What can I do to make use of WordPress as a headless CMS to use Next.js - (r)
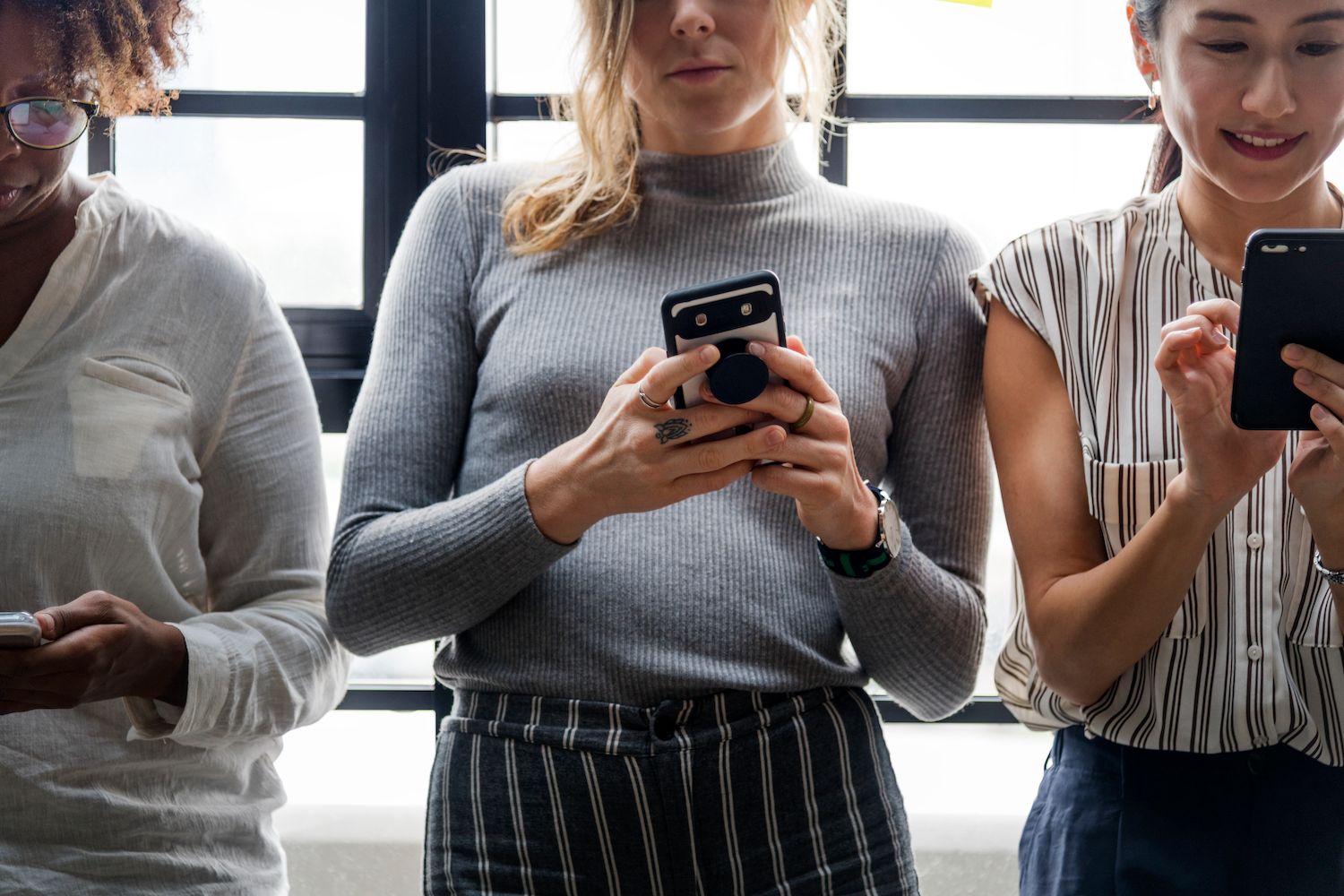
-sidebar-toc>
WordPress has existed for over 20 years, powering 42.7 percent of the websites that are on the Internet, according to W3Techs. It also holds a 62.5 percentage market share content management systems (CMSs) ahead of websites.
