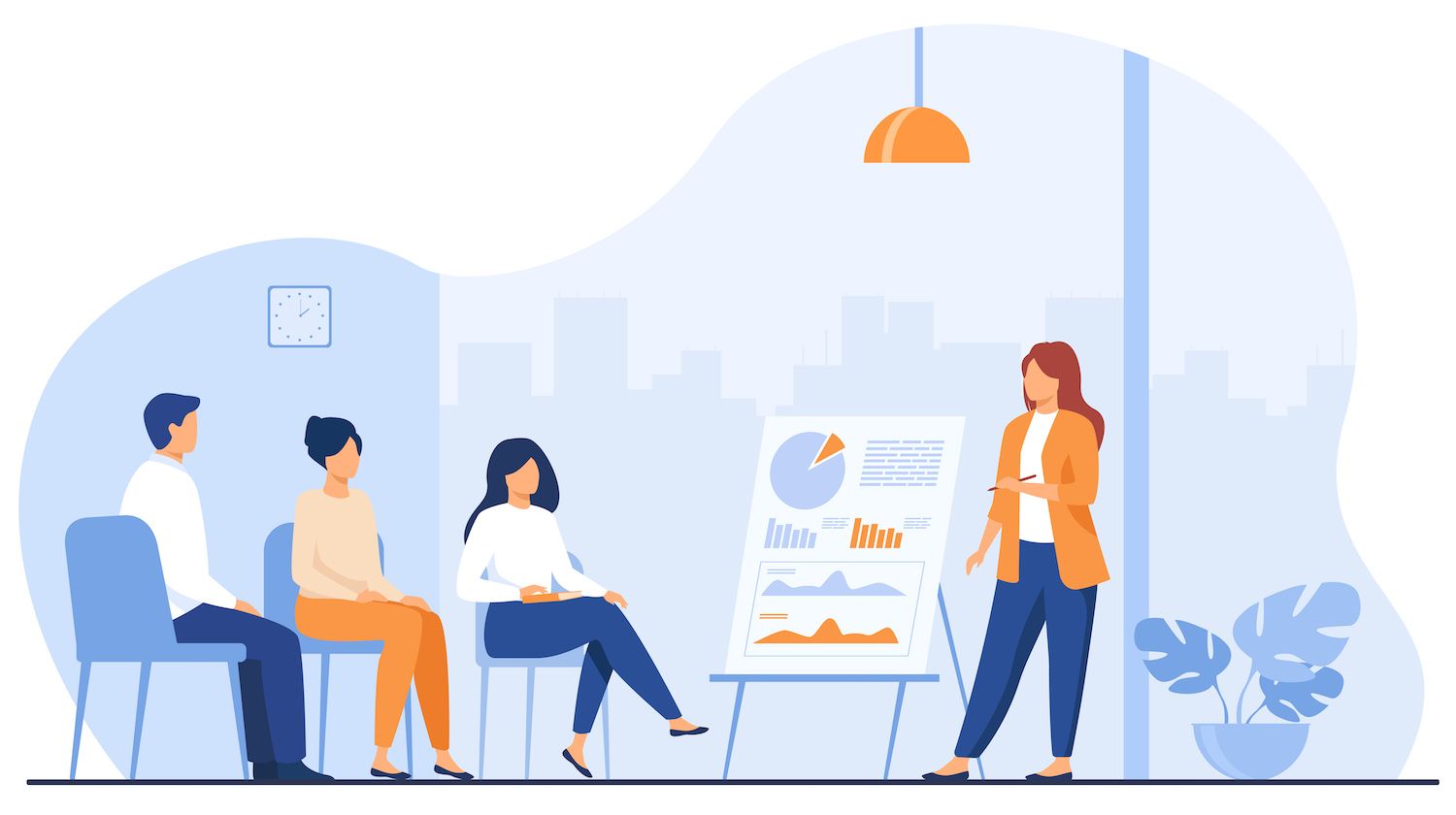
This approach allows for efficient and automated publishing to your WordPress site from your software.
