Master TypeScript development in Visual Studio Code - (r)
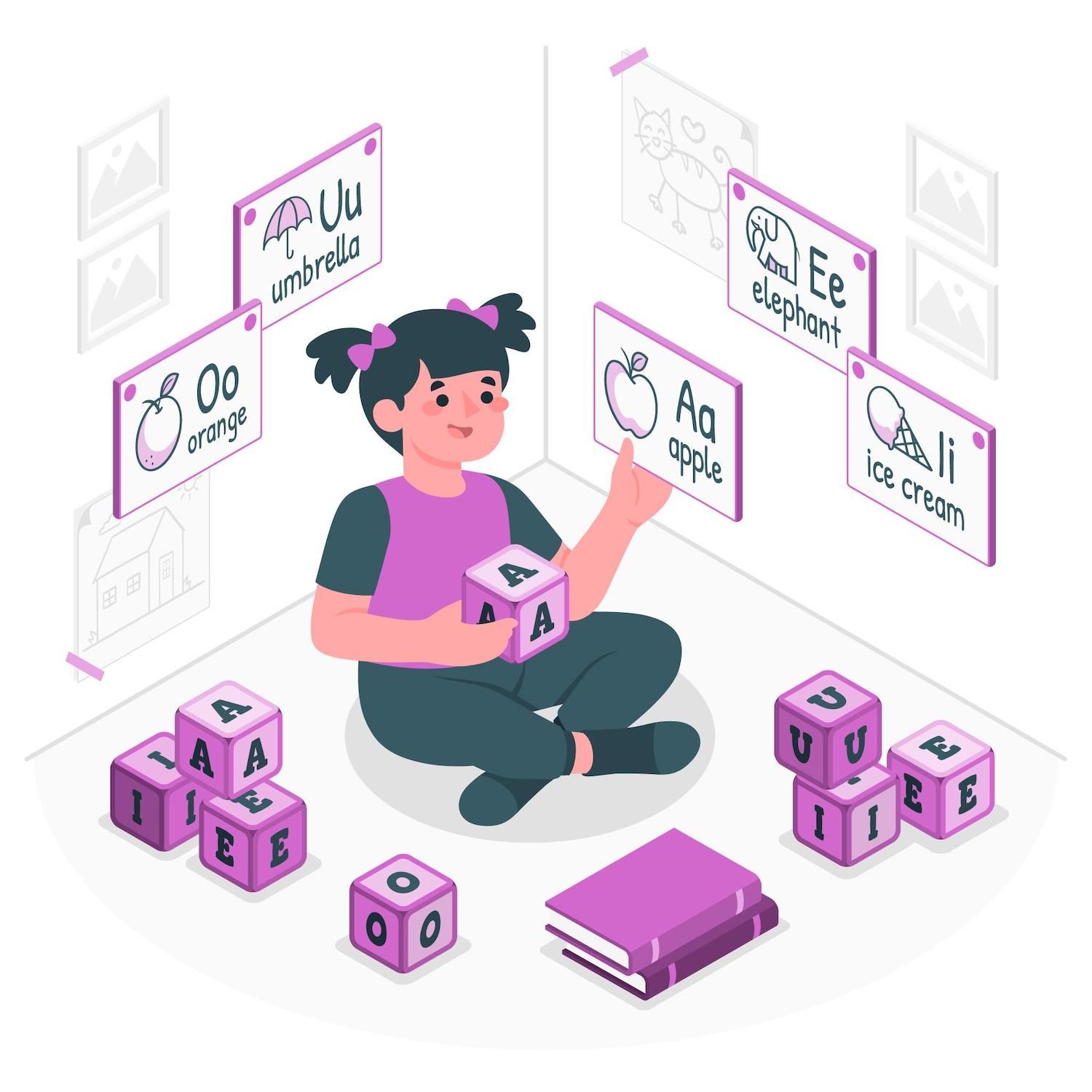
-sidebar-toc>
How do you set up Visual Studio Code for TypeScript development
This step-by-step tutorial shows how to set up Visual Studio Code for TypeScript development. The first step is to start an Node.js project with TypeScript create some code and run, compile, and analyze the TypeScript and run it in Visual Studio Code.
The prerequisites
Before you begin, be sure you have:
- Node.js installed and localized
- Visual Studio Code was downloaded and installed
node -v
This should give you the latest version of Node.js on your machine in the form of:
v21.6.1
Now let's get started with TypeScript using Visual Studio Code!
Download the TypeScript compiler
Visual Studio Code supports TypeScript development but doesn't include an integrated TypeScript compiler. As the TypeScript compiler transforms or transpiles -- TypeScript code into JavaScript and vice versa, it's an essential requirement for testing the performance of your TypeScript code. Also, tsc is a program that takes TypeScript Code as an input, and generates JavaScript code for output after which you use the JavaScript code by using Node.js or the Web browser.
Enter the below command into your terminal to install the TypeScript compiler globally on your PC:
npm install Typescript
Check the version that is installed of tsc:
tsc --version
If the command does not return an error, tsc is in use. Now you have all the information you need to build a TypeScript project!
Make a TypeScript project
Let's build a straightforward Node.js TypeScript project called hello-world. Open your terminal, and make a folder to store your project:
mkdir hello-world
cd hello-world
Inside hello-world, initialize a project using the following npm command
NPM init -y
It creates the package.json config file for your Node.js project. Time to see what the project consists of in Visual Studio Code!
Start Visual Studio Code and select the File > Open Folder...
