WordPress Data package used to control application states
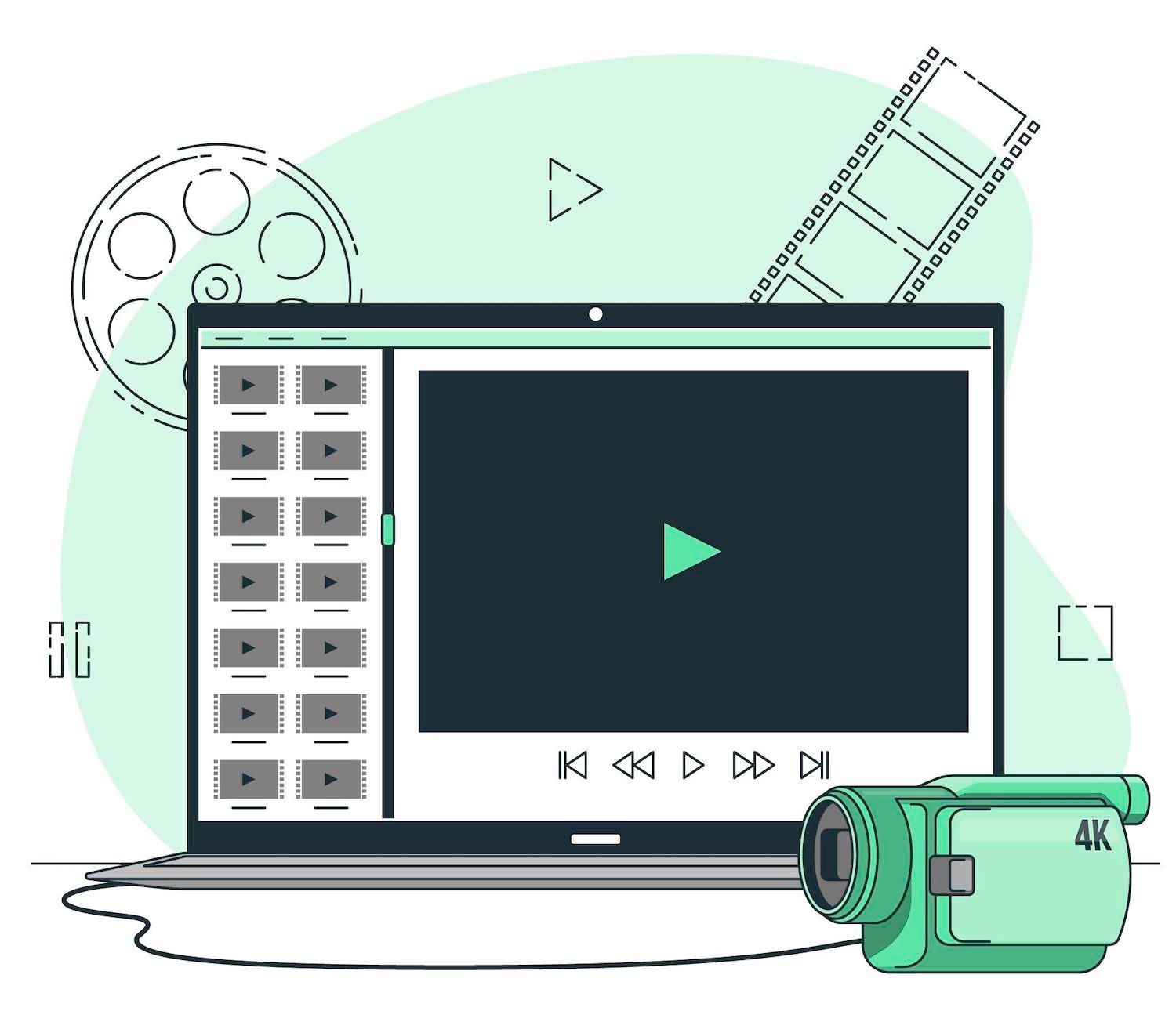
-sidebar-toc> -language-notice>
The management of the state of any WordPress app -- how it handles and manages data can be a challenge. With the development of your app, keeping track of the flow of data and making sure that updates are consistent across all components gets more challenging. The WordPress data package could be of help with this, as it has a an effective solution to managing state.
