What's new with PHP 7.2 (Now in beta)
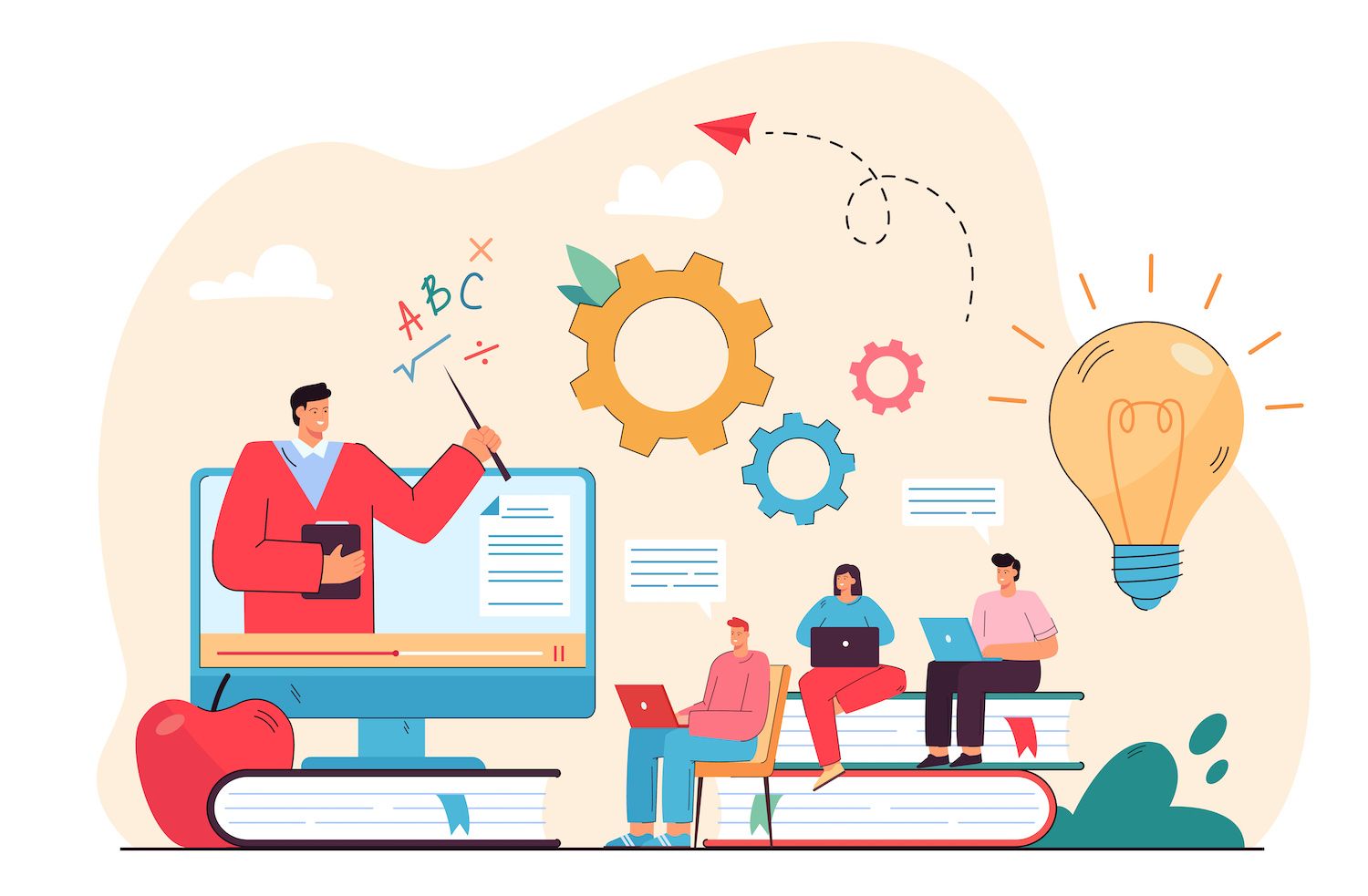
-language-notice>
PHP 7.2 was officially released as of November 30. The release has many new features, functions and improvements that will let us write more effective code. In this article we'll discuss some of the best functions and features of PHP 7.2. PHP 7.2.
It is possible to view the complete list of changes on the Requests For Comments page.
Improved Core
Argument type declarations
Since PHP 5 we're able to indicate in the declaration for a function, the type of argument which is to be used. If the argument type is of an incorrect type, then PHP will throw an error.