In the window that pops up, select from the hello-world project folder and then click Open. The project will look similar to this:
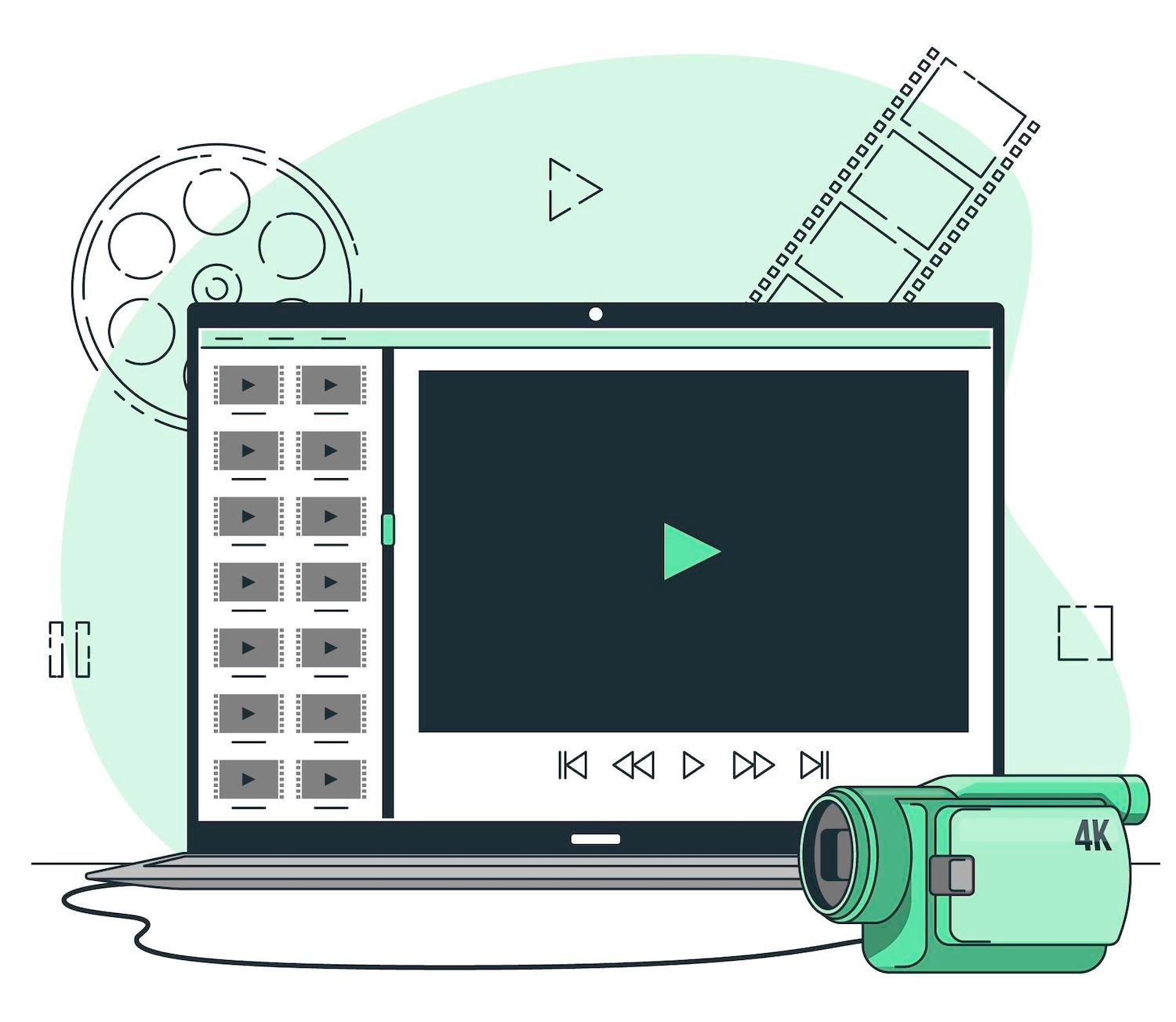
Currently, the project consists of only the package.json file initialized through the init function of npm
.
Choose the View > Terminal within the Visual Studio Code menu to get access to Visual Studio Code's built-in terminal. Then, run the following commands:
Npx tsc --init
It starts a TypeScript configuration file dubbed tsconfig.json in the project directory.
The tsconfig.json file allows you to modify the behaviour of the TypeScript compiler. It specifically provides you with the TypeScript compiler with instructions for changing to the TypeScript code. Without it, tsc wouldn't be able to build your Typescript project the way you'd prefer.
You can open tsconfig.json in Visual Studio Code in Visual Studio Code, and find comments for each setting. We want our tsconfig.json file to include these options:
"compilerOptions":
"target": "es2016",
"module": "commonjs",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true,
"sourceMap": true,
"outDir": "./build"
It's likely that the one difference you'll notice between the options above are the setting of the source mapping to the JavaScript generated by the program as well as the inclusion of an output directory.
"sourceMap": true,
"outDir": "./build"
Edit your tsconfig.json file.
Maps to Source is a requirement of Visual Studio Code compiler. Visual Studio Code compiler.
OutDir configuration. The OutDir configuration specifies the location where the compiler will place the transpiled files. By default, that's the root folder of the project. In order to avoid clogging your project folder with build files at every creation, you can set the folder to another like the build folder..
You're TypeScript project is now completed and is ready to be built. However, first you must have TypeScript code.