This article examines the WordPress data bundle, examining its key concepts, implementation methods, and the best methods.
Introducing the WordPress information package
WordPress data package -- officially @wordpress/data WordPress Data Package -- officially @wordpress/data
-- is an JavaScript (ES2015 and above) state management tool that provides a predictable and central way of managing applications' state. The right implementation can help in the creation of complicated user interfaces as well as manage information flow throughout your application.
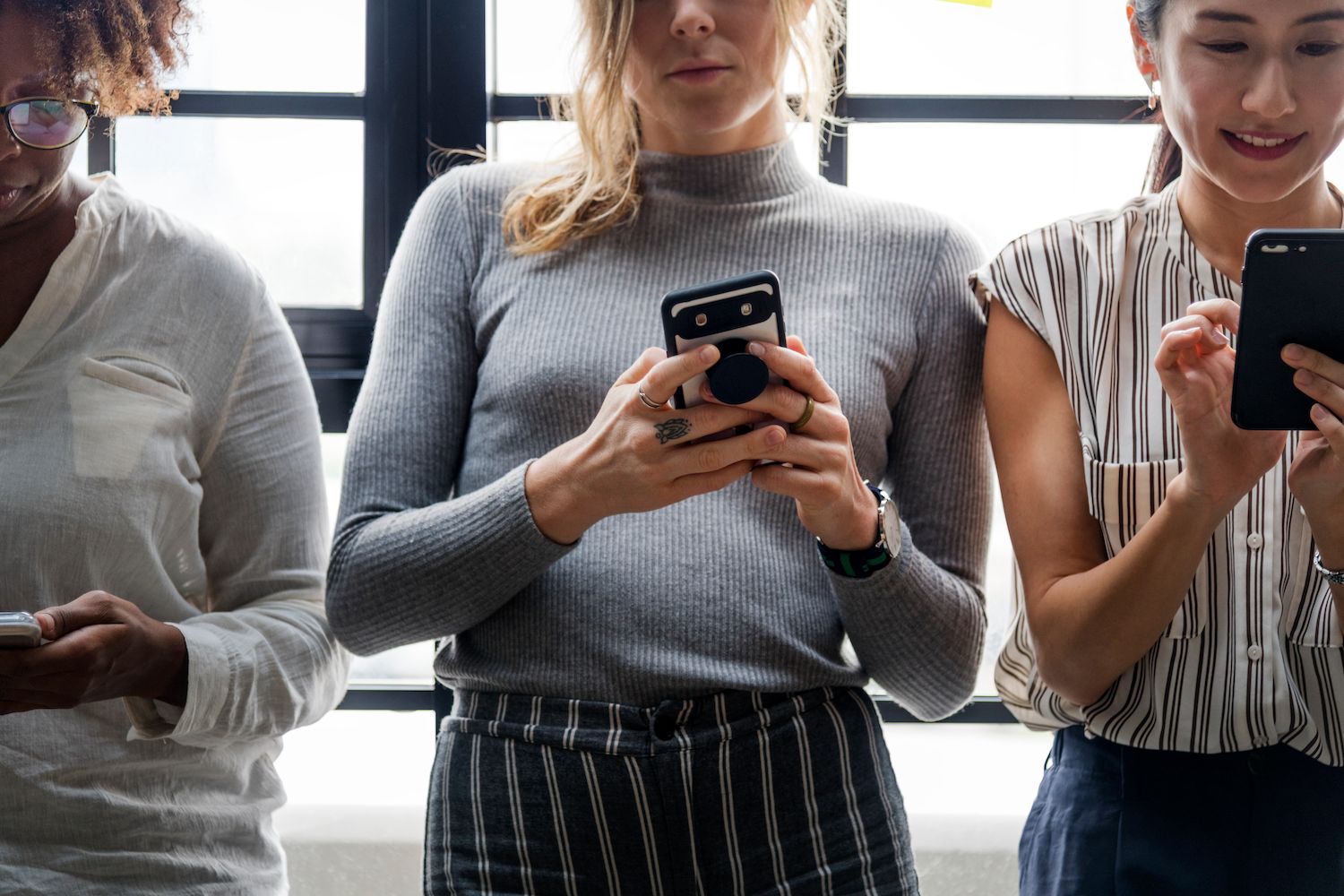
The connection between this package and Redux
While the WordPress data program draws an inspiration from Redux but it's not an exact port. There are many modifications to fit the WordPress ecosystem, with some significant differences between both solutions:
- The package for data is created to integrate seamlessly with WordPress APIs and functionality and functionality, something that standard Redux can't do without that adaption.