Argument type declarations (also known as type hints) indicate the nature of the variable likely to be passed on to a function or class method.
Here's an example:
class MyClass
public $var = 'Hello World';
$myclass = new MyClass;
function test(MyClass $myclass)
return $myclass->var;
echo test($myclass);
In this example, test function testing function is expecting to see an instanceof MyClass. A wrong data type could lead to the following fatal error:
fatal error Uncaught TypeError: Argument1 passed for testing() should be an instance from MyClass String provided, referred to in /app/index.php at line 12 and defined in /app/index.php:8
As of PHP 7.2 type hints can be utilized along with an object data type. Furthermore, this improvement allows to declare a generic object as part of the argument for a function or method. This is an example of this:
class MyClass
public $var = '';
class FirstChild extends MyClass
public $var = 'My name is Jim';
class SecondChild extends MyClass firstchild is a the new FirstChild;
$secondchild = the new SecondChild;
function test(object $arg)
return $arg->var;
echo test($firstchild) echo test($firstchild);echo test($secondchild);
In this case, we've invoked the test function twice and passed a different object on each occasion. This wasn't possible in previous PHP versions.
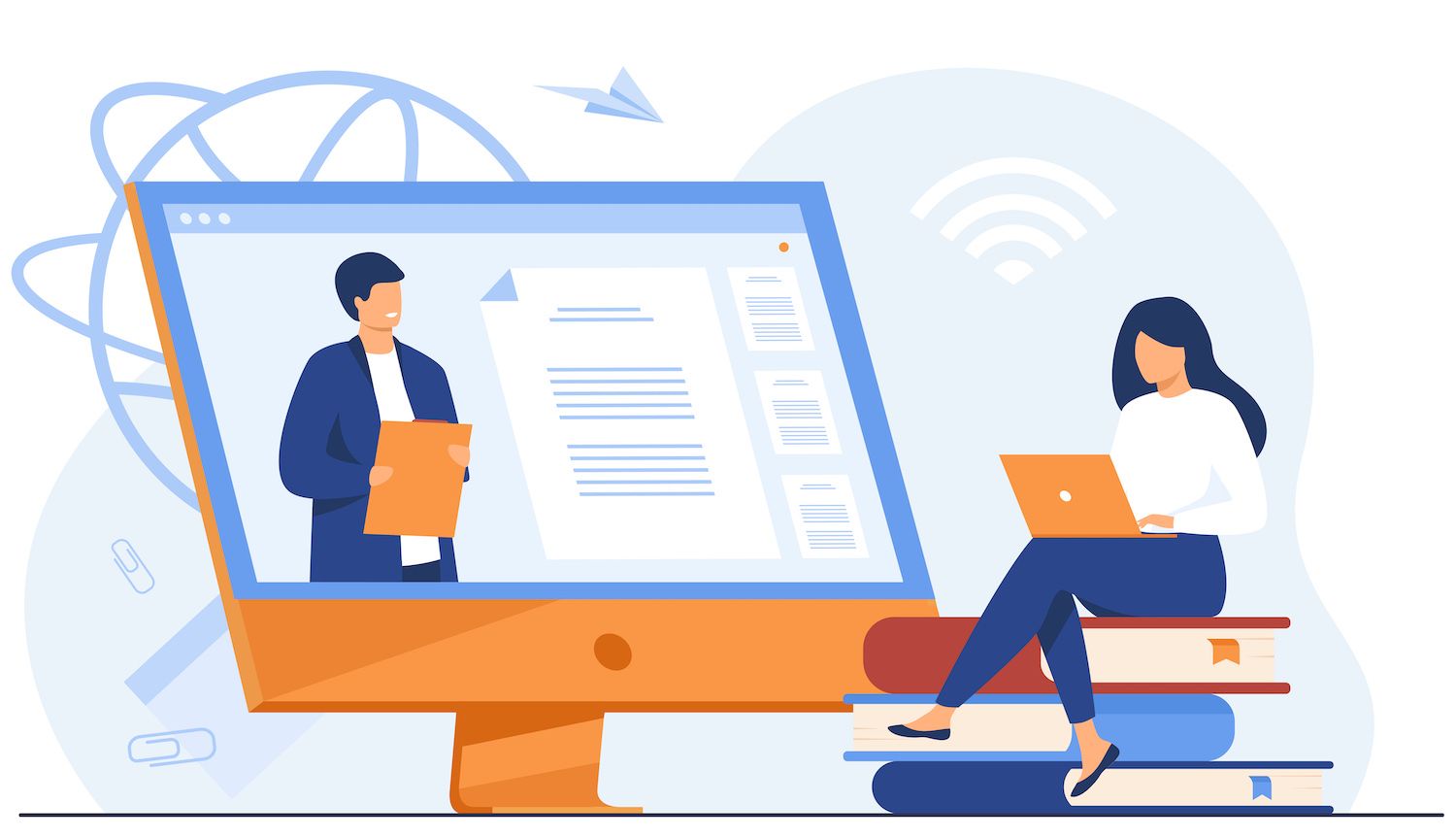
Object return type declarations
If declarations of argument type specify the expected format for function's arguments, the return declarations define what type is expected of the returning value.