Right-click in the Explorer section and choose New File... Type index.ts
and press Enter. Your project will now contain the TypeScript file called index.ts:
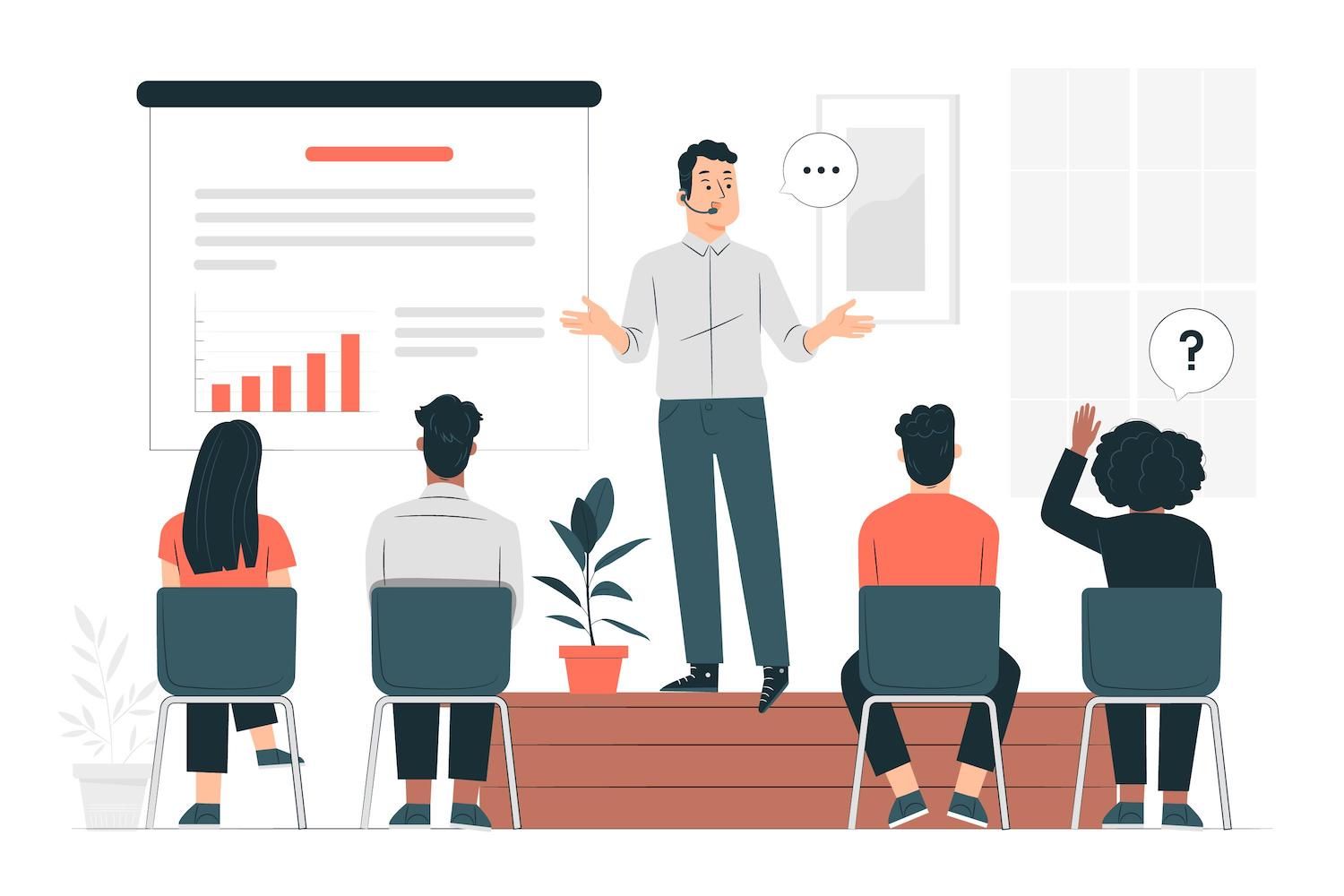
Let's get things started with the following TypeScript code:
const message: string = "Hello, World!" console.log(message)
This code snippet prints only the well-known "Hello, World! message.
Try IntelliSense for code completion
While writing the lines above in Visual Studio Code, you might have noticed some codes suggested by editors. It happens due to IntelliSense the most popular the Visual Studio Code's awesome tools.
IntelliSense comes with features such as the completion of code, information on docs, and parameter info on functions. IntelliSense offers suggestions on how you can complete your code while you type. This will dramatically increase your efficiency as well as accuracy. Watch it working on this page:
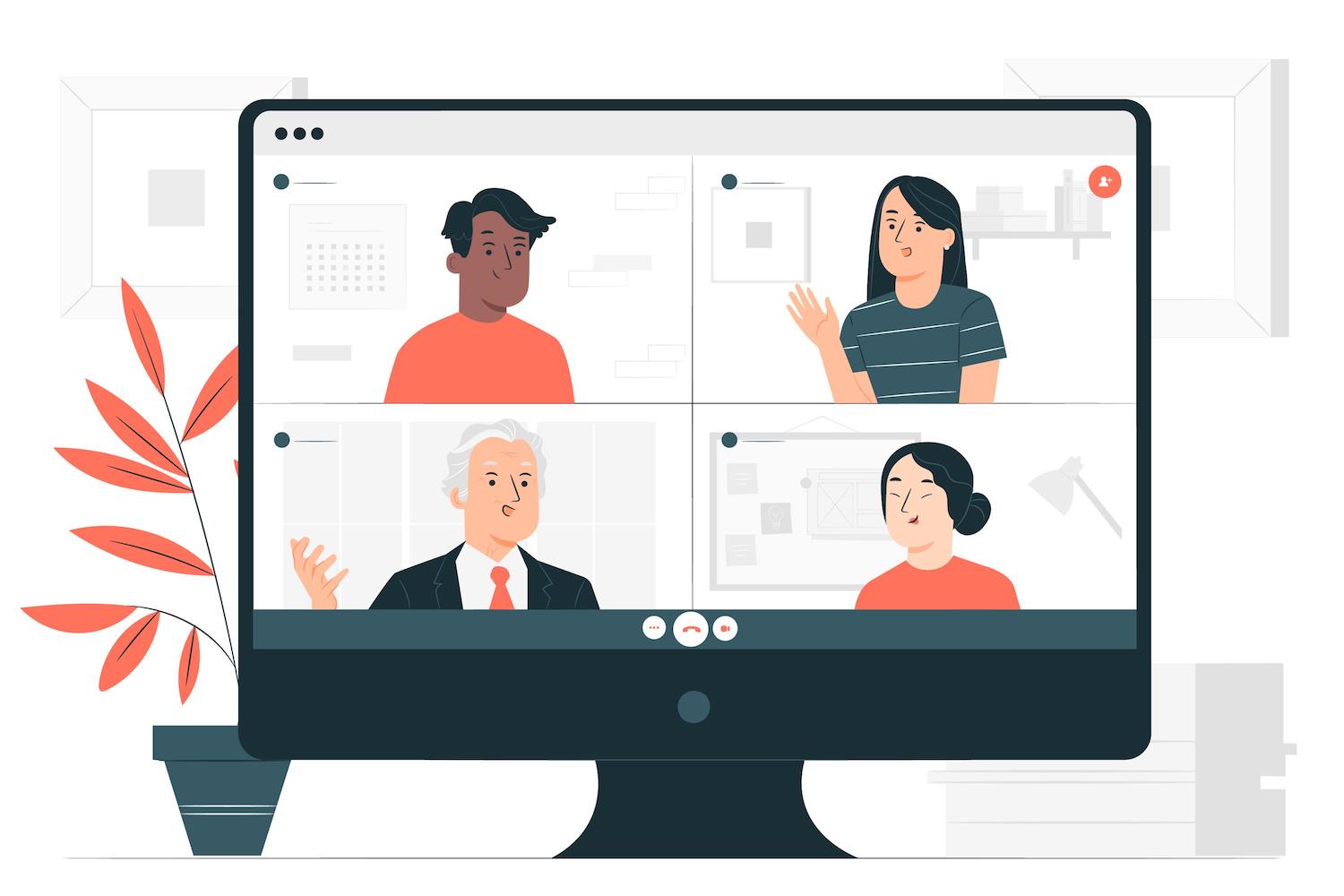
Keep in mind it is that Visual Studio Code comes with IntelliSense support for TypeScript applications right out of the box. You don't have to configure the feature by hand.