- Compared with Redux and Redux, the Data package has a much more efficient API. It makes it simpler to get started.
- The WordPress data package provides an centralized storage for data as well as UI state. This is a method to manage the flow of data and update within your app.
In most cases it is possible to use both using the REST API for fetching and updating the data stored on the server as well as the WordPress data pack to control those data inside your app.
Key concepts and terminology for the WordPress Data package
The WordPress data module provides an easy method of managing states. It refers to the information in stores. It represents the current condition of the application. It can include both UI state (such such as whether you have an open modal) as well as data state (such as a listing of blog posts).
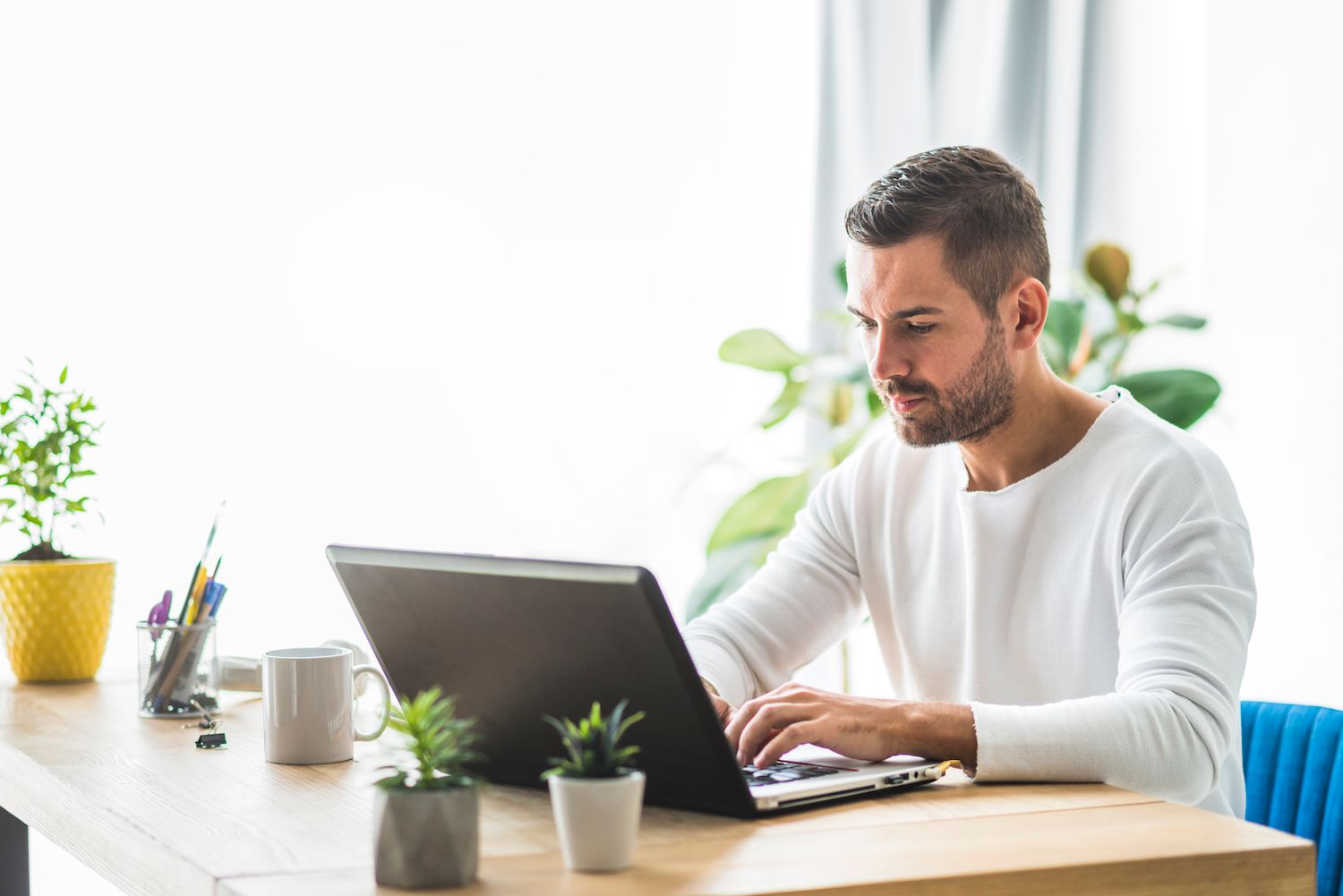
A store is the central hub of the WordPress data package. It is where you can store the whole site's state, and also provides methods to access and update the state. Within WordPress it is possible to have many stores. Each store will be responsible to a certain area of the site.
To manage those stores, you require to have a registry. This central object provides methods to create new stores as well as access your existing ones. The registry can hold the stores that store your app's data.
There are many strategies to cooperate with state:
- Actions are the actions that describe changes made to a state. They're plain JavaScript objects, and they can be the only method to trigger state changes. Most actions include a
kind
property, which describes the specific action. It may also include any additional information. - Reducers define how the state should be changed in response to certain actions. They use the state of the present and the action as inputs and then return a state object. Control functions allow reducers to manage complex async tasks with no side consequences.
It is essential to grasp these basic concepts because they work in tandem to build a strong state management system, with stores at its heart.