Making a Change to an Existing Post within WordPress
For updating a post using the WordPress API, make a PUT request to the /posts
endpoint of the WordPress API. It is also necessary to send the latest content for your WordPress post by submitting it in JSON format.
An update to an already published post on WordPress is as follows:
app.put("/update-post", async (req, res) =>
try
const postID = req.body.id
const resp = await axios.put(`https://yourdomain.com/wp-json/wp/v2/posts/$postID`, req.body)
if(resp.status !== 200) throw "Something went wrong"
catch (err)
console.log(err)
)
For example, you can update a post with an ID of 3
for example, in WordPress by making the following request within the Thunder Client:
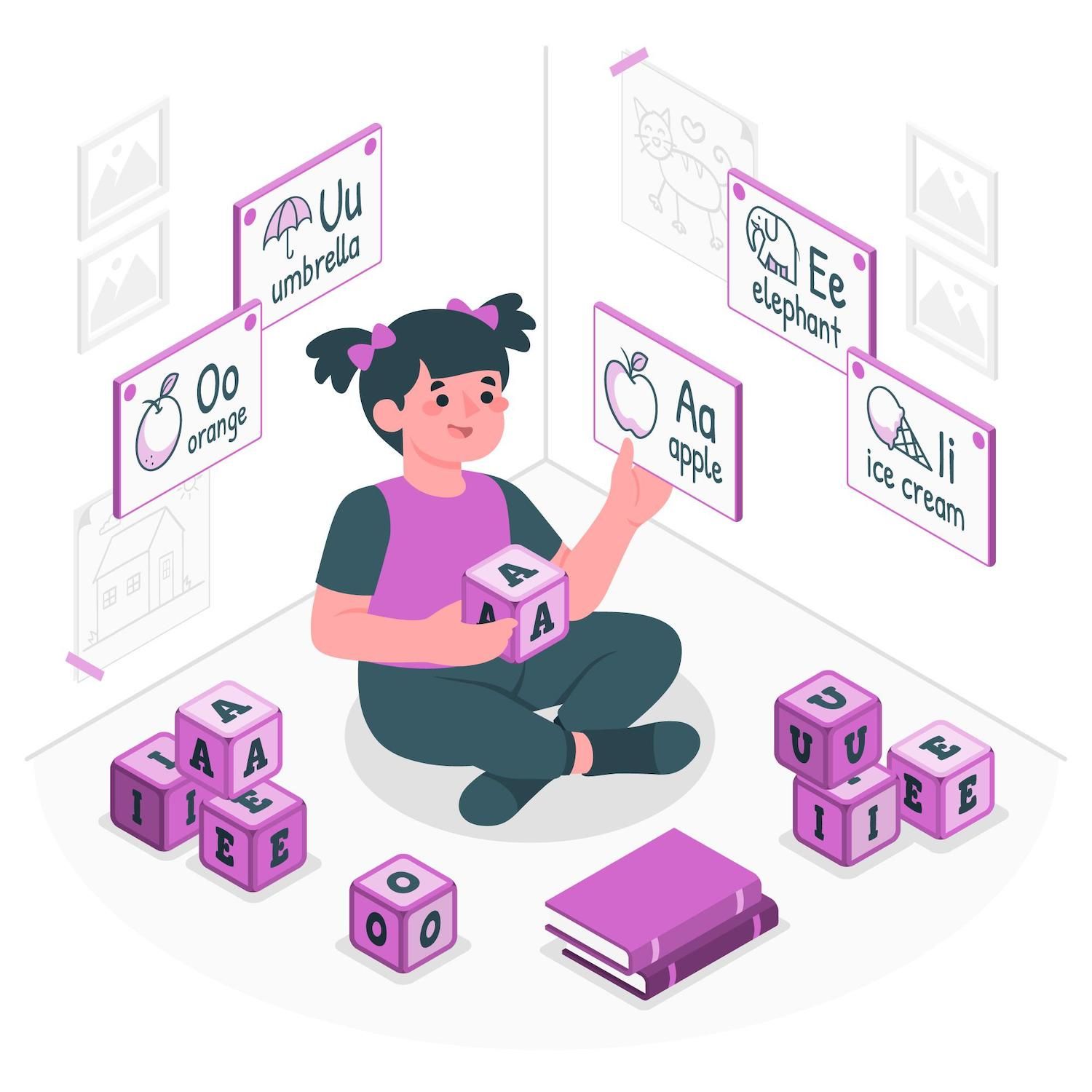
Removing an Post in WordPress
To delete a post in WordPress you must send a DELETE request to the /posts
endpoint using your unique ID for the post you want to erase.