If you've learned how to write TypeScript with ease in Visual Studio Code, let's compile it and see whether it's working.
Compiling TypeScript in Visual Studio Code
Start the terminal integrated in Visual Studio Code and run:
tsc -p .
This converts every TypeScript documents in the project into JavaScript. The "-p .
tells the compiler to utilize to use the tsconfig.json file located within the current directory. The output in this instance, index.js and index.js.map, the source map index.js.map -- is stored within the ./build directory.
You are able to verify that the transpiled JavaScript code works when you use this command on the terminal.
node ./build/index.js
Node.js will translate index.js and print to the terminal:
Hello, World!
A different method of starting the transpiler is by selecting Terminal > Launch Building Task... on the Visual Studio Code menu and choose the tsc:build - tsconfig.json option.
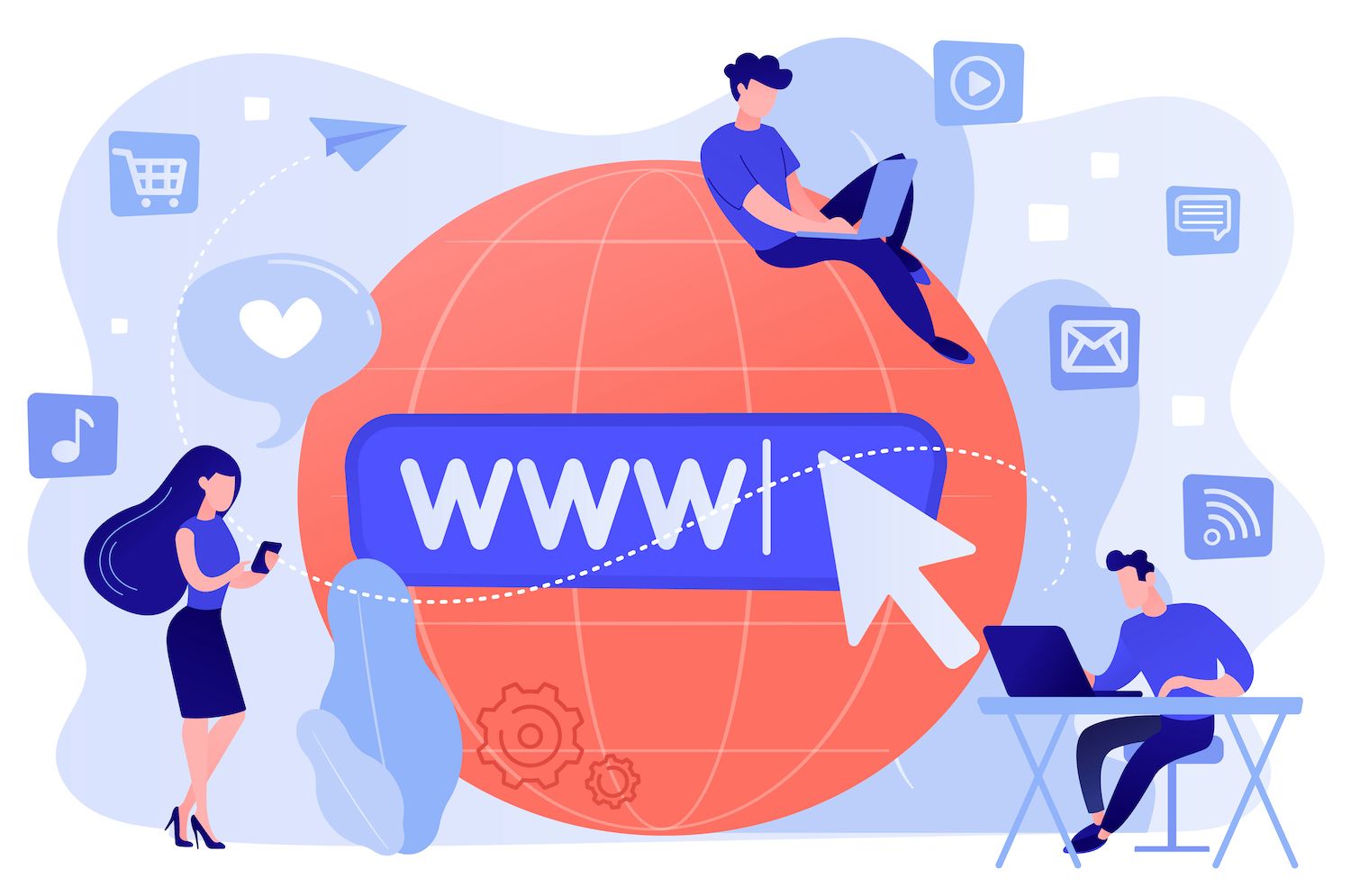
This command runs tsc -p .
behind the scenes and builds your code directly within the editor.
That's how you build your TypeScript project using Visual Studio Code. You now need find out how you can begin and then debug your code.
Run and debug TypeScript within Visual Studio Code
Visual Studio Code supports TypeScript debugging with its built-in Node.js debugger. Before you're able to utilize it, you'll need to create it. Click the Run and Debuggle icon located on the left sidebar, click Create a launch.json file then select Node.js.