Stores: the central center of the WordPress information package
Each store will have a distinctive namespace. Examples include Core
, core/editor
, and core/notices
. Third-party plugins will be able to register stores too, so you need to select unique namespaces in order to avoid conflict. Regardless, stores you register are registered in the default registry in most cases.
The central object has few responsibilities:
- Registering new stores.
- Giving access to stores already open.
- Managing subscriptions to state changes.
Although you rarely engage directly with the registry, it is important to must understand its importance in the way that the data package orchestrates state management across WordPress.
Basic interaction using WordPress data stores
If you use ES2015+ JavaScript and you work with a WordPress plugin or theme, you could include it in an dependency
NPM install @wordpress/data and save
In your code, you'll incorporate the functions you require from the package at the top of the file:
import select, send, or subscribe from '@wordpress/data';
Connecting to existing WordPress stores will require you to use some of the functions that you import. The state database can be accessed using select
, for instance:
const posts = select('core').getPosts();
The same applies to the dispatching of actions:
dispatch('core').savePost(postData);
Subscribers to state-wide changes use the same format but with a different style, but the idea is the same:
subscribe(() => const newPosts = select('core').getPosts(); // Update your UI based on the new posts );
But, it's not always the case that you use the standard stores. Most of the time, you'll use additional stores, or even register your own.
How to register for a WordPress data store
Determining the configuration of your store and then registering it using the WordPress database package begins with importing your store's configuration. register
function:
... import createReduxStore, register from '@wordpress/data' ...