app.delete("/delete-post", async (req, res) =>
try
const postID = req.body.id
const resp = await axios.delete(`https://yourdomain.com/wp-json/wp/v2/posts/$postID`)
if(resp.status !== 200) throw "Something went wrong"
catch (err)
console.log(err)
)
The result should look as follows:
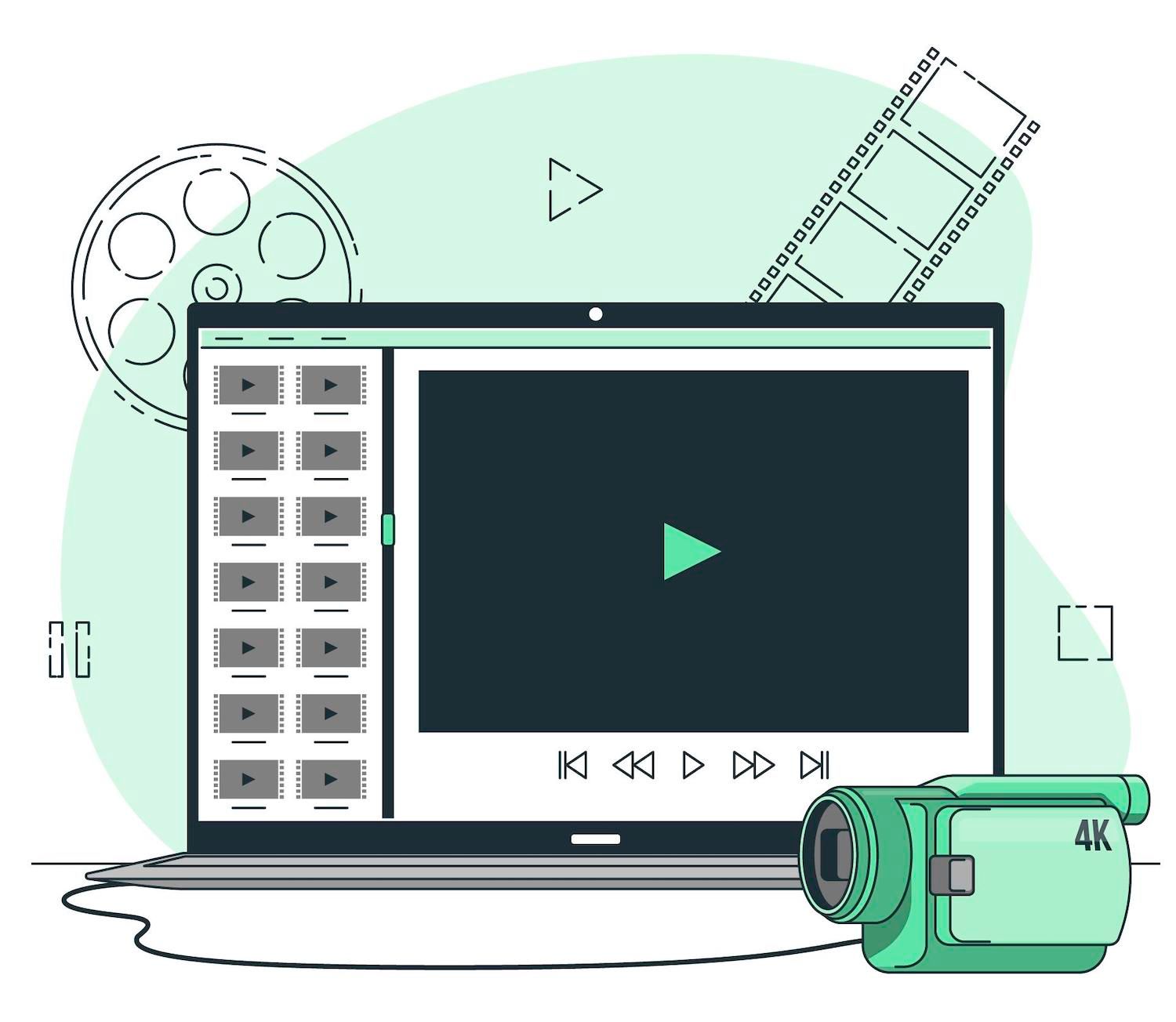
Moderating WordPress Comments Using Node.js
The Comment API lets you access and modify comments on your WordPress website through an application. Within that API serves as an interface for creating, listing, reading, updating, or deleting comments on the WordPress website.