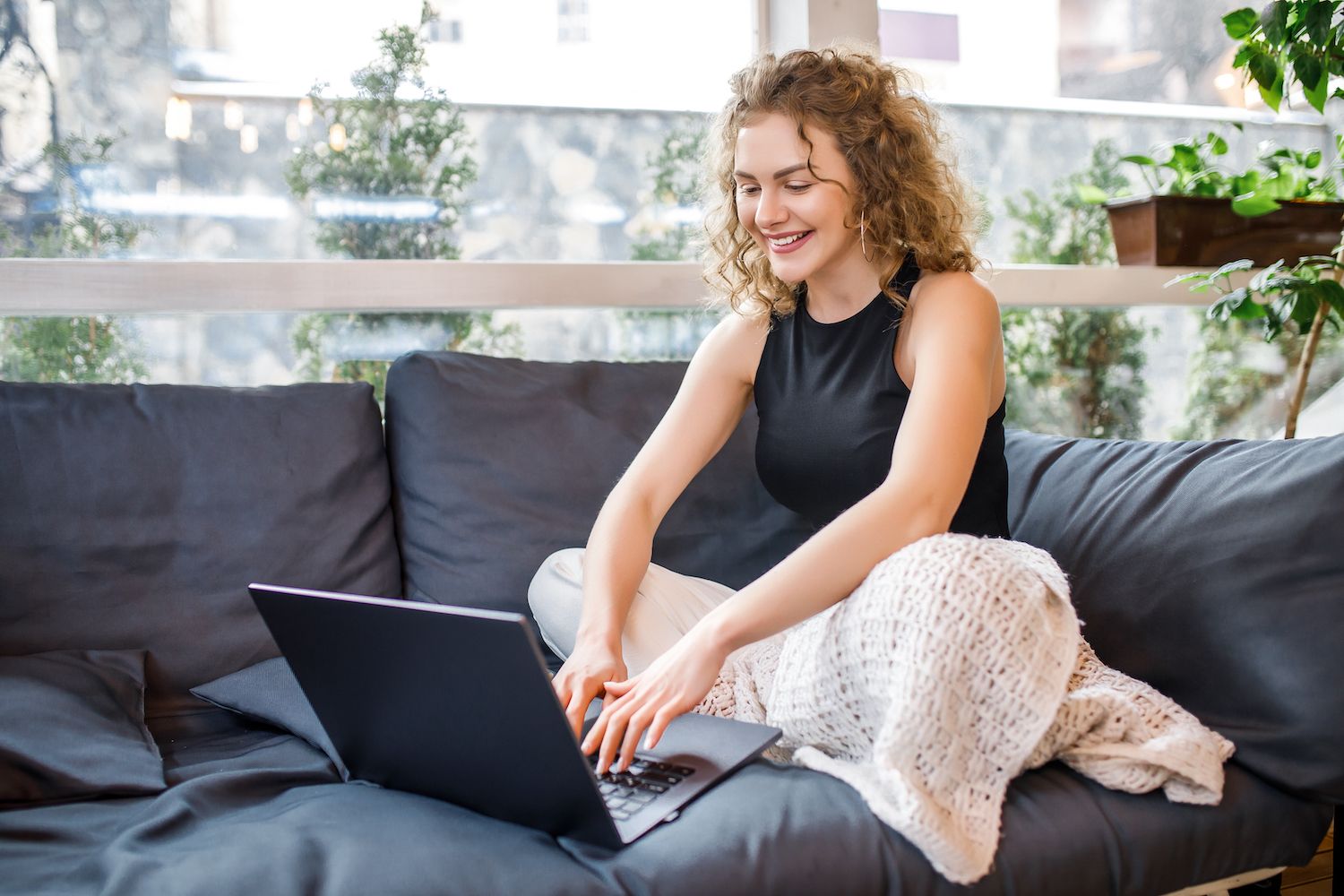
This creates an initial Node.js launch.json file that is the configuration files that Visual Studio Code Visual Studio Code debugger uses for debugging and launching an application. This config file specifies how to start the app, the command-line arguments to use, and the environment variables that need to be set.
In the Explorer section, launch.json is located within the .vscode folder of an application.
Open that file and make changes as follows:
// Use IntelliSense to discover possible attributes. Click to see the descriptions of attributes already in use. // For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
"type": "node",
"request": "launch",
"name": "Launch Program",
"skipFiles": [
"node_modules/**"
],
"program": "$workspaceFolder/index.ts",
"preLaunchTask": "tsc: build - tsconfig.json",
"outFiles": ["$workspaceFolder/build/**/*.js"]
]
Adjust your programs
, preLaunchTask
outFiles, and preLaunchTask outFiles
options, considering that
program
: Specifies the path leading to the entry point of the application to debug. When using TypeScript it must contain the principal file which will be executed during the launch of the application.preLaunchTask
is the name of the Visual Studio Code build task that will be run prior to launching the program. In the context of a TypeScript project, this would be the task to build.outFiles
contains the path for the converted JavaScript files generated by the process of building. Source map files created by tsc due to"sourceMap", a"sourceMap": true
config are used by debuggers to translate your TypeScript source code to the resulting JavaScript code. This lets you debug TypeScript code in real time.