Declarations of return type indicate the nature of a variable that is anticipated to be returned by a program.
Since PHP 7.2 We are now able to utilize return type declarations to describe the objects types of data. Here is an example:
class MyClass
public $var = 'Hello World';
$myclass = new MyClass;
function test(MyClass $arg) : object
return $arg;
echo test($myclass)->var;
Previous PHP versions throw the following fatal error:
Fatal error: Not caught TypeError: The return value of test() must be an instance of object, the instance MyClass returned in /app/index.php:10
In PHP 7.2 the code echos 'Hello World'.
Parameter Type Widening
PHP currently doesn't allow any variance in parameter types between child classes and their parent classes or interfaces. What does that mean?
Consider the following code:
In this case, we've removed the parameter type from the subclass. In PHP 7.0 this code produces the following warning:
Warning: Declaration of MyChildClass::myFunction($myarray) should be compatible with MyClass::myFunction(array $myarray) in %s on line 8
From PHP 7.2, we are allowed to omit an element of a class without breaking any code. This proposal will allow us to upgrade classes to make use of type hints within libraries without the need to update the subclasses.
The trailing commas of list syntax
A trailing comma after the final item of arrays is a valid syntax in PHP and at times, it's recommended for ease of use to join new elements and prevent error messages caused by a lack of a comma. Since PHP 7.2 we are allowed to use trailing commas within namespaces that are grouping.
Read the trailing commas in List Syntax for a closer view of this RFC and some samples of codes.
Security Improvements
Argon2 in password hash
Argon2 is a powerful hashing algorithm which was chosen as the winner of 2015 Password Hashing Competition, and PHP 7.2 is expected to bring it to us as an secure alternative to Bcrypt. Bcrypt algorithm.
The latest PHP version includes the password_argon2i constant which can be utilized for the password_* functions:
password_hash('password', PASSWORD_ARGON2I);
Contrary to Bcrypt, which takes only one cost element, Argon2 takes three cost elements that are categorized by the following:
- A memory price that defines the amount of KiB which should be used when the process of hashing (default values are 1
- The price for time that defines the number of times the algorithm is used. algorithm (defaults to 2.)
- A parameter for parallelism is a parameter that determines the amount of parallel threads which will be used for hashing (defaults to 2)
Three new constants define default cost factors:
- PASSWORD_ARGON2_DEFAULT_MEMORY_COST
- PASSWORD_ARGON2_DEFAULT_TIME_COST
- PASSWORD_ARGON2_DEFAULT_THREADS
Here is an example:
$options = ['memory_cost' => 1 4, 'threads' => 2];
password_hash('password', PASSWORD_ARGON2I, $options);
Check out Argon2 Password hash for more information.
Libsodium is a part of PHP Core
Beginning with the version 7.2, PHP includes the Sodium library in the PHP base. Libsodium is a cross-platform, cross-language library that can be used for encryption, decryption as well as encryption of passwords, signatures and much more.
The library was previously offered through PECL..
For a documented list of Libsodium functions, see the library quick Start guide.
Check out See PHP 7.2 This is the first programming language that has added Modern Cryptography to its Standard Library.
Deprecations
Below is a list of PHP 7.2 deprecated functions and features that will be removed as soon as PHP 8.0:
The __autoload function has been superseded by spl_autoload_register in PHP 5.1. A deprecation warning will occur if the function is encountered during compilation.
This $php_errormsg variable is created in the local scope when an error that is not fatal occurs. In PHP 7.2 error_get_last as well as error_clear_last should be used instead.
create_function() allows the creating of a function using named function generated along with a list of argument and body code that is provided as arguments. Because of security concerns as well as poor performance, the function is now deprecated and the use of enclosures is recommended rather.
mbstring.func_overload ini setting set to a non-zero value has been marked as deprecated.