It requires only one argument -- your store descriptor. You should then create a default state that allows the store to set its default values:
... const DEFAULT_STATE = todos: [], ; ...
Then, you need to create actions object, create an actions
object, specify a reduction
function for handling the state of affairs, and finally create the choosers
object with functions to connect to state information:
const actions = addTodo: (text) => ( type: 'ADD_TODO', text, ), ; const reducer = (state = DEFAULT_STATE, action) => switch (action.type) case 'ADD_TODO': return ...state, todos: [...state.todos, text: action.text, completed: false ], ; default: return state; ; const selectors = getTodos: (state) => state.todos, ;
In order to create your store's configuration create it by using an object called the createReduxStore
objects. This will initialize the actions such as selectors, controls and additional properties of the store you have created:
const store = createReduxStore('my-plugin/todos', reducer, actions, selectors, );
The most this object will need is a reducer in order to determine the form of the state and what it will change in response to other actions. Finally, you must register the store and call the store descriptor you define with createReduxStore
:
register(store);
Now you can use your store's custom interface as you would others:
import select, dispatch from '@wordpress/data'; // Add a new todo dispatch('my-plugin/todos').addTodo('Learn WordPress data package'); // Get all todos const todos = select('my-plugin/todos').getTodos();
The most important thing to consider when making use of the WordPress data bundle is how you make use of the various attributes and objects that are at your disposal.
Breaking down the five WordPress properties of the data store
The majority of what you do with the WordPress data pack happens "backwards" by defining the properties of a data store at a low level prior to the store itself. This createReduxStore
object is a great illustration, since it pulls together all the definitions that you create to make the descriptor used to register a store:
import createReduxStore from '@wordpress/data'; const store = createReduxStore( 'demo', reducer: ( state = 'OK' ) => state, selectors: getValue: ( state ) => state, , );
Other properties too require setup and configuration.
1. Actions
Actions are the main method to initiate the state of your store. They are plain JavaScript objects which describe the actions that will happen. This is why it could be a good idea to first create them, as you can decide what states you want to retrieve.
Const Actions = (, )
Action creators make use of arguments on their own and return an object that is passed to the reducer you define:
const actions = { updateStockPrice: (symbol, newPrice) => return type: 'UPDATE_STOCK_PRICE', symbol, newPrice ; ,
If you input the store's descriptor, you can dispatch actions creators to update the state value:
dispatch('my-plugin/todos').updateStockPrice('Y=', '150.37');
Take action objects as a guideline to the reducer about how to make state changes. As a minimum there is a good chance that you'll need to specify create and update, read and remove (CRUD) steps. There is also the possibility that you have a separate JavaScript document for types of actions and create an object for each of them, especially if you define these as constants.
2. Reducer
It's worth talking about the reducer because of its essential role along with actions. Its task is to define how the state should change according to the instruction it receives from an action. When you give it instructions from the action and the current state the state object can be returned as the state object in a different form and then pass it on the chain:
const reducer = (state = DEFAULT_STATE, action) => switch (action.type) case 'ADD_TODO': return ...state, todos: [...state.todos, text: action.text, completed: false ], ; case 'TOGGLE_TODO': return ...state, todos: state.todos.map((todo, index) => index === action.index ? ...todo, completed: !todo.completed : todo ), ; default: return state; ;
A reducer needs to be an pure function which means it can't modify the state that it accepts (rather, it should return to the user with changes). Reducers and actions have something in common in numerous aspects, therefore understanding the ways they interact is important.
3. Selectors
For access to the current state from a registered store it is necessary to use selectors. It's the primary way you "expose" the state of your store while also helping maintain your store's components separate from the store's internal structure:
const selectors = getTodos: (state) => state.todos, getTodoCount: (state) => state.todos.length, ;
They can be called by using select. select
function:
const todoCount = select('my-plugin/todos').getTodoCount();
But, the selector does not transfer the data to any other place: it simply reveals it and provides the ability to access it.