The understanding of headless WordPress
Headless WordPress means making use of WordPress solely to support its backend functions which includes managing and storing data -- employing a separate platform, like Next.js, for the frontend presentation.
This decoupling allows developers to utilize WordPress's powerful software for managing content while making the most of current front-end development tools, such as rendering on the server and static website generation using Next.js.
Preparing your WordPress site
If you don't already have a WordPress website, you can create one easily with . There are three ways for building your own WordPress site that you can use :
Let's use REST API to guide us through this. To retrieve your WordPress data in a JSON format, append /wp_json/wordpress/v2
to the WordPress website URL:
http://yoursite.com/wp-json/wp/v2
If the JSON API is not enabled when you visit http://yoursite.com/wp-json by default, you can enable it by opening Permalinks under Settings in the WordPress dashboard and selecting Post Name or any other one of your choice except Plain:
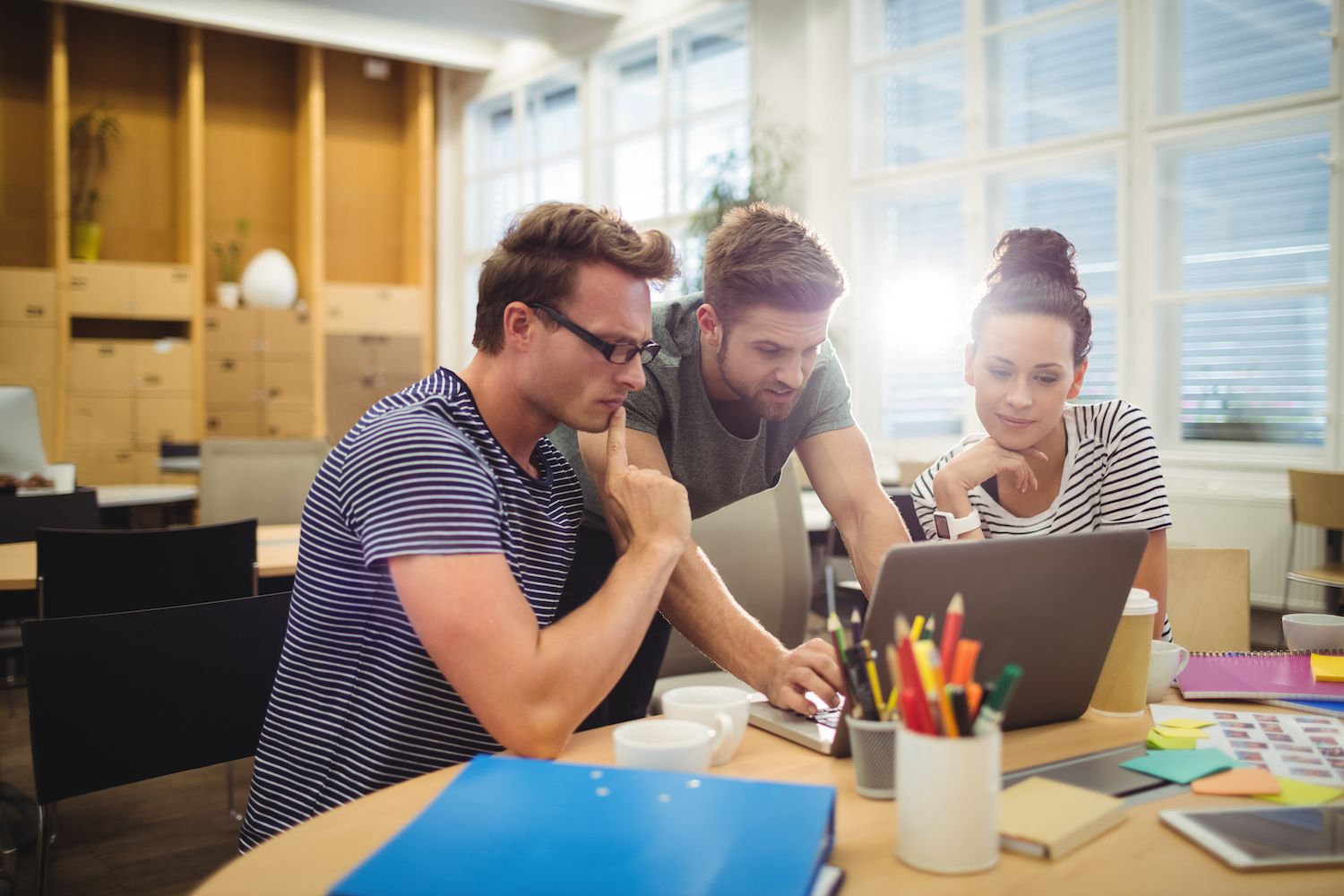
Configuring your Next.js environment
Next.js lets developers create websites with ease improving performance while also making development easier. One of its key features is the file-based routing feature, which helps in the creation of routes.