(unset) casting is an equation which always returns null and is considered useless.
parse_str() parses a query string into an array if the second argument is provided in the local symbol table when it is not used. Because setting variables within the functions' scope is not recommended for security reasons, using parse_str() without the second argument is likely to result in an advisory about deprecation.
GMP_random() is considered to be dependent on the platform and therefore will be discarded. You can use the gmp_random_bits() and GMP_random_rage() instead.
Each() is used to repeat an array much like foreach(), but foreach() is preferable for several reasons, including being 10 times faster. Now a deprecation will be given on the first attempt within an array.
Its assert() function checks the asserted assertion, and then takes proper actions if the outcome is FALSE. Utilizing assertion() with a string argument is no longer recommended because it creates the possibility of an RCE vulnerability. It is possible to use the zend.assertion ini option can be used to prevent the evaluation of assertion expressions.
$errcontext is an array containing local variables that are present when an error is generated. It's passed as the last argument to error handlers set by set_error_handler(). setting_error_handler() function.
What Does PHP 7.2 Define for WordPress User?
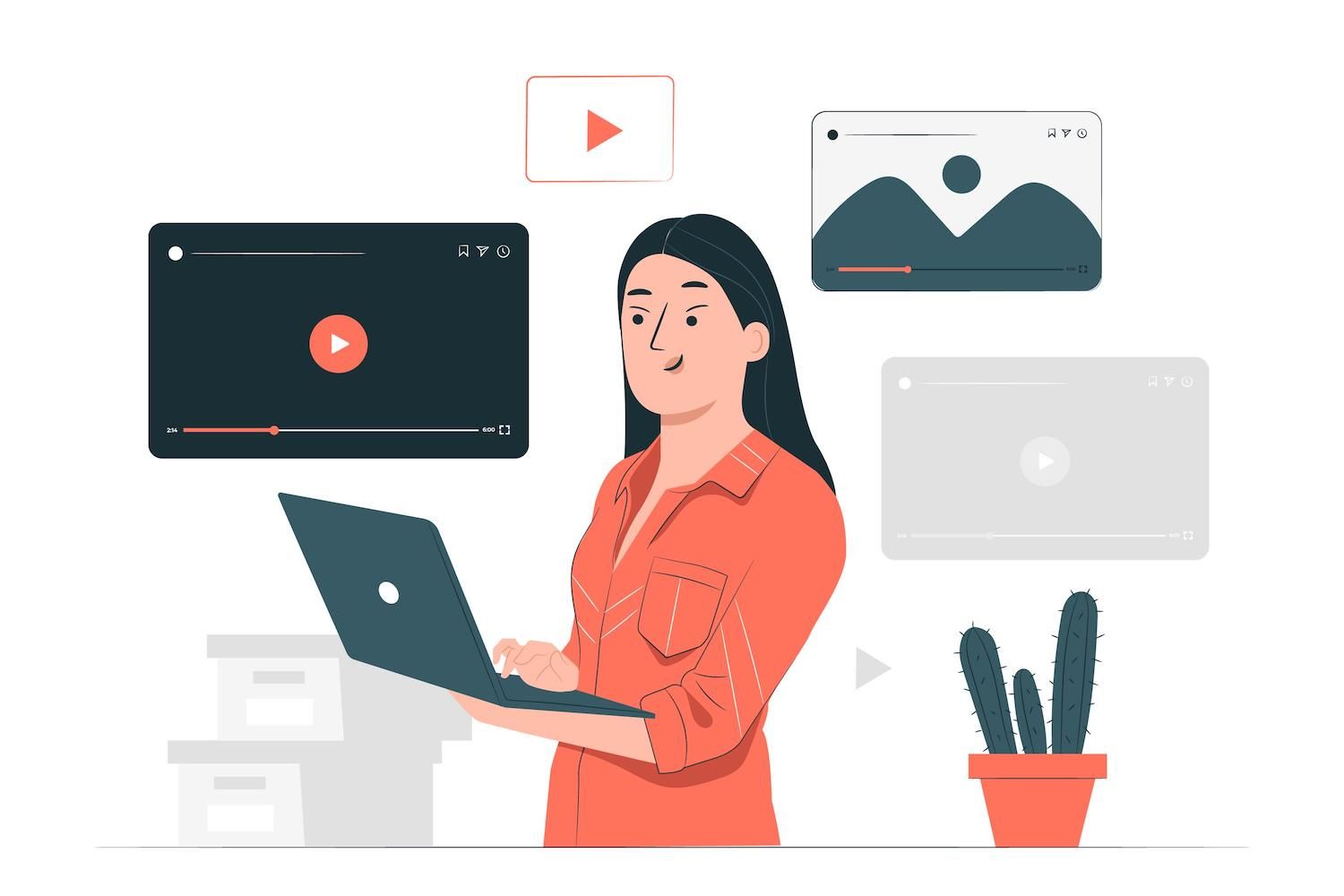
These numbers are particularly negative from a performance point the point of PHP 7 has shown to be significantly faster. These are a few statistics:
- The official PHP test results demonstrate that PHP 7 allows the system to run more than twice the number of requests in a second compared to PHP 5.6 that runs at nearly half of the latency.