Keep the launch.json file and open index.ts. Select the blank line just before that console.log()
line to set an endpoint. A red dot appears adjacent to the line. It looks like this:
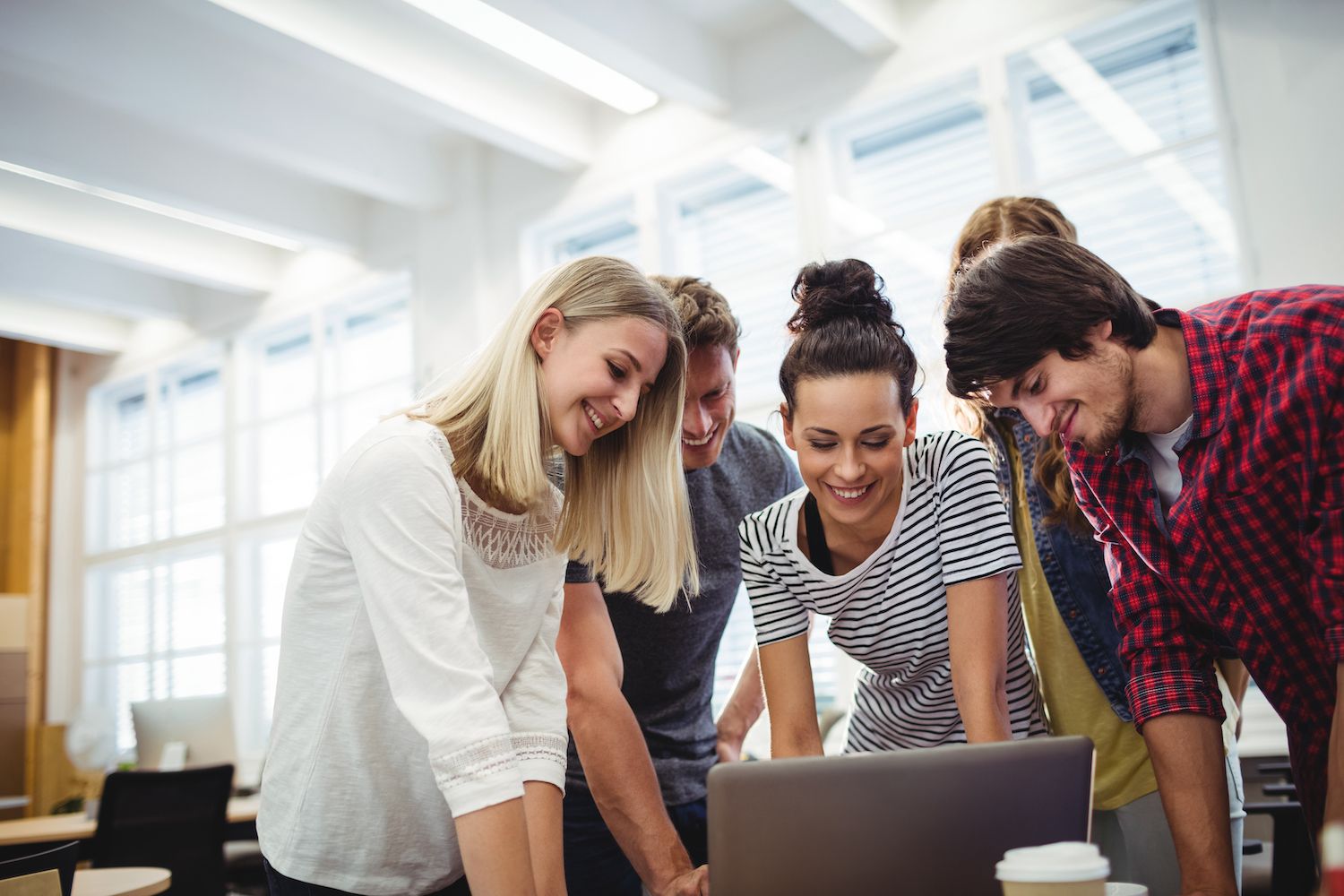
If you execute your code using the compiler, it's code is executed but stops at that point. With this breakpoint it is possible to confirm whether the Node.js debugger in Visual Studio Code is working exactly as you expect it to.
Go to the Run and Debuggle section again and click the green play button to launch the debugger. Wait for preLaunchTask
to run. When the code is completed, the program will launch with the program, then it ceases execution at the breakpoint that is set in.
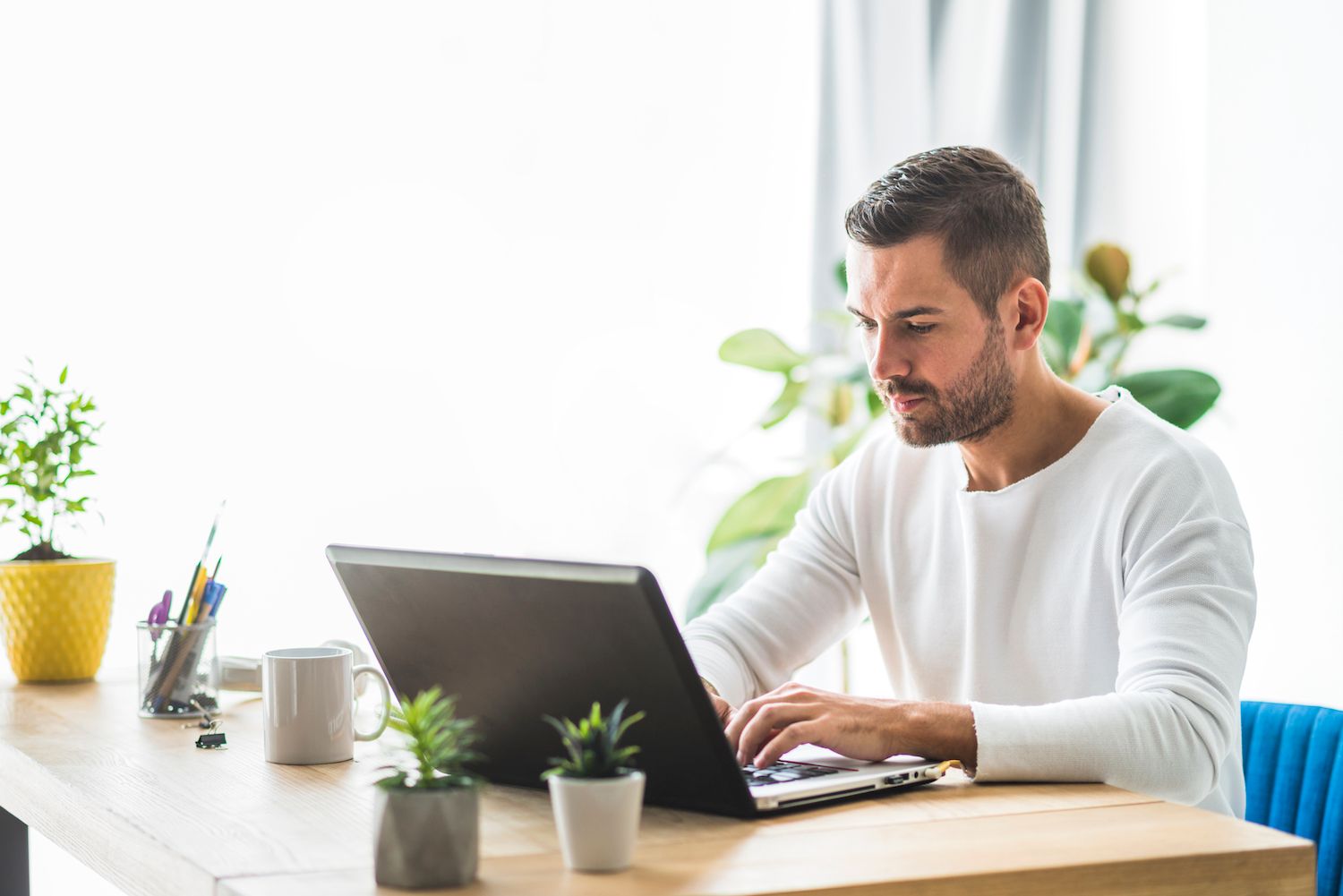
On the left in the image above it is possible to look at the current values of variables as of the break. Also, you can stop, step over the break, move in or out as well as restarting and stopping, as described in the Visual Studio Code document on debugging.