It is assumed that you do not want your WordPress site to display comments that include the phrase "Follow me." In this instance, you can make use of an regex
expression to examine every comment for the phrase before posting it.
For this, you must use the following code:
app.post("/add-comment", async (req, res) =>
try
let regex = /follow me/i;
let comment = req.body.comment
if(regex.test(comment)) throw "Oops! Contains the forbidden word"
const resp = await axios.post(`https://yourdomain/wp-json/wp/v2/comments`, req.body)
if(resp.status !== 200) throw "Something went wrong"
catch (err)
console.log(err)
)
With this route it is the case that only those comments that don't include the phrase "Follow me" get published on the website while comments like the one below aren't published:
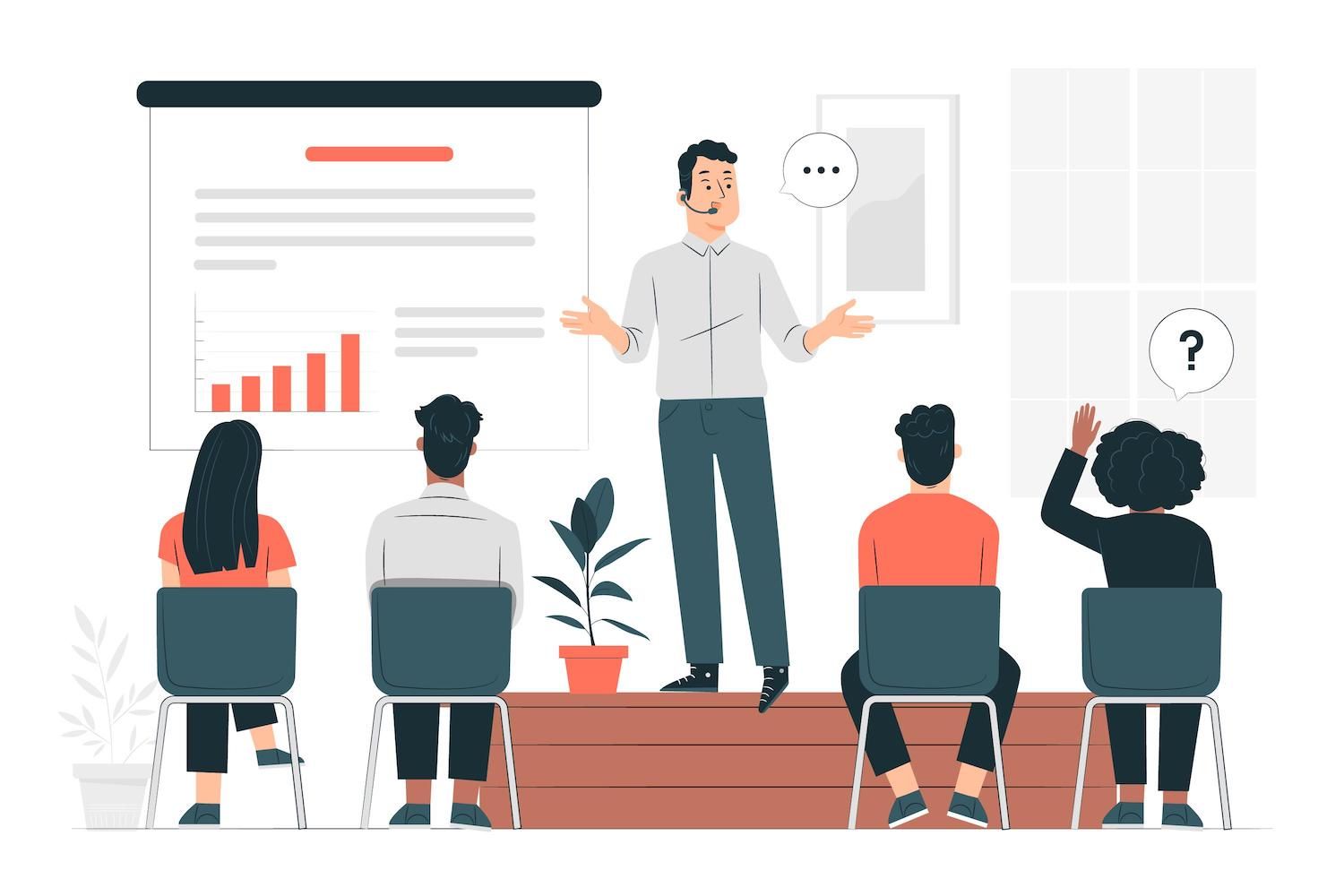
Customizing Sites for the needs of
Through storing user preferences, and also determining their location You can personalize the appearance of your WordPress pages for each user.
In Node.js it is possible to store information about users in cookies via the signup or login method of your backend application and place the cookie in the web browser of users, such so:
app.post("/sign-up", async (req, res) =>
// Sign up user
res.cookie("cookie_id", 123456)
res.cookie("lang", req.body.language)
res.status(200).json("Logged in.") )
Upon signing in, you are given the preferred language for your user and send it to the browser as cookies along with an identification number, the Cookie_ID
.