- Christian Vigh also published a PHP performance comparison that he concluded that PHP 5.2 is 400% slower than PHP 7.
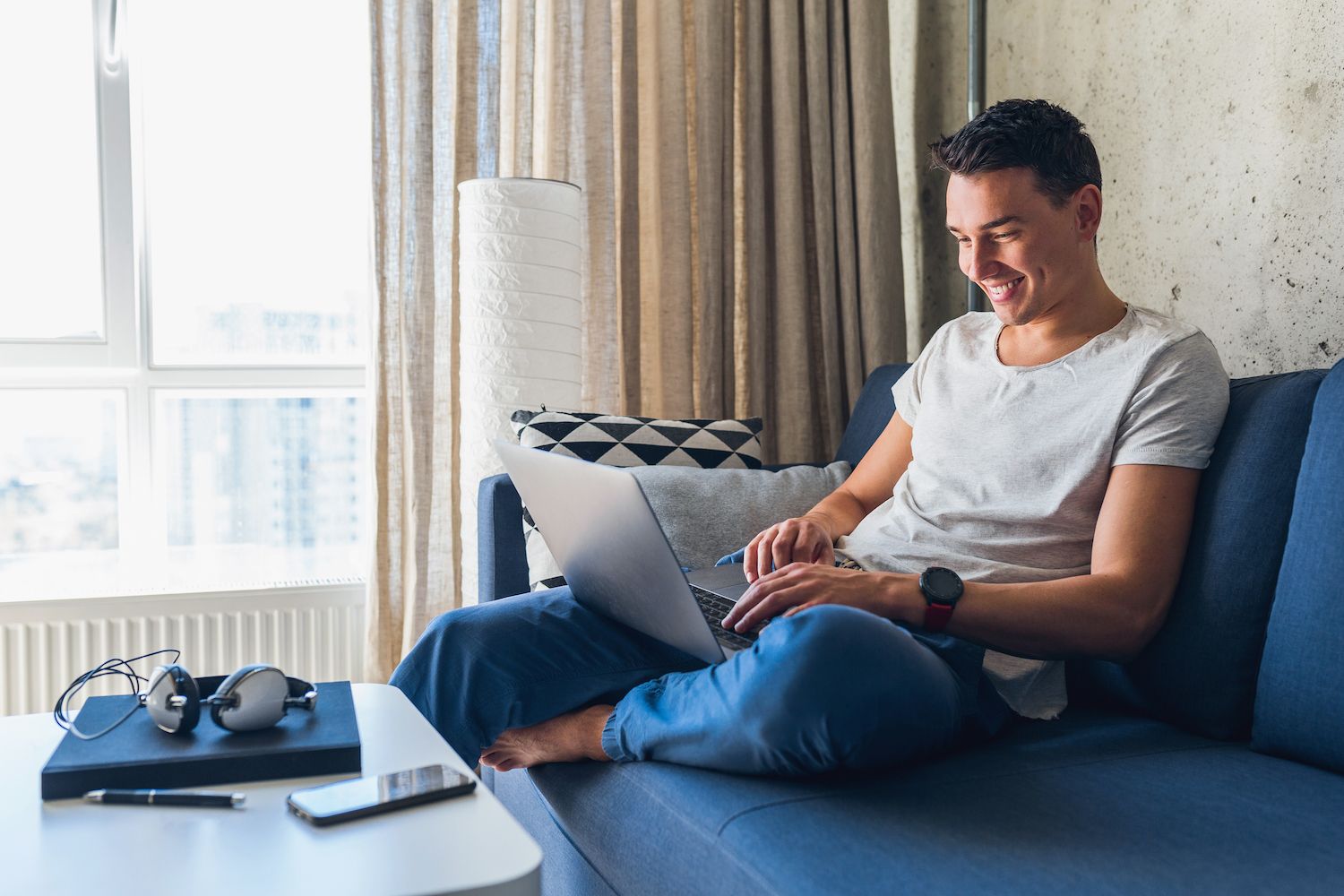
- WordPress 4.9.4 PHP 5.6 benchmark outcomes: 49.18 req/sec
- WordPress 4.9.4 PHP 7.0 benchmark result: 133.55 req/sec
- WordPress 4.9.4 PHP 7.1 benchmark outcomes: 134.24 req/sec
- WordPress 4.9.4 PHP 7.2 benchmark outcomes: 148.80 req/sec ?