In order to set up a Next.js project, use the following command to utilize its default responses
npx create-next-app@latest nextjs-wp-demo
- Visit this site's GitHub repository.
- Select Use this model >> Create the repository to copy the code for starter into an account within the account of your GitHub Account (check the box that says to include the entire branch).
- Pull the repository to your personal computer, then switch onto the starter-files branch using the following command:
Git checkout starter files
. - Install the necessary dependencies using the command
"npm install"
.
After the installation has been completed, launch the project on your local computer with the command npm run dev
. This makes the project available at http://localhost:3000/.
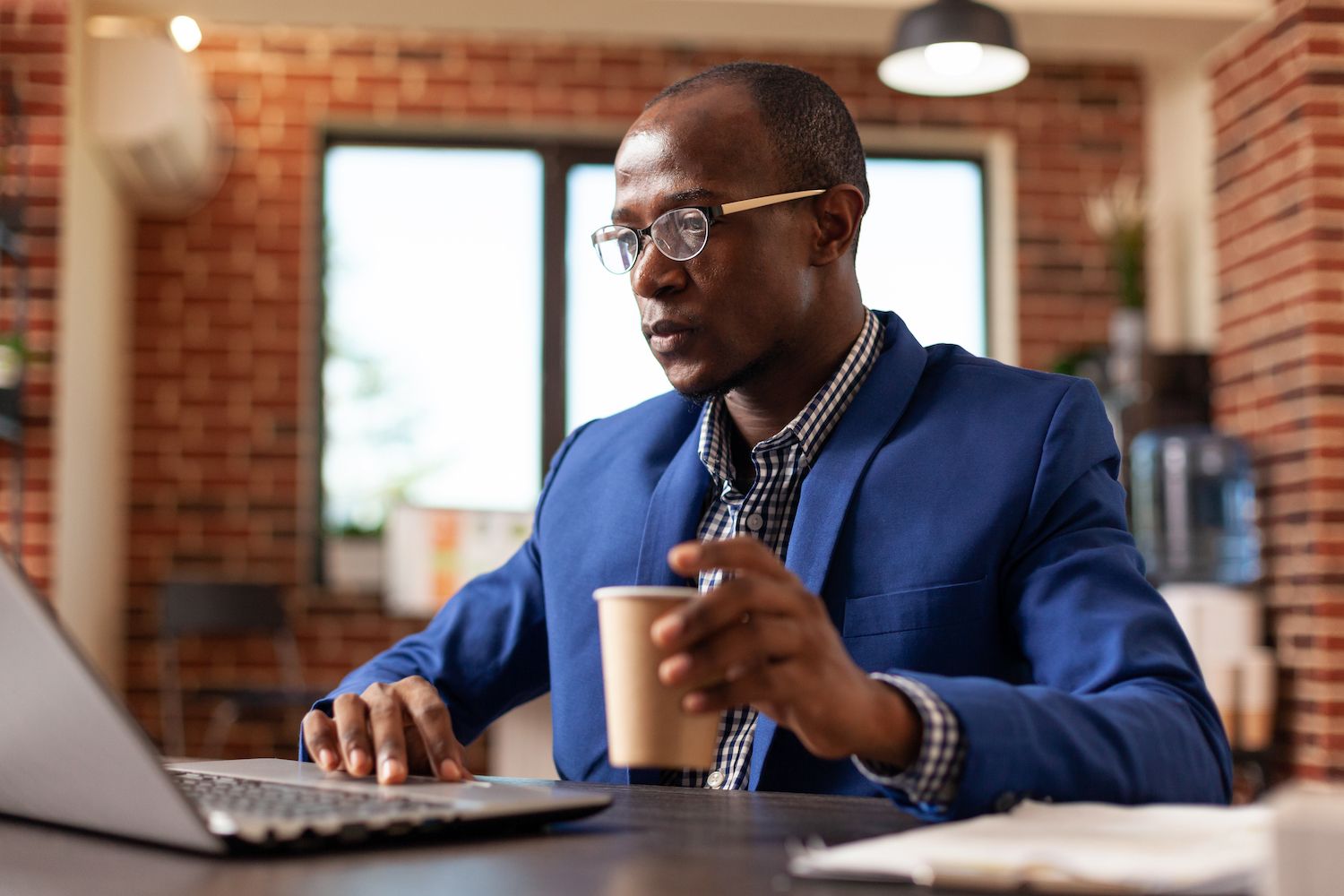
Knowing the scope of the project
App Router was introduced in Next.js 13. App Router was first introduced in Next.js 13 and replaced the existing pages directory used for routing. The routing process with the App Router is also about creating folders within your App directory. After that, you can nest a page.js file within the appropriate folder to define the route you want to take.
This project app is the core directory that you will be working with, and you will find the following file structure.
/
|-- /app
|-- /blog
|-- /[postId]
|-- page.js
|-- page.js
|-- globals.css
|-- layout.js
|-- navbar.js
|-- page.js
Three pages are designed The home page is designed that displays basic information and the blog page that will show all the posts in your WordPress CMS, and the dynamic page ( [postId]/page.js) for rendering individual posts.
There is also the navbar.js component, that is loaded in the layout.js file to build a layout to the project.
Transferring data directly from WordPress to Next.js
Through using the WordPress REST API, users are able to retrieve pages, posts and post types that you have created by making HTTP requests to specific endpoints.
Let's make a fetch request in the blog/page.js file to fetch all posts in your WordPress CMS and then finally make a request to fetch each post dynamically in the blog/[postId]/page.js based on the id
parameter passed.
When we are making request, it's recommended to add your JSON API address into the environment variables. This will ensure that the API's base URL is easily configurable and not hardcoded across many files.
Create a .env file in the root of your Next.js project. Include the following information:
NEXT_PUBLIC_WORDPRESS_API_URL=https://yoursite..cloud/wp-json/wp/v2
Make sure you change the URL to match the URL of your website's JSON API. Add .env
to your file, so that it won't push the file through your Git service provider.