With the language in the browser, you'll be able to utilize it to retrieve WordPress posts in the language of the user. It is necessary to translate your blog posts into WordPress first. An easy way to do this is by connecting WPML and SEO to your WordPress site.
After integration, subfolders are created to accommodate different languages:
- mydomain.com/en/
- mydomain.com/es/
- mydomain.com/fr/
If you search for WordPress post, you'll see the post's list in the preferred language of the user as it is stored in cookies.
app.get("/get-posts", async (req, res) =>
try
const lang = req.cookies.lang
const resp = await axios.get(`https://mydomain.com/$lang/wp-json/wp/v1/posts`)
if(resp.status !== 200) throw "Something went wrong"
catch (err)
console.log(err)
)
In this way, you get the list of posts based on the language the person registered with during registration.
Utilizing Custom Administration
Through extending the endpoint of the user, you can build an administration panel that is custom designed that allows you to manage WordPress user roles, users, as well as permissions. The Users API permits you to manage and modify user information on your WordPress site through an application that functions like the comment API.
In the example above, for instance, if you're trying to alter the user's role from "Administrator" to "Administrator," here's the route you can use:
app.put("/update-user", async (req, res) =>
try
const userID = req.body.id
const resp = await axios.put(`https://yourdomain/wp-json/wp/v2/users/$userID`, req.body)
if(resp.status !== 200) throw "Something went wrong"
catch (err)
console.log(err)
)
When you request for information, include an object containing the ID of the user who's record you wish to update along with the new details.