Use F5 to resume the execution You should then be able to see the following message on the Debug Console tab:
Hello, World!
That's the output you'd anticipate the application to do This is a sign that the program has been successfully executed.
You just learned how to configure Visual Studio Code for TypeScript programming. This tutorial may end there, but there's one more important thing to learn the best way to setup an extension within Visual Studio Code that can make writing quality code in TypeScript much easier.
How do I configure ESLint within Visual Studio Code
The most awaited Visual Studio Code extensions for TypeScript development is the ESLint extension.
ESLint is a popular static code analysis tool for JavaScript and TypeScript which helps developers find and fix common coding errors and ensure coding standards are adhered to. This extension can run ESLint directly in the editor.
Let's incorporate ESLint to Visual Studio Code in your TypeScript project.
First, initialize ESLint to your project by using the following terminal command:
npm init @eslint/config
During the configuration process, you will be asked a few questions that will aid in the creation of the ESLint Configuration file. The answers can be as follows:
What would you prefer to utilize ESLint? * Style What kind of modules does your project employ? * commonjs
Which framework is your project using? * none
Does your project use TypeScript? Do you know where your code be running? BrowserHow do you want to establish an aesthetic for your work? * GuideWhat style guidelines do you prefer to adhere to? * standard-with-typescript
What format do you want your config file to be in? * JSON
The installer will check for dependencies and ask if you want to install any of the packages which are not installed. You can respond like this:
Would you like to set up them now? * Yes
Which is the package manager you would like to install? * npm
When you've completed the process, you'll find the new .eslintrc.json file containing the following code in its initial form:
"env":
"browser": true,
"commonjs": true,
"es2021": true
,
"extends": "standard-with-typescript",
"overrides": [
],
"parserOptions":
"ecmaVersion": "latest"
,
"rules":
The .eslintrc.json file contains the settings used by ESLint to ensure specific code, style and quality standards. What a standard .eslintrc.json for a Node.js TypeScript project may look as:
"env":
"browser": true,
"commonjs": true,
"es2021": true,
// enable node support
"node": true
,
"extends": "standard-with-typescript",
"overrides": [
],
"parserOptions":
"ecmaVersion": "latest",
"project": "tsconfig.json"
,
"rules":
// force the code to be indented with 2 spaces
"indent": ["error", 2],
// mark extra spaces as errors
"no-multi-spaces": ["error"]
The time has come to install the ESLint extension to Visual Studio Code. Click the Extensions icon in the left menu, and then type "ESLint" into the search box
. Locate the ESLint extension, and then select to install it..
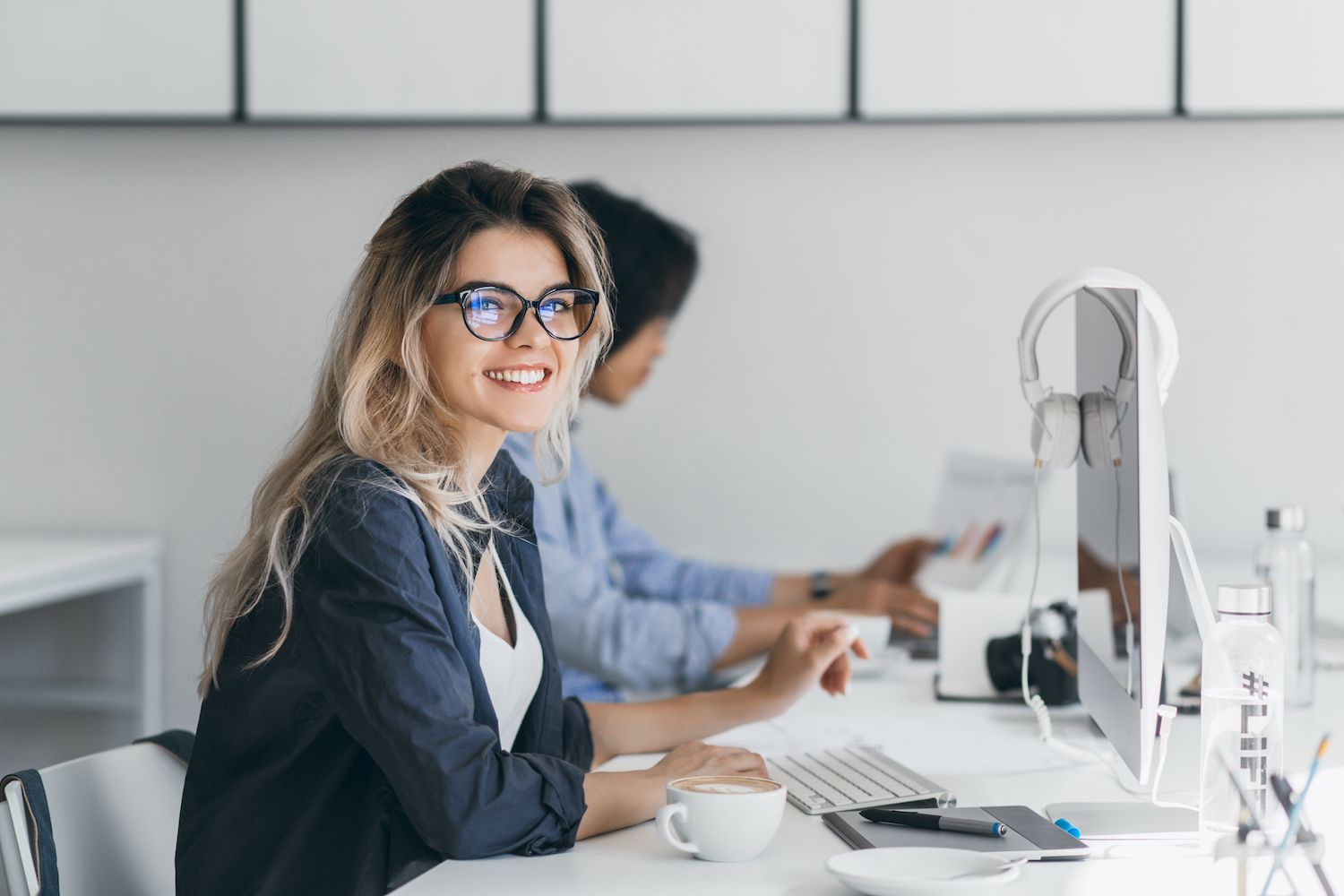
In order to enable the ESLint extension that will automatically check your TypeScript files after every save, make an settings.json file inside .vscode with the following content:
"editor.codeActionsOnSave":
"source.fixAll.eslint": true
,
"eslint.validate": [
"typescript"
],
"eslint.codeActionsOnSave.rules": null
Settings.json is the settings.json file contains the configuration used by Visual Studio Code to customize the behaviour of the editor and the extensions it comes with.