Selectors can receive as many arguments as necessary to get access to the state with accuracy. Its value depends on the results they accomplish inside the selector you define. As with actions, you might choose to create a separate file to hold all of your selections since you may have a large number of them.
4. Controls
Guiding the execution flow of the functionality of your website and executing the functions within it is when you utilize controls. The controls define the way in which you conduct execution flows for your actions. Take them for instance as assistants within the WordPress data bundle acting as intermediaries to gather the state that resolvers can use to process the state.
Controls are also able to handle any unwanted effects that occur in the store such as API calls, or interactions with APIs of browsers. They allow you to ensure that reducers are clean, while enabling you to handle complicated async processes:
const controls = FETCH_TODOS: async () => const response = await fetch('/api/todos'); return response.json(); , ; const actions = fetchTodos: () => ( type: 'FETCH_TODOS' ), ;
This cycle of fetching and returning data is crucial to the entire process. Without a signal from an action then you'll be unable to make use of the data.
5. Resolvers
Selectors expose a store's state but they do not explicitly transmit the data to any other location. Resolvers can meet criteria (and controls) in order to access the information. As with controls, they also handle asynchronous data fetching.
const resolvers = getTodos: async () => const todos = await controls.FETCH_TODOS(); return actions.receiveTodos(todos); , ;
The resolver ensures that the information you are looking to access is in the data store prior to running a selector. The tight connection between the resolver's and selector requires them to match names. This ensures that the WordPress data program can determine the resolver it should invoke according to the information that you have requested.
Furthermore The resolver will be able to receive the same arguments are passed into a selector function. It can also return, yield, or even dispatch actions objects.
Error handling when using the WordPress Data package
For example, if you dispatch actions that involve Asynchronous processes, a try-catch block could be a good option:
const StockUpdater = () => // Get the dispatch function const updateStock, setError, clearError = useDispatch('my-app/stocks'); const handleUpdateStock = async (stockId, newData) => try // Clear any existing errors clearError(); // Attempt to update the stock await updateStock(stockId, newData); catch (error) // Dispatch an error action if something goes wrong setError(error.message); ; return ( handleUpdateStock('AAPL', price: 150 )> Update Stock ); ;
For reducers, you can manage errors and then update the state:
const reducer = (state = DEFAULT_STATE; action) the state;
With selectors you could include error-checking to handle potential issues then look for any errors within the components prior to using the information. :
const MyComponent = () => // Get multiple pieces of state including error information const data, isLoading, error = useSelect((select) => ( data: select('my-app/stocks').getStockData(), isLoading: select('my-app/stocks').isLoading(), error: select('my-app/stocks').getError() )); // Handle different states if (isLoading) return Loading...; if (error) return ( Error loading stocks: error.message Try Again ); return ( /* Your normal component render */ ); ;
The utilizeSelect
and UseDispatch
tools give you a lot of power to handle errors within the WordPress data bundle. With both of these, you are able to send customized error messages to be used as arguments.
It is a good idea to make sure that you centralize your error states at the time of initial configuration as well as to maintain error boundaries at the component level. Utilizing error handling to load states can help make your code more simple and consistent.
How to integrate your WordPress database store to your site
There's plenty that the WordPress database package could do in order to assist you with managing state. The combination of all this can be a useful consideration. Take a look at a ticker, which updates financial data in real-time.