- WordPress 4.9.4 Results of HHVM benchmark: 144.76 req/sec
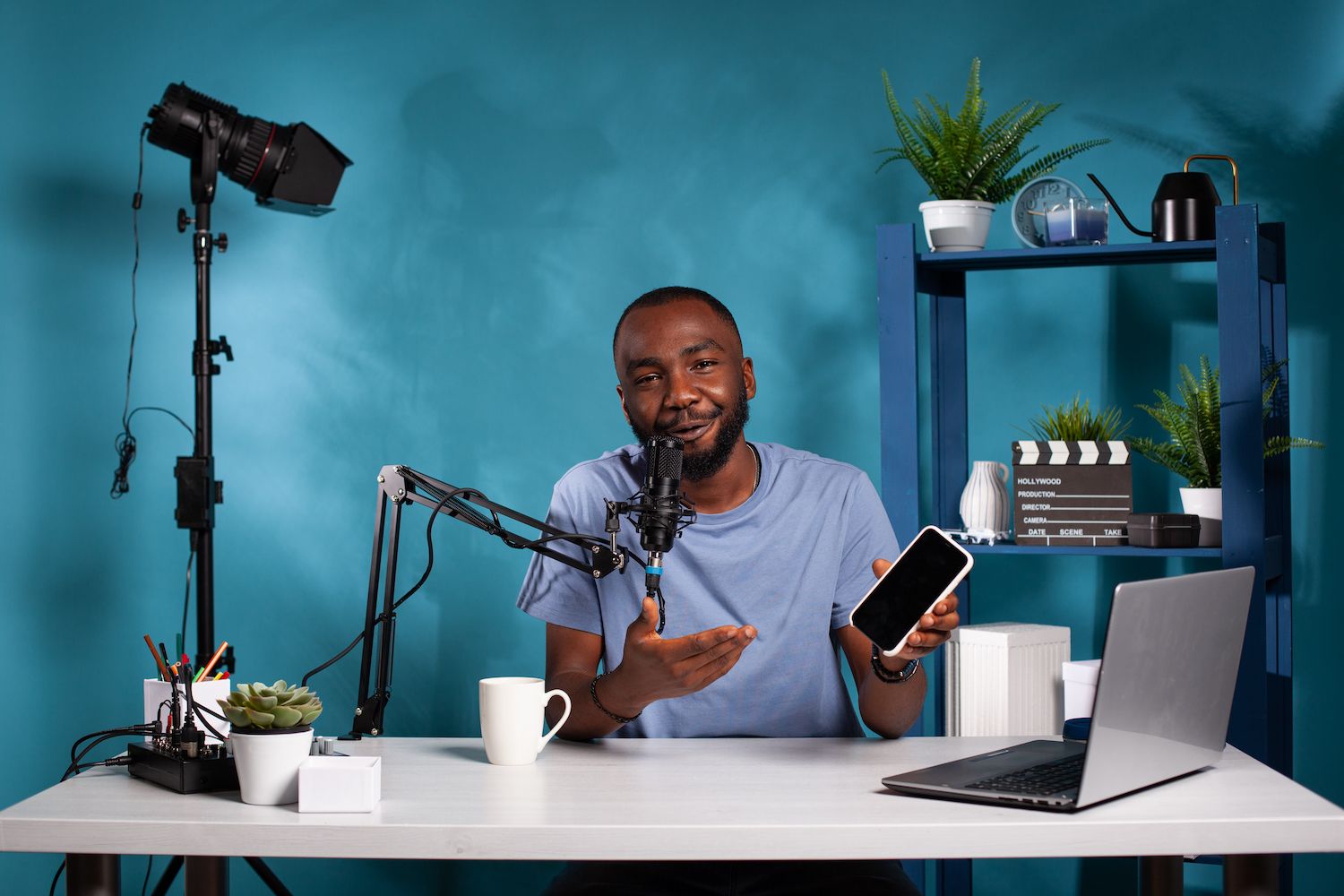
Update to PHP 7.2
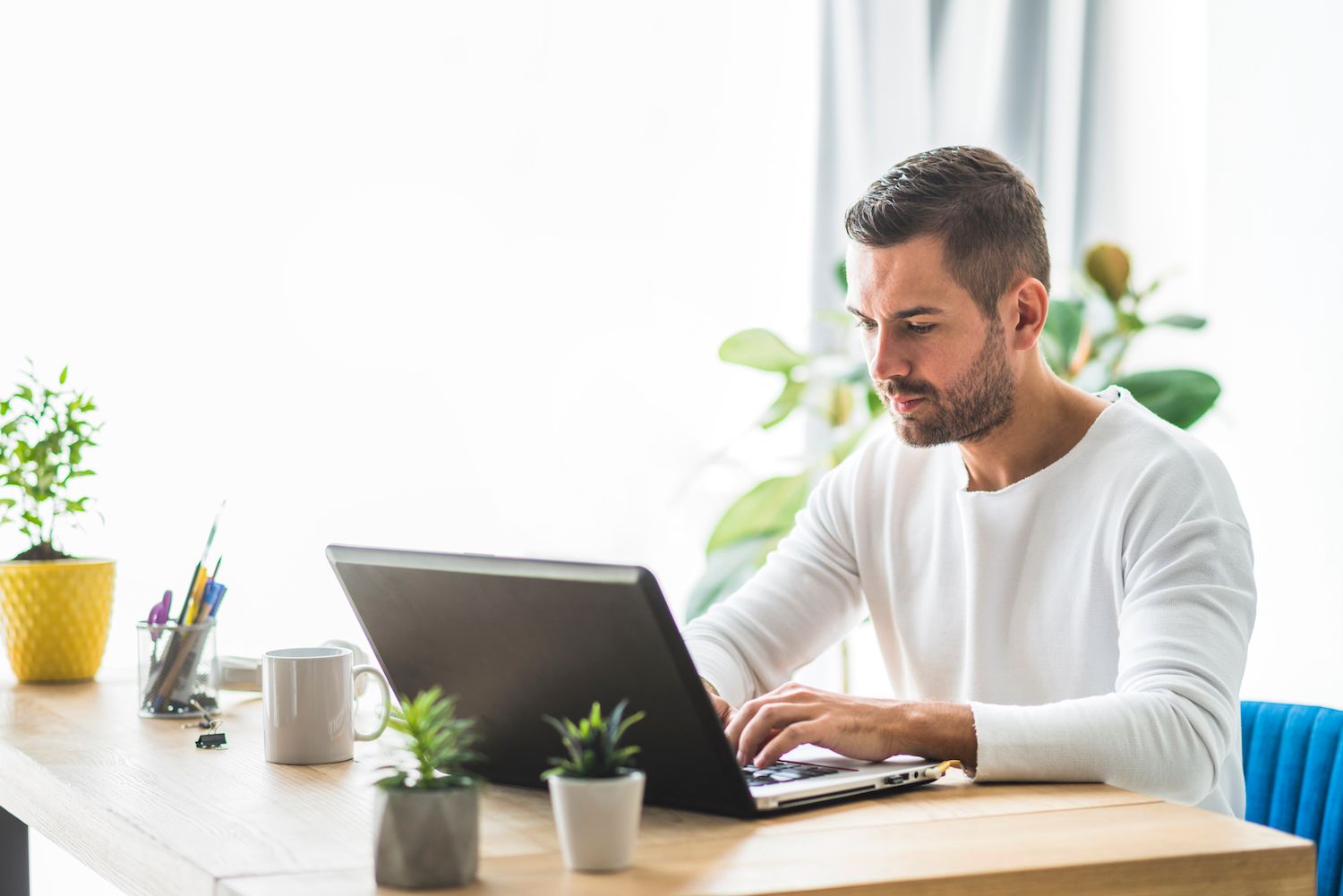
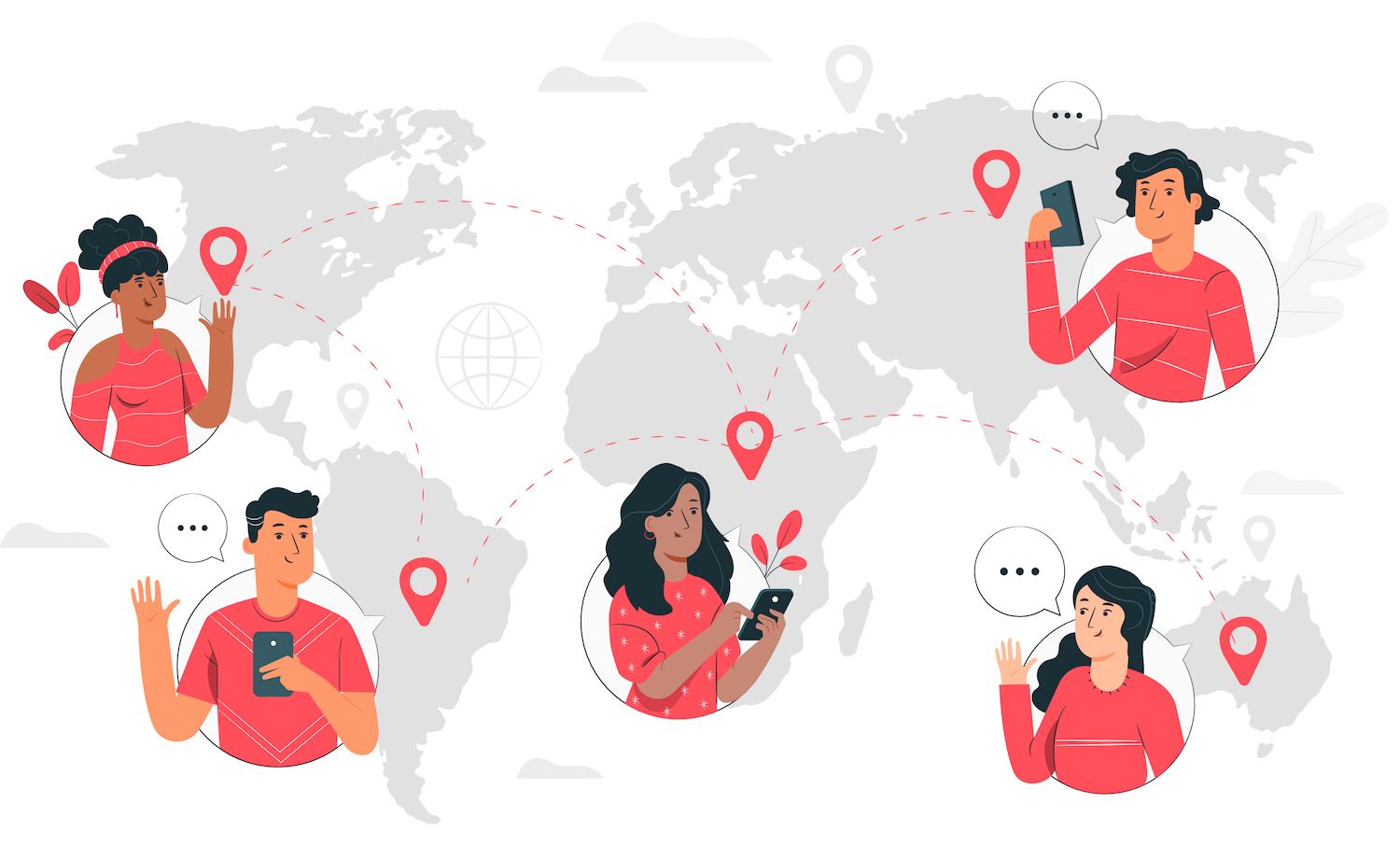
Conclusions
Are you ready to move into PHP 7.2? Perhaps, by now, you've at least completed the change to PHP 7. If not, now is a great moment to begin testing. Therefore, update your scripts, review your code and share your initial impressions with PHP 7.2.
Carlo Daniele
Carlo is a fervent lover of webdesign and front-end development. He has been playing with WordPress since more than twenty years. He also works in collaboration with Italian as well as European colleges and universities. He has written numerous articles and guides about WordPress that have been published on Italian and international websites, as well as on printed magazines. He is through LinkedIn.