All posts that are retrieved from WordPress to Next.js
To retrieve all the posts on your WordPress website, you need to create an asynchronous function named getPosts
in the blog/page.js file. The function utilizes the Fetch API to create an GET call to posts
page of the WordPress REST API.
async function getPosts()
const response = await fetch(
`$process.env.NEXT_PUBLIC_WORDPRESS_API_URL/posts`
);
const posts = await response.json();
return posts;
After receiving the response it converts the reply to JSON, forming an array of post objects. This array of posts
are rendered by your Next.js application. This will provide an ever-changing listing of blog entries retrieved directly from WordPress.
const BlogPage = async () =>
const posts = await getPosts();
return (
All Blog Posts
All blog posts are fetched from WordPress via the WP REST API.
posts.map((post) =>
return (
post.title.rendered
);
)
);
;
In the Next.js page component, use the function getPosts
in asynchronous fashion to get the latest posts. Next, create a map of all the posts and rendering the post's title as well as an excerpt inside a Link> component.
This not only displays the posts, but it also wraps them into a hyperlink that takes you to an in-depth view of the post. This is achieved by using Next.js's file-based routing, where the ID for each post will be used to determine the URL route dynamically.
Retrieve dynamic content from WordPress to Next.js
In the above code the posts are contained in a link which should help visitors get a more detailed overview of the content.
To display individual pages for posts, you utilize dynamic routing within Next.js to build an online page that pulls up and displays a single post using its ID. A dynamic webpage [postID]/page.js is already made in the stater-files script.
Create an GetSinglePost
function similar to getPosts
, to fetch one post by that uses the ID of the post passed as an input parameter.
async function getSinglePost(postId)
const response = await fetch(
`$process.env.NEXT_PUBLIC_WORDPRESS_API_URL/posts/$postId`
);
const post = await response.json();
return post;
With the dynamic component, you obtain the ID of the post from the URL parameters, then call getSinglePost
using this ID, and render the post's content.
const page = async ( params ) =>
const post = await getSinglePost(params.postId);
// ... the rest of the page code
;
It is then possible to add the fetched data:
const page = async ( params ) =>
const post = await getSinglePost(params.postId);
if (!post)
return Loading...;
return (
post.title.rendered
dangerouslySetInnerHTML= __html: post.content.rendered >
);
;
Access the entire program on the GitHub repository.
Deploying your Next.js application to for no cost
Static rendering in Next.js
To allow a static export in Next.js Version 13 or higher you must change your output
mode in next.config.js:
const nextConfig =
output: 'export',
;
From version 13, Next.js supports starting as a static site, and later, if desired, upgrading to utilize features that need servers. If you are using server-based features, building your pages doesn't produce static pages.
For example, in the dynamic route it is possible to fetch these data in a dynamic manner. You need to be able to generate all the posts statically. This can be done using the generatorStaticParams
function.
This function can be used along and dynamic segmentation of routes that create routes in a static manner at the build time instead of on-demand during request times. If you create, generateStaticParams
runs before the corresponding page layouts are generated.
Then, in [postID]/page.js, use the generateStaticParams
function to get all posts route:
export async function generateStaticParams()
const response = await fetch(
`$process.env.NEXT_PUBLIC_WORDPRESS_API_URL/posts`
);
const posts = await response.json();
return posts.map((post) => (
postId: post.id.toString(),
));
If you execute the build command the Next.js project will now generate the outside directory that contains the static files.
Setup Next.js to static site hosting
- Create or log in to an account to view your My dashboard.
- Authorize Git with the service you use.
- Go to the Static Websites in the sidebar left Click Static Sites, and click Add site.
- Select the repository as well as the branch that you want to use for deployment.
- Assign a unique name to your site.
- Set the build parameters using the format below:
- Build command:
NPM run build
- Node version:
18.16.0
- Publish directory:
out
- Finally Click Create site.
Summary
In this tutorial we will show you how you can use headless WordPress in a Next.js project to pull and display content dynamically. This technique allows for easy integration of WordPress posts into Next.js applications. This provides an updated and interactive user experience on the web.
The possibilities that Headless CMS API is not limited to post creation; it also allows the retrieval and management of pages, comments media, and more.
Joel Olawanle
Joel is Joel is a Frontend Developer working Technical Editor. He is a passionate educator who is a lover of open source and has written more than 200 technical articles majorly around JavaScript as well as its frameworks.