Your first step is to build a database of your personal data
import createReduxStore, register from '@wordpress/data'; const DEFAULT_STATE = stocks: [], isLoading: false, error: null, ; const actions = fetchStocks: () => async ( dispatch ) => dispatch( type: 'FETCH_STOCKS_START' ); try const response = await fetch('/api/stocks'); const stocks = await response.json(); dispatch( type: 'RECEIVE_STOCKS', stocks ); catch (error) dispatch( type: 'FETCH_STOCKS_ERROR', error: error.message ); , ; const reducer = (state = DEFAULT_STATE, action) => switch (action.type) case 'FETCH_STOCKS_START': return ...state, isLoading: true, error: null ; case 'RECEIVE_STOCKS': return ...state, stocks: action.stocks, isLoading: false ; case 'FETCH_STOCKS_ERROR': return ...state, error: action.error, isLoading: false ; default: return state; ; const selectors = getStocks: (state) => state.stocks, getStocksError: (state) => state.error, isStocksLoading: (state) => state.isLoading, ; const store = createReduxStore('my-investing-app/stocks', reducer, actions, selectors, ); register(store);
This process sets up a default state that includes loading and error state as well as your actions, reducers, as well as selectors. When you've defined them states, you are able to register your store.
Display the store's information
import useSelect, useDispatch from '@wordpress/data'; import useEffect from '@wordpress/element'; const StockTicker = () => const stocks = useSelect((select) => select('my-investing-app/stocks').getStocks()); const error = useSelect((select) => select('my-investing-app/stocks').getStocksError()); const isLoading = useSelect((select) => select('my-investing-app/stocks').isStocksLoading()); const fetchStocks = useDispatch('my-investing-app/stocks'); useEffect(() => fetchStocks(); , []); if (isLoading) return Loading stock data...; if (error) return Error: error; return ( Stock Ticker stocks.map((stock) => ( stock.symbol: $stock.price )) ); ;
The component includes useSelect and UseSelect
and UseDispatch
hooks (along with others) to handle information access, dispatching tasks as well as lifecycle management for components. Additionally, it sets specific error and loading state messages, along with some code that displays the ticker. With this in place the next step is to register the component with WordPress.
The component must be registered with WordPress
import registerBlockType from '@wordpress/blocks'; import StockTicker from './components/StockTicker'; registerBlockType('my-investing-app/stock-ticker', title: 'Stock Ticker', icon: 'chart-line', category: 'widgets', edit: StockTicker, save: () => null, // This will render dynamically );
The procedure follows what you'd normally do to sign up Blocks on WordPress It doesn't need an additional setup or implementation.
Monitoring state updates as well as interaction with users
When you've registered your Block, it is your responsibility to deal with user interactions as well as actual-time updates. It will require interactive controls as well as customized HTML as well as JavaScript:
const StockControls = () => const addToWatchlist, removeFromWatchlist = useDispatch('my-investing-app/stocks'); return ( addToWatchlist('AAPL')> Add Apple to Watchlist removeFromWatchlist('AAPL')> Remove from Watchlist ); ;
For real-time updates it is possible to set up an interval inside React's component:
useEffect(() => const updateStockPrice = dispatch('my-investing-app/stocks'); const interval = setInterval(() => stocks.forEach(stock => fetchStockPrice(stock.symbol) .then(price => updateStockPrice(stock.symbol, price)); ); , 60000); return () => clearInterval(interval); , [stocks]);
This approach keeps your component's data in sync with your store while maintaining a clear separation of responsibilities. The WordPress data pack will manage every state update and gives your application an enduring consistency.
Server-side rendering
function my_investing_app_render_stock_ticker($attributes, $content) // Fetch the latest stock data from your API $stocks = fetch_latest_stock_data(); ob_start(); ?> Stock Ticker
: $
'my_investing_app_render_stock_ticker' ));
This approach provides a complete integration of your data store with WordPress taking care of everything from initial rendering through real-time updates and user interactions.
Summary
The WordPress data package provides a complicated, but robust method to handle application state in your WordPress projects. Beyond the key concepts lies an array of operations, functions as well as arguments. Remember, though, that not all data has to go into an international store. the local component state still is a part of the code you write.
Do you see yourself using the WordPress data program frequently, or do you have another method of managing the state of your life? Share your opinions with us by leaving a comment below.
Steve Bonisteel
Steve Bonisteel is a Technical Editor for the site. He began his career in writing as a newspaper reporter, who was chasing ambulances and fire trucks. The journalist has covered Internet-related technology since the late 1990s.