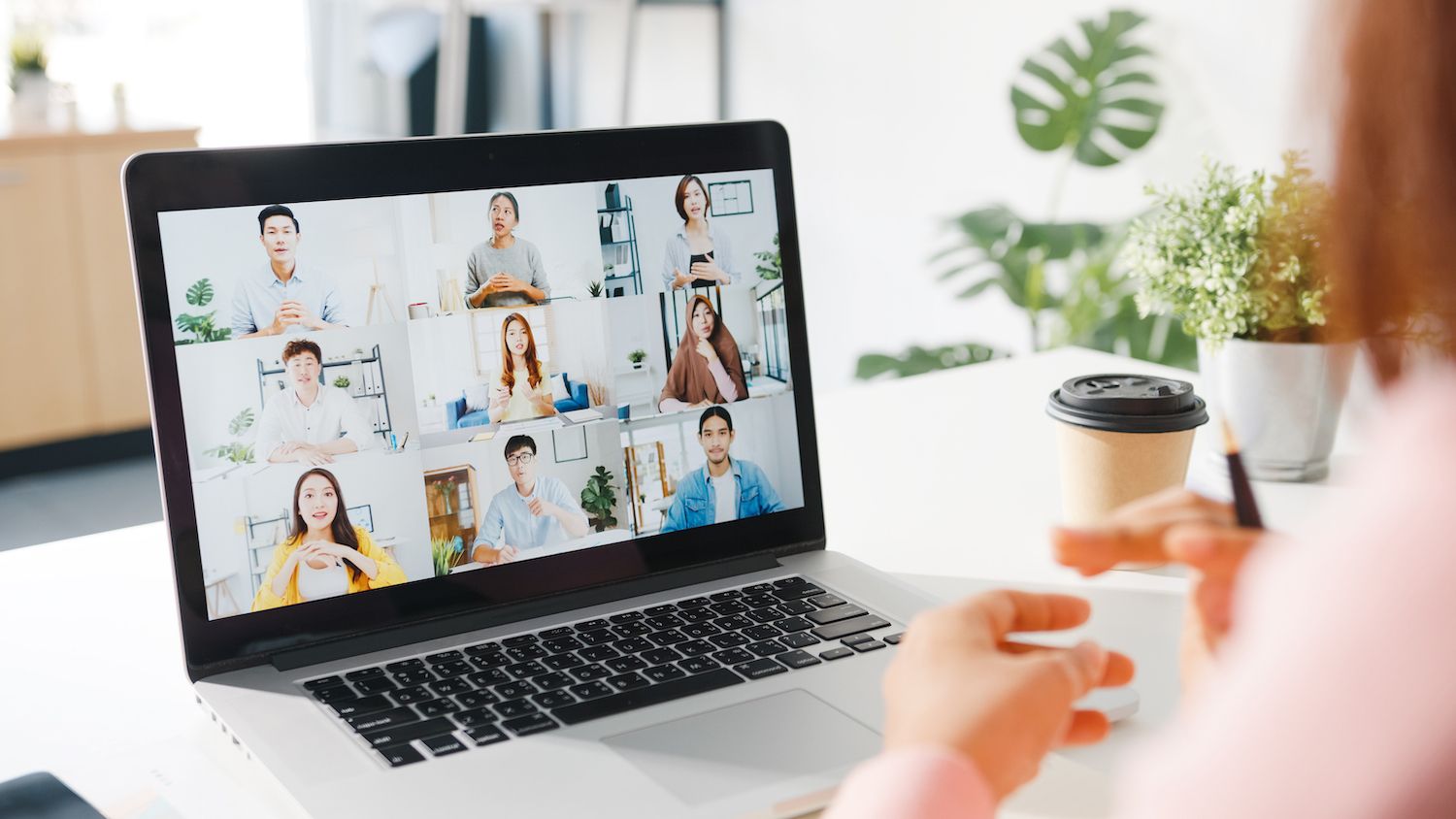
Summary
Integration of Node.js to the functionality of your WordPress site gives you the potential for greater functionality. You can make changes to posts, moderate comments and define user roles and add customization based on your user's country.
Apart from the features that we've already discussed the ability to add advanced searching, theme manipulation as well as post revisions. Do not hesitate to read the REST API Handbook and begin exploring.
What's your opinion on the WordPress REST API? Did you use it in some of your tasks? Let us know in the comment section below!
Jeremy Holcombe
Content and Marketing Editor at , WordPress Web Developer, and Content Writer. In addition to all things WordPress I like playing golf, at the beach, as well as movies. Also, I have height issues ;).