Restart Visual Studio Code to make it load the latest extension and configurations.
If you open index.ts and edit the code, you'll see new errors reported to the IDE. To fix code style mistakes it is recommended to save the file and ESLint instantly reformats your code as defined in .eslintrc.json.
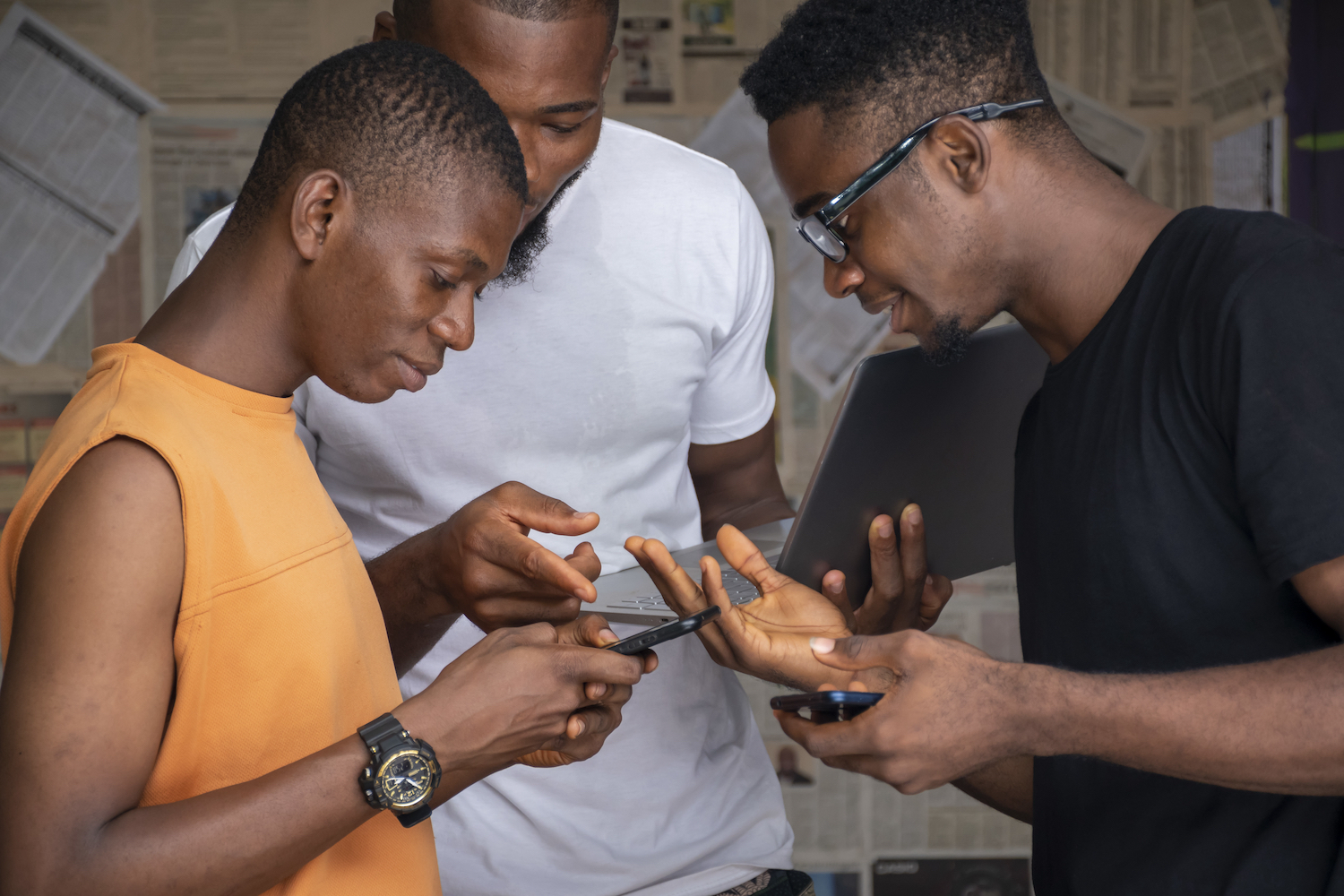
Now, nothing can stop you from creating high-quality code! The only thing left to do is implement your Node.js application to a reputable cloud hosting service like 's.
Summary
Thus, configuring Visual Studio Code for development using TypeScript is fairly straightforward--you have just discovered how to build the Node.js project in TypeScript then load it into Visual Studio Code, and use the IDE to write code assisted by IntelliSense. Also, you set up to use the TypeScript compiler, installed the Node.js compiler to debug TypeScript programming, as well as integrated ESLint into the project.
Antonello Zanini
Antonello is an engineer of software but prefers to be called an Technology Bishop. Sharing knowledge via writing is the goal of his work.